Introduction to ActionScript
ActionScript, an influential object-oriented programming language, emerged from the collaborative efforts of Macromedia Inc. and was later adopted by Adobe. It was initially designed as a control mechanism for simple two-dimensional vector animations in Adobe Flash. However, its role and capabilities have significantly evolved over time.
Early Years and Development
ActionScript’s roots can be traced back to the scripting language HyperTalk, which significantly influenced its early design. The language was crafted to complement Macromedia’s Flash authoring tool, primarily for creating basic animations and interactive elements on web pages. Its development closely paralleled JavaScript, with both languages being influenced by HyperTalk.
Core Capabilities in Programming
As a superset of ECMAScript, ActionScript shares syntax and semantics with JavaScript, making it familiar to many web developers. Initially, its functionality was limited, offering basic scripting abilities to control animations and interactivity in Flash content. However, subsequent versions, particularly ActionScript 3, marked a significant leap, introducing robust features suitable for complex web applications, desktop and mobile app development, and even basic robotics.
In its prime, ActionScript was pivotal in creating rich, interactive web experiences, including games, animated content, and media-rich applications. Despite the decline in Flash usage, ActionScript’s influence in web development and interactive design remains noteworthy.
Understanding ActionScript’s Core Features

In the realm of programming languages, ActionScript stands out for its object-oriented structure and close alignment with ECMAScript, the standard underlying JavaScript. This section delves into these core aspects, highlighting what makes ActionScript a versatile tool in the programmer’s toolkit.
Object-Oriented Programming Structure
At its core, ActionScript is object-oriented, meaning it models data and functions around “objects” rather than actions. This approach, common in modern programming, allows for more organized and modular code, making it easier to manage and scale complex applications. The language supports class-based inheritance, encapsulation, and polymorphism, providing a robust framework for building dynamic applications.
Integration with ECMAScript and JavaScript Influences
ActionScript’s development closely mirrored that of JavaScript, both being influenced by ECMAScript. This means ActionScript shares many similarities with JavaScript, making it relatively easy for web developers to transition between the two. Its syntax and semantics are consistent with ECMAScript standards, ensuring a familiar environment for developers accustomed to JavaScript’s intricacies.
The ECMAScript influence also extends to data types, functions, and object models, with ActionScript offering a rich set of built-in classes and functions. These include everything from basic data types like numbers and strings to more complex structures like arrays and objects, as well as a comprehensive set of APIs for handling tasks like event management and XML parsing.
The Evolution of ActionScript: From 1.0 to 3.0
ActionScript’s journey from a basic scripting tool to a fully-fledged programming language is marked by significant milestones, each contributing to its robustness and versatility.
Early Beginnings: ActionScript 1.0
Introduced with Flash 5 in 2000, ActionScript 1.0 was influenced by JavaScript and ECMAScript standards. It was a leap from simple, timeline-based controls to a more structured scripting language. This version supported basic programming constructs like variables, loops, and conditional statements, making it suitable for simple web animations and interactivity.
Advancements in ActionScript 2.0
Released with Flash MX 2004, ActionScript 2.0 brought class-based syntax and compile-time type checking to the forefront. This version was a response to the growing demand for a language capable of handling more complex applications. It retained compatibility with ActionScript 1.0 but introduced new concepts like class and extends, aligning more closely with object-oriented programming paradigms seen in Java and C++.
The Revolution of ActionScript 3.0
ActionScript 3.0, debuting with Adobe Flex 2.0 and Flash Player 9 in 2006, represented a fundamental overhaul. It introduced a new virtual machine (AVM2) for significantly improved performance. This version featured compile-time and run-time type checking, a unified event handling system, support for regular expressions, and a class-based inheritance system distinct from the prototype-based system of its predecessors. These enhancements enabled the creation of complex, high-performance applications and games, cementing ActionScript’s position in advanced web development.
Each iteration of ActionScript brought new capabilities and improvements, aligning with the evolving needs of developers and the industry. This evolution reflects ActionScript’s adaptability and its significant role in shaping interactive web experiences. The subsequent sections will delve deeper into ActionScript’s integration with Adobe Flash and AIR, its real-time application capabilities, and advanced features introduced over time.
ActionScript in Adobe Flash and AIR
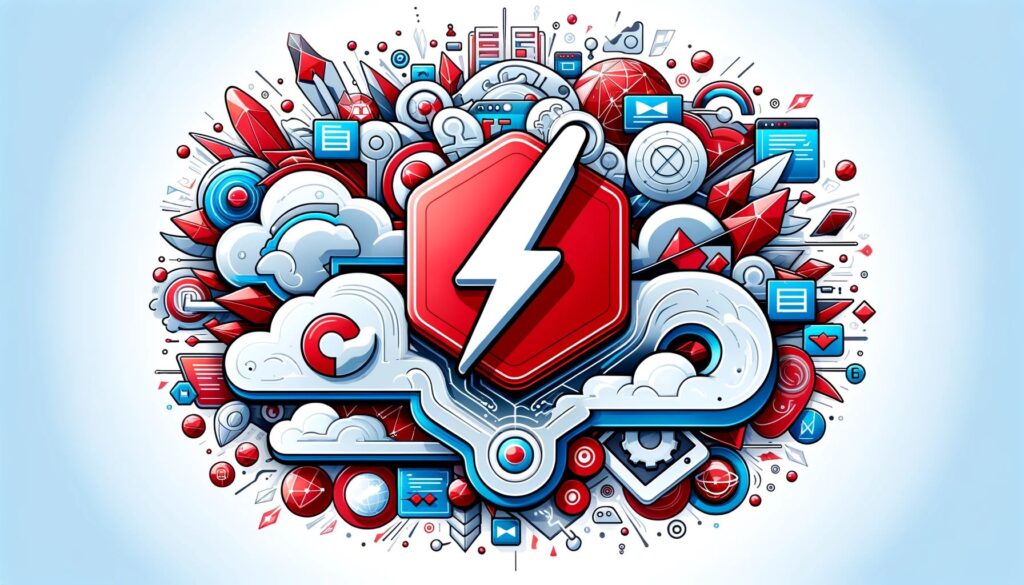
Role in Web-Based Animation and Gaming
ActionScript’s integration with Adobe Flash revolutionized web-based animation and gaming. Initially, it empowered developers to create rich, interactive animations and later evolved to support complex gaming environments. This was largely due to ActionScript’s ability to handle intricate graphics, user interaction, and multimedia elements seamlessly within a web browser.
Transition to Desktop and Mobile Applications with Adobe AIR
With the advent of Adobe AIR (Adobe Integrated Runtime), ActionScript extended its reach beyond web browsers to desktop and mobile applications. AIR allowed developers to use ActionScript for building standalone applications, which could run outside of web browsers, thus opening new avenues for software development.
Sample ActionScript Code
Consider a simple example of ActionScript code that creates a clickable button and responds to a user’s click event:
import flash.events.MouseEvent;
// Create a simple button
var myButton:SimpleButton = new SimpleButton();
myButton.x = 100;
myButton.y = 50;
addChild(myButton);
// Event listener for the button click
myButton.addEventListener(MouseEvent.CLICK, onClick);
// Function to handle the click event
function onClick(event:MouseEvent):void {
trace("Button clicked!");
}
In this code, we import necessary classes, create a button, position it, and add an event listener. When the button is clicked, the onClick function is triggered, printing a message to the console.
This example showcases the ease with which interactive elements can be integrated into applications using ActionScript. The language’s robust feature set, combined with the capabilities of Flash and AIR, has made it a tool of choice for many developers in creating engaging and interactive user experiences. The following sections will explore the specific applications of ActionScript in real-time scenarios and its advanced features.
Real-Time Applications of ActionScript
ActionScript’s capabilities extend far beyond animations and web games, making it a powerful tool for developing real-time applications. These include dynamic web content, interactive media, database applications, and even basic robotics.
Dynamic Web Content and Interactive Media
In the realm of dynamic web content, ActionScript excels in creating rich, interactive experiences. It’s capable of handling real-time data streaming, which is crucial for applications like online video players, chat applications, and live data visualizations. ActionScript’s powerful rendering capabilities allow for smooth animations and transitions, enhancing user engagement and experience.
Database Applications
ActionScript can interact with databases, making it possible to develop web applications that require real-time data processing and display. It’s particularly useful in scenarios where immediate feedback or interaction is required, such as in online booking systems or data dashboards.
Basic Robotics
In the field of basic robotics, ActionScript has found its use in controlling robotic components through the Make Controller Kit. This application demonstrates its versatility and potential in handling real-time control and feedback systems.
Sample ActionScript Code for a Real-Time Data Display
Here’s an example of how ActionScript can be used to fetch and display real-time data:
import flash.net.URLLoader;
import flash.net.URLRequest;
import flash.events.Event;
// Create a URL loader to fetch data
var loader:URLLoader = new URLLoader();
loader.addEventListener(Event.COMPLETE, onDataLoadComplete);
loader.load(new URLRequest("http://example.com/data"));
// Function to handle the data after loading
function onDataLoadComplete(event:Event):void {
var rawData:String = URLLoader(event.target).data;
trace("Data Loaded: " + rawData);
}
In this snippet, ActionScript is used to load data from a web source asynchronously. Once the data is loaded, the onDataLoadComplete function processes it, which could then be used to update the UI in real-time.
This ability to handle external data sources and process them in real-time underscores ActionScript’s utility in creating dynamic, responsive applications. The next sections will delve into the advanced features and performance enhancements introduced in ActionScript, further cementing its role in modern application development.
Advanced Features and Performance Enhancements in ActionScript

Enhanced Performance with JIT Compilation
One of the most significant advancements in ActionScript, particularly in version 3.0, is the introduction of Just-In-Time (JIT) compilation. This enhancement drastically improved the execution speed of ActionScript code, making it up to 10 times faster than its predecessors. JIT compilation translates ActionScript bytecode into native machine code at runtime, optimizing performance for complex applications and games.
Hardware Acceleration Support
ActionScript 3.0 also introduced limited support for hardware acceleration, leveraging technologies like DirectX and OpenGL. This allowed for more efficient rendering of graphics and animations, crucial for high-performance gaming and rich media applications.
Sample ActionScript Code Utilizing Enhanced Features
Here’s an example demonstrating ActionScript’s event handling and display list manipulation, highlighting performance and advanced capabilities:
import flash.display.Sprite;
import flash.events.Event;
var movingSprite:Sprite = new Sprite();
movingSprite.graphics.beginFill(0xFF0000);
movingSprite.graphics.drawCircle(50, 50, 50);
addChild(movingSprite);
addEventListener(Event.ENTER_FRAME, onEnterFrame);
function onEnterFrame(event:Event):void {
movingSprite.x += 1;
if (movingSprite.x > stage.stageWidth) {
movingSprite.x = 0;
}
}
This code creates a simple animation where a red circle moves across the screen. The Event.ENTER_FRAME listener updates the sprite’s position every frame, demonstrating how ActionScript can manage real-time graphics updates efficiently.
These advanced features, along with the language’s comprehensive API and enhanced event handling capabilities, make ActionScript a potent tool for developing high-performance applications. The final section will discuss the future and legacy of ActionScript, including its influence and relevance in today’s programming landscape.
Conclusion
ActionScript’s evolution from a basic scripting language to a robust tool for real-time applications marks its significant impact in web and multimedia development. Its legacy, rooted in its adaptability, object-oriented structure, and advanced features, continues to influence modern programming. Although its direct use may decline, the principles and innovations it introduced will persist in guiding developers, shaping future technologies and interactive digital solutions.