In the realm of web development, JavaScript stands as a cornerstone technology, instrumental in shaping the interactive experiences that users have come to expect from modern websites. Its role extends far beyond mere aesthetic enhancements; JavaScript is crucial for creating responsive, dynamic user interfaces that respond to user inputs in real-time, offering a seamless and engaging experience.
The Evolution of JavaScript in Web Development
JavaScript’s journey began in the mid-1990s, originally designed to add simple interactive elements to web pages. Over the years, it has evolved into a powerful, versatile language, capable of handling complex tasks including but not limited to:
- Animations and page transitions.
- Form validation and user feedback.
- Real-time updates without reloading the page (thanks to AJAX).
- Front-end development with advanced frameworks and libraries.
Why JavaScript for Interactive Web Applications?
The significance of JavaScript in building interactive web applications can be distilled into several key aspects:
- Cross-Platform Compatibility: JavaScript runs in every modern web browser, providing a consistent experience across different devices and platforms.
- Event-Driven Programming: JavaScript excels in responding to user actions like clicks, form submissions, and mouse movements, enabling developers to create highly reactive applications.
- Versatility and Flexibility: From front-end development with libraries like React and Angular to server-side with Node.js, JavaScript’s versatility is unparalleled.
- Community and Ecosystem: With one of the largest developer communities, JavaScript boasts an extensive ecosystem of libraries, tools, and frameworks, continuously evolving with new innovations.
Interactivity: A Key to Enhanced User Experience
The heart of JavaScript’s prowess lies in its ability to make web pages “alive”. This interactivity is a critical component in enhancing the user experience. Here’s how JavaScript achieves this:
- Dynamic Content Loading: JavaScript can update the content of a page in real-time without needing to reload the entire page. This is pivotal for applications like social media feeds, live sports scores, and stock market tickers.
- Responsive Feedback: Interactive forms with instant validation, search suggestions, and interactive galleries are all made possible with JavaScript, significantly improving user engagement.
- Animation and Effects: Subtle animations, smooth page transitions, and visual effects can be implemented, making the user interface more intuitive and enjoyable.
The Table of JavaScript Interactivity
To understand the scope of JavaScript’s capabilities, here’s a table summarizing its role in various interactive elements:
Interactive Element | JavaScript’s Role |
---|---|
Form Validation | Real-time input checking and feedback |
Dynamic Content | Updating content without reloading the page |
Animations | Smooth transitions and visual effects |
User Alerts | Custom pop-up messages and notifications |
Interactive Maps | Handling user inputs and displaying dynamic data |
Real-Time Updates | Live content updates, such as in chat apps or news feeds |
The Basics of JavaScript for Beginners
Embarking on the journey of learning JavaScript can be both exciting and daunting. This section aims to lay down the fundamental building blocks essential for any aspiring web developer. We’ll start with the basic syntax and constructs of JavaScript, and then introduce a few simple projects to help solidify these concepts.
Understanding JavaScript Syntax
The syntax of JavaScript is the set of rules that define how the language is written. Let’s look at some of the basic elements:
- Variables: Variables are containers for storing data values. In JavaScript, you can declare variables using
let
,const
, orvar
. For example:
let message = "Hello, World!";
console.log(message);
This code declares a variable named message
, assigns a string to it, and then prints it to the console.
- Functions: Functions are blocks of code designed to perform a particular task. Here’s a simple function in JavaScript:
function greet(name) {
return "Hello, " + name;
}
console.log(greet("Alice"));
This function, greet
, takes a name as an argument and returns a greeting message.
- Control Structures: These include if-else statements and loops, which control the flow of the program. For example, a simple loop to print numbers from 1 to 5:
for (let i = 1; i <= 5; i++) {
console.log(i);
}
- Events: JavaScript often interacts with HTML elements through events like clicks or key presses. For instance:
<button id="clickMe">Click me!</button>
<script>
document.getElementById("clickMe").onclick = function() {
alert("Button clicked!");
};
</script>
This code attaches an onclick
event to a button, triggering an alert when clicked.
Simple JavaScript Projects for Practice
- Building a To-Do List: Create a simple to-do list where users can add items and mark them as done. This project will teach you about DOM manipulation and event handling.
- Simple Calculator: Develop a basic calculator that performs addition, subtraction, multiplication, and division. This helps in understanding functions and user input handling.
- Interactive Quiz: Design a quiz with multiple-choice questions. This will involve more complex logic and may introduce you to arrays and objects.
Tips for JavaScript Beginners
- Practice Regularly: Like learning any language, regular practice is key. Try coding small projects or solving problems daily.
- Read Documentation: The Mozilla Developer Network (MDN) Web Docs are a fantastic resource for learning JavaScript.
- Join Communities: Engage with communities on platforms like Stack Overflow, GitHub, or Reddit. Learning from others’ experiences can be incredibly valuable.
- Keep It Simple: Start with small, manageable projects and gradually increase complexity.
Advanced JavaScript Techniques for Dynamic Content
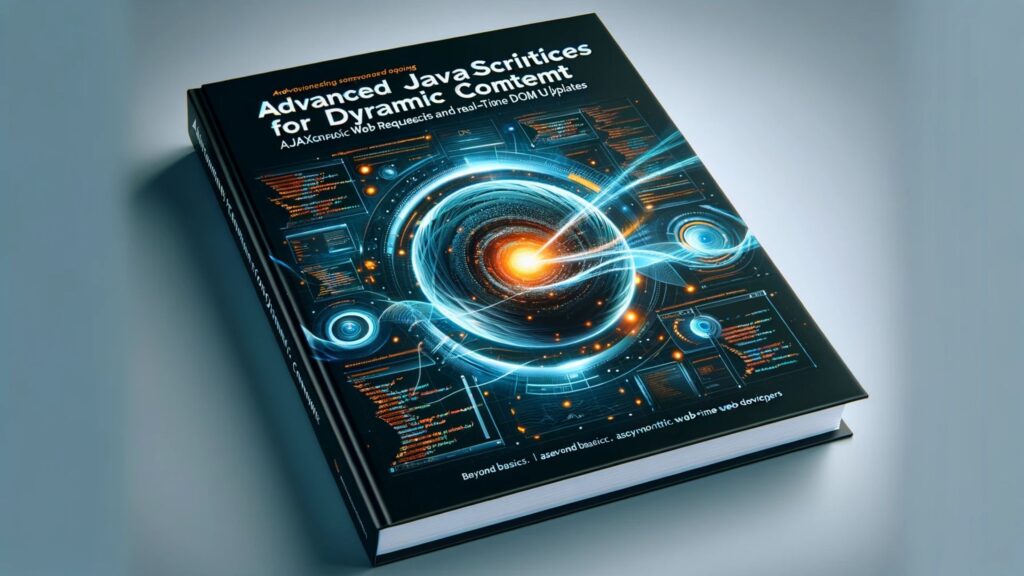
Moving beyond the basics, let’s explore some advanced techniques in JavaScript that are pivotal for creating more dynamic and interactive web content. These include AJAX for asynchronous web requests and manipulating the Document Object Model (DOM) to update web pages in real time.
AJAX: Asynchronous JavaScript and XML
AJAX stands for Asynchronous JavaScript and XML. It’s a set of web development techniques that enables web applications to send and retrieve data from a server asynchronously, without interfering with the display and behavior of the existing page. Here’s a basic example of how AJAX is used to fetch data from a server:
function fetchData() {
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
console.log(xhr.responseText);
}
};
xhr.open('GET', 'https://api.example.com/data', true);
xhr.send();
}
fetchData();
In this snippet, fetchData
function creates an XMLHttpRequest object, sets up a function to run when the server response is ready, and then sends an HTTP GET request to the server.
Manipulating the DOM for Real-Time Content Updates
The DOM is an essential concept in web development, providing a structured representation of the document (like HTML) and defining ways to access and manipulate it. JavaScript can dynamically update the content, structure, and style of a document. Here’s a simple example:
document.getElementById("demo").innerHTML = "Welcome to JavaScript!";
In this line, JavaScript is used to find an HTML element with the id demo
and change its content to “Welcome to JavaScript!”.
Advanced Event Handling
JavaScript’s ability to handle user-generated events can be used to create highly responsive and interactive user interfaces. Here’s an example of adding an event listener to a button:
<button id="myButton">Click Me!</button>
<script>
document.getElementById("myButton").addEventListener("click", function() {
alert("Button was clicked!");
});
</script>
This script makes the web page react to a click event on a button by displaying an alert.
Using Frameworks and Libraries
While pure JavaScript is powerful, frameworks and libraries like React, Angular, and Vue.js provide additional tools and structures to build more complex applications efficiently. They come with their own ways of handling state, rendering content, and managing user interactions.
For instance, a simple component in React might look like this:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
export default Counter;
In this React component, a simple counter is implemented using the useState
hook, showcasing how state can be managed in a functional component.
Frameworks and Libraries to Boost JavaScript Development
The landscape of JavaScript is enriched by a multitude of frameworks and libraries, each designed to streamline various aspects of web development. These tools not only accelerate the development process but also introduce new functionalities and best practices. Let’s delve into some of the most popular frameworks and libraries and see how they are applied in JavaScript development.
React: Building User Interfaces
React, developed by Facebook, is a declarative, efficient, and flexible JavaScript library for building user interfaces. It lets you compose complex UIs from small, isolated pieces of code called “components”. Here’s a glimpse into a simple React component:
import React from 'react';
function HelloComponent() {
return <h1>Hello, world!</h1>;
}
export default HelloComponent;
This code defines a React component, HelloComponent
, which simply renders “Hello, world!” in an <h1>
tag. React’s component-based architecture makes it easy to manage and reuse code.
Angular: A Platform for Building Mobile and Desktop Web Applications
Angular, developed by Google, is a platform and framework for building single-page client applications using HTML and TypeScript (a superset of JavaScript). Angular implements core and optional functionality as a set of TypeScript libraries that you import into your apps. A basic Angular component might look like this:
import { Component } from '@angular/core';
@Component({
selector: 'app-hello',
template: `<h1>Hello, Angular!</h1>`
})
export class HelloComponent {}
This snippet shows a basic Angular component with an HTML template displaying a greeting message.
Vue.js: The Progressive JavaScript Framework
Vue.js is a progressive framework for building user interfaces. Unlike other monolithic frameworks, Vue is designed from the ground up to be incrementally adoptable. A simple Vue instance looks like this:
var app = new Vue({
el: '#app',
data: {
message: 'Hello Vue!'
}
})
In this example, Vue is used to bind the message
data to a DOM element with the id app
.
Libraries like jQuery: Simplifying JavaScript
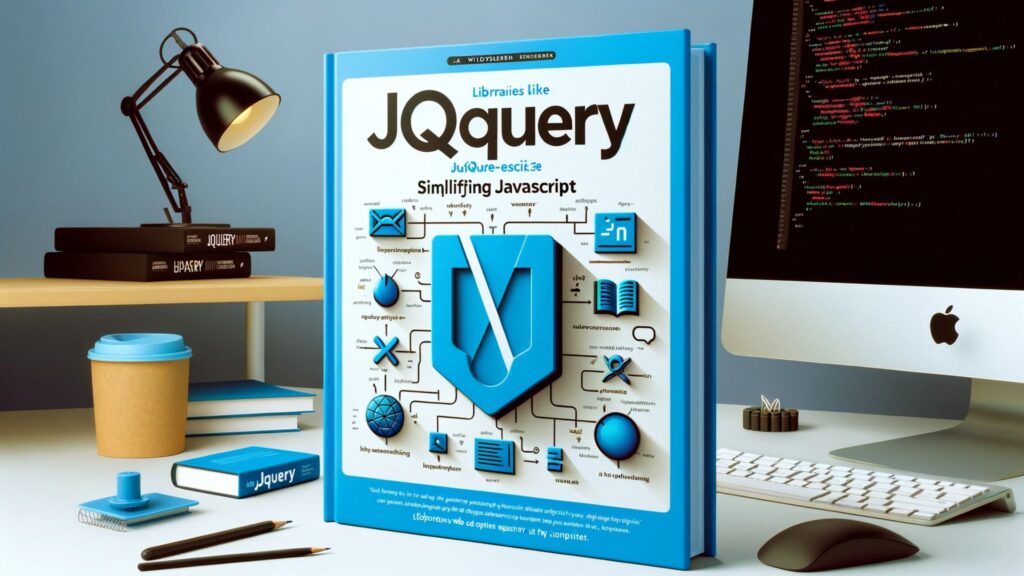
jQuery is a fast, small, and feature-rich JavaScript library. It makes things like HTML document traversal and manipulation, event handling, and animation much simpler with an easy-to-use API that works across a multitude of browsers. Here’s an example of using jQuery:
$(document).ready(function(){
$("#hideButton").click(function(){
$("p").hide();
});
});
In this jQuery example, when a button with the id hideButton
is clicked, all paragraph elements on the page are hidden.
Interactive Design Patterns in JavaScript
Interactive design patterns are strategies for designing interactive and responsive web applications. These patterns address common user interface problems and offer solutions to enhance user experience. Let’s explore some common interactive design patterns in JavaScript and their implementation.
The Model-View-Controller (MVC) Pattern
The MVC pattern is a software design pattern that separates an application into three interconnected components: the model (data), the view (user interface), and the controller (business logic). This separation helps in managing complex applications, as it encourages modular coding and provides a structured approach to design.
Example in JavaScript: Creating a simple MVC setup where the model updates the view through the controller.
// Model
const model = {
data: "Hello, MVC!"
};
// View
function updateView(message) {
document.getElementById("mvcOutput").innerText = message;
}
// Controller
function controller() {
updateView(model.data);
}
controller();
In this basic example, the model holds the data, the view updates the HTML, and the controller connects the two.
The Observer Pattern
The Observer pattern is a design pattern where an object (known as a subject) maintains a list of its dependents (observers) and notifies them automatically of any state changes. This pattern is particularly useful in event handling systems.
Example in JavaScript: Implementing a simple Observer pattern.
class Subject {
constructor() {
this.observers = [];
}
subscribe(observer) {
this.observers.push(observer);
}
notify(data) {
this.observers.forEach(observer => observer.update(data));
}
}
class Observer {
update(data) {
console.log("Received data:", data);
}
}
const subject = new Subject();
const observer = new Observer();
subject.subscribe(observer);
subject.notify("Notification from Subject");
Here, Subject
maintains a list of observers and notifies them, while Observer
receives and processes the data.
Single Page Application (SPA) Pattern
The SPA pattern involves rendering a single HTML page and dynamically updating it in response to user actions. This pattern avoids the need for reloading the entire page, leading to a smoother user experience.
Example with JavaScript: Using AJAX to fetch and display data without reloading the page.
function fetchData() {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
document.getElementById("spaContent").innerHTML = JSON.stringify(data);
})
.catch(error => console.error('Error:', error));
}
document.getElementById("loadDataButton").addEventListener("click", fetchData);
In this snippet, data is fetched and displayed in an element with the id spaContent
when a button is clicked.
Factory Pattern
The Factory pattern is a creational pattern that provides an interface for creating objects in a superclass, but allows subclasses to alter the type of objects that will be created.
Example in JavaScript: Implementing a basic Factory pattern.
class ProductFactory {
createProduct(type) {
if (type === "ProductA") {
return new ProductA();
} else if (type === "ProductB") {
return new ProductB();
}
}
}
class ProductA {
constructor() {
console.log("ProductA created");
}
}
class ProductB {
constructor() {
console.log("ProductB created");
}
}
const factory = new ProductFactory();
const productA = factory.createProduct("ProductA");
const productB = factory.createProduct("ProductB");
Here, ProductFactory
determines which product class to instantiate based on the provided type.
Optimizing Performance for JavaScript Web Applications
Performance optimization is critical in web development, as it directly impacts user experience and satisfaction. Efficient JavaScript code can lead to faster page loads, smoother interactions, and overall better performance. Let’s explore some best practices and tools for optimizing JavaScript web applications.
Best Practices for Efficient JavaScript Code
- Minimize DOM Manipulation: Excessive DOM manipulation is costly in terms of performance. Minimizing these operations can significantly enhance performance. For example, batch your DOM updates:
const fragment = document.createDocumentFragment();
for (let i = 0; i < 100; i++) {
const element = document.createElement('p');
element.textContent = `Item ${i}`;
fragment.appendChild(element);
}
document.body.appendChild(fragment);
This code uses a Document Fragment to batch DOM updates, reducing the number of reflow and repaint operations.
- Debounce and Throttle Event Handlers: These techniques are essential for optimizing events that fire frequently, like window resizing or scrolling.
- Debounce: Ensures that a function is only executed after a certain amount of time has passed since it was last called.
function debounce(func, wait) {
let timeout;
return function() {
const context = this, args = arguments;
clearTimeout(timeout);
timeout = setTimeout(() => func.apply(context, args), wait);
};
}
window.addEventListener('resize', debounce(function() {
console.log('Resize event handler called');
}, 250));
- Throttle: Ensures that a function is only called at most once in a specified time period.
function throttle(func, limit) {
let lastFunc;
let lastRan;
return function() {
const context = this;
const args = arguments;
if (!lastRan) {
func.apply(context, args);
lastRan = Date.now();
} else {
clearTimeout(lastFunc);
lastFunc = setTimeout(function() {
if ((Date.now() - lastRan) >= limit) {
func.apply(context, args);
lastRan = Date.now();
}
}, limit - (Date.now() - lastRan));
}
}
}
window.addEventListener('scroll', throttle(function() {
console.log('Scroll event handler called');
}, 1000));
- Lazy Loading of Images and Resources: Loading resources only when they are needed can significantly reduce initial load time and save bandwidth.
document.addEventListener("DOMContentLoaded", function() {
const lazyImages = [].slice.call(document.querySelectorAll("img.lazy"));
if ("IntersectionObserver" in window) {
let lazyImageObserver = new IntersectionObserver(function(entries, observer) {
entries.forEach(function(entry) {
if (entry.isIntersecting) {
let lazyImage = entry.target;
lazyImage.src = lazyImage.dataset.src;
lazyImage.classList.remove("lazy");
lazyImageObserver.unobserve(lazyImage);
}
});
});
lazyImages.forEach(function(lazyImage) {
lazyImageObserver.observe(lazyImage);
});
}
});
This snippet demonstrates the use of IntersectionObserver
to implement lazy loading of images.
Tools for Performance Testing and Debugging
- Google Chrome Developer Tools: Chrome DevTools provides a suite of web developer tools built directly into the Google Chrome browser. It has features for inspecting elements, debugging JavaScript, and analyzing performance.
- Lighthouse: An open-source, automated tool for improving the quality of web pages. It can audit for performance, accessibility, progressive web apps, and more.
- WebPageTest: A tool that provides detailed performance insights and allows you to test your site from different locations and on various devices.
Future Trends in JavaScript and Web Application Development
Staying ahead of the curve in web development involves understanding and adapting to emerging trends. The field of JavaScript and web application development is constantly evolving, with new frameworks, tools, and practices emerging regularly. Let’s explore some of the trends that are shaping the future of JavaScript web development.
1. The Rise of Frameworks like React, Vue, and Angular
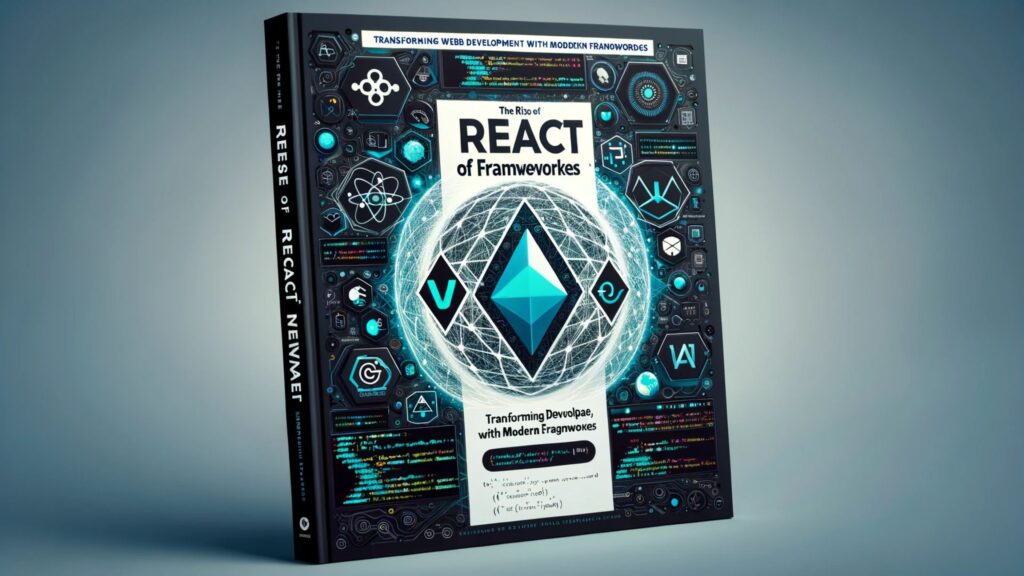
Modern JavaScript frameworks like React, Vue.js, and Angular are becoming increasingly popular for building scalable and maintainable web applications. They offer robust ecosystems, component-based architectures, and reactive data binding, making them ideal for developing complex web apps.
Example with React Hooks: React introduced Hooks in version 16.8, allowing you to use state and other React features without writing a class. Here’s a simple counter example using the useState
hook:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
export default Counter;
2. Server-Side Rendering (SSR) and Static Site Generators
Server-Side Rendering (SSR) and Static Site Generators are becoming more prevalent for their performance benefits and improved SEO. Frameworks like Next.js for React and Nuxt.js for Vue.js are popular choices.
Example with Next.js: Next.js provides a simple page-based routing system. Here’s how you can create a basic SSR page in Next.js:
// pages/index.js
function HomePage() {
return <div>Welcome to the Next.js!</div>
}
export default HomePage;
3. Progressive Web Apps (PWAs)
PWAs use modern web capabilities to deliver app-like experiences to users. They are installable, work offline, and are highly responsive.
Example PWA feature in JavaScript: Service Workers are a core technology behind PWAs. Here’s a basic service worker registration:
if ('serviceWorker' in navigator) {
navigator.serviceWorker.register('/service-worker.js').then(function(registration) {
console.log('ServiceWorker registration successful with scope:', registration.scope);
}, function(err) {
console.log('ServiceWorker registration failed:', err);
});
}
4. Web Components
Web Components are a suite of different technologies allowing you to create reusable custom elements — with their functionality encapsulated away from the rest of your code — and use them in your web apps.
Example with Custom Elements:
class MyElement extends HTMLElement {
connectedCallback() {
this.innerHTML = `<p>Hello, Web Components!</p>`;
}
}
customElements.define('my-element', MyElement);
5. TypeScript Adoption
TypeScript, a superset of JavaScript, adds static types to the language. It’s gaining popularity for its ability to catch errors early and provide a more robust coding experience.
TypeScript Example:
function greet(name: string): string {
return `Hello, ${name}!`;
}
console.log(greet("Alice"));
6. The Growing Importance of Performance and Accessibility
Web performance and accessibility are becoming critical factors in web development. Developers are focusing more on optimizing code and following accessibility guidelines to create inclusive and fast-loading websites.
Conclusion
As we reach the culmination of our exploration into JavaScript for interactive web applications, it is evident that this versatile language is the backbone of modern web development. The journey through JavaScript’s basics to its advanced implementations, coupled with the utilization of various frameworks and libraries, paints a vivid picture of its capabilities. JavaScript’s evolution from a simple scripting language to a powerful tool for creating sophisticated web applications underscores its significance in the digital landscape. The adaptability, community support, and continuous advancements in JavaScript ensure that it remains relevant and indispensable for developers looking to create engaging, efficient, and dynamic web experiences.
Looking towards the future, the landscape of web development is one of constant evolution, driven by technological advancements and emerging trends. As JavaScript continues to grow and adapt, so too must the skills and knowledge of those who wield it. Embracing the ever-changing nature of web development, developers are encouraged to stay curious, experiment, and continuously learn. Whether it’s optimizing performance, adhering to best practices, or exploring new frameworks and patterns, the possibilities with JavaScript are boundless. In this ever-evolving journey, the most exciting aspect is not just what JavaScript is today, but what it has the potential to become tomorrow, shaping the future of interactive web applications.