Introduction to Debugging in ActionScript
Welcome to the dynamic world of ActionScript, a robust programming language that powers countless interactive applications and animations. In this journey, one critical skill stands out as indispensable: debugging. Debugging in ActionScript is not just about fixing errors; it’s about understanding the nuances of the language and ensuring your application performs optimally.
The Essence of Debugging in ActionScript
At its core, debugging in ActionScript involves identifying and resolving bugs or flaws that impede the functionality or performance of applications. These issues can range from simple syntax errors to complex logical mishaps, each requiring a keen eye and strategic approach to resolve.
Why Debugging Matters
In the fast-paced world of software development, the efficiency and reliability of your code can make or break an application. Debugging ensures that your application not only runs smoothly but also provides a seamless user experience. It’s about preemptively addressing potential pitfalls and optimizing your code for the best possible performance.
A Brief History of ActionScript Development
ActionScript has evolved significantly since its inception, growing from a simple scripting tool in early Flash versions to a powerful, object-oriented programming language in ActionScript 3.0. This evolution has brought more sophisticated features, but also more complex debugging challenges. As the language has matured, so have the techniques and tools for debugging, allowing developers to more effectively pinpoint and solve issues in their code.
The Developer’s Mindset for Successful Debugging
Successful debugging in ActionScript starts with a developer’s mindset. It requires patience, attention to detail, and a systematic approach to problem-solving. Understanding the common types of bugs and their impact on applications is the first step in this process. The journey from identifying a bug to successfully resolving it is often iterative and requires a deep understanding of both the language and the specific application context.
Common Bugs in ActionScript and Their Impact

Navigating through the landscape of ActionScript development, one encounters various types of bugs that can impact the functionality and performance of applications. Understanding these common bugs is crucial for any developer aiming to create robust and efficient applications.
1. Syntax Errors: The Basics Gone Wrong
One of the most common bugs in any programming language, including ActionScript, are syntax errors. These are mistakes in the code’s structure, like missing semicolons, misused brackets, or typos in variable names. For instance, a simple typo such as funtcion instead of function can cause the script to fail, leading to non-functional code.
2. Logical Errors: When Things Don’t Add Up
Logical errors are more elusive and often harder to detect. These occur when the code is syntactically correct but doesn’t perform as expected due to flawed logic. For example, an improperly set conditional statement, like if (score = 100) instead of if (score == 100), might always execute because it assigns a value rather than comparing it.
3. Runtime Errors: The Unseen Pitfalls
Runtime errors manifest during the execution of the application and are often caused by unforeseen circumstances in the code’s environment. A common example in ActionScript is a null object reference error, where the code attempts to access a property or method of an object that hasn’t been instantiated.
4. Performance Issues: The Hidden Hurdles
Apart from syntactic and logical errors, performance issues are common in ActionScript, especially when dealing with complex animations or data-intensive operations. Poorly optimized code can lead to slow performance and unresponsive interfaces. For instance, an inefficient loop or redundant event listeners can significantly slow down an application.
Understanding these common bugs is the first step towards effective debugging. Each type of bug requires a specific approach to identify and resolve. In the upcoming sections, we will explore various tools and techniques to address these issues, enhancing your proficiency in debugging ActionScript applications.
The ActionScript Interpreter: Your First Line of Defense
In the realm of ActionScript development, the interpreter serves as a critical tool in the debugging process. The built-in ActionScript interpreter in Flash MX is a powerful feature that significantly streamlines code testing and debugging.
Functionality of the ActionScript Interpreter
The interpreter allows developers to check and automatically format their code before publishing their movie. This is immensely beneficial in catching syntax errors and other basic mistakes early in the development process. For instance, when you write a function in ActionScript:
function calculateTotal(price:Number, quantity:int):Number {
return price * quantity;
}
You can use the ActionScript interpreter to ensure that the syntax is correct and that the function behaves as expected. To invoke the interpreter, you can click the ActionScript panel (shortcut F9) and click the check mark at the top of the panel, or press Ctrl+T. This action triggers the interpreter to analyze your script, highlighting any syntax errors or basic logical flaws.
Early Error Detection
Using the interpreter for early error detection is a vital step. It helps in identifying simple mistakes that can be easily overlooked during manual code reviews. For example, if you accidentally write:
functon calculateTotal(price:Number, quantity:int):Number {
return price * quantity;
}
The interpreter will immediately flag the functon keyword as an error, allowing you to correct it to function. Such early detection saves a significant amount of time that might otherwise be spent looking for issues in more complex areas of your code.
Streamlining Development with Interpreter Checks
Regularly using the interpreter as part of your development workflow encourages a more disciplined coding practice. It aids in maintaining clean, error-free code, which is easier to manage and debug in later stages of development. By catching errors early and often, the interpreter helps in reducing the overall debugging time and enhances the quality of the final product.
The ActionScript interpreter is a vital tool for any developer working in this environment. It provides a first line of defense against common coding errors, streamlines the development process, and sets the stage for more advanced debugging techniques, which will be discussed in the upcoming sections.
Advanced Tools for Debugging ActionScript
Once basic errors are resolved using the ActionScript interpreter, developers can leverage a suite of advanced tools to delve deeper into debugging. These tools are integral in tracking down more complex issues that affect the performance and functionality of ActionScript applications.
The Output Window: Monitoring Script Activity
The Output window in Flash is an essential tool for debugging. It displays trace statements embedded in your ActionScript code, allowing you to monitor the internal state of your application. For instance:
trace("Total Price: " + calculateTotal(100, 5));
This line outputs the result of the calculateTotal function to the Output window, helping you verify the correctness of your function’s logic in real-time.
ActionScript Debugger: Step-by-Step Analysis
The ActionScript Debugger is another powerful tool that enables step-by-step execution of your code. It allows you to set breakpoints, step through code, and inspect the values of variables and properties at different stages of execution. For example, you can set a breakpoint inside a function to observe how variables change:
function updateScore(currentScore:int, bonus:int):int {
var newScore:int = currentScore + bonus;
// Set breakpoint here to inspect newScore
return newScore;
}
Using the debugger, you can stop at the breakpoint and examine the value of newScore, ensuring that the function behaves as expected.
NetConnection Debugger: Monitoring Client-Server Communication
For applications that involve client-server interactions, the NetConnection Debugger is invaluable. It monitors the Action Message Format (AMF) communication between the client and the server. This tool is particularly useful in debugging applications that use Flash Communication Server or Flash Remoting. By observing the data exchanged over the network, developers can pinpoint issues in the communication protocol or data serialization/deserialization processes.
These advanced debugging tools in ActionScript provide a more granular level of control and inspection, enabling developers to identify and resolve complex bugs that are not apparent at the surface level. The combination of the Output window, ActionScript Debugger, and NetConnection Debugger equips developers with a comprehensive set of tools to ensure their applications run flawlessly.
Trace Actions: Simplifying Debugging in ActionScript

Trace Actions are a fundamental aspect of debugging in ActionScript, offering a simple yet powerful way to monitor the execution flow and status changes in your application. They play a pivotal role in observing the behavior of functions and communication objects, providing real-time feedback and insights.
The Power of Trace Actions
Trace Actions allow developers to insert trace statements in their ActionScript code, which output messages to the Output window during runtime. This can be incredibly useful for verifying the execution of certain parts of the code. For example:
function login(user:String, password:String):Boolean {
trace("Attempting login for user: " + user);
// Code for login
if (successfulLogin) {
trace("Login successful for user: " + user);
return true;
} else {
trace("Login failed for user: " + user);
return false;
}
}
In this snippet, the trace statements provide feedback at each critical step of the login process, allowing you to monitor and verify the function’s behavior.
Tracking Status Changes and Errors
Trace Actions are particularly useful in tracking the status changes of network communications, such as with NetConnection and NetStream. They can be used to display messages from the server, status updates, or error messages, giving you a clear picture of what’s happening in your application. For instance:
netConnection.onStatus = function(info:Object):Void {
trace("NetConnection status: " + info.code);
if (info.code == "NetConnection.Connect.Success") {
// Handle successful connection
} else {
// Handle connection errors
}
};
Here, the trace output helps in diagnosing the status of the NetConnection, whether it’s successful or encounters an error.
Best Practices with Trace Actions
While trace statements are incredibly useful, it’s important to use them judiciously. Excessive use of trace actions can clutter the Output window and make it difficult to identify relevant information. A best practice is to use them selectively, focusing on critical parts of the code where monitoring is most needed.
In conclusion, Trace Actions are an indispensable tool in the ActionScript developer’s arsenal. They offer a straightforward approach to monitoring and diagnosing your application’s behavior, making them an essential part of the debugging process.
Using the Flash MX Debugger for Comprehensive Analysis
The Flash MX Debugger is an essential tool for ActionScript developers, offering an in-depth approach to debugging. This comprehensive tool provides advanced capabilities to inspect, track, and modify the state of an application during runtime, making it invaluable for resolving complex issues.
Deep Inspection of Application State
The Flash MX Debugger allows you to view and manipulate the properties of objects in your application. You can observe how data structures, like arrays or objects, change over time. For example:
var userInfo:Object = {name: "Alice", score: 120};
// Set a breakpoint here to inspect userInfo
By setting a breakpoint at this line, you can use the debugger to inspect the userInfo object, viewing its properties and their values.
Watching Variables and Properties
Another powerful feature of the Flash MX Debugger is the ability to watch variables and properties. You can set up watch expressions to monitor how specific variables change under different conditions. This is particularly useful for tracking down logical errors and understanding the flow of data through your application.
Navigating Through Code Execution
The step-through feature in the debugger allows you to execute code line by line. This is crucial for understanding the execution flow and pinpointing where things might be going awry. For instance, if a function is not returning the expected result, stepping through its execution can reveal where the logic deviates from the intended path.
Best Practices for Using the Flash MX Debugger
Effective use of the Flash MX Debugger requires a methodical approach. Start by setting breakpoints in areas of your code that are most likely to contain errors. Use the step-through feature to follow the execution path and watch expressions to monitor key variables. Always keep an eye on the Call Stack and Variables panels to gain a comprehensive view of your application’s state at any given point in time.
The Flash MX Debugger is a powerful tool that provides a detailed view of an application’s internal state. Its ability to inspect variables, step through code, and monitor the execution flow makes it an indispensable asset for any ActionScript developer aiming to produce error-free and efficient applications.
Leveraging the NetConnection Debugger for Client-Server Communication
In ActionScript development, especially in applications involving client-server interactions, the NetConnection Debugger emerges as a pivotal tool. It specifically targets debugging the communication layer, providing transparency into the messages exchanged between client and server.
Understanding the NetConnection Debugger
The NetConnection Debugger is designed to monitor Action Message Format (AMF) communication, which is essential in applications using Flash Communication Server or Flash Remoting. This tool allows you to see the data being sent and received over the network, giving you valuable insight into the interaction between the client and server.
Practical Use Cases
A common scenario where the NetConnection Debugger is invaluable is when debugging real-time data streaming or messaging applications. For example, if an application streams video content from a server, the debugger can be used to monitor the status of the stream, connection messages, and any errors that occur:
netConnection.onStatus = function(info:Object):Void {
trace("Stream status: " + info.code);
// Additional code to handle stream status
};
In this code snippet, the NetConnection Debugger would allow you to see the detailed status messages, helping to diagnose issues like connection failures or stream interruptions.
Benefits of Using the NetConnection Debugger
- Error Identification: Quickly pinpoint issues in client-server communication, such as connection problems or data serialization issues.
- Performance Optimization: Monitor the efficiency of data exchange processes, helping to optimize the performance of network-intensive applications.
- Real-Time Diagnostics: Provides real-time feedback on the communication process, allowing for immediate corrective actions.
Best Practices for Maximizing Effectiveness
To get the most out of the NetConnection Debugger, it’s important to have a clear understanding of the application’s communication architecture. Know what data should be sent and received, and use the debugger to confirm that the actual communication aligns with these expectations. Additionally, correlating the debugger’s output with other debugging tools like trace statements or the Flash MX Debugger can provide a more holistic view of the application’s behavior.
The NetConnection Debugger is an indispensable tool for developers working on applications with significant client-server interactions in ActionScript. Its ability to provide a clear window into the communication process is essential for diagnosing and resolving complex issues effectively.
Best Practices in Debugging ActionScript
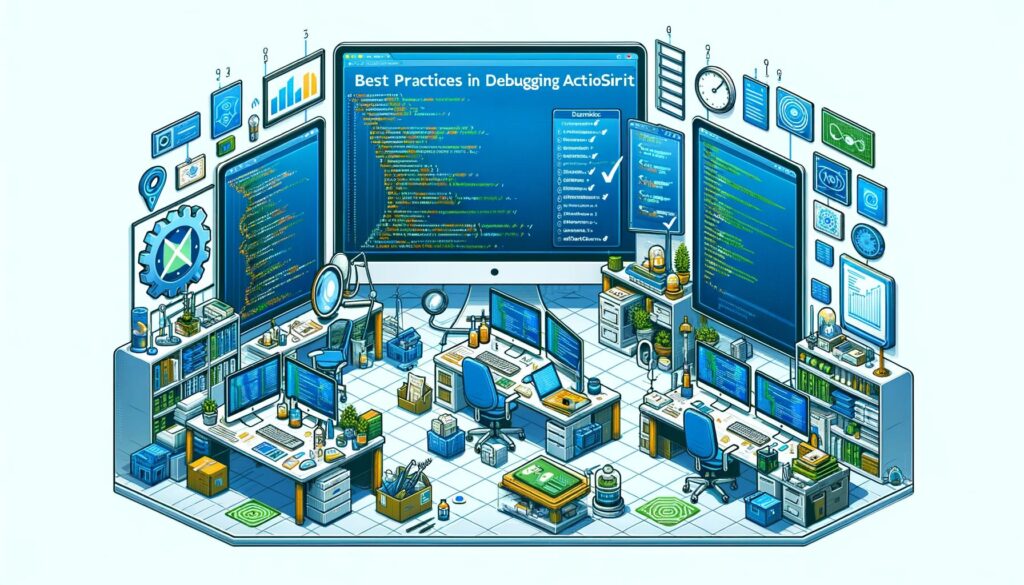
Mastering the art of debugging in ActionScript is not just about knowing the right tools; it’s also about adopting best practices that streamline the process and enhance efficiency.
- Understand the Codebase
Before diving into debugging, it’s crucial to have a thorough understanding of the codebase. Familiarity with the overall structure, key functions, and data flow makes it easier to identify where things might go wrong.
- Start with the Simplest Explanation
When encountering a bug, start by considering the simplest possible explanation. Check for common mistakes like syntax errors, misspelled variable names, or logical errors in conditionals.
- Use Breakpoints Strategically
Breakpoints are a powerful feature of the Flash MX Debugger. Use them strategically to pause execution and inspect the state of your application at critical points. This helps in understanding how variables and objects are being modified over time.
- Keep an Eye on Performance
While functional bugs are critical, don’t overlook performance issues. Inefficient loops, excessive memory usage, and redundant event listeners can degrade the performance of your application.
- Document and Track Bugs
Maintain a log of discovered bugs, including details about how they were identified and resolved. This not only helps in keeping track of what has been fixed but also aids in identifying patterns that might indicate deeper issues.
- Test Thoroughly
After fixing a bug, test the application thoroughly to ensure that the fix hasn’t introduced new issues. This is especially important in complex applications where different parts of the codebase are interdependent.
- Learn from Each Bug
Each bug presents a learning opportunity. Analyze why it occurred and how it was resolved. This helps in improving your coding practices and avoiding similar issues in future projects.
Incorporating these best practices into your debugging workflow can significantly enhance your effectiveness as an ActionScript developer. By approaching debugging methodically and learning from each challenge, you can ensure that your applications are not just functional, but also robust and efficient.
Conclusion: Streamlining Your ActionScript Development with Effective Debugging
As we conclude our exploration of debugging in ActionScript, it’s evident that effective debugging transcends mere error correction—it’s a fundamental aspect of crafting high-quality applications. This journey through various techniques underscores the importance of a comprehensive approach, integrating deep understanding, strategic tool use, and ongoing learning. Efficient debugging not only resolves issues but also fortifies your development process against future challenges, enhancing both application performance and user experience.
In the evolving world of ActionScript, staying abreast of the latest debugging trends and tools is crucial. Embracing continuous learning and adaptation in your debugging practices not only hones your individual skills but also contributes significantly to the broader field of software development. Ultimately, mastering the art of debugging is key to developing robust applications and establishing yourself as a skilled and reliable developer in the dynamic landscape of software development.