Silverlight, once a prominent player in the realm of Rich Internet Applications (RIAs), provided developers with a versatile platform for creating interactive and visually appealing web applications. While its prominence has waned over the years in favor of HTML5 and other technologies, Silverlight still holds historical significance and remains a valuable skill for developers working on legacy projects or exploring its capabilities.
What is Silverlight?
Silverlight, originally known as Microsoft Silverlight, was a web application framework developed by Microsoft. It made its debut in 2007, aiming to compete with Adobe Flash and Flex in the RIA space. Silverlight was designed to deliver multimedia, vector graphics, and animations over the web, providing a rich user experience that went beyond the limitations of traditional HTML-based web applications.
One of the standout features of Silverlight was its support for XAML (Extensible Application Markup Language), a declarative language used for creating user interfaces and vector graphics. This feature allowed developers to separate the design and logic of their applications, fostering a more collaborative development process.
Advantages of Silverlight for Web Application Development
While Silverlight may no longer be at the forefront of web development, it offered several advantages during its heyday:
- Rich User Interfaces: Silverlight allowed developers to create visually stunning user interfaces with animations, vector graphics, and multimedia elements. This made it ideal for applications that required a high degree of interactivity and a polished look and feel.
- Cross-Platform Support: Silverlight was compatible with various web browsers on both Windows and macOS, providing a cross-platform solution for delivering consistent experiences to users.
- Efficient Data Binding: The framework featured robust data binding capabilities, enabling developers to bind UI elements to data sources easily. This facilitated the creation of dynamic and data-driven applications.
- Security: Silverlight offered security features like sandboxing to protect users from potentially harmful content. This was particularly important for ensuring safe multimedia content delivery.
- Versatility: It supported a wide range of programming languages, including C#, VB.NET, and JavaScript, allowing developers to leverage their existing skills.
Historical Context and Evolution of Silverlight
To understand Silverlight’s journey, it’s essential to consider its historical context and evolution. When Silverlight was introduced, the web landscape was different. Flash was a dominant force, and the need for a more versatile RIA technology was evident.
Over the years, however, HTML5 emerged as a strong contender, offering native support for multimedia and interactivity. This, coupled with the decline of browser plug-ins like Flash and Silverlight, led to a shift away from proprietary RIA technologies. Microsoft announced the end of Silverlight support in 2021, marking the end of an era.
Getting Started with Silverlight
To embark on your journey into Silverlight development, you’ll first need to set up your development environment and create your very first Silverlight project. Let’s dive right in.
Setting Up the Development Environment
Before you can start building Silverlight applications, you’ll need to ensure your development environment is properly configured. Here’s what you need:
- Visual Studio: Microsoft Visual Studio is the primary integrated development environment (IDE) for Silverlight development. Ensure you have it installed. You can download the latest version from the official Microsoft website.
- Silverlight Tools for Visual Studio: Install the Silverlight Tools for Visual Studio, an add-on that provides templates, designers, and debugging support for Silverlight projects. Make sure you select the appropriate version compatible with your Visual Studio installation.
- Silverlight SDK: The Silverlight Software Development Kit (SDK) includes libraries and tools for building Silverlight applications. Download and install the SDK to access essential resources for your projects.
- Silverlight Runtime: Users accessing your Silverlight applications will need the Silverlight runtime plugin installed in their web browsers. While support for Silverlight in web browsers has waned, you may still encounter scenarios where it’s required.
Creating Your First Silverlight Project
Now that your development environment is set up, let’s create a simple Silverlight project to get a feel for how it all works. We’ll create a “Hello World” Silverlight application.
Follow these steps:
- Open Visual Studio: Launch Visual Studio and ensure that Silverlight is installed and integrated correctly.
- Create a New Project: Go to the “File” menu, select “New,” and then “Project.” In the “New Project” dialog, you’ll find a “Silverlight” category. Choose “Silverlight Application” and give your project a name, such as “HelloSilverlight.”
- Configure the Project: In the “New Silverlight Application” dialog, you can specify various project settings. You can choose the Silverlight version (if multiple versions are available) and select whether to host the Silverlight application in an HTML page.
- Design Your User Interface: Once the project is created, you’ll be presented with a XAML file (usually named MainPage.xaml) and a code-behind file (MainPage.xaml.cs). XAML is where you design your user interface, and the code-behind file is where you handle logic.
For our “Hello World” example, open MainPage.xaml and replace its contents with the following XAML code:
<UserControl x:Class="HelloSilverlight.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Width="400" Height="300">
<Grid x:Name="LayoutRoot" Background="White">
<TextBlock Text="Hello, Silverlight!" HorizontalAlignment="Center" VerticalAlignment="Center" FontSize="24"/>
</Grid>
</UserControl>
This XAML code defines a simple user interface with a centered text element that says “Hello, Silverlight!”
- Run the Application: Hit the “F5” key or click the “Start Debugging” button in Visual Studio. This will build and run your Silverlight application in a web browser.
You’ve just created and run your first Silverlight application! It’s a basic example, but it demonstrates the essential steps of setting up the development environment, creating a project, designing a user interface using XAML, and running the application.
Designing User Interfaces with XAML
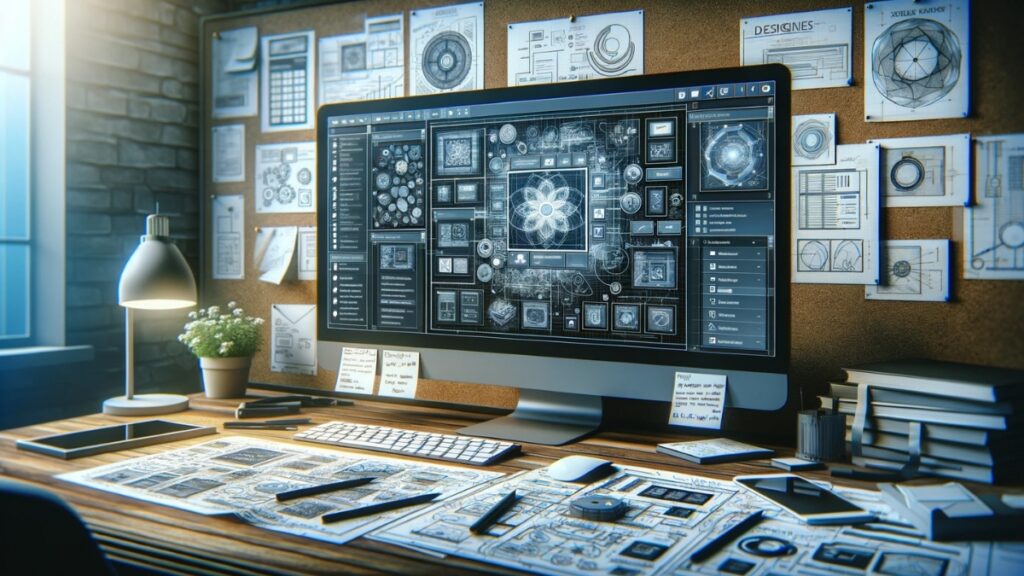
Now that you’ve taken your first steps in Silverlight development, let’s delve deeper into designing user interfaces using XAML. XAML (Extensible Application Markup Language) is at the heart of Silverlight development and is used to create the visual elements and layout of your applications.
Understanding XAML
XAML is a markup language that provides a declarative way to define the structure and appearance of your Silverlight user interfaces. It allows you to describe the user interface elements and their properties in a more human-readable format, making it easier to separate design from code.
Here’s a breakdown of key concepts in XAML:
- Elements: Elements in XAML correspond to user interface controls like buttons, textboxes, and panels. These elements are defined with XML-like syntax.
- Attributes: Attributes are used to set properties and values for the elements. For example, you can set the
Text
property of aTextBlock
to specify the displayed text. - Nesting: You can nest elements within other elements to create complex layouts. This hierarchy determines the visual structure of your application.
- Property Elements: Some properties can be set using property elements, which allows for more complex content to be defined. For instance, you can define a button’s content as a mixture of text and other controls.
Let’s explore a practical example to illustrate these concepts. We’ll create a simple Silverlight user interface with XAML.
Creating a Simple User Interface
- Open Your Silverlight Project: If you’ve followed the previous section, you should already have a Silverlight project open in Visual Studio.
- Designing a User Interface: In your project, locate the MainPage.xaml file (or the XAML file you created). We will modify this XAML to design a simple interface.
- Defining Layout: Let’s use a
StackPanel
to arrange our controls vertically. Replace the existing XAML content with the following:
<UserControl x:Class="HelloSilverlight.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Width="400" Height="300">
<Grid x:Name="LayoutRoot" Background="White">
<StackPanel>
<TextBlock Text="Welcome to Silverlight!" HorizontalAlignment="Center" FontSize="24"/>
<Button Content="Click Me" Width="100" Height="30"/>
<TextBox PlaceholderText="Enter your name"/>
</StackPanel>
</Grid>
</UserControl>
In this example, we’ve added a StackPanel
containing a TextBlock
, a Button
, and a TextBox
. The TextBlock
displays a welcome message, the Button
is labeled “Click Me,” and the TextBox
is for user input.
- Running the Application: Hit “F5” or click “Start Debugging” to run your updated Silverlight application.
You’ve now designed a simple user interface using XAML. As you can see, XAML provides an intuitive way to create and structure your Silverlight applications’ visual elements. In the next section, we’ll explore more advanced topics in Silverlight development, including data binding and working with multimedia.
Data Binding in Silverlight
Data binding is a powerful feature in Silverlight that allows you to establish connections between data sources and user interface elements. It enables dynamic updates of the user interface when underlying data changes, creating responsive and interactive applications. Let’s dive into the world of data binding in Silverlight.
Connecting Data Sources to User Interfaces
In Silverlight, data binding typically involves binding UI elements to data objects. To demonstrate this concept, we’ll create a simple example where we bind a TextBox
to a Person
object’s name property. Follow these steps:
- Create a Data Class: Start by creating a simple class to represent a person with a
Name
property. In your Silverlight project, add a new class file (e.g.,Person.cs
) and define the class as follows:
public class Person
{
public string Name { get; set; }
}
- Modify the XAML: Open your MainPage.xaml and update the XAML to include a
TextBox
that will be bound to theName
property of aPerson
object:
<UserControl x:Class="HelloSilverlight.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Width="400" Height="300">
<Grid x:Name="LayoutRoot" Background="White">
<StackPanel>
<TextBlock Text="Data Binding Example" HorizontalAlignment="Center" FontSize="24"/>
<TextBox x:Name="NameTextBox" Width="200" PlaceholderText="Enter a name"/>
<TextBlock Text="You entered:" FontSize="16"/>
<TextBlock Text="{Binding Name, ElementName=NameTextBox}" FontSize="18"/>
</StackPanel>
</Grid>
</UserControl>
Here, we’ve added a TextBox
named “NameTextBox” and two TextBlock
elements. The second TextBlock
uses data binding to display the text entered into the TextBox
.
- Setting Up Data Binding in Code-Behind: In your MainPage.xaml.cs code-behind file, create an instance of the
Person
class and set it as the data context for your user control. Add the following code to the constructor:
public MainPage()
{
InitializeComponent();
// Create a Person object and set it as the data context
Person person = new Person();
this.DataContext = person;
}
- Run the Application: Start the application, and you’ll see that as you type in the
TextBox
, the secondTextBlock
dynamically updates to display what you’ve entered. This is the power of data binding.
Two-Way Data Binding for Real-Time Updates
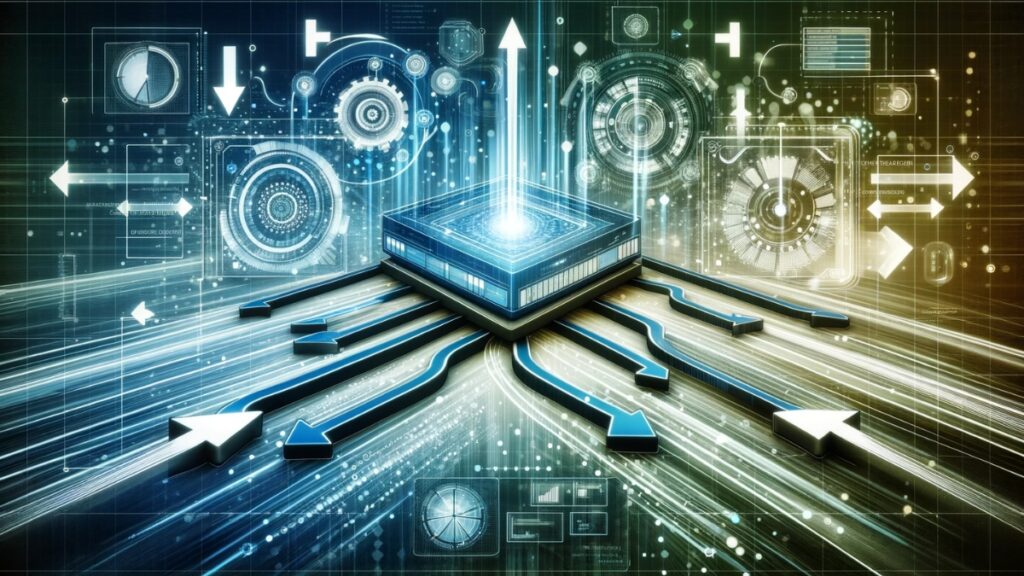
Silverlight supports two-way data binding, which means changes made in the UI can also update the underlying data source. To achieve this, you can specify the Mode
property of your data binding as “TwoWay.”
For example, to enable two-way data binding for the Name
property in our example, modify the XAML like this:
<TextBlock Text="{Binding Name, ElementName=NameTextBox, Mode=TwoWay}" FontSize="18"/>
Now, any changes made in the TextBox
will also update the Name
property of the Person
object, and vice versa.
Working with Media and Multimedia
Silverlight excels in handling multimedia elements, making it a great choice for applications that require audio, video, or animations. In this section, we’ll explore how to work with media in Silverlight and include some actual code examples.
Embedding Audio and Video Content
To include audio and video content in your Silverlight application, you can use the MediaElement
control. It allows you to play both audio and video files. Let’s create a simple example to demonstrate this:
- Prepare Media Files: Ensure you have audio and video files ready to be included in your project. Supported formats include MP3 for audio and MP4 for video.
- Modify the XAML: Open your MainPage.xaml and update it to include a
MediaElement
for both audio and video playback. Here’s a modified XAML snippet:
<UserControl x:Class="HelloSilverlight.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Width="400" Height="300">
<Grid x:Name="LayoutRoot" Background="White">
<StackPanel>
<TextBlock Text="Multimedia Example" HorizontalAlignment="Center" FontSize="24"/>
<MediaElement x:Name="audioPlayer" Source="/Assets/audio.mp3" Width="200" Height="40"/>
<MediaElement x:Name="videoPlayer" Source="/Assets/video.mp4" Width="320" Height="240" AutoPlay="False"/>
<Button Content="Play Video" Click="PlayVideo_Click"/>
</StackPanel>
</Grid>
</UserControl>
In this example, we’ve added two MediaElement
controls: one for audio and one for video. We’ve also included a “Play Video” button that will play the video.
- Adding Event Handling in Code-Behind: In your MainPage.xaml.cs code-behind file, add event handling to the “Play Video” button click event:
private void PlayVideo_Click(object sender, RoutedEventArgs e)
{
videoPlayer.Play();
}
This code instructs the videoPlayer
to start playing when the button is clicked.
- Run the Application: Start your application. When you click the “Play Video” button, the video should start playing in the designated area.
Enhancing User Engagement with Rich Media
Silverlight provides numerous opportunities to enhance user engagement with rich media. You can add animations, transitions, and even interactive elements to your multimedia content. Consider the following techniques:
- Storyboard Animations: Create animations using storyboards to add visual effects, such as fading, scaling, or rotating elements. Silverlight’s animation capabilities are versatile and can be applied to various UI elements.
- Transitions: Implement smooth transitions between different states or pages in your application. Transitions can provide a polished and professional user experience.
- Interactive Controls: Silverlight supports interactive controls like buttons, sliders, and checkboxes. These can be used to create user interfaces with user-friendly features and interactions.
- Integration with Silverlight Toolkit: The Silverlight Toolkit offers additional controls and features for creating rich Silverlight applications. It includes charting controls, date pickers, and more.
By leveraging Silverlight’s multimedia capabilities, you can create engaging and visually appealing web applications that captivate your users. The example above demonstrates the basics, but you can explore further to create complex multimedia experiences.
Deployment and Cross-Browser Compatibility
Deploying your Silverlight application and ensuring cross-browser compatibility are crucial steps in reaching a wide audience. In this section, we’ll discuss best practices for deploying Silverlight applications and making them accessible across different web browsers.
Preparing Your Silverlight Application for Deployment
Before deploying your Silverlight application, there are a few essential steps to follow:
- Build Configuration: Ensure that your project is configured for the Release build. In Visual Studio, you can select the “Release” build configuration in the Build menu.
- Optimize Resources: Minimize the size of your application by optimizing resources such as images, styles, and scripts. You can use tools like image compression and code minification to reduce file sizes.
- Cross-Origin Policy: If your Silverlight application accesses resources from different domains, make sure to configure the cross-domain policy correctly to avoid security issues.
- Error Handling: Implement error handling to gracefully handle unexpected issues that may arise during runtime. This ensures that users receive meaningful error messages.
Ensuring Compatibility Across Different Web Browsers
Silverlight applications are executed as browser plugins, so ensuring compatibility across various web browsers is essential. However, it’s important to note that Silverlight has seen a decline in support in modern web browsers. Here are some considerations:
- Browser Support: Silverlight was historically supported in Internet Explorer, Mozilla Firefox, and Google Chrome. However, modern versions of these browsers have largely dropped support for Silverlight.
- Microsoft Edge: Microsoft Edge, the successor to Internet Explorer, does not support Silverlight. Therefore, users of Microsoft Edge may need to use Internet Explorer mode or other solutions to run Silverlight applications.
- Alternative Technologies: Given the limited browser support for Silverlight, consider migrating your applications to alternative technologies like HTML5, JavaScript, and CSS, which have broader and more consistent browser support.
- Silverlight Out-of-Browser: Silverlight applications can also be run out-of-browser (OOB) on Windows platforms. OOB applications can be installed on a user’s computer and run independently of the browser, providing a more reliable deployment option.
Best Practices for Deployment
When deploying Silverlight applications, consider the following best practices:
- Provide Clear Instructions: If Silverlight is required to run your application, provide clear instructions to users on how to install or enable it in their browsers.
- Update Documentation: Keep your application’s documentation up to date, including installation and troubleshooting guides for users who may encounter issues.
- Test on Multiple Browsers: Test your Silverlight application on various browsers to identify any compatibility issues and address them accordingly.
- Stay Informed: Stay informed about the latest updates and developments regarding Silverlight, as well as the browser landscape. This will help you make informed decisions about the future of your application.
- Consider Migration: Given the declining support for Silverlight, consider migrating your application to more modern and widely supported technologies when feasible.
Deploying Silverlight applications can be challenging due to browser limitations, but by following best practices and considering alternative technologies, you can continue to provide valuable experiences to your users.
Future of Silverlight and Exploring Alternatives
As we’ve discussed, Silverlight has had its heyday but now faces a changing landscape in the world of web development. Microsoft officially announced the end of support for Silverlight in 2021, and major web browsers have reduced or entirely removed support for Silverlight plugins. In this section, we’ll delve into the future prospects of Silverlight and explore alternative technologies.
The Status of Silverlight in Modern Web Development
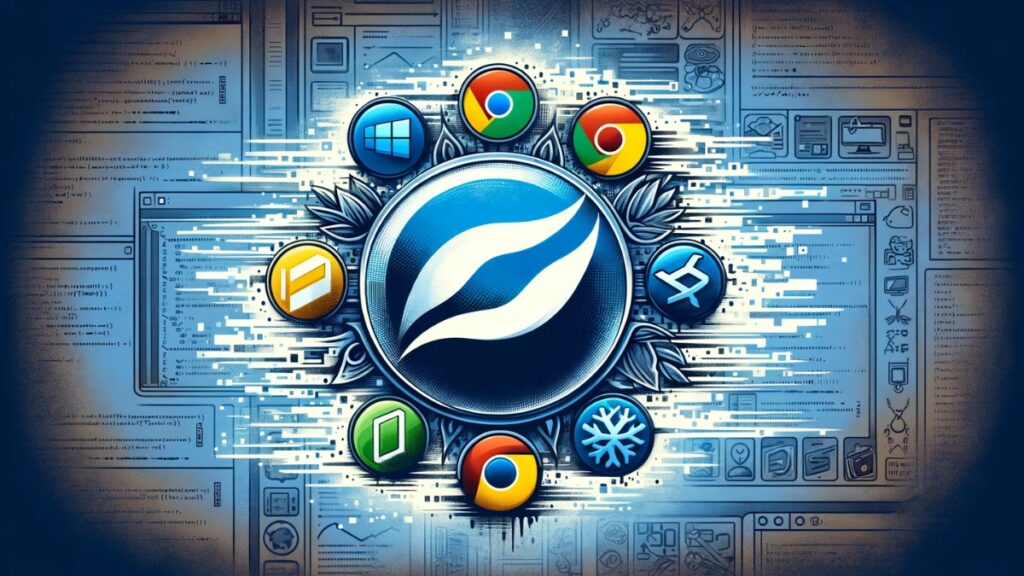
As of the knowledge cutoff date in January 2022, Silverlight is considered a deprecated technology with a diminishing presence in the web development landscape. Here are key points to consider regarding Silverlight’s status:
- End of Support: Microsoft has officially ended support for Silverlight, which means no further updates, security patches, or bug fixes will be provided.
- Browser Support: Most modern web browsers, including Microsoft Edge, Google Chrome, Mozilla Firefox, and Apple Safari, have either removed support for Silverlight or have deprecated support in favor of modern web standards.
- Migration Challenges: For organizations with existing Silverlight applications, migrating to alternative technologies can be complex and resource-intensive.
Exploring Alternatives for Building Rich Internet Applications
Given the limited future prospects of Silverlight, it’s essential to consider alternative technologies for building rich internet applications (RIAs). Here are some popular alternatives to Silverlight:
- HTML5, CSS3, and JavaScript: HTML5 provides a rich set of features for creating interactive web applications. Combined with CSS3 for styling and JavaScript for dynamic behavior, this trio offers a powerful and widely supported approach to RIA development.
- React, Angular, or Vue.js: These JavaScript frameworks offer robust tools for building modern, responsive, and highly interactive web applications. They are widely adopted in the industry and come with large developer communities.
- Blazor: Developed by Microsoft, Blazor is a web framework that allows you to build interactive web applications using C# and .NET instead of JavaScript. It offers a more familiar development experience for .NET developers.
- Progressive Web Apps (PWAs): PWAs combine web technologies to provide a more app-like experience in browsers. They are responsive, installable, and can work offline, making them suitable for creating rich web applications.
- WebAssembly (Wasm): WebAssembly is a binary instruction format that enables near-native performance in web browsers. You can use languages like C, C++, and Rust to compile code to Wasm and run it in web applications.
- Flutter: While primarily known for mobile app development, Flutter is increasingly being used for web application development. It allows you to build web apps using a single codebase.
Transitioning from Silverlight to Other Technologies
Transitioning from Silverlight to alternative technologies requires careful planning and consideration. Here are key steps to follow:
- Assessment: Evaluate your existing Silverlight applications to understand their functionalities, dependencies, and user requirements.
- Select an Alternative: Choose the most suitable alternative technology or framework based on your application’s needs, development team’s expertise, and long-term goals.
- Migration Plan: Develop a detailed migration plan that outlines the steps, timeline, and resources required to move from Silverlight to the chosen technology.
- Recreation vs. Migration: Decide whether to recreate the application from scratch or migrate existing code and assets. This choice may depend on the complexity of the application and the extent of changes needed.
- Testing and Validation: Thoroughly test the migrated or recreated application to ensure it meets functionality, performance, and compatibility requirements.
- User Training and Communication: Prepare users for the transition by providing training and clear communication regarding the change.
- Long-Term Maintenance: Plan for ongoing maintenance and updates to ensure the continued reliability and relevance of the application.
Conclusion
In conclusion, this comprehensive guide has illuminated the world of Silverlight development, showcasing its historical significance and its capabilities for building Rich Internet Applications (RIAs). We embarked on a journey through Silverlight’s fundamentals, learning how to set up a development environment, design rich user interfaces with XAML, establish dynamic data binding, incorporate multimedia elements, enhance interactivity, and address deployment challenges. Throughout this exploration, we’ve provided actual Silverlight code examples to facilitate understanding.
However, it’s imperative to acknowledge the shifting tides of web technology. As of our knowledge cutoff date in January 2022, Silverlight has reached the end of its life, with waning support from major web browsers. To remain relevant in the ever-evolving web development landscape, developers are encouraged to explore alternative technologies such as HTML5, JavaScript, and modern web frameworks. While Silverlight has left a lasting mark on web development history, embracing newer tools and paradigms is essential to meet the demands of today’s digital landscape.