Introduction to Scalability in ASP.NET
ASP.NET, developed by Microsoft, is a versatile framework for building dynamic web applications. It supports a range of programming languages like C#, F#, and VB.NET, offering a rich library and a consistent programming model that emphasizes security and efficiency. This makes it a popular choice for everything from small websites to complex enterprise systems. However, in the fast-paced digital world, a key challenge for ASP.NET developers is ensuring scalability – the ability of an application to handle increasing loads without compromising performance. Scalability involves not just accommodating more users or data but doing so efficiently, maintaining responsiveness and reliability.
Scalability in ASP.NET spans a spectrum from simple code optimizations to complex, cloud-native designs. Initially, developers may focus on optimizing code and database queries for better performance. As needs grow, more sophisticated strategies like load balancing, which distributes workload across multiple servers, become essential. At the cutting edge are cloud-native applications, designed from the ground up to leverage the cloud’s elasticity and power, allowing them to scale quickly and effectively in response to real-time demands. Understanding and implementing these scalability strategies is crucial for ASP.NET developers to create robust and future-proof web applications.
Understanding the Scalability Spectrum in ASP.NET
Definition and Significance of Scalability
Scalability in ASP.NET is the framework’s capacity to handle increased loads, whether it’s more data, users, or transactions, without a drop in performance. This concept is central to modern web application development, where user numbers and data volumes can fluctuate dramatically. Scalable ASP.NET applications can grow to meet demand while maintaining efficiency, a quality that’s essential for user satisfaction and operational stability.
Scalability is more than just handling a higher number of requests; it’s about doing so effectively, ensuring that each user’s experience remains consistent and reliable, regardless of the load on the system. This means an application should be able to scale up (increase its capacity) or scale out (distribute the load across multiple systems) as needed.
Performance vs. Scalability
Aspect | Performance | Scalability |
---|---|---|
Focus | How quickly a system can handle individual tasks. | How well a system can maintain performance as workload increases. |
Measurement | Response time and resource usage per task. | Consistency in performance under varying loads. |
Improvement Methods | Code optimization, efficient algorithms. | Adding resources, optimizing resource usage. |
Performance is about the speed and efficiency of processing a single request, while scalability is about handling multiple requests simultaneously. Both are crucial, but they require different approaches. Performance optimization often involves refining code and algorithms for speed and lower resource consumption. Scalability, on the other hand, might involve strategies like load balancing and efficient resource management to handle more requests without performance degradation.
Differentiating Between Performance and Scalability
Understanding the distinction between performance and scalability is crucial in ASP.NET development. A high-performance application may not necessarily be scalable if it cannot handle an increase in load without performance issues. Conversely, a scalable application might have moderate performance but maintains that level consistently as the number of users increases.
Common Scalability Bottlenecks in ASP.NET
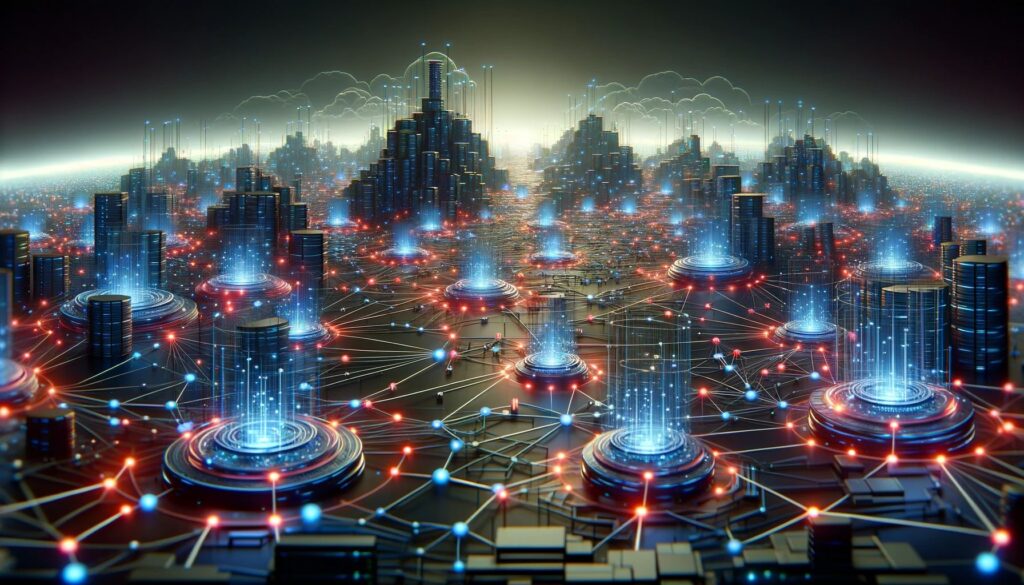
Identifying Typical Bottlenecks
In ASP.NET development, scalability bottlenecks refer to points in the application where the flow of data or processing is impeded, causing performance degradation as the load increases. Identifying these bottlenecks is crucial for creating scalable applications. Common bottlenecks include:
- Database Access: Excessive or inefficient database queries can significantly slow down an application, especially under heavy user loads.
- Network Latency: The time it takes for data to travel across the network can become a bottleneck, particularly in distributed architectures.
- Resource Contention: When multiple processes compete for limited resources like CPU, memory, or I/O, it can lead to performance issues.
- Application Design: Poorly designed applications, with monolithic architectures or unoptimized code, can hinder scalability.
- Third-party Services and APIs: Over-reliance on external services can introduce delays and reliability issues, affecting scalability.
How Increased User Load Affects Performance
User Load | Impact on Performance |
---|---|
Low | Minimal impact; resources are underutilized. |
Moderate | Some strain on resources; minor performance issues may arise. |
High | Significant impact; bottlenecks become apparent, leading to slow response times and potential system crashes. |
As user load increases, the impact on performance becomes more pronounced, revealing scalability issues. For example, an application might perform well with a small number of users but starts to slow down as more users access it simultaneously, indicating scalability bottlenecks.
Tackling Bottlenecks for Scalability
- Optimize Database Interactions: This involves refining SQL queries, using efficient data access patterns, and implementing proper indexing.
- Minimize Network Traffic: Techniques like data compression, efficient data serialization, and use of asynchronous communication can reduce the strain on network resources.
- Manage Resource Contention: Proper resource allocation and concurrency management can prevent processes from competing over resources.
- Refactor Application Design: Moving from a monolithic to a microservices architecture can improve scalability by distributing the load across multiple, smaller services.
- Caching: Implementing caching mechanisms can reduce the load on databases and external services by temporarily storing frequently accessed data.
- Use Load Balancers: Distributing requests across multiple servers can prevent any single server from becoming overwhelmed.
Addressing scalability bottlenecks in ASP.NET applications requires a multifaceted approach, involving everything from database optimization to application design. By understanding how increased user load affects performance and taking steps to mitigate these impacts, developers can ensure their applications are not only functional but also scalable.
Strategies for Code Optimization and Efficiency in ASP.NET
Best Practices for Writing Scalable ASP.NET Code
In ASP.NET development, writing scalable code is a foundational step towards building applications that can handle increased loads gracefully. Here are key best practices for code optimization:
- Efficient Memory Management: Avoid memory leaks by properly managing resources. Utilize using statements and dispose patterns where applicable.
- Asynchronous Programming: Implement async and await keywords for non-blocking operations, especially in I/O-bound processes, to prevent thread pool exhaustion.
- State Management: Minimize the use of session state or opt for out-of-process storage methods to reduce the memory footprint on the server.
- Minimize Server-Side Processing: Offload processing to the client side where possible, reducing server load and improving response times.
- Use Caching Wisely: Implement caching to store frequently accessed data, reducing database calls and improving response times.
- Optimize Database Access: Use Entity Framework efficiently, avoid N+1 queries, and use stored procedures or LINQ queries judiciously.
- Application Monitoring and Profiling: Regularly monitor application performance and use profiling tools to identify and address inefficiencies in the code.
Techniques to Minimize Code-Related Scalability Issues
Technique | Description | Impact on Scalability |
---|---|---|
Lazy Loading | Load only the necessary data when it’s needed. | Reduces initial load on the database and memory usage. |
Dependency Injection | Implementing inversion of control for better manageability and testing. | Improves application maintainability, aiding in scalability. |
Modular Design | Break down the application into smaller, manageable modules. | Facilitates scalability by allowing individual modules to scale as needed. |
API Rate Limiting | Implement limits on API calls to manage the load. | Prevents system overloads and ensures equitable resource distribution. |
Optimizing code for efficiency and scalability is a critical aspect of ASP.NET development. By adhering to best practices like efficient memory management, asynchronous programming, and wise use of caching, developers can significantly enhance application performance. Additionally, employing techniques like lazy loading, dependency injection, and modular design helps in minimizing code-related scalability issues. Regular application monitoring and profiling are also essential in identifying areas for improvement. These strategies collectively contribute to building scalable ASP.NET applications that perform well under varying loads and are prepared for future growth.
Load Balancing and System Affinity in ASP.NET
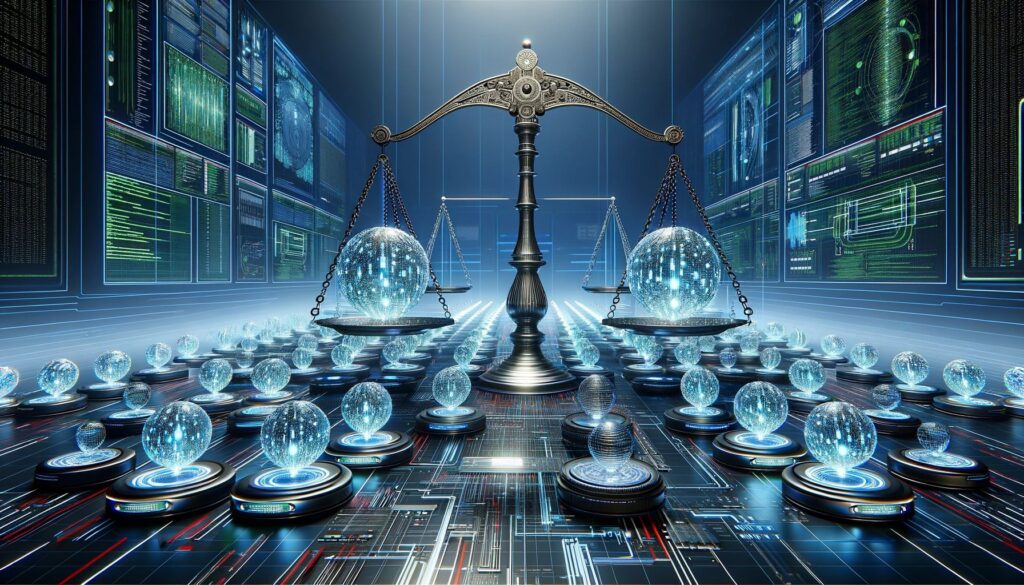
Role of Load Balancing in Managing Traffic
Load balancing is a critical strategy in ASP.NET scalability, designed to distribute incoming network traffic across multiple servers. This distribution helps to ensure that no single server bears too much load, preventing potential bottlenecks and improving application responsiveness. Especially in high-traffic scenarios, load balancing plays a pivotal role in maintaining application performance and availability.
Strategies for Effective Load Distribution
- Round Robin Method: This is one of the simplest forms of load balancing, where requests are distributed sequentially among the available servers. It’s easy to implement but may not account for the varying capacities of servers.
- Least Connections Method: This approach directs traffic to the server with the fewest active connections, assuming that fewer connections mean less load. It’s more dynamic than round-robin and can better handle uneven server capacities.
- Resource-Based Load Balancing: This strategy considers the actual load (CPU, memory usage) on each server and routes traffic to the server with the most available resources. It requires more sophisticated monitoring but can lead to more efficient load distribution.
- Geographic Load Balancing: This method routes traffic based on the geographic location of the user, directing requests to the nearest server. It’s beneficial for global applications to reduce latency.
System Affinity and Its Implications
System affinity, or session affinity, refers to directing a user’s requests to the same server for the duration of a session. It’s often used in applications that maintain session state on the server. While it simplifies session management, it can undermine the effectiveness of load balancing by potentially overloading certain servers.
Affinity Type | Description | Impact on Load Balancing |
---|---|---|
None | No affinity; requests can go to any server. | Maximizes load balancing efficiency but complicates session state management. |
Soft | Preferred affinity; requests try to go to the same server but can be rerouted. | Balances load distribution with session continuity. |
Hard | Strict affinity; requests must go to the same server. | Ensures session continuity but can limit load balancing effectiveness. |
Load balancing is a cornerstone in the scalability of ASP.NET applications, enabling the efficient distribution of traffic across multiple servers. Different methods like round-robin, least connections, resource-based, and geographic load balancing offer various approaches to managing server loads. System affinity, while useful for session state management, needs careful consideration to not counteract the benefits of load balancing. By implementing these strategies thoughtfully, ASP.NET developers can enhance application performance and reliability, even under high traffic conditions.
Data Management and Caching Solutions for ASP.NET Scalability
Efficient Data Management Strategies
In ASP.NET development, handling database scalability is a key challenge. Effective strategies include:
- Database Partitioning: Dividing the database into smaller sections for more efficient data management.
- Database Indexing: Implementing indexes to improve query performance while considering the impact on write operations.
- Normalization vs. Denormalization: Balancing data redundancy reduction and query complexity based on specific application needs.
- Scalable Database Architectures: Using cloud-based solutions that offer built-in scalability features, suitable for ASP.NET applications.
Caching Techniques for Enhanced Performance
Caching is crucial for reducing database load and speeding up data retrieval:
- In-Memory Caching: Storing data in the server’s memory for rapid access, which helps in reducing the number of database queries.
- Distributed Caching: Using an external cache store, like Redis or Memcached, which is shared across multiple servers. This is particularly useful in load-balanced environments and aids in maintaining data consistency across servers.
- Output Caching: Caching the output of pages or controllers to serve repeated requests quickly. This technique is beneficial for reducing server processing load and improving response times for static or semi-static content.
- Data Caching: Specifically caching data objects or query results that are frequently used. This minimizes the need for repeated database queries, improving data retrieval times.
Best Practices in Caching
- Implementing cache invalidation is crucial to maintain data accuracy.
- Utilizing cache dependencies helps automatically update related cache entries when data changes.
- Setting appropriate expiration times for cached items balances between data freshness and performance gains.
Data management and caching are key to scaling ASP.NET applications efficiently. By employing database strategies and various caching techniques, developers can effectively handle increased user loads, ensuring the application remains responsive and robust.
Designing for Cloud-Native Scalability in ASP.NET
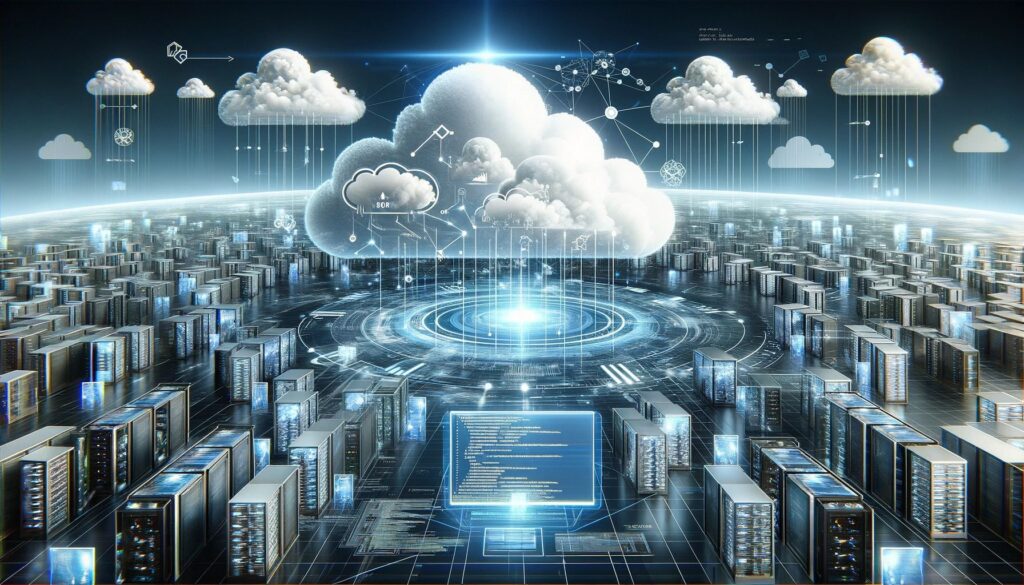
Embracing Cloud Technologies for Scalability
Cloud-native design is pivotal for scalable ASP.NET applications. This approach includes:
- Microservices Architecture: Breaking down applications into smaller, scalable services.
- Containerization: Using Docker-like containers for consistent deployment and easier scaling.
- Serverless Computing: Leveraging AWS Lambda or Azure Functions for scalable, event-driven code execution.
- Elasticity and Auto-Scaling: Utilizing cloud platforms for automatic resource adjustment according to demand.
Key Principles for Scalable Cloud-Native Applications
- Statelessness: Designing services without persistent local state to simplify scaling.
- Decoupling Components: Loosely coupling components for independent scaling and increased fault tolerance.
- Distributed Data Management: Implementing strategies like sharding for effective data distribution.
- CI/CD Practices: Employing Continuous Integration/Continuous Deployment for frequent and reliable updates.
Advantages of Cloud-Native Scalability
- Flexibility: Adapting to changing demands with minimal manual intervention.
- Cost-Efficiency: Only paying for used resources, especially in serverless models.
- High Availability: Leveraging cloud infrastructure for robust, redundant systems.
- Global Reach: Deploying near users to reduce latency and enhance experiences.
Cloud-native principles are essential in ASP.NET for building scalable, resilient applications. By incorporating microservices, containerization, and serverless computing, and adhering to statelessness and decoupling, developers can craft applications that efficiently scale and adapt to user demands.
Conclusion: Future of Scalability in ASP.NET
As we’ve explored throughout this article, the future of scalability in ASP.NET is intrinsically linked to a multitude of factors ranging from efficient code optimization to advanced cloud-native architectures. The landscape of web development is continually evolving, and ASP.NET developers must embrace these changes, adapting their strategies to meet the increasing demands of users and the complexity of modern systems. By understanding and implementing various scalability techniques — from optimizing database interactions and employing effective caching mechanisms to leveraging the power of cloud technologies — developers can ensure that their ASP.NET applications not only meet the current demands but are also poised to handle future challenges with resilience and agility. This comprehensive approach to scalability is key to building robust, efficient, and scalable web applications that stand the test of time and technology.