Introduction: Elevating Web Interactivity with Advanced Form Handling in ASP.NET
In the dynamic realm of web development, forms stand as the cornerstone of user interaction and data collection. They are the primary interface through which users communicate with web applications, making their design and functionality crucial for a positive user experience.
ASP.NET: A Robust Framework for Dynamic Form Handling
ASP.NET, a robust framework by Microsoft, offers extensive capabilities for creating and managing web forms, pivotal in harvesting user input and steering user engagement. This section highlights the framework’s strengths and how it empowers developers to build sophisticated forms.
Beyond Basics: Why Advanced Form Handling Matters
While basic form handling in ASP.NET might cover rudimentary needs, advanced techniques are essential for handling complex user interactions, ensuring data integrity, and enhancing the overall user experience. This part of the article will emphasize the importance of elevating form handling from basic to advanced levels.
Enhancing User Experience and Security through Advanced Techniques
Mastering advanced form handling techniques in ASP.NET allows developers to construct more interactive, secure, and efficient web applications. This section will outline how advanced form handling impacts both the user experience and the security of web applications.
Understanding Form Basics in ASP.NET
Forms in ASP.NET serve as a fundamental tool for data collection and user interaction. Before diving into advanced techniques, it’s important to grasp the basics of form handling in this versatile framework.
Form Fundamentals in ASP.NET
ASP.NET provides two primary environments for form development: MVC (Model-View-Controller) and Web Pages (Razor). Each offers unique features for form handling:
- MVC: Ideal for building dynamic, rich web applications. It separates the application into three main components – Model, View, and Controller – ensuring a clean separation of concerns. This architecture is particularly beneficial for complex form handling where data manipulation and presentation are distinct.
- Web Pages (Razor): Suited for simpler web applications, Razor syntax in ASP.NET Web Pages allows for embedding server-based code directly within HTML. It simplifies form creation and handling for straightforward scenarios.
Key Components of ASP.NET Forms
- HTML Helpers: These are methods that render HTML in the View component. They simplify the creation of HTML elements like text boxes, dropdown lists, and buttons linked to model properties.
- Model Binding: This feature automatically maps user input from forms to model properties, significantly reducing the amount of boilerplate code required for data transfer.
- Validation: ASP.NET provides robust validation tools, both on the client and server-side, ensuring data integrity and user-friendly error feedback.
Form Action Types: GET and POST Explained

Delving deeper into form handling in ASP.NET, it is crucial to understand the two primary form action types: GET and POST. Each plays a distinct role in how data is managed and transmitted in a web application.
GET Action: Retrieving Data
The GET action in ASP.NET is primarily used for retrieving data. It is characterized by data being appended to the URL as query parameters. This method is typically used for search forms or any scenario where bookmarking or sharing the URL is beneficial.
- Usage: Ideal for non-sensitive data retrieval.
- Visibility: Data is visible in the URL, making it inappropriate for sensitive information.
- Limitations: URL length restrictions can limit the amount of data sent.
POST Action: Submitting Data
On the other hand, the POST action is used for submitting data to the server for processing. This method transmits data as part of the request body, not in the URL, making it more secure for sensitive information.
- Usage: Preferred for submitting forms with sensitive or large amounts of data.
- Security: Better for sensitive data, as it does not appear in the URL.
- Capacity: No restrictions on data length, unlike GET.
Choosing Between GET and POST
The choice between GET and POST depends on the application’s needs:
- GET: Use when the data retrieval aspect is dominant, and there’s no sensitive data involved.
- POST: Opt for this when submitting data, especially when dealing with sensitive information or large datasets.
Enhancing Form Functionality with MVC
In ASP.NET, the Model-View-Controller (MVC) framework brings a sophisticated approach to form handling, providing enhanced functionality and flexibility. MVC architecture, with its clear separation of concerns, allows for more organized and maintainable code, especially in the context of complex forms.
MVC’s Role in Advanced Form Handling
MVC architecture divides the application into three interconnected components, each with a specific responsibility, which is particularly beneficial for form handling:
- Model: Represents the data and the business logic. In forms, it includes validation rules and data models.
- View: Handles the UI aspect. It’s where the form is rendered.
- Controller: Manages user interactions, processes form data, and returns responses.
Advanced Techniques in MVC Form Handling
ViewModel Enrichers: These are used to add additional information or perform processing on the model, which is particularly useful for POST actions. ViewModel Enrichers can simplify form submissions by pre-processing the data.
FormActionResult Class: An innovative approach to managing form submissions in MVC. It helps in structuring the handling of form submissions, covering various scenarios like success or failure.
Example:
public class FormActionResult<T> : ActionResult
{
public Action<T> Handler { get; set; }
public Func<T, ActionResult> SuccessResult;
public Func<T, ActionResult> FailureResult;
// Implementation...
}
Fluent Interface for Form Processing: This technique enhances code readability and intent expression. It involves chaining method calls in a way that resembles natural language, making the code more intuitive.
MVC Form Handling: A Practical Example
Consider a typical form submission scenario in an MVC application:
- Step 1: Form Rendering (GET Action): The user requests a form, which the MVC framework renders using data models and HTML helpers.
- Step 2: Form Submission (POST Action): The user submits the form, which is then processed by the controller. The controller validates the data against the model and decides the next step (success or failure).
- Step 3: Result Handling: Depending on the validation outcome, the controller either redirects to another view (on success) or returns the same view with error messages (on failure).
Advanced Techniques in Form Tag Helpers
In ASP.NET Core, Tag Helpers significantly simplify the process of writing Razor views, especially for form elements. They provide a more readable and HTML-like syntax compared to traditional HTML Helpers, enhancing the development experience and productivity.
Understanding Form Tag Helpers
Tag Helpers transform standard HTML tags into functional server-side code. Some essential Tag Helpers for form handling in ASP.NET Core include:
- Form Tag Helper: Automates the generation of form elements, including the action attribute pointing to MVC controller actions or named routes, and adds anti-forgery tokens for security.
- Form Action Tag Helper: Dynamically generates the
formaction
attribute for<button>
or<input type="image">
elements, controlling the submission endpoint of the form. - Input Tag Helper: Binds
<input>
elements to model properties, automatically generatingid
andname
attributes and setting the appropriate HTML5 input types based on model annotations. - Textarea Tag Helper: Similar to the Input Tag Helper but tailored for
<textarea>
elements, facilitating the handling of multi-line text inputs. - Validation Tag Helpers: Include the
Validation Message Tag Helper
andValidation Summary Tag Helper
, which display field-specific or summary validation messages, enhancing client-side and server-side form validation.
Practical Implementation of Tag Helpers
Here’s a practical example showcasing how these Tag Helpers can be used in a Razor view:
<form asp-action="SubmitForm" method="post">
<input asp-for="Name" type="text" />
<textarea asp-for="Description"></textarea>
<button type="submit">Submit</button>
<span asp-validation-for="Name"></span>
</form>
In this example, the asp-for
attribute in the Input and Textarea Tag Helpers binds these elements to the respective model properties. The Form Tag Helper sets the form’s action attribute to the “SubmitForm” action in the controller. Lastly, the Validation Message Tag Helper displays validation messages for the “Name” field.
Benefits of Using Tag Helpers in Form Handling
- Improved Readability: Tag Helpers maintain the HTML flow of Razor views, making them more readable and maintainable.
- Enhanced Productivity: They reduce the amount of code required to link form elements with model properties and validation logic.
- Seamless Integration: Tag Helpers integrate seamlessly with MVC features, including model binding and validation.
Security Measures in Form Handling
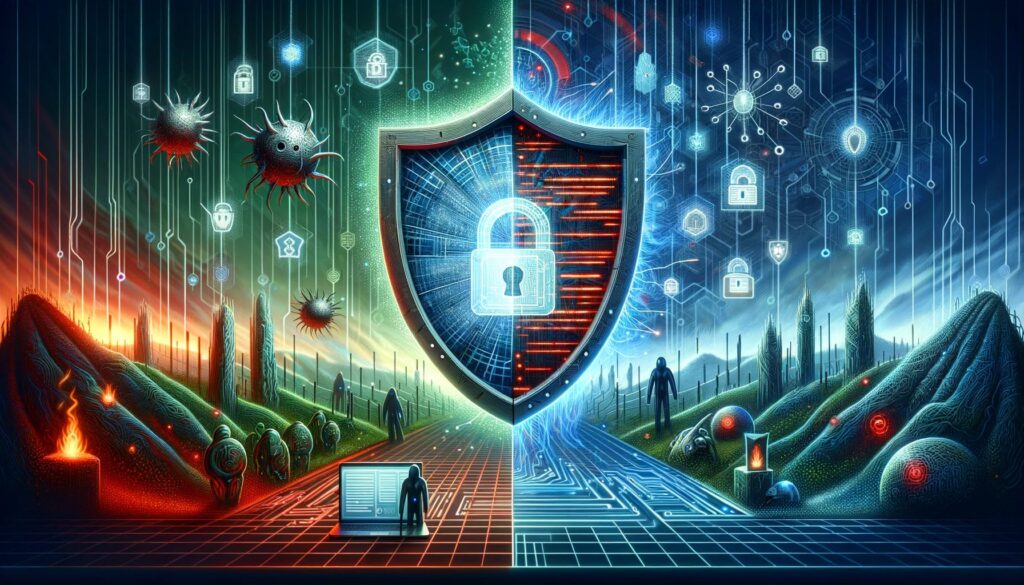
Security is a paramount concern in web application development, and form handling in ASP.NET is no exception. Implementing robust security measures is essential to protect sensitive user data and maintain the integrity of the application.
Cross-Site Request Forgery (CSRF) Protection
One of the key security challenges in form handling is protecting against CSRF attacks. ASP.NET provides built-in mechanisms to counter these threats:
- Anti-Forgery Tokens: ASP.NET uses anti-forgery tokens, automatically included in forms using the Form Tag Helper. These tokens ensure that the form submission is legitimate and originates from the application itself, not an external source.
Data Validation and Sanitization
- Server-Side Validation: Always validate user input on the server side. ASP.NET offers Data Annotation attributes that can be applied to model properties to define validation rules.
- Client-Side Validation: While server-side validation is essential, client-side validation improves user experience by providing immediate feedback. ASP.NET’s Validation Tag Helpers facilitate this process.
- HTML Encoding: Protect against cross-site scripting (XSS) attacks by encoding any HTML content submitted by the user. ASP.NET automatically encodes text content output from server-side code to prevent script injection.
Secure Data Transmission
- HTTPS Protocol: Ensure that all form data is transmitted over a secure HTTPS connection. This encrypts the data during transmission, protecting it from interception or tampering.
- SSL Certificates: Utilize SSL certificates to authenticate the identity of the application and establish a secure connection.
Optimizing Form Performance
Optimizing the performance of forms in ASP.NET is crucial for delivering a smooth and responsive user experience. Efficient form handling not only improves speed but also enhances overall application reliability.
Techniques for Performance Optimization
- Asynchronous Form Processing: Implement asynchronous methods in controllers to handle form submissions. This prevents blocking the main thread, allowing the server to handle more requests simultaneously.
- Minimizing Large Object Allocations: Avoid using large objects in forms, as they can lead to increased garbage collection and memory usage. Instead, opt for smaller, more efficient data structures.
- Efficient Data Binding: Optimize model binding in forms by only binding necessary fields. Over-binding can lead to performance issues and security risks.
- Caching Form Data: Cache static data used in forms, such as dropdown lists sourced from a database, to reduce database calls.
- Client-Side Scripting: Utilize client-side scripting for real-time validation and dynamic form behaviors to reduce server load.
Utilizing Compression and Minification
- Response Compression: Implement response compression techniques in ASP.NET to reduce the size of the data transmitted over the network, thus improving load times.
- Minifying Resources: Minify JavaScript and CSS files used in forms to decrease the size of these assets, enhancing page load performance.
Handling Long-Running Form Processes
Managing long-running tasks in ASP.NET forms is a critical aspect of ensuring application responsiveness and user satisfaction. Proper handling of such tasks is essential to prevent the UI from becoming unresponsive and to provide feedback to the user on the progress of their request.
Strategies for Managing Long-Running Tasks
- Asynchronous Processing: Implement asynchronous controllers and actions in ASP.NET. This approach allows the server to handle other requests while waiting for the long-running task to complete, thus avoiding blocking the UI or server thread.
- Background Services: For tasks that do not require immediate completion or user interaction, consider offloading them to background services. This approach is particularly effective for CPU-intensive tasks or processes that run independently of user actions.
- Real-Time Communication: Use technologies like SignalR for real-time communication with the client. This can be used to provide live updates on the progress of the long-running task, enhancing the user experience.
- Task Queues: Implement a task queue system where long-running operations are queued and processed sequentially or in parallel, depending on the requirement. This helps in managing resource utilization effectively.
- Timeouts and Feedback: Implement timeout logic and provide continuous feedback to the user. This could be in the form of a progress bar or periodic messages, informing the user about the status of their request.
Conclusion
The journey through advanced form handling in ASP.NET illuminates the importance of effective, secure, and efficient form management in web application development. From understanding the basics of GET and POST actions to implementing asynchronous processing and security measures, these practices are pivotal in creating robust, user-friendly, and responsive web applications.
Embracing these advanced techniques not only elevates the functionality of web forms but also ensures a seamless user experience and the integrity of the application. As technology evolves, staying abreast of these practices is essential for any ASP.NET developer committed to crafting high-quality, interactive, and secure web applications. This comprehensive exploration serves as a guide to mastering the art of form handling in ASP.NET, a skill indispensable in the modern web development landscape.