Introduction to CSS Grid
CSS Grid Layout, often simply referred to as CSS Grid, marks a revolutionary shift in the way web designers approach layout design. This powerful layout system for the web offers an entirely new way of arranging elements on a page, changing the game from the traditional methods used in web design.
Before the advent of CSS Grid, web layouts were typically constructed using techniques like floats and positioning, or frameworks like Flexbox. These methods, while effective, had their limitations, especially when it came to building complex, two-dimensional layouts. Designers often had to resort to hacks or complex CSS to achieve the desired layout, resulting in code that was hard to maintain and update.
CSS Grid, a module introduced in the CSS Level 3 specification, addresses these challenges head-on. It allows for the creation of complex web layouts that are both easier to understand and maintain. One of the key features of CSS Grid is its ability to layout elements in rows and columns, forming a grid system that is both flexible and easy to control.
This layout model provides a grid container where direct child elements can be positioned into customizable rows and columns. You can explicitly define the grid structure or let the browser automatically create it based on the content. This flexibility makes it particularly powerful for designing responsive and adaptive layouts.
Key Characteristics of CSS Grid:
- Two-Dimensional Layout: Unlike Flexbox, which is largely a one-dimensional system (either in rows or columns), CSS Grid lets you work in both rows and columns simultaneously.
- Flexibility: Grid layout provides the ability to create a layout structure that adapts to the content, ensuring a more dynamic and responsive design.
- Control: It gives designers precise control over how items are placed and aligned within the grid, both horizontally and vertically.
- Simplicity: With Grid, complex layouts that once required intricate and brittle CSS can now be achieved with less code and more clarity.
- Integration: It complements other CSS layout models like Flexbox and can be integrated seamlessly to create sophisticated designs.
- Browser Support: CSS Grid has gained wide support across modern browsers, making it a reliable choice for contemporary web design.
Why Use CSS Grid?
The CSS Grid Layout system is particularly useful for web pages that require a complex layout structure with multiple rows and columns. It simplifies the process of creating magazine-style layouts, forms, image galleries, and other advanced design patterns that were previously difficult to achieve.
Moreover, with the growing need for responsive design, CSS Grid proves invaluable. Its ability to rearrange layout items based on screen size and other factors without losing the integrity of the design is crucial for modern web development.
Understanding the Basics of CSS Grid
The foundation of mastering CSS Grid lies in grasping its core concepts. This section will break down the basic elements of the CSS Grid Layout and explain how they work together to form the backbone of any grid-based design.
The Grid Container
The first step in using CSS Grid is to define a grid container. This is done by setting an element’s display property to grid or inline-grid. The children of this container automatically become grid items, which can be placed into the grid layout.
.container {
display: grid;
}
Grid Items
Grid items are the direct children of a grid container. In a typical HTML structure, these would be any element directly enclosed within the grid container. Each item can be positioned and sized within the grid container, making up the overall layout.
Defining Columns and Rows
The real power of CSS Grid comes from its ability to define rows and columns. The grid-template-columns and grid-template-rows properties are used for this purpose. They allow you to specify the sizes of the columns and rows in the grid.
For example:
.container {
grid-template-columns: 100px 200px auto;
grid-template-rows: 50px auto;
}
In this example, the container is divided into three columns with widths of 100px, 200px, and the rest of the space (auto). Similarly, it has two rows with the first row being 50px high and the second taking up the remaining space.
The Fr Unit
One of the unique features of CSS Grid is the fr unit, which represents a fraction of the available space in the grid container. This unit allows for more flexible and responsive design layouts.
Example:
.container {
grid-template-columns: 1fr 2fr;
}
Here, the container is divided into two columns, where the second column is twice as wide as the first.
Grid Gaps
The gap property (formerly known as grid-gap) is used to create space between rows and columns. It helps in managing the layout’s spacing without having to adjust margins and paddings of individual items.
Example:
.container {
grid-gap: 10px;
}
This will create a 10px gap between both rows and columns.
Grid Lines
In a grid layout, lines are automatically created between columns and rows. These lines can be referenced to place and align grid items. Each line is numbered starting from 1 at the start of the grid, moving horizontally for columns and vertically for rows.
Putting It All Together
The combination of these elements forms the basis of CSS Grid. By understanding how to manipulate containers, items, rows, columns, gaps, and lines, you can start to build complex layouts with ease and precision.
Exploring Grid Template Columns and Rows
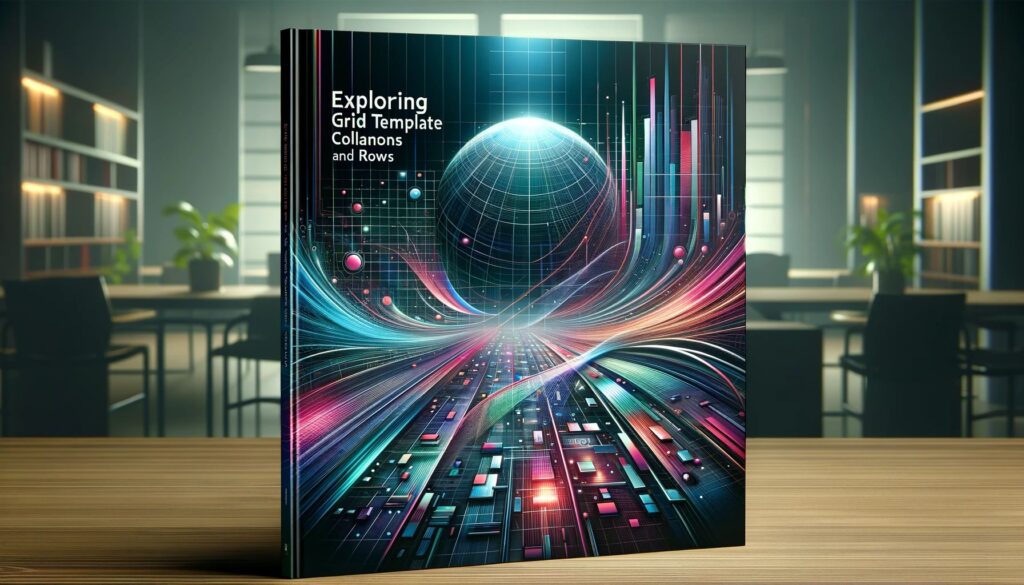
The heart of CSS Grid lies in its ability to define columns and rows in a highly customizable manner. This aspect of CSS Grid provides the framework upon which complex layouts are built. By understanding and effectively utilizing grid-template-columns and grid-template-rows, designers can create intricate and adaptable web structures.
Defining Columns and Rows
The properties grid-template-columns and grid-template-rows are used to define the number and size of columns and rows in a grid container. They accept a variety of values, allowing for precise control over the layout.
Columns:
.container {
grid-template-columns: 100px 1fr 2fr;
}
In this example, the grid container is divided into three columns. The first column is 100 pixels wide, the second takes up one fraction of the remaining space, and the third takes up two fractions, effectively being twice as wide as the second column.
Rows:
.container {
grid-template-rows: 50px auto 100px;
}
Here, the container is divided into three rows. The first row is 50 pixels high, the second row adjusts to the content’s size (auto), and the third row is 100 pixels high.
Using fr for Flexible Layouts
The fr unit, representing a fraction of the available space, is particularly useful for creating responsive layouts. It allows columns and rows to resize based on the container’s size, making the grid adapt to different screen sizes.
.container {
grid-template-columns: 1fr 3fr;
}
In this case, the first column occupies one part of the space, while the second occupies three parts, making the second column three times wider than the first.
Minmax Function for Responsive Columns and Rows
The minmax function is a powerful tool in grid layout. It allows setting a minimum and maximum size for rows and columns, providing flexibility and ensuring that content remains legible and accessible across different devices.
.container {
grid-template-columns: repeat(auto-fill, minmax(100px, 1fr));
}
This creates as many columns as can fit in the container, each with a minimum width of 100 pixels and a maximum width of 1fr.
Repeat Notation for Efficiency
The repeat function simplifies the definition of multiple columns or rows that have the same size. It is particularly useful in creating grids with a large number of similar tracks.
.container {
grid-template-columns: repeat(3, 1fr);
}
This creates three columns, each occupying one fraction of the available space.
Creating Advanced Layouts
Combining these properties allows for the creation of complex and responsive layouts. Designers can define the basic structure of their layout with grid-template-columns and grid-template-rows, then use fr units, the minmax function, and the repeat notation to ensure the layout is flexible and adapts to different screen sizes.
The Power of Grid Template Areas
Grid template areas offer an innovative way to visually structure your layout within the CSS Grid system. This feature enhances the readability and manageability of your grid layout, especially when dealing with complex designs.
Concept of Grid Template Areas
Grid template areas allow you to assign names to specific regions of your grid layout. This method involves two steps: defining the grid areas in the container and then assigning these areas to your grid items.
Defining Areas:
You use the grid-template-areas property on the grid container to create a visual map of your layout. Each area is represented by a name, and you can use a period (.) to represent an empty cell.
Example:
.container {
grid-template-areas:
"header header header"
"main main sidebar"
"footer footer footer";
}
This creates a layout with a header and footer spanning across three columns and a main content area next to a sidebar.
Assigning Areas to Items:
After defining the areas, you assign each grid item to an area using the grid-area property.
.header {
grid-area: header;
}
.main {
grid-area: main;
}
.sidebar {
grid-area: sidebar;
}
.footer {
grid-area: footer;
}
Benefits of Using Grid Template Areas
- Readability: It becomes easier to understand the layout structure at a glance, as the names are descriptive.
- Maintainability: Rearranging the layout only requires changes in the container’s grid-template-areas property, without the need to adjust the placement of each individual item.
- Responsiveness: You can redefine the grid-template-areas property within media queries to rearrange the layout for different screen sizes, enhancing the responsiveness of your design.
Responsive Design with Grid Template Areas
By adjusting grid-template-areas within media queries, you can dramatically change your layout for different devices without altering the HTML structure.
Example:
@media (max-width: 600px) {
.container {
grid-template-areas:
"header"
"main"
"sidebar"
"footer";
}
}
In this example, the layout shifts to a single column for screens smaller than 600 pixels.
Creating Complex Layouts
Grid template areas can be used to create more complex layouts as well. You can span areas across multiple rows or columns, overlap areas, or create intricate designs that were difficult to achieve with older CSS layout techniques.
Responsive Design with CSS Grid
Responsive design is crucial in today’s web development, ensuring that websites adapt seamlessly across different devices and screen sizes. CSS Grid brings a new level of ease and flexibility to creating these responsive designs.
Embracing Fluidity with CSS Grid
The inherent fluidity of CSS Grid makes it an ideal choice for responsive design. By using relative units like percentages or the fr unit for grid tracks, and combining these with media queries, you can create layouts that adapt to the screen size without the need for excessive code.
Example of Fluid Grid:
.container {
grid-template-columns: repeat(auto-fill, minmax(20%, 1fr));
}
This creates a grid layout where each column takes a minimum of 20% of the container width, but can expand equally to fill the available space.
Leveraging Media Queries
Media queries are a staple in responsive design, allowing you to apply different styles based on various conditions like screen width. CSS Grid’s structure works harmoniously with media queries, making it simpler to shift layouts at different breakpoints.
Example of Media Query with CSS Grid:
@media (max-width: 600px) {
.container {
grid-template-columns: 1fr;
}
}
In this example, the grid layout switches to a single column when the viewport width is 600 pixels or less.
Grid Template Areas and Responsiveness
Grid template areas shine in responsive design. You can redefine the grid-template-areas property within a media query to transform your page layout for different devices.
Responsive Design with Grid Template Areas:
.container {
grid-template-areas: "header" "main" "sidebar" "footer";
}
@media (min-width: 600px) {
.container {
grid-template-areas:
"header header"
"main sidebar"
"footer footer";
}
}
This shifts from a single-column layout on smaller screens to a more complex layout on larger screens.
The Role of auto-fill and auto-fit
The auto-fill and auto-fit keywords in CSS Grid offer powerful ways to handle responsive grids. They control how grid tracks behave in relation to the available space.
- auto-fill fills the row with as many columns as it can fit, even if they are empty.
- auto-fit collapses empty columns, resulting in a tighter layout.
Using auto-fill and auto-fit:
.container {
grid-template-columns: repeat(auto-fill, minmax(200px, 1fr));
}
This ensures that the container is filled with as many 200px columns as possible, adjusting the number based on the container’s width.
Accessibility in Responsive Design
While focusing on responsiveness, it’s important to maintain accessibility. CSS Grid’s layout does not disrupt the document flow, which is beneficial for screen readers and keyboard navigation. Ensuring that your responsive design maintains a logical tab order and content flow is paramount.
Combining CSS Grid with Other Layout Methods
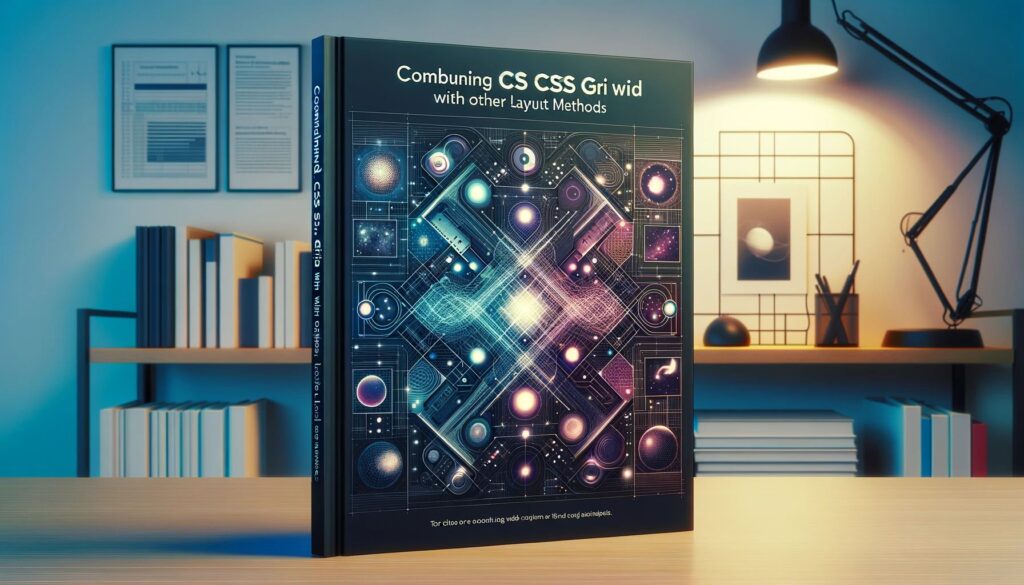
The versatility of CSS Grid is not just limited to its standalone capabilities. One of its strengths lies in its ability to be combined with other CSS layout techniques, such as Flexbox, to create even more powerful and flexible designs. This synergy allows developers to leverage the best features of each layout method for different aspects of their designs.
Integrating CSS Grid with Flexbox
Flexbox is ideal for laying out items in a single dimension – either in a row or a column. However, CSS Grid excels in two-dimensional layouts. By combining the two, you can manage complex layouts more effectively.
Example of Using Flexbox within a CSS Grid:
.container {
display: grid;
grid-template-columns: 1fr 2fr;
}
.sidebar {
display: flex;
flex-direction: column;
}
In this layout, the .container uses CSS Grid for its overall structure, while the .sidebar employs Flexbox to align its children vertically.
Nested Grids for Complex Layouts
Creating grids within grids, or nested grids, opens up possibilities for intricate layout patterns. You can define a grid for the main container and then specify another grid layout for one or more of its child elements.
Example of Nested Grids:
.container {
display: grid;
grid-template-columns: 1fr 1fr;
}
.item {
display: grid;
grid-template-columns: auto 1fr;
}
This creates a two-column grid for the .container, with each .item also being a grid with its own layout.
Using Grid and Other Positioning Methods
CSS Grid can also be combined with other positioning methods like absolute and relative positioning. This is useful for layering elements or for placing items in very specific locations within a grid cell.
Example of Combining Grid with Positioning:
.container {
display: grid;
grid-template-columns: 1fr 1fr;
position: relative;
}
.overlay {
position: absolute;
top: 10px;
left: 10px;
}
In this scenario, an overlay element is absolutely positioned within a grid container.
Practical Considerations
When combining CSS Grid with other layout methods, it’s important to consider the specific strengths and use-cases of each method. For instance, Flexbox is more suitable for components where the size of the items should influence the layout, while Grid is more suited for layouts where the grid structure dictates the size and position of its elements.
Accessibility and Performance
Maintaining accessibility and performance is crucial when combining layout techniques. Ensure that the use of multiple layout models does not hinder the website’s loading times or its navigability, especially for users relying on assistive technologies.
Optimizing Grid Performance
Efficient grid performance is pivotal in creating a smooth user experience. As websites become more complex with intricate layouts, it’s essential to ensure that these designs do not compromise on performance. This section provides strategies for optimizing the rendering and layout time when using CSS Grid.
Minimizing Grid Complexity
A key factor in grid performance is the complexity of the grid itself. The more complex the grid (e.g., many rows and columns, nested grids), the more work the browser has to do to calculate layouts.
Strategies for Reducing Complexity:
- Limit the Number of Grid Tracks: Use as few rows and columns as necessary. Excessive grid tracks can slow down layout calculations.
- Avoid Nested Grids When Possible: While nested grids are powerful, they can increase the layout complexity. Use them judiciously.
Efficient Use of Grid Template Areas
While grid-template-areas are incredibly useful for readability and maintainability, they can become less performant with a very large number of areas or overly complex arrangements.
Optimization Tips:
- Keep Areas Simple: Use grid template areas for simpler layouts and consider alternative methods for more complex designs.
- Use Named Lines for Complex Layouts: For more intricate designs, using named lines can sometimes be more efficient than grid-template-areas.
Leveraging Implicit Over Explicit Grids
CSS Grid offers both explicit and implicit grid controls. An explicit grid is defined with grid-template-rows and grid-template-columns, while an implicit grid is created automatically by the browser based on the number of grid items.
Performance Considerations:
- Use Implicit Grids for Dynamic Content: When dealing with dynamic content where the number of items is not predetermined, leveraging the implicit grid can be more efficient.
- Explicit Grids for Static Layouts: For static layouts, an explicit grid ensures better control and can be optimized more easily.
CSS Containment
The CSS contain property can be used to indicate to the browser that an element’s content is isolated from the rest of the page. This can improve rendering performance by allowing the browser to make optimizations knowing that certain changes will be confined to that element.
Example of Using CSS Containment:
.container {
contain: layout;
}
Conclusion
As we conclude this guide on CSS Grid, we recognize it as a transformative tool in web design, offering a blend of simplicity, control, and flexibility. It has redefined layout creation, allowing for both basic and complex structures with ease. The synergy of CSS Grid with other layout methods, like Flexbox, amplifies its utility, catering to nuanced design needs. Key to its adoption is its responsiveness, enabling designs that fluidly adapt across different devices. However, balancing design complexity with performance is crucial for optimal functionality. The essence of CSS Grid lies in its power to revolutionize web design, encouraging experimentation and exploration to harness its full potential. Embrace CSS Grid and elevate your web design to new heights.