In the realm of web development, Silverlight and AJAX stand out as two pivotal technologies that have significantly influenced the way web applications are designed and function. This section delves into the individual characteristics of Silverlight and AJAX, and how their integration can revolutionize web application development, offering a richer user experience and enhanced performance.
Silverlight: A Brief Overview
Silverlight, developed by Microsoft, is a powerful framework for building rich internet applications (RIAs). It’s primarily used for streaming media, multimedia, and graphics, and operates as a plugin for most web browsers. Silverlight facilitates the creation of highly interactive and visually appealing web applications. It leverages a subset of the .NET framework, embracing a XAML (eXtensible Application Markup Language) based architecture for defining user interfaces. This approach allows developers to craft sophisticated UI elements that are both scalable and lightweight.
Key features of Silverlight include:
- Rich Graphics and Media Handling: Silverlight excels in delivering high-quality video streams and engaging graphical content.
- Deep Zoom Technology: This feature allows users to interact with high-resolution images smoothly and seamlessly.
- Support for Various Programming Languages: It supports languages like C#, VB.NET, and others, making it versatile for developers.
AJAX: An Introduction
AJAX, which stands for Asynchronous JavaScript and XML, is not a technology in itself but a technique that uses a combination of existing technologies. It includes HTML or XHTML, Cascading Style Sheets (CSS), JavaScript, the Document Object Model (DOM), XML, XSLT, and the XMLHttpRequest object. AJAX allows web pages to be updated asynchronously by exchanging small amounts of data with the server behind the scenes. This means that it’s possible to update parts of a web page, without reloading the whole page.
Key features of AJAX include:
- Enhanced User Experience: AJAX minimizes the need for page refreshes, leading to smoother and more dynamic web pages.
- Reduced Server Load: Since only parts of the web page are updated, there’s less demand on the server, improving performance.
The Synergy of Silverlight and AJAX
When Silverlight and AJAX are combined, they create a compelling toolkit for web developers. Silverlight brings rich media and graphics, while AJAX contributes its asynchronous data handling and partial page updates. This integration leads to web applications that are not only visually impressive but also efficient and responsive.
Rich and Interactive UIs: Leveraging Silverlight’s graphical prowess with AJAX’s dynamic updates results in web applications with highly interactive and user-friendly interfaces.
Optimized Performance: The combination ensures that web applications are fast and responsive, with minimal server load and reduced bandwidth usage.
Setting Up Your Development Environment
To begin developing web applications with Silverlight and AJAX, it’s essential to set up a proper development environment. This setup involves installing the necessary software and tools, which are foundational for creating and testing your applications.
Essential Software and Tools
- Visual Studio: The primary integrated development environment (IDE) for building Silverlight and AJAX applications. Visual Studio provides a comprehensive set of tools for developing, debugging, and deploying applications.
- Silverlight Developer Runtime and SDK: The Software Development Kit (SDK) includes libraries, samples, tools, and documentation for developing Silverlight applications.
- ASP.NET AJAX Extensions and Control Toolkit: These are required for developing AJAX-enabled web applications. They provide a robust framework and a set of controls for rich, interactive web development.
Basic Setup Steps
- Install Visual Studio: Ensure you have Visual Studio installed. It’s the main platform where you’ll write, test, and debug your code.
- Download and Install Silverlight Tools: These tools integrate with Visual Studio and provide the necessary templates and debugging utilities for Silverlight development.
- Install ASP.NET AJAX Extensions: This step is crucial for enabling AJAX functionality in your web applications.
Creating a Basic Silverlight Application
Once your environment is set up, you can start building a simple Silverlight application. Here’s an example of a basic Silverlight application setup:
1. Start a New Project in Visual Studio: Choose a Silverlight application template to begin.
2. Define the UI with XAML: Silverlight uses XAML for its user interface layout. Here’s a simple XAML example:
<UserControl x:Class="SilverlightApplication.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Width="400" Height="300">
<Grid x:Name="LayoutRoot" Background="White">
<TextBlock Text="Hello, Silverlight!" />
</Grid>
</UserControl>
This code snippet creates a basic user interface with a single text block displaying “Hello, Silverlight!”
3. Implementing Code-Behind in C# or VB.NET: The logic for your Silverlight application is written in the code-behind file, typically in C# or VB.NET. For this example, the code-behind might simply initialize the component.
public partial class MainPage : UserControl
{
public MainPage()
{
InitializeComponent();
}
}
4. Running the Application: Once you’ve set up the XAML and code-behind, run the application in Visual Studio to see your Silverlight application in action.
Integrating AJAX
To add AJAX functionality to your Silverlight application:
- Use ASP.NET AJAX Control Toolkit: This toolkit provides extenders and controls that enhance your web forms with AJAX capabilities.
- Implement Partial Page Updates: Utilize the UpdatePanel control for partial page rendering, which is a key feature of AJAX. This allows parts of the page to be updated without a full page refresh.
- Client-Side Scripting: Use JavaScript for client-side logic, which is essential for AJAX’s asynchronous functionality.
Leveraging Silverlight Controls in ASP.NET Web Pages
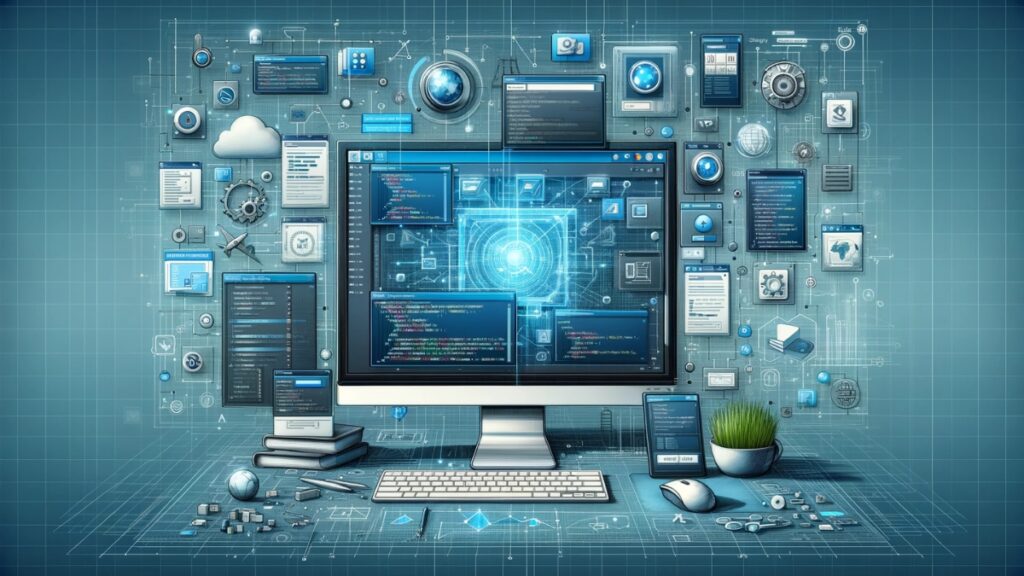
Integrating Silverlight controls into ASP.NET web pages can significantly enhance the richness and interactivity of your web applications. This process involves utilizing Silverlight’s capabilities within the ASP.NET framework to create a more dynamic and visually appealing user experience.
Using Silverlight Controls
Silverlight provides various controls that can be embedded into ASP.NET web pages. These controls allow developers to incorporate rich media content, like video and graphics, into their web applications. Here’s how you can use some of the basic Silverlight controls:
1. Embedding a Silverlight Application:
- Use the asp:Silverlight control to embed a Silverlight application within an ASP.NET web page.
- In your ASPX page, you can include the Silverlight control like this:
<asp:Silverlight ID="Xaml1" runat="server" Source="~/ClientBin/YourApplication.xap" MinimumVersion="3.0.40624.0" Width="300" Height="300" />
- This code snippet will embed a Silverlight application (YourApplication.xap) into your web page.
2. Adding a Media Player:
- The asp:MediaPlayer control can be used to integrate media playback functionalities.
- You can embed a media player to play audio or video files:
<asp:MediaPlayer ID="MediaPlayer1" runat="server" MediaSource="~/Media/YourMedia.wmv" AutoPlay="false" />
- This example adds a media player to your page, which is ready to play a media file (like YourMedia.wmv).
Integrating with ASP.NET Services
Silverlight applications can interact seamlessly with ASP.NET services, allowing for a robust back-end to support the rich front-end. This can be achieved by:
1. Creating Web Services:
- Develop ASP.NET web services that your Silverlight application can consume.
- These services can provide data or functionality to the Silverlight client.
2. Accessing Services from Silverlight:
- Use Silverlight’s networking capabilities to interact with these web services.
- For instance, you can use the WebClient or HttpWebRequest classes in Silverlight to call ASP.NET web services.
Example: Calling a Web Service from Silverlight
Here’s a simple example of how a Silverlight application can call an ASP.NET web service:
1. ASP.NET Web Service:
- Assume you have an ASP.NET web service that returns the current date and time.
- The web service might look like this:
[WebMethod]
public string GetCurrentDateTime()
{
return DateTime.Now.ToString();
}
2. Silverlight Client Code:
- In your Silverlight application, you can call this web service:
private void CallWebService()
{
WebClient webClient = new WebClient();
webClient.DownloadStringCompleted += new DownloadStringCompletedEventHandler(webClient_DownloadStringCompleted);
webClient.DownloadStringAsync(new Uri("http://yourdomain.com/YourService.asmx/GetCurrentDateTime"));
}
void webClient_DownloadStringCompleted(object sender, DownloadStringCompletedEventArgs e)
{
if (e.Error == null)
{
string result = e.Result; // This is the date and time returned from the web service
// Handle the result as needed
}
}
- This code sets up a WebClient to call the GetCurrentDateTime method of your web service and handle the response.
Creating Interactive User Interfaces with XAML and Canvas
Silverlight’s XAML and Canvas controls are integral for designing interactive and visually compelling user interfaces. XAML, which stands for eXtensible Application Markup Language, is used to create the layout of Silverlight applications, while the Canvas control provides a flexible space where visuals can be drawn, positioned, and manipulated dynamically.
Understanding XAML for UI Design
XAML is the language for designing user interfaces in Silverlight. It allows you to define UI elements in a declarative manner. Here’s an example of using XAML to create a simple user interface:
<UserControl x:Class="SilverlightApp.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Width="400" Height="300">
<Canvas>
<Rectangle Width="100" Height="100" Fill="Blue" Canvas.Left="50" Canvas.Top="50" />
<TextBlock Canvas.Left="160" Canvas.Top="70" Text="Hello, Silverlight!" Foreground="White"/>
</Canvas>
</UserControl>
In this example, a Canvas is used as the container, inside which a Rectangle and a TextBlock are placed. The Canvas.Left and Canvas.Top properties set the position of these elements within the canvas.
Leveraging the Canvas Control
The Canvas control in Silverlight is a layout container that allows for explicit positioning of child elements. It’s especially useful for creating graphics, custom drawings, and handling complex user interactions. Here’s a basic example of how to use Canvas in Silverlight:
<Canvas x:Name="MainCanvas">
<Ellipse Width="50" Height="50" Fill="Red" Canvas.Left="10" Canvas.Top="10"/>
<Ellipse Width="50" Height="50" Fill="Green" Canvas.Left="70" Canvas.Top="10"/>
<Ellipse Width="50" Height="50" Fill="Blue" Canvas.Left="130" Canvas.Top="10"/>
</Canvas>
This snippet creates a Canvas with three Ellipse elements, each positioned at different points.
Dynamic Interaction with JavaScript
To make the Canvas interactive, you can use JavaScript. For example, you can handle mouse events to move elements within the Canvas:
document.getElementById('MainCanvas').addEventListener('mousemove', function(event) {
// Logic to move elements within the canvas
});
This JavaScript snippet adds an event listener to the Canvas, enabling interaction based on mouse movements.
Enhancing User Experience with AJAX and Silverlight Integration
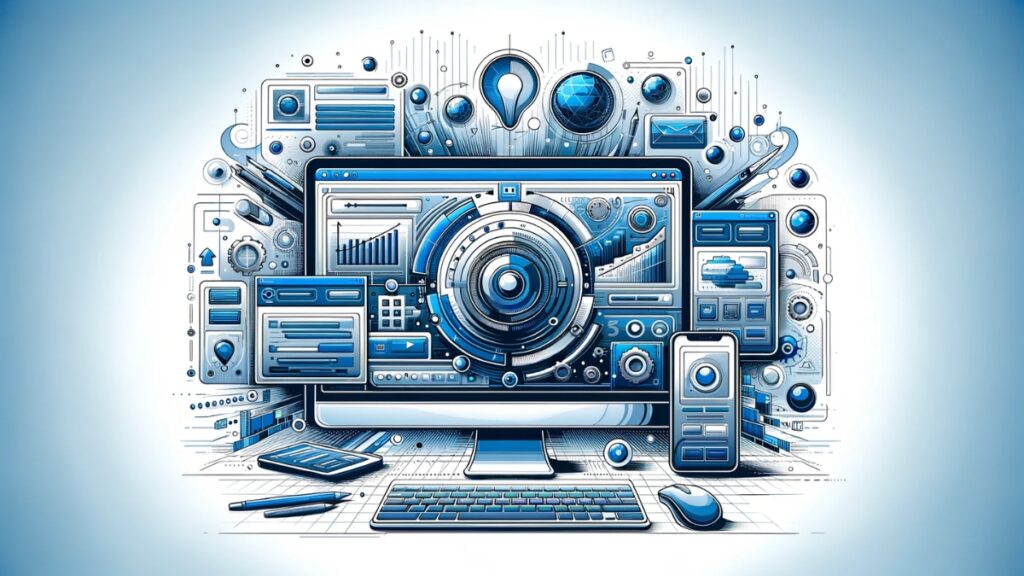
Integrating AJAX with Silverlight enriches the user experience by enabling dynamic content updates without the need for complete page reloads. This combination is particularly effective in creating responsive and interactive web applications.
Implementing AJAX in Silverlight Applications
Here’s how you can integrate AJAX with a Silverlight application:
1. Use AJAX to Fetch Data:
- Use JavaScript’s XMLHttpRequest to fetch data asynchronously.
- Update Silverlight components dynamically based on the AJAX response.
var xhr = new XMLHttpRequest();
xhr.open('GET', 'api/data', true);
xhr.onreadystatechange = function() {
if (xhr.readyState == 4 && xhr.status == 200) {
// Update Silverlight components with the received data
}
};
xhr.send();
In this example, an AJAX call is made to an API endpoint, and the response is used to update Silverlight components.
1. Handling Data on the Server Side:
On the server side, design your ASP.NET application to handle AJAX requests and respond with data in a format that Silverlight can consume, typically JSON or XML.
Example: Dynamic Data Loading
Imagine you have a Silverlight application that displays data in a grid. You can use AJAX to dynamically load and update this data without refreshing the entire page. The Silverlight application then parses this data and updates the grid accordingly.
Understanding the Role of RIA in Web Applications
Rich Internet Applications (RIAs) have transformed the web development landscape, offering end-users desktop-like application experiences within their web browsers. Silverlight, as a RIA technology, plays a crucial role in enhancing these experiences, especially when used in conjunction with AJAX.
Defining Rich Internet Applications (RIAs)
RIAs are web applications that have the features and functionality of traditional desktop applications. They offer a more engaging user experience with better responsiveness, rich media content, and interactive capabilities.
Silverlight, as a RIA platform, provides:
- High-Quality Video and Audio Streaming: Ideal for media-rich web applications.
- Advanced Graphics and Animation: Enables the creation of visually stunning user interfaces.
- Deep Zoom Technology: Allows users to interact with high-resolution images.
Comparing RIA Technologies
Other RIA technologies, like Adobe Flash and JavaFX, offer similar functionalities. However, Silverlight’s integration with .NET provides additional benefits, especially for developers already working within the Microsoft stack. It allows for the reuse of existing .NET libraries and skills, facilitating a smoother development process.
Example: A Rich Media Gallery in Silverlight
Consider creating a rich media gallery using Silverlight’s RIA capabilities. This gallery could dynamically display high-resolution images and videos, offering features like zoom and pan for an enhanced user experience.
Here’s a basic XAML code snippet for a Silverlight image gallery:
<UserControl x:Class="MediaGallery.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Width="640" Height="480">
<Grid x:Name="LayoutRoot">
<ItemsControl x:Name="GalleryItems">
<!-- Template for gallery items -->
<ItemsControl.ItemTemplate>
<DataTemplate>
<Image Source="{Binding ImagePath}" Stretch="Fill" />
</DataTemplate>
</ItemsControl.ItemTemplate>
</ItemsControl>
</Grid>
</UserControl>
In this code, ItemsControl is used to display a collection of images, where ImagePath is a property in your data model that points to the image location.
Best Practices for Building Robust Silverlight and AJAX Applications
Developing robust Silverlight and AJAX applications requires adhering to certain best practices to ensure performance, maintainability, and scalability.
Efficient Coding and Design
- Modular Design: Structure your application in a modular way to make it easier to manage and update.
- Data Binding and MVVM: Use data binding and the Model-View-ViewModel (MVVM) pattern for a clean separation of concerns.
- Error Handling: Implement comprehensive error handling to make your application more reliable and user-friendly.
Ensuring Cross-Browser Compatibility
Given the diversity of web browsers, it’s crucial to ensure your Silverlight and AJAX applications work seamlessly across all major browsers. This includes regular testing and updates based on browser version changes.
Responsive Design
Responsive design is essential in today’s multi-device world. Ensure your Silverlight applications resize and adjust properly on different screens, maintaining usability and aesthetics.
Example: Responsive Silverlight Application
Creating a responsive layout in Silverlight involves using fluid layouts that adjust to the size of the user’s screen. For instance, using Grid with relative sizing instead of fixed dimensions:
<Grid x:Name="LayoutRoot">
<Grid.RowDefinitions>
<RowDefinition Height="*" />
<RowDefinition Height="2*" />
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="*" />
<ColumnDefinition Width="2*" />
</Grid.ColumnDefinitions>
<!-- Place other controls here -->
</Grid>
Conclusion
Silverlight and AJAX have significantly shaped the landscape of web development, offering lessons in creating rich, interactive applications. While technology trends shift towards new standards like HTML5, CSS3, and JavaScript frameworks, the insights gained from Silverlight and AJAX remain relevant. They serve as a foundation for modern development practices, emphasizing rich user experiences, responsive design, and efficient data handling. As the web continues to evolve, the principles learned from these technologies will continue to inform and guide developers in crafting innovative web solutions.