In the realm of Java development, the persistence of data is a critical aspect that dictates the efficiency and scalability of applications. Two pivotal technologies in this domain are the Java Persistence API (JPA) and Hibernate. This section delves into their core concepts, illustrating why they are indispensable in modern Java-based enterprise solutions.
Understanding JPA and Hibernate
Java Persistence API (JPA) is a Java specification that provides a standardized way to access, persist, and manage data between Java objects and relational databases. JPA operates as a bridge between object-oriented domain models and relational database systems, ensuring that data is stored and retrieved efficiently and consistently.
Hibernate, on the other hand, is an open-source, object-relational mapping (ORM) tool for Java. It implements the JPA specifications and provides additional features that enhance data management and performance tuning. Hibernate simplifies the complexities of JDBC (Java Database Connectivity) such as handling database sessions, transactions, and query optimizations, making it a preferred choice for developers dealing with relational data storage in Java.
The Importance in Java Development
The significance of JPA and Hibernate in Java development lies in their ability to abstract the underlying database interactions, allowing developers to focus on the business logic rather than the intricacies of SQL queries and database connections. Key benefits include:
- Simplified Data Management: JPA and Hibernate manage the CRUD (Create, Read, Update, Delete) operations, reducing the amount of boilerplate code and potential for errors.
- Database Agnosticism: They provide a database-agnostic approach, meaning applications can switch between different database vendors with minimal changes to the code.
- Improved Performance: Features like lazy loading, caching, and batch processing optimize the performance of database operations.
- Scalability and Maintainability: With their well-structured approach to handling data, JPA and Hibernate contribute significantly to the scalability and maintainability of Java applications.
- Rich Query Capabilities: Hibernate, in particular, offers HQL (Hibernate Query Language), which is an object-oriented query language, making it easier to write complex queries.
A Glimpse into Real-World Applications
In real-world applications, JPA and Hibernate are used in various sectors including finance, e-commerce, healthcare, and more. They are instrumental in managing vast amounts of data, ensuring transactional integrity, and providing a seamless data access layer in enterprise applications.
Setting Up the Environment
Setting up the environment for working with JPA and Hibernate is a foundational step in leveraging these technologies effectively. This section guides you through configuring JPA and Hibernate within a Spring Boot application, a common setup in many Java projects.
Required Dependencies
To get started, add the following dependencies to your Maven pom.xml
file. These dependencies include the core Hibernate framework and Spring Boot’s starter for data JPA, which simplifies the configuration process.
<dependencies>
<!-- Hibernate Core -->
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>6.3.0.Final</version>
</dependency>
<!-- Spring Boot Starter Data JPA -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
<version>3.1.0</version>
</dependency>
</dependencies>
Configuration
Spring Boot simplifies the configuration of Hibernate as the default JPA provider. The application.properties
or application.yml
file in your Spring Boot project can be used to configure the database connection and JPA settings. Here’s an example using MySQL:
# DataSource settings
spring.datasource.url=jdbc:mysql://localhost:3306/mydatabase
spring.datasource.username=myuser
spring.datasource.password=mypassword
# JPA/Hibernate settings
spring.jpa.show-sql=true
spring.jpa.hibernate.ddl-auto=update
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQL5Dialect
In this configuration, spring.jpa.show-sql
is set to true
to display the SQL statements in the console, which is helpful for debugging. The spring.jpa.hibernate.ddl-auto
property is set to update
, which allows Hibernate to update the database schema based on entity classes.
EntityManager Configuration
The EntityManager
is the primary JPA interface used to interact with the persistence context. In a Spring Boot application, the EntityManager
is automatically configured and can be injected into your components. Here’s an example of a simple repository class using EntityManager
:
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import org.springframework.stereotype.Repository;
@Repository
public class BookRepository {
@PersistenceContext
private EntityManager entityManager;
public Book findBook(Long id) {
return entityManager.find(Book.class, id);
}
// Additional CRUD methods
}
Basic Concepts: Entities and Mappings
In the realm of JPA and Hibernate, entities and their mappings form the backbone of how Java classes correspond to database tables. This section explores how to define entities and map them effectively to leverage the power of JPA and Hibernate.
Defining JPA Entities
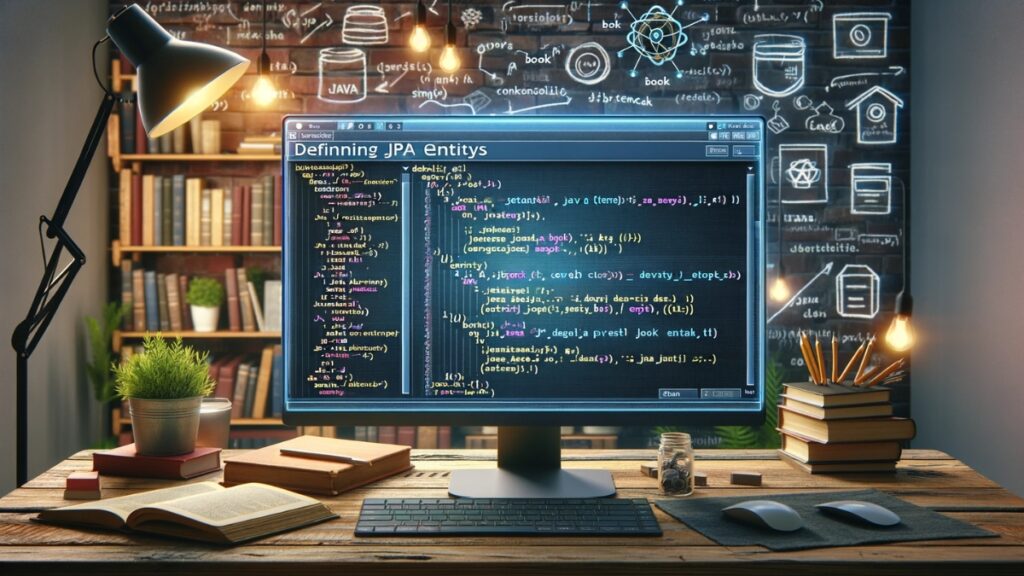
An entity in JPA is a lightweight, persistent domain object. To define a Java class as an entity, it needs to be annotated with @Entity
. This signals to JPA that the class should be mapped to a table in the database. Here’s a simple example of an entity representing a Book
:
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class Book {
@Id
private Long id;
private String title;
// Standard getters and setters
}
In this example, @Id
marks the id
field as the primary key of the entity. Each entity must have a primary key, which uniquely identifies each instance of the class in the database.
Mapping Fields to Columns
Mapping fields to database columns is done via annotations. The @Column
annotation is used to specify the details of a column in a database table. Here’s how you can customize mappings for the Book
entity:
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class Book {
@Id
private Long id;
@Column(name = "title", nullable = false, length = 100)
private String title;
// Standard getters and setters
}
In this example, the title
field is mapped to a column named “title” in the database. The nullable = false
attribute indicates that the column cannot be null, and length = 100
sets the maximum length of the column.
Specifying the Primary Key
Primary keys in entities can be assigned in different ways. The @GeneratedValue
annotation specifies how the primary key is populated, with various strategies like AUTO
, IDENTITY
, SEQUENCE
, or TABLE
. Here’s an example:
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
// Standard getters and setters
}
Entity Relationships in JPA
Entities often have relationships with other entities. JPA supports various types of relationships like @OneToOne
, @OneToMany
, @ManyToOne
, and @ManyToMany
. For instance, if a Book
has a Author
, it can be represented as a @ManyToOne
relationship:
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.ManyToOne;
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
@ManyToOne
private Author author;
// Standard getters and setters
}
In this setup, a Book
entity is associated with an Author
entity, representing a many-to-one relationship from the perspective of the Book
.
CRUD Operations with JPA and Hibernate
Performing Create, Read, Update, and Delete (CRUD) operations is a fundamental aspect of interacting with databases in JPA and Hibernate. Let’s explore how these operations can be implemented in a Java application.
Create Operation
To create or persist a new entity in the database, you use the EntityManager
‘s persist
method. Here’s an example of how to persist a new Book
entity:
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import org.springframework.transaction.annotation.Transactional;
public class BookRepository {
@PersistenceContext
private EntityManager entityManager;
@Transactional
public void addBook(Book book) {
entityManager.persist(book);
}
}
In this example, the @Transactional
annotation ensures that the operation is wrapped in a transaction.
Read Operation
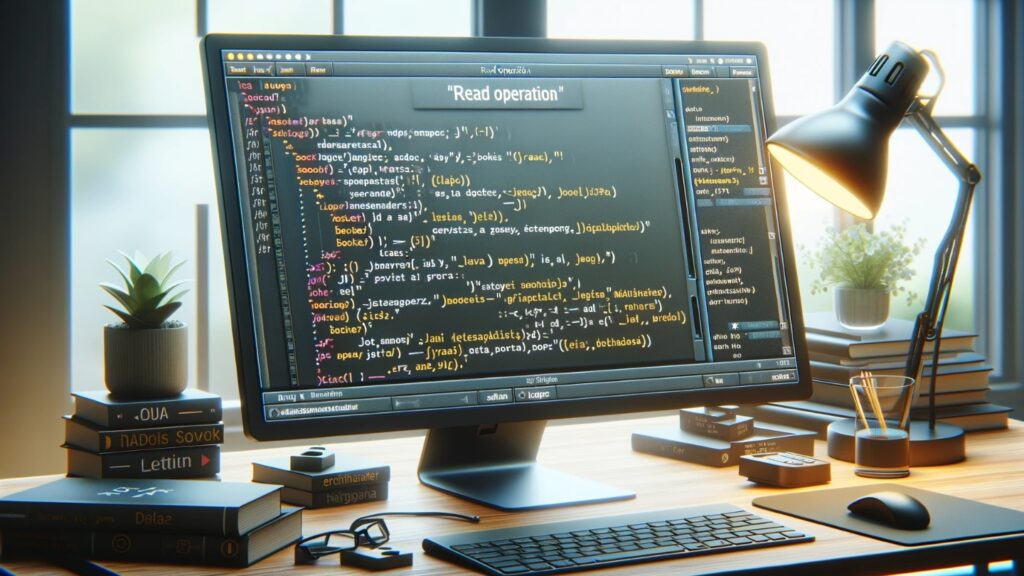
To read or retrieve an entity, you can use the find
method of EntityManager
. For instance, retrieving a Book
by its ID would look like this:
@Transactional(readOnly = true)
public Book findBookById(Long id) {
return entityManager.find(Book.class, id);
}
This method returns the Book
entity if found, or null
if no entity is found with the specified ID.
Update Operation
Updating an entity involves retrieving it, modifying its properties, and then letting the transaction commit. Since JPA tracks entity states within a transaction, no explicit ‘update’ or ‘save’ method call is needed. Here’s how you might update a Book
:
@Transactional
public void updateBookTitle(Long id, String newTitle) {
Book book = entityManager.find(Book.class, id);
if (book != null) {
book.setTitle(newTitle);
}
}
When the transaction commits, changes made to the book
entity will be automatically persisted.
Delete Operation
To delete an entity, you retrieve it and then use the remove
method of EntityManager
. Here’s an example of deleting a Book
:
@Transactional
public void deleteBook(Long id) {
Book book = entityManager.find(Book.class, id);
if (book != null) {
entityManager.remove(book);
}
}
This code retrieves a Book
by its ID and, if it exists, deletes it from the database.
Advanced Mapping and Relationships
In JPA and Hibernate, advanced mapping techniques and handling complex relationships between entities are key to building robust and scalable applications. Let’s explore some of these advanced concepts with practical examples.
Mapping JSON Documents in Hibernate
With Hibernate 6, mapping JSON documents to Java objects has become more straightforward. Here’s an example of mapping a JSON column to a Java object:
import javax.persistence.Embeddable;
import org.hibernate.annotations.JdbcTypeCode;
import java.io.Serializable;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import org.hibernate.type.SqlTypes;
@Embeddable
public class JsonData implements Serializable {
private String data;
// getters and setters
}
@Entity
public class MyEntity {
@Id
@GeneratedValue
private Long id;
@JdbcTypeCode(SqlTypes.JSON)
private JsonData jsonData;
// getters and setters
}
In this example, JsonData
is an @Embeddable
class mapped to a JSON column in the MyEntity
class.
Complex Relationship Mapping
Handling relationships like one-to-many and many-to-many can be achieved with JPA annotations. Here’s an example of a many-to-many relationship between Author
and Book
entities:
import javax.persistence.Entity;
import javax.persistence.ManyToMany;
import java.util.Set;
@Entity
public class Author {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@ManyToMany(mappedBy = "authors")
private Set<Book> books;
// getters and setters
}
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
@ManyToMany
private Set<Author> authors;
// getters and setters
}
In this setup, a Book
can have multiple Authors
, and an Author
can write multiple Books
.
Optimizing Relationships
Relationships in JPA can be optimized using concepts like lazy loading and cascading operations. Here’s an example of using lazy loading in a one-to-many relationship:
import javax.persistence.OneToMany;
import javax.persistence.FetchType;
@Entity
public class Author {
// ... other fields ...
@OneToMany(mappedBy = "author", fetch = FetchType.LAZY)
private Set<Book> books;
// getters and setters
}
In this example, the books
collection in the Author
entity is loaded lazily, meaning it’s loaded on-demand when accessed, rather than at the time the Author
entity is loaded.
Performance Optimization Techniques
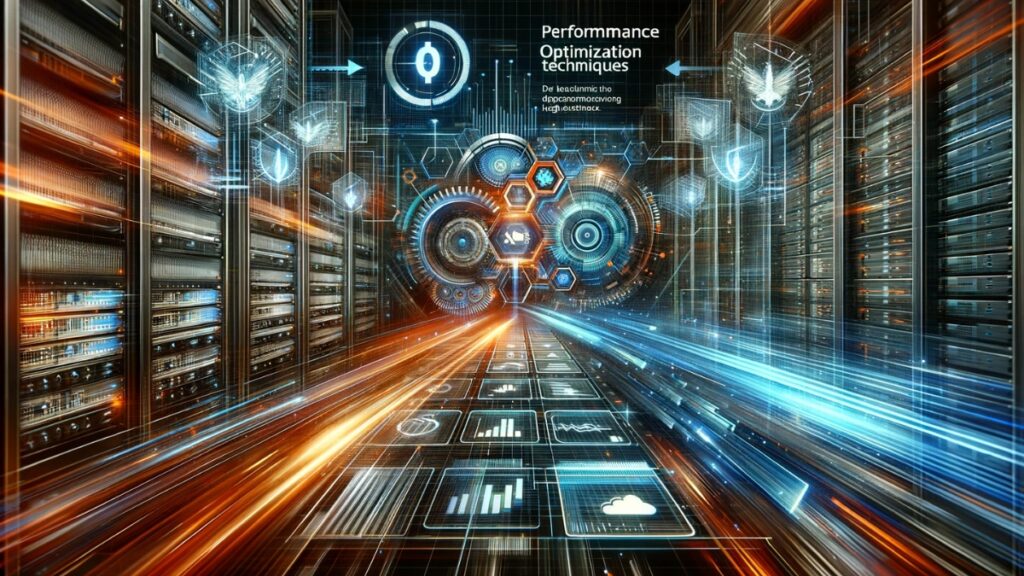
Optimizing performance in JPA and Hibernate is crucial for applications dealing with large datasets or requiring high throughput. This section covers some key strategies to enhance performance.
Batch Inserts and Updates
Batch processing can significantly improve the performance of bulk insert and update operations. In Hibernate, this can be configured via the hibernate.jdbc.batch_size
property. Here’s how to enable and use batch processing:
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.beans.factory.annotation.Value;
public class BatchRepository {
@PersistenceContext
private EntityManager entityManager;
@Value("${hibernate.jdbc.batch_size}")
private int batchSize;
@Transactional
public void batchInsert(List<Entity> entities) {
for (int i = 0; i < entities.size(); i++) {
entityManager.persist(entities.get(i));
if (i % batchSize == 0 && i > 0) {
entityManager.flush();
entityManager.clear();
}
}
}
}
In this example, entities are persisted in batches, reducing the number of database roundtrips. The flush
and clear
methods help manage memory efficiently during the process.
Lazy Loading and Fetch Strategies
Proper use of fetch strategies like lazy loading can significantly reduce the amount of unnecessary data loaded from the database. In JPA, you can control fetch strategies using FetchType.LAZY
or FetchType.EAGER
. Here’s an example of configuring lazy loading:
import javax.persistence.OneToMany;
import javax.persistence.FetchType;
@Entity
public class Author {
// ... other fields ...
@OneToMany(mappedBy = "author", fetch = FetchType.LAZY)
private Set<Book> books;
// getters and setters
}
This setup ensures that the books
collection is loaded only when explicitly accessed, rather than at the time the Author
entity is loaded.
Caching Strategies
Caching is another important aspect of performance optimization. Hibernate provides a powerful caching mechanism to reduce database hits. There are two levels of caching in Hibernate:
- First Level Cache: It’s enabled by default and tied to the
EntityManager
orSession
. - Second Level Cache: It’s a global cache shared across sessions and can be configured using cache providers like EHCache, Infinispan, or Hazelcast.
Here’s a basic example of enabling second-level caching for an entity:
import org.hibernate.annotations.Cache;
import org.hibernate.annotations.CacheConcurrencyStrategy;
@Entity
@Cache(usage = CacheConcurrencyStrategy.READ_WRITE)
public class Book {
// ... fields ...
}
In this example, the Book
entity is configured to use read-write caching strategy, making read operations faster and reducing the load on the database.
Handling Advanced Scenarios
As applications grow in complexity, JPA and Hibernate offer advanced features to handle sophisticated data models and transaction scenarios. This section explores some of these advanced capabilities with practical code examples.
Cascading Operations
Cascading is a powerful feature in JPA that propagates the state transition (like persist, delete) from a parent entity to its child entities. It’s useful in managing the state of related entities and can be particularly handy in one-to-many and many-to-one relationships. Here’s an example:
import javax.persistence.CascadeType;
import javax.persistence.OneToMany;
@Entity
public class Author {
// ... other fields ...
@OneToMany(mappedBy = "author", cascade = CascadeType.ALL)
private Set<Book> books;
// getters and setters
}
In this setup, operations like persist
or remove
on Author
will cascade to the Book
entities associated with it.
Handling Detached Entities
A common challenge in JPA is dealing with detached entities – instances that are no longer in sync with the database. To reattach a detached entity, you can use the merge
method of the EntityManager
. Here’s an example:
@Transactional
public Book updateDetachedBook(Book detachedBook) {
return entityManager.merge(detachedBook);
}
This method updates the entity in the database and returns a managed entity instance.
Advanced Querying
Beyond basic CRUD operations, JPA and Hibernate support advanced querying capabilities through JPQL (Java Persistence Query Language) and Criteria API. For complex queries, JPQL offers a way to write database queries using entity class and its fields instead of actual database tables. Here’s a simple JPQL example:
import javax.persistence.Query;
public List<Book> findBooksByAuthor(String authorName) {
Query query = entityManager.createQuery("SELECT b FROM Book b WHERE b.author.name = :name");
query.setParameter("name", authorName);
return query.getResultList();
}
The Criteria API provides a type-safe way to build queries programmatically. It’s particularly useful for dynamic queries based on varying runtime conditions:
import javax.persistence.criteria.CriteriaBuilder;
import javax.persistence.criteria.CriteriaQuery;
import javax.persistence.criteria.Root;
public List<Book> findBooksByTitle(String title) {
CriteriaBuilder cb = entityManager.getCriteriaBuilder();
CriteriaQuery<Book> cq = cb.createQuery(Book.class);
Root<Book> book = cq.from(Book.class);
cq.select(book).where(cb.equal(book.get("title"), title));
return entityManager.createQuery(cq).getResultList();
}
Conclusion
In conclusion, the exploration of Java Persistence API (JPA) and Hibernate provides a comprehensive insight into the effective management of data in Java applications. From setting up the environment and defining entities, to performing CRUD operations and handling complex relationships, these technologies offer a robust framework for dealing with relational data. Advanced features such as batch processing, lazy loading, caching, and cascading operations further enhance their utility in optimizing performance and handling sophisticated data models. As the field of Java development continues to evolve, the knowledge of JPA and Hibernate remains crucial for developers seeking to build scalable, efficient, and maintainable data-driven applications. This guide has aimed to equip you with a foundational understanding of these powerful tools, and as you delve deeper into their intricacies, they will undoubtedly become invaluable assets in your Java development toolkit.