Introduction to Multithreading and Concurrency
The realm of software development is ever-evolving, and with the advent of multi-core processors, understanding multithreading and concurrency has become essential for C++ programmers. This section will provide a comprehensive introduction to these concepts, elucidating their significance in modern C++ programming.
What are Multithreading and Concurrency?
Multithreading refers to the ability of a CPU to execute multiple threads concurrently. A thread, in its simplest form, is a sequence of programmed instructions that the CPU can execute independently. This means that a single process can have multiple threads running in parallel, each handling a different task, leading to more efficient execution of complex programs.
Concurrency, on the other hand, is a broader concept. It’s the ability of the program to deal with many things at once. In programming, concurrency allows different parts of a program to execute independently. It’s not just about parallel execution, but also about managing multiple tasks that might not necessarily run at the same time.
Importance in Modern C++ Programming
In today’s computing world, harnessing the power of multithreading and concurrency is no longer optional but a necessity. The reasons are manifold:
- Performance Optimization: By utilizing multiple threads, programs can perform more tasks in a given amount of time. This is crucial for performance-critical applications like video editing software, gaming engines, and scientific simulations.
- Responsiveness: In applications with a user interface, separating the UI thread from other long-running tasks ensures that the application remains responsive to user inputs.
- Resource Utilization: Modern CPUs come with multiple cores. Multithreading and concurrency allow programs to fully utilize these cores, thereby enhancing the efficiency of the hardware.
- Scalability: Applications designed with concurrency in mind can scale more effectively. They can handle an increase in workload simply by adding more resources, without the need to restructure the entire application.
Understanding Threads in C++
The concept of threads is fundamental to implementing multithreading in C++. This section delves into the basics of thread creation and management in C++, focusing on the std::thread library, a powerful feature introduced in C++11 that simplifies working with threads.
Basics of Thread Creation and Management
In C++, a thread is an executable unit of code. When a program starts, it begins with a single thread, known as the main thread. Additional threads can be created to perform various tasks concurrently. Here’s a basic example of creating a thread:
#include <iostream>
#include <thread>
void function() {
std::cout << "Thread is running\n";
}
int main() {
std::thread myThread(function); // Creating a thread
myThread.join(); // Waiting for the thread to finish
return 0;
}
In this example, myThread is created to execute the function. The join() method is used to block the main thread until myThread completes its execution. This is crucial to prevent the program from exiting before the thread has finished its task.
std::thread and Its Functionalities
The std::thread class provides several functionalities to manage threads:
- Thread Creation: As shown in the example above, std::thread can be used to create a new thread.
- Joining Threads: The join() function waits for a thread to finish its execution. It is essential to ensure that the main program waits for all threads to complete.
- Detaching Threads: The detach() method allows a thread to execute independently of the main thread. This is useful when the thread needs to run in the background, and its completion time is not a concern for the main program’s flow.
- Thread IDs: Each thread has a unique identifier, which can be obtained using the get_id() method. This is useful for debugging and managing multiple threads.
- Thread Safety: When dealing with multiple threads, it’s vital to ensure that they do not access shared resources simultaneously in a way that leads to conflicts or data corruption.
Creating and managing threads in C++ requires careful consideration of how these threads interact with shared data and resources. The next section will explore synchronization mechanisms in C++, which are crucial for ensuring safe and efficient data sharing between threads.
Synchronization Mechanisms in C++
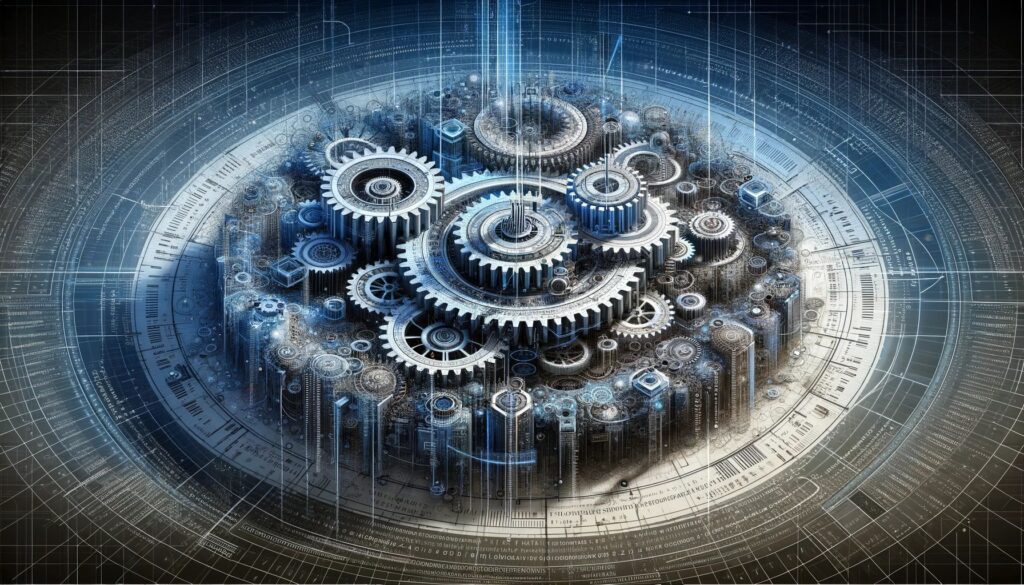
When dealing with multiple threads in a C++ program, ensuring safe access to shared resources is paramount. This is where synchronization mechanisms come into play. They are tools that help in coordinating the execution of threads to prevent race conditions, deadlocks, and other concurrency issues.
Mutexes, Locks, and Condition Variables
Mutex (Mutual Exclusion):
- A mutex is used to protect shared data. When a thread accesses shared data, it locks the mutex. This prevents other threads from accessing the same data until the mutex is unlocked.
- C++ provides several types of mutexes, like std::mutex, std::recursive_mutex, etc., each serving different use cases.
Locks:
- Locks are used in conjunction with mutexes. The std::lock_guard and std::unique_lock are two commonly used lock classes in C++.
- std::lock_guard is a scope-based lock, automatically locking the mutex when created and unlocking it when the scope ends.
- std::unique_lock is more flexible than std::lock_guard. It can lock and unlock the mutex multiple times within its scope, offering finer control.
Condition Variables:
- Condition variables are used for blocking a thread or multiple threads until a particular condition is met.
- They work in conjunction with a mutex. A thread waiting on a condition variable will be blocked until another thread signals the condition variable, indicating that the condition has been met.
Safe Data Sharing Between Threads
Consider the following simplified example demonstrating mutex and lock:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx; // Mutex for protecting shared data
int sharedData = 0;
void increment() {
std::lock_guard<std::mutex> lock(mtx);
++sharedData;
std::cout << "Data incremented to " << sharedData << std::endl;
}
int main() {
std::thread t1(increment);
std::thread t2(increment);
t1.join();
t2.join();
return 0;
}
In this example, std::lock_guard is used with std::mutex to ensure that only one thread at a time can access and modify sharedData. This prevents a race condition where two threads try to modify sharedData simultaneously.
Understanding and implementing these synchronization mechanisms are critical for writing effective multithreaded applications in C++. They ensure that the shared data is accessed in a controlled and safe manner, preventing data corruption and other concurrency-related issues.
Performance Considerations and Best Practices
Optimizing the performance of multithreaded applications in C++ requires a nuanced approach. This entails a mix of strategic planning and technical understanding of thread interactions and hardware.
Efficient Thread Management
Effective thread management is critical. This involves avoiding the overhead associated with creating and destroying threads, which can be resource-intensive. A practical approach is to use a thread pool to manage existing threads for recurring tasks. Additionally, balancing the workload evenly among threads is crucial to prevent scenarios where some threads are underutilized while others are overwhelmed.
Minimizing Lock Contention
Reducing lock contention is another key aspect. Employing fine-grained locks that cover smaller sections of code can significantly decrease waiting times for threads. Furthermore, lock-free programming, which uses lock-free data structures and algorithms, can be a viable alternative to traditional locking mechanisms, helping to reduce overhead.
Handling Race Conditions and Deadlocks
Ensuring that shared resources are adequately protected with mutexes is essential for preventing race conditions. To avoid deadlocks, strategies such as lock ordering or using std::lock to acquire multiple mutexes simultaneously can be effective.
Optimizing for CPU Cache Usage
Understanding and optimizing for CPU cache usage can yield significant performance improvements. This involves structuring data and access patterns to maximize cache locality, thus minimizing expensive memory accesses. Developers also need to be mindful of false sharing, where threads modify variables residing on the same cache line, and restructure data accordingly to avoid it.
Profiling and Monitoring
Regular use of profiling tools is indispensable for identifying performance bottlenecks and inefficient thread usage. Continuous monitoring of thread performance and resource utilization in real-world scenarios is also crucial for detecting issues that might not be apparent during testing.
Testing for Concurrency Issues
Conducting thorough testing under various conditions, including high loads and edge cases, is vital for ensuring the stability of multithreaded applications. Tools that simulate different thread interleavings are particularly useful for uncovering hidden concurrency issues.
Documentation and Code Clarity
Finally, maintaining clear documentation and commenting is essential, especially given the complexity of multithreaded logic. Writing readable and maintainable code not only facilitates future debugging and understanding but also aids in the overall maintainability of the software.
Advanced Topics in C++ Concurrency
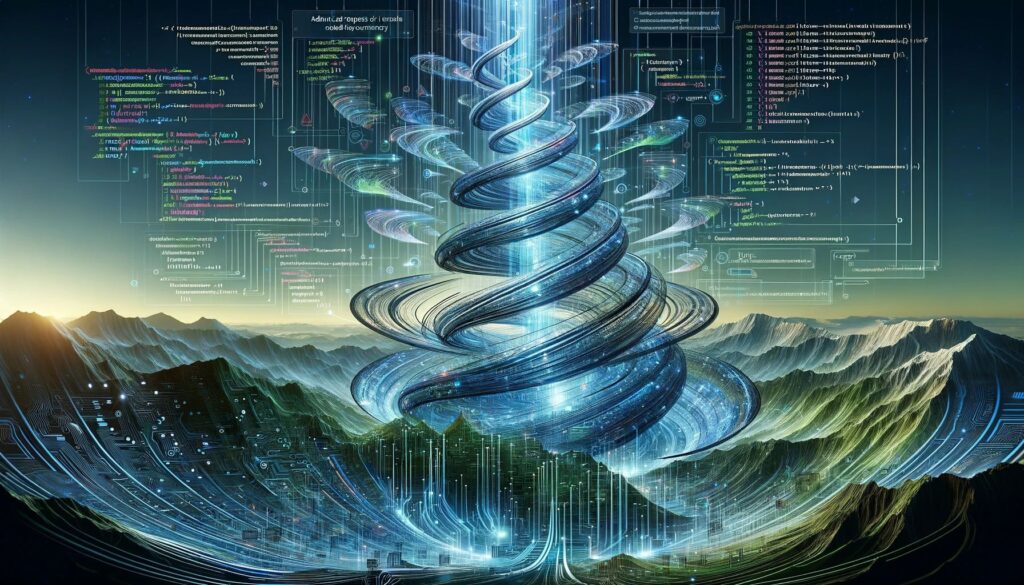
As we delve deeper into the realm of C++ concurrency, we encounter advanced topics that push the boundaries of what can be achieved with multithreaded programming. These topics not only enhance the capabilities of developers but also open up new possibilities for optimizing and scaling applications.
Future, Promise, and Async Programming
One of the significant advancements in C++ concurrency is the introduction of futures, promises, and asynchronous programming. These concepts, introduced in C++11, provide powerful tools for handling asynchronous operations.
- Futures and Promises: A future is an object that holds the result of an asynchronous operation, which may not be available yet. A promise, on the other hand, is an object that provides a way to fulfill a value to be used by a future. Together, they enable efficient communication between threads in asynchronous operations.
- Async Programming: The std::async function launches a task asynchronously and returns a future that will eventually hold the result of the task. This simplifies the process of starting new threads and managing their results.
Parallel Algorithms and Libraries
The introduction of parallel algorithms in C++17 marked a significant step forward in simplifying parallel programming. These algorithms allow developers to perform standard operations like sorting, searching, and numeric operations in parallel, without the complexity of manually managing threads.
- Parallel Execution Policies: Algorithms in the Standard Template Library (STL) can now be executed with different policies, such as std::execution::seq (sequential), std::execution::par (parallel), and std::execution::par_unseq (parallel and vectorized).
- Third-Party Libraries: Libraries like Intel TBB (Threading Building Blocks) and Microsoft’s PPL (Parallel Patterns Library) provide additional capabilities for parallel programming, offering various patterns and data structures optimized for concurrency.
Advanced Synchronization Techniques
Beyond basic mutexes and locks, advanced synchronization techniques offer more nuanced control over thread interaction.
- Reader-Writer Locks: std::shared_mutex introduced in C++17 allows multiple threads to read a shared resource simultaneously while still providing exclusive access for writing.
- Atomic Operations: Atomic operations on shared variables ensure safe manipulation without the need for locks. C++ provides std::atomic for this purpose, allowing lock-free programming for certain types of data.
Conclusion
Exploring multithreading and concurrency in C++ has highlighted its critical role in modern software development. As technology evolves, especially with the ubiquity of multi-core processors, mastering these concepts in C++ is essential. We’ve seen the importance of efficient thread management, the necessity of synchronization to avoid common issues like race conditions and deadlocks, and the diverse applications of multithreading across various domains. Performance optimization, employing best practices in thread management and synchronization, is key to creating reliable and efficient software.
Looking to the future, C++ continues to evolve, particularly in concurrency and parallel programming. This evolution demands continuous learning and adaptation from developers. Whether you are an experienced C++ programmer or just starting, understanding and applying these advanced concepts is crucial. It elevates your programming capabilities, opening new avenues for innovation and efficient software development. In the dynamic field of software development, embracing these advancements and challenges is essential for growth and success.