The world of web application development has been revolutionized by two powerful technologies: Java, a venerable and robust programming language, and the Spring Framework, a comprehensive toolset that complements Java’s capabilities. Together, they form an alliance that empowers developers to craft efficient, scalable, and secure web applications. This section delves into the synergy between Java and Spring, offering insights into how they have become indispensable tools in modern web development.
Java: The Cornerstone of Web Development
Java, developed by Sun Microsystems and now maintained by Oracle, stands out as one of the most widely-used programming languages in the world. Its design principles – “Write Once, Run Anywhere” (WORA) – ensure high portability across different computing platforms. Java’s key features that make it a preferred choice for web development include:
- Platform Independence: Java applications can run on any device or operating system that has the Java Virtual Machine (JVM).
- Object-Oriented Programming (OOP): Java’s OOP model simplifies complex problems into modular and manageable pieces.
- Memory Management: Automatic garbage collection in Java helps manage memory efficiently, reducing the risk of memory leaks and other related issues.
- Multithreading: Java’s built-in support for concurrent programming allows for handling multiple tasks simultaneously, enhancing application performance.
Spring Framework: Supercharging Java Applications
The Spring Framework is an open-source application framework that provides comprehensive infrastructure support for developing Java applications. Spring handles the “plumbing” of enterprise applications, allowing developers to focus on application-level business logic without unnecessary ties to specific deployment environments. Spring’s features include:
- Dependency Injection: Simplifies the way components are interconnected and promotes loose coupling.
- Aspect-Oriented Programming (AOP): Offers declarative programming to add additional behavior to code.
- Data Access Framework: Streamlines the integration with various database technologies.
- Spring MVC: A model-view-controller architecture for creating web applications.
- Spring Boot: Simplifies the bootstrapping and development of new Spring applications.
The Synergy Between Java and Spring in Modern Web Applications
The combination of Java and Spring Framework brings forth a robust ecosystem for web application development. This synergy provides several advantages:
- Enhanced Productivity: The Spring Framework’s ease of use, coupled with Java’s reliability, results in quicker development cycles.
- Scalability: Java’s multithreading capabilities, along with Spring’s efficient management of resources, make scaling applications seamless.
- Security: Spring’s security features, integrated with Java’s secure environment, fortify applications against various vulnerabilities.
- Diverse Ecosystem: Together, they offer a vast ecosystem of libraries, tools, and integrations, making it easier to extend functionalities and integrate with other systems.
What’s New in Java: Project Loom and Native Compilation
The Java ecosystem is constantly evolving, with recent advancements focusing on improving performance and simplifying application development. Two notable innovations are Project Loom and native compilation, which are set to redefine how Java handles concurrency and application packaging, respectively.
Project Loom: Rethinking Java Concurrency
Project Loom aims to significantly enhance Java’s concurrency model, addressing limitations of the traditional thread-based approach. Java’s platform threads, tied to the operating system’s threads, are limited by the number of available cores and are costly in terms of context switching. Project Loom introduces two key innovations:
- Virtual Threads (Green Threads): These are lightweight threads managed by the Java Virtual Machine (JVM) rather than the operating system. Virtual threads are designed to have a much lower overhead compared to platform threads, enabling Java applications to handle thousands, or even millions, of concurrent tasks efficiently.
- Structured Concurrency: This is a programming model that simplifies working with concurrent operations. It allows developers to treat a group of related concurrent operations as a single unit, making it easier to manage, debug, and scale concurrent applications.
Here’s an example demonstrating the use of virtual threads in Java:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class VirtualThreadExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newVirtualThreadPerTaskExecutor();
for (int i = 0; i < 10; i++) {
int finalI = i;
executor.execute(() -> System.out.println("Task " + finalI + " running on thread " + Thread.currentThread().getName()));
}
executor.shutdown();
}
}
In this snippet, Executors.newVirtualThreadPerTaskExecutor()
creates an executor service that uses virtual threads, enabling the efficient execution of multiple tasks concurrently.
Native Compilation with GraalVM
Native compilation in Java has been brought into the spotlight with the introduction of GraalVM. It provides an ahead-of-time (AOT) compilation feature, enabling Java applications to be compiled into native machine code. This approach offers several advantages:
- Improved Startup Time: Native compilation results in faster application startup, a significant benefit for cloud and microservice environments where quick scaling is essential.
- Reduced Memory Footprint: Native images generated by GraalVM are smaller, making them ideal for environments with limited resources.
- Increased Security: Smaller codebases imply fewer vectors for potential security vulnerabilities.
However, developers need to be cautious about using Java Reflection, as it can complicate the native compilation process. Frameworks like Spring are adapting to work seamlessly with GraalVM to leverage these benefits.
Spring Framework: The Backbone of Java Web Applications
![The world of web application development has been revolutionized by two powerful technologies: Java, a venerable and robust programming language, and the Spring Framework, a comprehensive toolset that complements Java's capabilities. Together, they form an alliance that empowers developers to craft efficient, scalable, and secure web applications. This section delves into the synergy between Java and Spring, offering insights into how they have become indispensable tools in modern web development.
Java: The Cornerstone of Web Development
Java, developed by Sun Microsystems and now maintained by Oracle, stands out as one of the most widely-used programming languages in the world. Its design principles - "Write Once, Run Anywhere" (WORA) - ensure high portability across different computing platforms. Java's key features that make it a preferred choice for web development include:
Platform Independence: Java applications can run on any device or operating system that has the Java Virtual Machine (JVM).
Object-Oriented Programming (OOP): Java's OOP model simplifies complex problems into modular and manageable pieces.
Memory Management: Automatic garbage collection in Java helps manage memory efficiently, reducing the risk of memory leaks and other related issues.
Multithreading: Java’s built-in support for concurrent programming allows for handling multiple tasks simultaneously, enhancing application performance.
Spring Framework: Supercharging Java Applications
The Spring Framework is an open-source application framework that provides comprehensive infrastructure support for developing Java applications. Spring handles the "plumbing" of enterprise applications, allowing developers to focus on application-level business logic without unnecessary ties to specific deployment environments. Spring's features include:
Dependency Injection: Simplifies the way components are interconnected and promotes loose coupling.
Aspect-Oriented Programming (AOP): Offers declarative programming to add additional behavior to code.
Data Access Framework: Streamlines the integration with various database technologies.
Spring MVC: A model-view-controller architecture for creating web applications.
Spring Boot: Simplifies the bootstrapping and development of new Spring applications.
The Synergy Between Java and Spring in Modern Web Applications
The combination of Java and Spring Framework brings forth a robust ecosystem for web application development. This synergy provides several advantages:
Enhanced Productivity: The Spring Framework's ease of use, coupled with Java's reliability, results in quicker development cycles.
Scalability: Java's multithreading capabilities, along with Spring's efficient management of resources, make scaling applications seamless.
Security: Spring's security features, integrated with Java's secure environment, fortify applications against various vulnerabilities.
Diverse Ecosystem: Together, they offer a vast ecosystem of libraries, tools, and integrations, making it easier to extend functionalities and integrate with other systems.
What's New in Java: Project Loom and Native Compilation
The Java ecosystem is constantly evolving, with recent advancements focusing on improving performance and simplifying application development. Two notable innovations are Project Loom and native compilation, which are set to redefine how Java handles concurrency and application packaging, respectively.
Project Loom: Rethinking Java Concurrency
Project Loom aims to significantly enhance Java's concurrency model, addressing limitations of the traditional thread-based approach. Java's platform threads, tied to the operating system's threads, are limited by the number of available cores and are costly in terms of context switching. Project Loom introduces two key innovations:
Virtual Threads (Green Threads): These are lightweight threads managed by the Java Virtual Machine (JVM) rather than the operating system. Virtual threads are designed to have a much lower overhead compared to platform threads, enabling Java applications to handle thousands, or even millions, of concurrent tasks efficiently.
Structured Concurrency: This is a programming model that simplifies working with concurrent operations. It allows developers to treat a group of related concurrent operations as a single unit, making it easier to manage, debug, and scale concurrent applications.
Here's an example demonstrating the use of virtual threads in Java:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public VirtualThreadExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newVirtualThreadPerTaskExecutor();
for (int i = 0; i < 10; i++) {
int finalI = i;
executor.execute(() -> System.out.println("Task " + finalI + " running on thread " + Thread.currentThread().getName()));
}
executor.shutdown();
}
}
In this snippet, Executors.newVirtualThreadPerTaskExecutor() creates an executor service that uses virtual threads, enabling the efficient execution of multiple tasks concurrently.
Native Compilation with GraalVM
Native compilation in Java has been brought into the spotlight with the introduction of GraalVM. It provides an ahead-of-time (AOT) compilation feature, enabling Java applications to be compiled into native machine code. This approach offers several advantages:
Improved Startup Time: Native compilation results in faster application startup, a significant benefit for cloud and microservice environments where quick scaling is essential.
Reduced Memory Footprint: Native images generated by GraalVM are smaller, making them ideal for environments with limited resources.
Increased Security: Smaller codebases imply fewer vectors for potential security vulnerabilities.
However, developers need to be cautious about using Java Reflection, as it can complicate the native compilation process. Frameworks like Spring are adapting to work seamlessly with GraalVM to leverage these benefits.
Spring Framework: The Backbone of Java Web Applications
The Spring Framework has established itself as a cornerstone in the Java ecosystem, especially for building web applications. It stands out for its comprehensive suite of features that simplify and accelerate the development of robust, enterprise-level applications.
Key Features of the Spring Framework
Dependency Injection: At the heart of Spring is its dependency injection mechanism, which promotes loose coupling and easier testing. It manages the creation and binding of dependencies within your application.
Spring MVC: The Model-View-Controller architecture in Spring MVC facilitates the development of well-structured web applications. It offers a powerful way to handle HTTP requests and responses.
Data Access: Spring provides extensive support for interacting with databases, including both relational and non-relational models. It simplifies the integration with JDBC and provides transaction management.
Spring Boot: A project within the Spring ecosystem, Spring Boot, takes an opinionated view of the Spring platform, which simplifies configuration and deployment. It offers a range of starters for easy dependency management.
Here is a simple example of a Spring Boot web application:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public MyApp {
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
}
@GetMapping("/")
public String home() {
return "Welcome to the Spring Boot application!";
}
}
In this code snippet, @SpringBootApplication signifies a Spring Boot application, and @RestController indicates that the serves RESTful web services. The home() method responds to HTTP GET requests at the root URL.
Spring Security: A Closer Look
Security is paramount in web application development, and Spring Security provides a comprehensive security solution for Java applications. It offers protection against common vulnerabilities and supports numerous authentication and authorization mechanisms.
Authentication and Authorization: Spring Security handles user authentication and restricts access to resources based on roles and permissions.
Protection Against Common Threats: It provides defenses against attacks like session fixation, clickjacking, and cross-site request forgery (CSRF).
Simplifying Data Access with Spring
Spring's data access frameworks, like Spring Data, make it easier to work with databases, whether it’s a relational database or a NoSQL store. It offers a consistent data access experience, reduces boilerplate code, and provides repository interfaces for various data stores.
Spring Data JPA: Simplifies the implementation of data access layers by reducing the amount of boilerplate code required.
Spring Data MongoDB: Offers easy integration with MongoDB, a popular NoSQL database.
Developer Productivity and Cloud-Readiness
Spring Boot, in particular, is designed to boost developer productivity with its convention-over-configuration approach. It offers a range of starters and auto-configurations to jumpstart application development. Furthermore, Spring's cloud-native support, including Spring Cloud, provides tools for developing microservices and deploying applications in cloud environments.
Developer Productivity with Spring Boot
Spring Boot is a groundbreaking project in the Spring ecosystem designed to enhance developer productivity and simplify the process of creating Spring applications. Its convention-over-configuration approach drastically reduces the amount of setup and configuration needed to start a project.
Key Features of Spring Boot
Auto-Configuration: Spring Boot automatically configures your application based on the libraries present on the classpath. This significantly reduces the need for specifying beans and XML configurations.
Standalone Applications: Spring Boot allows you to create standalone applications that can be run directly from the command line without needing an external servlet container.
Spring Initializr: A web-based tool that helps in generating a project structure with desired dependencies quickly.
Here is an example of a basic Spring Boot application that sets up a simple RESTful web service:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public SimpleApplication {
public static void main(String[] args) {
SpringApplication.run(SimpleApplication.class, args);
}
@GetMapping("/hello")
public String sayHello() {
return "Hello, Spring Boot!";
}
}
In this code, @SpringBootApplication signifies a Spring Boot application, while @RestController marks the as a controller where every method returns a domain object instead of a view. The sayHello method is mapped to a GET request at "/hello" and returns a simple string.
Spring Boot’s Production-Ready Features
Spring Boot comes with several built-in features that make applications production-ready:
Health Checks: It provides health check endpoints to monitor the status of your application.
Metrics: Spring Boot supports metrics collection to help you understand the performance and behavior of your application.
Externalized Configuration: Easy management of configuration outside your application code, such as in properties files, YAML files, environment variables, and command-line arguments.
Simplifying Dependency Management
Spring Boot's starters are a set of convenient dependency descriptors that you can include in your application. Each starter is tailored to support a specific type of development, such as web applications, data access, messaging, and more. They help in managing dependency versions and ensuring that your application has all the necessary Spring components.
Streamlining Application Deployment
Spring Boot simplifies the deployment process with embedded servlet containers like Tomcat, Jetty, or Undertow, allowing applications to run independently without requiring deployment to an external container. This feature, coupled with Spring Boot’s executable JAR format, makes deploying your application a straightforward process.
Security in Web Applications: Spring Security Deep Dive
Spring Security is a powerful and customizable authentication and access-control framework within the Spring ecosystem. It is vital for securing web applications against various security threats and vulnerabilities. Spring Security provides a comprehensive set of functionalities covering both authentication and authorization.
Authentication and Authorization with Spring Security
Authentication: It involves verifying the identity of a user or system. Spring Security supports multiple authentication mechanisms like form-based authentication, OAuth, LDAP, and more.
Authorization: This is about granting or denying permissions to authenticated users. Spring Security enables role-based and fine-grained access control to resources.
Here's a basic example to secure a Spring Boot application using Spring Security:
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@EnableWebSecurity
public SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password("{noop}password").roles("USER")
.and()
.withUser("admin").password("{noop}adminpass").roles("ADMIN");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasRole("USER")
.antMatchers("/", "/home").permitAll()
.and()
.formLogin();
}
}
In this code, @EnableWebSecurity activates Spring Security's web security support. The configure methods set up the in-memory authentication and define the authorization policies for different URL patterns.
Protection Against Common Security Threats
Spring Security provides out-of-the-box protection against common web application security threats, including:
Cross-Site Request Forgery (CSRF) Protection: Automatically enables CSRF protection to prevent attacks.
Session Management: Handles session fixation attacks, and allows configuring session creation and expiration policies.
Security Headers: Adds various HTTP headers for securing applications, like HTTP Strict Transport Security (HSTS), Content Security Policy (CSP), and X-Frame-Options.
OAuth2 and JWT in Spring Security
For modern web applications, Spring Security supports OAuth2 and JSON Web Tokens (JWT) for securing REST APIs. OAuth2 is widely used for authorization in client-server applications, and JWT is a token format used in OAuth2 for secure data transmission.
Data Access Made Easy with Spring
Spring significantly simplifies data access in web applications, supporting a wide range of data management activities, from simple database interactions to complex transaction management. This is achieved primarily through Spring Data, a high-level Spring project that abstracts data access in the application.
Spring Data JPA for Relational Databases
Spring Data JPA (Java Persistence API) is a part of the larger Spring Data family that makes it easier to implement JPA-based repositories. It reduces the amount of boilerplate code required and provides a more straightforward way to interact with relational databases.
Here's an example of how to define a simple repository using Spring Data JPA:
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Entity
public User {
@Id
private Long id;
private String name;
// standard getters and setters
}
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
List<User> findByName(String name);
}
In this example, the UserRepository interface extends JpaRepository, providing CRUD operations and the ability to define custom queries, like findByName, without implementing the method explicitly.
Spring Data MongoDB for NoSQL Databases
For applications using NoSQL databases, Spring Data MongoDB is an excellent choice. It provides an easy integration with MongoDB, a popular NoSQL database, allowing for flexible and scalable data storage.
Example of a simple MongoDB repository in Spring:
import org.springframework.data.mongodb.repository.MongoRepository;
import org.springframework.stereotype.Repository;
@Document
public Product {
@Id
private String id;
private String name;
// standard getters and setters
}
@Repository
public interface ProductRepository extends MongoRepository<Product, String> {
List<Product> findByName(String name);
}
This snippet shows how to define a ProductRepository for MongoDB. Similar to JPA, it extends MongoRepository, providing several methods for interacting with the database and the possibility to add custom query methods.
Transaction Management in Spring
Spring provides a consistent transaction management interface that can scale from local transactions (single database) to global transactions (multiple databases). It supports programmatic and declarative transaction management, giving developers the flexibility to choose the best approach for their application.
Embracing Cloud-Native Development with Spring
The push towards cloud-native development has been a significant trend in software engineering. Spring, particularly with Spring Boot and Spring Cloud, offers comprehensive support for building and deploying applications that are optimized for the cloud.
Spring Cloud for Microservices
Spring Cloud provides tools for developers to quickly build some of the common patterns in distributed systems (e.g., configuration management, service discovery, circuit breakers). It is beneficial in microservices architectures where it's crucial to handle service discovery, load balancing, and fault tolerance.
Consider an example of a service discovery setup using Spring Cloud:
@SpringBootApplication
@EnableEurekaServer
public ServiceDiscoveryApplication {
public static void main(String[] args) {
SpringApplication.run(ServiceDiscoveryApplication.class, args);
}
}
In this example, @EnableEurekaServer annotation is used to turn a Spring Boot application into a Eureka Service Discovery Server. Microservices register themselves with the Eureka server and can discover other services through it.
Spring Boot for Cloud-Native Applications
Spring Boot's ability to create stand-alone, production-grade applications aligns well with cloud-native development principles. It simplifies the deployment process, is container-friendly, and works seamlessly with cloud platforms.
Configuration Management in the Cloud
Spring Cloud Config provides server and client-side support for externalized configuration in a distributed system. This approach is crucial for cloud-native applications that need to be adaptable to varying environments without code changes.
Reactive Programming in Java: A New Paradigm
Reactive programming represents a paradigm shift in the way applications are built and data is handled, especially in Java. It's particularly useful for applications that deal with streams of data, especially when these streams need to be handled asynchronously or in a non-blocking manner. This is where Project Reactor and RxJava come into play.
Project Reactor and Spring WebFlux
Project Reactor is a reactive programming library for building non-blocking applications on the JVM and is the foundation for Spring's reactive support. Spring WebFlux, a part of the Spring Framework, provides reactive programming support for web applications.
Here is a basic example of a reactive REST endpoint using Spring WebFlux:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import reactor.core.publisher.Mono;
@SpringBootApplication
@RestController
public ReactiveApp {
public static void main(String[] args) {
SpringApplication.run(ReactiveApp.class, args);
}
@GetMapping("/reactive")
public Mono<String> reactiveHello() {
return Mono.just("Hello, Reactive World!");
}
}
In this example, Mono is a Reactor core type that represents a single value or an empty value asynchronously. The reactiveHello method defines a reactive API endpoint that returns a single string value.
RxJava: Reactive Extensions for the JVM
RxJava is another popular library for composing asynchronous and event-based programs using observable sequences. It extends the observer pattern to support data/events sequence and adds operators that allow you to compose sequences together declaratively.
Example of using RxJava to create a simple observable and subscriber:
import io.reactivex.rxjava3.core.Observable;
public RxJavaExample {
public static void main(String[] args) {
Observable<String> observable = Observable.just("Hello", "RxJava");
observable.subscribe(System.out::println);
}
}
This code creates an Observable that emits two strings and subscribes to it, printing each emitted item to the console.
Java in Data Science and Big Data
Java's robustness and performance make it a suitable choice for data science and big data applications. Its ability to handle large-scale data processing and integration with popular data science tools and libraries adds to its versatility.
Java for Data Processing and Machine Learning
Java's ecosystem includes several libraries and frameworks for data processing and machine learning. One such framework is Apache Hadoop, a popular choice for handling big data. It allows for distributed processing of large data sets across clusters of computers.
Here is a simple example of how Java can be used with Hadoop for data processing:
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
public HadoopExample {
public static TokenizerMapper extends Mapper<Object, Text, Text, IntWritable>{
// Map function
}
public static IntSumReducer extends Reducer<Text,IntWritable,Text,IntWritable> {
// Reduce function
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "word count");
// Job setup code
}
}
This basic example sets up a Hadoop job with mapper and reducer classes for a word count task, a common starting point in big data processing.
Eclipse Deeplearning4j for Neural Networks
Deeplearning4j is a deep learning library for Java and the JVM. It's designed for use in business environments and supports various neural network types, including convolutional and recurrent networks.
Here's a basic setup for a neural network using Deeplearning4j:
import org.deeplearning4j.nn.conf.NeuralNetConfiguration;
import org.deelearning4j.nn.multilayer.MultiLayerNetwork;
import org.deeplearning4j.nn.conf.layers.DenseLayer;
import org.deelearning4j.nn.conf.layers.OutputLayer;
MultiLayerNetwork model = new NeuralNetConfiguration.Builder()
.list()
.layer(new DenseLayer.Builder().nIn(numInputs).nOut(numHiddenNodes).build())
.layer(new OutputLayer.Builder().nIn(numHiddenNodes).nOut(numOutputs).build())
.build();
This code snippet demonstrates the creation of a simple neural network with input, hidden, and output layers using Deeplearning4j.
Conclusion: The Future of Java and Spring in Web Development
As we look towards the future, Java and the Spring Framework continue to stand as pivotal forces in the landscape of web development. Java, with its ongoing enhancements and evolution, remains a cornerstone for robust and scalable application development. The introduction of features like Project Loom and the advancements in native compilation demonstrate Java's commitment to staying at the forefront of technological innovation. These developments not only promise improved performance and efficiency but also open new avenues for Java in areas like concurrent programming and cloud-native applications.
The Spring Framework, paralleling Java's growth, is continuously evolving to embrace new trends and technologies. With its focus on simplifying the development process and enhancing productivity, Spring is adapting to the changing needs of developers and businesses alike. The integration of reactive programming, support for microservices architectures, and its continuous strides in security and data management, position Spring as an adaptable and powerful tool for modern web application development. As the digital landscape progresses, Java and Spring are poised to remain key players, adapting and growing to meet the ever-evolving challenges of web development.](https://www.geekpedia.com/wp-content/uploads/2023/12/Spring-Framework-The-Backbone-of-Java-Web-Applications-1024x585.jpg)
The Spring Framework has established itself as a cornerstone in the Java ecosystem, especially for building web applications. It stands out for its comprehensive suite of features that simplify and accelerate the development of robust, enterprise-level applications.
Key Features of the Spring Framework
- Dependency Injection: At the heart of Spring is its dependency injection mechanism, which promotes loose coupling and easier testing. It manages the creation and binding of dependencies within your application.
- Spring MVC: The Model-View-Controller architecture in Spring MVC facilitates the development of well-structured web applications. It offers a powerful way to handle HTTP requests and responses.
- Data Access: Spring provides extensive support for interacting with databases, including both relational and non-relational models. It simplifies the integration with JDBC and provides transaction management.
- Spring Boot: A project within the Spring ecosystem, Spring Boot, takes an opinionated view of the Spring platform, which simplifies configuration and deployment. It offers a range of starters for easy dependency management.
Here is a simple example of a Spring Boot web application:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class MyApp {
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
}
@GetMapping("/")
public String home() {
return "Welcome to the Spring Boot application!";
}
}
In this code snippet, @SpringBootApplication
signifies a Spring Boot application, and @RestController
indicates that the class serves RESTful web services. The home()
method responds to HTTP GET requests at the root URL.
Spring Security: A Closer Look
Security is paramount in web application development, and Spring Security provides a comprehensive security solution for Java applications. It offers protection against common vulnerabilities and supports numerous authentication and authorization mechanisms.
- Authentication and Authorization: Spring Security handles user authentication and restricts access to resources based on roles and permissions.
- Protection Against Common Threats: It provides defenses against attacks like session fixation, clickjacking, and cross-site request forgery (CSRF).
Simplifying Data Access with Spring
Spring’s data access frameworks, like Spring Data, make it easier to work with databases, whether it’s a relational database or a NoSQL store. It offers a consistent data access experience, reduces boilerplate code, and provides repository interfaces for various data stores.
- Spring Data JPA: Simplifies the implementation of data access layers by reducing the amount of boilerplate code required.
- Spring Data MongoDB: Offers easy integration with MongoDB, a popular NoSQL database.
Developer Productivity and Cloud-Readiness
Spring Boot, in particular, is designed to boost developer productivity with its convention-over-configuration approach. It offers a range of starters and auto-configurations to jumpstart application development. Furthermore, Spring’s cloud-native support, including Spring Cloud, provides tools for developing microservices and deploying applications in cloud environments.
Developer Productivity with Spring Boot
Spring Boot is a groundbreaking project in the Spring ecosystem designed to enhance developer productivity and simplify the process of creating Spring applications. Its convention-over-configuration approach drastically reduces the amount of setup and configuration needed to start a project.
Key Features of Spring Boot
- Auto-Configuration: Spring Boot automatically configures your application based on the libraries present on the classpath. This significantly reduces the need for specifying beans and XML configurations.
- Standalone Applications: Spring Boot allows you to create standalone applications that can be run directly from the command line without needing an external servlet container.
- Spring Initializr: A web-based tool that helps in generating a project structure with desired dependencies quickly.
Here is an example of a basic Spring Boot application that sets up a simple RESTful web service:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class SimpleApplication {
public static void main(String[] args) {
SpringApplication.run(SimpleApplication.class, args);
}
@GetMapping("/hello")
public String sayHello() {
return "Hello, Spring Boot!";
}
}
In this code, @SpringBootApplication
signifies a Spring Boot application, while @RestController
marks the class as a controller where every method returns a domain object instead of a view. The sayHello
method is mapped to a GET request at “/hello” and returns a simple string.
Spring Boot’s Production-Ready Features
Spring Boot comes with several built-in features that make applications production-ready:
- Health Checks: It provides health check endpoints to monitor the status of your application.
- Metrics: Spring Boot supports metrics collection to help you understand the performance and behavior of your application.
- Externalized Configuration: Easy management of configuration outside your application code, such as in properties files, YAML files, environment variables, and command-line arguments.
Simplifying Dependency Management
Spring Boot’s starters are a set of convenient dependency descriptors that you can include in your application. Each starter is tailored to support a specific type of development, such as web applications, data access, messaging, and more. They help in managing dependency versions and ensuring that your application has all the necessary Spring components.
Streamlining Application Deployment
Spring Boot simplifies the deployment process with embedded servlet containers like Tomcat, Jetty, or Undertow, allowing applications to run independently without requiring deployment to an external container. This feature, coupled with Spring Boot’s executable JAR format, makes deploying your application a straightforward process.
Security in Web Applications: Spring Security Deep Dive
Spring Security is a powerful and customizable authentication and access-control framework within the Spring ecosystem. It is vital for securing web applications against various security threats and vulnerabilities. Spring Security provides a comprehensive set of functionalities covering both authentication and authorization.
Authentication and Authorization with Spring Security
- Authentication: It involves verifying the identity of a user or system. Spring Security supports multiple authentication mechanisms like form-based authentication, OAuth, LDAP, and more.
- Authorization: This is about granting or denying permissions to authenticated users. Spring Security enables role-based and fine-grained access control to resources.
Here’s a basic example to secure a Spring Boot application using Spring Security:
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password("{noop}password").roles("USER")
.and()
.withUser("admin").password("{noop}adminpass").roles("ADMIN");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasRole("USER")
.antMatchers("/", "/home").permitAll()
.and()
.formLogin();
}
}
In this code, @EnableWebSecurity
activates Spring Security’s web security support. The configure
methods set up the in-memory authentication and define the authorization policies for different URL patterns.
Protection Against Common Security Threats
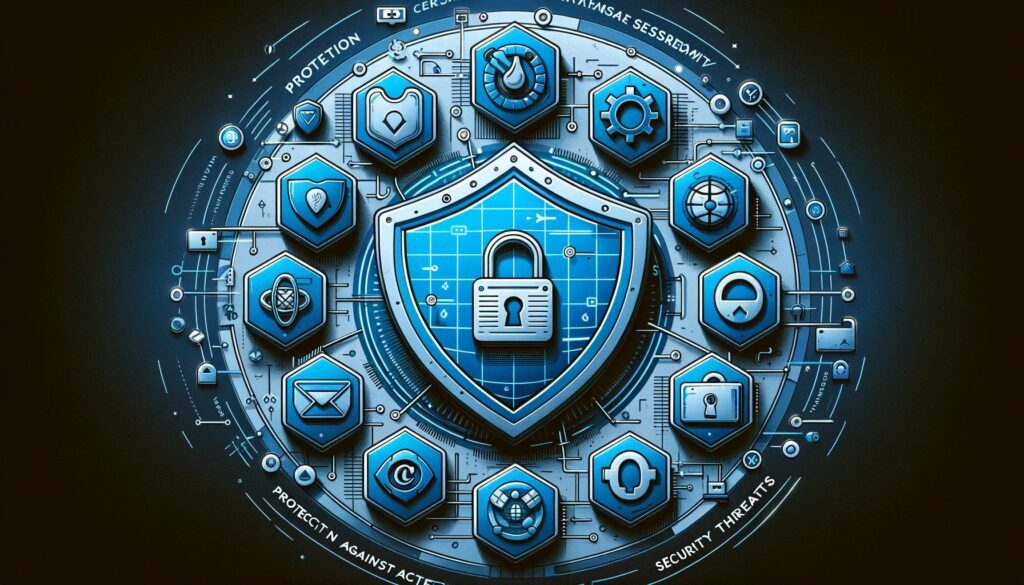
Spring Security provides out-of-the-box protection against common web application security threats, including:
- Cross-Site Request Forgery (CSRF) Protection: Automatically enables CSRF protection to prevent attacks.
- Session Management: Handles session fixation attacks, and allows configuring session creation and expiration policies.
- Security Headers: Adds various HTTP headers for securing applications, like HTTP Strict Transport Security (HSTS), Content Security Policy (CSP), and X-Frame-Options.
OAuth2 and JWT in Spring Security
For modern web applications, Spring Security supports OAuth2 and JSON Web Tokens (JWT) for securing REST APIs. OAuth2 is widely used for authorization in client-server applications, and JWT is a token format used in OAuth2 for secure data transmission.
Data Access Made Easy with Spring
Spring significantly simplifies data access in web applications, supporting a wide range of data management activities, from simple database interactions to complex transaction management. This is achieved primarily through Spring Data, a high-level Spring project that abstracts data access in the application.
Spring Data JPA for Relational Databases
Spring Data JPA (Java Persistence API) is a part of the larger Spring Data family that makes it easier to implement JPA-based repositories. It reduces the amount of boilerplate code required and provides a more straightforward way to interact with relational databases.
Here’s an example of how to define a simple repository using Spring Data JPA:
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Entity
public class User {
@Id
private Long id;
private String name;
// standard getters and setters
}
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
List<User> findByName(String name);
}
In this example, the UserRepository
interface extends JpaRepository
, providing CRUD operations and the ability to define custom queries, like findByName
, without implementing the method explicitly.
Spring Data MongoDB for NoSQL Databases
For applications using NoSQL databases, Spring Data MongoDB is an excellent choice. It provides an easy integration with MongoDB, a popular NoSQL database, allowing for flexible and scalable data storage.
Example of a simple MongoDB repository in Spring:
import org.springframework.data.mongodb.repository.MongoRepository;
import org.springframework.stereotype.Repository;
@Document
public class Product {
@Id
private String id;
private String name;
// standard getters and setters
}
@Repository
public interface ProductRepository extends MongoRepository<Product, String> {
List<Product> findByName(String name);
}
This snippet shows how to define a ProductRepository
for MongoDB. Similar to JPA, it extends MongoRepository
, providing several methods for interacting with the database and the possibility to add custom query methods.
Transaction Management in Spring
Spring provides a consistent transaction management interface that can scale from local transactions (single database) to global transactions (multiple databases). It supports programmatic and declarative transaction management, giving developers the flexibility to choose the best approach for their application.
Embracing Cloud-Native Development with Spring
The push towards cloud-native development has been a significant trend in software engineering. Spring, particularly with Spring Boot and Spring Cloud, offers comprehensive support for building and deploying applications that are optimized for the cloud.
Spring Cloud for Microservices
Spring Cloud provides tools for developers to quickly build some of the common patterns in distributed systems (e.g., configuration management, service discovery, circuit breakers). It is beneficial in microservices architectures where it’s crucial to handle service discovery, load balancing, and fault tolerance.
Consider an example of a service discovery setup using Spring Cloud:
@SpringBootApplication
@EnableEurekaServer
public class ServiceDiscoveryApplication {
public static void main(String[] args) {
SpringApplication.run(ServiceDiscoveryApplication.class, args);
}
}
In this example, @EnableEurekaServer
annotation is used to turn a Spring Boot application into a Eureka Service Discovery Server. Microservices register themselves with the Eureka server and can discover other services through it.
Spring Boot for Cloud-Native Applications
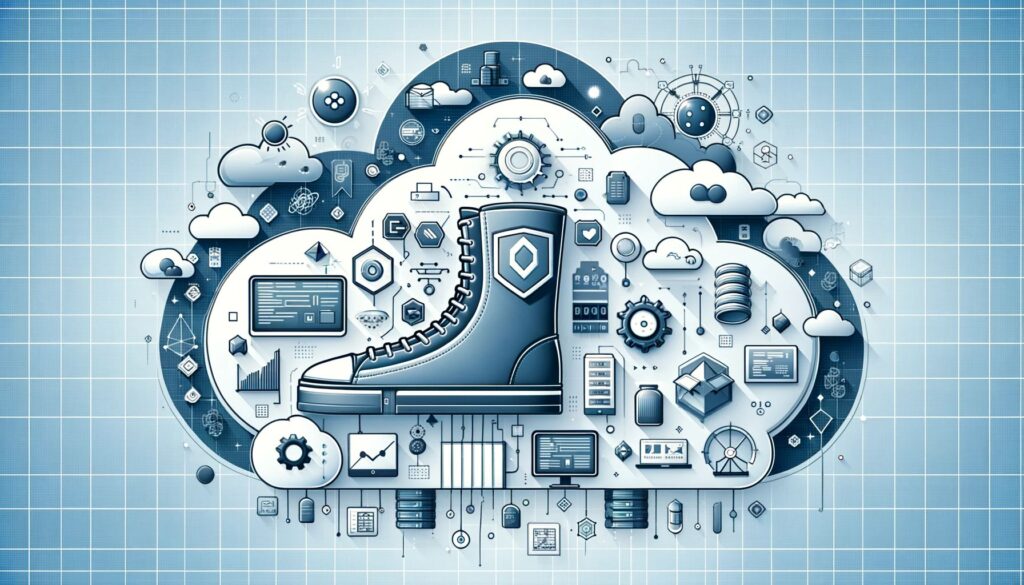
Spring Boot’s ability to create stand-alone, production-grade applications aligns well with cloud-native development principles. It simplifies the deployment process, is container-friendly, and works seamlessly with cloud platforms.
Configuration Management in the Cloud
Spring Cloud Config provides server and client-side support for externalized configuration in a distributed system. This approach is crucial for cloud-native applications that need to be adaptable to varying environments without code changes.
Reactive Programming in Java: A New Paradigm
Reactive programming represents a paradigm shift in the way applications are built and data is handled, especially in Java. It’s particularly useful for applications that deal with streams of data, especially when these streams need to be handled asynchronously or in a non-blocking manner. This is where Project Reactor and RxJava come into play.
Project Reactor and Spring WebFlux
Project Reactor is a reactive programming library for building non-blocking applications on the JVM and is the foundation for Spring’s reactive support. Spring WebFlux, a part of the Spring Framework, provides reactive programming support for web applications.
Here is a basic example of a reactive REST endpoint using Spring WebFlux:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import reactor.core.publisher.Mono;
@SpringBootApplication
@RestController
public class ReactiveApp {
public static void main(String[] args) {
SpringApplication.run(ReactiveApp.class, args);
}
@GetMapping("/reactive")
public Mono<String> reactiveHello() {
return Mono.just("Hello, Reactive World!");
}
}
In this example, Mono
is a Reactor core type that represents a single value or an empty value asynchronously. The reactiveHello
method defines a reactive API endpoint that returns a single string value.
RxJava: Reactive Extensions for the JVM
RxJava is another popular library for composing asynchronous and event-based programs using observable sequences. It extends the observer pattern to support data/events sequence and adds operators that allow you to compose sequences together declaratively.
Example of using RxJava to create a simple observable and subscriber:
import io.reactivex.rxjava3.core.Observable;
public class RxJavaExample {
public static void main(String[] args) {
Observable<String> observable = Observable.just("Hello", "RxJava");
observable.subscribe(System.out::println);
}
}
This code creates an Observable
that emits two strings and subscribes to it, printing each emitted item to the console.
Java in Data Science and Big Data
Java’s robustness and performance make it a suitable choice for data science and big data applications. Its ability to handle large-scale data processing and integration with popular data science tools and libraries adds to its versatility.
Java for Data Processing and Machine Learning
Java’s ecosystem includes several libraries and frameworks for data processing and machine learning. One such framework is Apache Hadoop, a popular choice for handling big data. It allows for distributed processing of large data sets across clusters of computers.
Here is a simple example of how Java can be used with Hadoop for data processing:
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
public class HadoopExample {
public static class TokenizerMapper extends Mapper<Object, Text, Text, IntWritable>{
// Map function
}
public static class IntSumReducer extends Reducer<Text,IntWritable,Text,IntWritable> {
// Reduce function
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "word count");
// Job setup code
}
}
This basic example sets up a Hadoop job with mapper and reducer classes for a word count task, a common starting point in big data processing.
Eclipse Deeplearning4j for Neural Networks
Deeplearning4j is a deep learning library for Java and the JVM. It’s designed for use in business environments and supports various neural network types, including convolutional and recurrent networks.
Here’s a basic setup for a neural network using Deeplearning4j:
import org.deeplearning4j.nn.conf.NeuralNetConfiguration;
import org.deelearning4j.nn.multilayer.MultiLayerNetwork;
import org.deeplearning4j.nn.conf.layers.DenseLayer;
import org.deelearning4j.nn.conf.layers.OutputLayer;
MultiLayerNetwork model = new NeuralNetConfiguration.Builder()
.list()
.layer(new DenseLayer.Builder().nIn(numInputs).nOut(numHiddenNodes).build())
.layer(new OutputLayer.Builder().nIn(numHiddenNodes).nOut(numOutputs).build())
.build();
This code snippet demonstrates the creation of a simple neural network with input, hidden, and output layers using Deeplearning4j.
Conclusion: The Future of Java and Spring in Web Development
As we look towards the future, Java and the Spring Framework continue to stand as pivotal forces in the landscape of web development. Java, with its ongoing enhancements and evolution, remains a cornerstone for robust and scalable application development. The introduction of features like Project Loom and the advancements in native compilation demonstrate Java’s commitment to staying at the forefront of technological innovation. These developments not only promise improved performance and efficiency but also open new avenues for Java in areas like concurrent programming and cloud-native applications.
The Spring Framework, paralleling Java’s growth, is continuously evolving to embrace new trends and technologies. With its focus on simplifying the development process and enhancing productivity, Spring is adapting to the changing needs of developers and businesses alike. The integration of reactive programming, support for microservices architectures, and its continuous strides in security and data management, position Spring as an adaptable and powerful tool for modern web application development. As the digital landscape progresses, Java and Spring are poised to remain key players, adapting and growing to meet the ever-evolving challenges of web development.