When you think about modern web development, Single Page Applications (SPAs) are a crucial element. They represent a shift from traditional webpage design, offering a more dynamic and fluid user experience. Essentially, an SPA is a web application or site that reloads parts of a page rather than refreshing the entire page when new content is needed. This results in a smoother, more app-like experience.
What is an SPA?
The term SPA might sound complex, but the concept is straightforward. In a typical website, navigating between pages involves loading new HTML pages from the server, which can cause delays and disrupt the user experience. An SPA, on the other hand, loads a single HTML page when you first visit the site. As you interact with the site, it dynamically updates the content using JavaScript, without the need to reload the entire page. This approach makes the website feel more responsive and quick.
Why JavaScript for SPAs?
JavaScript plays a pivotal role in SPAs. It’s the engine behind the dynamic loading of content and the seamless user interactions that characterize these applications. JavaScript allows developers to manipulate the Document Object Model (DOM), enabling the updating of content without page reloads. This manipulation is crucial for the SPA’s responsive feel.
Moreover, JavaScript’s compatibility with various frameworks and libraries makes it an ideal choice for SPA development. It’s versatile and widely supported, which means SPAs built with JavaScript can run smoothly across different browsers and devices.
The Advantages of SPAs
SPAs offer several benefits that make them popular among developers and users alike:
- Improved User Experience: By eliminating the need to reload the entire page, SPAs provide a smoother and faster experience. This is especially noticeable in applications that require frequent user interactions.
- Reduced Server Load: Since much of the content processing is done on the client side, SPAs can reduce the load on servers. This can lead to better performance, especially for applications with high user volumes.
- Development Efficiency: With the SPA architecture, developers often find it easier to manage and maintain their codebase. Frameworks and libraries tailored for SPAs further streamline the development process.
By understanding the basics of SPAs, you’re taking the first step into a field that’s shaping the future of web development. Whether you’re a seasoned developer or just starting, mastering SPAs can open up new opportunities and enhance the quality of your web projects.
Setting the Stage: Preparing Your Development Environment
Before you start building your SPA in JavaScript, it’s essential to set up your development environment correctly. This setup forms the foundation of your project, ensuring that you have all the necessary tools and files in place.
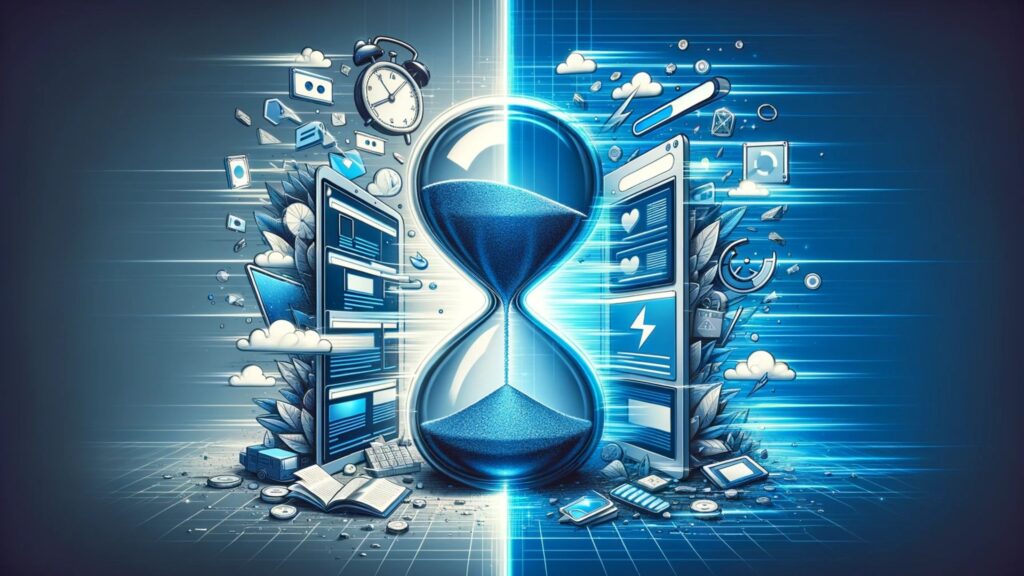
Essential Tools for SPA Development
First, let’s talk about the tools you need:
- Text Editor or IDE: Choose a text editor or Integrated Development Environment (IDE) where you will write your code. Popular options include Visual Studio Code, Sublime Text, and Atom. These editors offer features like syntax highlighting and auto-completion, which are very helpful in coding.
- Web Browser: A modern web browser like Chrome, Firefox, or Edge is crucial. These browsers come with developer tools that are invaluable for debugging JavaScript and inspecting the DOM.
- Node.js and NPM: Node.js is a JavaScript runtime that lets you run JavaScript on the server side. NPM (Node Package Manager) comes with Node.js and helps manage libraries and dependencies for your project.
- Git (Optional): For version control, Git is highly recommended. It allows you to track changes, revert to previous versions, and collaborate with others.
Creating the Basic File Structure
Next, you will create the basic structure of your SPA. This typically includes three main files:
1. index.html: This is the main HTML file. It acts as the single page in your SPA. Here, you will include the necessary HTML structure and links to your JavaScript and CSS files.
Example of a basic index.html:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Your SPA Title</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div id="app"></div>
<script src="app.js"></script>
</body>
</html>
2. styles.css: This CSS file will hold the styling for your SPA. Initially, it can be simple, but as your project grows, this file will help you maintain the visual aspect of your SPA.
Example of a basic styles.css:
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
background-color: #f4f4f4;
}
3. app.js: This JavaScript file is where the magic happens. You will write the JavaScript code that controls your SPA here. Initially, it might contain simple DOM manipulations or event listeners.
Example of a basic app.js:
document.addEventListener('DOMContentLoaded', function() {
// Your SPA's JavaScript code goes here
});
Getting Ready for Development
Once you have these tools and files set up, you’re ready to start building your SPA. Remember, the key to a successful SPA is not just in the coding, but also in how well you prepare your development environment. A good start sets the tone for the entire development process.
Core Concepts of a JavaScript SPA
In this part of our journey into building a Single Page Application (SPA) using JavaScript, we focus on two crucial concepts: DOM manipulation and client-side routing and state management. These concepts are the building blocks of any SPA.
Understanding DOM Manipulation and Its Role in SPAs
DOM (Document Object Model) manipulation is at the heart of how SPAs function. The DOM is a programming interface for web documents. It represents the page so that programs can change the document structure, style, and content. In an SPA, when you interact with the page, rather than reloading a new page, JavaScript manipulates the DOM to update the view dynamically.
For example, when you click a button in an SPA, instead of sending a request to the server for a new page, a JavaScript function might run to update the content of a div element on the current page. This process, where the page’s content is updated without a full page load, is what makes SPAs so responsive and quick.
JavaScript provides various methods for DOM manipulation, like getElementById, addEventListener, and innerHTML. These methods allow you to easily select elements on the page, respond to user actions, and update the content displayed to the user.
Implementing Client-side Routing in SPAs
In traditional websites, navigating to a new page involves a request to the server and loading a new document. However, in an SPA, client-side routing allows the user to navigate between different parts of the application without a full page refresh.
Client-side routing in SPAs is handled by JavaScript. When a user clicks a link, JavaScript catches the URL change and renders the appropriate content. For example, JavaScript can hide or show certain sections of the page, mimicking the experience of navigating to a different page.
This is achieved using the HTML5 History API, which allows you to change the URL without reloading the page. JavaScript listens for changes in the URL and updates the page content accordingly, giving the illusion of navigating between pages.
State Management in SPAs
State management is about keeping track of how data changes over time in your application. In an SPA, you need to manage the state on the client side. This can involve tracking things like user inputs, data retrieved from AJAX calls, and the current UI state.
JavaScript objects or more sophisticated state management libraries like Redux can be used to handle state. The idea is to have a central place where your application’s state is stored. Whenever the state changes, the view is updated to reflect these changes, ensuring that the user interface is always in sync with the underlying data.
For example, in a to-do list SPA, the state might consist of a list of tasks. When a new task is added, the state is updated, and the view is refreshed to show the new task. This keeps the UI consistent with the data.
Designing the SPA Layout and Structure
Creating a layout and structure for your Single Page Application (SPA) is a critical step in making it user-friendly and responsive. A well-thought-out design ensures that users can navigate your application smoothly and find what they need without confusion. Here, we’ll cover some tips for designing your SPA’s interface and using CSS techniques to manage views effectively.
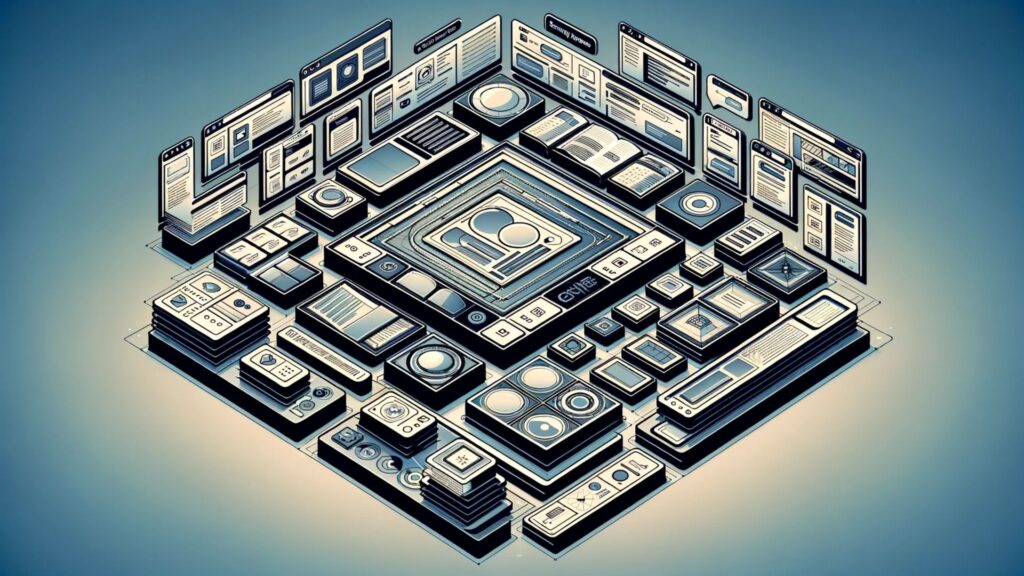
Designing User-Friendly and Responsive SPA Interfaces
- Keep It Intuitive: Your SPA’s layout should be straightforward. Users should be able to navigate easily without having to guess what to do next. Standard layouts with familiar elements like navigation bars, headers, and footers can be beneficial.
- Responsive Design: Ensure that your SPA looks good and functions well on all devices. Using a responsive design framework like Bootstrap can simplify this process. It helps your application adjust to different screen sizes, from mobile phones to desktop monitors.
- Loading Indicators: SPAs often fetch data asynchronously. It’s important to provide visual feedback, like a spinner or a progress bar, when data is being loaded. This keeps the user informed that the application is working in the background.
- Error Handling: Design your SPA to gracefully handle errors. For instance, if data fails to load, show a friendly error message and provide options to retry or go back.
CSS Techniques for Managing Views in a SPA
In an SPA, you often switch between different views without loading a new page. CSS plays a crucial role in showing and hiding these views.
1. Using CSS Classes for Show/Hide: You can use CSS classes to control the visibility of different sections of your SPA. For instance, you might have a class .hidden with display: none; to hide elements and remove the class to show them when needed.
Example:
.hidden {
display: none;
}
2. Transition Between Views: For a smoother user experience, use CSS transitions when switching views. This can be done by changing the opacity or using animations to slide elements in and out.
3. Grid Layout for Structure: Utilize CSS Grid or Flexbox to create a flexible and responsive layout. These CSS techniques allow you to define a grid-based layout system that adjusts automatically to screen sizes, making your SPA more responsive.
4. Consistency in Design: Maintain consistent styling throughout your SPA. Use a style guide or CSS variables to keep your color schemes, font sizes, and other design elements uniform.
5. Optimize for Speed: Keep your CSS efficient and minimal. Overly complex CSS can slow down your SPA, so it’s important to optimize your stylesheets for performance.
Data Retrieval and Management in SPAs
In Single Page Applications (SPAs), managing and retrieving data efficiently is key to ensuring a seamless user experience. This section will focus on using AJAX for data handling and implementing web storage for data management.
Making AJAX Calls and Handling JSON Data
1. Understanding AJAX in SPAs: AJAX (Asynchronous JavaScript and XML) allows your SPA to request, receive, and send data from and to a server asynchronously, without reloading the page. In SPAs, this means you can update the user interface in real-time as data is fetched or submitted.
2. Using JavaScript for AJAX: Modern JavaScript offers several methods for making AJAX calls, such as the fetch API or XMLHttpRequest. The fetch API is more modern and provides a more powerful and flexible feature set.
Example of a fetch request:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
3. Handling JSON Data: JSON (JavaScript Object Notation) is a common format for sending and receiving data in web applications. After retrieving data in JSON format via AJAX, you can easily parse and use it within your SPA. JavaScript’s JSON.parse() and JSON.stringify() methods are used for converting between JSON text and JavaScript objects.
Utilizing Web Storage for Efficient Data Management
- Leveraging Local Storage and Session Storage: Web storage, like local storage and session storage, provides a way to store key-value pairs in the browser. Local storage is persistent across sessions, while session storage is cleared when the session ends (like when a tab is closed).
- Benefits of Web Storage in SPAs: By using web storage, you can keep certain data on the client side, reducing the need for repetitive AJAX calls. This is especially useful for data that doesn’t change often, like user preferences or static data used across different parts of the SPA.
- Implementing Web Storage: Storing and retrieving data is straightforward. You can set items in storage and retrieve them when needed, thus enhancing the performance of your SPA by reducing server requests. Example of using local storage:
// Storing data
localStorage.setItem('user', JSON.stringify({ name: 'Alice', age: 25 }));
// Retrieving data
const user = JSON.parse(localStorage.getItem('user'));
console.log(user.name); // Output: Alice
4. Caching Strategies: For optimal performance, implement caching strategies. You can cache data fetched from AJAX calls in local storage and retrieve it from there on subsequent requests, falling back to a new AJAX call only if the data is not found or is outdated.
Advanced SPA Features and Best Practices
As you become more comfortable with the basics of Single Page Application (SPA) development, incorporating advanced features and following best practices can significantly enhance the performance and scalability of your application. This section will focus on templating, event delegation, and optimization techniques.
Incorporating Templating in SPAs
- Role of Templating: Templating engines allow you to define templates for your app’s views. These templates are then filled with data dynamically. This approach simplifies the process of rendering content, especially when dealing with large amounts of data or complex UI structures.
- Popular Templating Libraries: There are several JavaScript templating libraries available, such as Handlebars, Mustache, and EJS. These libraries provide a simple syntax to bind data to your HTML views. This method enhances code readability and maintainability.
Example of using Handlebars:
<script id="entry-template" type="text/x-handlebars-template">
<div class="entry">
<h1>{{title}}</h1>
<div class="body">{{body}}</div>
</div>
</script>
Event Delegation in SPAs
- Understanding Event Delegation: Event delegation is a technique where you assign a single event listener to a parent element, rather than individual listeners to each child. This approach is more efficient, especially for elements that dynamically change or get added to the DOM.
- Benefits: It improves performance by reducing the number of event listeners and handles dynamically added elements without the need to bind new listeners.
Example of event delegation:
document.getElementById('parent-element').addEventListener('click', function(e) {
if (e.target && e.target.matches('button.class')) {
// Handle button click
}
});
SPA Optimization Techniques for Performance and Scalability
- Optimizing Load Times: Use techniques like code splitting and lazy loading to reduce the initial load time of your SPA. This involves breaking your JavaScript bundle into smaller chunks and loading them only when needed.
- Caching Strategies: Implement effective caching strategies to reduce server load and speed up content delivery. This includes browser caching, service workers, and leveraging local storage for data caching.
- Minimizing HTTP Requests: Minimize the number of HTTP requests by combining files, using sprite sheets for images, and compressing assets. Each request adds to the load time, so reducing them can have a significant impact on performance.
- Server-Side Rendering (SSR): For SPAs, consider using server-side rendering for the initial page load. This can improve the load time and is beneficial for SEO as it allows web crawlers to index the content more effectively.
- Monitoring and Analysis: Regularly monitor your SPA’s performance using tools like Google Lighthouse or WebPageTest. Analyze the reports to identify bottlenecks and areas for improvement.
Testing and Debugging Your SPA
Effective testing and debugging are crucial for building a robust and reliable Single Page Application (SPA). This stage ensures that your application not only functions correctly but also provides a consistent experience across various platforms and browsers. Let’s explore the tools and strategies you can use to test and debug your SPA, as well as how to ensure cross-browser compatibility.

Tools and Strategies for Effective Testing and Debugging
- Unit Testing: Unit tests are essential for any SPA. They help you test individual components or functions in isolation. Frameworks like Jest or Mocha provide a robust testing environment where you can write and run tests to validate each part of your application.
- End-to-End Testing: Tools like Cypress or Selenium allow for end-to-end testing. This involves testing your SPA as a whole, simulating real user interactions to ensure all parts of your application work together seamlessly.
- Integration Testing: This tests the integration of different components of your SPA. It’s crucial to ensure that combined components or services work as expected.
- Using Developer Tools for Debugging: Modern browsers offer comprehensive developer tools for debugging. These tools allow you to inspect the DOM, debug JavaScript, view network requests, and analyze performance.
- Logging and Error Tracking: Implement logging and use tools like Sentry for real-time error tracking. This helps in quickly identifying and fixing issues that users encounter.
Ensuring Cross-Browser Compatibility
- Testing Across Browsers: Use tools like BrowserStack to test your SPA across different browsers and devices. This ensures that your application functions correctly and looks consistent on all platforms.
- Polyfills and Transpilers: Use polyfills to provide functionality that older browsers might not support. Transpilers like Babel allow you to write modern JavaScript while ensuring compatibility with older browsers.
- Responsive Design Testing: Ensure your SPA’s design is responsive and adapts to different screen sizes and resolutions. Tools like Chrome DevTools can simulate various devices for testing responsiveness.
Resolving Common Issues
- Handling Asynchronous Operations: SPAs heavily rely on asynchronous operations. Ensure you handle promises and async/await correctly to avoid uncaught exceptions or unexpected behavior.
- Addressing Performance Issues: If your SPA faces performance issues, consider optimizing resource loading, minimizing HTTP requests, and efficiently managing state and DOM updates.
- Solving Routing Problems: Client-side routing can sometimes be tricky, especially with browser history and URL changes. Ensure your routing logic is robust and handles all scenarios gracefully.
Testing and debugging are ongoing processes that require attention throughout the development cycle. By employing these strategies, tools, and best practices, you can build an SPA that is not only functional but also reliable and consistent across various platforms and browsers.
Conclusion
We’ve explored the intricate world of Single Page Applications (SPAs) in JavaScript, covering everything from setting up a development environment to implementing advanced features and best practices. We delved into core concepts like DOM manipulation and client-side routing, emphasized the importance of responsive design and efficient data management, and highlighted the necessity of rigorous testing and debugging for cross-browser compatibility. Looking ahead, the evolution of SPAs is set to embrace trends like Progressive Web Apps, Server-Side Rendering, and API-first development, all aimed at enhancing user experience and application performance. As we continue to witness technological advancements, the skills and principles in building SPAs will remain crucial in the ever-evolving landscape of web development.