Data science is a vast field that relies on understanding and manipulating data to extract meaningful insights. At the core of this process are two powerful tools: SQL and Python. SQL, or Structured Query Language, is the standard language for relational database management and data manipulation. Python, on the other hand, is a versatile programming language known for its readability and comprehensive libraries, making it a favorite among data scientists.
What is SQL?
SQL is a specialized language used to communicate with databases. It allows users to perform various operations like querying data, updating records, and managing databases. The language is highly efficient for managing structured data, where the relationships between different data entities are well defined. With SQL, data scientists can quickly retrieve and manipulate data stored in relational databases, a common scenario in many organizations.
Why Python for Data Science?
Python’s simplicity and elegance make it an ideal choice for data science. Its syntax is intuitive and easy to learn, which lowers the barrier to entry for newcomers. More importantly, Python boasts an extensive ecosystem of libraries and frameworks designed specifically for data analysis, machine learning, and scientific computing. Libraries like Pandas, NumPy, and SciPy provide robust tools to handle, analyze, and visualize data, while frameworks such as TensorFlow and Scikit-learn offer advanced machine learning capabilities.
The Synergy of SQL and Python in Data Science
Combining SQL’s powerful data retrieval capabilities with Python’s analytical tools creates a potent mix for data science. Data scientists can leverage SQL to extract and preprocess data from databases and then use Python for more complex data analysis and visualization tasks. This synergy allows for a more streamlined workflow, from data extraction to deriving insights.
Both SQL and Python are not just academic tools; they have vast applications in the real world. Industries like finance, healthcare, and retail use SQL to manage their vast databases. Python’s applications are even more widespread, ranging from web development to artificial intelligence. In the context of data science, these tools are used for tasks such as customer segmentation, predictive modeling, market basket analysis, and much more.
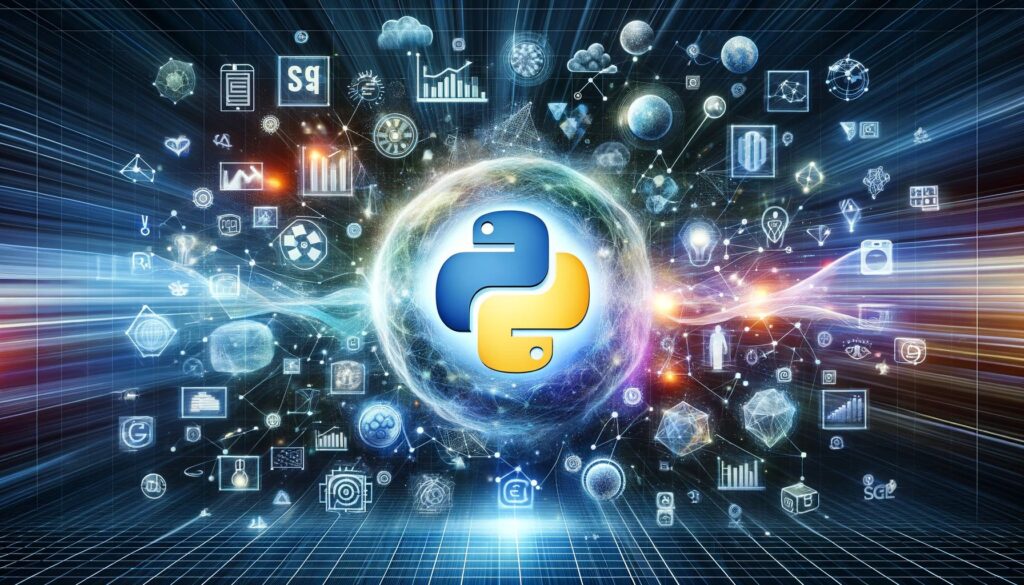
Setting Up Your Environment
Before you can start harnessing the power of SQL and Python for data science, you need to set up your environment. This involves installing the necessary software and libraries that will allow you to code, execute queries, and perform analysis. This section will guide you through the basic setup to get you started.
Installing Python
Python is widely used and supported on most operating systems, including Windows, Mac, and Linux. To begin, you need to install Python on your system. The most straightforward method is to download the latest version from the official Python website. Alternatively, distributions like Anaconda can simplify the process by managing both Python and the libraries you’ll need.
Choosing a Code Editor
Next, you’ll need a code editor or an Integrated Development Environment (IDE) where you can write and execute your Python code. Some popular options include:
- PyCharm: A powerful IDE with features like code completion and debugging.
- Jupyter Notebook: An open-source web application that allows you to create and share documents containing live code, equations, visualizations, and narrative text.
- Visual Studio Code: A versatile editor that supports Python and many other languages.
Installing SQL Dependencies
To interact with SQL databases using Python, you’ll need to install specific libraries. The choice of library might depend on the type of SQL database you are using (MySQL, PostgreSQL, SQLite, etc.). Some commonly used libraries include:
- PyMySQL: A pure Python MySQL client.
- SQLite: A C library that provides a lightweight disk-based database and is fully integrated with Python.
- SQLAlchemy: A SQL toolkit and Object-Relational Mapping (ORM) library for Python. It provides a full suite of well-known enterprise-level persistence patterns.
You can install these libraries using Python’s package manager, pip. For example:
pip install PyMySQL
pip install SQLAlchemy
Setting Up a Database
You’ll also need access to an SQL database where you can practice running queries. If you don’t have access to an existing database, you can install a local SQL server on your machine. MySQL and PostgreSQL are popular open-source databases you can start with. Follow the installation guide provided by the database you choose.
Testing Your Setup
Once everything is installed, it’s a good idea to test your setup to ensure that Python and the SQL libraries are working correctly. You can do this by writing a simple script in Python that connects to your SQL database and executes a basic query.
For example, here’s a simple Python script that connects to a MySQL database and fetches data:
import pymysql
# Connect to the database
connection = pymysql.connect(host='localhost',
user='your_username',
password='your_password',
db='your_database')
try:
with connection.cursor() as cursor:
# Execute a query
sql = "SELECT `id`, `name` FROM `users`"
cursor.execute(sql)
# Fetch all the rows
result = cursor.fetchall()
for row in result:
print(row)
finally:
connection.close()
Replace ‘your_username’, ‘your_password’, and ‘your_database’ with your actual MySQL username, password, and database name. If everything is set up correctly, this script should print the id and name of all users in the “users” table of your database.
Understanding SQL Basics
To effectively use SQL with Python for data science, you need a solid understanding of SQL basics. SQL is used to communicate with a database, allowing you to create, read, update, and delete (CRUD) the data. This section will introduce you to key SQL concepts and commands necessary for manipulating and retrieving data.
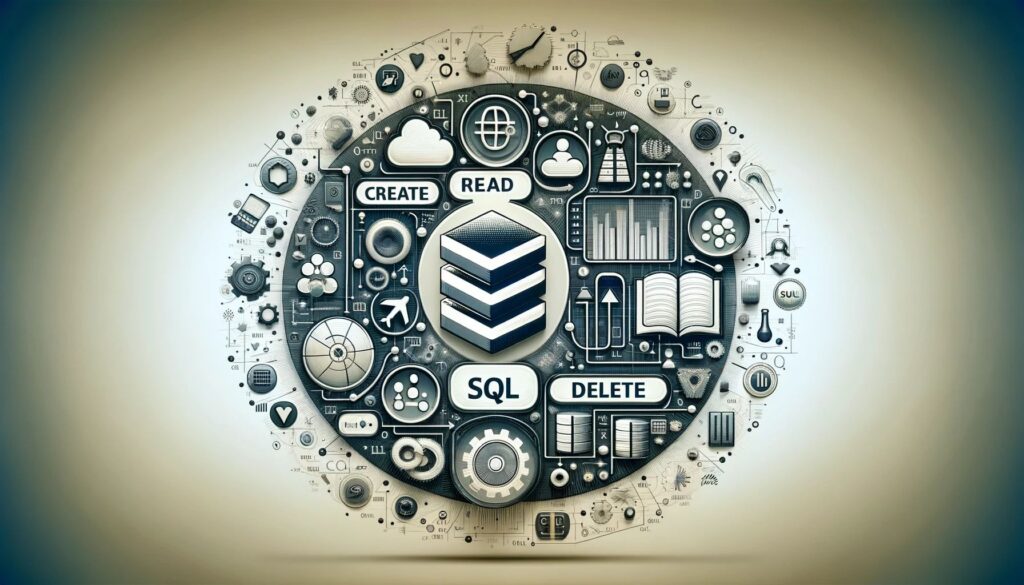
What is a Database?
A database is an organized collection of data. It’s typically structured in a way that makes the data easily accessible. Most databases you’ll encounter in the realm of data science are relational databases, which organize data into one or more tables.
Basic SQL Commands
- SELECT: This command is used to select data from a database. The data returned is stored in a result table, sometimes called the result-set.
SELECT column1, column2 FROM table_name;
- WHERE: This command is used to filter records. It’s used to extract only those records that fulfill a specified condition.
SELECT column1, column2 FROM table_name WHERE condition;
- INSERT INTO: This command is used to insert new records in a table.
INSERT INTO table_name (column1, column2) VALUES (value1, value2);
- UPDATE: This command is used to modify the existing records in a table.
UPDATE table_name SET column1 = value1 WHERE condition;
- DELETE: This command is used to delete existing records in a table.
DELETE FROM table_name WHERE condition;
- CREATE DATABASE/TABLE: These commands are used to create a new database or table.
CREATE DATABASE database_name;
CREATE TABLE table_name (column1 datatype, column2 datatype);
Understanding Data Types
In SQL, each column in a table is required to have a name and a data type. Data types define the kind of value that each column can hold: integer, text, date, etc. Some common data types you might encounter include:
- INT: A whole number (integer).
- VARCHAR(n): A string of text of up to n characters.
- DATE: A date in the format YYYY-MM-DD.
Working with Tables
Tables are a crucial component of databases. They hold all the data in rows and columns. Here’s how you might create a simple table:
CREATE TABLE users (
id INT AUTO_INCREMENT,
name VARCHAR(50),
email VARCHAR(50),
signup_date DATE,
PRIMARY KEY (id)
);
This command creates a new table called ‘users’ with columns for id, name, email, and signup date.
Retrieving Data
The most common task in SQL is retrieving specific data from a database. This is typically done with a SELECT statement. For instance:
SELECT name, email FROM users WHERE signup_date > '2020-01-01';
This command will return the name and email of all users who signed up after January 1, 2020.
Manipulating Data
Once data is stored in a database, you might need to update or delete it. The UPDATE and DELETE commands are used for this purpose. For instance:
UPDATE users SET email = '[email protected]' WHERE id = 1;
DELETE FROM users WHERE id = 1;
These commands will update the email of the user with id 1 and then delete the user from the ‘users’ table.
Now that you have a grasp of the basics of SQL, you can start experimenting with your own queries and tables. Understanding these fundamentals is crucial before moving on to more advanced querying techniques and integrating SQL with Python for data analysis and visualization, which we’ll explore in the following sections.
Diving into Python for Data Manipulation
After establishing a foundational understanding of SQL, it’s time to explore how Python can elevate your data manipulation capabilities. Python is not just a programming language; it’s a powerful tool that, when combined with SQL, can handle, analyze, and visualize data in ways that SQL alone cannot. This section will introduce Python’s primary libraries for data science and demonstrate how you can integrate Python with SQL databases.
Python Libraries for Data Science
- Pandas: Pandas is an open-source library providing high-performance, easy-to-use data structures, and data analysis tools for Python. Its primary data structure is called DataFrame, which allows you to store and manipulate tabular data in rows of observations and columns of variables.
- NumPy: NumPy is the fundamental package for scientific computing with Python. It contains a powerful N-dimensional array object and tools for integrating C/C++ and Fortran code. It’s also useful in linear algebra, random number capability, and Fourier transform capabilities.
- SciPy: SciPy is a library used for scientific and technical computing. It builds on NumPy and provides a large number of functions that operate on numpy arrays and are useful for different types of scientific and engineering applications.
Integrating Python with SQL Databases
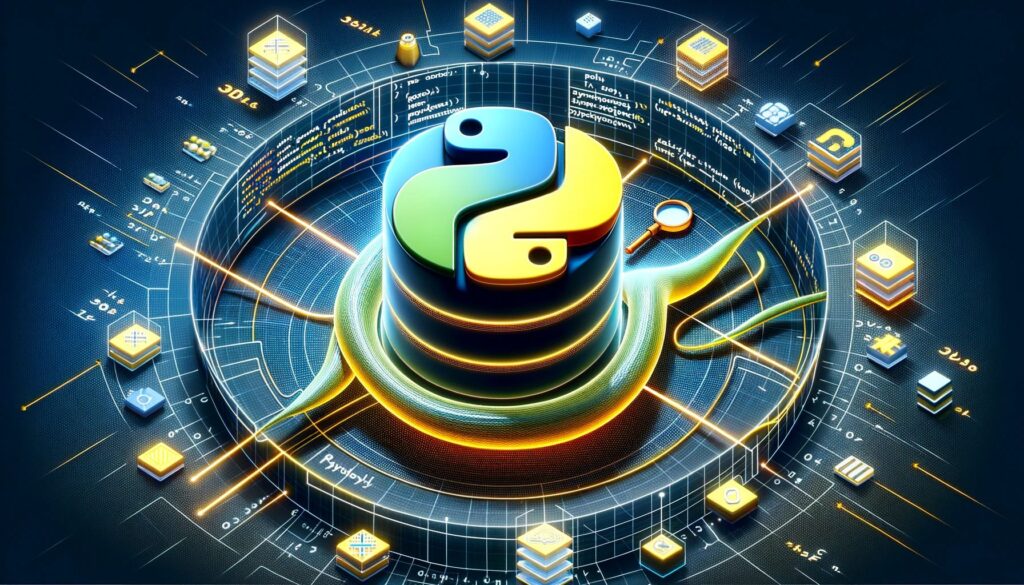
To connect Python with an SQL database, you’ll use libraries specifically designed for this purpose. The choice of library depends on the type of SQL database you are using. For instance, if you are working with MySQL, you might use PyMySQL, and for PostgreSQL, you might use psycopg2. Here’s how you can use these libraries to integrate Python with an SQL database:
- Connect to the Database: First, you need to establish a connection to your SQL database. Each library has its method to accomplish this, usually requiring you to provide details like the database server, database name, username, and password.
- Creating Cursors and Executing SQL: Once connected, you can create a cursor object and use it to execute SQL commands. The cursor allows you to interact with the database, executing commands and retrieving data.
- Fetching Data: After executing a SELECT command, you can use the cursor to fetch data. This might be one row, many rows, or all rows, depending on your needs.
Here’s a simple example of how you might connect to a MySQL database using Python and retrieve some data:
import pymysql
# Establishing the connection
connection = pymysql.connect(host='localhost',
user='your_username',
password='your_password',
db='your_database')
try:
with connection.cursor() as cursor:
# Executing a SQL command
cursor.execute("SELECT `id`, `name` FROM `users`")
# Fetching all rows
results = cursor.fetchall()
for row in results:
print(row)
finally:
connection.close()
Analyzing Data with Pandas
Once you’ve retrieved data from your SQL database, you can use Pandas to analyze it. You can load the data directly into a DataFrame and then perform various operations, such as filtering the data, calculating statistics, and creating visualizations. Here’s an example of how you might load SQL data into a Pandas DataFrame:
import pandas as pd
import pymysql
# Connect to the database
connection = pymysql.connect(host='localhost',
user='your_username',
password='your_password',
db='your_database')
# Query to select data
sql = "SELECT * FROM users"
# Loading data into a DataFrame
df = pd.read_sql(sql, connection)
# Display the DataFrame
print(df)
This script connects to a MySQL database, executes a SELECT command, and loads the results into a Pandas DataFrame, which you can then use for analysis.
Advanced Data Querying Techniques
Once you’re comfortable with the basics of SQL and Python, you can start exploring more advanced data querying techniques. These methods allow you to extract more complex and refined data from your databases, which can lead to deeper insights. This section will cover some advanced SQL querying techniques and show how you can use them in combination with Python.
Complex SQL Queries
Joins
- Purpose: Joins are used to combine rows from two or more tables, based on a related column between them.
- Types:
- Inner Join: Returns records that have matching values in both tables.
- Left (Outer) Join: Returns all records from the left table, and the matched records from the right table.
- Right (Outer) Join: Returns all records from the right table, and the matched records from the left table.
- Full (Outer) Join: Returns all records when there is a match in either left or right table.
- Example:
SELECT Orders.OrderID, Customers.CustomerName
FROM Orders
INNER JOIN Customers ON Orders.CustomerID = Customers.CustomerID;
Subqueries
- Purpose: A subquery is a query within another query. The outer query is called the main query, and the inner query is called the subquery.
- Example:
SELECT name
FROM users
WHERE id IN (SELECT user_id FROM orders WHERE amount > 100);
Views
- Purpose: A view is a virtual table based on the result-set of an SQL statement. It contains rows and columns, just like a real table, but the data comes from one or more tables.
- Example:
CREATE VIEW [Active Users] AS
SELECT name, email
FROM users
WHERE last_login > '2020-01-01';
Integrating Advanced SQL with Python
To utilize these advanced SQL techniques with Python, you’ll follow a similar process as with basic queries: connect to the database, create a cursor, execute the query, and fetch the results. Here’s how you might execute an advanced query, like a join, and work with the results in Python:
import pymysql
import pandas as pd
# Connect to the database
connection = pymysql.connect(host='localhost',
user='your_username',
password='your_password',
db='your_database')
try:
with connection.cursor() as cursor:
# Executing an advanced SQL command (Inner Join in this case)
sql = """
SELECT Orders.OrderID, Customers.CustomerName
FROM Orders
INNER JOIN Customers ON Orders.CustomerID = Customers.CustomerID;
"""
cursor.execute(sql)
# Fetching all rows
results = cursor.fetchall()
# Converting to DataFrame for easier manipulation and analysis
df = pd.DataFrame(results, columns=['OrderID', 'CustomerName'])
print(df)
finally:
connection.close()
In this script, you’re performing an inner join between Orders and Customers tables, fetching the results, and then converting those results into a Pandas DataFrame. Once in a DataFrame, you can perform various analyses and visualizations using Pandas and other Python libraries.
Data Analysis and Visualization
After retrieving and refining your data using SQL and Python, the next step is to analyze and visualize it to uncover patterns, trends, and insights. This section will focus on how you can use Python’s powerful libraries for data analysis and visualization, turning raw data into understandable and actionable information.
Data Analysis with Python
Python’s ecosystem has several libraries specifically designed for data analysis. Two of the most widely used are:
- Pandas:
- Purpose: Pandas provide data structures and functions needed to perform detailed analysis on datasets. It’s particularly useful for handling structured data where you want to perform operations like merging, reshaping, selecting, as well as slicing of data sets.
- Key Features:
- DataFrame: A two-dimensional, size-mutable, and potentially heterogeneous tabular data structure with labeled axes (rows and columns).
- Series: A one-dimensional array capable of holding any data type.
- Example Use: After loading your SQL data into a DataFrame, you might use Pandas to calculate statistics, like the mean or median of a column, or to filter out rows based on a condition.
- NumPy:
- Purpose: NumPy is primarily used for numerical calculations. It provides a high-performance multidimensional array object and tools for working with these arrays.
- Key Features:
- Array: A powerful N-dimensional array object.
- Broadcasting: A method used for vectorizing array operations, so they occur on whole arrays of data without the need for loops.
- Example Use: You might use NumPy in conjunction with Pandas for more complex numerical analysis, such as transforming data or performing statistical calculations.
Visualization with Python
Once you’ve analyzed your data, the next step is to visualize it. Visualization helps communicate data clearly and effectively through graphical means. Python offers multiple libraries for visualization, but two of the most popular are Matplotlib and Seaborn.
- Matplotlib:
- Purpose: Matplotlib is a plotting library for the Python programming language and its numerical mathematics extension, NumPy. It provides an object-oriented API for embedding plots into applications.
- Key Features:
- Plotting: Wide variety of plots and graphs, including line, bar, scatter, histogram, etc.
- Customization: Ability to customize every aspect of a plot.
- Example Use: You might use Matplotlib to create a histogram of a data column to understand its distribution or a line plot to see how a variable changes over time.
- Seaborn:
- Purpose: Seaborn is based on Matplotlib and provides a high-level interface for drawing attractive and informative statistical graphics.
- Key Features:
- Themes: Better default aesthetics and built-in themes.
- Complex Plots: Simplifies the creation of complex visualizations like heat maps, time series, and violin plots.
- Example Use: You might use Seaborn to create a heat map of correlation between different variables or a pair plot to understand the bivariate relationships in your dataset.
Example of Analysis and Visualization:
Let’s say you have a dataset of sales data in a Pandas DataFrame called sales_data
, and you’re interested in visualizing the monthly sales trends.
import pandas as pd
import matplotlib.pyplot as plt
# Sample data: Monthly sales data for a year
sales_data = pd.DataFrame({
'Month': ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'],
'Sales': [200, 220, 250, 275, 300, 320, 340, 360, 400, 420, 450, 480]
})
# Plotting the data
plt.figure(figsize=(10, 6))
plt.plot(sales_data['Month'], sales_data['Sales'], marker='o')
plt.title('Monthly Sales Trends')
plt.xlabel('Month')
plt.ylabel('Sales')
plt.grid(True)
plt.show()
This simple script uses Matplotlib to create a line plot of the sales data. You can see the monthly sales trends at a glance, with markers indicating the sales for each month.
By analyzing and visualizing your data, you can uncover patterns, identify trends, and make informed decisions. Python, with its powerful libraries and tools, makes this process efficient and insightful. In the next section, we’ll look at best practices and tips for optimizing your SQL and Python code for better performance and reliability.
Conclusion
In conclusion, the integration of SQL and Python forms a powerful combination for anyone in the field of data science. SQL’s robust data retrieval capabilities, coupled with Python’s extensive tools for analysis and visualization, provide a comprehensive environment for turning data into actionable insights. We’ve journeyed from setting up the environment and understanding SQL basics to advanced querying and efficient coding practices. This knowledge equips you with the skills necessary to handle complex data analysis tasks effectively.
As you continue to develop your expertise, remember that the learning journey in data science is ongoing. Leverage books, online courses, practice platforms, and communities to keep your skills sharp and stay abreast of the latest trends and techniques. Embrace challenges and projects to apply what you’ve learned and discover even deeper insights within your data.
Ultimately, the combination of SQL and Python in data science is not just about technical skills. It’s about the insights you can uncover and the value you can add through informed analysis. So, keep exploring, keep learning, and let your data guide you to new discoveries.