Introduction to ActionScript Security
ActionScript, a programming language used primarily for Adobe Flash applications, has evolved significantly since its inception. Originating as a simple scripting tool for enhancing web interactivity, ActionScript has grown into a robust language capable of creating complex applications. This development journey reflects the advancements in web technologies and the ever-increasing demand for interactive, multimedia-rich web content. Despite the gradual decline in Flash’s popularity due to the rise of HTML5 and mobile-first development, understanding ActionScript remains crucial for maintaining legacy systems and understanding the evolution of web technologies.
Why Security in ActionScript is Paramount
In the realm of programming, security is an omnipresent concern, and ActionScript is no exception. As with any technology used to create web applications, ActionScript is susceptible to a variety of security threats. These range from cross-site scripting (XSS) and cross-site request forgery (CSRF) to more sophisticated types of attacks aimed at exploiting vulnerabilities within the application logic or the Flash player itself.
The security of an ActionScript application is not just about protecting the code; it’s also about safeguarding the data and privacy of its users. With the increasing awareness and regulation around data protection (like GDPR and CCPA), ensuring that applications are secure against potential breaches has become a necessity. This is particularly true for applications handling sensitive user information, where a breach can lead to significant legal and reputational consequences.
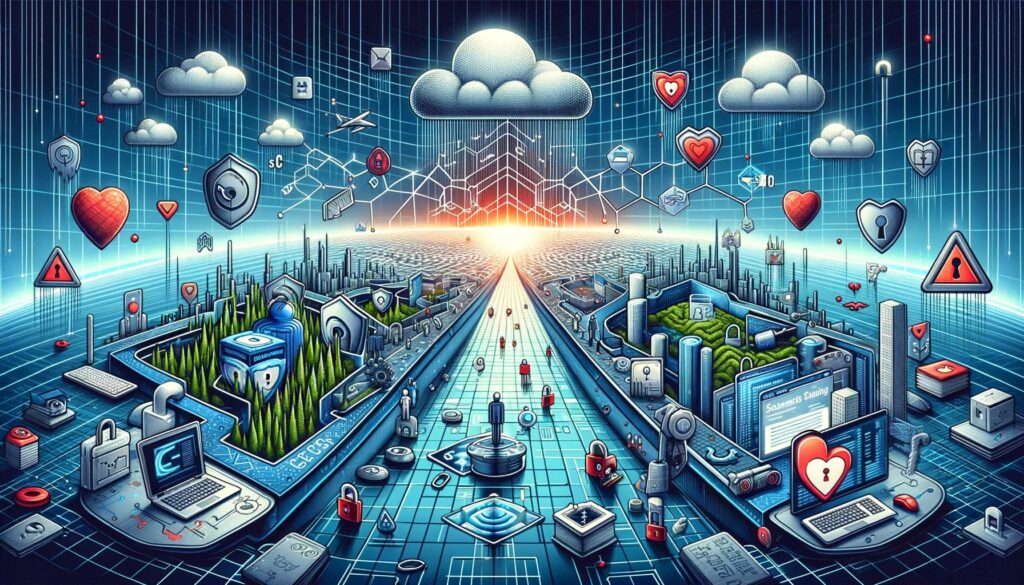
Understanding the Attack Surface in ActionScript Applications
The “attack surface” of an application refers to all the points where an unauthorized user can try to enter data to or extract data from an application. In ActionScript, this could involve:
- Input fields: Where data is entered by the user.
- Network connections: How the application communicates with servers.
- File interactions: How the application reads and writes local or network files.
- External interfaces: Integration with other systems or plugins.
Reducing the attack surface involves identifying these points and implementing robust security measures to protect them. It’s a continuous process of assessing and mitigating risks as new vulnerabilities are discovered and technology evolves.
Key Security Considerations in ActionScript
- Code Execution Vulnerabilities: This includes evaluating the risk of executing malicious code through ActionScript. Since Flash applications run on the client side, they can be used as vectors for various attacks.
- Data Storage and Transmission: Ensuring that sensitive data is stored and transmitted securely, using encryption and secure protocols, is vital. This includes considering how data is managed within the application and how it interacts with external servers and APIs.
- Third-party Libraries and Plugins: Dependencies on external libraries and plugins can introduce vulnerabilities. Regularly updating these and ensuring they come from reliable sources is essential for maintaining security.
- Compliance with Security Standards: Following best practices and standards, such as OWASP guidelines, helps in maintaining a high security standard in ActionScript development.
Common Vulnerabilities in ActionScript Programming
Understanding the Landscape of Security Threats
ActionScript, like any other programming language, is susceptible to a range of security vulnerabilities. These vulnerabilities can have significant implications for both the developers and the users of ActionScript applications. To ensure robust security, it’s crucial to recognize these vulnerabilities and adopt proactive measures to mitigate them.
Key Vulnerabilities in ActionScript
- Broken Authentication: This occurs when authentication credentials are compromised, allowing attackers to impersonate users and hijack sessions. In the context of ActionScript, this could involve manipulating Flash-based authentication systems.
- SQL Injection: A prevalent threat in web development, SQL injection attacks can also affect ActionScript applications that interact with databases. Attackers can execute unauthorized database commands by injecting malicious SQL code, potentially accessing or manipulating sensitive data.
- Cross-Site Scripting (XSS): ActionScript applications are vulnerable to XSS attacks, where malicious scripts are injected into web pages. These scripts can steal sensitive data like authentication cookies when executed in a victim’s browser.
- Cross-Site Request Forgery (CSRF): In CSRF attacks, authenticated users are tricked into executing actions they didn’t intend to, like changing personal information or initiating transactions, due to the application’s trust in user-initiated requests.
- Arbitrary File Uploads: Applications that allow users to upload files without strict validation are at risk. Attackers can upload files with arbitrary extensions, potentially leading to command injection or other malicious activities.
- XML External Entity (XXE) Injection: This type of attack involves exploiting XML processors to read arbitrary files from the server, leading to information disclosure or Denial of Service (DoS) attacks.
- Security Misconfiguration: Misconfigured security settings in ActionScript applications can expose them to various threats. This includes inadequate access controls, unprotected files and directories, and misconfigured HTTP headers.
- Server-Side Request Forgery (SSRF): SSRF attacks occur when an attacker abuses the functionality of a server, causing it to send a crafted request to an unintended destination. This can lead to sensitive data exposure or internal system attacks.
Addressing These Vulnerabilities
- Regular Security Audits: Conducting regular security audits and vulnerability scans can help identify and address weaknesses in ActionScript applications.
- Secure Coding Practices: Emphasizing secure coding practices, such as input validation and proper error handling, can prevent many of these vulnerabilities from being exploited.
- Keeping Up with Security Updates: Regularly updating ActionScript and its related libraries and frameworks can protect against known vulnerabilities.
- User Education: Educating users about security risks, such as phishing attacks and secure password practices, can also play a vital role in safeguarding ActionScript applications.
Implementing HTTPS in ActionScript Applications
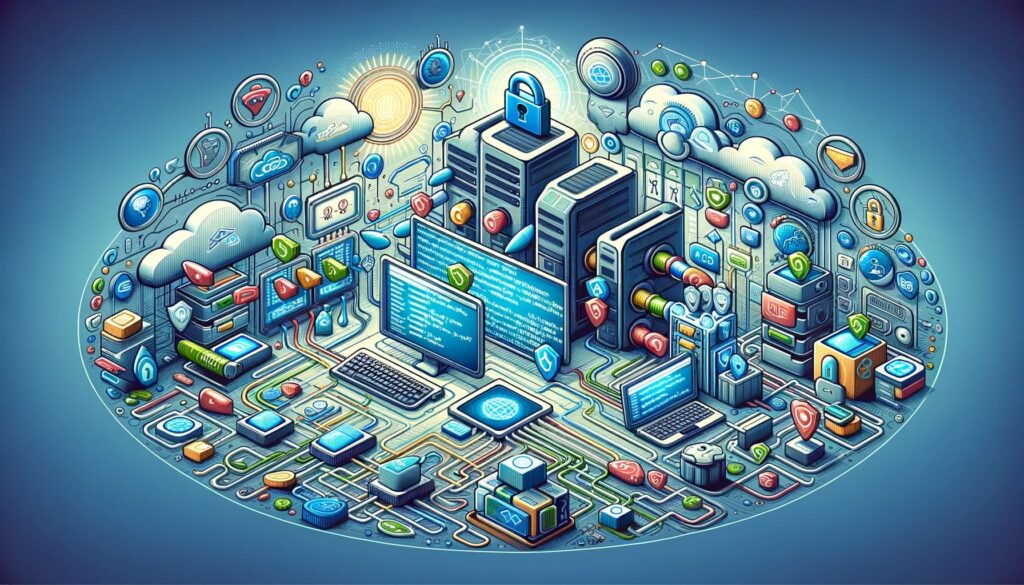
The Need for Secure Communication
With the increasing awareness of data security and privacy, HTTPS (Hypertext Transfer Protocol Secure) has become a standard for secure communication over the internet. For ActionScript applications, particularly those handling sensitive user information, implementing HTTPS is vital. It not only encrypts the data during transmission but also helps in authenticating the involved parties, thereby mitigating risks like man-in-the-middle attacks.
Step-by-Step Guide to Implementing HTTPS
- Acquire an SSL Certificate: The first step is to obtain an SSL (Secure Sockets Layer) certificate from a certified authority. This certificate is essential for establishing a secure connection.
- Configuring the Server: Configure your server to handle HTTPS requests. This involves installing the SSL certificate and setting up the server to redirect HTTP requests to HTTPS.
- ActionScript Code Adjustments: In your ActionScript code, make sure all external requests are made over HTTPS. For instance, when loading external resources or making API calls, use the
https://
prefix instead ofhttp://
.Example:var urlRequest:URLRequest = new URLRequest("https://example.com/api/data"); var urlLoader:URLLoader = new URLLoader(); urlLoader.load(urlRequest);
- Testing: After implementing HTTPS, thoroughly test your application to ensure that all parts of the application are functioning correctly over the secure protocol.
- Ongoing Maintenance: Regularly renew your SSL certificate and stay updated with the latest security practices to maintain the integrity of the HTTPS implementation.
Cross-Domain Policy in ActionScript with HTTPS
Cross-domain policy files in ActionScript are crucial when loading data from different domains. When using HTTPS, ensure that the cross-domain policy files are also served over HTTPS. This ensures that the policy files themselves are securely transmitted, reducing the risk of tampering.
Example of a cross-domain policy file request over HTTPS:
Security.loadPolicyFile("https://example.com/crossdomain.xml");
Cross-Domain Policy Files in ActionScript
Managing Cross-Domain Interactions
Cross-domain policy files in ActionScript are crucial for managing how your Flash application interacts with different domains. These XML files specify which domains are allowed to access your content, providing a layer of security against unauthorized access and data breaches.
Creating and Implementing a Cross-Domain Policy File
- Defining the Policy: Create an XML file that defines the allowed domains. For example:
<?xml version="1.0"?> <!DOCTYPE cross-domain-policy SYSTEM "http://www.adobe.com/xml/dtds/cross-domain-policy.dtd"> <cross-domain-policy> <allow-access-from domain="trusteddomain.com" /> </cross-domain-policy>
- Hosting the Policy File: Place this XML file at the root of your domain (e.g.,
https://yourdomain.com/crossdomain.xml
). - Referencing in ActionScript: In your ActionScript code, reference this policy file to allow cross-domain requests.
Example:Security.loadPolicyFile("https://yourdomain.com/crossdomain.xml");
- Testing and Validation: Ensure that your application correctly interacts with the specified domains and that unauthorized domains are blocked.
Best Practices for Cross-Domain Policies
- Limit Access: Only allow access from domains that need it. Avoid using wildcard entries like
*
unless absolutely necessary. - Serve Over HTTPS: Always serve your cross-domain policy file over HTTPS to prevent tampering.
- Regular Updates: Keep the policy file updated in line with changes to your application’s domain interactions.
ActionScript and Browser Security Considerations
Interaction Between ActionScript and Web Browsers
When developing ActionScript applications, especially those running within web browsers, it’s important to understand the security model of the browsers and how they interact with Flash content. This knowledge is crucial for both protecting the application and ensuring a seamless user experience.
Handling Mixed-Content and Cross-Scripting Issues
- Mixed-Content Issues: When a web page served over HTTPS includes resources (like Flash content) served over HTTP, it creates mixed-content issues. Browsers may block these resources or warn users, affecting functionality and trust.
- Solution: Ensure all resources, including Flash content, are served over HTTPS. Update your ActionScript code to request resources via HTTPS.
- Cross-Site Scripting (XSS) Protection: XSS is a significant threat where malicious scripts are injected into web pages. Browsers have built-in mechanisms to detect and block these attacks, but developers must also take proactive steps.
- ActionScript Approach: Validate all input and escape data before displaying it in the Flash application. Be cautious with any functionality that allows user-generated content.
- Cross-Origin Resource Sharing (CORS): This is a security feature that allows or denies scripts in a web page from interacting with resources from different origins.
- Implementation in ActionScript: Properly configure cross-origin policy files and headers to manage how Flash content interacts with resources from different domains.
Proactive Security Measures for ActionScript Applications
- Regular Security Audits and Updates: Conduct security audits to identify vulnerabilities. Regularly update the Flash player and ActionScript engine, as well as any third-party libraries used.
- Utilizing Security Tools and Resources: Leverage tools like static code analysis, penetration testing, and vulnerability scanners tailored for Flash and ActionScript.
- Educating Development Team: Ensure your development team is aware of the latest security threats and best practices in ActionScript programming. Encourage them to stay informed about new vulnerabilities and patches.
- Embedding Security in Development Lifecycle: Integrate security practices into the Software Development Life Cycle (SDLC). This includes secure coding practices, code reviews, and testing for security vulnerabilities at each stage of development.
- Community Engagement and Support: Participate in developer forums and communities. Engaging with the broader ActionScript and security community can provide insights into emerging threats and mitigation strategies.
Conclusion
Security in ActionScript programming is a multi-faceted challenge that requires a proactive and informed approach. By understanding the specific risks and vulnerabilities associated with ActionScript and browser interactions, developers can build more secure and robust applications. Regular updates, security audits, and an ongoing commitment to security education are key to maintaining the integrity and security of ActionScript applications. The goal is not only to protect the application but also to safeguard the end-users who interact with it.