Introduction to Advanced Data Handling in ASP
The realm of web development has seen significant evolution over the years, with ASP (Active Server Pages) emerging as a pivotal technology in this landscape. Initially developed by Microsoft, ASP has grown to become an essential tool for developers seeking to create dynamic, data-driven web applications. This article delves into the advanced techniques of data handling in ASP, offering insights into how these methods can revolutionize the way web applications process, manage, and present data.
The Evolution of ASP
ASP’s journey began as a simple scripting environment aimed at creating dynamic web pages. However, with the advent of ASP.NET, it transformed into a robust framework, capable of handling complex web applications. ASP.NET’s power lies in its ability to seamlessly integrate with the .NET framework, bringing a multitude of libraries and tools for sophisticated data handling.
The Significance of Data Handling in Web Development
Data handling is at the heart of modern web development. It involves not just the storage and retrieval of data, but also its efficient processing and secure transmission. In the context of ASP, data handling becomes even more crucial as it directly impacts the performance, scalability, and security of web applications.
ASP and Modern Data Handling Techniques
Modern data handling in ASP encompasses a range of techniques and practices. From employing advanced data models to integrating cutting-edge frameworks like the Entity Framework, ASP developers have a plethora of tools at their disposal. These tools and techniques not only enhance the efficiency of data handling but also ensure that applications can scale and adapt to the ever-changing demands of the digital world.
The Role of ASP in Dynamic Web Application Development
ASP plays a crucial role in the development of dynamic web applications. Unlike static websites, dynamic applications require constant interaction with databases and other data sources. ASP provides a seamless interface for this interaction, enabling developers to create responsive, data-centric applications.
Understanding ASP Data Models
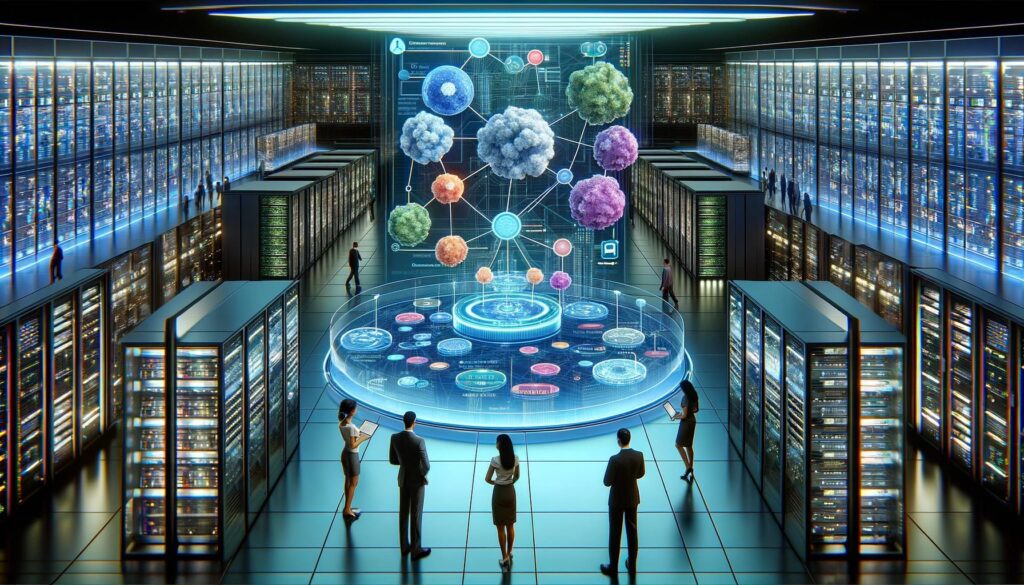
Data models serve as the blueprint for managing and organizing data in any web application. In ASP, these models are crucial for ensuring data is handled efficiently and effectively. This section explores the different data models used in ASP, shedding light on their roles, benefits, and applications.
The LINQ to SQL Model
LINQ to SQL is a core data modeling approach in ASP.NET. It provides a run-time infrastructure for managing relational data as objects. This model translates language-integrated queries into SQL queries, simplifying database operations. It’s particularly effective for applications that require direct, fine-tuned control over SQL queries while still offering the simplicity of object-oriented programming.
The ADO.NET Model
ADO.NET stands for ActiveX Data Objects .NET, and it’s another vital data handling model in ASP.NET. It’s designed for disconnected data architecture, meaning data can be accessed and manipulated without a constant database connection. This model is highly scalable and is ideal for applications that handle large datasets and require a high degree of performance optimization.
Entity Framework Advancements
The Entity Framework (EF) has emerged as a cornerstone in ASP.NET for data handling, thanks to its advanced features and optimizations. This section delves into the key advancements in the Entity Framework, highlighting how these enhancements have streamlined data management in ASP applications.
EF Core: The Modern Iteration
EF Core, the latest version of Entity Framework, represents a significant leap forward. Designed to be lightweight, extensible, and cross-platform, EF Core brings performance improvements and new features that cater to modern application requirements. Its ability to support a wide range of database engines enhances its versatility in various development scenarios.
Performance Improvements
One of the main focuses of EF Core is performance optimization. It includes several improvements, such as:
- Batched Saves: Allows multiple operations to be combined into a single round trip to the database, reducing the overhead of database interactions.
- Query Optimization: EF Core’s query processor is more efficient, translating queries into more optimized SQL statements.
- Lazy Loading: Enables entities to be automatically loaded from the database when accessed, reducing initial load times.
Code-First Approach
EF Core strengthens the code-first approach, allowing developers to define their database schema directly in the code without the need for an external designer or configuration files. This approach enhances developer productivity and makes it easier to maintain and evolve the database schema alongside the application code.
Migrations and Database Updates
EF Core simplifies database schema evolution with its migration feature. Migrations allow developers to apply incremental changes to the database schema and easily propagate these changes across different environments, ensuring consistency and ease of deployment.
Enhanced LINQ Capabilities
EF Core has expanded its LINQ (Language Integrated Query) capabilities, providing more intuitive and powerful ways to query and manipulate data. This includes better support for complex queries, group joins, and asynchronous query execution, making data operations more efficient and expressive.
Optimizing Data Access Performance in ASP
Efficient data access is crucial for the performance of ASP applications. This section focuses on the techniques and best practices for enhancing data access efficiency, ensuring fast and reliable application performance.
- Caching Strategies
Caching is a vital technique in ASP.NET, encompassing output caching, which stores page or component responses for quicker subsequent requests; data caching, involving the storage of objects like datasets to reduce database calls; and distributed caching, particularly useful for web farms, where data is shared across multiple servers. - Efficient Database Interaction
Optimizing database interactions is key to performance. This includes minimizing round trips by fetching only necessary data, using stored procedures for faster execution, and employing connection pooling to reuse existing connections, reducing the overhead of opening new ones for each request. - Asynchronous Programming
Asynchronous programming in ASP.NET is critical for handling multiple requests concurrently, improving throughput. This non-blocking approach to data operations enhances overall application responsiveness. - Entity Framework Core Optimizations
Within EF Core, several optimizations can be applied, such as disabling query tracking for read-only operations, eager loading to avoid the N+1 query problem, and proper indexing in the database to improve query performance. - Performance Monitoring and Profiling
Regular application monitoring and profiling using tools like MiniProfiler, Glimpse, or ASP.NET Core’s built-in diagnostics are essential to identify and address performance bottlenecks. - Best Practices for Data Modeling
Effective data modeling involves normalization to reduce redundancy and improve integrity, and choosing appropriate data types for fields to enhance storage efficiency and query performance.
Handling Large Datasets in ASP Applications
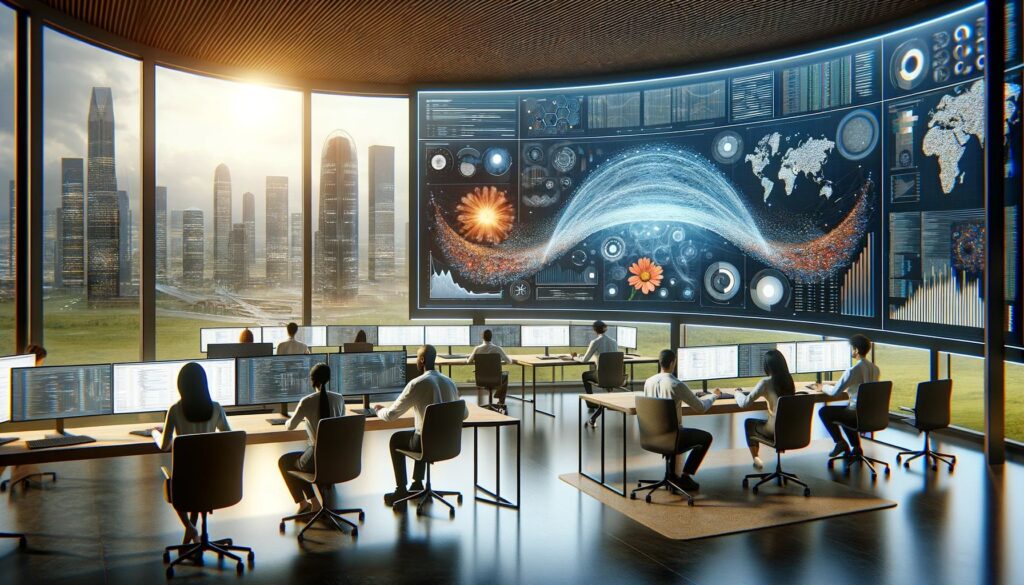
Dealing with large datasets is a common challenge in ASP applications. Efficient management and processing of these datasets are crucial for maintaining performance and scalability.
Strategies for Large Dataset Management
Paging Techniques: Implementing server-side paging is essential when displaying large datasets. Instead of loading all data at once, load only a subset corresponding to the current page view. This can significantly reduce the load time and memory usage.
Example Code Snippet for Paging in ASP.NET:
public ActionResult LargeDataset(int page = 1)
{
int pageSize = 10; // Number of items per page
var pagedData = dbContext.LargeData.OrderBy(d => d.Id)
.Skip((page - 1) * pageSize)
.Take(pageSize).ToList();
return View(pagedData);
}
Efficient Querying: Use LINQ or raw SQL queries optimized for performance. Avoid retrieving unnecessary columns or rows that are not required for the specific operation.
Asynchronous Data Operations: Employ asynchronous programming to handle data operations without blocking the main execution thread. This enhances the application’s responsiveness, especially when dealing with large volumes of data.
Using Data Partitioning: Splitting data into smaller, manageable partitions can improve query performance and scalability. Data partitioning strategies can be applied both at the database level and within the application logic.
Optimizing Indexing: Proper indexing in the database is crucial for quick data retrieval. Analyze query patterns and index the frequently queried columns to speed up search operations.
Memory Management: Monitor and manage memory usage carefully, especially when processing large data sets. Utilize garbage collection and object pooling techniques to maintain optimal memory usage.
Securing Data in ASP: Best Practices
Data security is paramount in ASP applications, especially when handling sensitive information. Employing robust security measures not only protects data but also builds trust with users. This section outlines essential practices for ensuring data security in ASP applications.
Implementing Robust Authentication and Authorization
- Utilize ASP.NET’s built-in identity system for user authentication.
- Implement role-based authorization to restrict access to sensitive data.
Example Code for Role-Based Authorization:
[Authorize(Roles = "Admin")]
public ActionResult ManageData()
{
// Data management logic for admins
}
Data Encryption and Hashing
- Encrypt sensitive data, such as user credentials and personal information.
- Use hashing for storing passwords. ASP.NET Core Identity uses secure hashing algorithms like bcrypt.
SQL Injection Prevention
- Use parameterized queries or Entity Framework to prevent SQL injection attacks.
- Avoid raw SQL queries; if necessary, ensure inputs are sanitized.
Cross-Site Scripting (XSS) Protection
- Utilize ASP.NET’s built-in mechanisms to automatically encode output.
- Be cautious when dealing with user input and employ content security policies.
Secure Data Transmission
- Enforce HTTPS to secure data in transit.
- Utilize SSL/TLS protocols for encrypting data exchanged between the client and server.
Regular Security Audits
- Conduct regular security audits and vulnerability assessments.
- Keep up-to-date with the latest security patches and updates for ASP.NET.
Integrating NoSQL Databases with ASP
The integration of NoSQL databases in ASP environments caters to applications requiring flexibility, scalability, and performance in handling unstructured or semi-structured data. This section focuses on how NoSQL databases can be effectively integrated into ASP applications.
Understanding NoSQL Databases
- NoSQL databases like MongoDB, Cassandra, or Redis, offer an alternative to traditional relational databases, especially for big data applications and real-time web applications.
- They are known for their schema-less data models, horizontal scalability, and efficient handling of large volumes of data.
Integration Strategies
- Choosing the Right NoSQL Database: Depending on the application’s requirements, choose a NoSQL database that best fits its data handling needs.
- Using ORM Tools for NoSQL: Tools like Entity Framework Core now support NoSQL databases, providing a familiar interface for data operations.
- API-Based Data Access: RESTful APIs can be used to interact with NoSQL databases, allowing for a flexible and decoupled architecture.
Example Code for Connecting to a MongoDB Database in ASP.NET:
public class BookService
{
private readonly IMongoCollection<Book> _books;
public BookService(IBookstoreDatabaseSettings settings)
{
var client = new MongoClient(settings.ConnectionString);
var database = client.GetDatabase(settings.DatabaseName);
_books = database.GetCollection<Book>(settings.BooksCollectionName);
}
public List<Book> Get() => _books.Find(book => true).ToList();
}
Performance Considerations
- NoSQL databases excel in scenarios with high read/write loads and can significantly improve performance for specific types of queries.
Data Consistency and Availability
- Understand the trade-offs between consistency, availability, and partition tolerance (CAP theorem) when working with NoSQL databases.
Conclusion
As we look ahead, ASP’s data handling is set to be influenced by advancements in cloud computing, AI, and ML, enhancing efficiency and user experience. Trends like serverless architectures, microservices, and the integration of IoT devices will further revolutionize data management, emphasizing modularity and real-time processing. Additionally, the increasing focus on data privacy will shape secure data handling practices. Staying updated with these developments is key for developers to keep their ASP applications competitive and relevant in the rapidly evolving digital landscape.