Silverlight, a powerful development tool from Microsoft, has significantly evolved over the years, especially in its animation capabilities. This section will delve into the depths of Silverlight’s animation features, illustrating how they have transformed the way developers create rich, interactive web applications.
The Capabilities of Silverlight in Animation
Silverlight stands out for its robust animation framework, which is a part of its broader offering as a multimedia and application development platform. At its core, Silverlight provides a rich set of animation features that enable developers to create complex, high-quality animations for web applications. These features include, but are not limited to:
- Storyboard Animations: Silverlight’s Storyboard functionality allows for the sequencing and control of animations. Storyboards can orchestrate the timing, duration, and interaction of multiple animations for a cohesive visual experience.
- Keyframe Animations: These animations offer precise control over the interpolation of values between frames, enabling the creation of complex and nuanced animations.
- Visual State Management: This allows for animations to be linked to the state of user interface elements, enhancing interactivity and user engagement.
Evolution of Animation Features in Silverlight
Silverlight has undergone several iterations, each bringing enhancements and new capabilities to its animation toolbox:
- Early Versions (Silverlight 1 and 2): Focused on basic animations, transitions, and interactivity, laying the groundwork for more advanced features.
- Silverlight 3 and Beyond: Introduced advanced concepts like easing functions for smoother transitions, 3D transformations, and enhanced control over animation timelines.
The advancements in Silverlight animations have been instrumental in enabling developers to create dynamic, visually appealing, and interactive web applications. These improvements not only enhanced the visual aspect of web applications but also contributed significantly to user experience and engagement.
Storyboard Fundamentals and Techniques in Silverlight
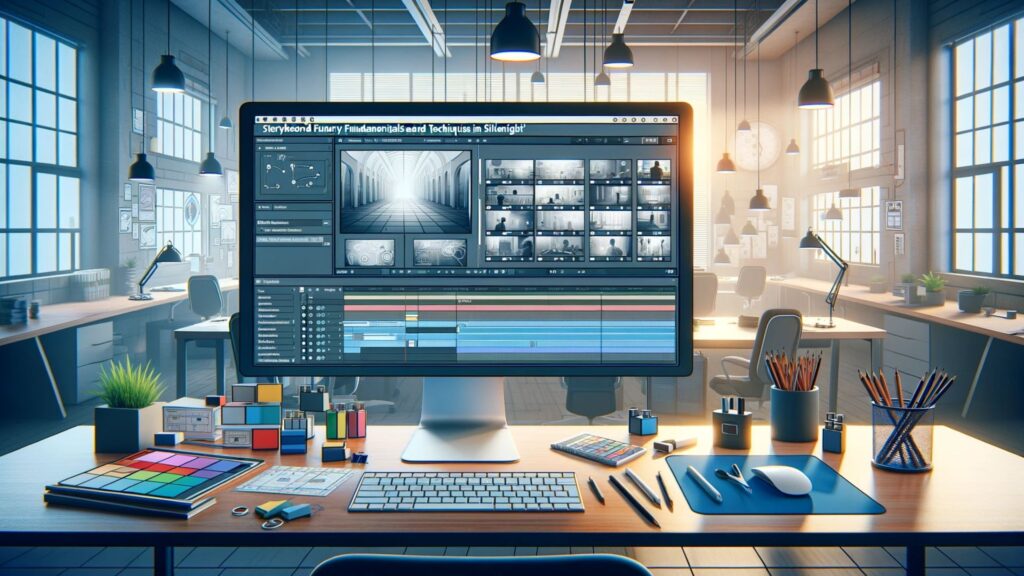
Understanding Storyboard in Silverlight
A Storyboard in Silverlight is a powerful tool for creating animations. It acts as a container for animations, managing the timing and execution of multiple animation sequences. Using Storyboards, developers can animate properties of Silverlight elements, such as opacity, position, color, and more.
Creating a Basic Storyboard Animation
To create a basic Storyboard animation in Silverlight, the following steps are involved:
1. Define the Storyboard: The Storyboard is defined within the Resources section of a Silverlight page. This includes setting up the timeline and linking it to the UI elements.
<UserControl.Resources>
<Storyboard x:Name="MyStoryboard">
<!-- Animation details go here -->
</Storyboard>
</UserControl.Resources>
2. Setting Up Animation Details: Within the Storyboard, developers define the type of animation (e.g., DoubleAnimation, ColorAnimation) and specify the target property to animate.
<DoubleAnimation Storyboard.TargetName="animatedElement"
Storyboard.TargetProperty="Opacity"
From="0.0" To="1.0" Duration="0:0:5"/>
This example animates the Opacity property of an element named “animatedElement” from 0.0 (fully transparent) to 1.0 (fully opaque) over a duration of 5 seconds.
3. Triggering the Animation: The animation can be triggered through an event (like a button click) or automatically on page load.
<Button Content="Start Animation" Click="StartAnimation"/
private void StartAnimation(object sender, RoutedEventArgs e)
{
MyStoryboard.Begin();
}
Advanced Techniques in Storyboard Animation
For more sophisticated animations, Silverlight offers several advanced techniques:
- KeyFrame Animations: Instead of a smooth transition between two values, keyframes allow for more control over the intermediate steps of an animation.
- Easing Functions: These functions help in creating more natural and realistic animations by modifying the speed of the animation over time.
- Controlling the Playback: Developers can control the playback of animations programmatically, including starting, pausing, resuming, and stopping animations.
Practical Example: Animating a UI Element
Consider a scenario where a UI element needs to slide in from the left and fade in simultaneously. This can be achieved using a Storyboard that contains two animations: one for the TranslateTransform.X property (for horizontal movement) and another for the Opacity property.
<Storyboard x:Name="SlideAndFadeInStoryboard">
<DoubleAnimation Storyboard.TargetName="myElementTransform"
Storyboard.TargetProperty="X"
From="-200" To="0" Duration="0:0:2"/>
<DoubleAnimation Storyboard.TargetName="myElement"
Storyboard.TargetProperty="Opacity"
From="0.0" To="1.0" Duration="0:0:2"/>
</Storyboard>
In this example, myElementTransform is the TranslateTransform applied to the element, and myElement is the UI element being animated. Both animations are synchronized to run over 2 seconds, creating a smooth slide and fade-in effect.
Keyframe-Based Animations for Dynamic Effects
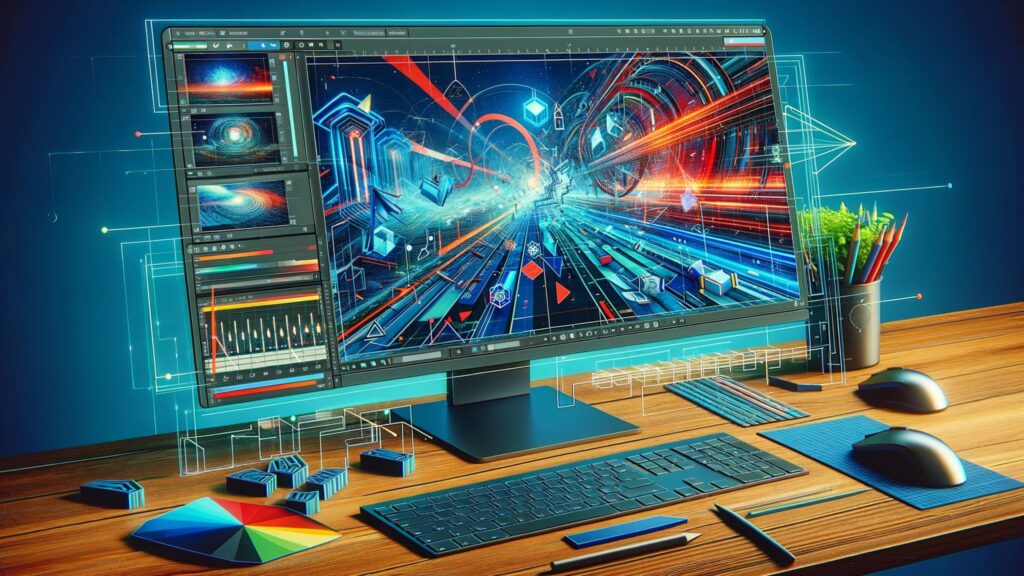
Keyframe-based animations in Silverlight offer a sophisticated approach to animating properties. Unlike basic animations that transition smoothly from a starting to an ending value, keyframe animations provide control over the intermediate values at specific times during the animation, allowing for more complex and dynamic effects.
Understanding Keyframe Animations
Keyframe animations in Silverlight are defined using KeyFrame classes, such as DoubleKeyFrame, ColorKeyFrame, and PointKeyFrame, depending on the type of property being animated. These keyframes specify values at specific points in time, giving developers fine-grained control over the animation timeline.
Implementing a Keyframe Animation
Here’s an example of a keyframe animation that changes the color of an element over time:
1. Defining the Storyboard and Animation:
<UserControl.Resources>
<Storyboard x:Name="ColorChangeStoryboard">
<ColorAnimationUsingKeyFrames Storyboard.TargetProperty="(Panel.Background).(SolidColorBrush.Color)"
Storyboard.TargetName="animatedElement">
<!-- Keyframes go here -->
</ColorAnimationUsingKeyFrames>
</Storyboard>
</UserControl.Resources>
2. Adding Keyframes to the Animation:
<DiscreteColorKeyFrame KeyTime="0:0:1" Value="Red"/>
<DiscreteColorKeyFrame KeyTime="0:0:2" Value="Green"/>
<DiscreteColorKeyFrame KeyTime="0:0:3" Value="Blue"/>
This sequence changes the color of the animatedElement to red, green, and then blue, each color change occurring at one-second intervals.
3. Triggering the Animation:
Similar to basic animations, keyframe animations are triggered by an event or programmatically.
<Button Content="Start Color Change" Click="StartColorChange"/>
private void StartColorChange(object sender, RoutedEventArgs e)
{
ColorChangeStoryboard.Begin();
}
Advanced Techniques with Keyframes
- Easing Keyframes: Easing functions can be applied to keyframes for more natural motion. For instance, an EasingDoubleKeyFrame can create a smooth acceleration or deceleration effect.
- Combining Multiple Animations: Keyframe animations can be combined with other types of animations within the same storyboard to create sophisticated effects.
Procedural Animations in Silverlight
Procedural animations in Silverlight offer a dynamic approach to animating visuals, particularly beneficial in scenarios requiring real-time updates or complex, physics-based movements. Unlike Storyboard or keyframe animations, procedural animations are generated programmatically during runtime, offering immense flexibility and control.
Concept of Procedural Animations
Procedural animations involve calculations and algorithms to generate animation data in real-time. This approach is widely used in gaming and simulations where unpredictability and complexity are key factors.
Implementing Procedural Animations
To implement procedural animations in Silverlight, developers typically use the CompositionTarget.Rendering event. This event fires before the screen is redrawn, making it an ideal place to update animation states.
1. Setting Up the CompositionTarget.Rendering Event:
public MainPage()
{
InitializeComponent();
CompositionTarget.Rendering += OnRendering;
}
2. Implementing the OnRendering Method:
The OnRendering method is where the animation logic is executed. Here, you can update properties of UI elements based on custom algorithms or time-based changes.
private void OnRendering(object sender, EventArgs e)
{
// Animation logic goes here
// Example: Moving an element in a circular path
double time = (DateTime.Now - startTime).TotalSeconds;
double x = centerX + Math.Cos(time) * radius;
double y = centerY + Math.Sin(time) * radius;
MyAnimatedElement.SetValue(Canvas.LeftProperty, x);
MyAnimatedElement.SetValue(Canvas.TopProperty, y);
}
This sample moves an element in a circular path, using the system time to calculate its position.
3. Stopping the Animation:
To stop the animation, simply detach from the CompositionTarget.Rendering event.
CompositionTarget.Rendering -= OnRendering;
Applications of Procedural Animations
Procedural animations are particularly useful in scenarios such as:
- Creating custom particle systems.
- Simulating natural phenomena.
- Implementing physics-based movements and interactions.
Leveraging Writeable Bitmaps and Image Blitting in Silverlight
Writeable Bitmaps and Image Blitting are advanced techniques in Silverlight that offer more control and efficiency in rendering animations, especially when dealing with a large number of moving parts or complex graphical transformations.
Understanding Writeable Bitmaps
A Writeable Bitmap in Silverlight is a dynamic bitmap image that can be modified programmatically. This feature is particularly useful for creating image-based animations where pixels need to be manipulated or updated frequently.
Using Writeable Bitmaps for Animations
1. Creating a Writeable Bitmap:
First, you create a Writeable Bitmap with the desired dimensions.
WriteableBitmap writableBitmap = new WriteableBitmap(width, height);
2. Rendering Content to the Bitmap:
You can render shapes, text, or other UI elements onto the bitmap.
writableBitmap.Render(uiElement, new TranslateTransform() { X = 100, Y = 50 });
writableBitmap.Invalidate(); // Refreshes the bitmap
In this example, uiElement is a UI element (like a Rectangle or TextBlock) that you want to render on the bitmap.
3. Updating the Bitmap in Real-Time:
For animations, the bitmap can be updated inside an event like CompositionTarget.Rendering.
CompositionTarget.Rendering += (s, e) =>
{
// Update bitmap content here
};
Image Blitting Techniques
Image Blitting involves manipulating bitmap data directly for rendering graphics. This is a highly efficient way to handle animations, especially when dealing with numerous small images like particles in a particle system.
1. Blitting Images onto the Bitmap:
Blitting is done by copying pixel data from one bitmap to another. In Silverlight, this can be achieved through array manipulation and pixel-by-pixel operations.
int[] sourcePixels = // Pixel data from source image
int[] destPixels = writableBitmap.Pixels;
// Blit (copy) pixels from source to destination
Array.Copy(sourcePixels, 0, destPixels, destPosition, sourcePixels.Length);
writableBitmap.Invalidate(); // Refreshes the bitmap
Color Animations and Visual Effects in Silverlight
Color animations in Silverlight offer a visually engaging way to enhance user interfaces and user experiences. By animating color properties, developers can create interactive and dynamic visual effects, making applications more attractive and intuitive.
Implementing Color Animations
Color animations can be applied to various properties like backgrounds, borders, and text. Here’s how to create a basic color animation in Silverlight:
1. Defining the Color Animation in XAML:
You start by defining the animation within a Storyboard in your XAML file.
<UserControl.Resources>
<Storyboard x:Name="ColorAnimationStoryboard">
<ColorAnimation Duration="0:0:3" To="Red"
Storyboard.TargetProperty="(Panel.Background).(SolidColorBrush.Color)"
Storyboard.TargetName="animatedElement"/>
</Storyboard>
</UserControl.Resources>
In this example, the color animation targets the background color of an element named “animatedElement,” changing it to red over 3 seconds.
2. Triggering the Animation:
The animation can be initiated through an event handler or directly when the page loads.
<Button Content="Start Color Change" Click="StartColorAnimation"/>
private void StartColorAnimation(object sender, RoutedEventArgs e)
{
ColorAnimationStoryboard.Begin();
}
Advanced Color Animation Techniques
- Gradient Animations: Silverlight supports animations of gradient colors, allowing for transitions between multiple colors in gradient brushes.
- Animating Opacity Along with Color: Combining color animations with opacity animations can create fading effects for smooth transitions between states.
Advanced Techniques and Performance Optimization in Silverlight Animations
To create sophisticated and performant animations in Silverlight, it’s important to employ advanced techniques and optimize for performance. This approach ensures animations are not only visually appealing but also efficient and smooth, enhancing the overall user experience.
Advanced Animation Techniques
1. Combining Multiple Animations:
Silverlight allows for the combination of different animation types within a single storyboard. This technique can be used to create complex effects.
<Storyboard x:Name="CombinedAnimationStoryboard">
<DoubleAnimation ... />
<ColorAnimation ... />
<!-- Other animations -->
</Storyboard>
Here, DoubleAnimation and ColorAnimation are combined to create a multi-effect animation.
2. Using Animation Easing Functions:
Easing functions in Silverlight provide more natural movement by varying the speed of an animation over its duration.
<DoubleAnimation.EasingFunction>
<BounceEase Bounces="2" Bounciness="3"/>
</DoubleAnimation.EasingFunction>
This example adds a bounce effect to the animation, making it more dynamic.
Performance Optimization Strategies
1. Reducing Complexity:
Simplifying animations by reducing the number of animated properties or the complexity of the animation can significantly improve performance.
2. Optimizing Resource Usage:
Efficiently managing resources, such as bitmaps and timers, and disposing of them when not needed, helps in maintaining optimal performance.
3. Profiling and Testing:
Regularly profiling and testing animations on different devices and browsers ensure consistent performance across platforms.
// Example of using the DispatcherTimer for profiling
DispatcherTimer timer = new DispatcherTimer();
timer.Interval = TimeSpan.FromSeconds(1);
timer.Tick += (s, e) =>
{
// Monitoring and logging performance metrics
};
timer.Start();
4. Balancing Quality and Performance:
Adjusting the frame rate or quality of animations can strike a balance between visual quality and performance.
// Example of adjusting frame rate
Timeline.DesiredFrameRateProperty.OverrideMetadata(
typeof(Timeline),
new FrameworkPropertyMetadata { DefaultValue = 30 }
);
This sets the global frame rate for animations to 30 frames per second.
Practical Application: Optimized Animations in a Real-World Scenario
Consider an application with a dashboard displaying data visualizations. To ensure smooth performance:
- Use simple, yet effective animations for data changes.
- Optimize resource usage by reusing animation storyboards.
- Employ easing functions for natural motion.
- Regularly test across devices to ensure consistent performance.
Conclusion
Silverlight has proven itself as a robust platform for creating advanced animations. The journey from basic storyboards to intricate procedural animations and optimized performance reflects Silverlight’s evolution in web application development. Its ability to handle complex animations with ease and efficiency has made it a preferred choice for developers aiming to enhance user engagement and experience. As technology progresses, we can anticipate further advancements in Silverlight’s animation capabilities, ensuring it remains at the forefront of dynamic web application design.