The landscape of web development is constantly evolving, with PHP and RESTful API development at its core. These technologies continue to play pivotal roles in shaping how web applications are designed, developed, and deployed. This section delves into the essence of PHP and RESTful APIs, highlighting their significance and the synergy they bring to modern web development.
Understanding PHP in Web Development
PHP, a server-side scripting language, recently marked its 25th anniversary, showcasing its remarkable evolution. Originally a template parsing system, PHP now boasts full object-oriented capabilities, an optional strict-typing system, a Just-In-Time compiler, extensive database support, and robust date and time processing tools. This enduring technology continues to play a vital role in modern web development, with its versatility and feature-rich nature making it a cornerstone of the industry.
The Role of RESTful APIs in Web Development
APIs have emerged as the foundational architecture for contemporary web and mobile applications. These APIs enable diverse software systems to communicate online using a standardized approach, streamlining data interchange. These qualities collectively contribute to the enduring popularity and utility of RESTful APIs in the digital landscape.
PHP and RESTful APIs: A Synergistic Relationship
The pairing of PHP and RESTful APIs is a powerful force in web development. PHP’s server-side scripting, coupled with RESTful APIs’ system connectivity, creates a robust platform for dynamic web apps. It excels in e-commerce, content management, and real-time data apps. PHP’s database integration and RESTful APIs’ request-handling prowess make them a top choice for fast, secure, and scalable web apps.
Current State of PHP in Web Development
PHP’s journey over the past 25 years has been remarkable, evolving from a simple scripting language to a powerful tool for creating sophisticated web applications. PHP’s widespread adoption is primarily due to its simplicity, flexibility, and continuous improvements, making it a preferred choice for web developers.
PHP’s Evolution and Adoption
PHP has continuously evolved to meet the demands of modern web development. With the introduction of PHP 8.0, developers now have access to features like:
- Just-In-Time (JIT) Compiler: Enhancing performance by compiling PHP code into machine code at runtime.
- Named Arguments: Improving code readability and flexibility.
- Union Types: Enabling PHP functions to accept multiple types of data.
PHP and Cloud Integration
The integration of PHP with cloud services marks a significant trend in its evolution. Cloud computing’s scalability and flexibility, combined with PHP’s efficiency, create a potent combination for web development. Businesses are increasingly leveraging PHP for cloud-based solutions, taking advantage of features like:
- Enhanced performance and scalability
- Reduced costs with efficient resource utilization
- Simplified deployment and management processes
PHP in IoT Applications
PHP’s role in the Internet of Things (IoT) is another emerging trend. IoT requires a dynamic programming environment, and PHP fits well in this space. PHP frameworks like Laravel and Symfony are increasingly being used for IoT applications due to their:
- Flexibility in handling diverse data types
- Efficient database integration capabilities
- Robust security features
PHP in the Developer Community
The PHP community has been a significant factor in the language’s success. With a vast array of resources, forums, and extensive documentation, the PHP community actively contributes to the language’s development and provides support for new and experienced developers alike.
RESTful API Development: Best Practices and New Approaches
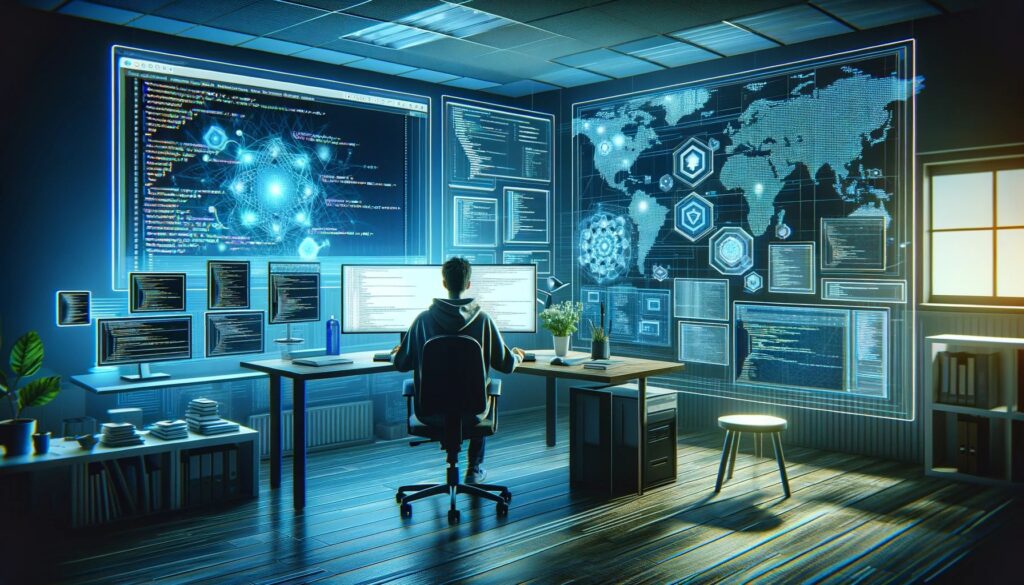
RESTful API development has become a cornerstone in creating scalable, efficient, and interoperable web applications. Emphasizing simplicity and statelessness, RESTful APIs facilitate seamless communication between diverse systems over the web.
Embracing Best Practices in RESTful API Development
The design and implementation of RESTful APIs involve adhering to key principles to ensure they are efficient, scalable, and maintainable:
- Resource Identification: Each resource, like a user or product, should have a unique URI.
- Statelessness: Each request from client to server must contain all the information needed to understand and process the request.
- Cacheability: Responses should be implicitly or explicitly labeled as cacheable or non-cacheable.
- Layered System: A client cannot ordinarily tell whether it is connected directly to the end server or to an intermediary along the way.
Leveraging New API Architectures
While REST APIs remain prevalent, the adoption of newer architectures like GraphQL, AsyncAPI, and gRPC is growing. These architectures address specific limitations of REST by offering more efficient data retrieval (GraphQL), better support for event-driven architectures (AsyncAPI), and improved performance (gRPC).
Code Example: Building a Simple RESTful API with PHP
Here’s a basic example of creating a RESTful API in PHP. This example demonstrates a simple API to manage a list of users:
<?php
// A simple RESTful API in PHP to manage users
header("Content-Type: application/json");
$users = [
['id' => 1, 'name' => 'Alice'],
['id' => 2, 'name' => 'Bob'],
];
$method = $_SERVER['REQUEST_METHOD'];
$uri = $_SERVER['REQUEST_URI'];
// Handling GET request to list users
if ($method == 'GET' && $uri == '/users') {
echo json_encode($users);
}
// Add more methods (POST, PUT, DELETE) to handle other CRUD operations
?>
This code provides a basic structure for handling HTTP GET requests. To develop a full-fledged RESTful API, you would expand this to include POST, PUT, and DELETE methods, alongside proper error handling and data validation.
The Shift Towards Low-Code and No-Code API Development
The proliferation of low-code and no-code API development tools is a significant trend. These tools allow developers and non-developers alike to create APIs through intuitive interfaces, dramatically simplifying the API development process and reducing the time to market. This democratization of API development is reshaping the landscape, making API creation more accessible to a broader audience.
Improvement in API Security Standards
With the increasing reliance on APIs, security has become paramount. Modern RESTful APIs incorporate robust security measures such as API keys, OAuth tokens, and HTTPS to ensure data integrity and privacy. The focus is on protecting sensitive data and complying with global data privacy regulations, which is crucial in maintaining user trust and meeting legal requirements.
The Role of Machine Learning and AI in APIs
The integration of Machine Learning (ML) and Artificial Intelligence (AI) in APIs marks a significant advancement in web development. This fusion is revolutionizing the capabilities of web applications by enabling sophisticated data analysis, predictive modeling, and intelligent automation.
Advancements in Machine Learning APIs
Machine Learning APIs are increasingly popular for their ability to add complex functionalities like natural language processing, image recognition, and predictive analytics with minimal effort. These APIs offer pre-trained models, allowing developers to incorporate advanced analytics without the need for in-house AI expertise. Major players like Google, IBM, and Microsoft provide comprehensive ML APIs that are both powerful and user-friendly. A prime example is Amazon’s Machine Learning API, which offers a range of pre-trained AI services for tasks like forecasting and computer vision.
Sample Code: Integrating an AI API in PHP
Consider an example where we integrate a simple AI-powered text analysis API into a PHP application. This example uses a hypothetical AI API that analyzes the sentiment of a text:
<?php
// PHP code to integrate an AI-powered text analysis API
function analyzeSentiment($text) {
$apiKey = 'YOUR_API_KEY';
$apiUrl = 'https://api.example.com/analyze';
$ch = curl_init($apiUrl);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query(['text' => $text]));
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $apiKey,
'Content-Type: application/x-www-form-urlencoded'
]);
$response = curl_exec($ch);
curl_close($ch);
return json_decode($response, true);
// Example usage
$text = 'PHP and AI integration in web development is exciting!';
$result = analyzeSentiment($text);
echo 'Sentiment: ' . $result['sentiment'];
?>
This code sends a text to an AI API for sentiment analysis and prints the result.
AI/ML APIs: Enhancing User Experience
The integration of AI/ML in APIs is not just about technology; it’s a strategic move to improve user experience significantly. Technologies like chatbots, recommendation systems, and automated customer service tools are becoming more accessible through these APIs. They enhance the functionality of web applications and provide users with more interactive and personalized experiences.
The Growing Market of AI/ML APIs
The AI/ML API market is expected to expand as more companies recognize the benefits of integrating advanced AI/ML features into their applications. These APIs abstract the complexity of AI/ML technologies, making them accessible to a wider range of developers and businesses.
Emerging Technologies in PHP and API Ecosystem

The PHP and API ecosystem is continuously evolving, adapting to emerging technologies that shape the future of web development. This adaptation is not only keeping PHP relevant but also expanding its capabilities in the realm of modern web applications.
PHP and IoT: A Growing Convergence
The Internet of Things (IoT) represents a major shift in how devices interact and communicate. PHP, with its server-side capabilities, is increasingly being used in IoT applications. PHP frameworks like Laravel and Symfony are well-suited for IoT implementations due to their robustness and ability to handle complex data processing tasks.
- Laravel: Offers an elegant syntax and tools that facilitate the integration with IoT devices, like handling real-time data.
- Symfony: Its modular nature makes it ideal for building scalable IoT applications that require a high level of customization.
Enhancing Cybersecurity with PHP
As digital transactions and data exchanges increase, the importance of cybersecurity in web development cannot be overstated. PHP’s role in enhancing web application security is crucial. PHP frameworks provide various security features like input validation, XSS (Cross-Site Scripting) protection, and CSRF (Cross-Site Request Forgery) protection.
PHP for Smart Chatbots
The integration of chatbots in web applications is another area where PHP is making significant strides. Utilizing AI and ML algorithms, PHP can be used to develop intelligent chatbots that enhance user engagement and provide automated customer support.
Example Code: PHP Chatbot Integration
Here’s an example of integrating a basic chatbot into a PHP application:
<?php
// Simple PHP code to integrate a chatbot
function chatbotResponse($userInput) {
$apiKey = 'YOUR_CHATBOT_API_KEY';
$apiUrl = 'https://api.chatbot.com/response';
$postData = json_encode(['query' => $userInput]);
$ch = curl_init($apiUrl);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $postData);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $apiKey,
'Content-Type: application/json'
]);
$response = curl_exec($ch);
curl_close($ch);
return json_decode($response, true);
}
// Example usage
$userInput = 'How can I track my order?';
$response = chatbotResponse($userInput);
echo 'Chatbot says: ' . $response['message'];
?>
PHP and Developer Experience (DX)
The emphasis on developer experience in the API market is also influencing PHP development. A seamless developer experience involves a developer-friendly API design, easy onboarding, and reusability. PHP frameworks are adapting to these needs by providing more intuitive tools and enhanced documentation to streamline the development process.
Where PHP and RESTful APIs are Headed
PHP and RESTful APIs are set to continue their evolution, adapting to the changing needs of the web development world. Emerging trends and technological advancements are shaping the trajectory of these technologies.
API-First Approach Gaining Momentum
The API-first design philosophy is becoming increasingly prevalent. This approach involves designing and defining APIs and schemas before beginning actual development, ensuring a more organized and efficient development process. It facilitates better collaboration among teams and leads to more consistent and well-documented APIs, thereby reducing development times and enhancing code reuse.
Enhancing Interoperability with OpenAPI
The adoption of standards like OpenAPI (formerly Swagger) is on the rise. OpenAPI provides a powerful way to describe RESTful APIs, making them more understandable and easier to integrate across different platforms. This standard is expected to gain more traction, promoting interoperability and easing the integration process between different systems.
Advanced PHP Features for Future Readiness
PHP, as a language, is expected to continue integrating advanced features to stay competitive and efficient. Features such as improved asynchronous programming capabilities and enhanced support for concurrent processing are likely to become more prominent. These advancements will enable PHP to handle more complex and high-performance applications, particularly in areas like real-time data processing and large-scale cloud-based systems.
AI and ML Integration in PHP
The integration of AI and ML within PHP applications is poised to grow. PHP developers will increasingly leverage AI and ML APIs to add intelligent features to web applications, such as predictive analytics, personalized content delivery, and automated decision-making processes.
Code Sample: Asynchronous Requests in PHP
Here’s an example showcasing the potential use of asynchronous programming in PHP. This code snippet demonstrates how PHP might handle asynchronous HTTP requests in the future:
<?php
// Hypothetical example of asynchronous requests in PHP
function fetchUrlAsync($url) {
// Asynchronous request handling
// This is a conceptual example and may not reflect actual PHP syntax
$promise = new AsyncHttpRequest($url);
return $promise->then(function ($response) {
return $response->getBody();
});
}
// Example usage
fetchUrlAsync('https://example.com/api/data')
->then(function ($data) {
echo 'Data received: ' . $data;
})
->catch(function ($error) {
echo 'Error: ' . $error->getMessage();
});
?>
This example, though hypothetical, gives an insight into how PHP might evolve to natively support asynchronous operations, enhancing its capabilities for handling non-blocking tasks and improving performance in web applications.
Conclusion
Embracing trends like API-first design, OpenAPI standards, and the integration of AI and ML, PHP is evolving to meet the demands of modern, complex applications. This evolution ensures PHP remains a robust, versatile choice for developers, driving innovation and efficiency in web development. The journey ahead for PHP and RESTful APIs is one of continual adaptation and growth, promising exciting developments in the web technology landscape.