In the realm of software development, integrating databases with applications is a fundamental skill, crucial for creating robust and dynamic systems. Visual Basic, a programming language developed by Microsoft, plays a significant role in this integration, especially for developers working with Windows-based applications. This section delves into the basics of Visual Basic (VB) in the context of database access, highlighting its importance and utility in modern software development.
The Role of Visual Basic in Database Integration
Visual Basic, with its user-friendly interface and versatile programming environment, is an excellent tool for creating applications that require database interactions. Its simplicity does not come at the expense of power; VB is capable of handling complex database operations with relative ease. The language’s integration with Microsoft Access and SQL Server makes it a preferred choice for many developers working on Windows platforms.
Key Characteristics of Visual Basic:
- Ease of Use: VB’s intuitive drag-and-drop interface allows developers to quickly design user interfaces.
- Rapid Application Development: VB accelerates the development process, enabling the quick creation of prototypes and applications.
- Strong Database Connectivity: VB provides robust tools for connecting to and manipulating databases.
Importance of Database Integration
In today’s data-driven world, the ability to efficiently store, retrieve, and manipulate data is vital for any application. Database integration allows applications to interact with data in a structured manner, facilitating tasks such as data entry, querying, and data analysis. This integration is essential for applications ranging from simple inventory management systems to complex analytical tools.
Benefits of Effective Database Integration:
- Data Management: Efficient organization and retrieval of data.
- Scalability: Ability to handle increasing amounts of data as the application grows.
- Data Integrity: Ensuring the accuracy and consistency of data across the application.
Visual Basic and Microsoft Access
Visual Basic’s integration with Microsoft Access is particularly noteworthy. Access serves as a powerful database management system, and when combined with VB, it offers a comprehensive environment for database application development. This synergy allows for the creation of applications that are not only efficient in data handling but also user-friendly, catering to a wide range of business needs.
Setting Up Your Environment
Before diving into the world of database access with Visual Basic, it’s crucial to set up your development environment correctly. This section will guide you through the necessary steps to ensure a seamless integration between Visual Basic and your chosen database system, whether it’s Microsoft Access or SQL Server.
Compatibility Check
One of the first considerations is compatibility between the versions of Microsoft Office and Visual Studio you are using. A mismatch in the bitness (32-bit or 64-bit) of these software packages can lead to compatibility issues and errors when connecting to a database.
Here’s what you need to ensure:
- If you are using a 32-bit version of Microsoft Office, make sure you are using Visual Studio 2019 or an earlier version.
- If you are using a 64-bit version of Microsoft Office, you should use Visual Studio 2022 or a later version.
Connecting to an Access Database
Microsoft Access is a widely used database management system, and integrating it with Visual Basic is a common scenario. Let’s walk through the steps to connect your Visual Basic application to an Access database:
- Open Visual Studio: Launch Visual Studio, and create or open your VB project.
- Access Database: Ensure you have your Access database file (.accdb) ready.
- Data Connection: In your project, go to the “Data” menu and select “Add New Data Source.”
- Choose Data Source Type: Select “Database” as your data source type.
- Select Database Model: Choose “Dataset” as the database model.
- Configure Data Connection: Click on “New Connection” to configure a new data connection.
- Set Data Source: Make sure “Microsoft Access Database File” is selected as the data source.
- Browse for Database: Browse and select your Access database file.
- Test Connection: Click on “Test Connection” to ensure the connection is successful.
- Select Database Objects: Expand the “Tables” node and select the tables or views you want to include in your dataset.
- Finish: Click “Finish” to complete the process. The dataset will be added to your project, and the selected tables will appear in the Data Sources window.
Congratulations! You’ve successfully set up a connection between your Visual Basic project and a Microsoft Access database. Now you can work with the data from these tables within your VB application.
Basic Database Operations in Visual Basic
Now that you have your Visual Basic environment set up to connect to a database, it’s time to explore fundamental database operations. In this section, we’ll cover how to manipulate records one at a time and leverage the Command Button Wizard to perform common programming tasks within your Visual Basic application.
Manipulating Records One at a Time
One of the essential aspects of database access is the ability to work with individual records. Visual Basic allows you to manipulate records in a database one at a time, making it easy to perform operations such as viewing, updating, or deleting specific data entries.
Here’s a basic example of how you can use Visual Basic to step through a set of records and perform an operation on each record:
Dim conn As New OleDbConnection("Your_Connection_String_Here")
Dim cmd As New OleDbCommand()
Dim reader As OleDbDataReader
Try
conn.Open()
cmd.Connection = conn
cmd.CommandText = "SELECT * FROM YourTableName"
reader = cmd.ExecuteReader()
While reader.Read()
' Access individual fields in the current record
Dim id As Integer = reader.GetInt32(0)
Dim name As String = reader.GetString(1)
' Perform operations on the record, e.g., display or update
Console.WriteLine($"ID: {id}, Name: {name}")
End While
Catch ex As Exception
Console.WriteLine("Error: " & ex.Message)
Finally
' Close the database connection
conn.Close()
End Try
In this code snippet:
- We establish a connection to the database using the
OleDbConnection
class. - We define an SQL command to select records from a specific table.
- We use a
While
loop to iterate through each record in the result set obtained from executing the SQL command. - Inside the loop, we access individual fields in the current record and perform operations as needed.
Using the Command Button Wizard
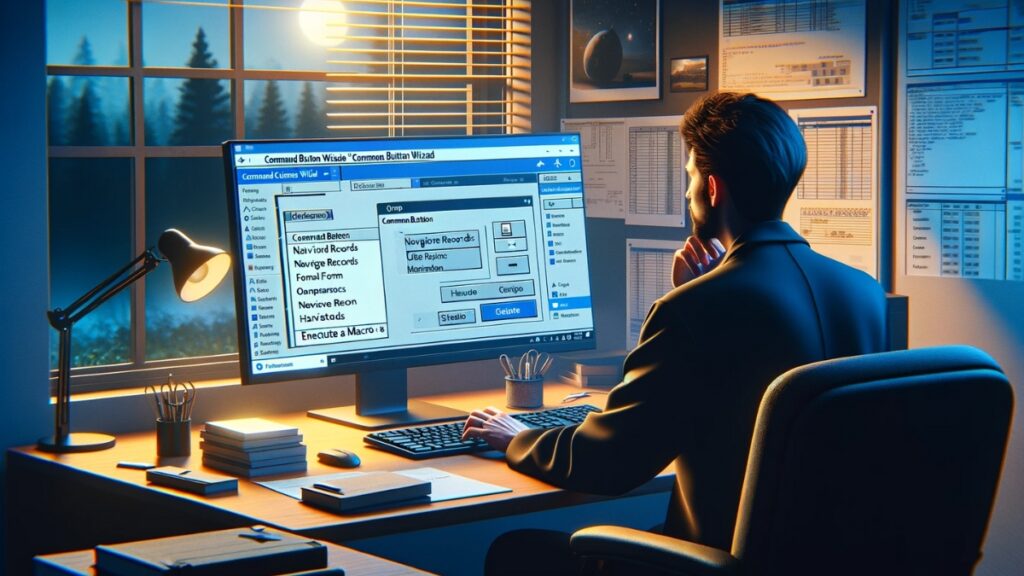
Visual Basic provides a helpful tool known as the Command Button Wizard, which simplifies the process of adding functionality to command buttons in your forms. This wizard assists in creating command buttons that perform specific tasks when clicked, such as saving data, navigating records, or executing custom actions.
Here are the steps to use the Command Button Wizard:
- In your Visual Basic project, open the form to which you want to add the command button in Design View.
- On the Design tab, access the Controls gallery and ensure that “Use Control Wizards” is selected.
- In the Controls gallery, click the “Button” control.
- Place the button on the form where you want it to appear.
- The Command Button Wizard will start automatically.
- On the first page of the wizard, select the action you want the button to perform, such as navigating records or executing a macro.
- Choose whether you want text or a picture to be displayed on the button.
- Enter a meaningful name for the command button.
- Complete the wizard, and the button will be created with the specified functionality.
The Command Button Wizard streamlines the process of adding interactivity to your forms, making it easier to create user-friendly database applications.
Advanced Data Handling Techniques
In the world of database access with Visual Basic, mastering advanced data handling techniques is essential for building robust and error-resilient applications. In this section, we’ll explore two critical aspects: implementing robust error handling and explicitly typecasting function return values. These techniques ensure data integrity and smooth operation of your Visual Basic applications.
Implementing Robust Error Handling
Error handling is a crucial part of any software development process, and Visual Basic provides powerful tools to handle errors gracefully. By anticipating and handling errors, you can prevent unexpected crashes and provide meaningful feedback to users. Here’s an example of how to implement error handling in your Visual Basic code:
Try
' Code that may cause an error
Dim result As Integer = 10 / 0 ' This will cause a runtime error
Catch ex As Exception
' Handle the error gracefully
MessageBox.Show("An error occurred: " & ex.Message, "Error", MessageBoxButtons.OK, MessageBoxIcon.Error)
End Try
In the code above:
- We enclose the code that may cause an error within a
Try...Catch
block. - If an error occurs, it’s caught by the
Catch
block, and we can take appropriate action, such as displaying an error message to the user.
By implementing robust error handling like this, you can prevent unexpected crashes and guide users on how to address errors.
Explicitly Typecasting Function Return Values
Visual Basic is known for its flexibility in handling data, but this flexibility can sometimes lead to unexpected results. To ensure data integrity, especially when working with functions that return values, it’s essential to explicitly typecast the return values to their expected data types.
Here’s an example of explicit typecasting when working with a function that returns a value:
' Define a function that returns a value
Function CalculateAverage(num1 As Double, num2 As Double) As Double
Return (num1 + num2) / 2
End Function
' Explicitly typecast the return value to Double
Dim result As Double = CDbl(CalculateAverage(5, 7))
In this code:
- We define a function
CalculateAverage
that returns a value of typeDouble
. - When calling the function, we use
CDbl
to explicitly typecast the return value toDouble
.
By explicitly typecasting return values, you ensure that the data type of the result matches your expectations, reducing the risk of unexpected errors.
These advanced data handling techniques in Visual Basic pave the way for more robust and reliable database applications. By implementing error handling and typecasting, you can enhance the overall quality of your code and provide a smoother experience for both developers and users.
Working with ADO Events
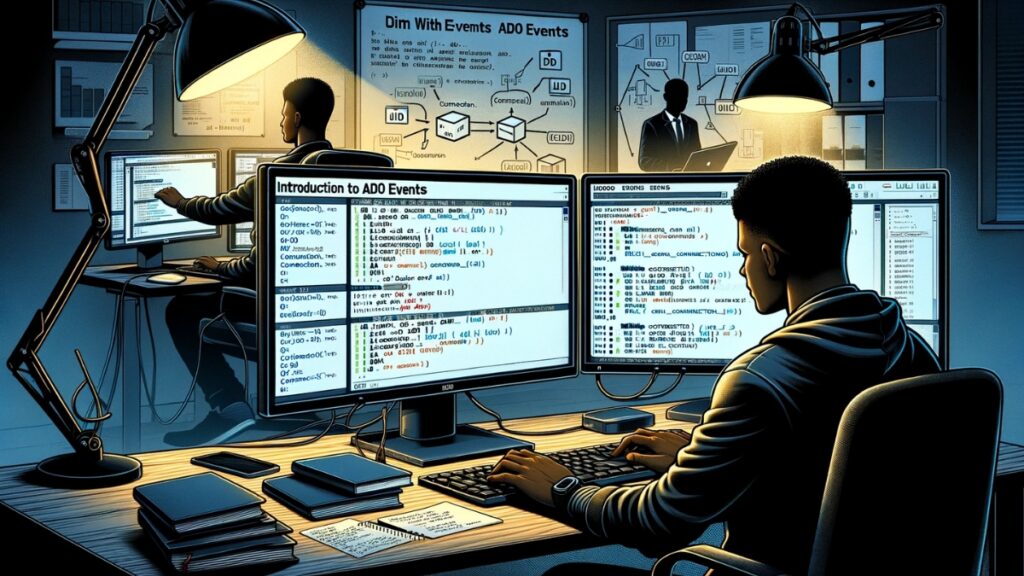
In the realm of database access with Visual Basic, handling ADO (ActiveX Data Objects) events is a powerful technique that allows you to respond to specific database-related events and execute custom logic accordingly. ADO events provide a way to enhance the interactivity and responsiveness of your Visual Basic applications. In this section, we’ll explore how to handle ADO events effectively.
Introduction to ADO Events
ActiveX Data Objects (ADO) is a technology that allows Visual Basic applications to interact with various data sources, including databases. ADO events are notifications triggered by specific actions or changes in the data source. By handling these events, you can execute code in response to database-related activities, such as connecting, disconnecting, or executing SQL statements.
Here’s a basic example of how to declare and handle an ADO event in Visual Basic:
Dim WithEvents conn As New ADODB.Connection
Private Sub Form_Load()
' Set up the connection string
Dim connStr As String = "Provider=SQLOLEDB;Data Source=ServerName;Initial Catalog=DatabaseName;Integrated Security=SSPI;"
conn.ConnectionString = connStr
' Open the database connection
conn.Open
' Handle the ConnectionComplete event
MsgBox("Database connection established.")
End Sub
Private Sub conn_ConnectionComplete(ByVal pError As ADODB.Error, adStatus As ADODB.EventStatusEnum, ByVal pConnection As ADODB.Connection)
If adStatus = adStatusOK Then
MsgBox("Connection was successful.")
Else
MsgBox("Connection failed.")
End If
End Sub
In this example:
- We declare a
conn
variable with theWithEvents
keyword to enable event handling for theADODB.Connection
class. - In the
Form_Load
event, we set up the connection string and open the database connection. - We handle the
ConnectionComplete
event, which is triggered when the connection is complete. Depending on the connection status, we display a message.
Common ADO Events
ADO events cover various aspects of database interactions. Here are some common ADO events you may encounter:
- ConnectionComplete: Triggered when the connection to the database is successfully established.
- BeginTransComplete: Raised when a transaction begins.
- CommitTransComplete: Fired when a transaction is successfully committed.
- RollbackTransComplete: Triggered when a transaction is rolled back.
- ExecuteComplete: Raised when an SQL statement or stored procedure execution is complete.
These events allow you to respond to database actions in real time, making your Visual Basic applications more interactive and dynamic.
Practical Applications
Handling ADO events is particularly valuable in scenarios where you need to respond to changes in the database or provide immediate feedback to users. For example, you can use ADO events to:
- Display a message when a database connection is established or fails.
- Notify users when a transaction is successfully completed.
- Update the user interface when data changes in the database.
Linking Data Sources to an Application
In the world of Visual Basic application development, one of the key skills is the ability to link data sources to your application. This step allows you to create forms and interfaces that interact seamlessly with your database, providing users with a dynamic and data-driven experience. In this section, we’ll explore how to link data sources to your Visual Basic applications effectively.
Displaying Data Sources in Visual Studio
Visual Studio provides a powerful feature called the “Data Sources” panel, which allows you to link data sources to your application and easily integrate database functionality. Here’s how to access and utilize this feature:
- Open Visual Studio: Launch Visual Studio and open your Visual Basic project.
- Show Data Sources Panel: In the Visual Studio menu, navigate to “Data” and select “Show Data Sources.” This action will display the Data Sources panel.
- Data Sources Panel: The Data Sources panel lists all the available data tables and columns from your connected data source, whether it’s a Microsoft Access database, SQL Server database, or another source.
- Select Data: Click on the ‘+’ sign next to a table to expand the list of data fields and columns associated with that table.
Adding Data Fields to Your Form
Once you have accessed the Data Sources panel and selected the data you want to work with, the next step is to add data fields to your Visual Basic form. Here’s how you can do that:
- Drag and Drop: From the Data Sources panel, simply drag and drop the data fields you want to use onto your form. For example, you can add fields like “CustomerID,” “CompanyName,” “Address,” and “Country” to your form.
- User Interface Elements: Visual Basic will automatically generate user interface elements for each data field you add. These elements could include textboxes, labels, and navigation controls, making it easy to create a user-friendly interface for interacting with your data.
Building a Database-Driven Application
Once you’ve added data fields to your form, Visual Basic does much of the heavy lifting for you. It creates the necessary data bindings and code to ensure that the data from your database is displayed and updated in real time. You can easily build applications that allow users to view, edit, and interact with the database seamlessly.
Running Your Application
To see your database-driven application in action, simply build and run your project (press F5). When the application starts, the data fields you added to your form will be populated with data from the linked database table. Users can interact with the data and make changes, and these changes can be saved back to the database if desired.
By linking data sources to your Visual Basic application, you empower it to become a powerful tool for working with databases. Whether you’re building a simple data entry form or a complex data analysis tool, understanding how to connect and utilize data sources is essential for creating effective data-driven applications.
Adding SQL Statements to a Visual Basic Application
In the realm of database access with Visual Basic, the ability to add and execute SQL (Structured Query Language) statements within your application is a powerful skill. SQL statements enable you to query and filter data from your database with precision and flexibility. In this section, we’ll explore how to add SQL statements to your Visual Basic application effectively.
The Importance of SQL in Database Access
SQL is the standard language for interacting with relational databases. It allows you to perform a wide range of operations, including selecting, filtering, updating, and deleting data. Incorporating SQL statements into your Visual Basic application expands its capabilities, enabling you to retrieve and manipulate data from your connected database.
Adding an SQL Query
To add an SQL query to your Visual Basic application, follow these steps:
- Select the Control: In your Visual Basic form, select the control (e.g., a textbox) to which you want to attach the SQL query. For this example, let’s assume you have a textbox named “txtFilter” where users can enter filter criteria.
- Add Query: With the control selected, go to the “Data” menu and choose “Add Query.”
- Query Builder: In the “Search Criteria Builder” dialog, you can specify the criteria for your query. For example, if you want to filter records where the “Country” field matches “France,” you would set the criteria as follows:
- Column Name: Select the “Country” column.
- Filter: Enter “France.”
- Generate SQL Statement: Click on “OK” to generate the SQL statement for your query. Visual Basic will create the SQL statement based on your criteria.
The generated SQL statement might look like this:
SELECT CustomerID, CompanyName, ContactName, ContactTitle, Address, City,
Region, PostalCode, Country, Phone, Fax FROM Customers WHERE (Country = 'France')
- Apply the SQL Statement: The generated SQL statement can be applied to a database connection or data adapter in your application. You can use it to filter and retrieve data based on user input or specific application requirements.
Example of Applying an SQL Query
Here’s an example of how you can apply an SQL query to filter data based on user input in your Visual Basic application:
Dim conn As New OleDbConnection("Your_Connection_String_Here")
Dim adapter As New OleDbDataAdapter()
Dim dataTable As New DataTable()
Try
conn.Open()
' Create an SQL command based on user input (e.g., txtFilter.Text)
Dim sql As String = "SELECT CustomerID, CompanyName, ContactName, Country FROM Customers WHERE Country = @Country"
Dim cmd As New OleDbCommand(sql, conn)
cmd.Parameters.AddWithValue("@Country", txtFilter.Text)
' Use a data adapter to fill a DataTable with the results
adapter.SelectCommand = cmd
adapter.Fill(dataTable)
' Bind the DataTable to a DataGridView for display
DataGridView1.DataSource = dataTable
Catch ex As Exception
MessageBox.Show("An error occurred: " & ex.Message, "Error", MessageBoxButtons.OK, MessageBoxIcon.Error)
Finally
conn.Close()
End Try
In this example:
- We create an SQL command with a parameterized query to filter data based on the user’s input (in this case, the country name).
- We use a data adapter to execute the SQL command and fill a DataTable with the results.
- Finally, we bind the DataTable to a DataGridView for display in the user interface.
By adding SQL statements to your Visual Basic application, you empower it to interact with your database dynamically, allowing users to filter and retrieve data as needed. This capability enhances the flexibility and usefulness of your database-driven applications.
Best Practices and Common Pitfalls
In the world of database access with Visual Basic, mastering best practices and avoiding common pitfalls is essential for creating efficient and error-free applications. In this section, we’ll explore important tips to write clean and effective code, ensuring that your Visual Basic applications perform reliably and provide a seamless user experience.
Use Parameterized Queries

One of the best practices in database access is to use parameterized queries whenever possible. Parameterized queries help prevent SQL injection attacks and ensure that data entered by users is treated as data, not executable code.
Here’s an example of a parameterized query in Visual Basic:
Dim conn As New OleDbConnection("Your_Connection_String_Here")
Dim adapter As New OleDbDataAdapter()
Dim dataTable As New DataTable()
Try
conn.Open()
' Create a parameterized SQL command
Dim sql As String = "SELECT CustomerID, CompanyName FROM Customers WHERE Country = ?"
Dim cmd As New OleDbCommand(sql, conn)
cmd.Parameters.AddWithValue("@Country", txtCountry.Text) ' Use parameters
' Use a data adapter to fill a DataTable with the results
adapter.SelectCommand = cmd
adapter.Fill(dataTable)
' Bind the DataTable to a DataGridView for display
DataGridView1.DataSource = dataTable
Catch ex As Exception
MessageBox.Show("An error occurred: " & ex.Message, "Error", MessageBoxButtons.OK, MessageBoxIcon.Error)
Finally
conn.Close()
End Try
Handle Errors Gracefully
Effective error handling is crucial for a robust application. Always implement error handling code to provide meaningful error messages to users and to log errors for debugging purposes. Use Try...Catch
blocks to handle exceptions gracefully.
Dispose of Resources
Proper resource management is vital. Ensure that you explicitly close database connections, dispose of objects, and release resources when they are no longer needed. Use the Using
statement to automatically dispose of objects when they go out of scope.
Using conn As New OleDbConnection("Your_Connection_String_Here")
' Code that uses the connection
End Using ' The connection is automatically closed and disposed of
Validate User Input
When accepting input from users, validate and sanitize it to prevent data integrity issues and security vulnerabilities. Use input validation techniques to ensure that the data entered meets expected criteria.
Optimize Database Operations
Efficiency is key when working with databases. Optimize your SQL queries, and avoid unnecessary data retrieval and processing. Use database indexes appropriately to speed up data retrieval.
Test Thoroughly
Test your Visual Basic application rigorously. Perform both unit testing and user acceptance testing to identify and fix issues. Ensure that your application works smoothly under various scenarios and handles edge cases gracefully.
Document Your Code
Maintain clear and well-documented code. Use meaningful variable and function names, add comments to explain complex logic, and create documentation that describes the purpose and usage of your code.
Avoid Hardcoding Credentials
Avoid hardcoding database connection strings and credentials in your code. Store them securely and consider using configuration files or environmental variables to manage sensitive information.
By following these best practices and avoiding common pitfalls, you can ensure that your Visual Basic applications are reliable, secure, and efficient in their interactions with databases. These principles will help you build high-quality database-driven applications that provide a seamless experience for users.
Conclusion
In conclusion, this guide has provided a comprehensive overview of database access with Visual Basic, equipping you with the essential skills to build robust and efficient applications. We began by setting up the development environment and establishing connections to databases, whether it’s Microsoft Access, SQL Server, or other data sources. We explored fundamental database operations, including manipulating records, using the Command Button Wizard, and implementing error handling and typecasting techniques to ensure data integrity. Furthermore, we delved into advanced topics such as handling ADO events, linking data sources to applications, adding SQL statements for precise data retrieval, and adhering to best practices for clean and secure coding. These skills are foundational for creating powerful, data-driven Visual Basic applications.
By following the principles outlined in this guide, you can confidently work with databases, query and manipulate data, and build user-friendly interfaces that enhance the user experience. Whether you are a beginner looking to get started or an experienced developer seeking to refine your skills, the world of database access with Visual Basic offers a wealth of opportunities to create dynamic and responsive applications that meet a wide range of business and data management needs. Keep exploring, practicing, and refining your database access skills, and you’ll be well-equipped to tackle complex data-driven projects with confidence.