You'll learn how to support drag and drop operations in an application - dragging and dropping files on a form or control, using key combinations, retrieving information about the dropped files...
Drag and drop is the most natural way of doing some operations in software applications like adding items to a list. Thanks to .NET implementing drag and drop in your program is a very simple task. So let’s cut to the chase.
Start a new C# Windows Application project which will create Form1, the form we’ll be working with.
First you have to change the AllowDrop property of the form to True.
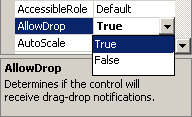
Change to the Events window now and scroll down to the DragEnter event:
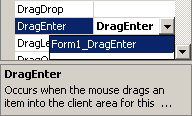
This is the first event we are interested in, double click it so it can be created.
Now I should tell you that the DragEnter event of the form fires up when you drag something (an icon) on the form but you don’t yet drop it there (release the mouse button).
In other applications we know,
what happens when you drag something on the form or over some control? Usually the cursor appears with a ‘plus’ (+) sign next to it to denote copying. But a arrow (typical shortcut arrow) can appear when creating a shortcut as a result of the drag and drop, or a rectangle when moving. Or all of them. Let’s see.
Let’s suppose that by default when dragging an icon on the form we want to move it, so on the DragEnter event use this:
private void Form1_DragEnter(object sender, System.Windows.Forms.DragEventArgs e)
{
   e.Effect = DragDropEffects.Move;
}
As you can see, we are using the DragEventArgs
e
Effect property. Now try to drag an icon on the form and you’ll see that next to the cursor the rectangle that symbolizes moving.
But there are applications which when you hold the Ctrl or Alt key pressed and dragging, they do something else like linking the file or copying it, and displaying the proper cursor. We can easily accomplish this using KeyState:
private void Form1_DragEnter(object sender, System.Windows.Forms.DragEventArgs e)
{
   // If ALT is pressed
   if((e.KeyState & 32) == 32)
   {
      // It shows a cursor with an arrow
      e.Effect = DragDropEffects.Link;
   }
   // If CTRL is pressed
   else if((e.KeyState & 8) == 8)
   {
      // It shows a cursor with a plus sign
      e.Effect = DragDropEffects.Copy;
   }
   // If SHIFT is pressed
   else if((e.KeyState & 4) == 4)
   {
      // It shows an unavailable cursor
      e.Effect = DragDropEffects.None;
   }
   // If neither one is pressed
   else
   {
      // It shows the rectangle
      e.Effect = DragDropEffects.Move;
   }
}
Ok, but there’s still something important we need to cover. How can you get the path of the file you have dropped?
Doubleclick the DragDrop event to get to the code. Here use the following:
private void Form1_DragDrop(object sender, System.Windows.Forms.DragEventArgs e)
{
   string[] files = (string[])e.Data.GetData(DataFormats.FileDrop);
   foreach(string file in files)
   {
      MessageBox.Show(file);
   }
}
The DragDrop event fires when you release the mouse button with an icon on top of a form, so exactly what we need.
Run the code now and drop a file on top of the form, the result is the path to the file displayed in a MessageBox:
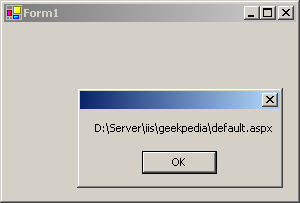
Using the path of the file you can retrieve that file’s information. And of course, the information doesn’t have to be displayed in a MessageBox, you can use a ListBox or a ListView. And something else I should point out is that you can drag and drop multiple files at once because files is an array and we are using foreach().