“PHP, standing for ‘Hypertext Preprocessor,’ has been a pivotal force in web development since its creation in 1995 by Rasmus Lerdorf. Initially designed as a simple set of CGI binaries for personal use, PHP rapidly evolved into a comprehensive server-side scripting language, integral to PHP Dynamic Website Development. Its journey from PHP/FI, a basic form interpreter, to the highly efficient PHP 7, showcases its remarkable transformation. Today, PHP is renowned for its ease of use, with syntax that integrates seamlessly into HTML, making it a go-to choice for both beginners and seasoned developers in dynamic website creation. Its open-source nature, coupled with extensive community support, has led to a rich ecosystem of frameworks and tools, further cementing its position as a cornerstone in the development of dynamic websites.”
PHP’s versatility is one of its standout features, allowing it to operate across various platforms and support a wide array of databases. This flexibility, combined with its compatibility with major servers, makes PHP a universally adaptable language. The language’s continuous enhancements in performance and functionality have kept it relevant in the fast-paced world of web technology. From small personal blogs to large-scale commercial websites, PHP’s scalability and robust set of built-in functions have made it an indispensable tool in creating interactive and efficient web applications.
Understanding the Basics of PHP for Dynamic Content
PHP’s fundamental role in web development is to generate dynamic content on web pages. This is achieved by embedding PHP code within HTML, allowing for a seamless blend of server-side scripting with front-end design. The beauty of PHP lies in its simplicity and efficiency in executing various web-related tasks.

PHP Syntax Essentials
At its core, PHP syntax is intuitive and user-friendly. A PHP script starts with <?php
and ends with ?>
. Within these tags, you can write your PHP code, which the server executes to generate dynamic content. Here’s a basic example:
<?php
echo "Hello, world!";
?>
This script outputs “Hello, world!” on the web page. The echo
statement is one of the most commonly used constructs in PHP, employed for displaying output.
Integrating PHP with HTML and CSS
One of PHP’s strengths is its ability to integrate seamlessly with HTML. You can insert PHP scripts anywhere in an HTML document. This integration allows for dynamic content generation, such as displaying user data, time-sensitive messages, or interactive forms. Here’s a simple example:
<!DOCTYPE html>
<html>
<head>
<title>My PHP Test</title>
</head>
<body>
<h1><?php echo "Welcome to My Website!"; ?></h1>
</body>
</html>
In this example, PHP is used within an HTML <h1>
tag to dynamically set the page’s heading. This integration extends to CSS as well, where PHP can dynamically set styles. For instance:
<style>
body {
background-color: <?php echo $backgroundColor; ?>;
}
}
</style>
Here, PHP sets the background color of the page based on a variable $backgroundColor
. This level of integration showcases PHP’s flexibility in web design, allowing developers to create highly responsive and personalized user experiences.
PHP’s simplicity in syntax and its powerful integration with HTML and CSS make it an ideal choice for developers looking to create dynamic web content. Whether it’s a simple blog or a complex e-commerce site, PHP provides the tools necessary to build engaging and interactive web experiences. As we progress further into PHP’s capabilities, its role in managing database interactions, user sessions, and advanced web functionalities becomes increasingly evident.
Database Interactions with PHP
A critical aspect of dynamic website development is the ability to interact with databases. PHP excels in this area, particularly with its native support for MySQL, a popular database management system. This synergy between PHP and MySQL enables the creation of robust and interactive web applications.
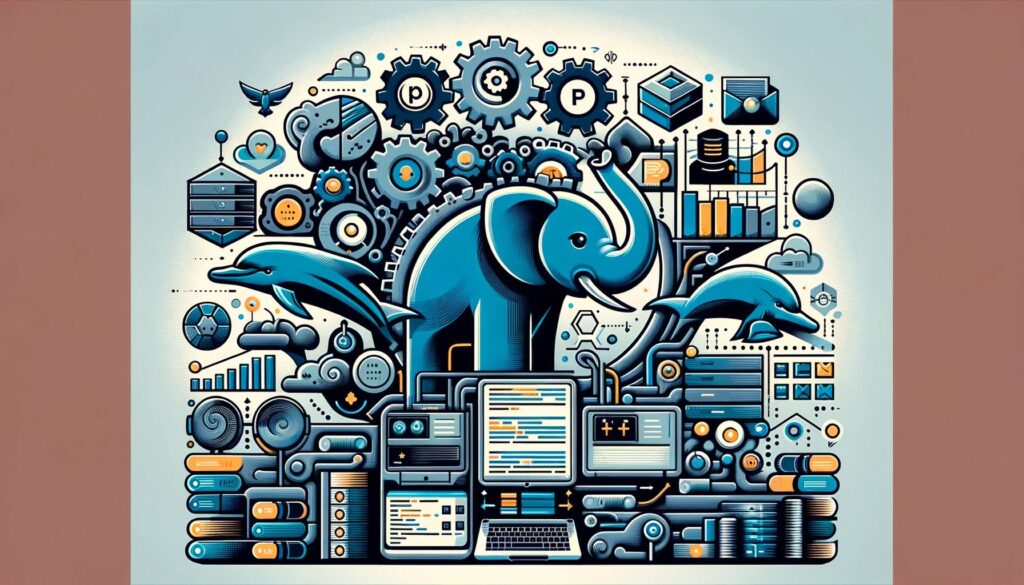
Connecting PHP to MySQL
The first step in database interaction is establishing a connection between PHP and the MySQL database. PHP provides functions like mysqli_connect()
for this purpose. Here’s a basic example of how to connect to a MySQL database using PHP:
<?php
$servername = "localhost";
$username = "username";
$password = "password";
$dbname = "myDatabase";
// Create connection
$conn = mysqli_connect($servername, $username, $password, $dbname);
// Check connection
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
echo "Connected successfully";
?>
In this script, mysqli_connect()
is used to connect to a MySQL database. The function takes parameters such as server name, username, password, and database name.
CRUD Operations in PHP
CRUD (Create, Read, Update, Delete) operations are fundamental in database management. PHP provides straightforward methods to execute these operations.
1. Create (Insert): To add new data to a database table.
$sql = "INSERT INTO MyTable (firstname, lastname) VALUES ('John', 'Doe')";
if (mysqli_query($conn, $sql)) {
echo "New record created successfully";
} else {
echo "Error: " . $sql . "<br>" . mysqli_error($conn);
}
2. Read (Select): To retrieve data from a database.
$sql = "SELECT id, firstname, lastname FROM MyTable";
$result = mysqli_query($conn, $sql);
if (mysqli_num_rows($result) > 0) {
while($row = mysqli_fetch_assoc($result)) {
echo "id: " . $row["id"]. " - Name: " . $row["firstname"]. " " . $row["lastname"]. "<br>";
}
} else {
echo "0 results";
}
3. Update: To modify existing data in a database.
$sql = "UPDATE MyTable SET lastname='Smith' WHERE id=1";
if (mysqli_query($conn, $sql)) {
echo "Record updated successfully";
} else {
echo "Error updating record: " . mysqli_error($conn);
}
4. Delete: To remove data from a database.
$sql = "UPDATE MyTable SET lastname='Smith' WHERE id=1";
if (mysqli_query($conn, $sql)) {
echo "Record updated successfully";
} else {
echo "Error updating record: " . mysqli_error($conn);
}
Each of these operations plays a vital role in managing the data of a website, whether it’s adding new user information, updating existing details, retrieving data for display, or removing outdated information. PHP’s built-in functions and its compatibility with MySQL make these tasks efficient and straightforward, allowing developers to focus on creating more complex and interactive web functionalities.
User Authentication and Session Management
User authentication and session management are key components in creating secure and personalized user experiences on dynamic websites. PHP provides robust tools for handling these aspects, ensuring both security and convenience for users.
Creating Secure Login Systems
A secure login system is the first line of defense in protecting user data and personalizing content. PHP handles user authentication by verifying user credentials (usually a username and password) against a database. Here’s a simplified overview of the process:
1. User Registration: Store user credentials securely in the database. It’s crucial to hash passwords using functions like password_hash()
for security.
$hashed_password = password_hash($password, PASSWORD_DEFAULT);
// Store $hashed_password in the database
2. User Login: When a user logs in, PHP retrieves the hashed password from the database and uses password_verify()
to compare it with the entered password.
if (password_verify($entered_password, $hashed_password_from_database)) {
// Password is correct, allow login
} else {
// Password is incorrect, deny access
}
3. Session Creation: Upon successful login, PHP starts a session to track user activity.
session_start();
$_SESSION['user_id'] = $user_id_from_database;
Managing User Sessions for Personalized Experiences
Sessions in PHP are a way to store information about a user’s interaction with the site. This information can be used to personalize the user experience. PHP uses a unique session ID for each user, ensuring that session data is kept separate and secure.
1. Starting a Session: A session is started with session_start()
. This function must be called before any output is sent to the browser.
session_start();
2. Storing Session Data: You can store and access session data using the global $_SESSION
array.
$_SESSION['username'] = 'JohnDoe';
3. Accessing Session Data: Retrieve data from the session as needed to personalize the user experience.
echo "Welcome, " . $_SESSION['username'];
4. Ending a Session: It’s important to manage sessions properly, especially for logging out users. Use session_destroy()
to end a session.
session_start();
// Destroy all session data
session_destroy();
User authentication and session management in PHP are essential for creating dynamic, secure, and user-centric web applications. By securely handling user credentials and effectively managing session data, PHP enables developers to build websites that are not only secure but also highly personalized, enhancing the overall user experience.
Advanced PHP Techniques for Dynamic Pages
As developers progress with PHP, they often explore advanced techniques to enhance user experience and website functionality. Two significant areas where PHP shows its strength are in its use with AJAX for creating seamless user experiences and the utilization of PHP frameworks for efficient development.
AJAX and PHP for Seamless User Experiences
AJAX (Asynchronous JavaScript and XML) combined with PHP is a powerful duo for creating dynamic, responsive web pages that don’t require page reloads for content updates. This combination is particularly useful in forms, search suggestions, and real-time data displays.
- How AJAX Works with PHP:
- A user action triggers an AJAX call on the client side.
- AJAX sends a request to a PHP script on the server without reloading the page.
- The PHP script processes the request, interacts with the database if needed, and sends back a response.
- The response is then handled by JavaScript to update the webpage content dynamically.
- Example Use Case: Live Search
- A user types in a search box.
- Each keystroke triggers an AJAX call that sends the input to a PHP script.
- The PHP script searches the database for matching entries and returns the results.
- The search results are dynamically displayed below the search box without reloading the page.
PHP Frameworks for Efficient Development
Frameworks in PHP provide structured, reusable code, helping developers build web applications faster and more efficiently. They offer tools and libraries for common tasks, reducing the need for repetitive coding.
- Popular PHP Frameworks:
- Laravel: Known for its elegant syntax, Laravel is great for tasks like authentication, routing, sessions, and caching.
- Symfony: Ideal for complex, large-scale enterprise projects, Symfony is known for its components that can be used in any PHP project.
- CodeIgniter: With a small footprint, CodeIgniter is a powerful framework for developers who need a simple and elegant toolkit to create full-featured web applications.
- Benefits of Using PHP Frameworks:
- Rapid Development: Frameworks come with built-in libraries and tools, speeding up the development process.
- Better Code Organization: MVC (Model-View-Controller) architecture in frameworks helps in organizing code better.
- Enhanced Security: Frameworks often have built-in protections against common security threats.
- Community Support: Popular frameworks have large communities, offering extensive resources and support.
By leveraging AJAX for real-time interactivity and utilizing PHP frameworks for structured and rapid development, PHP developers can significantly enhance the functionality and user experience of web applications. These advanced techniques not only streamline the development process but also open up new possibilities for creating sophisticated and interactive web applications.
Optimizing PHP for Performance and Security
Optimizing a PHP application is crucial for enhancing performance and ensuring security. By implementing best practices, developers can significantly improve the efficiency and safety of their PHP-based websites.
Best Practices for Secure PHP Coding
Security in PHP is paramount, especially when dealing with user data and authentication. Here are key practices to enhance security:
- Data Validation and Sanitization: Always validate and sanitize user input to prevent SQL injection and other forms of attacks. Use built-in PHP functions like
filter_var()
for sanitization andpreg_match()
for validation. - Use Prepared Statements for Database Queries: Using PDO (PHP Data Objects) or MySQLi, prepared statements ensure the safe execution of SQL queries, thereby preventing SQL injection attacks.
- Error Handling: Proper error handling prevents sensitive information from being exposed. Use custom error handling methods instead of relying on PHP’s default error reporting.
- Regular Updates: Keep PHP and its libraries up to date. Updates often include security patches for vulnerabilities.
Tips for Improving PHP Performance
Performance optimization is essential for a smooth user experience and efficient server resource usage. Here are effective strategies:
- Use Opcode Cache: Opcode caching like OPcache can significantly speed up PHP performance by storing precompiled script bytecode in shared memory, reducing the need for PHP to load and parse scripts on each request.
- Optimize Database Interactions: Efficient database queries and indexing can reduce the load time. Also, consider using persistent database connections to minimize connection overhead.
- Minimize Use of External Resources: Reduce the number of external scripts and libraries. Ensure to optimize them and load asynchronously if necessary, to prevent render-blocking.
- Content Caching: Implement caching strategies for static content to reduce server load and improve response time.
- Efficient Use of Loops and Algorithms: Optimize loops and algorithms to reduce execution time. Avoid unnecessary computations inside loops.
- Profile and Monitor Application Performance: Tools like Xdebug for profiling and monitoring can help identify bottlenecks in the application.
Conclusion
PHP firmly establishes itself as a versatile and powerful language in web development. Its evolution from a simple scripting tool to a robust platform for dynamic websites demonstrates continuous adaptability. The language’s ease of use, along with powerful features for database interaction, user authentication, and session management, makes it ideal for various applications. Advanced techniques involving AJAX and PHP frameworks enhance its capabilities, enabling the creation of sophisticated, interactive web applications.
Optimization strategies for performance and security ensure that PHP applications are not only functional but also efficient and secure. Real-world examples like WordPress, Facebook, and Magento showcase PHP’s scalability and flexibility, proving its effectiveness in handling everything from small personal blogs to large-scale commercial sites. With ongoing development and strong community support, PHP’s future remains bright, maintaining its relevance in the ever-evolving landscape of web technology.