In the evolving landscape of web development, the role of RESTful services has become increasingly vital. With the surge in demand for web applications that seamlessly integrate with various clients, including mobile devices and web browsers, the need for robust and scalable web services is more pronounced than ever. This scenario is where RESTful services shine, offering a standardized approach for designing networked applications. Moreover, the ASP.NET Web API framework emerges as a powerful tool for developers to build these RESTful services. It harnesses the full potential of REST principles, coupled with the rich features of the .NET framework, to create versatile and efficient web APIs. The following sections explore the core concepts of REST and the capabilities of the ASP.NET Web API, providing a roadmap for building state-of-the-art web services.
Understanding REST Principles
REST (Representational State Transfer) is an architectural style that defines a set of constraints for creating web services. RESTful services, or RESTful APIs, are designed to provide interoperability between computer systems on the internet. These services allow requesting systems to access and manipulate textual representations of web resources using a uniform and predefined set of stateless operations.
Key Characteristics of REST:
- Stateless: Each request from a client to a server must contain all the information needed to understand and process the request.
- Client-Server Architecture: Separation of concerns is the fundamental concept here. The client is not concerned with data storage, which remains internal to each server, and the server is not concerned with the user interface or user state.
- Cacheable: Clients can cache responses to improve performance, under certain conditions.
- Uniform Interface: This simplifies and decouples the architecture, enabling each part to evolve independently.
Overview of ASP.NET Web API Capabilities
ASP.NET Web API is a framework that makes it easy to build HTTP services that reach a broad range of clients, including browsers and mobile devices. It is an ideal platform for building RESTful applications on the .NET Framework.
ASP.NET Web API features:
- Flexibility: Supports various media types and is not limited to XML or JSON data formats.
- Routing: API routing is very flexible, and it is easy to define URI patterns that can map directly to service operations.
- Content Negotiation: The framework automatically selects the best media type formatter to handle the response data based on the request’s
Accept
header or the data type. - Self-hosting or IIS hosting: ASP.NET Web API can be hosted in IIS or in any other process that is capable of hosting .NET. This makes it a versatile choice for many types of web applications.
Setting Up Your Development Environment
To begin creating RESTful services with ASP.NET Web API, the first step is to set up the development environment. This setup involves installing the necessary tools and frameworks and initializing a new ASP.NET Web API project.
Installing Necessary Tools and Frameworks
- Visual Studio: Download and install Visual Studio. The Community Edition is free and sufficient for ASP.NET Web API development. Make sure to include the ASP.NET and web development workload during installation.
- .NET Framework: Ensure you have the latest .NET Framework installed, as it includes all the necessary libraries and runtime for ASP.NET Web API development.
- Postman or a Similar API Client: This tool is essential for testing your API endpoints. Postman allows you to make HTTP requests to your API and view responses without needing to create a front-end interface.
Creating a New ASP.NET Web API Project
- Open Visual Studio and select
Create a new project
.
- Selecting the Project Type:
- In the New Project dialog, go to the
ASP.NET Web Application
option. This template is specifically designed for building web applications, including RESTful services. - Ensure you choose
.NET Framework
(and not .NET Core) as the target framework for traditional ASP.NET Web API projects.
- In the New Project dialog, go to the
- Configuring the Web API Project:
- Once you select the template, you will be prompted to choose the project type. Select
Web API
. - This template automatically includes the necessary NuGet packages and project setup needed for a Web API.
- Once you select the template, you will be prompted to choose the project type. Select
- Project Structure:
- After creating the project, explore the Solution Explorer in Visual Studio to familiarize yourself with the structure of an ASP.NET Web API project.
- Key folders include
Controllers
,Models
, andApp_Start
.Controllers
will house your API controllers,Models
will contain your data models, andApp_Start
includes various configuration files.
- Understanding the Global.asax File:
- This file is crucial in ASP.NET applications. It contains code for application-level events fired by the ASP.NET application framework.
- In a Web API project, it configures the HTTP application and handles start-up tasks.
- Inspecting the WebApiConfig.cs File:
- Located in the
App_Start
folder, this file is where you configure API routes. - ASP.NET Web API uses a routing mechanism called
RouteAttribute
to define routes. It’s essential to understand how routing works in Web API to ensure your API endpoints are correctly exposed.
- Located in the
- Building Your First API Controller:
- Add a new controller by right-clicking on the
Controllers
folder and selectingAdd
->Controller
. Choose theWeb API Controller - Empty
option. - Implement a simple action method like
Get
in your controller. This method should return a simple message or a test data structure.
- Add a new controller by right-clicking on the
Here’s a basic example of a controller in ASP.NET Web API:
using System.Web.Http;
public class TestController : ApiController
{
public IHttpActionResult Get()
{
return Ok("Hello World from ASP.NET Web API");
}
}
- Running Your Project:
- Run your application by pressing F5 or clicking the
Start
button in Visual Studio. - Visual Studio will launch the application using IIS Express and open your default web browser, pointing to the base URL of your project.
- Run your application by pressing F5 or clicking the
- Testing with Postman:
- Open Postman and create a new request.
- Set the request method to
GET
and use the URL that your project is running on, appended with/api/test
(assuming your controller is namedTestController
). - Send the request and you should receive the “Hello World from ASP.NET Web API” response.
Designing the API: Models and Controllers
Defining Data Models
In ASP.NET Web API, models represent the data that your application deals with. These models are usually plain C# classes (POCOs), which are used to transfer data between different layers of your application.
- Creating a Model:
- In the Solution Explorer, right-click on the
Models
folder and selectAdd
->Class
. - Name the class according to the data it represents, such as
Product
orEmployee
.
- In the Solution Explorer, right-click on the
- Defining Model Properties:
- Inside your model class, define properties that represent the data fields. These properties should encapsulate the data attributes your API will accept and return.
Here’s a simple model example:
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
Implementing Controllers for Data Handling
Controllers in ASP.NET Web API are responsible for handling HTTP requests and returning responses. Each controller corresponds to a set of actions that can perform operations on the models.
- Creating a Controller:
- Right-click the
Controllers
folder, selectAdd
->Controller
, and choose theWeb API 2 Controller - Empty
option. - Name the controller appropriately, like
ProductsController
.
- Right-click the
- Writing Action Methods:
- Inside the controller, write action methods to handle different HTTP verbs (GET, POST, PUT, DELETE).
- Each method should perform operations like fetching, creating, updating, or deleting data.
Here’s an example of a controller with basic CRUD operations:
public class ProductsController : ApiController
{
private static List<Product> products = new List<Product>()
{
new Product { Id = 1, Name = "Apple", Price = 1.50M },
new Product { Id = 2, Name = "Banana", Price = 0.60M }
};
public IEnumerable<Product> Get()
{
return products;
}
public IHttpActionResult Get(int id)
{
var product = products.FirstOrDefault(p => p.Id == id);
if (product == null)
{
return NotFound();
}
return Ok(product);
}
public IHttpActionResult Post(Product product)
{
products.Add(product);
return Ok();
}
// PUT and DELETE methods can be added similarly
}
- Testing the Controller:
- Run the application and use Postman to test each of the controller’s action methods.
- Ensure that GET, POST, PUT, and DELETE requests are working as expected and returning appropriate responses.
Implementing Dependency Injection for Scalability
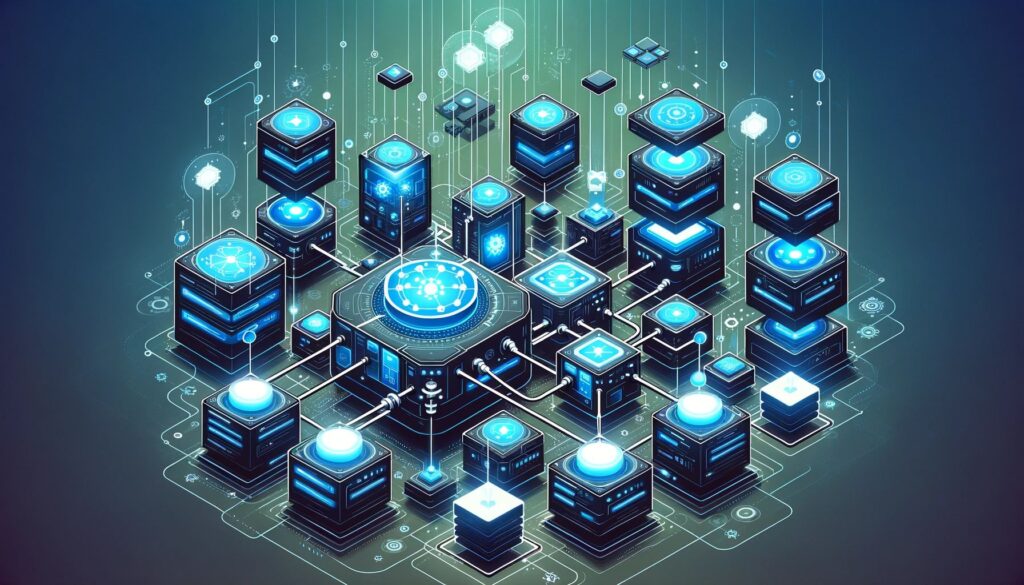
Dependency Injection (DI) in ASP.NET Web API promotes loose coupling and makes your application more modular and testable. By using DI, you can manage your dependencies centrally and provide your controllers with the necessary services easily.
Setting Up Dependency Injection
- Using an Inversion of Control (IoC) Container:
- ASP.NET Web API supports integration with various IoC containers like Unity, Ninject, or Autofac. For this example, let’s use Unity.
- Install the
Unity.AspNet.WebApi
package via NuGet to your project.
- Configuring the IoC Container:
- Create a new static class named
UnityConfig
in yourApp_Start
folder. - In
UnityConfig
, set up your container and register your types.
- Create a new static class named
Example UnityConfig.cs
:
using System.Web.Http;
using Unity;
using YourNamespace.Services; // Replace with your namespace
public static class UnityConfig
{
public static void RegisterComponents()
{
var container = new UnityContainer();
// e.g., container.RegisterType<IProductService, ProductService>();
// Register your types here
GlobalConfiguration.Configuration.DependencyResolver = new UnityDependencyResolver(container);
}
}
- Initializing DI in Application Start:
- In
Global.asax
, call theRegisterComponents
method ofUnityConfig
in theApplication_Start
method.
- In
protected void Application_Start()
{
// Other configurations...
UnityConfig.RegisterComponents();
}
Injecting Dependencies in Controllers
With your IoC container configured, you can now inject dependencies into your controllers through their constructors.
Example:
public class ProductsController : ApiController
{
private readonly IProductService _productService;
public ProductsController(IProductService productService)
{
_productService = productService;
}
// Action methods...
}
Database Integration and Entity Framework Usage
Integrating a database into your ASP.NET Web API project allows you to persist and manage data. The Entity Framework (EF) is a popular ORM (Object-Relational Mapper) that makes database interactions easy.
Configuring a Database Context
- Install Entity Framework:
- Add the Entity Framework to your project via NuGet:
EntityFramework
.
- Add the Entity Framework to your project via NuGet:
- Creating a Database Context Class:
- In your
Models
folder, create a new class for your database context, inheriting fromDbContext
.
- In your
Example MyApiContext.cs
:
using System.Data.Entity;
using YourNamespace.Models; // Replace with your namespace
public class MyApiContext : DbContext
{
public MyApiContext() : base("name=MyApiContext")
{
// Database configuration settings
}
public DbSet<Product> Products { get; set; }
// Add other DbSet properties for other entities
}
- Configuring Connection String:
- In the
Web.config
file, add a connection string for your database.
- In the
<connectionStrings>
<add name="MyApiContext" connectionString="YourConnectionStringHere" providerName="System.Data.SqlClient" />
</connectionStrings>
Implementing CRUD Operations with Entity Framework
With the database context in place, you can now implement CRUD operations in your controllers.
Example CRUD Operations in ProductsController
:
public IHttpActionResult Get()
{
using (var context = new MyApiContext())
{
var products = context.Products.ToList();
return Ok(products);
}
}
// Implement POST, PUT, DELETE similarly
Exception Handling and Global Error Management
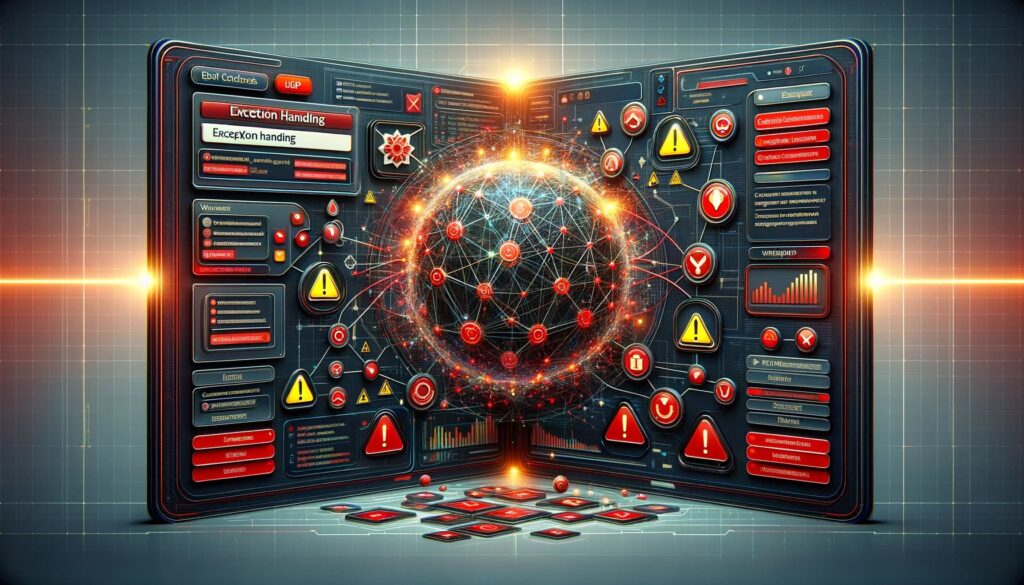
Proper exception handling in an ASP.NET Web API application is crucial for diagnosing issues and providing meaningful feedback to the client. Implementing a global error handling strategy ensures that uncaught exceptions are dealt with systematically.
Implementing Custom Exception Middleware
- Creating a Custom Exception Class:
- Create a class that will capture the necessary details when an exception occurs.
- This class could include properties for the error message, stack trace, and any other relevant information.
Example ErrorDetail.cs
:
public class ErrorDetail
{
public int StatusCode { get; set; }
public string Message { get; set; }
public override string ToString()
{
return Newtonsoft.Json.JsonConvert.SerializeObject(this);
}
}
- Creating Custom Exception Middleware:
- Create a middleware class that will handle exceptions and return the appropriate HTTP response.
Example ExceptionMiddleware.cs
:
public class ExceptionMiddleware
{
private readonly RequestDelegate _next;
private readonly ILogger<ExceptionMiddleware> _logger;
public ExceptionMiddleware(RequestDelegate next, ILogger<ExceptionMiddleware> logger)
{
_next = next;
_logger = logger;
}
public async Task Invoke(HttpContext context)
{
try
{
await _next(context);
}
catch (Exception ex)
{
_logger.LogError($"Something went wrong: {ex}");
await HandleExceptionAsync(context, ex);
}
}
private Task HandleExceptionAsync(HttpContext context, Exception exception)
{
context.Response.ContentType = "application/json";
context.Response.StatusCode = (int)HttpStatusCode.InternalServerError;
return context.Response.WriteAsync(new ErrorDetail
{
StatusCode = context.Response.StatusCode,
Message = "Internal Server Error. Please try again later."
}.ToString());
}
}
- Registering the Middleware:
- In the
Startup.cs
orConfigure
method, add your custom exception middleware to the pipeline.
- In the
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
// Other configurations...
app.UseMiddleware<ExceptionMiddleware>();
}
Securing Your API: Authentication and Authorization
Security is a critical aspect of any API. Implementing authentication and authorization ensures that only legitimate users can access your API.
Basic Authentication Methods
- Using ASP.NET Identity:
- ASP.NET Identity is a membership system that adds login functionality to your application. It supports user interface (UI) login functionality, storing hashed passwords, and user data.
- Implementing JWT Authentication:
- JSON Web Tokens (JWT) are an open standard (RFC 7519) that define a compact and self-contained way for securely transmitting information between parties as a JSON object.
- Configuring JWT in Startup:
- In the
Startup.cs
, configure JWT authentication.
- In the
Example configuration in Startup.cs
:
public void ConfigureServices(IServiceCollection services)
{
// Other configurations...
services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options =>
{
options.TokenValidationParameters = new TokenValidationParameters
{
ValidateIssuerSigningKey = true,
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8
.GetBytes("YourSuperSecretKey")), // Replace with your key
ValidateIssuer = false,
ValidateAudience = false
};
});
}
- Applying the Authentication Middleware:
- Ensure that the authentication middleware is added to the request processing pipeline.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
// Other configurations...
app.UseAuthentication();
}
Advanced API Features: Versioning, Caching, and Documentation
API Versioning
Versioning is essential for maintaining your API over time, especially as you make changes and enhancements that could break existing clients.
- Approaches to Versioning:
- There are various methods for versioning an API, such as URL versioning, query string versioning, and header versioning. Each method has its pros and cons.
- Implementing URL Versioning:
- This approach involves adding the version number in the URI path.
Example route configuration in WebApiConfig.cs
:
config.Routes.MapHttpRoute(
name: "Version1",
routeTemplate: "api/v1/products/{id}",
defaults: new { id = RouteParameter.Optional, controller = "V1Products" }
);
config.Routes.MapHttpRoute(
name: "Version2",
routeTemplate: "api/v2/products/{id}",
defaults: new { id = RouteParameter.Optional, controller = "V2Products" }
);
Caching
Caching improves the performance and scalability of your API by reducing the number of requests that need to go to the server.
- Server-Side Caching:
- Implement caching at the server level to store the responses of your API requests.
- ASP.NET Web API supports several caching mechanisms, including in-memory caching and distributed caching.
Example of in-memory caching in a controller:
public class ProductsController : ApiController
{
private static MemoryCache _cache = new MemoryCache(new MemoryCacheOptions());
public IHttpActionResult Get()
{
if (!_cache.TryGetValue("products", out List<Product> products))
{
products = FetchProductsFromDatabase();
_cache.Set("products", products, TimeSpan.FromMinutes(10)); // Cache for 10 minutes
}
return Ok(products);
}
}
Documentation with OpenAPI/Swagger
Documentation is crucial for API usability. OpenAPI/Swagger provides a user-friendly way to document your API’s functionality.
- Adding Swagger to Your Project:
- Install the
Swashbuckle.AspNetCore
NuGet package. - Configure Swagger in the
Startup.cs
file.
- Install the
Example configuration in Startup.cs
:
public void ConfigureServices(IServiceCollection services)
{
// Other services...
services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v1", new OpenApiInfo { Title = "My API", Version = "v1" });
});
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
// Other configurations...
app.UseSwagger();
app.UseSwaggerUI(c =>
{
c.SwaggerEndpoint("/swagger/v1/swagger.json", "My API V1");
});
}
- Accessing Swagger UI:
- Once configured, you can navigate to
/swagger
on your API’s URL to access the Swagger UI, which provides an interactive documentation of your API endpoints.
- Once configured, you can navigate to
Testing and Deploying Your API

Writing Unit Tests for API Endpoints
Testing is an integral part of the API development process, ensuring that your application behaves as expected.
- Setting Up a Test Project:
- Add a new test project to your solution, typically using xUnit, NUnit, or MSTest.
- Ensure it references your API project.
- Mocking Dependencies:
- Use mocking frameworks like Moq to simulate the behavior of complex objects.
- This is particularly important for testing components in isolation, such as testing controllers without relying on actual database connections.
Example unit test using xUnit and Moq:
public class ProductsControllerTests
{
[Fact]
public void Get_ReturnsAllProducts()
{
// Arrange
var mockRepo = new Mock<IProductRepository>();
mockRepo.Setup(repo => repo.GetAll()).Returns(GetTestProducts());
var controller = new ProductsController(mockRepo.Object);
// Act
var result = controller.Get();
// Assert
var actionResult = Assert.IsType<OkObjectResult>(result);
var returnValue = Assert.IsType<List<Product>>(actionResult.Value);
Assert.Equal(2, returnValue.Count);
}
private List<Product> GetTestProducts()
{
return new List<Product>
{
new Product { Id = 1, Name = "Apple", Price = 1.50M },
new Product { Id = 2, Name = "Banana", Price = 0.60M }
};
}
}
Deploying the API
Deployment involves moving your API from a development environment to a live server where it can be accessed by users.
- Choosing a Hosting Platform:
- Options include traditional web hosting, cloud platforms like Azure, AWS, or containerized solutions using Docker.
- Your choice will depend on factors such as scalability, cost, and infrastructure preferences.
- Configuring the Web Server:
- For IIS hosting, ensure that the IIS server is properly set up and that your application pool is configured to run .NET applications.
- For cloud-based or containerized deployments, follow the specific instructions for your chosen platform.
- Publishing the API:
- In Visual Studio, use the built-in publishing tools to deploy your API to the chosen server or platform.
- You may need to adjust connection strings and other settings in your
Web.config
orappsettings.json
files based on the production environment.
- Monitoring and Maintenance:
- After deployment, monitor your application’s performance and stability.
- Utilize logging and monitoring tools to stay informed of your API’s health and respond quickly to any issues.
Best Practices and Performance Optimization
After deploying your API, it’s essential to focus on best practices and performance optimization to ensure your API remains efficient and easy to maintain.
Ensuring Idempotent Operations
In RESTful services, it’s crucial to ensure that operations like GET, PUT, DELETE are idempotent, meaning multiple identical requests should have the same effect as a single request.
- Implementing Idempotent GET:
- GET requests should not change the state of the server. Make sure that your GET operations are only retrieving data without causing any side effects.
- Idempotent PUT and DELETE:
- PUT should update a resource and always produce the same result regardless of how many times it’s executed with the same data.
- DELETE should remove a resource and subsequent DELETE calls should return a status indicating the resource no longer exists (usually 404 Not Found).
Example of Idempotent PUT in a controller:
[HttpPut("{id}")]
public IActionResult Put(int id, [FromBody] Product product)
{
if (!ProductExists(id))
{
return NotFound();
}
UpdateProduct(id, product); // Implement this method to update the product
return NoContent(); // No content indicates success but no data returned
}
private bool ProductExists(int id)
{
// Check if product exists in the database
}
Optimizing Data Transfer with DTOs
Data Transfer Objects (DTOs) are simple objects that should be used to transfer data between processes. Using DTOs helps to optimize data transfer by including only necessary data.
- Creating DTOs:
- Define DTOs that contain only the data required for specific operations.
- This reduces the amount of data transferred, especially important for high-latency networks.
Example DTO:
public class ProductDto
{
public int Id { get; set; }
public string Name { get; set; }
// Exclude unnecessary properties like detailed descriptions, etc.
}
- Mapping Entities to DTOs:
- Use tools like AutoMapper to map your domain entities to DTOs.
- This simplifies the code and automates the mapping process.
Applying Data Validation Techniques
Proper data validation is critical to ensure that only valid data is processed by your API.
- Using Data Annotations:
- Apply data annotations to your models or DTOs to enforce validation rules.
Example with Data Annotations:
public class ProductDto
{
[Required]
public int Id { get; set; }
[Required]
[StringLength(100)]
public string Name { get; set; }
}
- Implementing Custom Validation Logic:
- For more complex validation scenarios, implement custom validation logic within your API endpoints.
Conclusion
As we conclude our guide on creating RESTful services with ASP.NET Web API, it’s important to reflect on the journey we’ve taken. From the initial understanding of REST principles and the capabilities of ASP.NET Web API, through the setup of the development environment and the implementation of basic and advanced concepts, we’ve covered a significant ground. This journey has included exploring dependency injection, database integration, exception handling, security, performance optimization, and the crucial steps of testing and deployment.
The key takeaway from this guide is the importance of a solid foundation in both theory and practice. By understanding the underlying principles and then applying them through hands-on implementation, you’ve equipped yourself with the skills necessary to build robust and scalable web services.
Remember, the field of web development is dynamic and ever-evolving. Continuous learning, staying updated with the latest trends, and adapting to new technologies and methodologies are crucial for your growth and success as a developer.
Additional Resources
To further advance your skills and knowledge in ASP.NET Web API and RESTful services, consider the following resources:
- Official Microsoft Documentation: The ASP.NET Web API documentation on Microsoft’s official website is a rich source of information, offering detailed guides and tutorials.
- Online Courses and Tutorials: Platforms like Udemy, Pluralsight, and Coursera have a variety of courses ranging from beginner to advanced levels, providing in-depth knowledge and practical examples.
- Community and Forums: Participating in discussions on platforms like Stack Overflow, Reddit, and GitHub can provide insights into real-world problems and solutions, as well as keeping you informed about the latest trends and best practices.
- Books: Reading comprehensive books such as “Pro ASP.NET Web API” can provide deeper insights and cover aspects that might not be available in online tutorials and documentation.