Introduction to ActionScript in E-commerce Development
ActionScript, a scripting language developed by Adobe Systems, has played a pivotal role in the evolution of web applications, particularly in the realm of e-commerce. This language, rooted in the ECMAScript standard, shares many commonalities with JavaScript, making it familiar and accessible to a wide range of developers. Over the years, ActionScript has evolved significantly, expanding its capabilities from simple animations to the creation of rich, interactive web applications.
The Early Days: From Animations to Rich Web Experiences
ActionScript’s journey began as a tool for enhancing the functionality of animations created in Adobe Flash, then known as Macromedia Flash. In its initial versions, Flash primarily focused on animation, offering limited interactivity features. This meant that the scripting capabilities were basic, only allowing for simple user interactions. However, as the internet and web technologies progressed, so did the demands for more dynamic and interactive web content. This need led to the significant expansion of ActionScript’s capabilities.
A Turning Point: ActionScript for E-commerce
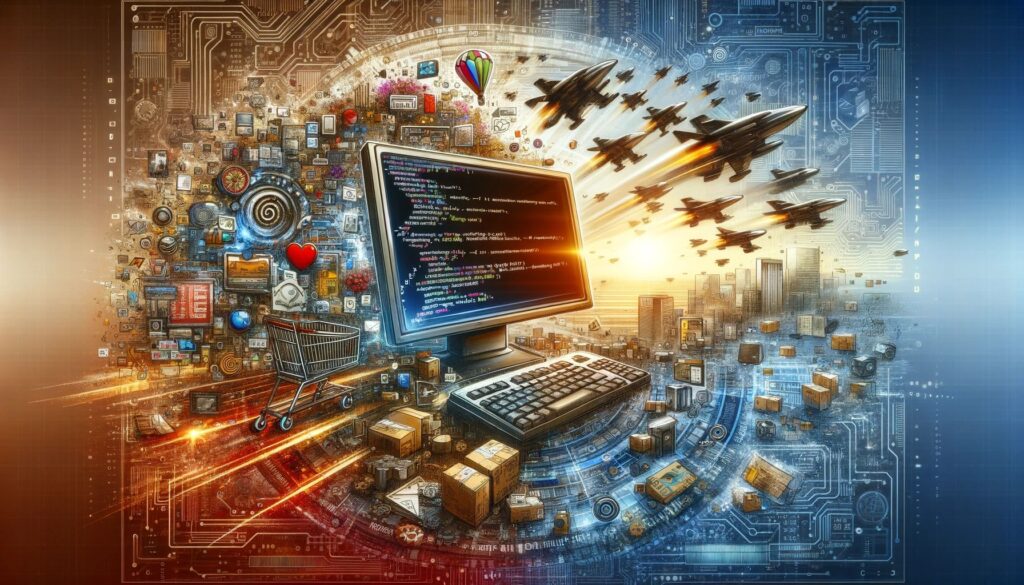
With the advent of Flash MX 2004, ActionScript 2.0 was introduced, marking a significant leap in the language’s development. This version was more suited to the creation of complex Flash applications, which included e-commerce websites. The scripting language allowed developers to save time by scripting functionalities instead of animating them, offering a higher level of flexibility and efficiency in web development. This feature was particularly beneficial for e-commerce sites, where dynamic content and user interaction are crucial.
Advancements with ActionScript 3.0
The release of ActionScript 3.0, aligned with Flash Player 9, brought about another major enhancement in the language’s capabilities. This version was designed to be compiled and run on the newly written ActionScript Virtual Machine 2 (AVM2), resulting in significantly faster execution speeds — up to 10 times faster than the previous versions. The just-in-time compiler enhancements in ActionScript 3.0 enabled more complex and performance-intensive applications, ideal for modern e-commerce platforms that require high responsiveness and dynamic content handling.
Application in E-commerce Web Development
The use of ActionScript in e-commerce websites has allowed for the creation of highly interactive and engaging user interfaces. Its ability to handle multimedia content, including video and audio streaming, has opened up new avenues for showcasing products and services in an interactive manner. Moreover, the integration of ActionScript with Adobe AIR extends its utility beyond web browsers, allowing e-commerce platforms to reach users on desktop and mobile devices, thus broadening the potential customer base.
Understanding ActionScript Versions: From 1.0 to 3.0
ActionScript’s evolution has been marked by significant changes in its structure and capabilities, each version bringing new features and improvements suited for complex web applications like e-commerce platforms.
ActionScript 1.0: The Beginning
ActionScript 1.0, introduced with Flash 5, was based on ECMAScript and allowed for basic procedural programming and object-oriented programming. This version was straightforward, making it suitable for simple interactive features on websites. A typical code snippet in ActionScript 1.0 might look like this:
// Basic button click interaction
on (release) {
// Navigate to a different frame on the timeline
gotoAndStop("productsPage");
}
This code represents a basic interaction where clicking a button would navigate the user to a different part of the Flash timeline, possibly showing a products page in an e-commerce context.
ActionScript 2.0: Advancing the Capabilities
With Flash MX 2004, ActionScript 2.0 was introduced, offering a more robust and object-oriented approach. It allowed for more sophisticated programming techniques, making it suitable for more complex applications. A sample code snippet in ActionScript 2.0 could be:
// A simple ActionScript 2.0 class
class ProductDisplay {
private var productName:String;
public function ProductDisplay(name:String) {
this.productName = name;
}
public function display():Void {
trace("Displaying product: " + productName);
}
}
var myProduct:ProductDisplay = new ProductDisplay("Smartphone");
myProduct.display();
This code defines a class for displaying a product, demonstrating the object-oriented capabilities of ActionScript 2.0.
ActionScript 3.0: A Leap Forward
ActionScript 3.0, aligned with Flash Player 9, was a complete overhaul of the language, optimized for performance and aligned with modern programming standards. It provided a more powerful platform for developing rich Internet applications, including e-commerce sites. A basic example of ActionScript 3.0 code is:
// ActionScript 3.0 event handling
package {
import flash.display.Sprite;
import flash.events.MouseEvent;
public class ProductButton extends Sprite {
public function ProductButton() {
this.addEventListener(MouseEvent.CLICK, onClick);
}
private function onClick(event:MouseEvent):void {
trace("Product clicked");
}
}
}
In this snippet, a class is defined that responds to a mouse click, a common requirement for interactive elements in an e-commerce site.
Each version of ActionScript brought its own strengths and was suited for different stages of e-commerce web development. ActionScript 1.0 was great for simple interactivities, ActionScript 2.0 introduced more structured coding practices, and ActionScript 3.0 provided a powerful environment for creating complex and high-performance web applications. Understanding these differences is crucial for developers to choose the right version for their specific e-commerce project needs.
ActionScript and Adobe AIR: Expanding Beyond Web Browsers
The introduction of Adobe AIR marked a significant shift in the capabilities of ActionScript, extending its utility beyond traditional web browsers into desktop and mobile application development. This advancement opened up new possibilities for e-commerce platforms, allowing them to offer richer, more engaging experiences across various devices.
Desktop and Mobile Development with Adobe AIR
Adobe AIR enables ActionScript developers to create standalone applications for desktop and mobile platforms. This means e-commerce applications can be built to run natively on a user’s device, providing a more integrated and responsive experience compared to traditional web apps. An example of ActionScript code used in an Adobe AIR project might involve creating a native window:
import flash.display.NativeWindow;
import flash.display.NativeWindowInitOptions;
var windowOptions:NativeWindowInitOptions = new NativeWindowInitOptions();
var myWindow:NativeWindow = new NativeWindow(windowOptions);
myWindow.activate();
This snippet shows how ActionScript can be used to initiate and control native application windows, a capability that’s essential for desktop applications.
ActionScript in Database Applications
Beyond traditional e-commerce websites, ActionScript’s flexibility allows it to be utilized in database applications. This can be especially useful for managing large inventories, customer data, and transaction records in e-commerce systems. For example, ActionScript can interface with a database to retrieve product information:
// Code to connect and retrieve data from a database
var loader:URLLoader = new URLLoader();
loader.addEventListener(Event.COMPLETE, loadComplete);
loader.load(new URLRequest("http://example.com/api/products"));
function loadComplete(event:Event):void {
var products:Array = JSON.parse(loader.data) as Array;
// Process and display products
}
This code demonstrates how ActionScript can be used to interact with web APIs, a common method for retrieving data from databases in modern web applications.
Utilizing ActionScript in Basic Robotics
A lesser-known application of ActionScript is in the field of basic robotics. The Make Controller Kit, for instance, can be programmed using ActionScript, enabling developers to create interactive physical systems that could be integrated into an e-commerce context, like automated inventory management or interactive displays.
ActionScript for Interactive and Dynamic E-commerce Features
In the world of e-commerce, engaging the customer is key. ActionScript shines in this area, providing a wide range of capabilities for creating interactive and dynamic features on e-commerce websites.
Creating Interactive User Interfaces
Interactive user interfaces are crucial in keeping potential customers engaged and guiding them through the buying process. ActionScript can be used to create interactive product galleries, custom shopping carts, and personalized user experiences. For instance:
// Interactive product gallery
var gallery:ProductGallery = new ProductGallery();
addChild(gallery);
gallery.loadProducts(["product1.jpg", "product2.jpg", "product3.jpg"]);
In this example, an interactive product gallery is created, which could enhance the shopping experience by allowing customers to browse products in a more engaging way.
Dynamic Content Management
E-commerce sites often need to display a large amount of dynamic content, such as product listings, which are regularly updated. ActionScript can handle this dynamically, updating content in real-time based on user interactions or changes in the database.
// Dynamic content loading
function loadProductDetails(productId:int):void {
var loader:URLLoader = new URLLoader();
loader.load(new URLRequest("http://example.com/api/product/" + productId));
loader.addEventListener(Event.COMPLETE, onProductLoadComplete);
}
function onProductLoadComplete(event:Event):void {
var productDetails:Object = JSON.parse(event.target.data);
// Display product details
}
This code snippet demonstrates how ActionScript can dynamically load and display product details, an essential feature for any e-commerce platform.
Best Practices in ActionScript for E-commerce Sites
Developing e-commerce sites with ActionScript requires a careful approach to ensure optimal performance, user experience, and maintainability. Here are some best practices that developers should consider when using ActionScript in e-commerce development.
Optimizing Performance and Responsiveness
E-commerce websites must be fast and responsive to ensure a smooth shopping experience. ActionScript developers should:
- Efficiently Manage Memory: Avoid memory leaks by properly disposing of objects and listeners when they are no longer needed.
- Optimize Asset Loading: Use loaders effectively to manage and prioritize the loading of external assets such as images and videos.
- Leverage Caching: Store reusable data in cache to reduce server requests and loading times.
Enhancing User Experience
The user experience is critical in e-commerce. ActionScript can enhance this through:
- Interactive Product Displays: Create interactive galleries and 3D product views to engage customers.
- Seamless Navigation: Ensure that site navigation is intuitive and efficient, reducing the number of clicks to reach desired products or information.
- Personalized Experiences: Use data tracking to provide personalized recommendations and content.
Tips for Mobile Device Compatibility
With the increasing use of mobile devices for online shopping, ensuring your e-commerce site is mobile-friendly is essential.
- Responsive Design: Ensure your ActionScript content scales properly on different screen sizes and resolutions.
- Touch Interactions: Implement touch-friendly interfaces, considering the difference in interaction compared to mouse events.
- Optimized Performance: Be mindful of the performance and memory constraints of mobile devices.
Accessibility Considerations
Accessibility is not only a legal requirement in many regions but also expands your market reach.
- Keyboard Navigation: Ensure that all interactive elements are accessible via keyboard.
- Screen Reader Compatibility: Use appropriate tags and descriptions for screen readers.
- Contrast and Font Size: Ensure that your text is readable and your site is navigable for users with visual impairments.
Integrating ActionScript with Other Adobe Tools
Leveraging the synergy between ActionScript and other Adobe tools can greatly enhance the capabilities of an e-commerce platform.
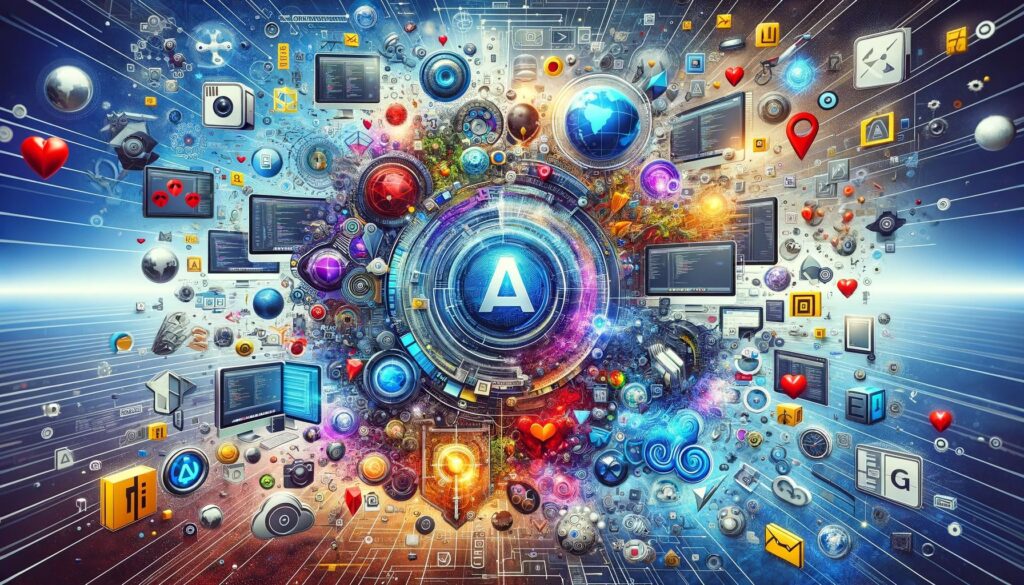
Using ActionScript with Adobe Premiere Pro and After Effects
- Rich Media Content: Utilize Adobe Premiere Pro and After Effects for creating high-quality video content for product demonstrations and promotions.
- Animation Integration: Seamlessly integrate animations created in After Effects into your ActionScript projects for dynamic visual effects.
Enhancing Multimedia Capabilities
- Audio Integration: Use Adobe’s audio tools to incorporate background music or product-related audio clips that enhance the shopping experience.
- Video Streaming: Implement video streaming features using ActionScript for product launches or live demonstrations.
Future of ActionScript in E-commerce Development
The landscape of web development is constantly evolving, and so is the role of ActionScript in e-commerce.
The Shift Towards Newer Technologies
While ActionScript has been a staple in web development, newer technologies like HTML5, CSS3, and JavaScript frameworks are becoming more prevalent. However, ActionScript still holds a niche for certain types of interactive applications and games.
Case Studies of Successful E-commerce Websites Using ActionScript
Several e-commerce platforms have effectively used ActionScript to create engaging user experiences. Studying these case studies can provide valuable insights into how ActionScript can be utilized creatively and effectively.
- Interactive Product Configurators: Websites that allow customers to customize products in real-time using interactive tools developed in ActionScript.
- Engaging Marketing Campaigns: Innovative marketing campaigns using rich media and interactive content to attract and retain customers.
Conclusion
In conclusion, ActionScript’s journey through the evolution of web development, particularly in the e-commerce sector, highlights its versatility and enduring relevance. From its early days of enhancing simple web animations to its current capabilities in creating rich, interactive e-commerce platforms, ActionScript has demonstrated its adaptability and strength. Its integration with Adobe AIR and other Adobe tools has further extended its utility, enabling developers to craft comprehensive and immersive online shopping experiences. While newer technologies continue to emerge and reshape the web development landscape, ActionScript’s established role in creating dynamic, interactive content ensures it remains a valuable asset in the developer’s toolkit, especially for specific applications where its unique features are most beneficial.
The future of ActionScript in e-commerce development may be influenced by the broader trends in web technology, yet its legacy in advancing web interactivity will continue to inform and inspire. For developers and businesses, understanding the nuances of ActionScript, from its version differences to its best practices in modern web development, is crucial for leveraging its full potential. As the digital marketplace grows increasingly competitive, the ability to create engaging, responsive, and user-friendly e-commerce platforms is paramount. ActionScript, with its rich history and proven capabilities, remains a tool worth considering for those looking to innovate and captivate in the ever-evolving world of e-commerce.