Rust, a modern programming language, is gaining popularity due to its emphasis on safety, performance, and concurrency. It’s favored for its ability to write fast, reliable code without common errors. With a growing community and industry support, Rust’s popularity is on the rise.
The Emergence of a Multi-Paradigm Language
Rust, a multi-paradigm programming language, is rapidly gaining acclaim in the tech world for its unique blend of safety, speed, and concurrency. Born out of Mozilla Research, Rust has evolved to offer a compelling mix of high-level language features with the performance of a low-level language. It’s renowned for its focus on memory safety without sacrificing performance, a balance not often found in programming languages.
Adoption by Tech Giants
The language’s robustness and reliability have attracted major tech players. Google, for instance, has incorporated Rust into significant projects, recognizing its potential to enhance performance and security. Similarly, Mozilla’s Servo web engine, a notable project, leverages Rust’s capabilities to achieve impressive speeds and reliability. The integration of Rust into parts of the Linux kernel further solidifies its position as a language of choice for system-level programming.
Rust’s Unique Features
What sets Rust apart are its core features:
- Memory Safety: Rust is designed to be memory-safe. The compiler plays a crucial role in this, refusing to compile code that breaches safety guarantees. This feature significantly reduces common programming errors, such as buffer overflows and null pointer dereferencing.
- Performance: Rust offers performance comparable to C and C++, making it ideal for system-level and performance-critical applications.
- Concurrency: Rust’s ownership and borrowing concepts provide a unique approach to managing concurrency, offering safer concurrent programming without the fear of data races.
- Zero-Cost Abstractions: Rust’s abstractions are designed to impose no additional runtime overhead. This means that high-level abstractions compile to low-level code as efficient as hand-written equivalents.
Rust’s Growing Ecosystem
The Rust ecosystem is rapidly expanding, with a growing number of libraries and tools being developed. From web frameworks to embedded systems, the versatility of Rust is evident in its wide range of applications. The Rust community is known for its dedication to improving the language, contributing to its libraries, and providing extensive documentation and support.
// Example Rust code demonstrating a simple Fibonacci sequence generator
fn fibonacci(n: u32) -> u32 {
match n {
0 => 0,
1 => 1,
_ => fibonacci(n - 1) + fibonacci(n - 2),
}
}
fn main() {
let n = 10; // Change the value of n to generate a different Fibonacci sequence
for i in 0..n {
println!("Fibonacci({}) = {}", i, fibonacci(i));
}
}
The Safety Paradigm of Rust Programming
Rust’s approach to safety is foundational to its design and operation, setting it apart in the programming landscape. The language’s safety guarantees are not just a feature but a core philosophy, ensuring developers can write more reliable and secure code.
Memory Safety
At the heart of Rust’s safety paradigm is memory safety. Rust achieves this through its unique ownership model, which effectively manages memory without the need for a garbage collector. This system ensures safe memory access and helps prevent common errors like dangling pointers and buffer overflows.
Null and Type Safety
Rust also enforces null and type safety. The language’s type system is designed to catch errors at compile time, thus preventing null reference exceptions that are common in other languages. The compiler’s strict type checking further aids in preventing runtime errors and ensures that code behaves as expected.
Thread Safety
Concurrency in Rust is handled with a focus on safety. The language’s ownership and borrowing rules extend to its concurrency model, preventing data races by design. This makes Rust an ideal choice for writing multi-threaded applications where safety and performance are critical.
Compiler’s Role in Enforcing Safety
Rust’s compiler is known for being strict and helpful. It plays a crucial role in enforcing safety by refusing to compile code that violates safety principles. This means that a lot of potential runtime errors are caught during compilation, significantly reducing the likelihood of bugs in production code.
Rust in AI and Machine Learning: A Promising Union
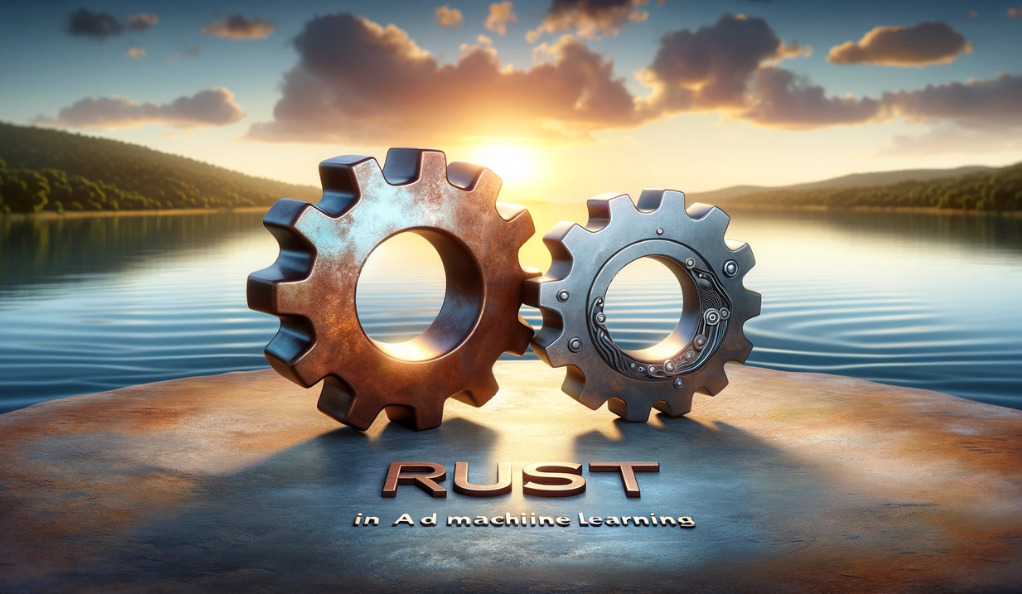
Rust’s integration into the fields of Artificial Intelligence (AI) and Machine Learning (ML) is opening new frontiers. Its emphasis on performance and memory safety aligns perfectly with the demands of AI and ML applications.
Performance and Memory Safety
In AI and ML, efficient resource management is crucial, especially when dealing with large datasets and complex algorithms. Rust’s memory safety and zero-cost abstractions make it a strong candidate for these applications. Its ability to manage resources efficiently without a garbage collector ensures that AI and ML applications run faster and more reliably.
Growing Tools and Libraries
The Rust ecosystem is rapidly adapting to the needs of AI and ML. With an increasing number of libraries and tools being developed, Rust is becoming more accessible for AI and ML development. These tools are not only focused on performance but also on providing safe and efficient ways to handle AI and ML workloads.
Case Studies and Emerging Trends
There are already several case studies where Rust has been successfully used in AI and ML projects. These instances highlight Rust’s potential in handling complex computations and data-intensive tasks efficiently. As more developers explore Rust for AI and ML, we can expect to see innovative applications and breakthroughs in these fields.
AI Tools Transforming Rust Development
The integration of AI tools into Rust development is revolutionizing the way programmers approach coding in this language. These tools are enhancing code generation, optimization, and learning processes, making Rust programming more accessible and efficient.
OpenAI Codex and Rust
One of the most notable AI tools in the Rust ecosystem is OpenAI Codex, which powers GitHub Copilot. This tool is particularly adept at generating code within mainstream development environments. For Rust developers, this means enhanced productivity, as the AI can generate boilerplate code, suggest code optimizations, and even identify potential issues.
Advanced Learning with AI Assistance
Learning Rust can be a daunting task, given its unique features and strict compiler. AI tools have made this learning curve less steep. By providing real-time feedback, code suggestions, and automated error detection, these tools help both novice and experienced programmers refine their Rust coding skills more efficiently.
AI-Driven Code Optimization
Beyond just code generation, AI tools are now capable of optimizing Rust code. They can analyze code for inefficiencies, suggest better algorithms, and even refactor code for improved performance and readability. This not only saves time but also ensures that the Rust codebases are maintainable and efficient.
Optimizing Rust Code with AI Integration
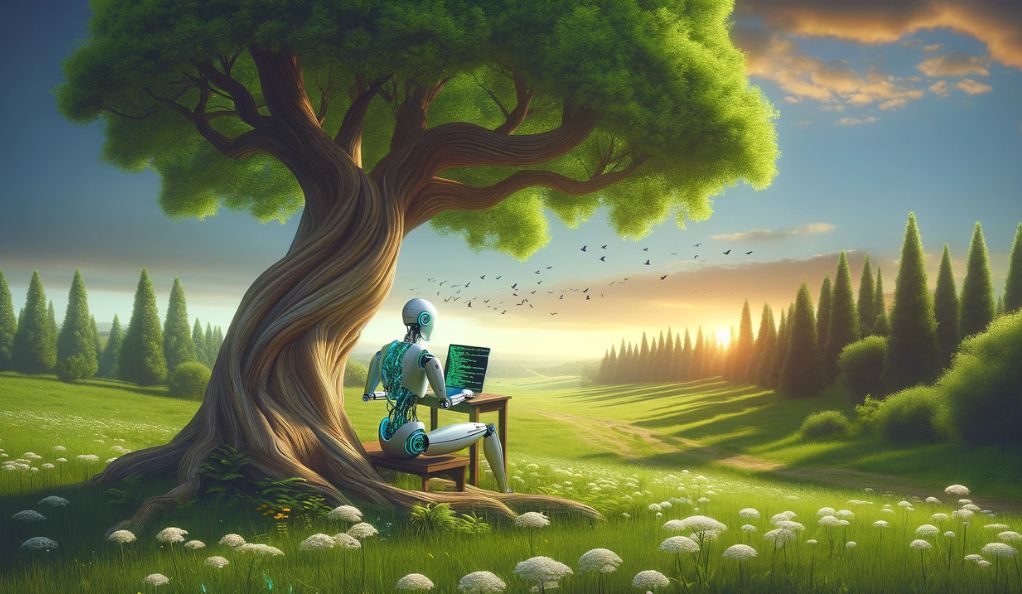
The integration of Artificial Intelligence (AI) in Rust programming has led to significant advancements in code optimization. AI tools are now playing a crucial role in enhancing the efficiency, readability, and maintainability of Rust code.
AI-Driven Tools for Rust Code Enhancement
A variety of AI-driven tools are available for optimizing Rust code. These tools leverage machine learning algorithms to analyze code patterns, suggest optimizations, and even refactor code. By doing so, they enhance the code’s performance and make it more readable and maintainable.
Maintainability and Performance
One key aspect of these AI tools is their ability to improve code maintainability. They can identify complex code segments and suggest simplifications, making the code easier to understand and modify. Additionally, these tools optimize code for performance, ensuring that Rust applications run efficiently.
Predicting and Preventing Errors: The AI Advantage
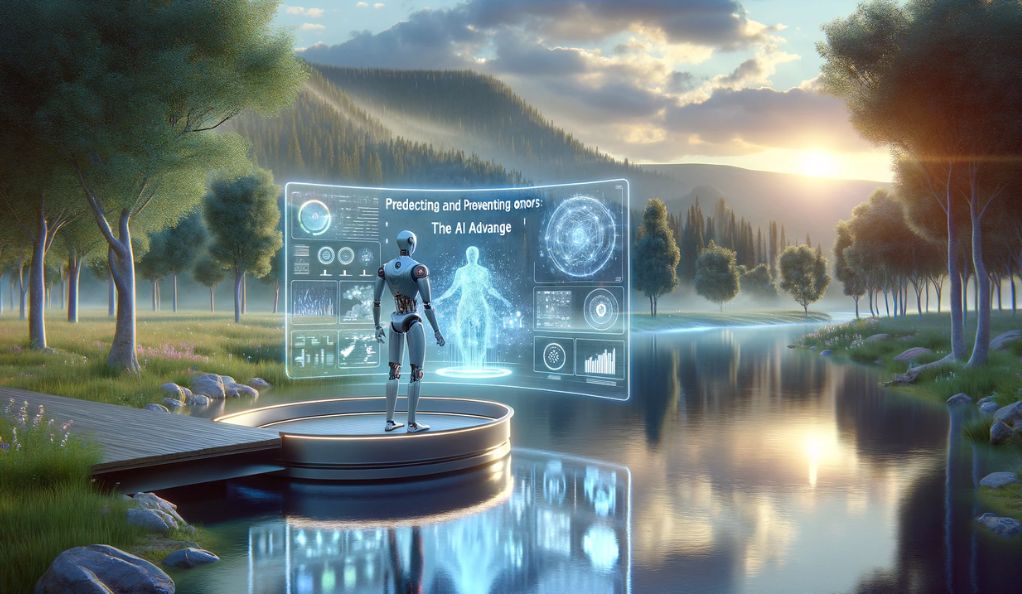
The integration of Artificial Intelligence (AI) in Rust programming goes beyond code optimization—it plays a pivotal role in error prediction and prevention. This capability is crucial in enhancing the reliability and security of Rust applications.
AI in Error Detection
AI tools have the capability to analyze Rust code and predict potential errors before they occur. By examining code patterns and historical data, these tools can identify anomalies or practices that are likely to lead to errors. This proactive approach helps developers fix issues before they manifest into larger problems.
Enhancing Rust’s Safety Features
Rust is already known for its strong safety features, but AI takes this a step further. With AI’s predictive capabilities, the safety net that Rust provides is significantly strengthened. This is particularly beneficial in complex applications where manual error detection can be challenging.
Real-World Applications
In real-world scenarios, AI-driven error prediction tools have been instrumental in identifying and resolving potential vulnerabilities in Rust code. These tools help maintain high standards of code quality and security, which is especially important in security-sensitive projects.
Future Prospects: Rust and AI Synergy
The synergy between Rust programming and Artificial Intelligence (AI) is poised to reshape the landscape of software development. As both fields continue to evolve, their integration holds immense potential for future technological advancements.
Evolving Landscape of Rust with AI
The continuous development in the Rust ecosystem, coupled with the rapid advancements in AI, is leading to more sophisticated and efficient software solutions. The integration of AI in Rust is not just enhancing current programming practices but also opening up new possibilities for innovative applications.
Predictions for Rust and AI
The future of Rust programming, enhanced with AI, is likely to see more intuitive development environments, smarter error handling, and even more robust security features. The use of AI in code optimization and error prediction is expected to become more refined, making Rust an even more powerful tool for developers.
Potential in Future Tech Solutions
The combination of Rust’s performance and safety features with AI’s predictive and optimization capabilities holds great promise for future tech solutions. This could range from more sophisticated machine learning applications to safer, more efficient system-level programming. The potential for Rust and AI in areas like IoT, cloud computing, and big data is particularly exciting.
Conclusion
Rust programming, now synergized with Artificial Intelligence (AI), marks a transformative era in software development. This integration brings together Rust’s memory safety, performance, and concurrency with AI’s prowess in predictive analytics and code optimization. The result is a powerful toolkit for developers, fostering the creation of more robust, efficient, and error-resistant software. This blend is particularly vital in today’s rapidly evolving technological landscape, where reliability and efficiency are paramount.
Looking ahead, the combination of Rust and AI is set to significantly influence future software development trends. The synergy of these technologies is unlocking exciting possibilities, ranging from cutting-edge machine learning applications to enhanced security and efficiency in system-level programming. This convergence is not just a trend but a visionary direction for the future of software development, promising smarter, safer, and more sophisticated solutions.