Pointers in C are a critical component that sets this programming language apart in its capability for efficient and powerful coding. They offer a unique approach to handling memory and data, providing unparalleled control and flexibility in various programming scenarios.
What is a Pointer?
In the realm of C programming, a pointer is a powerful tool. It is, fundamentally, a variable, but unlike a regular variable that holds a value, a pointer holds the address of another variable. This unique characteristic allows pointers to directly access and manipulate the memory where variables are stored, offering a deeper level of control over the data.
Understanding pointers is crucial for efficient C programming. They enable direct interaction with memory, leading to more effective and dynamic code. This capability is particularly vital for systems programming, where memory management is key, and in applications like data structures, dynamic memory allocation, and more.
Importance of Pointers in C Programming
Pointers in C are not just a feature but a backbone in many respects. Here’s why they are so pivotal:
- Memory Efficiency: Pointers allow for the manipulation of data in memory directly, which can lead to more memory-efficient programs.
- Dynamic Data Structures: They are fundamental in creating dynamic data structures like linked lists, trees, and graphs.
- Functionality Enhancement: Pointers enable functions to modify actual data rather than a copy, enhancing functionality and efficiency.
- System-Level Operations: They provide a way to interact with hardware, enabling system-level programming, which is crucial in operating systems and embedded systems.
Understanding pointers involves getting familiar with concepts like memory addresses, dereferencing, pointer arithmetic, and more. This foundational knowledge sets the stage for more advanced and powerful programming capabilities in C.
Basic Concepts of Pointers

Pointer Declaration and Initialization
Declaring a pointer in C is similar to declaring a regular variable, with the addition of an asterisk (*) symbol. This asterisk denotes that the variable is a pointer, designed to store a memory address. For example, declaring a pointer to an integer looks like this:
int *pointerToInt;
In this line, pointerToInt is capable of holding the address of an integer variable. To initialize this pointer, we usually assign it the address of a regular integer variable using the address-of operator (&):
int number = 10;
pointerToInt = &number;
Here, pointerToInt is assigned the address of number. Now, pointerToInt doesn’t just point to any integer, but specifically to number.
Understanding the Address-of Operator (&)
The address-of operator (&) is a unary operator in C that provides the memory address of a variable. It’s fundamental in linking a pointer to the location of a variable. For instance:
int value = 5;
int *pointer = &value;
In this code snippet, pointer is assigned the memory address of value. The &value expression yields the address where value is stored in memory.
The Role of Dereferencing in Pointers
Dereferencing is the process of accessing the value at a memory address. In C, this is done using the dereference operator (*). When applied to a pointer, it accesses the value stored at the pointer’s memory address. Here’s an example:
int value = 10;
int *pointer = &value;
int dereferencedValue = *pointer;
In this case, dereferencedValue will be 10, the value stored at the memory address held by pointer. This capability of accessing and modifying data directly through pointers is what makes them so powerful in C programming.
Understanding these basic concepts of pointers – declaration, the address-of operator, and dereferencing – is crucial for any C programmer. They form the foundation upon which more complex pointer operations are built, as we will see in the subsequent sections.
Pointers and Memory Management
Effective memory management is a crucial skill in C programming, and pointers play a key role in this area. They allow programmers to allocate, manage, and free memory dynamically, which is essential in creating efficient and scalable programs.
Addressing Common Misconceptions and Pitfalls
A common misconception with pointers is that they are inherently complex and error-prone. While pointers do require careful handling, understanding their behavior and following best practices can greatly reduce the risk of errors. One common mistake is forgetting to initialize a pointer, which can lead to undefined behavior or segmentation faults. Always initialize pointers, either to NULL or to a valid memory address, to avoid such issues.
Using Pointers for Dynamic Memory Allocation
Dynamic memory allocation is a process where memory is allocated during the runtime. In C, this is achieved using functions like malloc(), calloc(), realloc(), and free(). Pointers are used to store the address of dynamically allocated memory. For example:
int *dynamicArray = (int*)malloc(5 * sizeof(int));
if (dynamicArray != NULL) {
// Use the allocated memory
for (int i = 0; i < 5; i++) {
dynamicArray[i] = i;
}
// Don't forget to free the memory once done
free(dynamicArray);
}
In this example, malloc() allocates memory for an array of 5 integers, and dynamicArray points to the first element of this array. It’s essential to check if malloc() returns NULL, which indicates that the memory allocation failed. After using the allocated memory, it is vital to release it using free() to prevent memory leaks.
Memory Leaks and How to Prevent Them
Memory leaks occur when a program loses the reference to a block of dynamically allocated memory without freeing it. This can happen if a pointer is reassigned to another memory address without first releasing the memory it points to. Avoiding memory leaks requires diligent management of memory allocation and deallocation. Tools like Valgrind can be used to detect memory leaks in C programs.
The understanding of pointers in the context of memory management is crucial for writing efficient and reliable C programs. It involves not only allocating and deallocating memory but also ensuring that memory leaks and other common issues are addressed proactively.
Pointers and Data Structures
Pointers are particularly powerful when used in conjunction with data structures. Their ability to store and manipulate memory addresses makes them ideal for constructing complex structures such as arrays, linked lists, and trees.
Pointers in Arrays and Strings
In C, arrays and pointers are closely related. The name of an array acts as a pointer to its first element. This relationship allows pointers to be used effectively in accessing and manipulating array elements. Consider this example:
int numbers[3] = {10, 20, 30};
int *ptr = numbers;
for(int i = 0; i < 3; i++) {
printf("numbers[%d] = %d\n", i, *(ptr + i));
}
Here, ptr is a pointer to the first element of the numbers array. Using pointer arithmetic (ptr + i), we can access each element of the array.
Integrating Pointers with Structs
Pointers can also be used with structures to create dynamic and complex data structures. A common use is in linked lists, where each node contains a pointer to the next node. For example:
struct Node {
int data;
struct Node *next;
};
struct Node *head = NULL; // Pointer to the first node of the list
In this snippet, struct Node defines a linked list node with an integer data and a pointer to the next node. head is a pointer to the first node in the list.
Understanding Pointers to Pointers
Pointers to pointers are an advanced concept in C, which can be used for various purposes, including creating multidimensional arrays or managing dynamic arrays of pointers. A pointer to a pointer is declared using two asterisks (e.g., int **ptr;). This means that ptr is a pointer to another pointer.
In the context of data structures, understanding how pointers interact with arrays and structures is crucial. It allows for more dynamic and memory-efficient programs, especially when dealing with large amounts of data or complex data manipulations.
Advanced Pointer Concepts

Advanced usage of pointers in C programming opens up a range of possibilities, including pointer arithmetic, function pointers, and more complex data manipulations.
Pointer Arithmetic Explained
Pointer arithmetic allows the manipulation of the memory address that a pointer holds. This is particularly useful when dealing with arrays and dynamically allocated memory. Consider the following example:
int array[] = {10, 20, 30, 40, 50};
int *ptr = array;
for (int i = 0; i < 5; i++) {
printf("Element %d: %d\n", i, *(ptr + i));
}
In this code, ptr points to the first element of array. By incrementing ptr (ptr + i), we can traverse the array, accessing each element.
Function Pointers and their Applications
Function pointers are another intriguing aspect of C pointers. They allow storing the address of a function, which can then be called through the pointer. This is useful for callbacks, implementing function tables, and more. Here’s an example:
int add(int a, int b) {
return a + b;
}
int main() {
int (*operation)(int, int) = &add;
int result = operation(5, 3);
printf("Result: %d\n", result);
return 0;
}
In this snippet, operation is a pointer to a function that takes two integers as arguments and returns an integer. It points to the add function and is used to call it.
Pointers within Structures and Linked Lists
Pointers find extensive use in data structures, particularly in dynamically linked structures like linked lists and trees. For example, in a singly linked list, each node contains data and a pointer to the next node:
struct Node {
int data;
struct Node *next;
};
struct Node *head; // Pointer to the head of the list
In this example, head is a pointer to the first node (Node) of the list. Each Node consists of some data and a pointer to the next Node.
Exploring advanced pointer concepts broadens the horizon of what can be achieved with C programming. These concepts are pivotal in creating efficient and flexible programs, especially when dealing with complex data structures and dynamic memory allocation.
Best Practices and Considerations in Using Pointers
Utilizing pointers effectively in C programming involves adhering to certain best practices and being aware of potential pitfalls. These practices not only enhance code quality but also prevent common errors associated with pointer usage.
Error Handling and Null Pointers
One of the cardinal rules when working with pointers is to always check if a pointer is NULL before dereferencing it. A NULL pointer points to no valid memory location, and attempting to access such a pointer will likely result in a segmentation fault or a crash. Here’s an example:
int *ptr = getPointer(); // Assume this function returns an int pointer
if (ptr != NULL) {
// Safe to dereference ptr
printf("%d\n", *ptr);
}
In this code, before dereferencing ptr, there is a check to ensure it’s not NULL.
Ensuring Pointer Safety and Robust Programming
Pointer safety involves being cautious with pointer arithmetic and always ensuring that pointers point to valid memory locations. It’s also crucial to avoid “dangling pointers” – pointers that refer to memory that has been freed or is no longer valid. This can be mitigated by setting pointers to NULL after freeing the memory they point to.
Tips for Efficient Usage of Pointers
- Initialization: Always initialize pointers. Uninitialized pointers can point to random memory locations, leading to unpredictable behavior.
- Consistent Use of const: When a pointer should not modify the data it points to, use the const keyword. This is a good practice for both safety and readability.
- Memory Management: Be vigilant with memory allocation and deallocation. Every malloc or calloc should have a corresponding free to avoid memory leaks.
- Pointer Type Matching: Ensure the pointer type matches the type of the data it points to. Mismatched pointer types can lead to incorrect memory access and data interpretation.
By adhering to these best practices, programmers can effectively leverage the power of pointers while minimizing the risk of bugs and memory-related issues.
Practical Examples and Exercises
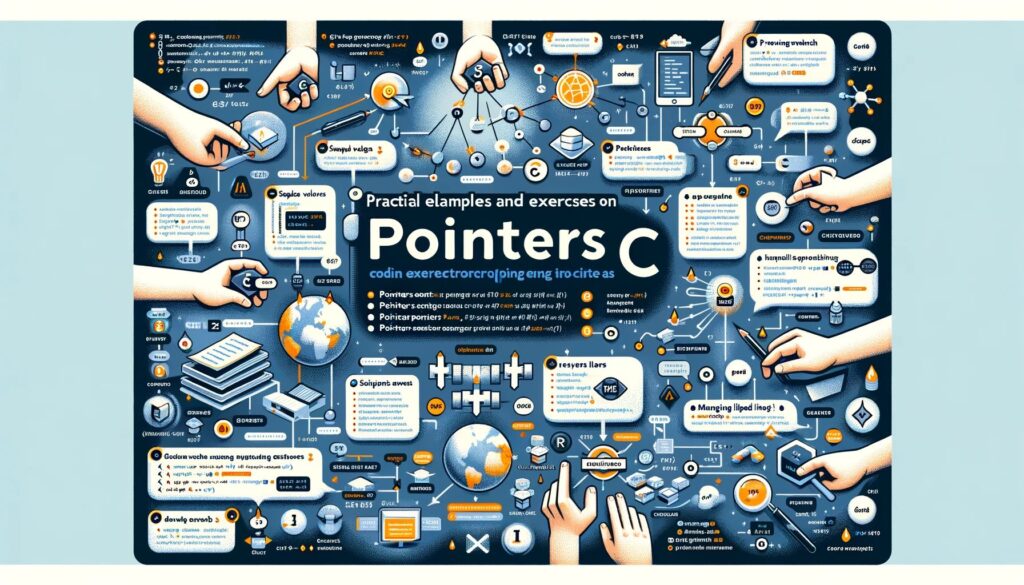
To consolidate your understanding of pointers in C, it’s beneficial to engage with practical examples and exercises. These not only reinforce the concepts discussed but also provide hands-on experience in applying them in real-world scenarios.
Simple Programs to Illustrate Pointer Usage
A basic example can involve a program that uses pointers to swap the values of two variables. This demonstrates the ability of pointers to manipulate data directly:
void swap(int *x, int *y) {
int temp = *x;
*x = *y;
*y = temp;
}
int main() {
int a = 10, b = 20;
swap(&a, &b);
printf("a = %d, b = %d\n", a, b);
return 0;
}
In this example, swap uses pointers to change the values of a and b directly.
Advanced Pointer-based Solutions
For more advanced practice, one could write a program using pointers to reverse an array. This kind of exercise challenges the understanding of pointer arithmetic and memory manipulation:
void reverseArray(int *array, int size) {
int *start = array;
int *end = array + size - 1;
while (start < end) {
int temp = *start;
*start = *end;
*end = temp;
start++;
end--;
}
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
reverseArray(arr, 5);
// Code to print the reversed array
return 0;
}
Exercises for Hands-On Practice
For further practice, consider these exercises:
- Create a dynamic array using pointers and implement functions to add, delete, and search for elements.
- Write a program using function pointers to implement a simple calculator.
- Develop a linked list implementation using pointers, including functions to insert, delete, and traverse the list.
Engaging with these practical examples and exercises will deepen your understanding of pointers in C. They illustrate the versatility of pointers and provide a platform for developing strong programming skills.
Conclusion
In conclusion, pointers in C are a fundamental and powerful feature that, when mastered, can significantly enhance a programmer’s ability to write efficient and effective code. From basic operations like declaration and dereferencing to more complex applications in dynamic memory management, data structures, and function pointers, the journey through understanding pointers is both challenging and rewarding. By adhering to best practices and engaging with practical examples, programmers can avoid common pitfalls and unlock the full potential of pointers. Whether you are a beginner or an experienced coder, a deep understanding of pointers is invaluable in the world of C programming, opening doors to more advanced programming techniques and a better grasp of how computers operate at a fundamental level.