Progressive Web Apps (PWAs) represent a seismic shift in the web development landscape, redefining the capabilities of web applications. They are fast, reliable, and engaging, blurring the lines between web content and native applications. PWAs leverage modern web technologies to offer users a native app-like experience directly in their web browsers.
At the heart of PWAs lies the concept of delivering a high-quality user experience irrespective of device or network conditions. This approach ensures that PWAs can work offline or on low-quality networks, load quickly, and integrate seamlessly with the user’s device, providing functionalities like push notifications and home screen icons.
The Role of JavaScript in PWA Development
JavaScript plays a pivotal role in enabling the dynamic and powerful features of PWAs. As a versatile programming language, JavaScript provides the functionality necessary to create responsive and interactive web applications. In the context of PWAs, JavaScript is used for:
- Service Workers: These are JavaScript files that run separately from the main browser thread, intercepting network requests, caching or retrieving resources from the cache, and delivering push messages. They are the key technology behind a PWA’s ability to work offline and load quickly.
- Web App Manifest: This is a JSON file that controls how the PWA appears to the user and how it can be launched. JavaScript is used to link this manifest file, allowing developers to customize the appearance of the PWA, including its home screen icon, page orientation, and display mode.
- Push Notifications: JavaScript is used to implement push notifications in PWAs, engaging users effectively by sending them timely updates and information.
- Background Sync: With JavaScript, developers can implement background sync features in PWAs, allowing web apps to synchronize data in the background, enhancing the app’s offline capabilities.
- Accessing Device Features: Modern JavaScript APIs enable PWAs to access device features such as camera, geolocation, and motion sensors, making web apps more interactive and functional.
Leveraging JavaScript for Enhanced PWA Features
JavaScript’s flexibility and the extensive ecosystem of libraries and frameworks make it an ideal choice for developing sophisticated PWAs. Frameworks like React, Angular, and Vue.js are commonly used in PWA development, providing a structured way to build dynamic web applications. These tools streamline the development process, offer reusable components, and ensure that the PWA is maintainable and scalable.
Key Benefits of PWAs
Progressive Web Apps (PWAs) offer a myriad of benefits that make them an attractive choice for businesses and developers. Let’s delve into some of these key advantages:
1. Quick Market Reach and Cross-platform Compatibility
PWAs can be developed, tested, and deployed much faster than traditional mobile apps. This speed is largely due to the use of a single codebase for multiple platforms. For instance, a PWA built with JavaScript can run on any device with a web browser, regardless of the operating system. This universality significantly widens market reach.
JavaScript Example:
// Basic service worker registration in JavaScript
if ('serviceWorker' in navigator) {
navigator.serviceWorker.register('/service-worker.js')
.then(function(registration) {
console.log('Service Worker registered with scope:', registration.scope);
})
.catch(function(error) {
console.log('Service Worker registration failed:', error);
});
}
This snippet showcases how a service worker is registered in a PWA, a fundamental step in enabling offline capabilities and push notifications.
2. Enhanced User Engagement and Lightweight Experience
PWAs are designed to be engaging and responsive, offering a user experience akin to native apps. The use of service workers allows content to be cached and loaded quickly, even on poor network conditions, thereby enhancing user engagement.
Moreover, PWAs are typically smaller in size compared to native apps, resulting in a more lightweight experience. This smaller footprint means faster downloads and less storage space required on users’ devices.
JavaScript Example:
// Example of caching files with a service worker
self.addEventListener('install', function(event) {
event.waitUntil(
caches.open('v1').then(function(cache) {
return cache.addAll([
'/index.html',
'/styles.css',
'/script.js',
'/images/logo.png'
]);
})
);
});
This code demonstrates how to cache essential files during the service worker’s install event, ensuring they are available for offline use.
3. Lower Development Costs
Building a PWA can be more cost-effective than developing separate native applications for different platforms. JavaScript, a language familiar to many web developers, is used for PWA development, thus reducing the need to hire specialists for different native platforms.
4. Improving SEO
PWAs are indexed by search engines, which can improve a website’s visibility and SEO ranking. The fast loading times and mobile-friendly nature of PWAs also contribute positively to SEO.
JavaScript Example:
// Sample manifest file link in HTML
<link rel="manifest" href="/manifest.json">
This line in the HTML links to the Web App Manifest, a key element in defining how the PWA appears to users and search engines.
Latest Features and Capabilities in PWAs

1. New Browser Capabilities: Window Controls Overlay and Widgets
Recent advancements in browser capabilities have further enhanced the potential of PWAs. One such feature is the Window Controls Overlay, which allows PWAs to utilize the full screen, including the area typically reserved for window controls. This enhances the app-like feel of PWAs.
JavaScript Example for Window Controls Overlay:
if ('windowControlsOverlay' in navigator) {
navigator.windowControlsOverlay.addEventListener('geometrychange', () => {
const { titlebarAreaRect } = navigator.windowControlsOverlay;
console.log('Titlebar area dimensions:', titlebarAreaRect);
});
}
This snippet demonstrates how to detect and respond to changes in the window controls overlay’s geometry, allowing for dynamic UI adjustments.
Another noteworthy feature is the integration of PWAs with Windows 11 Widgets, allowing apps to be more deeply integrated into the operating system, enhancing accessibility and user engagement.
2. Service Worker Advancements: Enhanced Offline Capabilities
Service workers are at the core of a PWA’s functionality. Recent advancements have expanded their capabilities, especially in terms of offline functionality and background processing.
JavaScript Example for Service Worker:
// Example of using service workers for offline capabilities
self.addEventListener('fetch', function(event) {
event.respondWith(
caches.match(event.request)
.then(function(response) {
if (response) {
return response;
}
return fetch(event.request);
}
)
);
});
This code allows a PWA to serve cached content when offline, falling back to network requests when online.
3. Expanding App Capabilities: Device APIs
Modern JavaScript APIs have made it possible for PWAs to access more device features, such as geolocation, camera, and motion sensors, making them more functional and versatile.
JavaScript Example for Geolocation API:
// Getting the current position using Geolocation API
if ("geolocation" in navigator) {
navigator.geolocation.getCurrentPosition(function(position) {
console.log("Latitude is :", position.coords.latitude);
console.log("Longitude is :", position.coords.longitude);
});
} else {
console.log("Geolocation is not supported by this browser.");
}
This code snippet demonstrates how a PWA can access the user’s current location, subject to their permission.
Design Trends in PWA Development
Progressive Web Apps are not just about functionality; their design plays a crucial role in user experience. Recent trends in PWA design focus on enhancing usability, especially on mobile devices.
1. Mobile-Optimized Navigation Menus
With the increasing use of mobile devices, PWAs are adopting navigation menus that are easy to access with one hand. This often involves placing key navigation elements at the bottom of the screen.
JavaScript and CSS Example for Bottom Navigation:
// Basic CSS for bottom navigation
.bottom-nav {
position: fixed;
bottom: 0;
width: 100%;
// Add more styling as needed
}
// JavaScript to enhance bottom navigation functionality
document.querySelector('.bottom-nav').addEventListener('click', function(event) {
// Handle navigation click events
});
This code demonstrates a simple implementation of a bottom navigation bar, a common trend in modern PWA design.
2. Strategic Element Placement
Another design trend is the strategic placement of tappable elements. These elements are positioned within easy reach of the user’s thumb, enhancing the app’s usability on larger screens.
CSS Example for Element Placement:
/* Styling for easy-to-reach tappable elements */
.tappable-area {
position: absolute;
bottom: 20%;
left: 10%;
/* Additional styling */
}
This CSS ensures that key interactive elements are placed within the thumb’s reach on larger screens.
3. Utilizing Screen Space Efficiently
Efficient use of screen space is paramount in PWA design. This includes appropriately sizing buttons and text, reducing paddings, and reorganizing elements to avoid clutter.
CSS Example for Efficient Screen Space Usage:
/* Optimizing screen space usage */
.content {
padding: 10px;
font-size: 16px;
/* Additional styling for content */
}
.button {
padding: 10px 20px;
font-size: 14px;
/* Additional button styling */
}
These styles demonstrate how to effectively use screen space by optimizing padding and font sizes.
PWAs vs. Traditional Websites and Native Apps

The debate between PWAs, traditional websites, and native apps centers on performance, user experience, and technical capabilities. Understanding these differences is key to choosing the right approach for your project.
1. Speed and Performance
PWAs often outperform traditional websites in terms of loading speed and smoothness, thanks to service workers. They also rival native apps in performance, especially with advancements in browser technologies.
JavaScript Example for Service Worker Caching:
// Service worker caching for faster load times
self.addEventListener('install', event => {
event.waitUntil(
caches.open('my-pwa-cache').then(cache => {
return cache.addAll([
'/index.html',
'/main.css',
'/main.js',
// other assets
]);
})
);
});
This code snippet shows how a service worker can cache essential assets, ensuring that a PWA loads quickly, even on subsequent visits.
2. User Experience
PWAs offer a more seamless user experience compared to traditional websites by mimicking native app features like offline access and push notifications. However, native apps still lead in terms of overall smoothness and access to advanced device features.
JavaScript Example for Push Notifications:
// Registering for push notifications in a PWA
navigator.serviceWorker.ready.then(function(registration) {
if (!registration.pushManager) {
console.log('Push manager unavailable.');
return;
}
registration.pushManager.subscribe({ userVisibleOnly: true })
.then(function(subscription) {
console.log('Push subscription successful:', subscription);
})
.catch(function(error) {
console.log('Push subscription failed:', error);
});
});
This example demonstrates registering a service worker for push notifications, enhancing user engagement.
3. Accessibility and Reach
PWAs are accessible through web browsers, offering a broader reach compared to native apps that require downloading from app stores. This aspect makes PWAs a powerful tool for businesses aiming for a wider audience.
Building Secure and Efficient PWAs
Security and efficiency are paramount in PWA development. Adhering to best practices in these areas ensures a trustworthy and smooth user experience.
1. Implementing HTTPS for Security
Using HTTPS is essential for PWAs to ensure secure data transmission. It prevents intruders from tampering with or eavesdropping on communications between the user and the site.
JavaScript Example for Checking HTTPS:
// Ensure the PWA is served over HTTPS
if (location.protocol !== 'https:') {
console.log('Please use HTTPS for enhanced security');
}
This code snippet checks if the PWA is served over HTTPS, highlighting the importance of secure connections.
2. Service Worker Security Considerations
Service workers can intercept network requests, making them a crucial point for implementing security measures. Ensuring they are updated and managed correctly is vital for maintaining the integrity of the PWA.
JavaScript Example for Updating Service Workers:
// Updating service workers for security
self.addEventListener('install', event => {
self.skipWaiting();
});
self.addEventListener('activate', event => {
event.waitUntil(clients.claim());
});
This script ensures that the latest version of the service worker is activated as soon as it’s installed, keeping the PWA updated and secure.
3. Efficient Loading and Performance Optimization
Efficient use of resources is a hallmark of PWAs. Techniques like lazy loading and code splitting are employed to optimize performance and speed.
JavaScript Example for Lazy Loading:
// Lazy loading images in a PWA
document.addEventListener('DOMContentLoaded', function() {
let lazyImages = [].slice.call(document.querySelectorAll('img.lazy'));
if ('IntersectionObserver' in window) {
let lazyImageObserver = new IntersectionObserver(function(entries, observer) {
entries.forEach(function(entry) {
if (entry.isIntersecting) {
let lazyImage = entry.target;
lazyImage.src = lazyImage.dataset.src;
lazyImage.classList.remove('lazy');
lazyImageObserver.unobserve(lazyImage);
}
});
});
lazyImages.forEach(function(lazyImage) {
lazyImageObserver.observe(lazyImage);
});
} else {
// Fallback for browsers without IntersectionObserver support
}
This code demonstrates how to implement lazy loading for images, where images are loaded as they enter the viewport, reducing initial load time.
Future Trends and Opportunities in PWA Development
The landscape of Progressive Web Apps is continuously evolving, with new trends and opportunities emerging that shape their future development.
1. Advancements in Web Technologies
The advancement of web technologies like WebAssembly and WebGPU promises to further enhance the capabilities of PWAs. These technologies enable more complex and computationally intensive tasks to be performed in the browser, bringing PWAs closer to native app performance.
JavaScript Example for WebAssembly:
// Loading a WebAssembly module in a PWA
WebAssembly.instantiateStreaming(fetch('module.wasm'), {})
.then(result => {
result.instance.exports.exportedFunction();
});
This code snippet demonstrates how to load and instantiate a WebAssembly module, enabling high-performance computations in a PWA.
2. Increased Focus on Progressive Enhancement
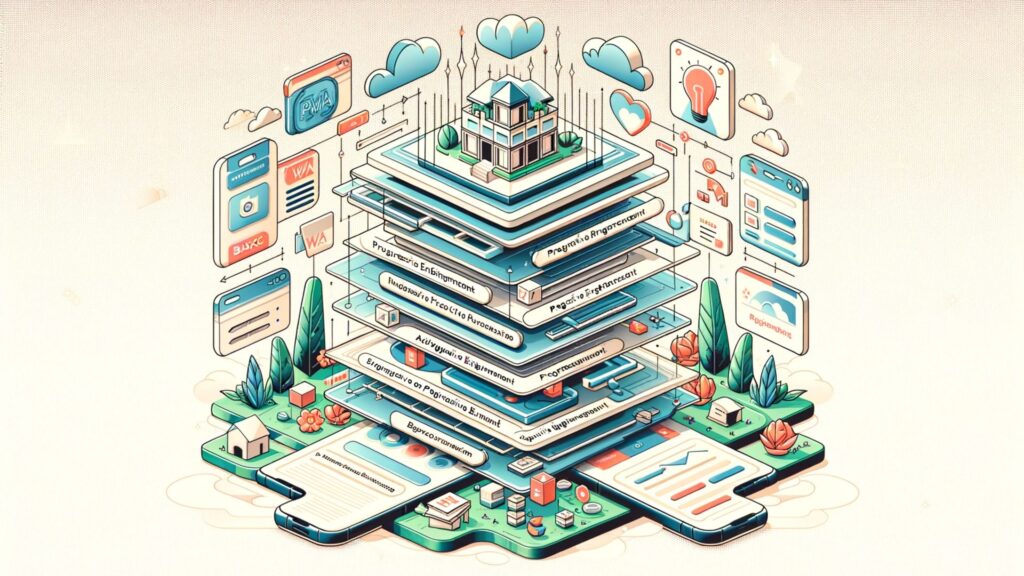
Progressive enhancement, focusing on core webpage content first and then adding advanced features, is becoming more prominent in PWA development. This approach ensures that the PWA remains accessible and functional, even on older browsers or less capable devices.
JavaScript Example for Progressive Enhancement:
// Feature detection for progressive enhancement
if ('serviceWorker' in navigator) {
// Service worker supported: register it
navigator.serviceWorker.register('/service-worker.js');
} else {
// Service worker not supported: Load a fallback script
loadFallbackScript();
}
This example shows how to use feature detection to apply progressive enhancement principles in a PWA.
3. Integration with Emerging Technologies
PWAs are expected to integrate more seamlessly with emerging technologies such as AR/VR, IoT, and AI. This integration will open new avenues for creating immersive and interactive web experiences.
4. Enhanced Offline Capabilities
Future PWAs are likely to offer even more sophisticated offline capabilities, utilizing advanced caching strategies and background synchronization to provide a seamless offline experience.
JavaScript Example for Advanced Caching:
// Advanced caching strategies using service workers
self.addEventListener('fetch', event => {
event.respondWith(
caches.match(event.request)
.then(cachedResponse => {
if (cachedResponse) {
// Use cached response if available
return cachedResponse;
}
// Otherwise, fetch from network and cache the response
return fetch(event.request).then(response => {
return caches.open('dynamic-cache').then(cache => {
cache.put(event.request, response.clone());
return response;
});
});
})
);
});
This script illustrates an advanced caching strategy where fetched responses are dynamically cached for future use.
Conclusion
The journey of Progressive Web Apps (PWAs) marks a significant evolution in the web development landscape. Embracing the core principles of the web—such as universality, flexibility, and accessibility—PWAs have redefined expectations for what web applications can achieve. By leveraging modern JavaScript capabilities, they offer a near-native experience, bridging the gap between web and mobile apps. This convergence has opened up new horizons for businesses and developers, allowing them to create engaging, high-performance applications that reach a broader audience without the constraints of traditional app development.
Looking ahead, the future of PWAs is undoubtedly promising. As web technologies continue to advance, we can expect PWAs to become even more sophisticated, integrating seamlessly with emerging tech trends like AR/VR, IoT, and AI. The key to success in this evolving landscape will be continuous learning and adaptation. Developers who stay up-to-date with the latest advancements in JavaScript and web technologies will be well-positioned to leverage the full potential of PWAs. For businesses, the message is clear: embracing PWAs is not just about keeping pace with current trends, but about being prepared for the future of digital engagement.