ASP.NET Core is a cross-platform, open-source framework for building modern web applications. It offers high performance, flexibility, and a robust set of tools for web development, making it a popular choice among developers.
Overview of ASP.NET Core
ASP.NET Core marks a pivotal advancement in Microsoft’s web development platforms, embracing open-source and cross-platform capabilities. Tailored for contemporary, cloud-native, and internet-connected apps, it prioritizes high performance and scalability. Key benefits include enhanced runtime efficiency, a modular architecture, and a unified approach for web UI and APIs. Its cross-platform compatibility empowers developers to create applications that effortlessly operate on Windows, Linux, and macOS, bridging gaps in the modern web application landscape.
Why ASP.NET Core for Scalable Applications
- Cross-Platform Support: ASP.NET Core’s cross-platform compatibility enables scalable applications across various environments, including Docker containers for microservices.
- High Performance: ASP.NET Core excels in benchmarks, ensuring efficient resource utilization and the ability to handle more requests.
- Modular Architecture: Its modularity streamlines application development by reducing overhead and potential points of failure.
- Development Environment: ASP.NET Core integrates with tools like Visual Studio and Visual Studio Code, simplifying complex application building and scaling.
- Community Support: An active open-source community provides updates, security patches, and performance enhancements.
- Advanced Features: Features like async programming and dependency injection aid in scalable, maintainable app development.
- Robust Security: ASP.NET Core includes built-in security, crucial for secure and scalable applications.
Understanding ASP.NET Core Performance
Understanding ASP.NET Core performance is vital for developing fast and efficient web applications. It involves optimizing code, minimizing resource consumption, and leveraging features like asynchronous programming and caching.
Importance of Performance in Scalability
In ASP.NET Core, performance is integral, not an afterthought. The framework prioritizes responsiveness under increased loads, impacting user experience and resource efficiency. It achieves this through optimized runtime, efficient memory management, and asynchronous processing. This allows applications to handle more requests and concurrent users with the same hardware resources, ensuring scalability and a seamless user experience.
Best Practices for Optimal Performance
1. Asynchronous Programming: ASP.NET Core’s support for asynchronous programming allows for non-blocking I/O operations. This approach is crucial for scalability as it helps in efficiently utilizing server resources. Instead of tying up threads waiting for I/O operations to complete, asynchronous methods free up these threads to handle other requests.
Example: Consider an operation to read from a database. Using synchronous methods, a thread would be blocked until the database operation is complete. With asynchronous programming, the thread can be released back to the thread pool to serve other requests while the database operation is ongoing.
public async Task<IActionResult> GetDataAsync()
{
var data = await _databaseService.ReadDataAsync();
return View(data);
}
2.Efficient Memory Management: Avoiding large object heap (LOH) allocations is vital. Objects over 85,000 bytes are allocated on the LOH in .NET, leading to costly garbage collections. ASP.NET Core encourages practices to minimize LOH allocations, like using ArrayPool for large arrays.
3.Minimize Resource Intensive Operations: Heavy computations or long-running operations should be minimized or offloaded. If necessary, these operations can be performed in the background or on separate services to ensure the main application remains responsive.
4.Implement Caching: Implementing caching strategies is crucial. Storing frequently accessed data in memory or distributed caches can significantly reduce database load and improve response times.
5.Optimize Data Access: Optimize database interactions by using efficient queries and minimizing data transfer. Entity Framework Core, for example, should be used judiciously with well-optimized LINQ queries to avoid unnecessary data loading.
6.Utilize Response Compression: Response compression reduces the size of data transmitted over the network. ASP.NET Core supports response compression middleware which can be easily added to the application pipeline.
7.Monitor and Profile Application Performance: Regular monitoring and profiling help in identifying bottlenecks. Tools like Application Insights and profiling in Visual Studio can provide insights into performance issues.
8.Leverage HTTP/2 Features: HTTP/2 offers several performance benefits over HTTP/1.1, including header compression and multiplexing. ASP.NET Core supports HTTP/2, and leveraging these features can lead to performance improvements.
Implementing Effective Caching Strategies
Caching is a powerful technique in ASP.NET Core that significantly enhances the scalability and performance of web applications. It involves storing copies of frequently accessed data in a fast-access storage layer, which reduces the need to repeatedly fetch this data from slower storage layers, like databases or file systems.
Types of Caching in ASP.NET Core
1. In-Memory Caching: This involves storing data in the memory of the web server. It’s fast and ideal for data that doesn’t change often and is shared across multiple requests or users.
Example: Storing a list of categories in an e-commerce application that doesn’t change frequently.
public IActionResult Index()
{
var categories = _memoryCache.GetOrCreate("categories", entry =>
{
entry.SlidingExpiration = TimeSpan.FromMinutes(30);
return _repository.GetCategories();
});
return View(categories);
}
2. Distributed Caching: For applications running on multiple servers, distributed caching helps in synchronizing the cache across all servers. Redis and SQL Server are popular choices for distributed caching in ASP.NET Core.
3. Response Caching: This involves caching the response of HTTP requests. It can be used to store the rendered output of pages, thus reducing the need for regeneration of content for each request.
4. Data Caching: This is similar to in-memory caching but more specific to data retrieved from databases or external services. It is commonly used for caching query results.
5. Cache Dependencies: ASP.NET Core allows setting up dependencies in the cache, so the cached data is invalidated automatically when the dependent data changes.
How Caching Improves Scalability
- Reduced Database Load: By caching data, the frequency of database queries decreases, reducing the load on the database server.
- Improved Response Time: Cached data can be served much faster than data retrieved from a database or an external service, improving the application’s response time.
- Better Resource Utilization: Caching reduces the amount of processing and memory use on the server, freeing up resources for handling more user requests.
Best Practices for Implementing Caching
- Cache Appropriate Data: Not all data is suitable for caching. Data that is frequently accessed and does not change often is an ideal candidate for caching.
- Manage Cache Lifetime: Set appropriate expiration times for cache items. This ensures that the cache is updated regularly and doesn’t serve stale data.
- Use Distributed Caching for High Availability: In a load-balanced environment, use distributed caching to ensure consistency across different instances of the application.
- Monitor Cache Effectiveness: Regularly monitor the cache hit and miss rates to adjust caching strategies for optimal performance.
Implementing caching in ASP.NET Core applications is a balance between memory use and data freshness. Done correctly, it can significantly boost the performance and scalability of web applications, providing a better user experience and more efficient resource utilization.
Load Balancing and Asynchronous Programming
Load balancing and asynchronous programming are pivotal in building scalable ASP.NET Core applications. These techniques ensure that the application can handle a high number of simultaneous requests without compromising performance.
Benefits of Load Balancing
Load balancing is vital in distributing network traffic across multiple servers, preventing bottlenecks, and enhancing application availability and reliability. In microservices architecture, where components run on separate servers, load balancing is especially crucial. ASP.NET Core supports load balancing at different levels, from basic round-robin DNS methods to advanced hardware or cloud-based solutions with features like SSL termination and session persistence, providing flexibility in optimizing application performance and scalability.
Asynchronous Programming in ASP.NET Core
Asynchronous programming in ASP.NET Core allows applications to initiate lengthy tasks and continue processing other tasks without waiting for the initial one to finish. This is essential for maintaining a responsive user interface in web applications. ASP.NET Core streamlines asynchronous programming using the async and await keywords in C#, facilitating code that is both straightforward to write and maintain while capitalizing on the performance gains of non-blocking I/O operations.
Example of Asynchronous Programming in ASP.NET Core
Here’s a basic example demonstrating an asynchronous action method in an ASP.NET Core MVC controller. This method asynchronously fetches data from a database using Entity Framework Core.
public class HomeController : Controller
{
private readonly MyDbContext _context;
public HomeController(MyDbContext context)
{
_context = context;
}
public async Task<IActionResult> Index()
{
var products = await _context.Products.ToListAsync();
return View(products);
}
}
In this example, ‘ToListAsync()‘ is an asynchronous method provided by Entity Framework Core. It fetches data from the ‘Products‘ table without blocking the main thread. The use of ‘await‘ ensures that the method’s execution pauses until the data is fetched, without blocking the thread.
Implementing Load Balancing in ASP.NET Core
While ASP.NET Core doesn’t directly manage load balancing, it is designed to work efficiently in load-balanced environments. When deploying an ASP.NET Core application in a load-balanced setup, consider the following:
- Session State: If your application uses session state, configure it to use a distributed cache. This ensures that session data is available across all instances of the application.
- Sticky Sessions: Some load balancers support sticky sessions, which can be useful if you need to maintain user context on a specific server.
- Health Checks: Implement health checks to allow the load balancer to monitor the health of your application instances.
Database Optimization for Scalability
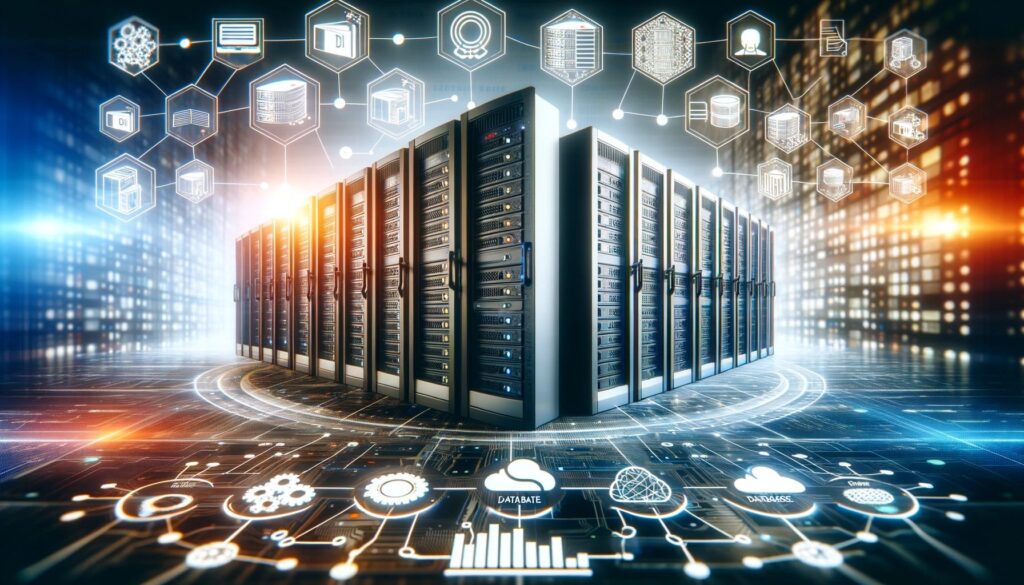
Optimizing database performance is critical in scaling ASP.NET Core applications. As the volume of data and number of users increase, efficient database interactions become crucial to maintain application responsiveness and prevent bottlenecks.
Scaling Database Performance
Database scaling in ASP.NET Core applications involves managing increased loads through vertical scaling (upgrading hardware) or horizontal scaling (adding servers). Entity Framework Core, commonly used for database interactions, plays a crucial role. Optimizing Entity Framework Core queries and adopting efficient database design principles can yield substantial performance enhancements, ensuring effective handling of growing workloads in your application.
Example of Optimizing Database Queries with Entity Framework Core
Consider a scenario where you need to retrieve a specific set of data from a large table. Instead of fetching the entire dataset, you can optimize the query to retrieve only the required data.
public async Task<IActionResult> GetProductsAsync()
{
var products = await _context.Products
.Where(p => p.Category == "Electronics")
.ToListAsync();
return View(products);
}
In this example, the .Where clause filters the products to only include those in the “Electronics” category, reducing the amount of data retrieved and processed.
Database Sharding and Replication Techniques
- Database Sharding: This involves dividing a database into smaller, more manageable pieces, called shards. Each shard contains a subset of the total data and can be hosted on separate servers. Sharding is effective for horizontal scaling and can improve performance by distributing the load across multiple servers.
- Database Replication: Replication involves creating copies of a database on different servers. Read replicas can be used to distribute read queries across multiple servers, thus reducing the load on the primary database server.
Best Practices for Database Optimization
- Indexing: Vital for query performance; create indexes on frequently used columns.
- N+1 Queries: Beware of Entity Framework Core’s N+1 query issue; retrieve data efficiently in a single query.
- Connection Pooling: Efficiently manage DB connections with connection pooling to avoid open/close overhead.
- Monitoring & Logging: Use tools like SQL Server Profiler to monitor and log DB performance, identifying bottlenecks.
Utilizing Azure for ASP.NET Core Apps
Azure provides a comprehensive set of services that can significantly enhance the scalability and performance of ASP.NET Core applications. Utilizing Azure services like Azure App Service, Azure SQL Database, and Azure Storage can streamline the process of deploying, managing, and scaling applications.
Creating and Managing Azure Services
Azure App Service is a fully managed platform for building, deploying, and scaling web apps. It offers auto-scaling, high availability, and supports both Windows and Linux-based environments. Additionally, Azure SQL Database, a fully managed relational cloud database service, provides built-in features like automatic scaling, backups, and high availability.
Example: Deploying an ASP.NET Core Application to Azure App Service
Deploying an ASP.NET Core application to Azure App Service involves several steps:
- Create an Azure App Service Plan: This defines the region, features, cost, and compute resources of your web app.
- Publish the ASP.NET Core Application: Use Visual Studio or the Azure CLI to deploy your application to the created App Service.
In Visual Studio, right-click on the project and select “Publish”. Choose “Azure” as the target, select the existing App Service, and follow the prompts to deploy. - Configure Application Settings: Set application-specific settings in the Azure portal, like connection strings and application keys.
Connecting Azure Services for Enhanced Scalability
- Azure SQL Database: Configure your ASP.NET Core application to use Azure SQL Database for scalable and secure data storage. Utilize features like Geo-Replication for high availability.
- Azure Blob Storage: For storing large amounts of unstructured data, like images and videos, use Azure Blob Storage. This integrates seamlessly with ASP.NET Core applications.
- Azure Redis Cache: Implement Azure Redis Cache for distributed caching, which is essential for high-performance and scalable ASP.NET Core applications.
Security Role Assignments and Configuration
Azure offers various security features to protect your application. Utilize Azure Active Directory for authentication, implement role-based access control (RBAC) for managing access to resources, and use Azure Key Vault to securely store application secrets.
Monitoring and Diagnostics
Utilize Azure Monitor and Application Insights for monitoring the performance and diagnosing issues in your ASP.NET Core application. These tools provide valuable insights into your application’s operations, helping you make informed decisions for scaling and performance tuning.
Tools and Frameworks for ASP.NET Development
ASP.NET Core development is enhanced by a variety of tools and frameworks that streamline the building of scalable applications. These tools assist in various aspects of development, from coding and debugging to deployment and monitoring.
Overview of Essential ASP.NET Tools
- Visual Studio: A feature-rich IDE for ASP.NET Core with advanced debugging and Git support.
- Entity Framework Core: Simplifies DB access by mapping it to C# objects.
- Bootstrap: Front-end framework for responsive web app UI development.
- jQuery: Versatile JavaScript library for simplified client-side scripting.
- Angular, React, Vue.js: Front-end frameworks for dynamic SPAs integrated with ASP.NET Core.
How These Tools Contribute to Scalability
- Visual Studio: Its powerful debugging and performance profiling tools help identify and fix performance bottlenecks, which is crucial for scaling applications.
- Entity Framework Core: Efficiently handles data access, allowing for scalable database operations, especially when combined with LINQ for optimized querying.
- Bootstrap, jQuery, Angular, React, and Vue.js: These tools/frameworks facilitate the development of dynamic and responsive user interfaces that scale well across different devices and screen sizes.
Example: Integrating Bootstrap with ASP.NET Core
Integrating Bootstrap into an ASP.NET Core project enhances UI scalability and responsiveness. Here’s a basic example of how to include Bootstrap in an ASP.NET Core MVC view.
1.Install Bootstrap: You can add Bootstrap to your project via npm, Bower, or simply by including it in your HTML file.
<!-- Include Bootstrap CSS -->
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
2. Use Bootstrap Classes in Razor View: Utilize Bootstrap’s CSS classes in your Razor views to create responsive layouts.
<div class="container">
<div class="row">
<div class="col-md-12">
<h2>Welcome to ASP.NET Core with Bootstrap</h2>
</div>
</div>
</div>
Security and Maintenance Best Practices
Ensuring robust security and implementing effective maintenance practices are crucial for the long-term scalability and reliability of ASP.NET Core applications.
Ensuring Application Security
ASP.NET Core offers various built-in security features that are essential for protecting applications from common vulnerabilities:
1. Authentication and Authorization: Implementing authentication and authorization ensures that only authenticated and authorized users can access specific resources. ASP.NET Core provides various options like cookie-based authentication, JWT (JSON Web Tokens), and external providers like OAuth.
Example: Setting up JWT Authentication in ASP.NET Core.
services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options =>
{
options.TokenValidationParameters = new TokenValidationParameters
{
ValidateIssuer = true,
ValidateAudience = true,
ValidateLifetime = true,
ValidateIssuerSigningKey = true,
ValidIssuer = Configuration["Jwt:Issuer"],
ValidAudience = Configuration["Jwt:Audience"],
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(Configuration["Jwt:Key"]))
};
});
2. Data Protection: Use ASP.NET Core’s data protection APIs to protect data such as passwords and personal information. This includes encryption of sensitive data and secure storage of keys.
3. Cross-Site Scripting (XSS) Protection: ASP.NET Core provides features to prevent XSS attacks by encoding text output and offering features like Content Security Policy (CSP) headers.
4. Cross-Site Request Forgery (CSRF) Protection: Use anti-forgery tokens in forms to prevent CSRF attacks. ASP.NET Core includes easy-to-use anti-forgery support.
Ongoing Maintenance for Scalable ASP.NET Core Apps
1. Regular Updates: Keep the .NET Core framework and all dependencies up to date. This ensures that the application uses the latest features and security fixes.
2. Logging and Monitoring: Implement comprehensive logging and use monitoring tools like Application Insights to track the application’s health and performance. This helps in early detection of issues and facilitates troubleshooting.
Example: Setting up Application Insights.
services.AddApplicationInsightsTelemetry(Configuration["ApplicationInsights:InstrumentationKey"]);
3. Performance Optimization: Regularly analyze and optimize the performance of the application. This includes profiling the application, optimizing code, and reducing resource consumption.
4. Database Maintenance: Regularly update database schemas, optimize queries, and perform database indexing to ensure efficient data access.
5. Automated Testing and CI/CD: Implement automated tests and continuous integration/continuous deployment (CI/CD) pipelines to ensure code quality and streamline deployment processes.
6. Disaster Recovery Planning: Implement backup and recovery strategies to handle potential data loss scenarios. This is critical for maintaining data integrity and availability.
Conclusion
This article has provided a comprehensive guide to building scalable applications using ASP.NET Core, highlighting the importance of understanding performance best practices, leveraging effective caching strategies, utilizing load balancing and asynchronous programming, optimizing database performance, integrating Azure services, and harnessing key development tools and frameworks. Implementing these strategies ensures that ASP.NET Core applications are not only high-performing but also scalable and secure, ready to meet the evolving demands of modern web development.