The landscape of web development is continually evolving, and at the forefront of this evolution is full-stack development — a methodology where developers have the proficiency to work on both the front-end and back-end portions of an application. This approach not only enhances development efficiency but also ensures a seamless integration of various parts of a web application.
Understanding Full-Stack Development
Full-stack development involves a comprehensive understanding of both client-side (frontend) and server-side (backend) development. In the context of web applications, the frontend is what users interact with directly, while the backend involves server, application, and database interactions.
The ASP.NET and Angular Combo
ASP.NET, a mature framework developed by Microsoft, is renowned for its robustness and scalability in building dynamic web applications. It’s a server-side framework that runs on Windows and is known for its performance and security features.
Angular, on the other hand, is a platform and framework for building single-page client applications using HTML and TypeScript. Developed by Google, it’s known for creating dynamic, modern web applications. Angular achieves this by extending HTML’s syntax, which allows for the application’s components to be expressed clearly and succinctly.
Why Combine ASP.NET and Angular?
The combination of ASP.NET and Angular is powerful for several reasons:
- Scalability and Performance: ASP.NET is known for its scalability and performance, which, when combined with Angular’s efficient client-side rendering, results in a highly responsive and fast application.
- Robust Architecture: Both Angular and ASP.NET follow a modular architecture, which makes it easier to manage and scale large applications.
- Community and Ecosystem: With both technologies being backed by tech giants (Microsoft for ASP.NET and Google for Angular), they have large communities and extensive libraries and tools available.
- Security: ASP.NET has built-in features for authentication and authorization, while Angular provides mechanisms to prevent common web vulnerabilities, making the combination suitable for building secure applications.
- Cross-Platform Development: ASP.NET’s cross-platform nature, thanks to .NET Core, allows applications to be run on various operating systems, while Angular’s platform-independent nature makes it ideal for the frontend.
The Versatility of Full-Stack Development with ASP.NET and Angular
Full-stack development with ASP.NET and Angular offers a comprehensive approach to building web applications. It allows developers to:
- Design interactive and dynamic user interfaces with Angular.
- Implement robust server-side logic and database handling with ASP.NET.
- Create applications that are scalable, maintainable, and secure.
The Target Audience for This Combination
This combination is ideal for developers looking to build enterprise-level applications, as well as for those interested in creating scalable, secure, and high-performance web applications. Both beginners and experienced developers can benefit from learning these technologies, given their widespread use and the demand in the job market.
Setting Up Your Development Environment for ASP.NET and Angular
To embark on building full-stack applications using ASP.NET and Angular, the first critical step is to establish a solid development environment. This setup encompasses the installation of necessary tools, frameworks, and extensions that will enable efficient development of both the frontend and backend parts of your application.
Essential Tools and Frameworks
- Visual Studio: An integrated development environment (IDE) from Microsoft. Ideal for ASP.NET development, it provides a comprehensive set of tools and features to streamline the development process. Make sure to install the latest version to get the most updated features.
- Node.js and npm: Node.js is a runtime environment for executing JavaScript code server-side. npm is Node’s package manager, and it’s crucial for managing dependencies in Angular projects. Install the latest versions of Node.js and npm from the official Node.js website.
- Angular CLI: The Angular Command Line Interface (CLI) is a powerful tool to initialize, develop, scaffold, and maintain Angular applications. You can install Angular CLI globally on your machine using npm:
npm install -g @angular/cli
- .NET Core SDK: The Software Development Kit for .NET Core is necessary for developing ASP.NET applications. It includes the runtime and command-line tools for creating .NET Core applications. Download and install it from the .NET official site.
- Git: Version control is crucial in software development. Git helps you manage source code history. Install Git from its official website.
Configuring the IDE
After installing Visual Studio:
- Install ASP.NET and web development workload: Open Visual Studio Installer and ensure that the “ASP.NET and web development” workload is selected. This workload includes the necessary tools for developing ASP.NET applications.
- Configure External Tools: Integrate Git for source control management within Visual Studio for better version control.
Setting Up an Angular Environment
- Create a New Angular Project: Use Angular CLI to create a new project. Open your command line interface and run:
ng new your-angular-project-name
This command scaffolds a new Angular application with all the default configurations.
- Running the Angular Application: Navigate to the project directory and start the development server:
cd your-angular-project-name
ng serve
This will compile the application and make it available on localhost:4200
.
Setting Up an ASP.NET Core Environment
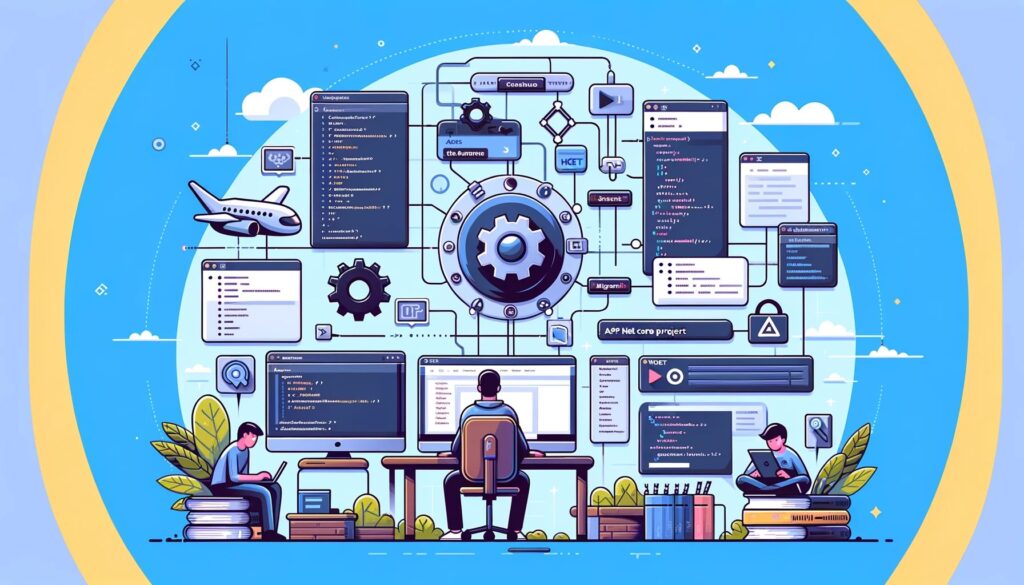
- Create a New ASP.NET Core Project: Using Visual Studio, create a new ASP.NET Core Web API project. This project will serve as the backend for your full-stack application.
- Understanding the Project Structure: Familiarize yourself with the ASP.NET Core project structure, including understanding the purpose of the
Startup.cs
,Program.cs
, and other fundamental files. - Adding NuGet Packages: Depending on your application’s needs, you might need to add various NuGet packages. This can be done through the NuGet Package Manager in Visual Studio.
- Running the ASP.NET Core Application: Run the application in Visual Studio using IIS Express. This will host your API locally for development purposes.
Integrating ASP.NET Core with Angular
- Enable CORS: To allow the Angular application to communicate with the ASP.NET Core API, enable Cross-Origin Resource Sharing (CORS) in the Startup.cs of your ASP.NET project:
public void ConfigureServices(IServiceCollection services)
{
// ...
services.AddCors(options =>
{
options.AddPolicy("AllowAllOrigins",
builder => builder.AllowAnyOrigin().AllowAnyMethod().AllowAnyHeader());
});
// ...
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
// ...
app.UseCors("AllowAllOrigins");
// ...
}
- Setting Up API Calls: In your Angular application, use Angular’s HttpClient to make API calls to your ASP.NET Core backend.
Crafting the Angular Frontend
With the development environment set up, the next step in building a full-stack application with ASP.NET and Angular is to craft the Angular frontend. Angular excels in creating dynamic, interactive user interfaces. This section will guide you through the fundamental aspects of building the frontend of your application.
Understanding Angular Architecture
Angular applications are structured around components and services. A component controls a patch of the screen (a view) and services provide reusable logic independent of views.
- Components: The building blocks of Angular applications. Each component consists of an HTML template, a TypeScript class, and a CSS file for styles.
- Services: Provide logic that isn’t associated with views and can be shared across components.
Creating Components
Components are crucial for your application. They define the user interface and the logic behind it. Here’s how to create a new component:
- Use Angular CLI: To generate a new component, run:
ng generate component your-component-name
This command creates a new folder with your component name containing the necessary files.
- Component Structure: A typical component consists of:
- A TypeScript file (
.ts
): Contains the logic. - An HTML file (
.html
): The template of the component. - A CSS file (
.css
or.scss
): Styles for your component.
- A TypeScript file (
- Implementing the Template: Write your HTML code in the template file. This can include data binding, directives, and more.
- Adding Logic: In the TypeScript file, define variables and functions that your component will use.
Managing State and Data Binding
Angular’s two-way data binding is a powerful feature that allows automatic synchronization between the model and the view.
- ngModel Directive: Use the ngModel directive for two-way data binding. For example, in your component template:
<input [(ngModel)]="yourModel">
This binds the input value to the yourModel property in your component.
- Using Services for State Management: Implement services to manage and share data across components.
Routing and Navigation
Angular’s Router allows you to navigate from one view to the next as users perform application tasks.
- Setting Up Routing: In your main module file (typically
app.module.ts
), importRouterModule
and define your routes. - Router Outlet: Use
<router-outlet></router-outlet>
in your template to mark where the router should display views. - Navigation: Use the
Router
service in your component to navigate between views.
Integrating with ASP.NET Core Backend
- HttpClient: Use Angular’s
HttpClient
to make HTTP requests to your ASP.NET Core backend. - Service Creation: Create a service to handle API calls. For example:
import { HttpClient } from '@angular/common/http';
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class ApiService {
constructor(private http: HttpClient) { }
getSomeData() {
return this.http.get('/api/your-endpoint');
}
}
- Using the Service: Inject the service into your components to use the API methods.
Developing the ASP.NET Core Backend
After setting up the Angular frontend, our focus shifts to developing the ASP.NET Core backend. ASP.NET Core is a robust, high-performance framework for building server-side applications. It’s crucial for handling database operations, business logic, and serving API requests from the Angular frontend.
Creating a Web API Project
Start by creating a new ASP.NET Core Web API project. This can be done through Visual Studio by choosing ‘Create a new project’ and then selecting ‘ASP.NET Core Web API’. This setup provides a basic structure for your API.
Understanding the Project Structure
A typical ASP.NET Core project contains several key files and folders:
- Startup.cs: Configures services and the app’s request pipeline.
- Program.cs: Hosts the main entry point for the application.
- Controllers: Contains the API controllers which handle HTTP requests.
- Models: Where you define your data models.
Building a Controller
- Creating a Controller: In the ‘Controllers’ directory, add a new controller. Use the MVC controller or API controller template provided by Visual Studio.
- Defining Routes: Use attributes like
[HttpGet]
and[HttpPost]
to define routes. For example:
[ApiController]
[Route("[controller]")]
public class SampleController : ControllerBase
{
[HttpGet]
public IActionResult Get()
{
// Implementation
}
}
Connecting to a Database
- Entity Framework Core: Utilize Entity Framework Core for ORM (Object-Relational Mapping). This allows you to interact with your database using C# objects.
- Setting Up a Connection String: Define your database connection string in
appsettings.json
. - Creating Data Models: Create C# classes in the Models directory, and use Entity Framework tools to scaffold a database context and generate database tables.
Implementing Business Logic
- Services and Repositories: Create services and repositories to encapsulate business logic and data access logic.
- Dependency Injection: ASP.NET Core’s built-in dependency injection feature should be used to inject these services into your controllers.
Handling CORS
To allow your Angular frontend to access the API, configure CORS in the Startup.cs
file. For example:
public void ConfigureServices(IServiceCollection services)
{
services.AddCors(options =>
{
options.AddPolicy(name: "AllowSpecificOrigin",
builder =>
{
builder.WithOrigins("http://example.com",
"http://www.contoso.com");
});
});
// Other services
}
Creating API Endpoints
Define methods in your controllers to serve as API endpoints. For instance, to retrieve data:
[HttpGet("get-data")]
public async Task<IActionResult> GetData()
{
// Logic to get data
}
Testing the API
Use tools like Postman or Swagger to test your API endpoints. Ensure they respond correctly to HTTP requests.
Bridging Frontend and Backend: Integration Techniques
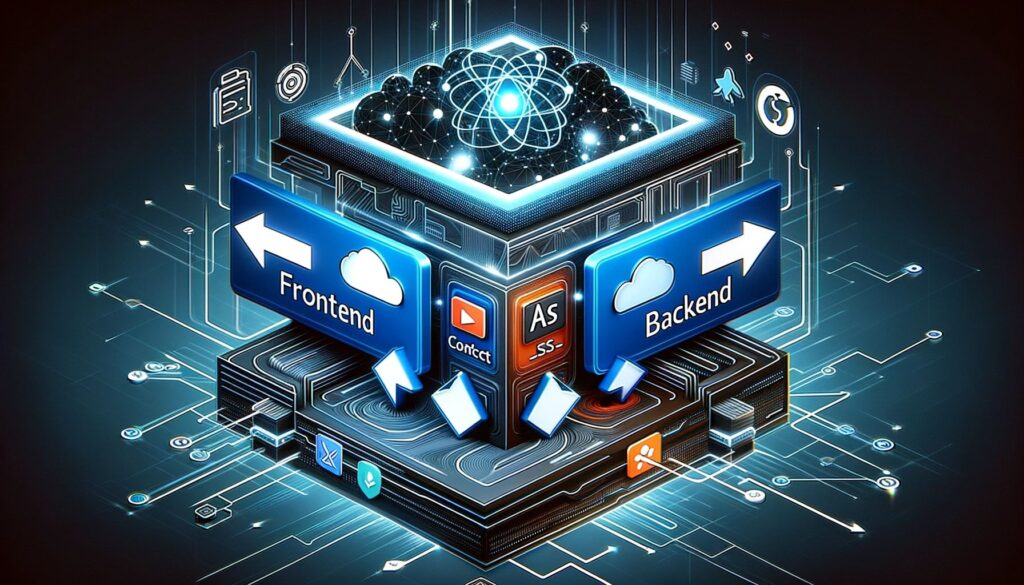
Integrating the Angular frontend with the ASP.NET Core backend is a pivotal step in creating a cohesive and functional full-stack application. This process involves setting up effective communication channels between the two parts of your application. By doing this, you can ensure that your Angular frontend can send requests to and receive responses from your ASP.NET Core backend.
Setting Up Angular to Communicate with ASP.NET Core
- Using HttpClient: Angular’s HttpClient module is used for making HTTP requests to your backend. First, import HttpClientModule in your main module (app.module.ts):
import { HttpClientModule } from '@angular/common/http';
@NgModule({
imports: [
// ... other imports
HttpClientModule
],
})
export class AppModule { }
- Creating a Service for API Calls: Create a service in Angular to handle the API calls. For instance:
import { HttpClient } from '@angular/common/http';
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class DataService {
constructor(private http: HttpClient) { }
getSomeData() {
return this.http.get('http://localhost:5000/api/sample');
}
}
Replace '
http://localhost:5000/api/sample
'
with your actual ASP.NET Core API endpoint.
Handling Requests in ASP.NET Core
In your ASP.NET Core backend, ensure that your controllers are ready to accept and respond to requests from your Angular application.
- Create API Endpoints: Define methods in your controllers to serve as API endpoints. For example:
[Route("api/[controller]")]
public class SampleController : ControllerBase
{
[HttpGet]
public ActionResult<IEnumerable<string>> Get()
{
return new string[] { "value1", "value2" };
}
}
- Enable CORS: Make sure to enable CORS in your ASP.NET Core application to allow requests from your Angular app’s origin.
Testing the Integration
- Testing with Angular: Use the service you created to make requests to your ASP.NET Core backend and log the responses. This can be done from an Angular component:
export class AppComponent {
constructor(private dataService: DataService) {}
ngOnInit() {
this.dataService.getSomeData().subscribe(data => {
console.log(data);
});
}
}
- Using Development Tools: Tools like the browser’s developer console and network tab can be very helpful to debug and monitor the requests and responses.
- Debugging in Visual Studio: Utilize breakpoints in your ASP.NET Core controllers to ensure that the incoming requests from the Angular frontend are being received and processed correctly.
Security Measures in Full-Stack Development
Ensuring the security of your full-stack application, comprising the Angular frontend and the ASP.NET Core backend, is paramount. This involves implementing various security mechanisms to protect against common vulnerabilities and threats.
Implementing Frontend Security in Angular
- Sanitizing Data to Prevent XSS Attacks: Angular comes with built-in protection against cross-site scripting (XSS) attacks. However, when manually manipulating the DOM or working with innerHTML, additional care should be taken to sanitize inputs.
import { DomSanitizer } from '@angular/platform-browser';
constructor(private sanitizer: DomSanitizer) {}
sanitizeHtml(content) {
return this.sanitizer.bypassSecurityTrustHtml(content);
}
- Using HTTPS: Make sure all API requests use HTTPS to secure data in transit. This can be configured in the service handling API requests.
getData() {
return this.http.get('https://yourdomain.com/api/data');
}
- Validation: Perform client-side validation to improve user experience but never rely on it for security. All data must be validated again on the server side.
Securing the ASP.NET Core Backend
- Securing API Endpoints with Authentication and Authorization: Use ASP.NET Core Identity for user authentication and authorization. Implement JWT (JSON Web Tokens) for a stateless, secure API.
services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options =>
{
options.TokenValidationParameters = new TokenValidationParameters
{
ValidateIssuerSigningKey = true,
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8
.GetBytes(Configuration["TokenKey"])),
ValidateIssuer = false,
ValidateAudience = false
};
});
- Cross-Site Request Forgery (CSRF) Protection: ASP.NET Core provides built-in CSRF protection. Ensure that it’s properly configured especially if your application involves form submissions.
- Securing Data Access: Use Entity Framework Core’s built-in security features to prevent SQL injection attacks. Always use parameterized queries or LINQ to interact with the database.
public async Task<IActionResult> GetItem(int id)
{
var item = await _context.Items.FirstOrDefaultAsync(i => i.Id == id);
// ...
}
- Data Encryption: Secure sensitive data using encryption both at rest and in transit. ASP.NET Core includes APIs for encrypting data.
Best Practices for Security
- Keep Dependencies Updated: Regularly update Angular, ASP.NET Core, and other dependencies to their latest versions to incorporate security patches.
- Least Privilege Principle: Grant minimal permissions needed for the application to function, especially in database access and API permissions.
- Logging and Monitoring: Implement logging to monitor and record suspicious activities or breaches.
Performance Optimization: Best Practices for Speed and Efficiency
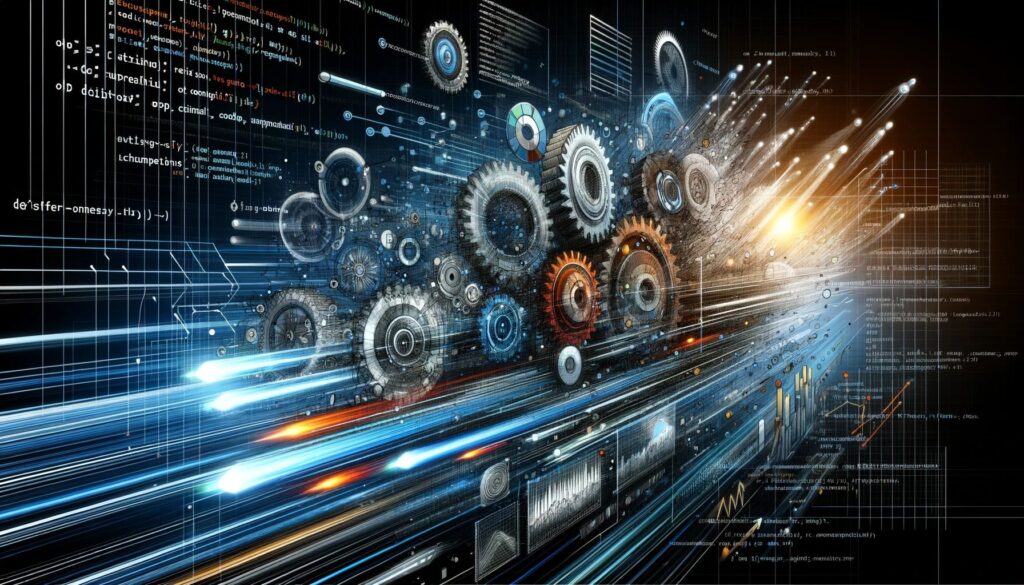
Optimizing the performance of your full-stack application built with Angular and ASP.NET Core is crucial for delivering a smooth and responsive user experience. Here are some strategies and techniques to enhance the speed and efficiency of your application.
Performance Optimization in Angular
- Lazy Loading: Implement lazy loading to load feature modules only when needed, reducing the initial load time of the application.
const routes: Routes = [
{
path: 'feature',
loadChildren: () => import('./feature/feature.module').then(m => m.FeatureModule)
}
];
- TrackBy Function in ngFor: Use
trackBy
with*ngFor
to improve the performance of list rendering.
<div *ngFor="let item of items; trackBy: trackByFn">
{{item.name}}
</div>
trackByFn(index, item) {
return item.id;
}
- Optimizing Change Detection: Utilize Angular’s
OnPush
change detection strategy to minimize unnecessary checks.
@Component({
selector: 'app-sample',
changeDetection: ChangeDetectionStrategy.OnPush,
templateUrl: './sample.component.html'
})
export class SampleComponent {}
Performance Optimization in ASP.NET Core
- Response Caching: Implement response caching to store responses temporarily and serve them for subsequent requests.
[ResponseCache(Duration = 60)]
public IActionResult Index()
{
// ...
}
- Asynchronous Programming: Use async and await for non-blocking I/O operations.
public async Task<IActionResult> GetDataAsync()
{
var data = await _dbContext.Data.ToListAsync();
return Ok(data);
}
- Optimizing Data Access: Use Entity Framework Core efficiently by avoiding unnecessary includes, selecting only required fields, and handling large datasets with pagination.
var users = await _dbContext.Users.Select(u => new { u.Id, u.Name }).ToListAsync();
General Best Practices for Performance
- Minimize Use of Heavy Libraries: Evaluate the necessity of third-party libraries. If only a small part of a library is needed, look for lighter alternatives.
- Optimize Assets: Minimize and compress assets, including images, CSS, and JavaScript files.
- Use Content Delivery Network (CDN): Serve static assets through a CDN to reduce load times.
- Server-Side Rendering (SSR): For Angular, consider using Angular Universal for server-side rendering to improve initial load times and SEO.
- Database Optimization: Regularly review and optimize your database queries and indexes.
- Code Splitting in Angular: Split your code into bundles to load only the necessary code for the current route.
Deployment and Maintenance of Your Full-Stack Application
Deploying and maintaining a full-stack application built with Angular and ASP.NET Core involves several key steps to ensure that your application is securely and efficiently available to users. This section will cover the essentials of deploying both parts of your application and maintaining them in a production environment.
Preparing for Deployment
- Build the Angular Application: Compile your Angular application for production. Use the Angular CLI build command with the –prod flag, which enables optimizations like minification and ahead-of-time (AOT) compilation.
ng build --prod
- Preparing ASP.NET Core for Production: Ensure your ASP.NET Core app is ready for production. This includes setting appropriate environment variables, connection strings, and any necessary configuration changes in appsettings.Production.json.
- Testing: Perform thorough testing in a production-like environment to catch any potential issues before going live.
Deployment Strategies
- Hosting Options:
- Frontend (Angular): Deploy your built Angular application to a web server or a content delivery network (CDN). Popular choices include Netlify, Vercel, and Amazon S3.
- Backend (ASP.NET Core): Deploy your ASP.NET Core application to a cloud provider like Azure, AWS, or a dedicated hosting environment.
- Continuous Integration/Continuous Deployment (CI/CD): Implement CI/CD pipelines using tools like Azure DevOps, Jenkins, or GitHub Actions to automate the build and deployment process.
- Docker Containers: Consider containerizing your application with Docker. This simplifies deployment and ensures consistency across environments.
# Example Dockerfile for ASP.NET Core
FROM mcr.microsoft.com/dotnet/core/sdk:3.1 AS build-env
WORKDIR /app
# Copy csproj and restore as distinct layers
COPY *.csproj ./
RUN dotnet restore
# Copy everything else and build
COPY . ./
RUN dotnet publish -c Release -o out
# Build runtime image
FROM mcr.microsoft.com/dotnet/core/aspnet:3.1
WORKDIR /app
COPY --from=build-env /app/out .
ENTRYPOINT ["dotnet", "yourapp.dll"]
Post-Deployment Maintenance
- Monitoring and Logging: Implement logging and monitoring solutions to keep track of your application’s performance and health. Use tools like ELK Stack, Datadog, or Azure Monitor.
- Updates and Patch Management: Regularly update your application and its dependencies to patch security vulnerabilities and performance issues.
- Database Maintenance: Regularly back up your database and perform maintenance tasks such as indexing and query optimization.
- Handling Downtime and Rollbacks: Have strategies in place for handling downtime and rolling back deployments in case of critical failures.
- User Feedback and Issue Tracking: Set up a system for collecting user feedback and tracking issues. Tools like Sentry, JIRA, or Zendesk can be helpful.
Future Trends and Advancements in Full-Stack Development
The world of full-stack development, particularly with technologies like Angular and ASP.NET Core, is constantly evolving. Staying ahead of the curve involves understanding and adapting to emerging trends and advancements. Here, we explore potential future directions that could shape the landscape of full-stack development.
Emerging Technologies and Frameworks
- Progressive Web Apps (PWAs): PWAs are gaining traction for their ability to deliver a native app-like experience. Angular already offers excellent support for building PWAs, and this trend is likely to grow.
- Blazor for WebAssembly: Blazor, a framework for building interactive web UIs with C#, has introduced WebAssembly support. This could open new possibilities for .NET developers in the realm of full-stack development.
- Microfrontend Architecture: The concept of microfrontends – breaking down frontend apps into smaller, more manageable pieces – might see increased adoption, offering a parallel to microservices in backend development.
- AI and Machine Learning Integration: The integration of AI and machine learning into web applications can provide advanced features like personalized user experiences, predictive analytics, and intelligent data processing.
Advancements in Tooling and Ecosystems
- Enhanced Development Tools: Tools that offer better integration between frontend and backend development, such as improved debugging across the stack and more unified workflows, are likely to emerge.
- Cloud-Native Technologies: The shift towards cloud-native development is accelerating. This includes serverless architectures, container orchestration with Kubernetes, and cloud-specific optimizations.
- DevOps and Automation: The role of DevOps is becoming more crucial. Automated testing, CI/CD pipelines, infrastructure as code, and other DevOps practices will continue to streamline the development process.
Language and Framework Enhancements
- .NET 6 and Beyond: With .NET 5 and the upcoming .NET 6, there is a push towards a unified framework. This unification will likely bring more features and optimizations beneficial for full-stack development.
- Angular Updates: Angular continues to evolve with regular updates. Future versions are expected to bring more performance improvements, enhanced modularity, and possibly new approaches to state management.
Security and Compliance
- Data Privacy Regulations: With increasing focus on user privacy (GDPR, CCPA, etc.), full-stack development will need to incorporate stronger privacy and security measures.
- Advanced Security Practices: As security threats evolve, so will the security practices in web development. This includes more robust encryption, secure coding practices, and automated vulnerability scanning.
Conclusion
In this exploration of “ASP.NET and Angular: Building a Full-Stack App,” we’ve navigated the intricacies of combining Angular and ASP.NET Core, two powerful technologies, to develop dynamic, robust web applications. This journey has taken us through the essential steps of setting up a development environment, delving into the creation of an engaging Angular frontend and a solid ASP.NET Core backend, and culminating in the deployment and maintenance of a seamless application. The synergy between Angular and ASP.NET Core transcends their individual capabilities, enabling developers to create scalable, secure, and responsive applications that meet contemporary needs.
As technology continues to evolve, staying abreast of trends like serverless architectures, real-time applications, and cross-platform development is vital. These trends underscore the dynamic nature of full-stack development and the necessity for continuous learning. This guide not only equips developers with the foundational skills to build end-to-end solutions but also encourages embracing the ongoing journey of growth in the ever-changing landscape of web development. With Angular and ASP.NET Core, developers are well-positioned to make impactful contributions in the world of web development.