JavaScript has been the backbone of web development for decades, shaping the way we interact with the web. As the complexity of web applications grew, the need for structured and efficient ways to build them led to the birth of JavaScript frameworks. These frameworks provide developers with robust tools and libraries, making it easier to create responsive, scalable, and maintainable web applications.
The Rise of JavaScript Frameworks
JavaScript frameworks emerged as a solution to tackle the challenges in building complex user interfaces and handling the vast amount of JavaScript code in a structured manner. They offer predefined templates and functions, allowing developers to focus more on the creative aspect of web development rather than getting bogged down by technicalities.
Core Benefits of Using JavaScript Frameworks
- Efficiency: Streamlines the development process by reducing the amount of code needed.
- Safety: Many frameworks come with built-in security features.
- Cost-Effective: Reduces development time, thereby cutting costs.
- Responsiveness: Facilitates the building of applications that work seamlessly across multiple devices.
- Community Support: Large communities often support these frameworks, providing valuable resources and support.
Popular JavaScript Frameworks: React, Vue, and Angular
React, Vue, and Angular are three of the most popular JavaScript frameworks in the modern development landscape:
- React: Developed by Facebook, React is more of a library than a full-fledged framework. It’s known for its component-based architecture, which makes building dynamic user interfaces a breeze.
- Vue: Vue is renowned for its simplicity and flexibility. It is a progressive framework that is particularly easy to integrate into projects due to its incremental adoptability.
- Angular: Angular, maintained by Google, is a comprehensive solution and is often chosen for enterprise-level applications. It’s a full-fledged framework with a wide range of features including two-way data binding, modular development, and an extensive suite of tools.
Choosing the Right Framework
Selecting the right framework depends on various factors like project requirements, team expertise, and the specific use case. Each framework has its strengths and is suited for different types of projects. React’s component-based approach is excellent for dynamic, high-performance user interfaces. Vue, with its simplicity, is ideal for quick development cycles and smaller projects. Angular is well-suited for large-scale enterprise applications due to its robust feature set.
Understanding React: The Popular Choice
React has cemented its position as one of the most popular JavaScript libraries for building user interfaces. Created by Facebook in 2013, React stands out for its efficient and flexible approach to UI development. Its core principle is the use of components – reusable, self-contained units of code that define the UI elements.
Key Features of React
- Component-Based Architecture: This architecture allows for the encapsulation of individual pieces of the UI, which can be reused and managed independently.
- Virtual DOM: React utilizes a virtual DOM, a lightweight copy of the actual DOM. This enables efficient updates and rendering, enhancing the performance of applications.
- JSX (JavaScript XML): JSX is a React extension that allows writing HTML structures in the same file as JavaScript code. This blending of HTML and JavaScript enhances the development experience.
- One-Way Data Binding: React’s one-way data flow provides better control throughout the application. It helps in debugging and makes the code more predictable.
Sample React Code
A basic example of a React component might look something like this:
import React from 'react';
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
export default Welcome;
In this simple component, ‘Welcome’ takes a property (props
) and renders a greeting message. Components like these can be composed together to build complex UIs.
React Ecosystem and Tools
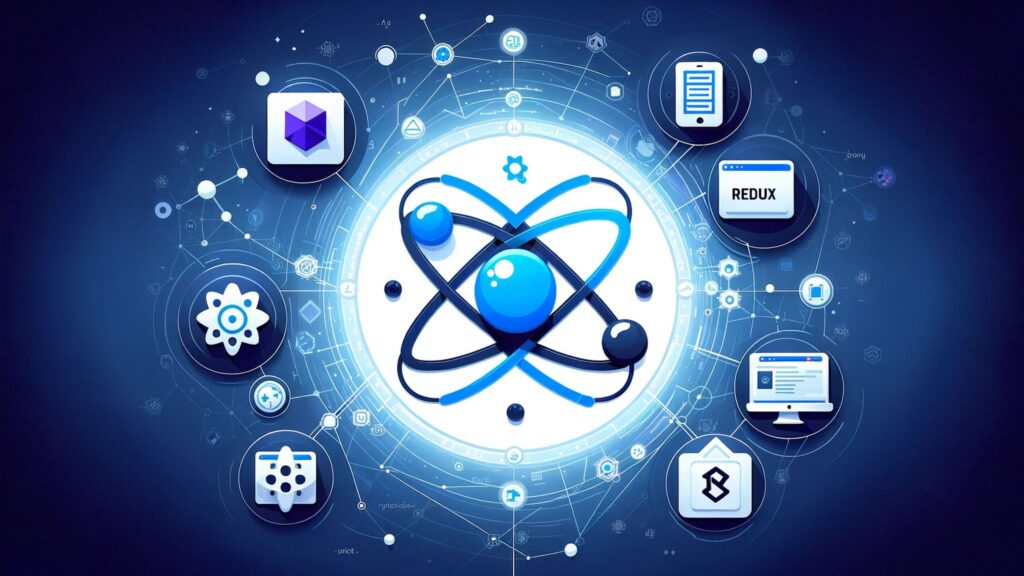
React’s ecosystem is rich and diverse, offering various tools and libraries to augment its core functionalities:
- Redux: A state management tool often used in React applications for managing application state more predictably.
- React Router: This library helps in adding navigation functionality to React applications.
- React Native: A framework for building native mobile apps using React.
React’s Popularity and Use Cases
React’s popularity can be attributed to its simplicity, efficiency, and the strong backing of Facebook. It’s widely used in single-page applications (SPAs) where dynamic content needs to be updated without reloading the entire page. Big names like Facebook, Instagram, and Airbnb use React in their applications.
Vue: The Progressive Framework
Vue.js, often referred to simply as Vue, has rapidly gained popularity in the JavaScript community for its progressive approach to building user interfaces. Created by Evan You in 2014, Vue is designed to be incrementally adoptable, making it as easy as possible to start using for small parts of an application and scalable enough for full-fledged applications.
Simplicity and Flexibility
Vue’s core library focuses on the view layer only, making it easy for developers to pick up and integrate with other libraries or existing projects. Vue is also perfectly capable of powering sophisticated Single-Page Applications when used in combination with modern tooling and supporting libraries.
Reactive Data Binding
Vue’s most distinctive feature is its reactive data binding system. Data binding in Vue is simple and intuitive, allowing developers to create dynamic interfaces quickly. The syntax is declarative, meaning that you can describe how the UI should look based on your application state, and Vue takes care of the rest.
Here’s an example of a simple Vue component:
<template>
<div>
<h1>{{ greeting }}</h1>
<button v-on:click="greet">Greet</button>
</div>
</template>
<script>
export default {
data() {
return {
greeting: 'Hello World'
}
},
methods: {
greet() {
this.greeting = 'Hello Vue!'
}
}
}
</script>
In this example, we have a greeting
data property and a greet
method that updates this property. The v-on
directive is used to handle the click event on the button.
Ecosystem and Community
Vue’s ecosystem is rapidly growing, with tools like Vuex for state management and Vue Router for client-side routing. The community around Vue is vibrant and active, contributing to a large collection of resources, plugins, and tutorials.
Use Cases and Adoption
Vue is particularly favored for its ease of learning and simplicity, making it a popular choice among both beginners and experienced developers. It’s used by companies like Alibaba, Xiaomi, and Expedia.
Angular: The Enterprise Favorite

Angular, developed and maintained by Google, is a powerful JavaScript framework used for building rich single-page applications. Released in 2016 as a complete rewrite of AngularJS, Angular provides a robust set of tools and features for developing complex client-side applications.
Comprehensive Framework
Unlike React and Vue, which are more library-like in their approach, Angular is a full-fledged framework. It includes everything you need to build a complex application, including dependency injection, routing, forms management, and more, all within a cohesive package.
TypeScript: A Core Aspect
Angular applications are built using TypeScript, a superset of JavaScript that adds static typing and object-oriented features. TypeScript enhances code quality and readability, which is particularly beneficial for larger projects.
Here’s a simple Angular component example:
import { Component } from '@angular/core';
@Component({
selector: 'app-hello-world',
template: `<h1>Hello World!</h1>`
})
export class HelloWorldComponent {}
In this example, the @Component
decorator defines the metadata for the component, including its selector and template. This component can then be used within other parts of an Angular application.
Strong Tooling and Ecosystem
Angular is known for its strong tooling. The Angular CLI (Command Line Interface) is a powerful tool that simplifies tasks like project creation, configuration, and deployment. Angular also has a rich ecosystem of third-party tools and integrations, as well as comprehensive documentation.
Use in Enterprise Applications
Angular’s architecture and tooling make it particularly well-suited for enterprise-scale applications. Its emphasis on code consistency and maintainability appeals to larger teams working on complex projects. Companies like Microsoft, Upwork, and Autodesk use Angular for their web applications.
Comparative Analysis: React vs. Vue vs. Angular
When selecting a JavaScript framework or library for a project, it’s crucial to understand the differences and similarities between React, Vue, and Angular. Each has its unique strengths and use cases, and the decision often depends on the specific needs of the project and the preferences of the development team.
React: Flexible and Efficient
React’s greatest strength lies in its simplicity and flexibility. It allows for the creation of complex user interfaces through a component-based approach. React is not a full-fledged framework, but rather a library focused on the view layer, which can be integrated with other libraries for state management, routing, etc.
// Example of a React component
function WelcomeMessage() {
const [name, setName] = React.useState("User");
return (
<div>
<h1>Welcome, {name}!</h1>
<input type="text" onChange={(e) => setName(e.target.value)} />
</div>
);
}
Vue: Progressive and Intuitive
Vue is appreciated for its progressive nature, meaning it can be adopted incrementally. It is highly intuitive and straightforward, making it a good choice for developers new to JavaScript frameworks. Vue’s template syntax and reactivity system make it easy to develop interactive UIs.
// Example of a Vue component
Vue.createApp({
data() {
return { name: 'User' };
},
template: `
<div>
<h1>Welcome, {{ name }}!</h1>
<input v-model="name" type="text" />
</div>
`
}).mount('#app');
Angular: Robust and Scalable

Angular is a full-scale framework offering a comprehensive solution for developing large-scale applications. It encompasses a wide range of features, including dependency injection, end-to-end tooling, and a suite of pre-built material design components. Angular is well-suited for enterprise-level applications due to its robustness and scalability.
// Example of an Angular component
import { Component } from '@angular/core';
@Component({
selector: 'app-welcome',
template: `
<div>
<h1>Welcome, {{ name }}!</h1>
<input [(ngModel)]="name" type="text" />
</div>
`
})
export class WelcomeComponent {
name = 'User';
}
Performance and Community Support
- Performance: React and Vue are known for their high performance, especially in handling dynamic content updates. Angular, while being slightly bulkier, offers strong performance for enterprise-scale applications.
- Community and Ecosystem: React has the backing of Facebook and a vast community. Vue, while community-driven, has seen significant growth in its ecosystem. Angular, supported by Google, has an extensive ecosystem and is often preferred in the corporate environment.
Learning Curve and Ease of Use
- React: Offers a moderate learning curve. Understanding JSX and the React ecosystem can be challenging for beginners.
- Vue: Known for its ease of learning. Its simplicity and clear documentation make it accessible to beginners.
- Angular: Has a steeper learning curve due to its comprehensive nature and the need to understand TypeScript and MVC architecture.
The Future of JavaScript Frameworks
As the web continues to evolve, so do JavaScript frameworks. React, Vue, and Angular continue to adapt and grow, but the landscape is also witnessing the emergence of new players and technologies. Understanding these trends is crucial for developers and businesses looking to stay ahead in the fast-paced world of web development.
Emerging Trends
- Server-Side Rendering (SSR): Frameworks are increasingly focusing on improving SEO and load times. React with Next.js, Vue with Nuxt.js, and Angular Universal all offer SSR capabilities, making applications more efficient and SEO-friendly.
- Web Components: The concept of web components is gaining traction. These are encapsulated, reusable components that work across modern browsers and can be used in various frameworks.
- Static Site Generation (SSG): SSG is becoming popular for its speed and reliability. Frameworks are now integrating SSG capabilities, as seen with Next.js for React and Nuxt.js for Vue.
- TypeScript Adoption: TypeScript’s popularity is on the rise. Angular already uses TypeScript, and React and Vue have increased support for it, acknowledging its benefits in enhancing code quality and maintainability.
- JAMstack Architecture: The JAMstack architecture (JavaScript, APIs, and Markup) is gaining popularity for building fast and secure sites. It leverages client-side JavaScript, reusable APIs, and prebuilt Markup, fitting well with React, Vue, and Angular.
- AI and Machine Learning Integration: Incorporating AI and machine learning in web applications is becoming more mainstream. Frameworks will likely evolve to better support these integrations.
The Future of React, Vue, and Angular
- React: React is likely to continue its focus on enhancing the developer experience and performance. The adoption of concurrent mode and server components could be game-changers.
- Vue: Vue 3 introduced the Composition API, offering better reusability and organization of code. Future versions may focus on improved TypeScript support and performance enhancements.
- Angular: Angular might continue to streamline its performance and tooling, making it more attractive for large-scale applications. Improved integration with Web Components could also be on the horizon.
Staying Ahead
For developers and companies, staying updated with these trends is crucial. Participating in community discussions, following the updates from framework creators, and experimenting with new releases are great ways to stay ahead.
Conclusion
In the dynamic world of web development, the choice between React, Vue, and Angular is more than a technical decision; it’s a strategic one. Each framework brings its unique strengths, philosophies, and communities to the table. React, with its vast ecosystem and flexibility, continues to be a favorite for many developers. Vue, known for its simplicity and ease of learning, is perfect for projects that require rapid development and a gentle learning curve. Angular, with its comprehensive feature set and robust architecture, remains the go-to choice for large-scale enterprise applications. The decision ultimately hinges on the specific needs and goals of your project, the skill set of your development team, and the long-term vision for your application.
As technology continues to advance, staying informed and adaptable is key. The landscape of JavaScript frameworks is constantly evolving, with new trends and innovations emerging regularly. Embracing these changes, experimenting with new tools and practices, and continually learning will ensure that developers and organizations can build efficient, scalable, and modern web applications that meet the demands of today’s users and anticipate the needs of tomorrow. Whether you choose React, Vue, Angular, or another framework, the focus should always be on creating engaging, performant, and user-friendly web experiences.