Java, a stalwart in the programming world, has carved its niche in various domains, including desktop application development. The language’s platform-independent nature, robust security features, and rich library ecosystem make it a preferred choice for developers. A significant milestone in Java’s journey in desktop applications is the evolution and adoption of JavaFX, a cutting-edge toolkit designed for building rich internet applications with immersive user interfaces.
The Role of Java in Desktop Application Development
Java’s history in desktop application development is marked by its consistent adaptability and evolution. Initially, Java applets brought Java to web browsers, demonstrating the language’s cross-platform capabilities. However, as technology progressed, the need for more sophisticated desktop applications arose, and Java answered this call with Swing, an integral part of its foundation. But Java didn’t stop there. It continued to evolve, addressing the needs of modern desktop applications, leading to the creation of JavaFX.
The Emergence and Impact of JavaFX
JavaFX represents a leap forward in Java’s capabilities for desktop application development. Launched as a successor to Swing, JavaFX offers a plethora of modern UI components, a dedicated scene builder, and extensive support for multimedia and graphical content. Unlike its predecessor, JavaFX was built with the intention of simplifying the design and deployment of modern, feature-rich desktop applications.
JavaFX has significantly influenced the Java community by:
- Enabling Modern UI Design: JavaFX comes with an array of built-in UI components and supports CSS styling, which allows for the creation of aesthetically pleasing and functional user interfaces.
- Facilitating Cross-Platform Development: True to Java’s philosophy, JavaFX applications can run seamlessly on various platforms, including Windows, macOS, and Linux, without the need for platform-specific modifications.
- Enhancing Developer Productivity: With tools like the JavaFX Scene Builder, developers can design interfaces visually, reducing the time and complexity involved in UI development.
- Supporting Multimedia and Graphics: JavaFX has robust support for graphics and multimedia, making it an ideal choice for applications that require rich content and interactive features.
- Integration with Other Java Technologies: JavaFX can be seamlessly integrated with other Java libraries and frameworks, making it a versatile tool in a developer’s toolkit.
Why JavaFX? Advantages Over Other Frameworks
JavaFX stands out in the Java ecosystem as a superior framework for building modern desktop applications. It offers several advantages over its predecessor, Swing, and other Java UI frameworks, which we will explore in this section.
Rich User Interface Components
JavaFX comes equipped with a wide array of built-in UI components that are not only advanced but also customizable. These components range from basic elements like buttons and text fields to more complex ones like charts and tables. The added benefit of CSS styling allows developers to create visually appealing interfaces with ease. For example, customizing a button in JavaFX can be as simple as:
Button button = new Button("Click Me");
button.setStyle("-fx-background-color: #FF5733; -fx-text-fill: white;");
This snippet demonstrates how straightforward it is to style a button with a specific background color and text color using CSS in JavaFX.
Enhanced Graphics and Multimedia Capabilities
JavaFX provides extensive support for graphics and multimedia, which is a significant leap from Swing. It includes features for 2D and 3D graphics, allowing for the creation of visually rich and interactive applications. The framework also supports audio and video, making it a go-to choice for applications that require multimedia integration.
Scene Builder: Streamlining UI Development
One of the most notable tools in JavaFX is the Scene Builder, a visual layout tool that allows developers to design their UIs without writing extensive code. It significantly speeds up the development process and makes UI design more accessible, especially for those who are more visually inclined.
FXML: Separating UI and Logic
JavaFX utilizes FXML, an XML-based language, for defining user interfaces. This separation of UI and logic makes the code more maintainable and readable. For example, defining a simple layout in FXML might look like:
<VBox xmlns:fx="http://javafx.com/fxml">
<Button text="Click Me" />
<Label text="Welcome to JavaFX!" />
</VBox>
This code snippet shows a VBox layout with a button and a label, defined cleanly in FXML.
Animation and Special Effects
JavaFX’s animation framework is powerful and straightforward to use, allowing for the creation of smooth transitions and dynamic UI elements. Special effects like drop shadows, blurs, and reflections can be easily implemented to enhance the visual appeal of applications.
Cross-Platform Consistency
JavaFX applications maintain a consistent look and feel across different platforms, adhering to Java’s write once, run anywhere (WORA) philosophy. This cross-platform consistency is crucial for applications that are deployed on various operating systems.
Community and Future-Proofing
With the backing of the Java community and continuous enhancements, JavaFX is well-positioned for future developments in desktop application technology. Its integration with Java ensures it stays up-to-date with the latest Java features and best practices.
Designing with JavaFX: Tools and Techniques
JavaFX provides a comprehensive set of tools and techniques that streamline the process of designing and developing desktop applications. This section delves into the key aspects that make JavaFX a preferred choice for developers seeking to create both functional and visually appealing user interfaces.
Leveraging Scene Builder for Rapid UI Design
The JavaFX Scene Builder is an invaluable tool for developers, enabling the rapid construction of UIs without writing extensive code. It’s a drag-and-drop interface that lets you visually design the UI and generates FXML markup, which can be integrated with your Java code. For instance, creating a layout with buttons, labels, and text fields is intuitive in Scene Builder, and it generates FXML similar to:
<AnchorPane>
<Button layoutX="50" layoutY="50" text="Submit"/>
<TextField layoutX="50" layoutY="100"/>
<Label layoutX="50" layoutY="150" text="Enter your name:"/>
</AnchorPane>
CSS Styling in JavaFX
JavaFX’s support for CSS styling offers a familiar approach for web developers and provides immense flexibility in customizing UI components. You can create a separate CSS file and apply styles to your JavaFX components, giving you the power to design sophisticated and attractive interfaces. For example, styling a label might look like this:
Label label = new Label("Styled Label");
label.getStyleClass().add("myLabelStyle");
And in your CSS file:
.myLabelStyle {
-fx-font-size: 16px;
-fx-font-weight: bold;
-fx-text-fill: #2a2a2a;
}
Animation and Special Effects
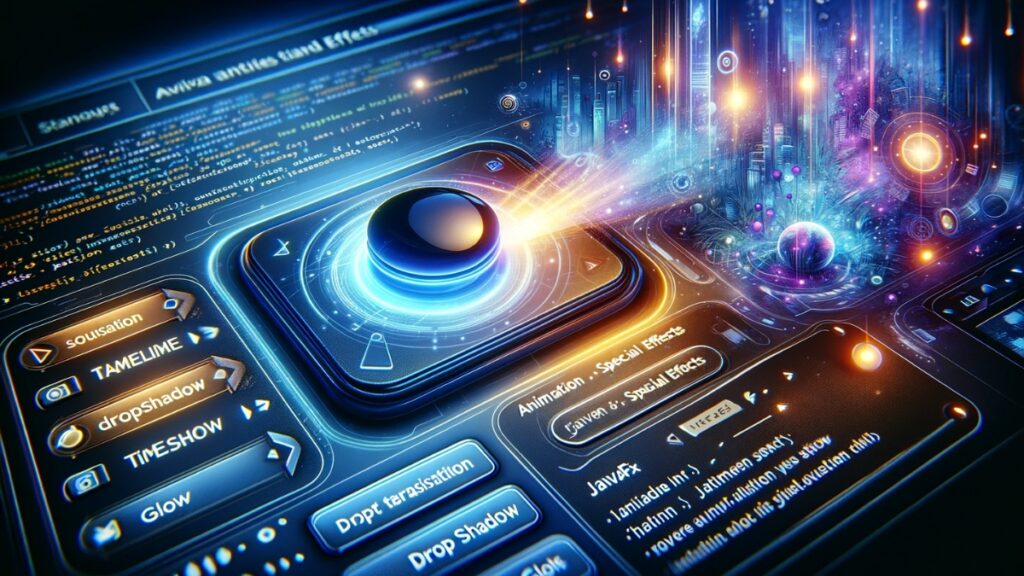
JavaFX simplifies the creation of animations and the application of special effects, enhancing the user experience. The framework provides classes such as Timeline
and Transition
for creating animations, and effects like DropShadow
and Glow
for adding visual flair. Here’s a simple example of a fade transition on a button:
FadeTransition fade = new FadeTransition(Duration.seconds(3), button);
fade.setFromValue(1.0);
fade.setToValue(0.0);
fade.play();
Event Handling and Data Binding
JavaFX’s event handling and data binding mechanisms are robust and easy to implement. They enable responsive applications that can react to user inputs and changes in data. Binding a property, for instance, allows automatic updates between the UI and the data model. For example, binding a slider’s value to a label:
Slider slider = new Slider();
Label label = new Label();
label.textProperty().bind(slider.valueProperty().asString());
Integrating Multimedia
JavaFX makes integrating multimedia elements like images, audio, and video straightforward. The MediaPlayer
and MediaView
classes, for example, are used to add and control video playback in a JavaFX application.
Advanced Graphics with Canvas and 3D
For more advanced graphical requirements, JavaFX offers the Canvas
API for drawing directly within an application and supports 3D graphics. This capability allows developers to create complex visualizations and user interfaces that were not possible with older Java UI frameworks.
Canvas canvas = new Canvas(150, 150);
GraphicsContext gc = canvas.getGraphicsContext2D();
gc.setFill(Color.RED);
gc.fillRect(10, 10, 130, 130);
This code snippet demonstrates how to create a simple canvas and draw a red square on it.
JavaFX and Cross-Platform Development
JavaFX excels in creating applications that are truly cross-platform. This means that an application developed with JavaFX can run on multiple operating systems like Windows, macOS, and Linux without any need for platform-specific modifications. This cross-platform capability is inherent in Java’s design philosophy and is brilliantly extended by JavaFX.
Ensuring Compatibility Across Platforms
JavaFX’s ability to maintain a consistent look and feel across different platforms is one of its most significant advantages. This consistency is achieved through the use of Java’s virtual machine (JVM), which abstracts the underlying operating system differences. Here’s a basic example of a JavaFX application that can run on any platform:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.stage.Stage;
public class CrossPlatformApp extends Application {
@Override
public void start(Stage primaryStage) {
Label label = new Label("Welcome to JavaFX!");
Scene scene = new Scene(label, 200, 100);
primaryStage.setTitle("Cross-Platform Application");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
This code creates a simple JavaFX application with a label. The application will look and function the same way, regardless of the operating system.
Handling Platform-Specific Nuances
While JavaFX applications are inherently cross-platform, there may be scenarios where you need to handle certain platform-specific nuances. JavaFX provides the capability to detect the operating system at runtime, allowing developers to make adjustments if necessary. For example:
String os = System.getProperty("os.name").toLowerCase();
if (os.contains("win")) {
// Windows-specific code
} else if (os.contains("mac")) {
// macOS-specific code
} else if (os.contains("nix") || os.contains("nux")) {
// Linux/Unix-specific code
}
This snippet demonstrates how to detect the operating system and execute platform-specific code, if needed.
Leveraging JavaFX’s Cross-Platform Libraries
JavaFX’s library of UI components is designed to work across all major platforms. This means that developers can focus on building the functionality of their applications without worrying about compatibility issues. The standardization of components ensures that they behave consistently, regardless of the platform on which the application is running.
Real-World Example: Cross-Platform Application
Consider a real-world scenario where a JavaFX application is used for data visualization. The application can pull data from a server and display it in charts and graphs. This application would be able to run on a Windows machine in an office, a Mac used by a remote worker, or a Linux system in a data center, all without any change to the codebase.
Integrating JavaFX with Other Java Technologies
JavaFX’s integration with other Java technologies is a key factor in its versatility and effectiveness for desktop application development. This integration allows developers to leverage the vast ecosystem of Java libraries and frameworks, enhancing the capabilities of JavaFX applications.
Utilizing Java Libraries and Frameworks
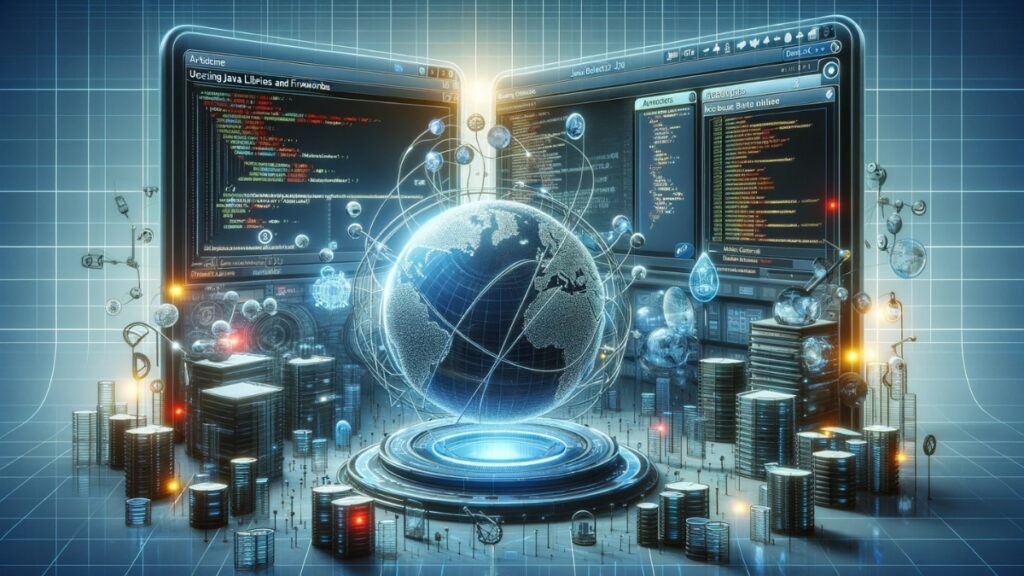
JavaFX applications can seamlessly incorporate standard Java libraries and frameworks, enabling a wide range of functionalities from database connectivity to network communication. For example, integrating JavaFX with JDBC (Java Database Connectivity) allows for robust database operations. Here’s a simple example of connecting to a database in a JavaFX application:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnector {
public Connection connect() {
Connection conn = null;
try {
// Database URL and credentials
String url = "jdbc:postgresql://localhost/testdb";
String user = "username";
String password = "password";
conn = DriverManager.getConnection(url, user, password);
System.out.println("Connected to the database successfully.");
} catch (SQLException e) {
System.out.println(e.getMessage());
}
return conn;
}
}
This code establishes a connection to a PostgreSQL database, which can then be used within a JavaFX application to retrieve or store data.
Leveraging JavaFX in Enterprise Applications
JavaFX can be integrated into Java EE (Java Enterprise Edition) environments, making it suitable for creating client-side interfaces in larger, distributed applications. This integration allows JavaFX applications to interact with Java EE backend services, such as EJB (Enterprise JavaBeans) and JPA (Java Persistence API).
Combining JavaFX with Spring Framework
The Spring Framework, widely used for building enterprise applications, can also be integrated with JavaFX. This combination allows developers to leverage the dependency injection and aspect-oriented programming features of Spring, enhancing the structure and maintainability of JavaFX applications. A simple example of this integration can be seen in setting up a Spring context in a JavaFX application:
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class SpringJavaFXIntegration {
private static ApplicationContext context;
public static void main(String[] args) {
context = new ClassPathXmlApplicationContext("applicationContext.xml");
// Launch JavaFX application
Application.launch(JavaFXApp.class, args);
}
public static ApplicationContext getApplicationContext() {
return context;
}
}
In this example, a Spring application context is created and can be used within a JavaFX application.
JavaFX and Web Integration
JavaFX also provides support for integrating web content into desktop applications. The WebView
and WebEngine
classes in JavaFX allow embedding web pages within a JavaFX application, creating hybrid applications that combine web and desktop technologies.
import javafx.scene.Scene;
import javafx.scene.web.WebView;
import javafx.stage.Stage;
public class WebIntegrationExample extends Application {
@Override
public void start(Stage primaryStage) {
WebView webView = new WebView();
webView.getEngine().load("https://www.example.com");
primaryStage.setScene(new Scene(webView, 800, 600));
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
This code demonstrates embedding a web page inside a JavaFX application using WebView
.
JavaFX for High-Performance Applications
Optimizing performance is crucial in desktop application development, and JavaFX provides various features and best practices to help developers create efficient and responsive applications.
Efficient Use of System Resources
JavaFX applications can be optimized to use system resources more effectively, improving performance, especially on devices with limited capabilities. For instance, using property bindings and observable collections can help in reducing unnecessary computations:
ListView<String> listView = new ListView<>();
ObservableList<String> items = FXCollections.observableArrayList("Item 1", "Item 2", "Item 3");
listView.setItems(items);
This code demonstrates the use of an ObservableList
to update a ListView
in JavaFX, which is more efficient than manually updating the list view when the data changes.
Performance Tuning with JavaFX Canvas and Animation
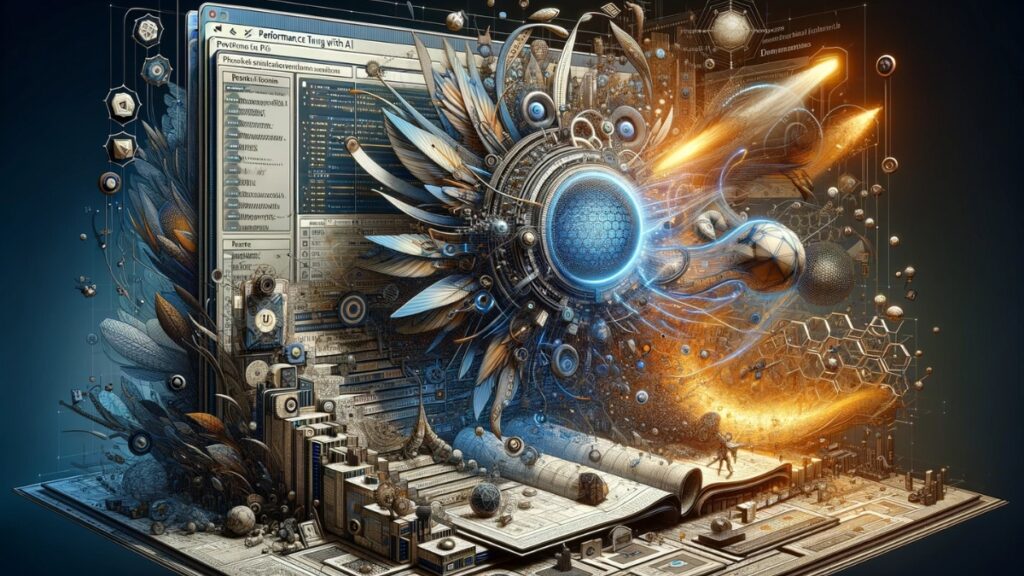
For applications requiring extensive graphical rendering or animations, JavaFX’s Canvas
API can be used for more fine-grained control over rendering, which can lead to performance improvements. Managing the animation timeline and reducing the frame rate for less critical animations can also optimize performance.
Canvas canvas = new Canvas(300, 300);
GraphicsContext gc = canvas.getGraphicsContext2D();
gc.setFill(Color.BLUE);
gc.fillRect(10, 10, 280, 280);
// Add canvas to the scene
In this example, a Canvas
is used for custom drawing, which can be more efficient than using multiple individual JavaFX nodes for complex scenes.
Leveraging Multi-Threading
JavaFX applications can take advantage of Java’s multi-threading capabilities to perform resource-intensive tasks in the background, ensuring that the UI remains responsive. The Platform.runLater()
method can be used to update UI components safely from other threads.
new Thread(() -> {
// Perform long-running task...
String result = "Task completed";
Platform.runLater(() -> {
// Update UI with result
label.setText(result);
});
}).start();
This snippet demonstrates running a long-running task in a separate thread and then updating the UI with the result.
Garbage Collection and Memory Management
Proper memory management is critical for the performance of JavaFX applications. Developers should be mindful of object creation and disposal, and leverage Java’s garbage collection effectively to avoid memory leaks.
Profiling and Optimization Tools
JavaFX developers can use various profiling and optimization tools to identify performance bottlenecks and optimize their code. Tools like VisualVM, JProfiler, or Java Mission Control can provide insights into memory usage, CPU utilization, and other performance metrics.
Security Considerations in JavaFX Desktop Applications
Security is a paramount concern in application development, and JavaFX, built on the robust Java platform, offers various features to ensure the security of desktop applications. However, developers must also implement best practices to safeguard against potential vulnerabilities.
Leveraging Java’s Built-in Security Features
Java’s security model is designed to protect against various types of attacks, and these features extend to JavaFX applications. For example, the use of the Java Security Manager can help in controlling what resources an application can access:
System.setSecurityManager(new SecurityManager());
Setting a security manager helps in enforcing a security policy, controlling the operations that can be performed by different parts of the application.
Secure Communication and Data Handling
For applications that communicate over the network or handle sensitive data, implementing secure communication protocols such as HTTPS and using encryption for data storage and transmission is crucial.
URL url = new URL("https://example.com/api/data");
HttpsURLConnection conn = (HttpsURLConnection) url.openConnection();
// Configure HTTPS connection settings
In this snippet, a secure HTTPS connection is established to communicate with a web service.
Input Validation and Sanitization
Proper validation and sanitization of user inputs are essential to prevent common vulnerabilities like SQL injection and cross-site scripting (XSS). JavaFX applications should validate all inputs, especially when interacting with databases or executing system commands.
Regular Updates and Dependency Management
Keeping the Java runtime environment and all dependencies up to date is vital for security. Regular updates ensure that security patches are applied, protecting the application from known vulnerabilities.
Security Testing and Auditing
Performing regular security audits and testing, including static code analysis and penetration testing, can help in identifying and mitigating potential security issues in JavaFX applications.
Conclusion: The Power and Versatility of JavaFX for Desktop Applications
JavaFX stands as a powerful and versatile framework for desktop application development, blending the robustness of the Java platform with a rich set of features. It enables the creation of visually appealing, responsive user interfaces, and ensures cross-platform compatibility, adhering to Java’s write once, run anywhere philosophy. With its efficient resource management, integration with the broader Java ecosystem, and strong focus on security, JavaFX empowers developers to build high-performance, feature-rich applications. As the technology landscape evolves, JavaFX continues to adapt, offering tools and flexibility for innovative and high-quality desktop application development, making it an ideal choice for a wide range of applications, from simple tools to complex enterprise software.