Visual Basic for Applications (VBA) is a powerful programming language integrated into Microsoft Excel. It enables users to automate various tasks, ranging from simple data manipulations to complex analytical processes. Understanding the basics of VBA and recognizing the importance of automating tasks in Excel can significantly enhance your productivity and data handling capabilities.
The Basics of Visual Basic
VBA, a subset of the popular Visual Basic programming language, is tailored for the Office suite. It’s designed to be accessible for beginners, yet powerful enough for advanced users. Here’s what makes it stand out:
- Integrated Development Environment (IDE): VBA comes with a built-in editor in Excel, providing a familiar and accessible workspace.
- Event-Driven Programming: VBA reacts to specific user actions or Excel events, allowing automation to be context-sensitive.
- Access to Excel Objects: VBA can manipulate almost every aspect of Excel, including cells, ranges, worksheets, and even the Excel interface itself.
Why Automate Tasks in Excel?
Automation in Excel isn’t just about saving time; it’s about enhancing accuracy, consistency, and the capability to handle complex tasks. Key benefits include:
- Efficiency: Automate repetitive tasks like data entry, formatting, and calculations.
- Accuracy: Minimize human errors in complex calculations and data manipulations.
- Scalability: Handle large datasets and complex operations more effectively.
- Customization: Tailor Excel’s functionality to suit specific needs that aren’t covered by standard features.
Excel and VBA: A Powerful Combination
Combining Excel’s data handling capabilities with VBA’s automation potential creates a powerful tool for any data analyst, accountant, or Excel user. From automating mundane tasks like formatting to developing complex algorithms for data analysis, VBA opens up a world of possibilities.
Setting Up Your Environment for VBA
Before diving into the world of automation with VBA, it’s crucial to set up your Excel environment correctly. This setup is a one-time process and will pave the way for all your future VBA endeavors.
Enabling Developer Tab in Excel
The Developer tab is where you access the VBA Editor and other developer tools in Excel. It’s not visible by default, so here’s how you can enable it:
- Open Excel and click on the
File
tab. - Choose
Options
to open the Excel Options dialog box. - In the Excel Options dialog box, click on
Customize Ribbon
. - In the right pane, check the box next to
Developer
and clickOK
.
The Developer tab will now appear in the Excel ribbon, providing easy access to the VBA Editor and other advanced tools.
Navigating the VBA Editor
To open the VBA Editor, you can either press Alt + F11
or use the Visual Basic
button on the Developer tab. Once inside, you’ll see a few key areas:
- Project Explorer: Displays your open workbooks and their components (worksheets, modules, etc.).
- Properties Window: Shows properties of the selected item in the Project Explorer.
- Code Window: Where you write and view VBA code.
Writing Your First VBA Script
Let’s create a simple macro that demonstrates the power of VBA. This script will automatically insert the current date into a selected cell:
- In the VBA Editor, right-click on your workbook in the Project Explorer.
- Choose
Insert
>Module
. This creates a new module where you can write your code. - In the new module window, enter the following VBA code:
Sub InsertCurrentDate()
ActiveCell.Value = Date
End Sub
- Close the VBA Editor and return to Excel.
- To run your macro, go to the Developer tab, click
Macros
, selectInsertCurrentDate
, and then clickRun
.
This simple example introduces the basic structure of a VBA subroutine (Sub) and demonstrates how you can manipulate cell values with VBA.
Fundamentals of VBA Programming
Venturing into the world of VBA programming begins with understanding its core components. Here, we will explore variables, data types, and basic procedures, which are the building blocks of any VBA program.
Variables and Data Types
In VBA, a variable is a storage location in your computer’s memory that can hold data. Each variable has a specific data type, which determines the kind of data it can hold. Here are some common data types in VBA:
- Integer: For whole numbers.
- String: For text.
- Boolean: For True/False values.
- Date: For dates.
Here’s an example of how to declare and use variables in VBA:
Sub VariableExample()
Dim myNumber As Integer
Dim myText As String
Dim myDate As Date
Dim isCompleted As Boolean
myNumber = 10
myText = "Hello, Excel!"
myDate = Date
isCompleted = True
End Sub
Writing Your First VBA Script with Variables
Let’s expand on our earlier script by adding variables. This time, we’ll create a macro that inserts a personalized greeting into a cell, using a String variable:
Sub PersonalizedGreeting()
Dim userName As String
userName = "Excel User" ' You can change this to any name
ActiveCell.Value = "Hello, " & userName & "!"
End Sub
This script introduces the concept of concatenating strings using the &
operator, a fundamental concept in programming.
Procedures: Subs and Functions
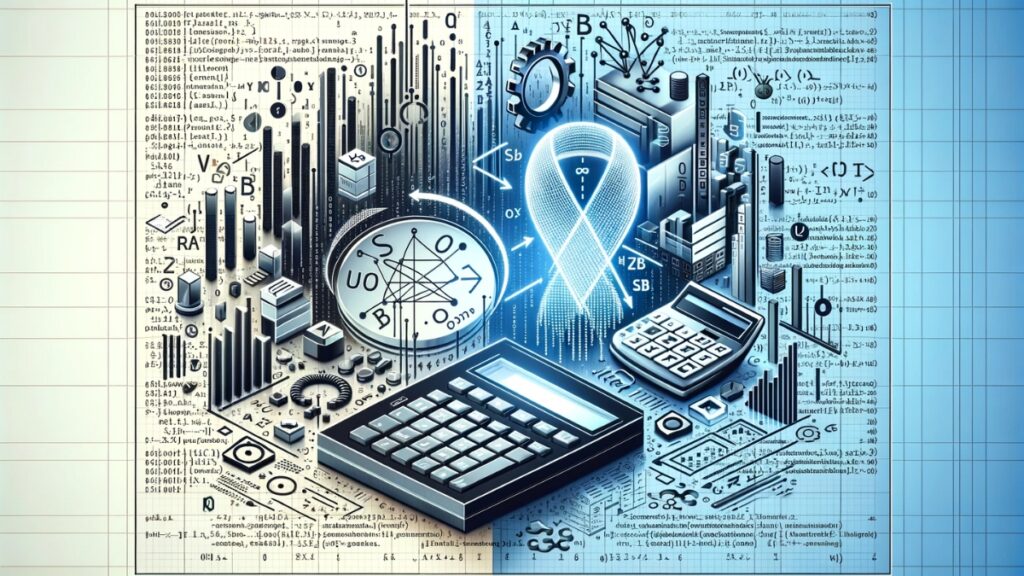
In VBA, there are two main types of procedures: Subroutines (Sub) and Functions. Subroutines perform actions, while Functions return values and can be used in cell formulas.
Here’s an example of a simple Function in VBA:
Function AddNumbers(number1 As Integer, number2 As Integer) As Integer
AddNumbers = number1 + number2
End Function
You can use this function in Excel like any other formula: =AddNumbers(5, 3)
, and it would return 8
.
Automating Repetitive Tasks
One of the primary uses of VBA in Excel is to automate repetitive tasks. This not only saves time but also reduces the risk of human errors. We’ll explore how to automate a common task and provide a step-by-step guide.
Identifying Tasks Suitable for Automation
Tasks ideal for automation usually involve repetitive actions, such as:
- Formatting cells or rows based on specific criteria.
- Inserting or modifying data across multiple worksheets.
- Generating regular reports from a dataset.
Automating a Sample Task: Formatting Cells
Let’s say you often need to highlight cells in a column that contain values greater than a specific threshold. Doing this manually can be time-consuming, especially with large datasets. We can automate this with VBA.
Here’s the VBA script to do it:
Sub HighlightHighValues()
Dim cell As Range
Dim threshold As Integer
threshold = 50 ' Set your threshold value here
For Each cell In Selection
If cell.Value > threshold Then
cell.Interior.Color = RGB(255, 0, 0) ' Highlight in red
Else
cell.Interior.ColorIndex = 0 ' No fill
End If
Next cell
End Sub
To use this script:
- Select the range of cells you want to format.
- Run the
HighlightHighValues
macro.
This script iterates through each cell in the selected range, checks if the cell’s value is greater than the threshold, and changes the cell’s background color if it is.
Enhancing the Script: User Input
Let’s enhance our script by allowing the user to input the threshold value. We’ll use the InputBox
function to get user input:
Sub HighlightHighValuesWithInput()
Dim cell As Range
Dim threshold As Integer
threshold = InputBox("Enter the threshold value", "Threshold Value", 50)
For Each cell In Selection
If cell.Value > threshold Then
cell.Interior.Color = RGB(255, 0, 0) ' Highlight in red
Else
cell.Interior.ColorIndex = 0 ' No fill
End If
Next cell
End Sub
Now, when you run the macro, a dialog box will prompt you to enter the threshold value, making the script more dynamic and user-friendly.
Working with Excel Objects in VBA
Excel VBA allows you to manipulate different objects like ranges, cells, worksheets, and workbooks. Understanding how to interact with these objects is key to effective automation.
Understanding Range, Cells, Worksheets, and Workbooks
In VBA, everything in Excel is considered an object, which includes cells, ranges, worksheets, and entire workbooks. Here’s a quick overview:
- Range: A cell, a row, a column, or a selection of cells.
- Cells: Individual cells in a worksheet.
- Worksheets: The sheets within a workbook.
- Workbooks: The entire Excel file.
Manipulating Data and Formatting with VBA
Let’s create a script that demonstrates how to manipulate these objects. This script will automatically format a range of cells and write a summary in a new worksheet.
Sub FormatAndSummarizeData()
Dim sourceSheet As Worksheet
Dim summarySheet As Worksheet
Dim dataRange As Range
Dim cell As Range
Dim total As Double
Set sourceSheet = ThisWorkbook.Sheets("Data") ' Change to your data sheet name
Set dataRange = sourceSheet.Range("A1:A10") ' Change to your data range
Set summarySheet = ThisWorkbook.Sheets.Add(After:=ThisWorkbook.Sheets(ThisWorkbook.Sheets.Count))
summarySheet.Name = "Summary"
total = 0
For Each cell In dataRange
total = total + cell.Value
cell.Interior.Color = RGB(255, 255, 0) ' Highlight cells in yellow
Next cell
summarySheet.Range("A1").Value = "Total"
summarySheet.Range("B1").Value = total
End Sub
This script performs the following actions:
- Defines the data range on a specified sheet.
- Creates a new worksheet for the summary.
- Iterates through the range, calculates the total, and applies formatting.
- Writes the total value in the new summary sheet.
Advanced Data Manipulation
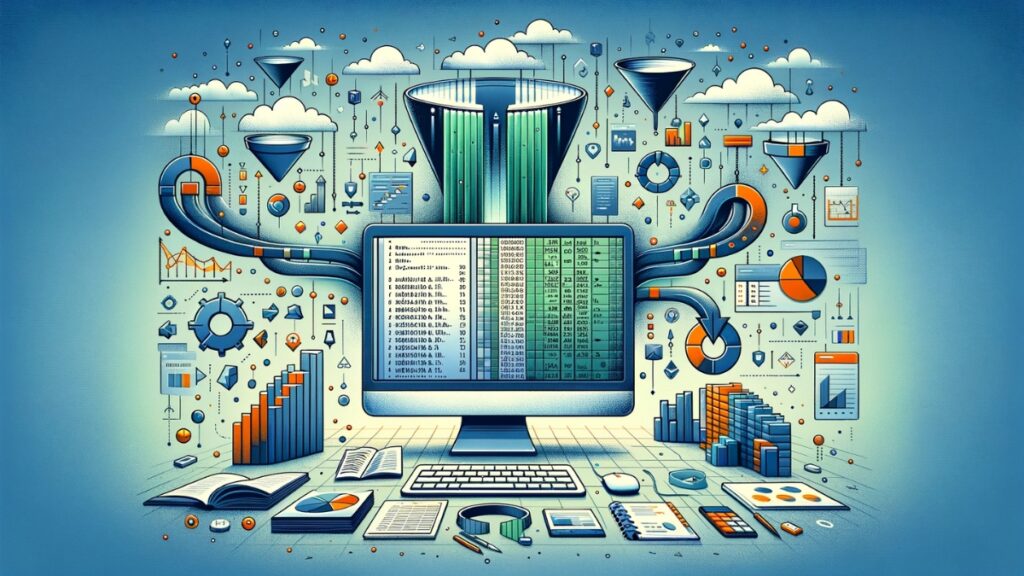
You can also perform more complex data manipulations using VBA. For example, filtering data based on certain criteria or sorting data. Here’s a simple example of sorting data:
Sub SortData()
Dim dataRange As Range
Set dataRange = ThisWorkbook.Sheets("Data").Range("A1:B10") ' Adjust range as needed
dataRange.Sort Key1:=dataRange.Cells(1, 1), Order1:=xlAscending, Header:=xlYes
End Sub
This script sorts the data in the range A1:B10 of the “Data” sheet in ascending order based on the values in the first column.
Error Handling and Debugging in VBA
Writing error-free code on the first try is rare, even for experienced programmers. That’s why understanding error handling and debugging in VBA is essential. It helps you identify and fix issues in your code, ensuring it runs as intended.
Common Errors in Excel VBA
Errors in VBA can arise from various sources, such as syntax mistakes, incorrect object references, or runtime issues. Some common errors include:
- Compile errors: Caused by syntax issues, like missing end statements or misspelled keywords.
- Runtime errors: Occur when the code is syntactically correct but encounters problems during execution, like dividing by zero or referencing a non-existent worksheet.
- Logical errors: These are the hardest to detect because the code runs without crashing, but it doesn’t produce the expected result.
Techniques for Effective Debugging
Effective debugging involves systematically checking your code to find where things go wrong. Here are some techniques:
- Using the Immediate Window: Useful for printing values of variables at different points in your code.
- Breakpoints: Pause the execution of your code so you can examine the current values of variables.
- Step Through Execution: Run your code line by line to observe how different parts are executing.
Implementing Basic Error Handling
Let’s enhance the SortData
subroutine from the previous section with basic error handling:
Sub SortDataWithErrorHandling()
On Error GoTo ErrorHandler
Dim dataRange As Range
Set dataRange = ThisWorkbook.Sheets("Data").Range("A1:B10")
dataRange.Sort Key1:=dataRange.Cells(1, 1), Order1:=xlAscending, Header:=xlYes
Exit Sub
ErrorHandler:
MsgBox "An error occurred: " & Err.Description
End Sub
In this script, On Error GoTo ErrorHandler
tells VBA to jump to the ErrorHandler
label if an error occurs. The error handler displays a message box with the error description.
Debugging with Breakpoints and the Immediate Window
Now, let’s use breakpoints and the Immediate Window to debug a script. Suppose we have a script that sometimes doesn’t produce the correct output:
Sub CalculateSum()
Dim total As Integer
Dim i As Integer
For i = 1 To 10
total = total + i
Next i
Debug.Print "Total is: " & total ' Print to Immediate Window
End Sub
You can set a breakpoint on the Debug.Print
line, run the script, and when it pauses, check the value of total
in the Immediate Window. This simple technique helps identify issues with the logic in your code.
Advanced VBA Techniques for Data Analysis
VBA can be a powerful tool for data analysis in Excel, allowing you to automate complex tasks, create custom functions, and enhance your data’s interactivity and functionality.
Creating Custom Functions
Custom functions, also known as User Defined Functions (UDFs), can be created in VBA to perform calculations or operations that are not available with standard Excel functions. These UDFs can then be used directly in Excel cells.
Example: Custom Function for Average Calculation
Here’s an example of a custom function to calculate the average of a range, excluding any zeros:
Function AverageExcludingZeros(rng As Range) As Double
Dim cell As Range
Dim total As Double
Dim count As Integer
total = 0
count = 0
For Each cell In rng
If cell.Value <> 0 Then
total = total + cell.Value
count = count + 1
End If
Next cell
If count > 0 Then
AverageExcludingZeros = total / count
Else
AverageExcludingZeros = 0
End If
End Function
This function can be used in Excel like any other function: =AverageExcludingZeros(A1:A10)
Automating Data Analysis and Report Generation
Automation of data analysis and report generation can save considerable time. Let’s create a macro that analyzes a dataset and generates a report.
Example: Data Analysis and Report Generation
Suppose you have a dataset of sales figures and want to generate a monthly report:
Sub GenerateMonthlySalesReport()
Dim wsData As Worksheet, wsReport As Worksheet
Dim lastRow As Long, i As Long
Dim totalSales As Double
Set wsData = ThisWorkbook.Sheets("SalesData")
Set wsReport = ThisWorkbook.Sheets("MonthlyReport")
lastRow = wsData.Cells(wsData.Rows.Count, "A").End(xlUp).Row
totalSales = 0
For i = 2 To lastRow ' Assuming row 1 has headers
totalSales = totalSales + wsData.Cells(i, "B").Value ' Column B has sales figures
Next i
wsReport.Range("B1").Value = "Total Sales"
wsReport.Range("B2").Value = totalSales
End Sub
This macro calculates the total sales from the “SalesData” sheet and writes the result to the “MonthlyReport” sheet.
Best Practices and Tips for Efficient VBA Coding
Writing maintainable and efficient code is crucial for long-term success in VBA programming. Here are some best practices and tips to enhance your VBA code’s performance and readability.
Writing Maintainable Code
Maintainable code is easier to understand, debug, and update. Here are some key practices:
- Use Descriptive Variable Names: Choose names that reflect the purpose of the variable.
- Consistent Indentation: Use indentation to make your code structure clear.
- Comment Your Code: Include comments to explain the purpose of complex sections.
Example: Well-Commented Code
Sub CalculateSalesTax()
' Declare variables
Dim totalSales As Double
Dim salesTaxRate As Double
Dim salesTax As Double
' Initialize variables
totalSales = 1000 ' Example sales amount
salesTaxRate = 0.05 ' 5% sales tax
' Calculate sales tax
salesTax = totalSales * salesTaxRate
' Output the result
MsgBox "The sales tax is: $" & salesTax
End Sub
This example demonstrates clear variable names, proper indentation, and comments explaining each section.
Optimizing VBA Performance
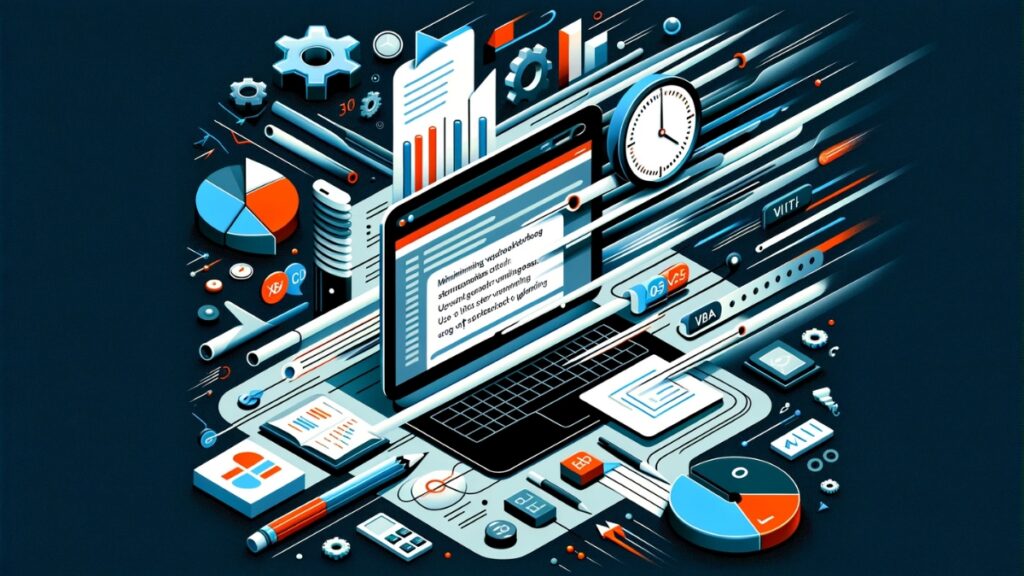
Optimizing your code can significantly improve the speed of your VBA scripts, especially when working with large datasets.
- Avoid Repeatedly Accessing the Worksheet: Minimize interactions with the worksheet within loops.
- Use
With
Statements: Useful for performing multiple operations on a single object. - Turn Off Screen Updating: Temporarily disable screen updates while your macro runs.
Example: Optimized Code for Performance
Sub OptimizePerformanceExample()
Application.ScreenUpdating = False ' Turn off screen updating
With ThisWorkbook.Sheets("Data")
.Range("A1").Value = "Optimized"
.Range("A2:A10").FillDown
End With
Application.ScreenUpdating = True ' Turn on screen updating
End Sub
This script minimizes screen updates and uses a With
statement for efficient range operations.
Conclusion
In this comprehensive guide, we’ve journeyed through the essentials of using Visual Basic for Applications (VBA) in Excel to automate tasks. From setting up your environment, understanding the fundamentals of VBA programming, to mastering advanced techniques for data analysis, we’ve covered a range of topics to enhance your Excel proficiency. By implementing these practices, you can transform how you interact with Excel, turning routine data tasks into efficient, automated processes. Remember, the key to mastering VBA lies in practice and continuous learning. As you begin to integrate these techniques into your daily workflows, you’ll discover new ways to leverage Excel’s capabilities, ultimately leading to more sophisticated and time-efficient data management strategies.
The journey with Excel VBA doesn’t end here. As with any programming language, there’s always more to learn and explore. The versatility of VBA in Excel allows for endless possibilities in data manipulation and automation. Whether you’re a beginner or an experienced user, the skills you’ve gained through this guide will serve as a strong foundation for your continued exploration and innovation in Excel.
Additional Resources
For those looking to deepen their understanding of VBA and explore more complex applications, here are some valuable resources:
- Excel VBA Programming For Dummies: A comprehensive book offering in-depth tutorials and practical examples.
- Microsoft’s VBA Documentation: Official documentation from Microsoft, providing detailed information on various VBA aspects.
- Online Courses: Platforms like Udemy, Coursera, and LinkedIn Learning offer courses ranging from beginner to advanced levels.
- VBA Forums and Online Communities: Websites like Stack Overflow and MrExcel provide platforms for asking questions and sharing knowledge.
- YouTube Tutorials: Visual learners may benefit from the multitude of step-by-step video tutorials available on YouTube.
- Practice Projects: The best way to learn is by doing. Try automating tasks in your current Excel workflows or create your projects.
Remember, the key to mastering any skill is consistent practice and a willingness to explore new challenges. Excel VBA offers a dynamic environment to develop your programming and data analysis skills, opening up a world of possibilities for automating and optimizing your data-related tasks.