Deploying ASP.NET applications on Azure is a journey that combines the robustness of Microsoft’s ASP.NET framework with the flexibility and scalability of Azure’s cloud services. This process not only enhances the performance and availability of web applications but also brings in the benefits of cloud computing such as cost-efficiency, agility, and global reach. Understanding how to effectively deploy ASP.NET apps on Azure can significantly impact the success and efficiency of web applications.
Why Choose Azure for ASP.NET Apps?
Azure, Microsoft’s cloud computing service, provides a comprehensive and flexible platform that supports a wide range of programming languages, tools, and frameworks, including ASP.NET. By deploying ASP.NET apps on Azure, developers can enjoy several benefits:
- Scalability: Azure makes it easy to scale web applications up or down based on demand. This ensures that applications remain responsive during peak times and cost-efficient during quieter periods.
- Integrated Development Environment: With tools like Visual Studio, developers get a seamless experience for building, testing, and deploying applications directly to Azure.
- Security and Compliance: Azure provides advanced security features and compliance protocols to ensure that applications and data are protected.
- Global Reach: Azure’s vast network of data centers allows applications to be hosted near the user base, reducing latency and improving performance.
- Cost-Effective Solutions: Azure offers various pricing models that cater to different needs and budgets, including pay-as-you-go options that can minimize upfront costs.
Understanding ASP.NET and Azure Compatibility
ASP.NET is a widely used framework for building web applications and services. When deploying ASP.NET apps on Azure, it’s essential to understand the compatibility and specific configurations required:
- ASP.NET Versions: Ensure that the version of ASP.NET used in your application is supported by Azure. Azure continuously updates its services to support the latest ASP.NET versions.
- App Service Plans: Choose the right Azure App Service plan that aligns with your application’s requirements in terms of performance, scale, and price.
- Database Services: If your application uses a database, Azure offers SQL Database and Azure Cosmos DB services that can be seamlessly integrated.
Preparing for Azure Deployment
Before deploying an ASP.NET app to Azure, certain preparatory steps are crucial:
- Update and Test Your Application: Ensure your application is updated to the latest ASP.NET version and thoroughly tested.
- Configure App Settings: Adjust configuration settings for Azure deployment, including connection strings and application settings.
- Choose Deployment Method: Azure offers several deployment methods like FTP, Azure DevOps, GitHub Actions, etc. Select the one that suits your workflow.
Preparing Your ASP.NET App for Azure Deployment
The preparation phase is crucial in the deployment process, ensuring that your ASP.NET application is primed for a smooth transition to Azure. This stage involves a series of steps to optimize your application for cloud deployment, addressing aspects like code efficiency, resource organization, and environmental settings.
Code Optimization and Cleanup
Start by refining your codebase:
- Update Libraries and Frameworks: Ensure that all libraries, frameworks, and packages are up to date. This includes upgrading to the latest stable version of ASP.NET.
- Refactor and Optimize Code: Simplify and optimize your code for better performance. Remove unused code, split large methods into smaller, more manageable ones, and ensure adherence to coding standards.
- Implement Asynchronous Programming: For improved scalability and performance, utilize ASP.NET’s support for asynchronous programming. Here’s a basic example:
public async Task<ActionResult> Index()
{
var data = await GetDataAsync();
return View(data);
}
In this example, GetDataAsync
represents an asynchronous operation, like fetching data from a database.
Configuration and Resource Management
Proper configuration and resource management are vital:
- App Settings and Connection Strings: Externalize configuration settings like connection strings and API keys. Use Azure’s application settings to securely store and manage these configurations.
- Database Migrations: If your application uses Entity Framework, ensure all database migrations are up to date. You can use the following Entity Framework commands to manage migrations:
Enable-Migrations
Add-Migration InitialCreate
Update-Database
- Static Files and Content: Organize static files (images, stylesheets, scripts) efficiently. Azure Blob Storage can be used for storing static content, reducing load on the web server.
Testing and Debugging
Testing is critical:
- Unit and Integration Testing: Conduct comprehensive unit and integration testing to catch and fix bugs early in the development cycle.
- Performance Testing: Test the application’s performance to ensure it can handle the expected load. Tools like Azure Load Testing can simulate traffic to your application.
- Security Audits: Perform security audits to identify and fix vulnerabilities. ASP.NET applications should be tested for common security threats like SQL injection, XSS, and CSRF attacks.
Environment-Specific Settings
Finally, tailor your application for the Azure environment:
- Environment Variables: Use environment variables for different deployment stages (development, staging, production). Azure App Service allows setting environment-specific variables.
- Logging and Diagnostics: Implement logging and diagnostic capabilities to monitor the application’s health and performance. Azure provides built-in monitoring and analytics tools like Azure Monitor and Application Insights.
- Dependency Checks: Ensure all dependencies, such as external APIs or database services, are accessible in the Azure environment.
Azure Deployment Options and Tools
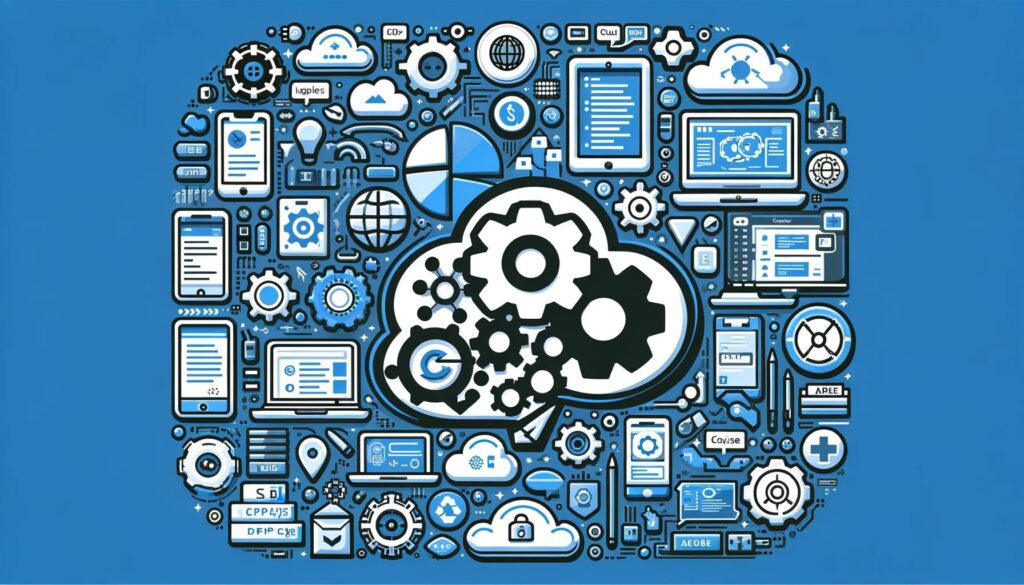
Deploying an ASP.NET application on Azure involves selecting the right tools and services that align with your application’s requirements and your team’s workflow. Azure provides various deployment options, each offering different levels of control and flexibility.
Azure App Service
Azure App Service is a fully managed platform for building, deploying, and scaling web applications. Here’s how you can deploy an ASP.NET application to Azure App Service using Visual Studio:
- Publishing from Visual Studio:
- Right-click on your ASP.NET project in Visual Studio and select ‘Publish’.
- Choose ‘Azure’ as the target, and then select ‘Azure App Service’.
- If you haven’t already, you’ll be prompted to sign in to your Azure account.
- Select or create a new Azure App Service and fill in the necessary details like the resource group and plan.
- Click ‘Publish’. Visual Studio will handle the process of uploading your application to Azure.
// This is a conceptual code snippet showing the deployment process
var azureService = new AzureAppService();
azureService.Deploy("YourAspNetApp");
Azure Kubernetes Service (AKS)
For applications that require more control and scalability, Azure Kubernetes Service (AKS) is an excellent option. It provides a managed Kubernetes environment for deploying containerized applications:
- Creating Docker Containers:
- First, create a Dockerfile in your ASP.NET application project. This file defines how your application will be containerized.
# Use the ASP.NET Core image
FROM mcr.microsoft.com/dotnet/aspnet:5.0 AS base
WORKDIR /app
EXPOSE 80
# Build your application
FROM mcr.microsoft.com/dotnet/sdk:5.0 AS build
WORKDIR /src
COPY ["YourAspNetApp.csproj", "./"]
RUN dotnet restore "YourAspNetApp.csproj"
COPY . .
WORKDIR "/src/."
RUN dotnet build "YourAspNetApp.csproj" -c Release -o /app/build
FROM build AS publish
RUN dotnet publish "YourAspNetApp.csproj" -c Release -o /app/publish
# Final stage/image
FROM base AS final
WORKDIR /app
COPY --from=publish /app/publish .
ENTRYPOINT ["dotnet", "YourAspNetApp.dll"]
- Use Docker commands to build and run the container locally for testing:
docker build -t youraspnetapp .
docker run -d -p 8080:80 --name myapp youraspnetapp
- Deploying to AKS:
- Create an AKS cluster in Azure.
- Push your Docker image to a container registry like Azure Container Registry (ACR).
- Deploy the containerized app to AKS using Kubernetes commands or Azure CLI.
Azure Functions
For smaller, event-driven components, Azure Functions offers a serverless compute service. This is ideal for microservices architecture or when you need to run small pieces of code in response to events.
- Creating an Azure Function in Visual Studio:
- In Visual Studio, create a new Azure Functions project.
- Write the function code. The function can be triggered by various events like HTTP requests, queue messages, etc.
- Publish the function directly to Azure from Visual Studio.
public static class MyHttpTrigger
{
[FunctionName("MyHttpTrigger")]
public static async Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Function, "get", "post", Route = null)] HttpRequest req,
ILogger log)
{
log.LogInformation("C# HTTP trigger function processed a request.");
// Function logic here
}
}
Azure DevOps
For a complete CI/CD pipeline, Azure DevOps provides tools for version control, project management, testing, building, and deploying applications.
- Setting Up a Pipeline:
- Create a new pipeline in Azure DevOps.
- Connect your source code repository.
- Define build and release pipelines that include steps for building the ASP.NET application, running tests, and deploying to Azure.
Step-by-Step Guide to Deploying Your App
Deploying an ASP.NET app to Azure is a streamlined process, especially when using Visual Studio and Azure DevOps. This section provides a step-by-step guide to deploy your app, ensuring a smooth transition to the cloud.
Deploying via Visual Studio to Azure App Service
- Prepare the ASP.NET Application:
- Make sure your application is ready for deployment. This includes any last-minute code checks, database migrations, and configuration settings.
- Publishing Setup in Visual Studio:
- Open your ASP.NET project in Visual Studio.
- Right-click on the project in the Solution Explorer and select ‘Publish’.
- Choose ‘Azure App Service’ as the publish target.
- If prompted, sign in to your Azure account.
- Select an existing App Service or create a new one, specifying the details like subscription, resource group, and app service plan.
- Configure the settings, such as connection strings and environment variables, specific to the Azure environment.
Here’s a snippet to illustrate how you might set up a connection string in your ASP.NET app:
public void ConfigureServices(IServiceCollection services)
{
services.AddDbContext<ApplicationDbContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString("DefaultConnection")));
}
- Deployment:
- After configuring, click ‘Publish’. Visual Studio will package your application and deploy it to the specified Azure App Service.
- Once the deployment is complete, Visual Studio will provide you with the URL to access your application.
Automated Deployment with Azure DevOps
For a more automated approach, especially for teams practicing continuous integration and deployment, Azure DevOps is the tool of choice.
- Setting Up a Build Pipeline:
- In Azure DevOps, create a new build pipeline linked to your code repository (e.g., GitHub or Azure Repos).
- Define the build process, which typically includes restoring NuGet packages, building the solution, running tests, and generating artifacts (the compiled app).
- Creating a Release Pipeline:
- Create a release pipeline that takes the artifacts from the build pipeline.
- Define the deployment process, which includes steps for deploying the artifacts to Azure App Service.
- Set up trigger rules for the pipeline, such as deploying on every successful build or on manual approval.
- Monitoring the Deployment:
- Once the pipeline is triggered, you can monitor the deployment process in Azure DevOps.
- After deployment, check the live site to ensure everything is running as expected.
Using Azure DevOps, you can automate the entire lifecycle of your application, from code commits to deployment, ensuring that your application is always in a deployable state.
Post-Deployment Checks
After deployment, it’s important to perform certain checks:
- Functionality Testing:
- Verify that the application functions correctly in the Azure environment. This includes checking all endpoints, user flows, and integrations.
- Performance Monitoring:
- Use Azure Monitor and Application Insights to track the performance of your application. Look for any unusual activity or performance issues that may need addressing.
- Security Audits:
- Conduct post-deployment security checks to ensure that your application is secure in its new environment.
Database Integration and Management in Azure
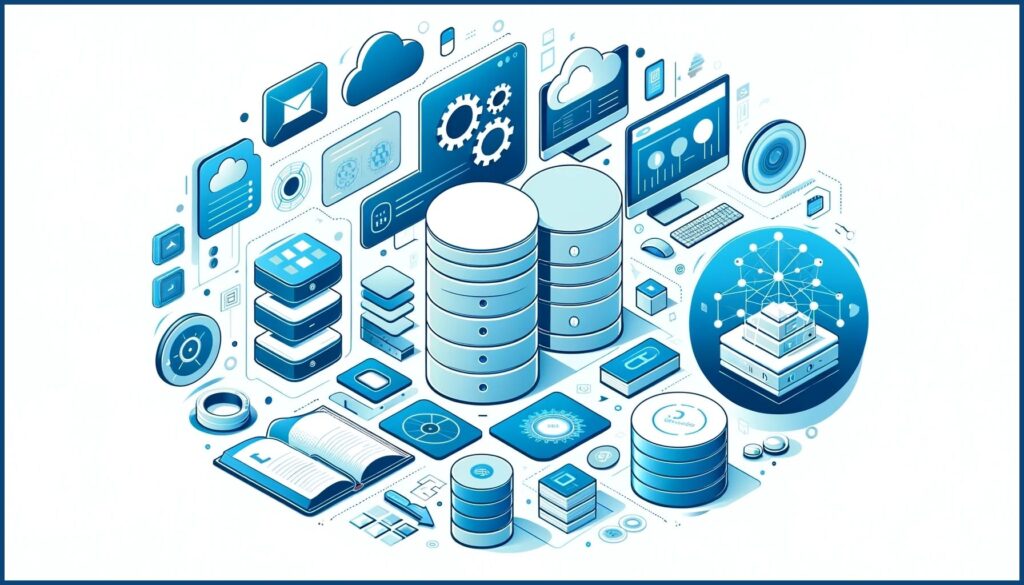
Integrating and managing databases is a pivotal aspect of deploying ASP.NET applications on Azure, especially for applications that rely heavily on data operations. Azure offers several options for database services, most notably Azure SQL Database and Azure Cosmos DB, each catering to different needs.
Integrating Azure SQL Database
Azure SQL Database is a fully managed relational database service that is highly compatible with ASP.NET applications. Here’s how to integrate it:
- Creating an Azure SQL Database:
- In the Azure portal, create a new SQL Database, specifying the server, database name, and pricing tier.
- Note the server URL, database name, and authentication credentials, as you will need these for your connection string.
- Configuring Connection Strings in ASP.NET:
- In your ASP.NET application, update the connection string to point to the Azure SQL Database. This can be done in the appsettings.json file or through the Azure App Service application settings for more security
{
"ConnectionStrings": {
"DefaultConnection": "Server=tcp:your_server.database.windows.net,1433;Initial Catalog=your_database;Persist Security Info=False;User ID=your_username;Password=your_password;MultipleActiveResultSets=False;Encrypt=True;TrustServerCertificate=False;Connection Timeout=30;"
}
}
- Entity Framework Core Integration:
- If you’re using Entity Framework Core, ensure that your DbContext is configured to use the SQL Server provider.
services.AddDbContext<ApplicationDbContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString("DefaultConnection")));
- Migrating Database Schema:
- Use Entity Framework Core migrations to create the database schema in Azure SQL Database.
Update-Database
Working with Azure Cosmos DB
Azure Cosmos DB is a globally distributed, multi-model database service. It’s ideal for applications that require a NoSQL database with high throughput and global distribution.
- Creating a Cosmos DB Account:
- In the Azure portal, create a new Azure Cosmos DB account, selecting the API depending on your needs (e.g., SQL API, MongoDB API).
- Configuring the Cosmos DB SDK in ASP.NET
- Install the necessary NuGet packages for Cosmos DB in your ASP.NET project.
- Configure the Cosmos DB client in your application, providing the endpoint URL and authentication key.
var cosmosClient = new CosmosClient("YourEndpointUrl", "YourAuthKey");
- Data Operations:
- Perform data operations like creating databases, containers, and items using the Cosmos DB SDK.
Database database = await cosmosClient.CreateDatabaseIfNotExistsAsync("YourDatabaseName");
Container container = await database.CreateContainerIfNotExistsAsync("YourContainerName", "/partitionKeyPath");
Security and Backup
- Security: Configure firewalls and virtual networks in Azure to secure your database. Use Azure Active Directory (AAD) for authentication where possible.
- Backup and Restore: Leverage Azure’s built-in backup and restore capabilities to protect your data against loss.
Security Practices for ASP.NET Apps on Azure
Ensuring the security of your ASP.NET application in Azure is paramount. This includes protecting both the application and its data from potential threats. Azure provides several security features and best practices to enhance the security posture of your applications.
Implementing HTTPS
Secure communication is essential for protecting data in transit. Azure App Service provides easy SSL/TLS implementation.
- Enforce HTTPS:
- In your ASP.NET application, enforce HTTPS to ensure all communications are encrypted.
public void Configure(IApplicationBuilder app)
{
app.UseHttpsRedirection();
// Other middleware configurations
}
- SSL/TLS Certificates:
- Azure App Service allows the binding of SSL/TLS certificates to your domain. You can purchase certificates from Azure or upload your own.
Managing Authentication and Authorization
Azure offers integrated authentication and authorization capabilities, making it easier to manage user access.
- Azure Active Directory (AAD):
- Integrate Azure AD to manage user authentication and authorization. Azure AD provides a robust, secure, and scalable identity service.
public void ConfigureServices(IServiceCollection services)
{
services.AddAuthentication(AzureADDefaults.AuthenticationScheme)
.AddAzureAD(options => Configuration.Bind("AzureAd", options));
// Other service configurations
}
- Role-Based Access Control (RBAC):
- Implement RBAC in your application to ensure users have access only to the resources necessary for their role.
Securing Data
Data security is crucial, especially when dealing with sensitive information.
- Azure SQL Database Security:
- Use features like Advanced Data Security, Transparent Data Encryption, and SQL Threat Detection to secure your database.
- Securing Application Settings:
- Store sensitive data like connection strings and API keys in Azure Key Vault instead of hardcoding them in your application.
public void ConfigureServices(IServiceCollection services)
{
var keyVaultEndpoint = new Uri(Environment.GetEnvironmentVariable("VaultUri"));
services.AddAzureKeyVault(keyVaultEndpoint, new DefaultAzureCredential());
// Other service configurations
}
- Input Validation and Anti-Forgery Tokens:
- Validate user inputs to prevent common web vulnerabilities like SQL injection and cross-site scripting (XSS).
- Use anti-forgery tokens in your ASP.NET applications to prevent cross-site request forgery (CSRF) attacks.
[ValidateAntiForgeryToken]
public async Task<IActionResult> Create([Bind("Id,Name,Email")] User user)
{
if (ModelState.IsValid)
{
// Process data
}
return View(user);
}
Monitoring and Auditing
- Azure Monitor and Application Insights: Utilize Azure Monitor and Application Insights for real-time monitoring of your application. They provide valuable insights into the performance and health of your application.
- Audit Logs: Keep track of activities with Azure Audit Logs. Monitoring these logs can help in identifying suspicious activities early.
By implementing these security measures, you can significantly enhance the security of your ASP.NET application on Azure. It is important to adopt a holistic approach to security, encompassing both the application and its underlying infrastructure.
Automating Deployment with Azure DevOps
Automating the deployment process of your ASP.NET application using Azure DevOps can significantly streamline your workflow, ensuring consistent and reliable deployments. Azure DevOps provides an integrated set of features for continuous integration (CI) and continuous deployment (CD), allowing for automated builds, tests, and deployments.
Setting Up a CI/CD Pipeline in Azure DevOps
- Creating a Build Pipeline:
- In Azure DevOps, create a new build pipeline and connect it to your source code repository (e.g., GitHub, Azure Repos).
- Configure the build pipeline to include tasks for restoring NuGet packages, building the ASP.NET solution, running tests, and generating build artifacts.
trigger:
- master
pool:
vmImage: 'windows-latest'
steps:
- task: NuGetToolInstaller@1
- task: NuGetCommand@2
inputs:
restoreSolution: '**/*.sln'
- task: VSBuild@1
inputs:
solution: '**/*.sln'
msbuildArgs: '/p:DeployOnBuild=true /p:WebPublishMethod=Package /p:PackageAsSingleFile=true /p:SkipInvalidConfigurations=true'
platform: 'Any CPU'
configuration: 'Release'
- Creating a Release Pipeline:
- Set up a release pipeline that picks up the artifacts from the build pipeline.
- Define the deployment process, including steps for deploying the artifacts to the Azure App Service.
- Configure pre-deployment and post-deployment conditions, such as approvals or gates.
- In Azure DevOps, you can define these steps in a GUI interface, making it easy to set up and visualize your deployment pipeline.
- Automating Database Migrations:
- If your application uses a database, include steps in your release pipeline to handle database migrations. This can be done using Entity Framework migrations or SQL scripts.
Update-Database -ConnectionString $env:SQLAZURECONNSTR_DefaultConnection -SourceMigration $InitialDatabaseState
- In this script,
Update-Database
is a PowerShell cmdlet from the Entity Framework, and$env:SQLAZURECONNSTR_DefaultConnection
is an environment variable in Azure DevOps that stores the connection string to the Azure SQL Database.
Integrating Tests and Quality Gates
- Automated Testing: Incorporate various types of automated tests in your CI/CD pipeline, such as unit tests, integration tests, and UI tests. Azure DevOps can run these tests as part of the build process.
- Quality Gates: Set up quality gates to ensure that only code that meets certain criteria (e.g., passing all tests, meeting code coverage thresholds) is deployed.
Monitoring and Feedback
- Monitoring Deployments: Use Azure DevOps to monitor the progress and success of your deployments. It provides detailed logs and reports for every build and release.
- Feedback Loops: Establish feedback loops using Azure DevOps and Azure Monitor. This ensures that any issues in the deployment process are quickly identified and addressed.
By leveraging Azure DevOps for CI/CD, you can automate the build and deployment processes, reduce manual errors, and ensure that your ASP.NET application is always in a deployable state. This automation not only saves time but also increases the reliability and consistency of your deployments.
Monitoring and Optimizing App Performance
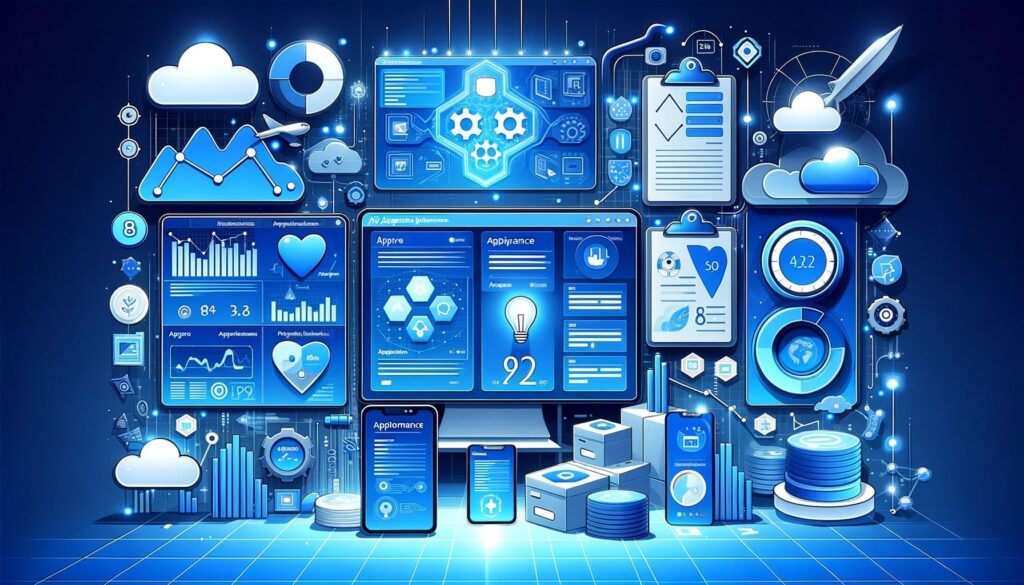
After deploying your ASP.NET application on Azure, monitoring its performance and optimizing it based on real-time data is essential. Azure provides various tools and services to help you monitor your application’s health and performance, ensuring it operates efficiently and reliably.
Implementing Application Insights
Application Insights, a feature of Azure Monitor, is an extensible Application Performance Management (APM) service for developers. It helps you understand how your app is performing and how it’s being used.
- Adding Application Insights to Your ASP.NET App:
- In Visual Studio, you can easily add Application Insights to your project. This involves installing the Application Insights SDK via NuGet and configuring it in your application.
public void ConfigureServices(IServiceCollection services)
{
services.AddApplicationInsightsTelemetry(Configuration["ApplicationInsights:InstrumentationKey"]);
// Other service configurations
}
In the above code, InstrumentationKey
is your unique Application Insights resource key.
- Custom Telemetry Data:
- You can extend Application Insights to collect custom telemetry data that’s specific to your application’s needs.
var telemetry = new TelemetryClient();
telemetry.TrackEvent("CustomEventName", new Dictionary<string, string> { { "Property", "Value" } });
Azure Monitor and Log Analytics
Azure Monitor collects, analyzes, and acts on telemetry data from your Azure and on-premises environments. Azure Log Analytics is a tool within Azure Monitor that helps you to query and visualize log data from your application.
- Setting Up Log Analytics:
- In the Azure portal, create a Log Analytics workspace.
- Integrate your ASP.NET application with the workspace to start collecting logs.
- Querying Logs for Insights:
- Use the Kusto Query Language (KQL) in Log Analytics to write queries and analyze the log data.
- Create dashboards for visualizing log data and gaining insights into application performance.
AppRequests
| where TimeGenerated > ago(1d)
| summarize Count = count() by bin(TimeGenerated, 1h), Success
| render timechart
The above Kusto query visualizes the count of successful and unsuccessful requests to your app over the last day, grouped by hour.
Performance Optimization
- Scaling: Based on the insights gained from monitoring tools, you might need to scale your application up or out. Azure App Service provides easy scaling options.
- Database Optimization: Use the performance recommendations in Azure SQL Database for tuning your database queries and indexes.
- Caching: Implement caching mechanisms like Azure Cache for Redis to improve performance for frequently accessed data.
Automated Alerts
- Setting Up Alerts: Configure automated alerts in Azure Monitor for critical performance metrics or error conditions. This ensures that you are promptly informed of potential issues.
Monitoring and optimizing an ASP.NET application on Azure is a continuous process. By leveraging the powerful tools provided by Azure, you can ensure that your application is not only running but thriving in the cloud environment.
The comprehensive guide provided so far covers all the crucial aspects of deploying, securing, monitoring, and optimizing ASP.NET applications on Azure. With these guidelines and best practices, you can confidently manage the lifecycle of your application on the Azure platform.
Troubleshooting Common Deployment Issues
Deploying an ASP.NET application on Azure can sometimes encounter issues. Effective troubleshooting is key to quickly resolving these problems and ensuring your application runs smoothly. Here are some common issues and how to address them:
Issue 1: Deployment Failures
If your deployment fails, the first step is to check the deployment logs in Azure.
- Viewing Logs in Azure Portal:
- Navigate to your App Service in the Azure portal.
- Under the “Development Tools” section, select “App Service Logs”.
- Enable application logging and view the logs for any errors during deployment.
- Common Causes:
- Misconfigured settings in the
web.config
file or incorrect connection strings. - Compatibility issues with Azure App Service, like unsupported frameworks or libraries.
- Misconfigured settings in the
Issue 2: Database Connectivity Problems
Troubleshooting database connectivity issues often involves checking connection strings and network configurations.
- Testing Connection Strings:
- Ensure that the connection strings in your application settings are correct.
- Test the connection string locally to see if the issue is with Azure or your application.
- Azure SQL Database Firewall Settings:
- In the Azure portal, navigate to your SQL Database.
- Under “Security”, check the “Firewall and virtual networks” settings.
- Ensure that your App Service is allowed to connect to the database.
Issue 3: Performance Issues
Performance issues post-deployment can stem from inadequate resources or inefficient application code.
- Application Insights:
- Use Application Insights to diagnose performance issues.
- Check for long-running requests, database query delays, or high memory usage.
- Scaling Up or Out:
- Consider scaling up your App Service plan for more CPU or memory.
- Alternatively, scale out to add more instances for load balancing.
Set-AzAppServicePlan -ResourceGroupName "YourResourceGroup" -Name "YourAppServicePlan" -Tier "Standard" -WorkerSize "Medium"
This PowerShell command scales up your App Service plan to the Standard tier with medium-sized instances.
Issue 4: SSL/TLS Certificates Not Working
If your SSL/TLS certificates are not functioning correctly:
- Certificate Binding:
- Check the SSL settings in your App Service in the Azure portal.
- Ensure that the certificate is correctly bound to your custom domain.
- HTTPS Redirection:
- Verify that your application is properly configured to redirect HTTP to HTTPS.
public void Configure(IApplicationBuilder app)
{
app.UseHttpsRedirection();
// Other middleware configurations
}
By systematically addressing these common issues, you can effectively troubleshoot and resolve deployment challenges in Azure. Remember, a proactive approach to monitoring and regular application maintenance can prevent many of these issues from occurring in the first place.
This concludes our comprehensive guide on deploying ASP.NET applications on Azure. From initial setup and security considerations to performance monitoring and troubleshooting, following these guidelines will help you leverage Azure’s capabilities to the fullest, ensuring a robust and efficient cloud presence for your ASP.NET applications.
Conclusion: Mastering ASP.NET Deployment on Azure for Long-Term Success
In conclusion, deploying ASP.NET applications on Azure signifies a strategic shift towards utilizing the cloud’s scalability, security, and efficiency. This comprehensive journey, encompassing automation through Azure DevOps, vigilant security practices, continuous performance monitoring, and a user-centric approach, not only enhances application management but also aligns it with evolving user needs and expectations. Embracing Azure’s dynamic ecosystem for your ASP.NET applications prepares you for the future, ensuring readiness for emerging technologies and trends in cloud computing. This approach sets a solid foundation for growth, innovation, and long-term success in the rapidly evolving digital landscape.