Introduction to Advanced PHP
PHP, a server-side scripting language designed for web development, has undergone significant evolution since its inception in 1995. As the complexity of web applications grew, so did the need for more sophisticated programming features. The introduction of advanced concepts like Namespaces, Traits, and Interfaces marked a significant leap in PHP’s capabilities, allowing developers to build more robust, scalable, and maintainable applications.
Why Advanced Features?
In the early days, PHP was simple, with a primary focus on small to medium-sized applications. However, as technology advanced and the demand for complex applications increased, PHP’s basic features were no longer sufficient. Developers needed tools that could handle a higher level of abstraction, promote code reuse, and manage large codebases efficiently. This need led to the inclusion of advanced features that supported object-oriented programming (OOP) practices, a paradigm centered around objects rather than actions, and data rather than logic.
Namespaces, Traits, and Interfaces: The Pillars of Advanced PHP
- Namespaces: As PHP applications grew larger, code organization became a challenge. Namespaces address this issue by allowing developers to group logically related classes, interfaces, constants, and functions under a named hierarchy. This system prevents code conflicts and makes the codebase more readable and maintainable.
- Traits: PHP supports single inheritance, meaning a class can inherit only from one parent class. Traits overcome this limitation by enabling developers to reuse sets of methods freely in several independent classes. They provide a way to reduce code duplication and ensure a cleaner and more organized structure.
- Interfaces: An interface is a contract that defines what methods a class should implement, without specifying how these methods should be handled. Interfaces are crucial in enforcing a structure and ensuring that certain blocks of code adhere to a particular protocol. They are particularly useful when different classes need to implement the same functionality in varied ways.
Understanding Namespaces
In this section, we’ll explore how namespaces can significantly improve the structure and maintainability of your PHP code.
What are Namespaces?
Namespaces are a fundamental feature in PHP that allow for better organization of code. They enable you to group related classes, interfaces, functions, and constants together. This organization is vital in avoiding name collisions between code components that might otherwise share the same name. Think of a namespace as a directory structure for your classes and interfaces; it’s a way to ensure that one class called File in one part of your program doesn’t accidentally interfere with another File class elsewhere.
Declaring and Using Namespaces
To declare a namespace in PHP, you use the namespace keyword at the top of your PHP file. It’s important to note that it must be the first command in the file, with the exception of any declare statements. Here’s a simple example:
namespace MyProject\Tools;
class File {...}
In this snippet, the File class is part of the MyProject\Tools namespace. To use this class elsewhere, you’d reference it by its fully qualified name unless you import it using the use keyword:
use MyProject\Tools\File;
$file = new File();
This approach keeps your code clean and avoids the potential for naming conflicts.
Benefits of Using Namespaces
- Organization: Namespaces allow you to group related code, making your application more organized and manageable.
- Avoiding Name Conflicts: They prevent naming collisions by segregating your code into distinct areas.
- Better Autoloading: Modern PHP relies heavily on autoloading classes. Namespaces make autoloading more straightforward and efficient.
The Power of PHP Traits
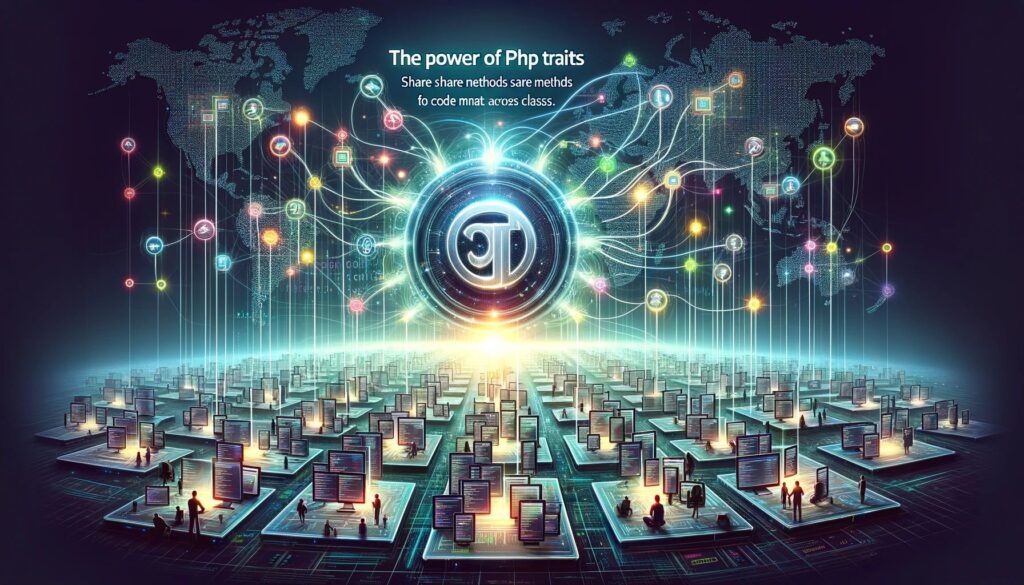
Traits in PHP offer a streamlined solution for code reuse and flexibility. By allowing developers to share methods across classes without complex inheritance, traits enhance code maintainability and readability, paving the way for more modular and adaptable applications.
Defining Traits
Traits in PHP are a method of code reuse. They are similar to classes but are intended to group functionality in a fine-grained and consistent way. They allow you to create reusable code components that can be included in multiple classes. Unlike classes, however, traits cannot be instantiated on their own.
Here’s how you might declare a trait:
trait Sharable {
public function share($item){
echo "Sharing {$item}";
}
}
Using Traits in Your Classes
To use a trait in a class, you use the use keyword inside the class. For instance:
class Post {
use Sharable;
// Other class properties and methods
}
Now, any Post object will have access to the share method defined in the Sharable trait.
Advantages of Using Traits
- Code Reuse: Traits help in reducing code duplication. Instead of repeating the same method in multiple classes, you can define it once in a trait and include it wherever needed.
- Flexibility: They provide a lot of flexibility and can be used to extend the functionality of classes in a way that might not be possible with traditional inheritance.
- No Inheritance Conflicts: Since PHP does not support multiple inheritances, traits offer a way to import methods from multiple sources without conflicts.
Diving Deep into Interfaces
Interfaces in PHP are crucial for defining consistent, enforceable contracts for classes. They promote a clear structure and flexible architecture, ensuring that disparate classes can work together seamlessly while maintaining code integrity.
The Concept of Interfaces
Interfaces are a way to ensure that certain classes adhere to a particular contract. An interface is not a class but a type of contract that classes can sign. When a class implements an interface, it agrees to implement all the methods declared within that interface.
Here’s a simple interface declaration:
interface Shareable {
public function share();
}
Implementing Interfaces in PHP
To implement an interface, a class must use the implements keyword. A class that implements an interface must provide an implementation for all of the interface’s methods:
class Post implements Shareable {
public function share(){
echo "Sharing post";
}
}
Benefits of Using Interfaces
- Consistency: Interfaces ensure that different classes offer the same set of methods, promoting consistency.
- Flexibility: They allow for flexible and loosely coupled designs. You can change the implementations of your interfaces without changing how your classes use them.
- Documentation: They serve as a clear guide to what a class should do, acting as a form of documentation.
Best Practices for Using Namespaces, Traits, and Interfaces
Adopting best practices for Namespaces, Traits, and Interfaces is key to maximizing their benefits. It ensures organized, efficient, and robust PHP applications, enhancing both code quality and developer productivity.
Effective Use of Namespaces
- Consistent Structure: Adopt a naming convention that mirrors your project’s directory structure to maintain consistency and predictability.
- Alias Long Namespaces: Use the use keyword with an alias to shorten long namespace names in your code, enhancing readability.
- Avoid Global Space Conflicts: Always define a namespace for your classes, even if it’s a simple project, to prevent conflicts with PHP’s internal classes and third-party libraries.
Maximizing Traits
- Single Responsibility: Each trait should have a single responsibility. Just like with classes, if you find a trait growing too large, consider breaking it into smaller, more focused traits.
- Conflict Resolution: Be mindful of name conflicts when using multiple traits. PHP allows you to resolve conflicts manually, but it’s better to design your traits to avoid these conflicts altogether.
- Complementary to Classes: Remember, traits are meant to complement, not replace, inheritance. Use them to share methods among classes, not to define entire classes.
Implementing Interfaces Effectively
- Define Clear Contracts: Ensure your interfaces define a clear and consistent contract that implementing classes must follow. Avoid changing an interface once it’s widely used, as this will break all implementing classes.
- Type Hinting: Use interfaces for type hinting in functions and methods to ensure that passed objects adhere to a specific contract.
- Separation of Concerns: Use interfaces to separate high-level policy from low-level implementation details, allowing you to change the implementation without affecting the policy.
Integrating Namespaces, Traits, and Interfaces
Integrating these three components effectively can dramatically improve the quality and maintainability of your code. Here’s how you might bring them together in a real-world scenario:
Scenario: Imagine you’re building a content management system with various types of content, like posts, pages, and products, each shareable on different platforms.
- Namespaces for Organization: Organize your classes into namespaces based on their functionality, like CMS\Content for content-related classes and CMS\Sharing for sharing-related functionality.
- Traits for Common Behaviors: Create traits for shared behaviors across different classes. For instance, a Sharable trait in the CMS\Sharing namespace could provide a method to share content items on social media.
- Interfaces for Contracts: Define interfaces for each type of content. For example, a ContentInterface in the CMS\Content namespace might require methods like getTitle() and getDescription(). Each content type (post, page, product) implements this interface, ensuring they all provide the necessary methods.
Example Integration:
namespace CMS\Content;
interface ContentInterface {
public function getTitle();
public function getDescription();
}
namespace CMS\Sharing;
trait Sharable {
public function share($platform) {
// Implementation for sharing on specified platform
}
}
namespace CMS\Content;
class Post implements ContentInterface {
use \CMS\Sharing\Sharable;
public function getTitle() { /* ... */ }
public function getDescription() { /* ... */ }
}
In this scenario, Namespaces keep your code organized, Traits allow you to reuse the sharing functionality, and Interfaces ensure that all content types adhere to a specific structure.
Conclusion
In this article, we’ve explored some of the advanced features of PHP — Namespaces, Traits, and Interfaces — and how they can be used to create more structured, efficient, and scalable applications. By understanding and implementing these concepts, you can take your PHP development to new heights, producing code that’s not just functional but also clean, maintainable, and robust.
Remember, the journey of learning never ends, especially in a field as dynamic as web development. Keep experimenting, keep learning, and most importantly, keep coding!