In the realm of software development, the landscape is continually evolving, with new technologies and frameworks emerging at a rapid pace. However, amidst this constant flux, Windows application development remains a crucial domain, powered significantly by C# and Windows Presentation Foundation (WPF). This section delves into the current state of Windows desktop development and the pivotal role played by C# and WPF.
The Landscape of Windows Desktop Development
As of 2023, Windows application development, though seemingly overshadowed by web and mobile platforms, retains its importance due to the robust and diverse needs of desktop users. Historically, desktop development was predominantly focused on PC-centric architectures, but the present scenario is more integrated, often involving web components. Yet, desktop applications still hold sway in many enterprise, commercial, and specialized software domains.
C# and WPF: Pillars of Windows Applications
C#, a language developed by Microsoft, is the cornerstone of Windows application development. It’s a language that has matured over the years, becoming more versatile and powerful with each iteration. The recent versions of C# have brought numerous enhancements, making it a language well-suited for modern desktop development. The language’s popularity is evident, with a significant percentage of developers using the latest versions for both closed-source and open-source projects.
WPF, on the other hand, stands as a testament to Microsoft’s commitment to rich, interactive user interfaces. As a framework for building Windows desktop applications, WPF allows developers to create visually appealing UIs with greater ease. It employs XAML (Extensible Application Markup Language) for UI design, enabling a clean separation of presentation from business logic. This clear demarcation not only streamlines development but also facilitates maintenance and scalability.
The Significance of WPF in the .NET Ecosystem
WPF, integrated into the .NET framework, provides developers with a comprehensive set of tools and libraries to build sophisticated desktop applications. Its compatibility with .NET MAUI (Multi-platform App UI) and other cross-platform frameworks further extends its relevance, allowing WPF-based applications to reach a wider audience beyond the Windows ecosystem.
Understanding Windows Forms and WPF
Windows Forms and Windows Presentation Foundation (WPF) are two key technologies for developing desktop applications in the Windows ecosystem. Each has its unique strengths and use cases, which are important to understand for effective application development.
Windows Forms: The Traditional Approach
Windows Forms, a part of the .NET Framework, is a GUI class library that offers a simpler way to create Windows-based applications. It has been a staple in the toolkit of Windows developers for creating form-based applications since the early days of .NET. Windows Forms applications are straightforward to design, with a drag-and-drop surface in Visual Studio, making it an excellent choice for rapid application development.
Despite being older, Windows Forms remains relevant due to its simplicity and directness, especially for applications that don’t require a complex user interface or extensive graphics. It provides a vast library of controls such as buttons, text boxes, and labels, which can be easily added to forms.
WPF: Advancing to Rich UI Experiences
WPF, introduced with .NET Framework 3.0, represents a significant advancement in the way Windows applications are built, especially regarding user interface design. It uses XAML for defining UI elements, providing a more flexible and powerful way to design user interfaces compared to Windows Forms. WPF supports advanced graphics and animations, making it ideal for applications requiring dynamic and modern UIs.
One of the key advantages of WPF is its separation of UI and business logic. This is achieved through data binding, a feature that allows developers to connect UI elements to data sources, thereby making UIs dynamic and responsive to underlying data changes without extensive coding.
Sample C# Code in WPF
To illustrate the power of WPF, consider a simple example where a button’s content changes based on user input:
<Window x:Class="WpfApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="200" Width="300">
<Grid>
<TextBox x:Name="InputTextBox" HorizontalAlignment="Left" Height="23" Margin="10,10,0,0" TextWrapping="Wrap" Text="Enter Text" VerticalAlignment="Top" Width="120"/>
<Button Content="Click Me" HorizontalAlignment="Left" Margin="140,10,0,0" VerticalAlignment="Top" Width="75" Click="Button_Click"/>
</Grid>
</Window>
In the C# code behind:
private void Button_Click(object sender, RoutedEventArgs e)
{
Button button = (Button)sender;
button.Content = InputTextBox.Text;
}
This example demonstrates how a button’s content in a WPF application can be dynamically updated based on user input, showcasing the ease of binding and event handling in WPF.
C# – The Powerhouse of Windows Applications
C# has cemented its position as a premier language for Windows application development. Its evolution and features make it an indispensable tool for developers working on Windows platforms, especially when combined with WPF for desktop applications.
The Role of C# in Windows Development
C#, developed by Microsoft, is a multi-paradigm programming language that is both powerful and flexible. It’s strongly typed, supports object-oriented programming, and offers advanced features like LINQ (Language Integrated Query) and async programming. These features make C# particularly well-suited for developing complex Windows applications that require robust backend logic and high-performance.
Latest Features and Updates in C#
Recent versions of C# have introduced numerous enhancements that are highly beneficial for desktop application development. These include improved asynchronous programming capabilities, pattern matching enhancements, and more robust null checking. Such features enable developers to write more efficient, clean, and maintainable code.
C# Sample in a WPF Application
To demonstrate the synergy between C# and WPF, let’s consider an example of a simple WPF application with C#. This application will feature a button that, when clicked, updates a label with the current time – showcasing basic event handling and UI interaction in a WPF application.
XAML Code:
<Window x:Class="WpfTimeApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Time App" Height="150" Width="300">
<StackPanel>
<Button x:Name="UpdateTimeButton" Click="UpdateTimeButton_Click">Update Time</Button>
<Label x:Name="TimeLabel">Time will be shown here</Label>
</StackPanel>
</Window>
C# Code Behind:
private void UpdateTimeButton_Click(object sender, RoutedEventArgs e)
{
TimeLabel.Content = DateTime.Now.ToString("HH:mm:ss");
}
In this example, clicking the “Update Time” button triggers the UpdateTimeButton_Click
event handler, which updates the TimeLabel
‘s content to the current time. This illustrates how C# and WPF work together to create interactive and responsive UIs.
Designing UIs with WPF
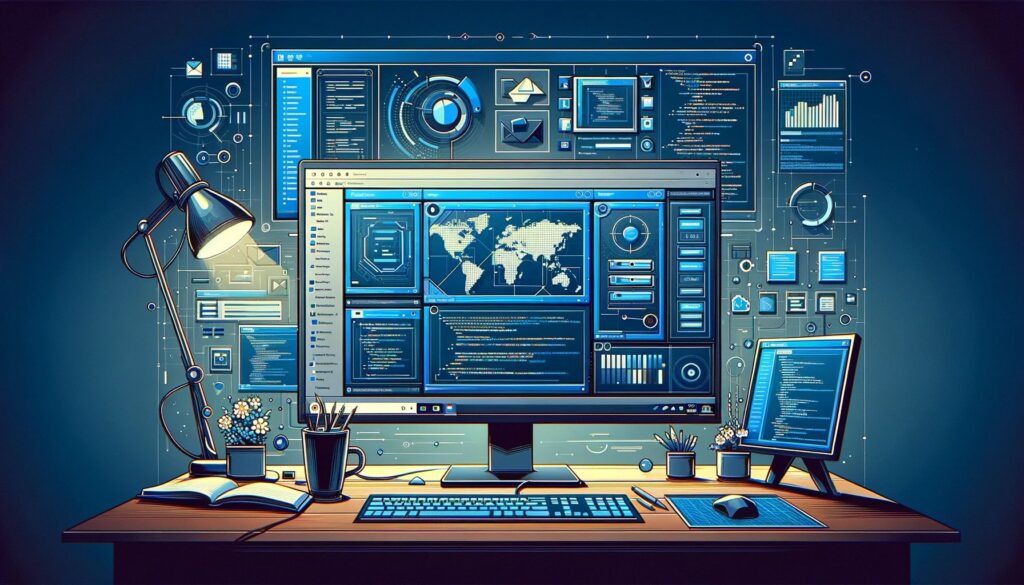
Windows Presentation Foundation (WPF) is renowned for its ability to create sophisticated and visually appealing user interfaces. It leverages XAML, a powerful markup language, to design UIs, making it a preferred choice for applications that demand a high level of visual finesse.
Key Features of WPF for UI Design
WPF introduces a vector-based rendering engine, allowing applications to scale seamlessly across devices without losing graphical fidelity. This is crucial in an era where high-DPI displays are common. Additionally, WPF’s support for advanced graphics, animations, and 3D rendering opens up possibilities for dynamic and interactive UIs.
Data binding in WPF is another powerful feature, enabling seamless communication between the UI and business logic layers. This makes the UI dynamic, automatically updating in response to data changes, thereby reducing the need for boilerplate code.
Advanced UI Elements and Data Binding in WPF
WPF’s control library includes a range of advanced UI elements such as custom controls, templates, styles, and animations. These elements can be extensively customized, giving developers the ability to create unique user experiences tailored to specific application requirements.
Example: Creating a Data-Bound List in WPF
To illustrate the use of data binding in WPF, let’s create a simple application with a list box that is bound to a data source. This example demonstrates how data binding can be used to display a list of items in the UI.
XAML Code:
<Window x:Class="DataBoundListApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Data Bound List" Height="350" Width="525">
<Grid>
<ListBox x:Name="ItemsListBox"/>
</Grid>
</Window>
C# Code Behind:
public MainWindow()
{
InitializeComponent();
List<string> items = new List<string> { "Item 1", "Item 2", "Item 3" };
ItemsListBox.ItemsSource = items;
}
In this example, the ListBox
named ItemsListBox
is bound to a list of strings defined in the C# code. This demonstrates how WPF’s data binding can simplify the process of displaying data in the UI.
Integrating WPF with .NET MAUI for Cross-platform Reach
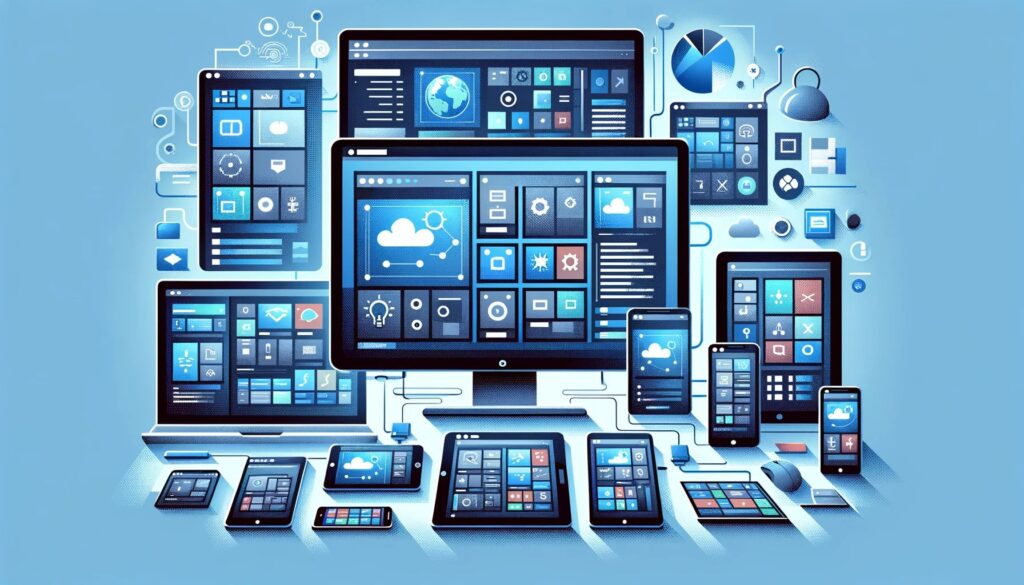
With the advent of .NET Multi-platform App UI (.NET MAUI), Microsoft has expanded the reach of traditional Windows-based applications. .NET MAUI allows developers to create applications that run not only on Windows but also on Android, iOS, and macOS, all from a single code base. This integration is particularly significant for WPF applications, offering a pathway to cross-platform functionality.
.NET MAUI and WPF Compatibility
.NET MAUI is an evolution of Xamarin.Forms and is designed to work seamlessly with existing .NET ecosystems, including WPF. It extends the capabilities of WPF by providing a unified framework for building UIs that can run on multiple platforms while leveraging native platform capabilities.
The Future of WPF in Multi-platform Development
The integration of WPF with .NET MAUI is a significant step in the evolution of WPF, as it opens up new possibilities for applications to be more versatile and accessible. This combination is particularly appealing for enterprises that want to maintain a single codebase for their applications across various platforms.
Example: A Simple .NET MAUI Application
To demonstrate how .NET MAUI can be used to create a cross-platform application, consider a simple example of an application with a single button that displays a greeting. This example will show the basic structure without platform-specific details.
XAML Code (Shared in .NET MAUI Project):
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MauiApp.MainPage">
<VerticalStackLayout Spacing="25" Padding="30">
<Button x:Name="GreetButton" Text="Greet" Clicked="OnGreetButtonClicked"/>
<Label x:Name="GreetingLabel"/>
</VerticalStackLayout>
</ContentPage>
C# Code Behind:
public partial class MainPage : ContentPage
{
public MainPage()
{
InitializeComponent();
}
private void OnGreetButtonClicked(object sender, EventArgs e)
{
GreetingLabel.Text = "Hello, .NET MAUI!";
}
}
In this example, clicking the “Greet” button triggers the OnGreetButtonClicked event, updating the GreetingLabel text. This code is shared across all platforms, demonstrating the power of .NET MAUI in creating cross-platform applications.
Best Practices and Patterns in WPF and C#
When developing applications with WPF and C#, adhering to best practices and design patterns is crucial for creating efficient, maintainable, and scalable software. These practices not only enhance code quality but also streamline the development process.
Effective Design Patterns for Robust Development
Design patterns provide a tested and proven approach to solving common software design problems. In WPF and C# development, some of the most effective patterns include:
- MVVM (Model-View-ViewModel): This pattern is particularly well-suited for WPF applications. It separates the UI (View) from the business logic (Model) with an intermediary (ViewModel), facilitating easier maintenance and testing.
- Singleton: Ensures a class has only one instance and provides a global point of access to it. This is useful for managing resources like database connections.
- Factory Method: Useful for creating objects without specifying the exact class of the object that will be created. This adds flexibility to your code, especially when dealing with complex object creation.
Performance Optimization in WPF Applications
Optimizing the performance of WPF applications involves several key considerations:
- Resource Management: Efficiently manage resources like brushes, templates, and styles to improve rendering performance.
- Data Binding Optimization: Use lightweight data binding where possible and avoid unnecessary bindings to enhance UI responsiveness.
- UI Virtualization: Implement virtualization for controls like ListView and DataGrid to enhance the performance when dealing with large datasets.
C# Sample Implementing MVVM Pattern
To illustrate the MVVM pattern, consider a simple WPF application where the ViewModel updates a property that the View binds to:
ViewModel (C# Code):
public class MainViewModel : INotifyPropertyChanged
{
private string _message;
public string Message
{
get { return _message; }
set
{
_message = value;
OnPropertyChanged(nameof(Message));
}
}
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged(string propertyName)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
View (XAML Code):
<Window.DataContext>
<local:MainViewModel />
</Window.DataContext>
<Grid>
<TextBlock Text="{Binding Message}" />
</Grid>
In this example, the TextBlock
in the View is bound to the Message
property in the MainViewModel
. When Message
is updated, the UI automatically reflects these changes due to the data binding.
Conclusion
C# and WPF remain relevant and adaptable for Windows application development. C# is a versatile language, and WPF offers powerful UI capabilities. Together, they enable developers to create robust, aesthetically pleasing, and user-friendly applications using best practices like MVVM.
Looking ahead, WPF’s integration with .NET MAUI facilitates cross-platform development, making C# and WPF essential tools that adapt to changing software development needs. Staying updated on these technologies is crucial for success in Windows app development.