Introduction to Deep Learning
Deep Learning is an advanced subset of artificial intelligence (AI) that mimics the workings of the human brain in processing data and creating patterns for use in decision making. It’s a field that has revolutionized many aspects of technology and our daily lives, from voice recognition systems to medical diagnostics.
Defining Deep Learning
At its core, deep learning is a method of teaching computers to learn by example, much like humans do. It’s a type of machine learning, a broader concept that refers to computers learning from data. However, deep learning is unique in its ability to process vast amounts of unstructured data, learning complex patterns at multiple levels of abstraction. This ability is powered by neural networks, which are algorithms modeled loosely after the human brain.
How Deep Learning Differs from Traditional Machine Learning
While traditional machine learning algorithms linearly analyze data, deep learning’s neural networks enable a hierarchical level of learning. This hierarchy allows the system to handle more complex, abstract tasks by breaking them down into simpler ones. In essence, where traditional algorithms might struggle with tasks like recognizing faces or understanding natural language, deep learning excels.
The Importance of Deep Learning in Today’s Tech Landscape
Deep learning has become a cornerstone of modern technology, driving advances in fields as diverse as autonomous vehicles, language translation, and medical image analysis. Its importance lies in its ability to learn and improve from experience, adapt to new data, and produce results that are increasingly accurate and human-like. This technology is not just a passing trend but a fundamental shift in how machines interact with the world, offering significant benefits in efficiency, accuracy, and automation.
Understanding Neural Networks
Neural networks form the backbone of deep learning. They are inspired by the biological neural networks that constitute animal brains. At a basic level, a neural network in AI is a series of algorithms that endeavors to recognize underlying relationships in a set of data through a process that mimics the way the human brain operates.
Basics of Neural Networks
A neural network consists of layers of interconnected nodes, or neurons, each of which performs a simple computation. The simplest form of a neural network has three layers:
- Input Layer: This is where the network receives its input data.
- Hidden Layers: These layers perform computations using weights and biases, adjusted during the learning process. They are called ‘hidden’ because unlike the input and output layers, they do not directly interact with the external environment.
- Output Layer: The final layer that provides the output of the model, based on the computations and transformations that occurred in the hidden layers.
The real power of neural networks lies in these hidden layers, where complex patterns and features are extracted from the input data.
Types of Neural Networks
There are various types of neural networks designed for different tasks. Some of the most common include:
- Convolutional Neural Networks (CNNs): Primarily used in image recognition and processing, they are adept at picking up patterns like edges, shapes, and textures.
- Recurrent Neural Networks (RNNs): Ideal for sequence data such as time series or natural language, RNNs have a memory feature that retains information from previous inputs.
- Autoencoders: Used for unsupervised learning tasks, such as feature learning and dimensionality reduction.
How Neural Networks Learn and Adapt
The learning process in neural networks involves adjusting the weights of the connections based on the error of the output compared to the expected result. This process is known as backpropagation. It uses algorithms like gradient descent to minimize the error by adjusting the weights. Over time, this enables the network to make more accurate predictions or decisions.
Key Concepts and Terminology
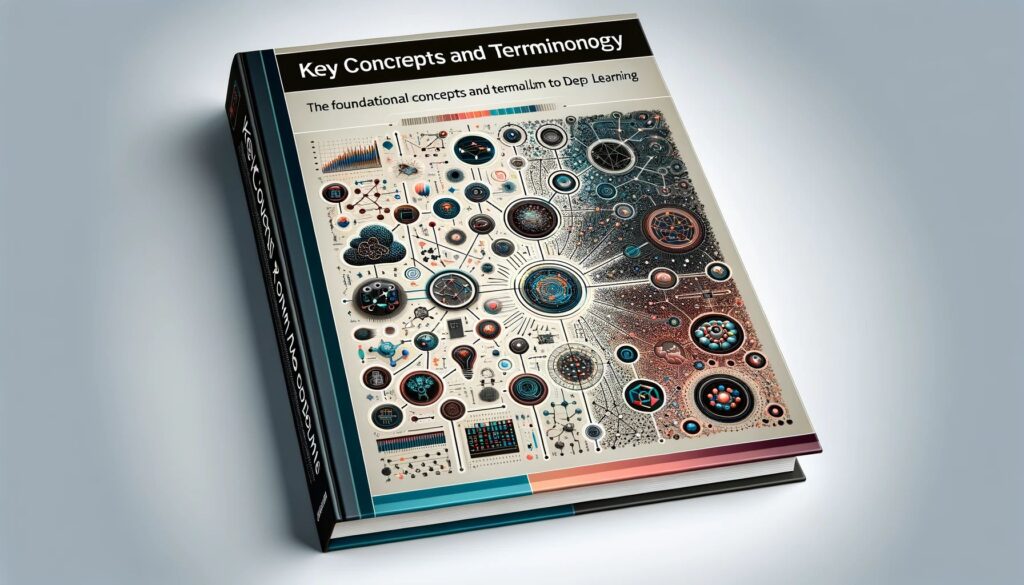
Deep learning, while a complex field, is built upon a foundation of key concepts and terminology that are essential to grasp for anyone delving into this area. Understanding these fundamental elements will provide a clearer picture of how deep learning models function and learn.
Understanding Layers, Neurons, and Activation Functions
- Layers: As mentioned, a neural network is composed of layers. Each layer consists of units or neurons. The input layer receives the initial data, while the hidden layers process the data, and the output layer presents the final output.
- Neurons: These are the basic units of a neural network. Each neuron receives input, processes it, and passes an output to the next layer. The processing involves weighing the input, adding a bias, and then applying an activation function.
- Activation Functions: These are mathematical equations that determine the output of a neural network. They add non-linearity to the model, enabling it to learn more complex patterns. Common examples include the Sigmoid, Tanh, and ReLU functions.
The Role of Data in Training Models
Data is the cornerstone of any deep learning model. The quality and quantity of data directly impact the model’s performance. Training data is used to teach the model, validation data to tune the parameters, and test data to evaluate its performance. Deep learning models require a substantial amount of data to learn effectively, making data collection and preprocessing critical steps in the development process.
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Activation
# Create a simple neural network with one hidden layer
model = Sequential([
Dense(5, input_shape=(3,), activation='relu'), # Input layer with 3 inputs and hidden layer with 5 neurons
Dense(2, activation='softmax') # Output layer with 2 outputs
])
# Compile the model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Dummy data (3 features per sample)
data = [[0.1, 0.2, 0.3], [0.2, 0.2, 0.3], [0.3, 0.4, 0.5]]
labels = [0, 1, 0] # Corresponding labels
# Train the model
model.fit(data, labels, epochs=10)
Overfitting and Regularization Explained
- Overfitting: This occurs when a model learns the training data too well, including its noise and outliers, which reduces its ability to perform well on new, unseen data. It’s a common issue in deep learning due to the complexity of the models.
- Regularization: To prevent overfitting, techniques like dropout, L1, and L2 regularization are used. These methods adjust the learning process to ensure the model remains general and applicable to new data.
Tools and Libraries for Deep Learning
The deep learning landscape is rich with tools and libraries, each offering unique features and capabilities. Understanding these tools is vital for anyone looking to enter the field.
Overview of TensorFlow, PyTorch, and Keras
- TensorFlow: Developed by Google, TensorFlow is a comprehensive library for numerical computation and machine learning. It is known for its flexibility and scalability, especially in large-scale deep learning applications.
- PyTorch: Created by Facebook’s AI Research lab, PyTorch is favored for its dynamic computational graph and user-friendly interface. It’s particularly popular in research and development due to its ease of use and intuitive design.
- Keras: Keras is a high-level neural networks API running on top of TensorFlow. It’s designed for easy and fast experimentation with deep neural networks and is known for its user-friendliness.
import torch
import torch.nn as nn
import torch.nn.functional as F
# Define a simple neural network
class SimpleNet(nn.Module):
def __init__(self):
super(SimpleNet, self).__init__()
self.fc1 = nn.Linear(3, 5) # Input layer with 3 inputs, hidden layer with 5 neurons
self.fc2 = nn.Linear(5, 2) # Output layer with 2 outputs
def forward(self, x):
x = F.relu(self.fc1(x))
x = self.fc2(x)
return x
# Create a network instance and a sample input tensor
net = SimpleNet()
sample_input = torch.tensor([0.1, 0.2, 0.3])
# Forward pass
output = net(sample_input)
print(output)
This code demonstrates how to set up a basic neural network in PyTorch, showcasing its straightforward and intuitive approach to deep learning.
Choosing the Right Tool for Your Project
Selecting the right library depends on various factors:
- Project Complexity: TensorFlow is ideal for large-scale, complex projects, while Keras suits simpler, quick-to-deploy applications.
- Ease of Use: PyTorch and Keras are more beginner-friendly, with simpler syntax and a more intuitive approach to building neural networks.
- Community and Support: TensorFlow and PyTorch have large, active communities, making finding help and resources easier.
Community and Resources for Learning
Each of these tools has a robust community and a wealth of resources for learning:
- Official Documentation: Always the best place to start, providing comprehensive guides and tutorials.
- Online Courses and Tutorials: Platforms like Coursera, Udemy, and YouTube offer extensive courses covering these libraries.
- Community Forums: Websites like Stack Overflow, Reddit, and the respective tool’s community forums are invaluable for troubleshooting and advice.
Getting Started with Deep Learning Projects
Starting a deep learning project can be both exciting and challenging, but with the right approach, you can begin your journey in this field effectively.
Setting Up Your Environment
Before you dive into deep learning projects, you’ll need to set up your development environment. Here are the basic steps:
- Choose a Programming Language: Python is the most widely used language for deep learning due to its rich libraries and frameworks like TensorFlow and PyTorch.
- Install Required Libraries: Install the deep learning libraries of your choice. You can use Python package managers like pip or conda for this purpose.
- Select a Development Environment: You can use popular integrated development environments (IDEs) like Jupyter Notebook, PyCharm, or VSCode for coding.
- Data Preparation: Gather and preprocess your data. Ensure it’s well-structured and suitable for your project.
Best Practices for Beginners
- Start with Tutorials: Begin with beginner-friendly tutorials provided by TensorFlow, PyTorch, or Keras. These tutorials often cover the basics and guide you through simple projects.
- Experiment with Small Datasets: Start with small datasets to avoid overwhelming yourself. Once you gain confidence, you can move on to larger datasets.
- Understand Model Selection: Select the appropriate model architecture for your task. For image classification, convolutional neural networks (CNNs) are commonly used, while recurrent neural networks (RNNs) are suitable for sequence data.
- Hyperparameter Tuning: Experiment with different hyperparameters like learning rate, batch size, and optimizer to fine-tune your model’s performance.
Simple Project Ideas to Get Started
- Image Classification: Create a deep learning model that can classify images into predefined categories. You can use datasets like CIFAR-10 or MNIST for practice.
- Sentiment Analysis: Build a sentiment analysis model that can determine whether a given text is positive, negative, or neutral. Use datasets of movie reviews or tweets for this project.
- Predictive Text Generation: Develop a model that generates human-like text based on a given prompt. You can use language models like GPT-2 for this task.
- Object Detection: Create a model that can detect and locate objects within images or videos. The COCO dataset is a good resource for this project.
- Recommendation Systems: Build a recommendation system that suggests products, movies, or music based on user preferences. Collaborative filtering and matrix factorization techniques can be explored.
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Embedding, LSTM, Dense
# Create a simple LSTM-based sentiment analysis model
model = Sequential([
Embedding(input_dim=10000, output_dim=128, input_length=100),
LSTM(128),
Dense(1, activation='sigmoid')
])
# Compile the model
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# Train the model with text data
model.fit(train_text, train_labels, epochs=10)
This code illustrates how to build a basic sentiment analysis model, a great starting point for a deep learning project.
Challenges and Ethical Considerations
Deep learning, like any powerful technology, presents challenges and ethical considerations that must be addressed to ensure responsible and unbiased development.
Addressing Bias in Models
One of the significant challenges in deep learning is bias in models. Models trained on biased or incomplete data can perpetuate and even exacerbate existing biases. For example, if a model is trained on historical hiring data that is biased towards a particular gender or race, it may make biased hiring decisions in the future.
To mitigate bias, developers must:
- Ensure diverse and representative training data.
- Regularly audit models for bias.
- Use techniques like re-sampling, re-weighting, or adversarial training to reduce bias.
Ethical Implications of Deep Learning
- Privacy: Deep learning models can process vast amounts of personal data. Protecting user privacy is essential, and models should comply with data protection laws and regulations.
- Job Displacement: Automation driven by deep learning can lead to job displacement in certain industries. Preparing the workforce for these changes is crucial.
- Autonomous Weapons: Concerns have been raised about the use of deep learning in autonomous weapons systems. Ethical guidelines and regulations are needed to address this issue.
- Explainability: Ensuring that AI models are explainable and transparent is vital. Users and stakeholders should understand how decisions are made.
- AI Safety: Research into the safety of AI systems is ongoing. Ensuring that AI systems do not cause harm is a top priority.
Conclusion
In conclusion, we’ve explored the fundamentals of deep learning, from neural networks to key concepts like layers, neurons, and activation functions. We’ve introduced essential tools and libraries such as TensorFlow, PyTorch, and Keras, along with their applications in diverse industries. Getting started in deep learning involves setting up your environment, experimenting with tutorials, and gradually taking on small projects. However, it’s essential to be aware of the challenges, including bias in models and ethical considerations. To further your learning journey, consider enrolling in online courses, reading relevant books, joining communities, staying updated with research, and applying your knowledge through hands-on projects. Deep learning is a dynamic field, and with these resources, you’re well-equipped to embark on an exciting exploration of its possibilities.