Silverlight, developed by Microsoft, is a powerful tool for building rich, interactive web applications. Its framework is designed to bring a new level of interactivity and user experience to the browser, rivaled only by standalone desktop applications. In this section, we delve into what makes Silverlight a standout choice for developing data-driven applications.
What is Silverlight?
Silverlight is a versatile technology for creating engaging web and mobile applications. It’s a browser plugin, similar in some respects to Adobe Flash, but with a focus on enabling .NET languages and development tools. This integration with .NET provides a robust environment for developers who are familiar with Microsoft technologies.
Key Features of Silverlight for Data-Driven Applications
- Rich UI Capabilities: Silverlight allows for the creation of visually appealing user interfaces with animations, vector graphics, and media playback.
- .NET Framework Integration: It leverages the .NET framework, enabling developers to write applications in C#, VB.NET, and other .NET languages.
- Powerful Data Binding: Silverlight offers strong data binding capabilities, crucial for data-driven applications. It allows UI components to be bound to data sources, making it easier to display, edit, and interact with data.
- Deep Zoom: This feature provides the ability to interactively view high-resolution images. It’s particularly useful in applications that require image analysis or detailed visualizations.
- Cross-Browser and Cross-Platform: Silverlight applications are not just limited to Windows; they can run on various browsers and operating systems including Mac OS and Linux.
- Advanced Networking Capabilities: Silverlight enables secure and scalable communication options, including support for WCF (Windows Communication Foundation) services.
The Importance of Silverlight in Data-Driven Applications
Data-driven applications are those that heavily rely on data manipulation, retrieval, and presentation. In these applications, the user interface often needs to be dynamic, updating in response to the underlying data changes. Silverlight excels in this area due to its advanced data binding and UI capabilities. Whether it’s a business dashboard, an interactive data report, or a complex data visualization, Silverlight provides the tools necessary to build these applications efficiently.
Setting Up Your Development Environment
To begin developing data-driven applications with Silverlight, it’s essential to set up the right development environment. This setup will ensure you have all the necessary tools and frameworks for efficient Silverlight development.
Tools and Frameworks
Visual Studio is the primary IDE for Silverlight development, offering robust debugging and design tools. The Silverlight Developer Runtime and SDK are essential for running and building Silverlight applications, respectively. Additionally, Expression Blend complements Visual Studio by enhancing graphical interface design, simplifying the creation of visually appealing user interfaces in the Silverlight development environment.
Basic Setup Steps
- Install Visual Studio: Start by installing Visual Studio. You can use any edition that supports Silverlight development, including the Community edition, which is free for individual developers.
- Install Silverlight Developer Runtime and SDK: After setting up Visual Studio, install the Silverlight Developer Runtime and SDK. These are critical for compiling and running Silverlight applications.
- Install Expression Blend: Although optional, Expression Blend is highly recommended for designers focusing on UI/UX of Silverlight applications.
Creating Your First Silverlight Project
- Open Visual Studio: Launch Visual Studio and select ‘New Project’.
- Choose a Silverlight Application: Navigate to the ‘Silverlight’ category and select ‘Silverlight Application’. Name your project and choose a location for it.
- Project Configuration: In the New Project dialog, you have the option to host the Silverlight application in a new Web site. This can be useful for testing and deployment.
- Exploring the Project Structure: Once the project is created, explore the Solution Explorer to understand the structure of a Silverlight application. Familiarize yourself with the main XAML file and the code-behind file.
Basic Silverlight Application Code
Here’s a snippet of a basic Silverlight application code that demonstrates a simple data binding:
<UserControl x:Class="SilverlightApp.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="400">
<Grid x:Name="LayoutRoot" Background="White">
<TextBlock Text="{Binding Message}" HorizontalAlignment="Center" VerticalAlignment="Center"/>
</Grid>
</UserControl>
This is a fundamental example of data binding in Silverlight.
Understanding Data Binding in Silverlight
Data binding is a powerful feature in Silverlight that allows developers to link UI elements to data sources, making it easier to display, edit, and update data. Understanding and utilizing data binding is crucial for creating dynamic, data-driven applications.
The Basics of Data Binding
In Silverlight, data binding involves connecting a property of a UI element to a property of an object (the data source). When the data source changes, the UI element automatically reflects these changes, and vice versa.
Types of Data Binding
- One-Way Binding: The UI element gets updated when the data source changes. This is useful for displaying data that doesn’t require user interaction.
- Two-Way Binding: Changes in the UI element update the data source, and changes in the data source update the UI element. Ideal for forms and interactive interfaces.
- One-Time Binding: The UI element is set with the data source’s value only once, when the application loads.
Implementing Data Binding in Silverlight
Here’s an example of implementing two-way data binding in a Silverlight application:
<UserControl x:Class="SilverlightApp.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="400">
<Grid x:Name="LayoutRoot" Background="White">
<TextBox Text="{Binding Path=Name, Mode=TwoWay}" Width="200" />
</Grid>
</UserControl>
The Mode=TwoWay ensures that any changes in the TextBox will update the Name property, and any changes in the Name property will reflect in the TextBox.
The ViewModel
The ViewModel in MVVM (Model-View-ViewModel) architecture plays a crucial role in data binding. It acts as an intermediary between the View (UI) and the Model (data), providing properties and commands that the View can bind to.
public class MainViewModel : INotifyPropertyChanged
{
private string _name;
public string Name
{
get { return _name; }
set
{
_name = value;
OnPropertyChanged("Name");
}
}
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged(string propertyName)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
In this ViewModel example, the Name property is bound to the UI. The OnPropertyChanged method is called whenever the Name property is changed, ensuring the UI stays in sync with the data.
Working with WCF Services in Silverlight
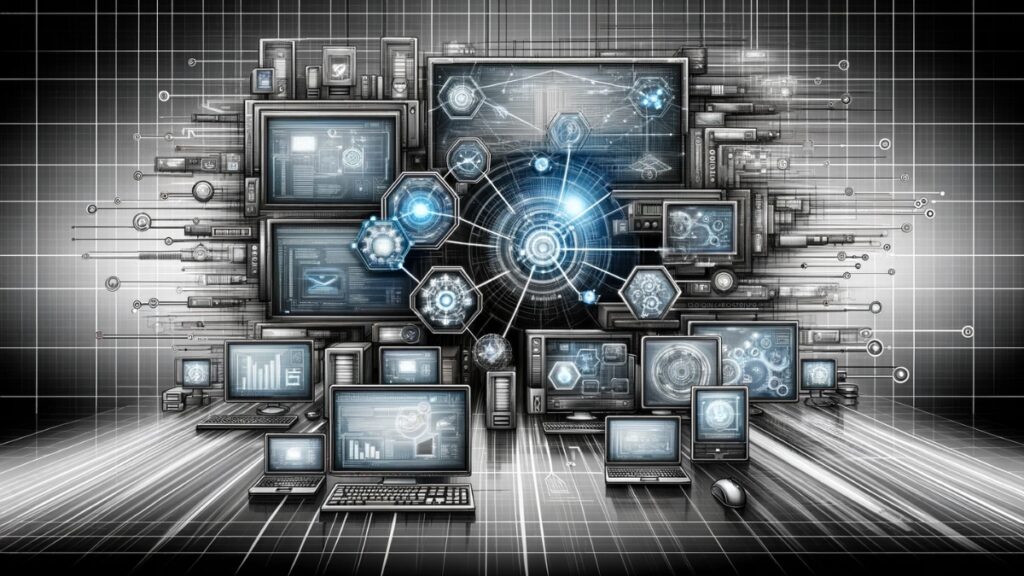
Interacting with web services is a common requirement in data-driven applications. Silverlight provides excellent support for consuming Windows Communication Foundation (WCF) services, enabling applications to communicate with server-side logic and databases.
Creating a Silverlight-Enabled WCF Service
WCF services can be tailored to work seamlessly with Silverlight clients. Here’s how you can create a basic WCF service for a Silverlight application:
- Creating the Service: In Visual Studio, add a new WCF Service to your web project. This will create a .svc file along with an interface (IService1.cs) and its implementation (Service1.svc.cs).
- Configuring the Service for Silverlight: Modify the binding in the WCF service configuration to basicHttpBinding, as Silverlight supports only basic HTTP communication.
Implementing the WCF Service
The service might have a simple operation like fetching data. For instance:
[ServiceContract]
public interface IService1
{
[OperationContract]
string GetData(int value);
}
public class Service1 : IService1
{
public string GetData(int value)
{
return $"You entered: {value}";
}
}
Consuming the WCF Service in Silverlight
1. Adding Service Reference: In your Silverlight project, add a reference to the WCF service. This generates proxy classes in the Silverlight application, enabling you to call the WCF service methods.
2. Calling the Service: You can now call the WCF service from your Silverlight code. For instance:
public partial class MainPage : UserControl
{
private Service1Client _client;
public MainPage()
{
InitializeComponent();
_client = new Service1Client();
_client.GetDataCompleted += new EventHandler<GetDataCompletedEventArgs>(_client_GetDataCompleted);
_client.GetDataAsync(123); // Sample data
}
void _client_GetDataCompleted(object sender, GetDataCompletedEventArgs e)
{
if (e.Error == null)
{
MessageBox.Show(e.Result);
}
}
}
In this code, we create an instance of the generated proxy client and call the GetDataAsync method. We also handle the GetDataCompleted event to receive the data from the service.
Binding Service Data to UI
You can bind the data received from the service to UI elements in Silverlight. For example, if the service returns a list of items, you can bind this list to a ListBox or a DataGrid.
Error Handling and Debugging
It’s crucial to handle errors effectively when dealing with asynchronous service calls. Always implement error handling in your service callbacks to catch and deal with any issues that arise during the service call.
Advanced Data Binding Techniques
After mastering basic data binding and WCF service integration, advancing to more sophisticated data binding techniques can significantly enhance the functionality and responsiveness of your Silverlight applications.
Hooking into the Data Binding Process
Silverlight’s data binding model allows for a deeper level of interaction by hooking into the binding process. This can be particularly useful for scenarios like validation or formatting data.
Implementing Value Converters
Value converters are an integral part of advanced data binding. They allow you to manipulate or transform the data as it passes between the source and the target. Here’s an example of a simple value converter that changes the text color based on a condition:
public class ColorConverter : IValueConverter
{
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
int input = (int)value;
return input > 10 ? Colors.Red : Colors.Green;
}
public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture)
{
throw new NotImplementedException();
}
}
You can use this converter in XAML as follows:
<UserControl.Resources>
<local:ColorConverter x:Key="ColorConverter"/>
</UserControl.Resources>
<TextBlock Text="{Binding Path=Value}" Foreground="{Binding Path=Value, Converter={StaticResource ColorConverter}}"/>
Data Binding and Collections
Binding to collections and handling changes dynamically is a common requirement.
public class ViewModel
{
public ObservableCollection<string> Items { get; private set; }
public ViewModel()
{
Items = new ObservableCollection<string>();
// Add items to the collection
}
}
In XAML, you can bind a ListBox to this collection:
<ListBox ItemsSource="{Binding Items}"/>
Whenever items are added or removed from the Items collection, the UI will automatically update to reflect these changes.
Using Data Templates for Customized Display
Data templates in Silverlight allow you to define a custom visual structure for displaying your data.
<DataTemplate x:Key="ProductTemplate">
<StackPanel Orientation="Horizontal">
<TextBlock Text="{Binding Name}"/>
<TextBlock Text="{Binding Price}"/>
</StackPanel>
</DataTemplate>
<ListBox ItemsSource="{Binding Products}" ItemTemplate="{StaticResource ProductTemplate}"/>
Implementing the Data Grid and DataForm
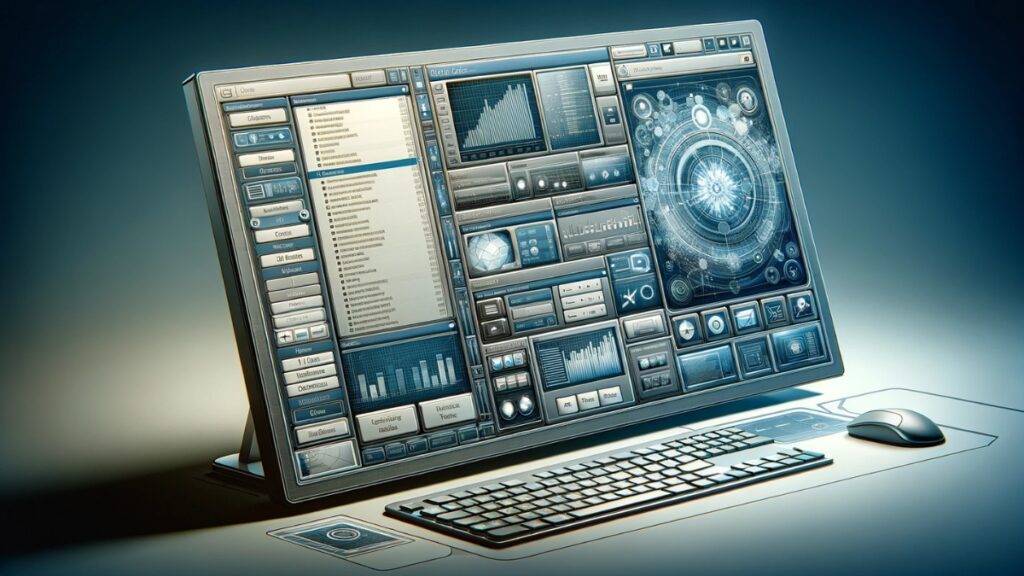
The Data Grid and DataForm are powerful controls in Silverlight that facilitate the display and editing of collections and complex data structures. They are essential for data-driven applications where user interaction with data is a key requirement.
The Data Grid
The Data Grid control is used for displaying and manipulating tabular data.
Basic Data Grid Implementation
Here’s how you can implement a simple Data Grid in Silverlight:
<sdk:DataGrid x:Name="dataGrid" AutoGenerateColumns="True" ItemsSource="{Binding Products}" />
In the above XAML, AutoGenerateColumns=”True” instructs the Data Grid to automatically create columns for each property in the Products collection. The ItemsSource is bound to a collection named Products in the ViewModel.
Customizing Columns
To customize the columns of the Data Grid, you can set AutoGenerateColumns to False and define your columns manually:
<sdk:DataGrid x:Name="dataGrid" AutoGenerateColumns="False" ItemsSource="{Binding Products}">
<sdk:DataGrid.Columns>
<sdk:DataGridTextColumn Header="Product Name" Binding="{Binding Name}" />
<sdk:DataGridTextColumn Header="Price" Binding="{Binding Price}" />
</sdk:DataGrid.Columns>
</sdk:DataGrid>
The DataForm
The DataForm control simplifies the process of displaying and editing the details of a single item from a collection.
Basic DataForm Implementation
Here’s an example of using DataForm to display and edit the details of a selected item from a Data Grid:
<sdk:DataForm x:Name="dataForm" AutoGenerateFields="True" CurrentItem="{Binding SelectedProduct, Mode=TwoWay}" />
In this example, the CurrentItem of the DataForm is bound to a property SelectedProduct in the ViewModel. This property represents the item selected in the Data Grid.
Linking Data Grid and DataForm
To create a master-detail interface, you can link the Data Grid and DataForm together. When a user selects an item in the Data Grid, the DataForm shows the details of that item for editing.
Here’s how you can link them:
public class ViewModel : INotifyPropertyChanged
{
private Product _selectedProduct;
public ObservableCollection<Product> Products { get; private set; }
public Product SelectedProduct
{
get { return _selectedProduct; }
set
{
_selectedProduct = value;
OnPropertyChanged("SelectedProduct");
}
}
// Implementation of INotifyPropertyChanged
}
In the XAML, bind the SelectedItem of the Data Grid to the SelectedProduct property of the ViewModel:
<sdk:DataGrid x:Name="dataGrid" ItemsSource="{Binding Products}" SelectedItem="{Binding SelectedProduct, Mode=TwoWay}" />
Interacting with Services
In Silverlight applications, effectively communicating with various services is crucial for fetching, updating, and managing data. Beyond WCF services, Silverlight can interact with RESTful services, SOAP services, and other web APIs.
Consuming RESTful Services
RESTful services are a popular choice due to their simplicity and ease of use. Silverlight can consume these services to perform CRUD (Create, Read, Update, Delete) operations on data.
Basic RESTful Service Consumption
Here’s an example of consuming a RESTful service in Silverlight using WebClient:
public void GetData()
{
WebClient webClient = new WebClient();
webClient.DownloadStringCompleted += new DownloadStringCompletedEventHandler(webClient_DownloadStringCompleted);
webClient.DownloadStringAsync(new Uri("http://example.com/api/data"));
}
void webClient_DownloadStringCompleted(object sender, DownloadStringCompletedEventArgs e)
{
if (e.Error == null)
{
string result = e.Result;
// Process result
}
}
Configuring Cross-Domain Calls
Silverlight enforces cross-domain access policies for security reasons. To make a service call to a domain other than the one hosting the Silverlight application, you need a client access policy file (clientaccesspolicy.xml) in the root of the target domain.
Example of clientaccesspolicy.xml:
<?xml version="1.0" encoding="utf-8"?>
<access-policy>
<cross-domain-access>
<policy>
<allow-from http-request-headers="*">
<domain uri="*"/>
</allow-from>
<grant-to>
<resource path="/" include-subpaths="true"/>
</grant-to>
</policy>
</cross-domain-access>
</access-policy>
This XML file allows all domains () to access the services hosted on the server.
Handling Data Serialization and Deserialization
When receiving data from services, it often comes in a serialized format like JSON or XML. Silverlight provides mechanisms to deserialize this data into usable objects.
JSON Serialization Example
If the RESTful service returns JSON, you can use the DataContractJsonSerializer to deserialize the JSON string into a .NET object:
public void DeserializeJson(string jsonString)
{
using (MemoryStream stream = new MemoryStream(Encoding.Unicode.GetBytes(jsonString)))
{
DataContractJsonSerializer serializer = new DataContractJsonSerializer(typeof(MyObject));
MyObject obj = (MyObject)serializer.ReadObject(stream);
// Use the deserialized object
}
}
[DataContract]
public class MyObject
{
[DataMember]
public string Property1 { get; set; }
[DataMember]
public int Property2 { get; set; }
}
In this example, MyObject is a custom class that represents the structure of the JSON data.
Cross-Domain Policy in Silverlight Applications
One of the unique challenges in Silverlight applications is handling cross-domain policy restrictions. These restrictions are in place for security reasons, preventing Silverlight applications from accessing services on domains other than the one from which they were downloaded.
Understanding Cross-Domain Restrictions
Silverlight applications, by default, can only access services on the same domain. To access services on a different domain, the service’s domain must explicitly allow it through a policy file.
Policy Files
There are two types of policy files:
- clientaccesspolicy.xml: Specific to Silverlight, it provides a highly configurable way to specify cross-domain access rules.
- crossdomain.xml: Common in Flash applications but also supported by Silverlight, offering a simpler set of rules.
Implementing a Cross-Domain Policy File
To allow a Silverlight application to access your service, you must place a clientaccesspolicy.xml file at the root of your domain.
Example clientaccesspolicy.xml:
<?xml version="1.0" encoding="utf-8"?>
<access-policy>
<cross-domain-access>
<policy>
<allow-from http-request-headers="SOAPAction">
<domain uri="http://clientdomain.com"/>
</allow-from>
<grant-to>
<resource path="/services" include-subpaths="true"/>
</grant-to>
</policy>
</cross-domain-access>
</access-policy>
This file allows access to the /services path for any requests coming from http://clientdomain.com.
Debugging Cross-Domain Issues
Debugging cross-domain issues can be tricky. Tools like Fiddler can be used to monitor HTTP traffic and verify whether the Silverlight application is attempting to access resources across domains and whether the policy files are being requested and received correctly.
Serialization and Entity Framework Integration
In a Silverlight application, integrating with databases often involves working with the Entity Framework and managing data serialization. This section will guide you through the basics of using Entity Framework in a Silverlight application and how to handle serialization effectively.
Using Entity Framework with Silverlight
Entity Framework is a popular ORM (Object-Relational Mapping) tool that allows developers to work with relational data using domain-specific objects.
Basic Integration Steps
- Create an Entity Data Model: In your web project, create an Entity Data Model (EDM) that maps to your database schema.
- Expose Data via WCF Services: Create WCF services that expose the data from your EDM to the Silverlight client.
public class ProductService : IProductService
{
private MyEntities _context = new MyEntities();
public List<Product> GetProducts()
{
return _context.Products.ToList();
}
}
1. Consume WCF Service in Silverlight: In your Silverlight application, add a service reference to the ProductService and use it to fetch data.
public partial class MainPage : UserControl
{
private ProductServiceClient _client;
public MainPage()
{
InitializeComponent();
_client = new ProductServiceClient();
_client.GetProductsCompleted += OnGetProductsCompleted;
_client.GetProductsAsync();
}
private void OnGetProductsCompleted(object sender, GetProductsCompletedEventArgs e)
{
if (e.Error == null)
{
ProductsList.ItemsSource = e.Result;
}
}
}
Handling Data Serialization
When data is transferred between the client and server in a Silverlight application, it’s serialized into a format like XML or JSON. Silverlight provides built-in support for data serialization.
JSON Serialization Example
public void SerializeToJson(Product product)
{
DataContractJsonSerializer serializer = new DataContractJsonSerializer(typeof(Product));
using (MemoryStream stream = new MemoryStream())
{
serializer.WriteObject(stream, product);
string json = Encoding.UTF8.GetString(stream.ToArray());
// Use the JSON string
}
}
public Product DeserializeFromJson(string json)
{
DataContractJsonSerializer serializer = new DataContractJsonSerializer(typeof(Product));
using (MemoryStream stream = new MemoryStream(Encoding.UTF8.GetBytes(json)))
{
return (Product)serializer.ReadObject(stream);
}
}
Debugging and Optimizing Silverlight Applications
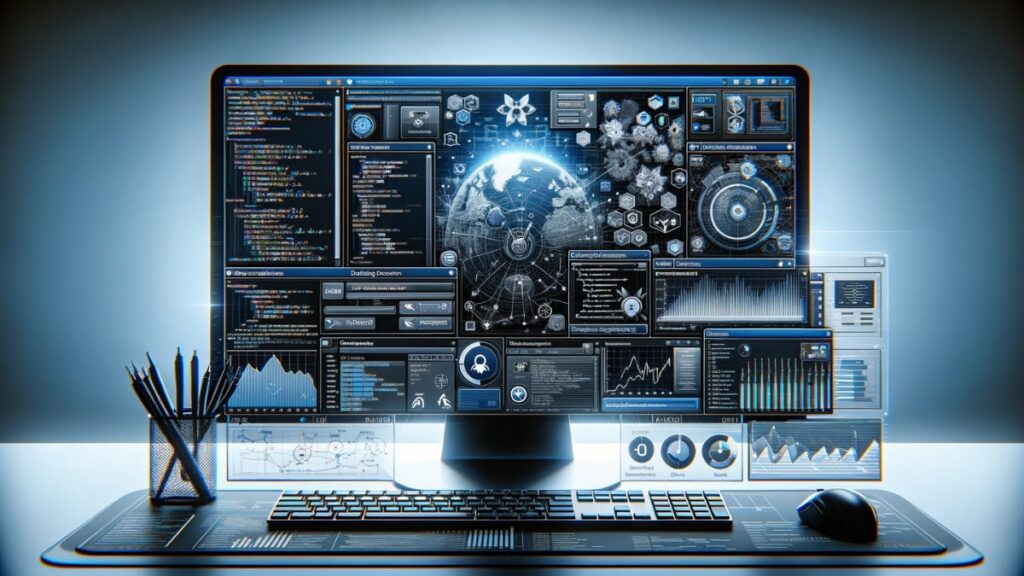
Optimizing and debugging are crucial for ensuring the smooth functioning of Silverlight applications, especially those dealing with complex data operations.
Techniques in Debugging
1. Using Visual Studio Debugger: The most common tool for debugging Silverlight applications is the Visual Studio Debugger. It allows you to set breakpoints, inspect variables, and step through the code.
- Example: Setting a breakpoint in a data retrieval method to inspect the returned data.
private void OnGetProductsCompleted(object sender, GetProductsCompletedEventArgs e)
{
// Set a breakpoint here to inspect 'e.Result'
if (e.Error == null)
{
ProductsList.ItemsSource = e.Result;
}
}
2. Debugging WCF Service Calls: For debugging WCF service calls, tools like Fiddler can be used to inspect the request and response messages.
- Tip: Check the SOAP messages in Fiddler to ensure that the requests and responses are correctly formatted.
3. Silverlight Developer Runtime: It provides detailed error messages that are crucial for debugging.
Performance Optimization
1. Data Binding Optimization: Efficient data binding is crucial for performance, especially when dealing with large datasets.
- Use INotifyPropertyChanged and ObservableCollection to ensure that only necessary UI updates are made.
public class ProductViewModel : INotifyPropertyChanged
{
// Implement INotifyPropertyChanged to update UI efficiently
}
2. Asynchronous Operations: Ensure all I/O operations, especially service calls, are asynchronous to prevent UI freezing.
- Example: Use asynchronous methods for WCF service calls.
_client.GetProductsAsync(); // Async call to prevent UI blocking
3. Optimizing XAML: Unnecessary complexity in XAML can lead to performance issues. Simplify your XAML by removing unused controls and optimizing data templates.
4. Caching: Implement caching for data that doesn’t change frequently to reduce the number of service calls.
- Example: Cache product data if it changes infrequently.
private void CacheProducts(List<Product> products)
{
// Implement caching logic
}
5. Minimizing Resource Usage: Optimize images and other resources used by the application. Large resources can significantly impact the performance and loading time.
Conclusion
Building data-driven Silverlight applications requires a solid foundation in data binding, service integration, and optimization. Mastering these skills empowers developers to create dynamic and responsive user interfaces, interact seamlessly with various services, and ensure optimal performance. Debugging tools and techniques play a crucial role in identifying and resolving issues, while optimization strategies enhance the application’s efficiency. By consistently applying these principles, developers can deliver robust and user-friendly data-driven Silverlight applications that meet the demands of modern web development.