Entity Framework Core (EF Core) has become a cornerstone of data access strategies in .NET applications, offering a powerful Object-Relational Mapping (ORM) toolset that bridges the gap between object-oriented programming and relational databases. This section provides an overview of EF Core, emphasizing its evolution, features, and why it’s a critical tool for developers working in the C# ecosystem.
The Essence of EF Core as an ORM Tool
At its core, Entity Framework Core is an ORM framework, which means it enables developers to work with data in a database using .NET objects, thus eliminating the need for most of the data-access code that developers typically need to write. EF Core tracks changes in these objects and translates operations on them into database queries, streamlining the interaction with the database.
Key Features of EF Core:
- LINQ Queries: EF Core allows the use of LINQ (Language Integrated Query) to query the database, providing a comfortable and familiar syntax for C# developers.
- Change Tracking: It keeps track of changes made to objects so that it can update the database accordingly.
- Migrations: EF Core provides a set of tools to update the database schema based on changes in the application’s data model.
The Evolution of EF Core
EF Core has undergone significant evolution since its inception. The journey from the early versions to the latest EF Core 8 has seen numerous enhancements, making it more robust, efficient, and adaptable to various data access requirements.
- Early Versions: Focused on establishing a reliable data access platform with basic ORM capabilities.
- EF Core 3.x and 5: Introduced major performance improvements and many new features, including many-to-many relationships, improved LINQ translations, and more.
- EF Core 6: Brought much-needed performance enhancements and features like temporal tables, JSON column mapping, and improved LINQ queries.
- EF Core 7: Focused on JSON columns, aggregate type mapping for complex data structures, and other improvements.
- EF Core 8: The latest version, as of writing, offers enhanced SQL translations, better support for SQLite, native support for DateOnly and TimeOnly data types, and more.
Why EF Core is Essential for C# Developers
EF Core is not just an ORM; it represents a strategic choice for .NET developers, especially those using C#. Its deep integration with .NET Core and ASP.NET Core makes it an indispensable tool for modern web and cloud applications. The ability to work with a variety of databases, from SQL Server to SQLite and in-memory databases, further enhances its versatility.
New Features in EF Core 8

The release of Entity Framework Core 8 marks a significant milestone in the development of this ORM tool. This version introduces a range of features and improvements that enhance its functionality, performance, and ease of use.
Enhanced SQL Translations and Math Functions
EF Core 8 has worked closely with the .NET team to introduce new generic math methods implemented on double and float types. These methods are also translated to SQL, providing a more seamless interaction with the database. This enhancement means that developers can use familiar .NET math functions in their LINQ queries, and EF Core will translate them into the corresponding SQL functions.
Example: Converting Degrees to Radians
In C#, you can now write:
var radiansQuery = context.WeatherForecasts
.Select(forecast => MathExtensions.DegreesToRadians(forecast.Degree));
This LINQ query would be translated by EF Core into a SQL query using the RADIANS function.
Improvements in SQLite Scaffolding and Data Type Handling
SQLite scaffolding in EF Core 8 has seen improvements. Previously, when reverse-engineering a SQLite database, the resultant entity types included only basic data types (long, double, string, byte[]). EF Core 8 uses additional information like data format and column type name to determine a more appropriate .NET type for the model.
Example: Improved Type Mapping
For a column defined in SQLite as BOOLEAN, EF Core 7 would map this to a byte[] in C#. However, EF Core 8 correctly maps it to a bool type in the model.
Sentinel Values and Database Defaults
EF Core 8 introduces enhancements in handling sentinel values and database defaults. This improvement makes it easier to work with default values in the database and understand when EF should send or not send a value for a column.
Example: Using Database Defaults
In C#, you can define a default value for a property like this:
modelBuilder.Entity<MyEntity>()
.Property(e => e.Status)
.HasDefaultValue("Active");
This tells EF Core to use the default value “Active” for the Status property when inserting new records, unless specified otherwise.
Advancements in EF Core 7
While focusing on the latest version, it is also essential to acknowledge the advancements made in EF Core 7, as they lay the foundation for the features in EF Core 8.
JSON Column Mapping
EF Core 7 introduced the ability to map JSON columns, bringing the capabilities of working with document databases into EF Core. This feature is especially useful for working with complex data types and hierarchical data structures.
Example: Mapping JSON Columns
In EF Core 7, you can map a complex type to a JSON column:
modelBuilder.Entity<Post>()
.OwnsOne(post => post.Metadata, ownedNavigationBuilder =>
{
ownedNavigationBuilder.ToJson();
});
This allows for storing and querying JSON data within a relational database using EF Core.
Aggregate Type Mapping for Complex Data Structures
EF Core 7 also enhanced its support for aggregate types containing nested types and collections. This feature is crucial for applications that deal with complex data models.
Example: Mapping Aggregate Types
modelBuilder.Entity<Post>().OwnsOne(
post => post.Metadata, ownedNavigationBuilder =>
{
ownedNavigationBuilder.ToJson();
ownedNavigationBuilder.OwnsMany(metadata => metadata.TopSearches);
});
This code snippet demonstrates how EF Core 7 can map complex aggregates with nested collections to JSON.
Raw SQL Queries and Performance in EF Core 8
Entity Framework Core 8 introduces significant enhancements in the area of raw SQL queries and performance, making it more versatile and efficient for developers.
Expanded Capabilities for Raw SQL Queries
In EF Core 8, raw SQL queries can now return any mappable CLR (Common Language Runtime) type, not just those included in the Entity Framework model. This feature expands the flexibility of EF Core, allowing developers to work with a broader range of data types and structures.
Example: Querying with Raw SQL
var result = context.Set<MyCustomType>()
.FromSqlRaw("SELECT Id, Name FROM MyTable")
.ToList();
This code snippet demonstrates how developers can execute a raw SQL query and map the results to a custom CLR type, even if it’s not part of the EF model.
Considerations for Lazy-Loading in Untracked Queries
EF Core 8 also added support for lazy-loading of navigation properties in un-tracked queries. However, it’s important to note that lazy-loading will only work until the DbContext used to execute the no-tracking query is disposed of.
Example: Lazy-Loading in Untracked Queries
var myEntity = context.MyEntities
.AsNoTracking()
.FirstOrDefault(e => e.Id == myEntityId);
// Navigation properties can be lazy-loaded here
In this code, navigation properties of myEntity can be lazy-loaded. However, once the DbContext is disposed, lazy-loading will no longer be available for this instance.
Native Support for DateOnly and TimeOnly Data Types
EF Core 8 brings native support for DateOnly and TimeOnly data types for SQL Server. This is a step forward from previous versions, where these types could only be used by installing a community NuGet package.
Example: Using DateOnly and TimeOnly Types
modelBuilder.Entity<MyEntity>()
.Property(e => e.MyDate)
.HasColumnType("date"); // Mapping to DateOnly type
modelBuilder.Entity<MyEntity>()
.Property(e => e.MyTime)
.HasColumnType("time"); // Mapping to TimeOnly type
This code snippet shows how to define properties in an entity that correspond to the DateOnly and TimeOnly types, which are now natively supported in EF Core 8.
EF Core in Cloud Native and Mobile Environments
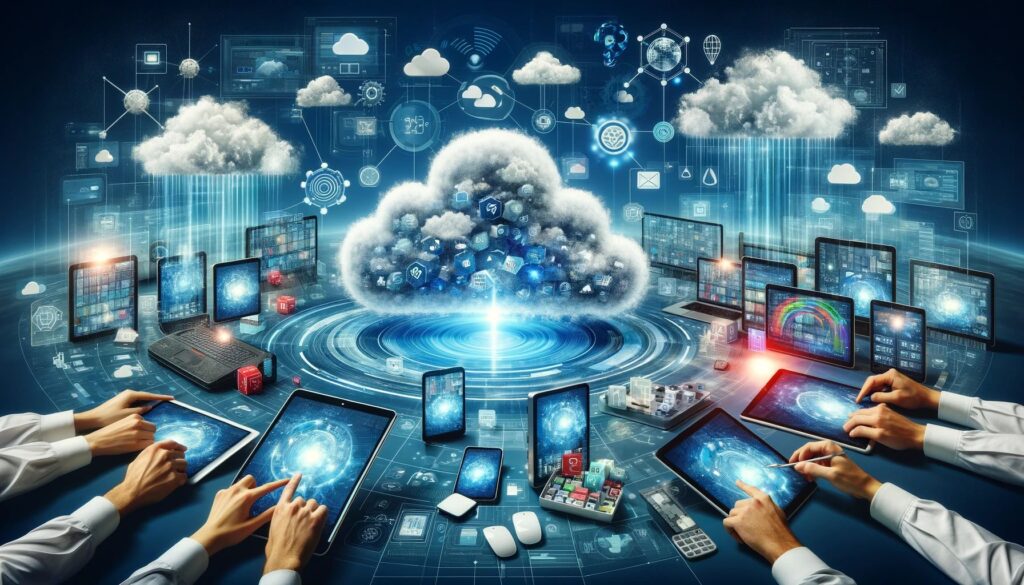
Entity Framework Core is increasingly being used in diverse environments, including cloud-native applications and on mobile devices. The latest versions, especially EF Core 8, have made significant strides in adapting to these varied environments.
Adapting EF Core for Microservices and Mobile Devices
Microservices and mobile applications often demand smaller application sizes, faster startup times, and minimal reliance on dynamic code generation. To accommodate these requirements, EF Core has been optimized for better performance and reduced overhead.
Example: Configuration for Microservices
When configuring EF Core for a microservice, you might opt for a lightweight DbContext and minimal tracking to enhance performance:
public class MyMicroserviceDbContext : DbContext
{
public MyMicroserviceDbContext(DbContextOptions<MyMicroserviceDbContext> options)
: base(options)
{ }
// Define DbSets for your entities
}
// In Startup.cs or Program.cs
services.AddDbContext<MyMicroserviceDbContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString("MyDbConnection"))
.UseQueryTrackingBehavior(QueryTrackingBehavior.NoTracking));
This configuration helps to reduce memory footprint and improve response times, essential for microservices.
Ahead-of-Time (AOT) Compilation and Trimming in EF Core
Ahead-of-time compilation and trimming are crucial for applications that need to start quickly and run with minimal resource consumption. EF Core’s enhancements in these areas make it more suitable for cloud-native and mobile environments.
Example: AOT Compilation with EF Core
AOT compilation involves pre-compiling the application to native code, which can significantly reduce startup times:
<Project Sdk="Microsoft.NET.Sdk">
<!-- Add AOT compilation settings -->
<PropertyGroup>
<PublishReadyToRun>true</PublishReadyToRun>
<PublishTrimmed>true</PublishTrimmed>
<PublishSingleFile>true</PublishSingleFile>
</PropertyGroup>
</Project>
This project file snippet demonstrates how to enable AOT compilation and trimming in a .NET application using EF Core.
Best Practices in EF Core Project Structure
Adopting best practices in structuring EF Core projects is crucial for maintaining clean, testable, and maintainable code. These practices include organizing the project into layers, ensuring security, and effectively managing database schema evolution.
Organizing Projects for Cleanliness and Testability
A well-structured project in EF Core should separate concerns and encapsulate Entity Framework specifics. This approach promotes cleanliness and facilitates easier testing.
Example: Layered Architecture in EF Core
A typical EF Core project might be divided into the following layers:
- Domain Layer: Contains business entities.
- Infrastructure Layer: Handles database configuration and EF Core specifics.
- Application Layer: Contains business logic and interacts with the infrastructure layer.
// Domain Project (Domain Layer)
public class MyEntity
{
// Entity properties and business logic
}
// Infrastructure Project (Infrastructure Layer)
public class MyDbContext : DbContext
{
public DbSet<MyEntity> MyEntities { get; set; }
// EF Core configurations
}
// Application Project (Application Layer)
public class MyService
{
private readonly MyDbContext _context;
public MyService(MyDbContext context)
{
_context = context;
}
// Business logic utilizing the DbContext
}
Security in Application Design
Incorporating security principles in EF Core applications involves practices like adhering to the principle of least privilege and safeguarding sensitive data.
Example: Secure DbContext Configuration
public class SecureDbContext : DbContext
{
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
// Use secure connection strings and settings
optionsBuilder.UseSqlServer("Your_Secure_Connection_String");
}
// Define DbSets and configurations
}
This snippet shows a DbContext configured with a secure connection string, which is a fundamental aspect of secure EF Core application design.
Schema Evolution with Migration Bundles and Docker
Automating schema evolution in EF Core using migration bundles and Docker can significantly streamline the process of database schema changes, especially in continuous integration/continuous deployment (CI/CD) pipelines.
Example: Using Migration Bundles
Migration bundles in EF Core allow bundling all migrations into a single executable, simplifying the deployment process.
// In the Package Manager Console
PM> Add-Migration InitialCreate
PM> dotnet ef migrations bundle
Querying and Performance Optimization
Effective querying and performance optimization are key areas where Entity Framework Core provides extensive support. Understanding how to efficiently query data and optimize performance is essential for building responsive and scalable applications.
Techniques for Efficient Querying in EF Core
EF Core offers various strategies to ensure efficient data querying. This includes understanding how to use eager, lazy, and explicit loading, as well as writing efficient LINQ queries.
Example: Eager Loading to Prevent N+1 Problem
Eager loading is a technique used to load related data alongside the main query, thus avoiding multiple roundtrips to the database, commonly known as the N+1 problem.
var blogs = context.Blogs.Include(b => b.Posts).ToList();
In this example, when blogs are queried, their related posts are also loaded in the same query, which is more efficient than lazy loading in scenarios where related data is needed immediately.
Avoiding Common Pitfalls like the N+1 Problem
The N+1 problem is a common pitfall in ORMs where an initial query is followed by N additional queries, one for each returned row. Being aware of this issue and knowing how to avoid it is crucial.
Example: Addressing the N+1 Problem with Projections
var blogsAndPosts = context.Blogs
.Select(b => new { Blog = b, Posts = b.Posts })
.ToList();
This code uses a projection to fetch both blogs and their posts in a single query, effectively avoiding the N+1 problem.
Implementing Eager and Explicit Loading Effectively
While eager loading is useful, it’s not always the best choice, especially for large datasets. Explicit loading can be a better alternative in such cases.
Example: Explicit Loading
var blog = context.Blogs.Single(b => b.Id == blogId);
context.Entry(blog).Collection(b => b.Posts).Load();
In this example, the posts related to a specific blog are loaded explicitly only when needed, which can be more efficient in certain scenarios.
Code-First Approach with EF Core in .NET 8
Adopting a code-first approach with Entity Framework Core in .NET 8 allows for a more agile development process, where you define your database schema using C# classes. This approach is particularly advantageous when you want your application logic to drive your database structure.
Implementing a Code-First Approach
In the code-first approach, you start by defining your entity classes and DbContext. EF Core then generates the database schema based on these classes.
Example: Defining Entity and DbContext
public class MyEntity
{
public int Id { get; set; }
public string Name { get; set; }
// Other properties
}
public class MyDbContext : DbContext
{
public DbSet<MyEntity> MyEntities { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder options)
{
options.UseSqlServer("Your_Connection_String");
}
}
In this example, MyEntity is an entity class, and MyDbContext is the EF Core context class with a DbSet property.
Registering DbContext and Managing Database Connections
Properly registering your DbContext in your application’s service container is crucial for effective data access.
Example: Registering DbContext in ASP.NET Core
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddDbContext<MyDbContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString("DefaultConnection")));
}
}
This code snippet demonstrates how to register MyDbContext in an ASP.NET Core application.
Building and Managing Services in a .NET 8 Environment
In a .NET 8 application, services and dependencies are typically configured in the Program.cs file, following the new minimal hosting model introduced in .NET 6.
Example: Service Configuration in .NET 8
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddDbContext<MyDbContext>(options =>
options.UseSqlServer(builder.Configuration.GetConnectionString("MyDatabase")));
builder.Services.AddScoped<IMyService, MyService>();
var app = builder.Build();
// Configure the HTTP request pipeline and endpoints
app.MapControllers();
app.Run();
In this example, MyDbContext and a service IMyService are registered in the DI container, and the application is configured and run.
Conclusion
Entity Framework Core (EF Core) has become an essential tool in the .NET ecosystem, especially in recent versions like EF Core 8. These updates emphasize performance, flexibility, and ease of use, with enhanced SQL translations, support for new data types, and improved querying and optimization.
EF Core’s adaptability shines in handling complex data structures, optimizing queries, and embracing a code-first approach. It fits well in various scenarios, including cloud-native apps, microservices, and traditional web apps, making it a versatile choice for data management challenges.