Unity has emerged as one of the most popular game development platforms, renowned for its versatility and user-friendly interface. At the heart of Unity’s scripting capabilities lies C#, a powerful programming language that enables developers to breathe life into their game ideas. This section delves into the fundamentals of Unity as a game development tool and the integral role of C# in crafting immersive gaming experiences.
Unity: A Versatile Platform for Game Development
Unity stands out in the game development world due to its extensive support for a wide range of platforms, including PC, consoles, mobile devices, and VR/AR systems. Its real-time 3D development capabilities allow creators to visualize and iterate on their ideas quickly. Unity’s engine is adept at handling both 2D and 3D games, making it a versatile choice for developers with diverse project requirements.
Key Features of Unity:
- Cross-Platform Support: Unity’s ability to deploy games across multiple platforms is one of its most compelling features. Developers can build their games once and publish them on various platforms, including Windows, macOS, iOS, Android, and many others.
- Intuitive User Interface: The Unity Editor presents a user-friendly interface that lowers the entry barrier for new developers while offering powerful tools for experienced programmers.
- Asset Store: Unity hosts a vast Asset Store, providing an array of assets such as textures, models, animations, and even entire project templates, which can significantly speed up the development process.
The Role of C# in Unity Game Development
C# (pronounced “C Sharp”) is the primary programming language used in Unity for scripting. It’s a high-level, object-oriented language developed by Microsoft, part of the .NET framework. C# in Unity is used to define the behavior of game objects, control game logic, and interact with the game environment.
Why C# in Unity?
- Ease of Learning: C# is known for its clear syntax and structured nature, making it an ideal language for beginners in game development.
- Powerful and Flexible: Despite being easy to learn, C# is incredibly powerful. It supports advanced programming concepts like encapsulation, inheritance, and polymorphism, crucial for creating complex games.
- Community and Documentation: The Unity developer community is vast and active. Coupled with comprehensive documentation, new developers find it easier to learn and troubleshoot issues in their projects.
- Integration with Unity: C# scripts in Unity interact seamlessly with the engine, allowing developers to easily manipulate game objects and their properties.
Getting Started with Unity and C#
Before diving into game development with Unity and C#, it’s essential to set up the correct environment. Installing Unity is straightforward. Developers can download Unity Hub, a management tool that helps to install different versions of the Unity Editor and manage projects. Once Unity is installed, it integrates seamlessly with C#, enabling developers to start scripting immediately.
Unity’s scripting environment is designed to be intuitive. As you write C# scripts, Unity compiles them and makes them a part of your game project. These scripts are attached to game objects within the Unity Editor, defining their behaviors and interactions.
Setting Up Your Development Environment
The first step in harnessing the power of Unity and C# for game development is setting up the right environment. This involves installing Unity, familiarizing yourself with its interface, and understanding the basics of how C# scripts interact within this environment.
Installing Unity and Configuring for C# Development
- Download and Install Unity Hub: Unity Hub is a central place where you can manage multiple Unity versions and projects. Download it from the Unity website and install it on your computer.
- Install the Unity Editor: Through Unity Hub, install the Unity Editor. You can choose the version that best suits your needs, although the latest stable release is generally recommended.
- Set Up Visual Studio for Unity: Unity integrates with Visual Studio, which serves as the primary IDE for C# scripting. Make sure to install Visual Studio along with the Unity workload, which includes essential tools and features for Unity development.
Familiarizing with the Unity Editor Interface
- Unity Editor Layout: The Unity Editor comprises several panels and windows. Key areas include the Scene view for visualizing and arranging game objects, the Game view for testing your game, the Hierarchy window for managing game objects, the Project window for accessing assets, and the Inspector window for modifying the properties of game objects and components.
- Creating a New Project: Start by creating a new project in Unity. Choose a template that matches the type of game you are planning to develop, like 3D or 2D.
- Exploring the Project Structure: Unity projects have a standard structure, with folders like Assets, where you store all your game assets, and Scripts, where you keep your C# scripts.
Writing Your First C# Script in Unity
- Creating a C# Script: In the Project window, right-click, select Create > C# Script. Name your script, and it will appear in the list of assets.
- Basic Script Structure: C# scripts in Unity start with importing necessary namespaces, like
UnityEngine
. The script then contains a class that inherits fromMonoBehaviour
, Unity’s base class for all scripts.
using UnityEngine;
public class MyFirstScript : MonoBehaviour
{
// Script content goes here
}
- Attaching Scripts to Game Objects: Drag and drop your script onto a game object in the Hierarchy window. This links your script to the object, allowing the script to control its behavior.
- Editing Scripts: Double-click on the script asset to open it in Visual Studio. Here, you can write and edit your C# code, which Unity will compile and execute as part of your game.
With your development environment set up and a basic understanding of the Unity Editor and C# scripting, you’re ready to start exploring the possibilities of game development with Unity. In the next sections, we’ll dive deeper into the specifics of C# programming within Unity, including variables, data types, and the fundamental structures that form the backbone of game scripting.
Basics of C# in Unity
Understanding the basics of C# is crucial for effective game development in Unity. This segment will explore fundamental concepts like variables, data types, and basic structures that are essential in C# programming for Unity.
Understanding Variables and Data Types
In C#, variables are used to store information. Variables have a type, a name, and a value. The type determines what kind of data the variable can hold.
- Common Data Types: Some of the basic data types in C# include:
int
: For storing integers.float
: For storing floating-point numbers.string
: For storing sequences of characters.bool
: For storing true/false values.
- Variable Declaration: Declaring a variable in C# involves specifying its type and name, and optionally setting a value.
int playerScore = 100;
float playerSpeed = 5.0f;
string playerName = "Hero";
bool isGameOver = false;
Conditional Statements
Conditional statements allow you to execute different code based on certain conditions.
- If-Else Statement: The most basic conditional statement in C#.
if (playerScore > 50)
{
Debug.Log(“You’re doing great!”);
}
else
{
Debug.Log(“Try harder!”);
}
Loops
Loops are used to repeat actions until a certain condition is met.
- For Loop Example: A simple loop that repeats an action a specific number of times.
for (int i = 0; i < 10; i++)
{
Debug.Log("Loop iteration: " + i);
}
Functions or Methods
Functions (also known as methods) are blocks of code that perform a specific task and are executed when called.
- Creating a Function: A simple function in C#.
void StartGame()
{
Debug.Log("Game Started!");
playerScore = 0;
}
- Calling a Function: Functions are called by their name followed by parentheses.
StartGame();
Understanding these basic concepts of C# is the first step towards building complex game logic in Unity. As you become more familiar with these fundamentals, you’ll find that they form the building blocks of more advanced game development concepts.
Unity’s Scripting Essentials
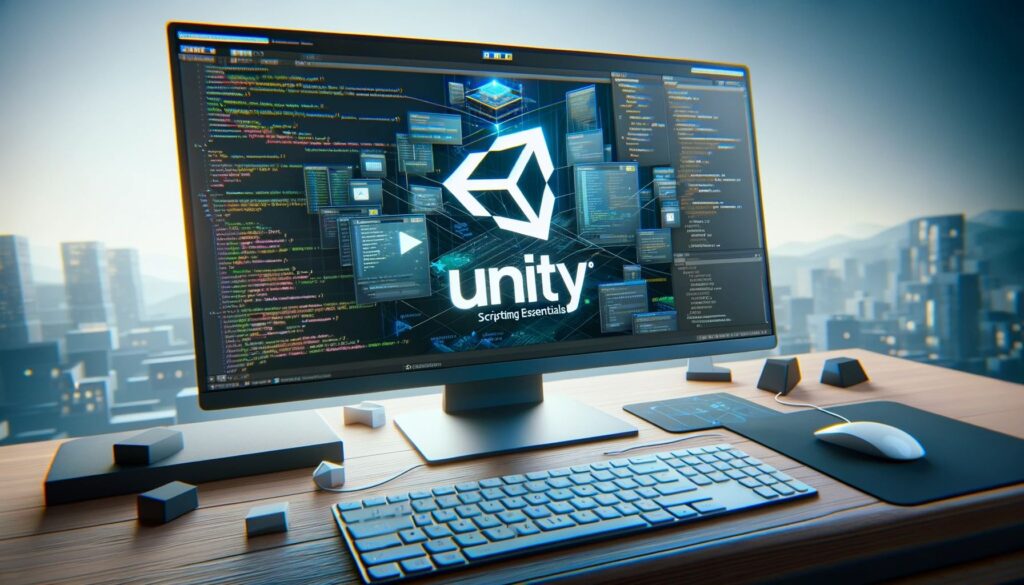
Diving deeper into Unity and C#, it’s essential to understand how scripting integrates into the game development process. Unity’s scripting model revolves around several key concepts and functions that are pivotal for game behavior and mechanics.
MonoBehaviour and the Unity Lifecycle
The MonoBehaviour
class is the base class from which all Unity scripts derive. It provides a range of event-driven methods that are automatically called by Unity during the lifecycle of a game object.
- Key MonoBehaviour Methods:
Awake()
: Called when the script instance is being loaded.Start()
: Called before the first frame update, often used for initialization.Update()
: Called once per frame, perfect for game logic that needs to be checked continuously.
public class PlayerController : MonoBehaviour
{
void Start()
{
// Initialization code here
}
void Update()
{
// Code to move the player, check for collisions, etc.
}
}
Interacting with Game Objects
In Unity, everything in your scene is a game object. Scripts are used to define the behavior of these objects.
- Accessing Components: Game objects are made up of components such as
Transform
,Rigidbody
, or custom scripts. You can access and modify these components using C#.
public class Mover : MonoBehaviour
{
void Start()
{
Rigidbody rb = GetComponent<Rigidbody>();
rb.velocity = new Vector3(0, 0, 10);
}
}
- Instantiating Game Objects: You can create new game objects during runtime. This is commonly used for spawning enemies, bullets, etc.
public class Spawner : MonoBehaviour
{
public GameObject enemyPrefab;
void Start()
{
Instantiate(enemyPrefab, new Vector3(0, 0, 0), Quaternion.identity);
}
}
Unity Event Functions
Unity provides several event functions that are called in response to specific actions or events in the game lifecycle.
- Collision Events:
OnCollisionEnter
,OnCollisionExit
, andOnCollisionStay
are used to detect and respond to collisions.
void OnCollisionEnter(Collision collision)
{
if (collision.gameObject.tag == "Enemy")
{
Destroy(collision.gameObject);
}
}
- Input Handling: Detecting player input is crucial for interactive games. Unity simplifies this process with functions like
Input.GetKeyDown
.
void Update()
{
if (Input.GetKeyDown(KeyCode.Space))
{
// Perform action when Space key is pressed
}
}
Understanding these scripting essentials in Unity is crucial for creating dynamic and responsive game mechanics. The flexibility of C# in Unity allows developers to craft unique gameplay experiences, from simple 2D platformers to complex 3D environments.
Enhancing Game Mechanics with C#
Moving forward in our journey through Unity and C#, let’s focus on how we can use C# to enhance the mechanics of a game. This involves more than just making objects move or interact; it’s about creating a rich, interactive environment that feels alive and responsive to the player.
Implementing Player Controls
One of the first steps in bringing a game to life is enabling player interaction, typically through controls.
- Basic Movement: Here’s an example of how you might allow a player to move an object using the keyboard.
public class PlayerMovement : MonoBehaviour
{
public float speed = 5.0f;
void Update()
{
float moveHorizontal = Input.GetAxis("Horizontal");
float moveVertical = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveHorizontal, 0.0f, moveVertical);
transform.Translate(movement * speed * Time.deltaTime);
}
}
In this script, Input.GetAxis
is used to get input from the keyboard (or a gamepad), and the object’s position is updated accordingly.
Working with Unity’s Rigidbody for Physics-Based Movement
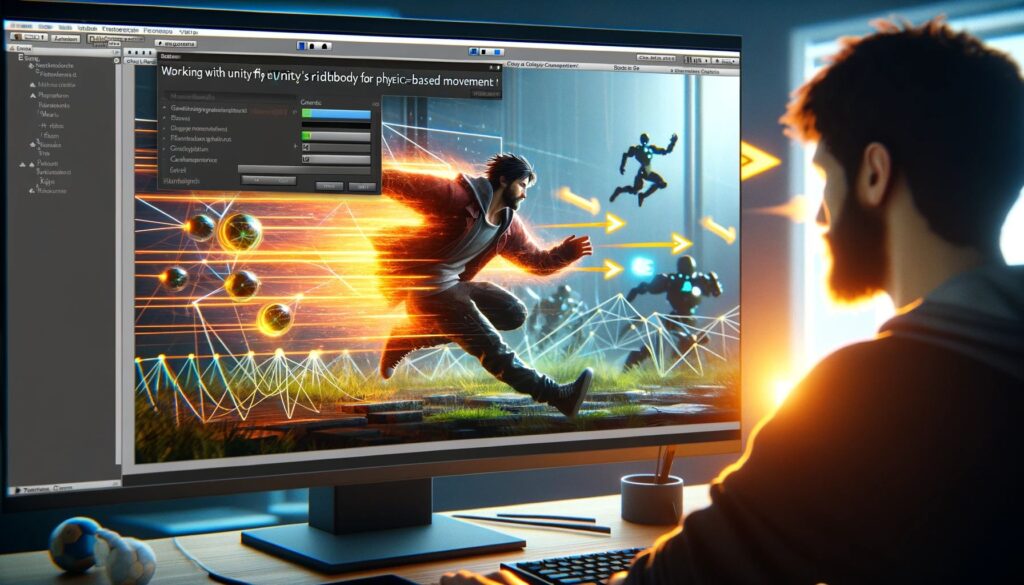
To add a sense of realism through physics, Unity’s Rigidbody
component is used. It allows objects to act under the laws of physics.
- Adding Force and Movement: Here’s how you might apply force to a Rigidbody to move a game object.
public class PlayerController : MonoBehaviour
{
public float thrust = 10.0f;
private Rigidbody rb;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void FixedUpdate()
{
if (Input.GetKey(KeyCode.W))
{
rb.AddForce(Vector3.forward * thrust);
}
}
}
In this example, AddForce
is used in the FixedUpdate
method to apply a continuous force to the Rigidbody, moving the object forward when the player presses the ‘W’ key.
Interaction with Environment and Objects
Interactions are not limited to player movement. Objects in the game world can interact with each other and the environment.
- Detecting Collisions: Detecting and responding to collisions is a fundamental part of game mechanics.
void OnCollisionEnter(Collision collision)
{
if (collision.gameObject.CompareTag("Collectible"))
{
Destroy(collision.gameObject);
// Increment score or perform other actions
}
}
This script checks for collisions with objects tagged as “Collectible” and destroys them, which can be part of a scoring system or a game objective.
Creating Dynamic and Responsive Environments
Finally, creating a dynamic and responsive game environment is key to an engaging game experience. This can include changing environmental conditions, triggering events based on player actions, or creating a world that evolves over time.
- Triggering Events: Here’s a simple way to trigger an event when a player enters a specific area.
void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Player"))
{
// Trigger an event, like opening a door or revealing a secret
}
}
This script uses OnTriggerEnter
to detect when the player enters a trigger zone, which can then be used to initiate various in-game events.
By mastering these aspects of C# scripting in Unity, you can create games that are not only fun to play but also rich in mechanics and interaction. The possibilities are nearly endless, limited only by your imagination and understanding of the tools at your disposal.
Advanced C# Features for Game Development
As you grow more comfortable with the basics of Unity and C#, it’s time to explore advanced features that can significantly enhance your game’s complexity and appeal. This includes implementing artificial intelligence, managing physics simulations, and handling advanced player interactions.
Implementing Artificial Intelligence (AI)
AI can transform your game, adding depth and challenge by simulating intelligent behavior in non-player characters (NPCs).
- Basic AI Movement: Here’s a simple way to make an enemy character follow the player.
public class EnemyAI : MonoBehaviour
{
public Transform player;
public float moveSpeed = 2.0f;
void Update()
{
transform.LookAt(player);
transform.position += transform.forward * moveSpeed * Time.deltaTime;
}
}
In this script, the enemy character will continuously move towards the player’s position, simulating a basic chase behavior.
Handling Physics Simulations
Unity’s physics engine allows for realistic simulation of physical interactions in the game world.
- Using Rigidbody for Complex Movements: Beyond basic movement, the Rigidbody component can be used for more complex physics-based behaviors like jumping.
public class PlayerController : MonoBehaviour
{
public float jumpForce = 5.0f;
private Rigidbody rb;
public class PlayerController : MonoBehaviour
{
public float jumpForce = 5.0f;
private Rigidbody rb;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
if (Input.GetButtonDown("Jump") && Mathf.Abs(rb.velocity.y) < 0.01f)
{
rb.AddForce(Vector3.up * jumpForce, ForceMode.Impulse);
}
}
}
This script allows the player to jump, but only when they are not already moving upwards or downwards, preventing double jumps in mid-air.
Advanced Player Interactions
Enhancing player interaction can significantly improve the gaming experience, making it more immersive and engaging.
- Interactive Game Elements: For instance, you can create a script that allows players to interact with objects in the game world.
public class InteractableObject : MonoBehaviour
{
void OnMouseDown()
{
// Code to interact with the object
// This could be picking up an item, opening a door, etc.
}
}
This script uses the OnMouseDown
method, which is called when the player clicks on an object with this script attached. It’s a simple way to add interactivity to objects in your game.
Networking and Multiplayer
Creating multiplayer games in Unity using C# involves managing network states, synchronizing player actions, and ensuring a consistent experience across different clients.
- Basic Network Setup: Unity provides the tools necessary for building multiplayer games, but this topic is quite advanced and involves a thorough understanding of networking principles.
// This is a simplified example and real-world networking in Unity is more complex
public class NetworkPlayer : MonoBehaviour
{
void Update()
{
if (isLocalPlayer)
{
// Handle local player movement or actions
}
}
}
In this snippet, isLocalPlayer
would be a boolean indicating if this instance of the script is running on the local player’s machine. This is a fundamental concept in Unity networking, ensuring that each player’s game handles only their character.
Exploring these advanced aspects of C# in Unity opens up a new realm of possibilities for your games. You can create more dynamic, responsive, and complex games that are both challenging and engaging for players.
Best Practices and Common Pitfalls
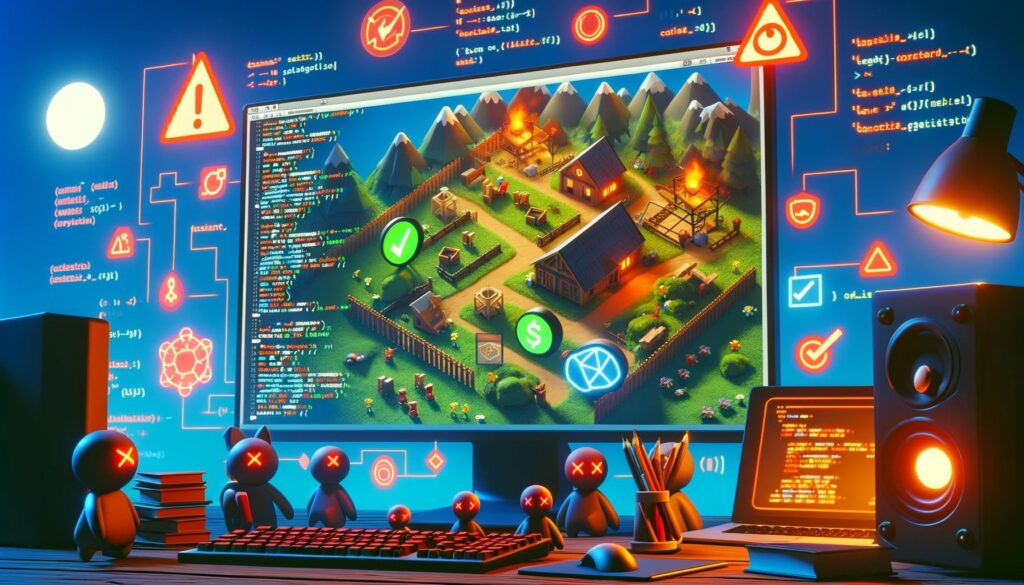
While creating a game using C# in Unity, it’s important to follow best practices to ensure your project is efficient, maintainable, and delivers a smooth gaming experience. This section will cover common pitfalls to avoid and optimization techniques to implement in your Unity and C# game development journey.
Effective Debugging
Debugging is an essential part of game development. Unity provides various tools to help you identify and fix issues in your code.
- Unity’s Debug Class: The
Debug
class is your best friend when it comes to debugging. You can useDebug.Log
to print messages to the console for debugging purposes.
void Update()
{
Debug.Log("Updating...");
}
- Unity’s Inspector: Utilize the Inspector window to inspect and modify variables during runtime. This allows you to fine-tune your game without altering the code.
Optimization Techniques
Optimizing your game is crucial for ensuring it runs smoothly on a variety of devices and platforms.
- Object Pooling: Instead of constantly creating and destroying objects (e.g., bullets, enemies), use object pooling to reuse them. This reduces memory overhead and improves performance.
public class ObjectPool : MonoBehaviour
{
public GameObject prefab;
private List<GameObject> pool = new List<GameObject>();
void Start()
{
for (int i = 0; i < poolSize; i++)
{
GameObject obj = Instantiate(prefab);
obj.SetActive(false);
pool.Add(obj);
}
}
public GameObject GetObject()
{
foreach (GameObject obj in pool)
{
if (!obj.activeInHierarchy)
{
obj.SetActive(true);
return obj;
}
}
return null;
}
}
- Batching: Unity can automatically batch similar objects to reduce draw calls. Ensure your materials and shaders are set up for batching.
- Avoiding Expensive Operations in Update: Heavy calculations in the
Update
method can impact performance. Consider usingFixedUpdate
for physics-related updates andLateUpdate
for camera and follow functions.
void FixedUpdate()
{
// Perform physics-related calculations here
}
void LateUpdate()
{
// Perform camera and follow updates here
}
Avoiding Common Pitfalls
Here are some common pitfalls to be aware of and avoid:
- Memory Leaks: Always clean up unused objects, materials, and textures to prevent memory leaks. Use Unity’s profiler to identify memory issues.
- Overusing Physics: Physics calculations can be computationally expensive. Avoid having too many physics objects with complex colliders and interactions.
- Inefficient Asset Management: Organize your project’s assets efficiently. Use asset bundles for large projects to load assets on demand.
- Ignoring Cross-Platform Considerations: Test your game on different devices and platforms to ensure compatibility. Optimize graphics and controls for various screen sizes and resolutions.
- Lack of Code Comments and Documentation: Document your code with comments and maintain clear naming conventions to make it more readable and maintainable.
By following these best practices and avoiding common pitfalls, you can create high-quality Unity games with C# that run smoothly, provide a great user experience, and are easier to maintain and scale.
Leveraging Unity Community and Resources
Unity has a vast and active community of developers who are always willing to help and share their knowledge. Take advantage of the following resources:
- Unity Forums: Unity’s official forums are a great place to ask questions, seek advice, and find solutions to common issues.
- Online Tutorials and Courses: Many online platforms offer tutorials and courses on Unity and C# game development, such as Unity Learn, Udemy, and Coursera.
- GitHub Repositories: Explore open-source Unity projects on GitHub to learn from other developers’ code and practices.
- Unity Asset Store: The Asset Store offers a wide range of assets, scripts, and tools that can save you time and effort in your game development journey.
- Unity Documentation: Unity provides extensive documentation that covers all aspects of the engine and scripting in detail.
As you continue your Unity and C# game development adventure, don’t hesitate to reach out to the community and utilize these resources to enhance your skills and overcome challenges.
Conclusion
Mastering C# in Unity opens the door to captivating game development. This guide has provided the essential foundations and advanced techniques needed for success.
From setting up your environment to harnessing C# for game logic and AI, you’ve gained the skills to breathe life into your creations. Effective debugging, optimization, and community resources ensure a smooth development journey. Now, equipped with Unity and C#, you’re ready to turn your gaming visions into reality and captivate players worldwide. Happy coding! 😊