Explore advanced PowerShell scripting in this introductory guide. Learn essential techniques for efficient automation, scripting best practices, and harness the full power of PowerShell for managing and automating your IT tasks.
Windows PowerShell: A Brief Overview
PowerShell, Microsoft’s task automation framework, integrates an advanced command-line shell with a scripting language built on .NET technology. It empowers IT professionals and system administrators to control and automate the administration of Windows and applications running on Windows Server environments.
Evolving Beyond Basic Scripting
Initially perceived as just a powerful command-line device, PowerShell has evolved. It now offers advanced scripting capabilities, allowing users to create complex scripts that perform a wide range of functions, from simple automation to complex data processing tasks. The versatility of PowerShell lies in its ability to integrate with various technologies, including cloud services, GUI applications, and network systems, making it an indispensable device for modern IT operations.
The Importance of Advancing Scripting Skills
As technology landscapes become increasingly complex, the need for advanced scripting skills is more pronounced. Mastering PowerShell scripting allows IT professionals to:
- Automate Repetitive Tasks: Reducing manual intervention for routine tasks, thereby enhancing efficiency.
- Manage Systems Effectively: From server configurations to deploying updates, PowerShell scripts provide a scalable way to manage systems across varied environments.
- Integrate with Diverse Technologies: Whether it’s Azure cloud services or local Windows servers, PowerShell scripts can interact seamlessly, providing a unified scripting solution.
- Enhance Security: Automating security checks and responses with PowerShell scripts can significantly improve the security posture of an organization.
- Customize Solutions: Advanced scripting enables tailored solutions for specific organizational needs, be it data analysis, system configuration, or user management.
Understanding PowerShell’s Advanced Capabilities
Delve into PowerShell’s advanced capabilities with this comprehensive guide. Gain insights into scripting, automation, module development, and unleash the full potential of PowerShell for managing complex IT environments and tasks.
Exploring Capabilities Beyond Basic Scripting
PowerShell’s scripting capabilities extend far beyond basic command execution, offering a powerful system for developing complex automation scripts. Advanced PowerShell scripting involves utilizing its full range of functionalities, including cmdlets, modules, and the .NET framework, to create scripts that can handle intricate tasks and workflows. This section explores some of these advanced features that distinguish PowerShell from traditional scripting environments.
Integration with GUI for Enhanced User Interaction
One of PowerShell’s standout capabilities is its ability to integrate with Graphical User Interfaces (GUIs). This integration allows scripts to interact with users in a more intuitive way, making it easier to collect input, display information, and guide users through complex processes. Here are a few ways PowerShell achieves this:
- Windows Forms and WPF: PowerShell can use .NET Windows Forms and Windows Presentation Foundation (WPF) to create custom dialog boxes, windows, and other graphical elements.
- Input Dialogs: Simple input dialogs can be created for quick user input.
- Progress Bars: Scripts can include progress bars to give users feedback during long-running operations.
Example: Creating a Simple Input Dialog in PowerShell
Add-Type -AssemblyName Microsoft.VisualBasic
Add-Type -AssemblyName System.Windows.Forms
$userInput = [Microsoft.VisualBasic.Interaction]::InputBox("Enter your input", "Input Dialog", "Default Text")
This script snippet demonstrates creating a basic input dialog using the .NET Framework, illustrating how PowerShell can leverage GUI elements for user interaction.
Writing Advanced Functions and Scripts in PowerShell
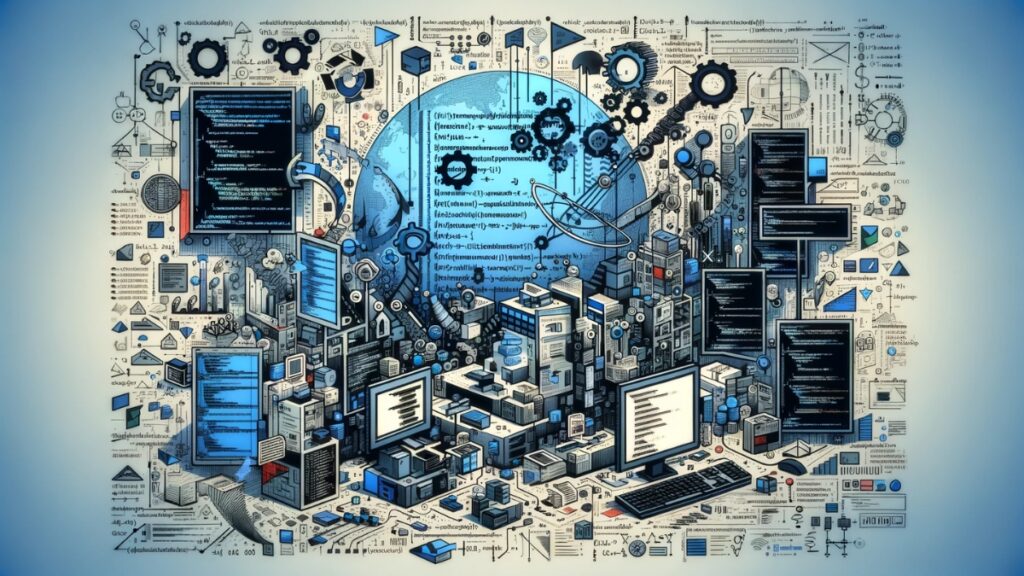
Learn to craft advanced PowerShell functions and scripts. This comprehensive guide covers parameterization, error handling, module development, and more, enabling you to create powerful and efficient automation solutions.
Creating Robust, Reusable Scripts
Advanced PowerShell scripting transcends simple command execution, focusing on creating robust, reusable scripts that can be easily maintained and adapted. This requires a structured approach to scripting, encompassing best practices in coding, error handling, and modular design.
1. Modular Script Design:
- Purpose: Breaking down scripts into smaller, reusable modules or functions.
- Benefits: Enhances readability, simplifies debugging, and promotes reuse.
- Implementation: Use functions to encapsulate specific tasks, making scripts more organized and easier to update.
2. Error Handling and Debugging:
- Purpose: To ensure scripts run smoothly and handle unexpected situations gracefully.
- Techniques: Utilize try-catch blocks, error logging, and PowerShell’s built-in debugging devices.
- Example:
try {
# Attempt to execute a command
} catch {
# Handle errors here
}
3. Parameterization:
- Purpose: To make scripts flexible and adaptable to different scenarios.
- Implementation: Use parameters to allow users to input or modify values without altering the script code.
- Example: Defining a function with parameters:
function Get-Data ($param1, $param2) {
# Function code here
}
Best Practices in Script Writing
To enhance the effectiveness and reliability of PowerShell scripts, adhere to these best practices:
- Consistent Coding Style: Maintain a consistent coding style for ease of reading and maintenance. This includes naming conventions, indentation, and commenting.
- Thorough Documentation: Document each script and function, explaining its purpose, parameters, return values, and usage examples.
- Testing and Validation: Rigorously test scripts in different environments and scenarios. Include validation checks for parameters to prevent errors due to invalid inputs.
- Version Control: Use version control systems like Git to track changes, collaborate with others, and maintain a history of script development.
Dynamic Parameters and Dynamic-Param Usage in PowerShell
Enhancing Scripts with Dynamic Parameters
Dynamic parameters in PowerShell add a layer of flexibility to your scripts, enabling them to adapt based on runtime conditions. Unlike regular parameters that are static and defined at the time of function or script creation, dynamic parameters can be added based on the context or the values of other parameters.
Understanding Dynamic Parameters
- Definition: Dynamic parameters are script parameters that are added at runtime based on conditions or other parameter values.
- Usage: They are particularly useful in scenarios where the script needs to provide options based on user input or environmental conditions.
Creating Dynamic Parameters with Dynamicparam
The Dynamicparam keyword in PowerShell allows the addition of parameters dynamically. Here’s a simplified structure of how it’s implemented:
function Get-DynamicData {
[CmdletBinding()]
Param(
[Parameter(Mandatory=$true)]
$MainParameter
)
DynamicParam {
# Create dynamic parameters based on $MainParameter value
}
begin {
# Script block to process parameters
}
}
In this structure, the DynamicParam block defines the conditions under which additional parameters are created and added to the function.
Practical Applications
Dynamic parameters are invaluable in scripts that need to adjust their behavior based on external factors. For instance, a script that interacts with different databases might offer different parameter options based on the selected database type.
Effective Loops and Performance in Scripting
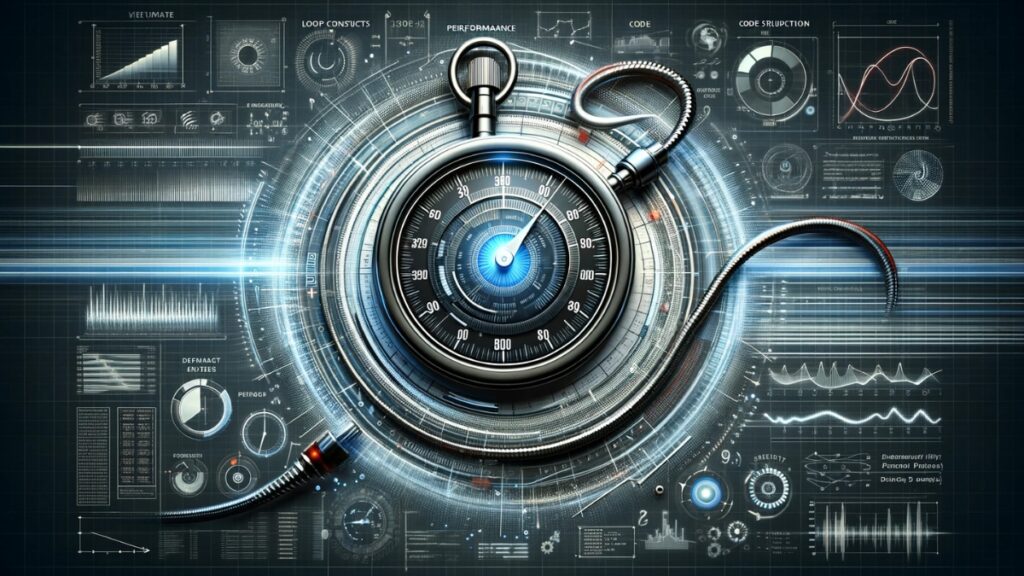
Boost scripting efficiency with effective loops and performance techniques. This guide provides insights into optimizing PowerShell scripts for faster execution, enhancing your ability to manage tasks efficiently in complex IT environments.
Optimizing Scripts with Effective Loop Structures
In PowerShell, loops are fundamental constructs used for iterating over collections or repeating tasks until a condition is met. Effective use of loops can significantly enhance the performance and efficiency of scripts.
Types of Loops in PowerShell
PowerShell supports several types of loops, each with its specific use case:
1. For Loop: Ideal for iterating a set number of times, often used when the number of iterations is known beforehand.
for ($i = 0; $i -lt 10; $i++) {
# Code to execute
}
2. Foreach Loop: Used to iterate over each item in a collection or array.
foreach ($item in $collection) {
# Code to execute for each item
}
3. While Loop: Executes as long as a specified condition is true. Useful when the number of iterations is not known in advance.
while ($condition) {
# Code to execute as long as condition is true
}
4. Do-While and Do-Until Loops: These loops will execute the code block at least once before checking the condition.
do {
# Code to execute
} while ($condition)
Performance Considerations for Large-Scale Scripting
When dealing with large datasets or complex tasks, loop performance becomes crucial. Here are some tips to enhance loop performance in PowerShell scripts:
- Limit the Use of Pipelines in Loops: While pipelines are a powerful feature in PowerShell, their overuse inside loops can impact performance. Use direct method calls where possible.
- Avoid Unnecessary Operations Inside Loops: Minimize the number of operations performed inside a loop, especially resource-intensive ones.
- Use Parallel Processing: For independent iterations, consider using parallel processing techniques to speed up execution.
Documenting Functions and Scripts for Clarity
The Importance of Documentation in PowerShell Scripts
Effective documentation is a critical aspect of advanced PowerShell scripting. It enhances the readability, maintainability, and usability of scripts, especially in environments where scripts are shared or used by multiple people. Well-documented scripts allow others (and yourself at a later time) to understand the purpose, functionality, and usage of the script with ease.
Methods for Clear and Effective Documentation
- Inline Comments: These are brief explanations within the script code, providing context or explaining the purpose of specific sections or lines of code.
- Function Help Comments: PowerShell supports a special comment syntax for documenting functions, which can be displayed using the Get-Help cmdlet. This includes descriptions of the function, parameters, examples, inputs, and outputs.
- External Documentation: For complex scripts or modules, external documentation (like a README file) may be necessary to provide a comprehensive overview, including setup instructions, dependencies, and more detailed usage guidelines.
Documenting a PowerShell Function – An Example
<#
.SYNOPSIS
Short description of the function.
.DESCRIPTION
A more detailed description of the function and its behavior.
.PARAMETER Param1
Description of the first parameter.
.PARAMETER Param2
Description of the second parameter.
.EXAMPLE
An example of how to use the function.
.INPUTS
Inputs to the function, if any.
.OUTPUTS
Expected output of the function.
.NOTES
Additional information about the function.
.LINK
Reference to more information or related scripts.
#>
function My-PowerShellFunction {
# Function code goes here
}
PowerShell and Azure: Automating Cloud Environments
Leveraging PowerShell for Azure Cloud Services
PowerShell scripts can be incredibly effective for managing and automating tasks in Azure, Microsoft’s cloud computing service. By utilizing Azure-specific cmdlets, scripts can automate a wide range of cloud operations, from deploying virtual machines to managing cloud resources.
Automating Lab Deployment in Azure IaaS
One of the key applications of PowerShell in Azure is automating the deployment of lab environments in Azure Infrastructure as a Service (IaaS). This can greatly simplify the setup of test environments, development labs, or training setups.
- Scripting Virtual Machine Creation: PowerShell scripts can automate the process of setting up virtual machines, including configuring network settings, storage options, and installing necessary software.
- Resource Group Management: Scripts can manage Azure resource groups, organizing resources for easy management and cost tracking.
Migrating On-Premises Websites and Databases to Azure
Another critical use case is the migration of on-premises applications, such as websites and databases, to Azure. PowerShell can streamline this migration process by automating various tasks.
- Database Migration: Automating the transfer of database data from on-premises servers to Azure SQL Database or other Azure-based database services.
- Website Deployment: Scripting the deployment of web applications to Azure App Service or other Azure hosting solutions.
Summary and Advanced Scripting Resources
As we conclude our exploration of “Windows PowerShell: Advanced Scripting Techniques,” let’s recap the key points covered in this series and look at some resources for further learning.
Recap of Key Points Covered:
- Advanced Scripting Capabilities: We delved into PowerShell’s advanced capabilities, highlighting its power beyond basic scripting, especially its GUI integration and interaction with Azure.
- Writing Advanced Functions and Scripts: Emphasis was placed on creating robust, reusable, and well-documented scripts, following best practices in scripting.
- Dynamic Parameters and Dynamic-Param: We explored the flexibility and adaptability of scripts through dynamic parameters, adding a layer of sophistication to PowerShell scripts.
- Effective Loop Structures: The importance of efficient loop structures was discussed, focusing on their impact on script performance, especially in large-scale scripting.
- Documentation: The significance of documenting scripts for clarity and maintainability was highlighted, emphasizing best practices in both inline and external documentation.
- PowerShell in Azure: The series explored the use of PowerShell in automating tasks within Azure, showcasing its effectiveness in cloud environment management.
Further Resources for Advanced PowerShell Scripting:
- PowerShell Documentation: The official PowerShell documentation by Microsoft provides comprehensive guides, cmdlet references, and tutorials.
- Online Courses and Tutorials: Websites like Pluralsight, Udemy, and Coursera offer courses ranging from beginner to advanced levels in PowerShell scripting.
- Books: Books like “Windows PowerShell in Action” and “Learn Windows PowerShell in a Month of Lunches” are excellent resources for in-depth learning.
- Community Forums: Interface like Stack Overflow and the PowerShell subreddit offer a community-based approach to learning where you can ask questions and share knowledge.
- Script Repositories: Websites like GitHub host a plethora of PowerShell scripts and modules, providing real-world examples and community contributions.
- Official Microsoft Learning Paths: Microsoft offers structured learning paths and modules specifically for PowerShell on Microsoft Learn.
Final Thoughts
Advanced scripting in Windows PowerShell showcases its remarkable power and versatility. From automating tasks to managing complex environments, it’s a vital equipment for modern IT. Mastering efficient scripting, GUI integration, and Azure usage highlights its role in simplifying and streamlining IT operations. As technology evolves, enhancing scripting skills remains crucial, keeping PowerShell relevant and valuable in the IT landscape.
Exploring PowerShell’s advanced capabilities highlights the need for continuous learning. Abundant resources, like official documentation and community forums, support mastering PowerShell. Whether automating small tasks or deploying large-scale environments, these skills provide a strong foundation. As IT evolves, PowerShell remains a key equipment for professionals.