Introduction to Database Connectivity in C++
The integration of databases in C++ applications represents a fundamental aspect of modern software engineering. This synergy allows programs to store, retrieve, and manipulate data with high efficiency, supporting a wide range of applications from small desktop programs to large-scale enterprise systems.
The Role of Databases in C++ Applications
Databases are essential for managing the vast amounts of data that modern applications handle. They offer structured storage, efficient data retrieval, and powerful query capabilities. In C++, connecting to databases enables developers to build applications that can interact with stored data, perform complex analyses, and maintain data integrity and security.
Historical Perspective
The journey of database connectivity in C++ began with early databases, where the interaction was primarily through embedded SQL or proprietary APIs. As technology evolved, so did the approaches to database connectivity. The introduction of standards like ODBC (Open Database Connectivity) and JDBC (Java Database Connectivity, often used in tandem with C++ for client-server models) provided more unified and efficient ways to connect with various database systems.
Evolution of Techniques and Tools
Over time, C++ has seen the development of numerous libraries and APIs designed to simplify database interactions. Libraries like SQLite, MySQL Connector/C++, and PostgreSQL’s libpq offer C++ programmers direct and streamlined access to respective database systems. These tools abstract the complexities of direct database communication, allowing developers to focus more on application logic rather than low-level data handling.
Significance in Modern Applications
In today’s application landscape, database connectivity is not just a feature but a necessity. From handling user data in web services to performing real-time data analysis in financial systems, C++ applications rely heavily on databases for various critical functions. The ability to efficiently connect and interact with databases is a key skill for C++ developers.
Key Concepts and Components
Understanding database connectivity in C++ involves several key concepts:
- Database Drivers: These are specific to each database system (e.g., SQLite, MySQL, PostgreSQL) and are essential for establishing a connection between the C++ application and the database.
- SQL (Structured Query Language): The standard language for interacting with relational databases. C++ applications use SQL queries to communicate with databases.
- Connection Handling: This includes opening, managing, and closing connections to a database. Effective connection management is crucial for the performance and stability of C++ applications.
- Data Retrieval and Manipulation: Fetching data from databases and updating or inserting new data are fundamental operations in database connectivity.
- Error Handling: Robust error handling mechanisms are vital to gracefully manage exceptions and issues during database interactions.
Setting Up Your C++ Environment for Database Interaction
Establishing a robust C++ environment for database connectivity is a foundational step in developing database-driven applications. This section guides you through selecting the right tools and libraries and setting up your development environment to seamlessly integrate C++ with various database systems.
Choosing the Right Tools and Libraries
The choice of tools and libraries for database connectivity in C++ largely depends on the database system you plan to interact with. Here are some popular options:
- SQLite: Ideal for lightweight applications, embedded systems, or situations where a full-fledged database server is not required. SQLite is renowned for its simplicity, reliability, and ease of integration.
- MySQL Connector/C++: A standard choice for applications that require interaction with MySQL databases. It provides a direct interface to MySQL, offering both high performance and a comprehensive set of features.
- PostgreSQL libpq: The official C library for PostgreSQL, libpq is suitable for applications that need to connect to PostgreSQL databases. It offers advanced features and supports a wide range of PostgreSQL-specific functionalities.
Configuring the Development Environment
The configuration of the development environment involves installing the necessary libraries and setting up your IDE (Integrated Development Environment) or code editor. Here’s a step-by-step guide to get you started:
- Install the Database System: Begin by installing the database system (e.g., SQLite, MySQL, PostgreSQL) on your machine. Ensure you have the server running and accessible.
- Install C++ Database Libraries: Download and install the corresponding C++ libraries for your chosen database system. For example, if you’re using MySQL, install the MySQL Connector/C++.
- Set Up Your IDE or Code Editor: Choose an IDE or code editor that supports C++ development, such as Visual Studio, Eclipse, or Code::Blocks. Configure the IDE to recognize the installed database libraries. This usually involves setting the library paths and linking the required files in your project settings.
- Test the Setup: Create a simple C++ program that attempts to connect to the database. This step is crucial to verify that the environment is correctly set up and the libraries are properly linked.
Essential Configuration Parameters
When setting up database connectivity in C++, certain key parameters need to be configured:
- Database URL/Path: The location of the database. For example, in SQLite, this could be the file path to the database file.
- User Credentials: Username and password for databases that require authentication, like MySQL or PostgreSQL.
- Port Number: The port number on which the database server listens.
- Additional Parameters: Depending on the library and database, you might need to configure additional parameters like timeout values, encryption options, or connection pool settings.
Understanding the Basics of SQL and C++ Integration
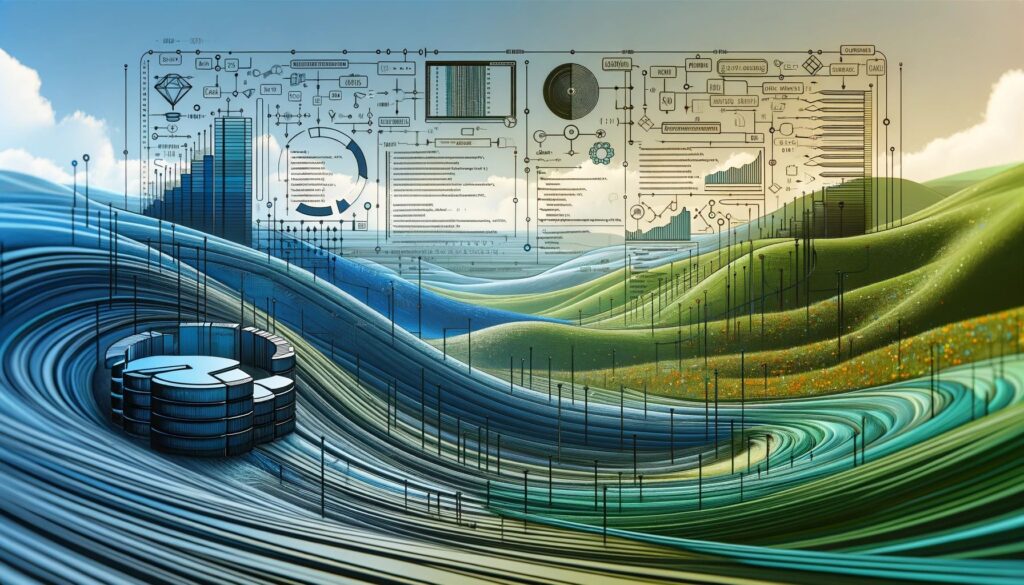
Integrating SQL within C++ applications is a critical skill for database connectivity. This section delves into the fundamentals of using SQL in C++ and provides examples to illustrate these concepts in action.
The Essentials of SQL in C++
SQL (Structured Query Language) is the standard language used to communicate with databases. Its primary purpose is to retrieve, insert, update, and delete data within a database. Here’s a quick overview of the core SQL operations and their purpose:
- SELECT: Retrieves data from one or more tables.
- INSERT: Adds new records to a table.
- UPDATE: Modifies existing records in a table.
- DELETE: Removes records from a table.
Incorporating SQL into C++ Programs
Integrating SQL into C++ involves establishing a connection to the database, executing SQL queries, and processing the results. Here’s a typical workflow:
- Establish a Database Connection: Use the appropriate library to connect to the database (as discussed in the previous section).
- Prepare SQL Queries: Formulate your SQL query as a string within your C++ code.
- Execute Queries: Use the database library’s functions to execute the query.
- Process Results: Handle the data returned from SELECT queries, or confirm the success of INSERT, UPDATE, and DELETE operations.
Example: Basic Database Operations
Here’s a simple example demonstrating basic SQL operations in C++ using SQLite:
#include <sqlite3.h>
// Function to execute a query and handle results
void executeQuery(sqlite3* db, const char* sql) {
char* errMsg;
int rc = sqlite3_exec(db, sql, callback, 0, &errMsg);
if (rc != SQLITE_OK) {
std::cerr << "SQL error: " << errMsg;
sqlite3_free(errMsg);
}
}
int main() {
sqlite3* db;
sqlite3_open("example.db", &db);
// Create a table
const char* createTable = "CREATE TABLE employees (id INTEGER PRIMARY KEY, name TEXT, age INTEGER);";
executeQuery(db, createTable);
// Insert data
const char* insertData = "INSERT INTO employees (name, age) VALUES ('John Doe', 30);";
executeQuery(db, insertData);
// Select data
const char* selectData = "SELECT * FROM employees;";
executeQuery(db, selectData);
sqlite3_close(db);
return 0;
}
In this example, we create a table, insert a record, and then select data from the database. The executeQuery function encapsulates the logic for executing SQL statements and handling errors.
Handling SQL Results in C++
When executing a SELECT query, you will typically receive a result set. In C++, handling this data often involves iterating over the rows in the result set and extracting the values of each column. Different libraries provide various mechanisms for this, such as iterators, cursors, or callback functions.
Best Practices for SQL in C++
- Prepared Statements: Use prepared statements to execute SQL queries more efficiently and securely. They prevent SQL injection attacks and optimize performance by pre-compiling the SQL statement.
- Transaction Management: Use transactions to ensure data integrity, particularly when performing multiple related database operations.
- Error Handling: Robust error handling is crucial for detecting and responding to database errors, such as connection issues or query failures.
- Resource Management: Ensure that database connections and resources are properly closed or released after use to avoid memory leaks and other resource management issues.
Advanced Database Operations with C++
As developers grow more proficient in database connectivity with C++, they can explore advanced database operations that enable more complex and efficient data manipulation. This section covers such advanced techniques, including complex queries, optimization strategies, and security considerations.
Complex Queries and Data Manipulation
Advanced database operations often involve more intricate SQL queries. These can include:
- Join Operations: Combining data from multiple tables into a single result set. For instance, using INNER JOIN, LEFT JOIN, or FULL JOIN to correlate data across tables based on a related column.
- Subqueries: Queries nested within other queries. They are useful for performing operations that require multiple steps, such as filtering data based on aggregate functions.
- Stored Procedures and Functions: These are SQL scripts stored in the database that can be executed by the C++ application. They are beneficial for encapsulating complex logic within the database.
- Triggers: Automated actions triggered by specific events in the database, such as inserting or updating a row.
- Batch Operations: Performing multiple insertions or updates in a single operation, which can significantly enhance performance by reducing the number of round-trips to the database.
Example: Executing a Complex Join Query
// Assuming a connection 'db' is already established
const char* joinQuery = "SELECT employees.name, departments.name FROM employees INNER JOIN departments ON employees.dept_id = departments.id;";
executeQuery(db, joinQuery);
This example demonstrates a basic join operation between two tables, employees and departments, linking them via the dept_id field.
Database Optimization Strategies
- Indexing: Proper use of indexes can significantly improve the performance of queries, especially in large datasets.
- Query Optimization: Analyzing and refining SQL queries to ensure they execute efficiently. This can involve using EXPLAIN statements to understand how queries are executed.
- Caching Results: Storing frequently accessed data in memory to reduce database access times.
- Connection Pooling: Reusing database connections instead of opening new ones for each query, reducing overhead and improving performance.
Security Considerations
- SQL Injection Protection: Using prepared statements and parameterized queries to prevent SQL injection attacks.
- Data Encryption: Encrypting sensitive data both in transit and at rest.
- Access Control: Implementing robust authentication and authorization mechanisms to control access to the database.
- Auditing and Logging: Keeping detailed logs of database operations for security auditing and troubleshooting.
Best Practices for Advanced Operations
- Regularly Review and Optimize Queries: Continuously monitor and optimize your SQL queries for performance and efficiency.
- Use Database-Specific Features Judiciously: While leveraging database-specific features can be powerful, it can also lead to vendor lock-in. Striking the right balance is key.
- Continuously Monitor Database Performance: Regularly monitor your database’s performance metrics to identify potential bottlenecks or inefficiencies.
- Stay Updated with Security Best Practices: Database security is an ever-evolving field. Keeping abreast of the latest security trends and best practices is crucial.
Error Handling and Debugging in Database Connectivity
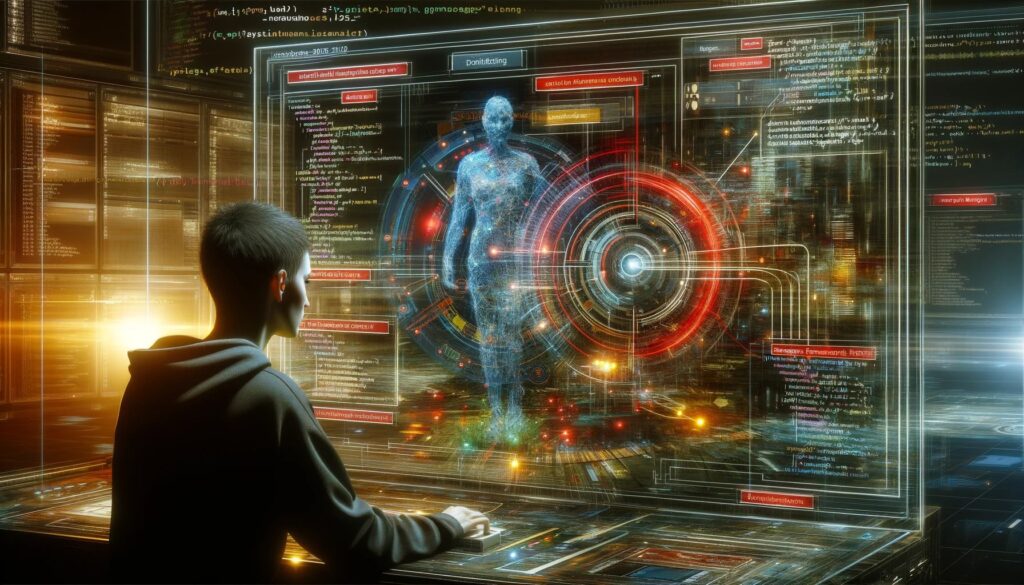
Effective error handling and debugging are crucial in database connectivity to ensure the robustness and reliability of C++ applications. This section discusses strategies for identifying and resolving common issues in database interactions.
Understanding Common Database Errors
Database operations in C++ can fail due to various reasons, such as:
- Connection Errors: Issues in establishing a connection with the database server, often due to incorrect credentials, network issues, or server unavailability.
- Syntax Errors in SQL: Errors in SQL query syntax, which could lead to failed execution.
- Data Integrity Violations: Constraints like unique keys, foreign keys, or not-null constraints being violated.
- Resource Limitations: Running out of memory or exceeding storage limits.
Strategies for Effective Error Handling
- Proper Use of Exceptions: C++ provides exception handling mechanisms that should be used to capture and handle database-related errors.
- Checking Return Values: Always check the return values of database function calls to detect failures.
- Using Database-Specific Error Reporting Mechanisms: Most database libraries provide functions to retrieve detailed error messages. For instance, in SQLite, sqlite3_errmsg() returns the last error message.
- Transaction Management: Use transactions to handle multi-step operations, allowing rollback in case of errors.
Debugging Techniques
- Logging: Implement detailed logging at various points in your database interaction code to capture the state and flow of execution.
- Using Debuggers: Utilize IDE debuggers to step through your code and inspect variables and database connections.
- SQL Query Analysis: Analyze complex SQL queries separately using tools like SQL Fiddle or database-specific query analyzers.
- Profiling and Monitoring Tools: Use profiling tools to monitor database performance and identify bottlenecks.
Example: Basic Error Handling in C++
Here’s a simple example using SQLite:
int result = sqlite3_exec(db, sql, callback, 0, &errMsg);
if (result != SQLITE_OK) {
std::cerr << "SQL Error: " << errMsg;
sqlite3_free(errMsg);
// Handle error (e.g., rollback transaction, retry, etc.)
}
In this example, we check the return value of sqlite3_exec and output an error message if it indicates a failure.
Optimizing Database Performance in C++ Applications
Optimizing database performance is vital for ensuring that C++ applications remain efficient and responsive, particularly when dealing with large datasets or high transaction volumes. This section outlines key strategies to enhance the performance of database operations in C++.
Indexing for Performance
- Purpose of Indexing: Indexes are used to speed up the retrieval of rows from a database table. An index creates an entry for each value and a reference to the corresponding row, much like an index in a book.
- Best Practices:
Index columns that are frequently used in the WHERE clause of queries.
Avoid over-indexing, as this can slow down write operations (INSERT, UPDATE, DELETE).
Regularly monitor and optimize indexes based on query patterns.
Query Optimization
Writing Efficient Queries: The way SQL queries are written can significantly impact performance.
- Use specific column names instead of SELECT *.
- Minimize the use of subqueries and complex joins where possible.
- Use query execution plans to understand and optimize query performance.
Caching and Prepared Statements:
- Implement caching to store and reuse query results, reducing the number of database hits.
- Use prepared statements to improve performance and security, especially for repeated queries.
Connection Pooling
- What is Connection Pooling?: Connection pooling involves maintaining a pool of database connections that can be reused, rather than opening and closing a new connection for each query.
- Advantages:
Reduces the overhead of creating and closing connections.
Improves application performance, especially under high load. - Implementing Connection Pooling: Many C++ database libraries support connection pooling, and it can typically be configured with parameters like pool size and connection timeout.
Memory and Resource Management
- Managing Resources: Ensure efficient use of resources such as memory and file handles. This includes properly releasing any resources used by database connections and queries.
- Monitoring and Profiling: Use tools to monitor memory usage and performance bottlenecks. Profiling tools can help identify inefficient queries or memory leaks in the application.
Conclusion
Mastering database connectivity in C++ is a multifaceted skill essential for developing robust and scalable software. This article has navigated through setting up environments, basic and advanced SQL integrations, and optimization strategies, concluding with real-world applications. Fundamental understanding of SQL, database connections, and CRUD operations lay the groundwork for proficiency in this area. Advanced techniques like complex queries and performance optimization significantly enhance application capabilities. Robust error handling and debugging ensure reliability, while prioritizing security through practices like using prepared statements and encryption is crucial. As technology evolves, staying updated with developments in database technologies and programming methods remains important. Practical application and continuous skill refinement through real-world projects are recommended for developers to fully grasp and apply these concepts. This comprehensive exploration into database connectivity with C++ serves as a valuable resource for both novice and seasoned developers in their software development endeavors.