Introduction to Data Structures and Algorithms in C++
C++ stands as a cornerstone in the world of programming, renowned for its efficiency and control over system resources. At the heart of this powerful language lies the implementation of data structures and algorithms, which are critical for developing robust and efficient software solutions.
The Role of C++ in Data Structures and Algorithms
C++ is a language that blends the high-level functionality of its predecessors with the low-level control of memory management. This unique combination makes it an ideal choice for implementing data structures and algorithms. The language’s features, such as classes and templates, provide the necessary tools to create complex data structures while ensuring code reusability and maintainability.
Data structures in C++ are ways of organizing and storing data so that they can be accessed and modified efficiently. They are the backbone of creating efficient algorithms, which are sets of instructions or steps to solve specific problems. The effectiveness of these algorithms heavily relies on how data is structured.
Importance in Software Development and Problem Solving
In software development, choosing the right data structure and algorithm can drastically impact the performance and scalability of an application. For instance, a well-chosen data structure can reduce the complexity of a task, leading to faster execution times and reduced memory usage.
Let’s consider a simple example: searching for an element in a list. If the list is unsorted, a linear search might be the only solution, which has a complexity of O(n), where ‘n’ is the number of elements. However, if the list is sorted, a binary search can be used, reducing the complexity to O(log n), a significant improvement for large datasets.
C++ and Modern Software Challenges
In the realm of modern computing, where data is vast and computing efficiency is paramount, C++ shines. Its ability to handle complex data structures and algorithms makes it a go-to language for high-performance applications like game development, real-time systems, and scientific computing. Moreover, C++’s continuous evolution, with the introduction of modern features in its recent versions, keeps it relevant in addressing contemporary software challenges.
Fundamentals of Data Structures in C++
Data structures are fundamental to programming in C++, providing the framework for storing and organizing data efficiently. In this part of our journey, we explore some of the basic yet crucial data structures: arrays, linked lists, stacks, and queues. Each of these structures serves a unique purpose, and understanding their implementation in C++ is key to developing efficient software.
Arrays: The Building Block
An array is one of the simplest and most widely used data structures in C++. It is a collection of elements, all of the same type, stored in contiguous memory locations. Arrays are particularly useful when you need to access elements by their index quickly.
In C++, arrays can be declared as follows:
int myArray[10]; // Declares an array of 10 integers
The primary advantage of arrays is their fast access time. However, this comes with a limitation – their size is fixed and needs to be known at compile time.
Linked Lists: Dynamic Data Handling
Linked lists are a step up from arrays in terms of flexibility. A linked list is a collection of nodes, where each node contains data and a pointer to the next node in the sequence. This structure allows for efficient insertion and deletion of elements without reallocating or reorganizing the entire structure.
In C++, a basic linked list node can be represented as:
struct Node {
int data;
Node* next;
};
Linked lists are ideal for situations where the size of the data set is unknown or changes frequently.
Stacks: Last-In, First-Out
Stacks are a type of container adaptor with a LIFO (Last In, First Out) structure. This means that the last element added to the stack is the first one to be removed. Stacks are useful in scenarios where you need to reverse items or parse expressions (like in compiler algorithms).
A simple stack implementation in C++ might look like this:
#include <stack>
std::stack<int> myStack; // Declaring a stack of integers
Queues: First-In, First-Out
Opposite to stacks, queues are a FIFO (First In, First Out) data structure. Elements are added to the back and removed from the front. Queues are essential in scenarios like task scheduling, where the order of tasks matters.
A queue in C++ can be implemented as follows:
#include <queue>
std::queue<int> myQueue; // Declaring a queue of integers
Advanced Data Structures in C++
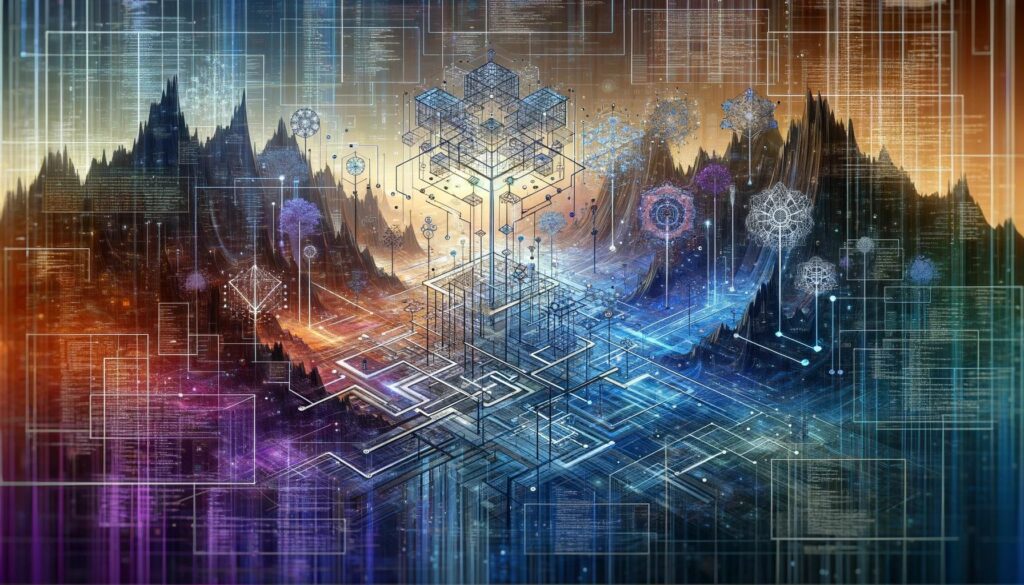
After exploring the basics, it’s time to delve into more advanced data structures in C++. These structures, including trees, graphs, and hash tables, are pivotal for handling complex data and solving intricate problems in computer science. Their implementation in C++ allows developers to tackle a broader range of challenges with greater efficiency.
Trees: Hierarchical Data Representation
Trees are non-linear data structures that simulate a hierarchical tree structure, with a root value and subtrees of children, represented as nodes. They are crucial in scenarios where data naturally forms a hierarchy, such as file systems or organizational structures.
Types of Trees in C++
- Binary Trees: Every node has at most two children.
- Binary Search Trees (BST): A binary tree where the left child contains only nodes with values less than the parent, and the right child only values greater.
- AVL Trees: A self-balancing BST where the difference between heights of left and right subtrees cannot be more than one for all nodes.
Here is a simple BST node structure in C++:
struct TreeNode {
int value;
TreeNode *left, *right;
TreeNode(int x) : value(x), left(nullptr), right(nullptr) {}
};
Graphs: Interconnected Data
Graphs are a step beyond trees in complexity. They consist of a set of nodes (or vertices) connected by edges. Graphs are incredibly useful in representing and solving problems involving interconnected data, like social networks, network topologies, and routing algorithms.
Representing Graphs in C++
Graphs can be represented in C++ using:
- Adjacency Matrix: A 2D array where the element at matrix[i][j] is 1 if there is an edge from node i to node j.
- Adjacency List: A list of lists, where each list represents a node and contains a list of all the nodes connected to it.
Hash Tables: Efficient Data Retrieval
Hash tables are used for storing key-value pairs. They provide efficient retrieval through a unique key. Underlying this efficiency is a hash function that converts keys into indexes of an array.
In C++, hash tables can be implemented using the unordered_map container, which utilizes a hash table internally.
#include <unordered_map>
std::unordered_map<std::string, int> hashMap;
Basic Algorithms and Their Implementation in C++
In this exploration of fundamental algorithms, we dive into the core of computational efficiency and problem-solving in C++.
Sorting Algorithms
Sorting, a fundamental operation in programming, is often a preliminary step in complex algorithms. We focus on three popular sorting algorithms in C++: Bubble Sort, Merge Sort, and Quick Sort. Bubble Sort is a simple algorithm that compares and swaps adjacent elements if they are in the wrong order. It’s easy to implement and suitable for small datasets. Merge Sort is a more efficient, stable, and comparison-based algorithm, ideal for large lists. It divides the list into halves, sorts them, and then merges them. Quick Sort, known for its efficiency, places elements of an array in order systematically. While it’s faster on large datasets, its worst-case scenario needs consideration.
Search Algorithms
Efficient search algorithms are crucial for performance, especially in applications handling large data volumes. Binary Search, an effective method in sorted arrays, locates the target value by comparing it to the middle element of the array. Its efficiency is marked by its O(log n) time complexity. Depth-First Search (DFS) and Breadth-First Search (BFS) are essential for traversing or searching tree or graph data structures. DFS explores as far as possible along each branch before backtracking, useful in puzzle solving and topological sorting. BFS, on the other hand, explores all neighbor nodes at the current depth before moving to the next level, often used in finding the shortest path in unweighted graphs and level order tree traversal. Both have a time complexity of O(V + E) for graphs.
Algorithm Optimization Techniques
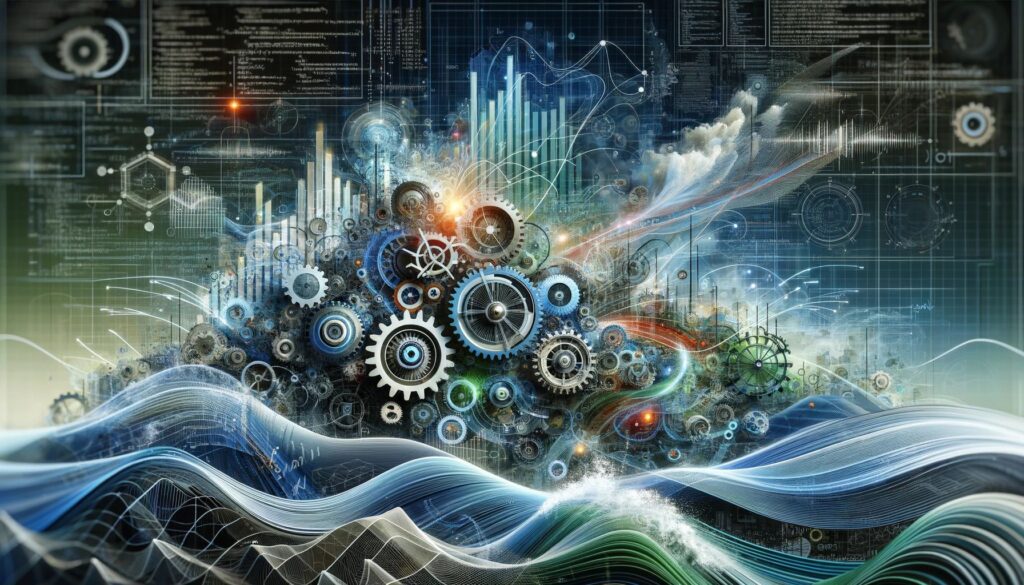
Optimization is a crucial aspect of algorithm design and implementation in C++. It involves enhancing the algorithm to improve its efficiency, typically in terms of time and space complexity. Understanding and applying optimization techniques is key to developing high-performance applications in C++.
Time Complexity Analysis
Time complexity is a measure of the amount of time an algorithm takes to complete as a function of the length of the input. It’s commonly expressed using Big O notation, which provides an upper limit on the time for an algorithm in the worst-case scenario.
For Example:
- A linear search algorithm has a time complexity of O(n), where ‘n’ is the number of elements. It implies that in the worst case, the algorithm might check each element once.
- A more efficient binary search algorithm, on the other hand, has a time complexity of O(log n), indicating a significant reduction in time, especially for large datasets.
Space Complexity Analysis
Space complexity refers to the amount of memory space required by an algorithm to run. Like time complexity, it’s essential to minimize space usage, especially in memory-constrained environments or when dealing with large data sets.
For Instance:
- An iterative implementation of an algorithm might have a lower space complexity than a recursive implementation, as recursion often requires additional stack space for each recursive call.
Tips for Optimizing C++ Code
- Choose the Right Data Structures: Selecting the appropriate data structure can significantly impact the performance of an algorithm. For example, using hash tables for frequent search operations can be more efficient than a list or an array.
- Avoid Unnecessary Computations: Reusing results of calculations that don’t change within a loop or avoiding redundant calculations can save time.
- Efficient Memory Management: Utilizing smart pointers and being mindful of memory allocation and deallocation can help in managing memory more efficiently in C++.
- Loop Unrolling: This technique involves expanding a loop to reduce the loop control overhead, which can lead to performance improvements in certain scenarios.
- Use Recursion Judiciously: While recursion can simplify code, it can also lead to increased space complexity and stack overflow issues for deep recursive calls. Iterative solutions might be more efficient in some cases.
- Compiler Optimizations: Modern C++ compilers come with optimization flags that can significantly enhance performance. Understanding and utilizing these can yield substantial benefits.
Best Practices and Common Pitfalls in Implementing Data Structures and Algorithms in C++
Navigating through the intricacies of C++ programming requires an awareness of both the best practices to follow and the common pitfalls to avoid.
Coding Standards and Style Guides
In C++, adhering to coding standards and style guides is imperative. These standards ensure readability, maintainability, and consistency, which are particularly crucial in collaborative coding environments. Employing consistent naming conventions like camelCase for variables and functions, and PascalCase for class names, aids in clarity. Proper code formatting, including consistent indentation and bracket styles, enhances readability. Furthermore, commenting and thorough documentation are essential for conveying the purpose and functionality of different segments of the code.
Common Errors to Avoid
When implementing data structures and algorithms in C++, several common pitfalls should be vigilantly avoided. Memory management is a prime area of concern, with memory leaks arising from neglected delete or delete[] operations after allocating dynamic memory. Dangling pointers, resulting from deleted or deallocated objects without resetting the pointer to nullptr, can lead to undefined behavior. The choice of data structures is another critical area; improper use or selecting an inappropriate structure for the problem at hand can lead to inefficient solutions. Simplifying solutions rather than overcomplicating them with unnecessary complexity is advisable. Additionally, a thorough consideration of edge cases in algorithm implementation is essential to prevent unexpected behavior or crashes. Lastly, overlooking the analysis of time and space complexity of algorithms can result in non-scalable and inefficient solutions.
Conclusion
In summary, the journey through data structures and algorithms in C++ is fundamental to effective software development. From basic structures like arrays to complex ones like graphs, and from simple sorting algorithms to more intricate searching techniques, each element plays a vital role in efficient problem-solving. Understanding these concepts is essential, but equally important is applying best practices and avoiding common pitfalls to ensure robust and maintainable code.
This exploration not only enhances technical skills but also fosters a deeper understanding of computational thinking. For students, programmers, and professionals alike, mastering these aspects of C++ is a stepping stone towards creating more sophisticated and efficient software solutions. As the field of technology continues to evolve, the principles outlined in this guide will remain a valuable resource for navigating the challenges of modern software development.