In the ever-evolving digital world, the security of web applications is paramount. PHP, being one of the most popular scripting languages for web development, is often a target for cyber-attacks. This makes understanding and implementing PHP security best practices crucial for developers and organizations alike.
The Imperative of PHP Security
PHP’s widespread use in web development, from small-scale projects to large-scale enterprise applications, exposes it to various security vulnerabilities. The consequences of a security breach can range from data theft and financial loss to reputational damage and legal implications. As such, securing PHP applications is not just a technical necessity but a business imperative.
Emerging Threats in the PHP Landscape
The PHP landscape is constantly challenged by emerging threats. These include SQL injection, Cross-Site Scripting (XSS), Cross-Site Request Forgery (CSRF), and more. Each year brings new types of vulnerabilities, making it critical for developers to stay informed and proactive in their security approach.
Always Code with Security in Mind
The foundation of PHP security lies in the mindset and practices of the developer. Coding with security as a priority helps prevent vulnerabilities from being introduced into the codebase in the first place.
Implementing Secure Coding Practices from the Start
Secure coding in PHP is about more than just following a checklist; it’s a philosophy that should be integrated into every aspect of development. Here are some key practices:
- Input Validation: Treat all user input as untrustworthy. Use PHP’s filter_var() function to validate email addresses, URLs, or other specific data types.
- Error Handling: Craft error messages that don’t disclose sensitive information. Instead of detailed error outputs, use generalized messages and log the details for internal review.
- Use of Frameworks and Libraries: Frameworks like Laravel or Symfony come with built-in security features. Use these devices to your advantage but understand their workings and limitations.
Secure Coding Examples
Here are some examples of secure PHP coding practices:
1. Input Validation:
if (!filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo "Invalid email format";
}
This code snippet validates an email address, ensuring it conforms to a standard email format.
2. Error Handling:
try {
// Code that may throw an exception
} catch (Exception $e) {
error_log($e->getMessage());
echo "An error occurred. Please try again later.";
}
This approach logs detailed error information for internal use while providing a user-friendly message.
Common PHP Vulnerabilities
Understanding common vulnerabilities helps in writing more secure code:
- SQL Injection: Occurs when attackers manipulate a SQL query via untrusted user input.
- Cross-Site Scripting (XSS): Happens when malicious scripts are injected into web pages.
- Cross-Site Request Forgery (CSRF): Involves unauthorized commands being transmitted from a user that the web application trusts.
Validating and Sanitizing User Input
Proper validation and sanitization of user input are critical for PHP security. These practices are essential in preventing common vulnerabilities such as SQL Injection and Cross-Site Scripting (XSS).
Techniques to Prevent SQL Injection and XSS Attacks
- Input Validation: Ensure the data fits the expected format. Use PHP functions like filter_input() or preg_match() for pattern matching.
- Prepared Statements for SQL Queries: Use prepared statements with PDO (PHP Data Objects) or MySQLi to securely handle SQL queries.
- Example of Prepared Statements with PDO:
$stmt = $pdo->prepare("SELECT * FROM users WHERE email = :email");
$stmt->execute(['email' => $email]);
This code snippet prevents SQL injection by using named placeholders in SQL statements.
3. Sanitization of User Input: Use PHP functions like htmlspecialchars() to prevent XSS by encoding special characters in user input.
- Example of XSS Prevention:
echo htmlspecialchars($user_input, ENT_QUOTES, 'UTF-8');
This converts special characters to HTML entities, preventing them from being executed as part of the HTML.
Importance of Data Validation and Sanitization
Validating and sanitizing user input are vital for several reasons:
- Security: They protect against malicious data that can exploit vulnerabilities.
- Data Integrity: They ensure the data received is what the application expects.
- User Trust: They contribute to creating a secure environment for users.
Using SSL/TLS for Secure Data Transmission
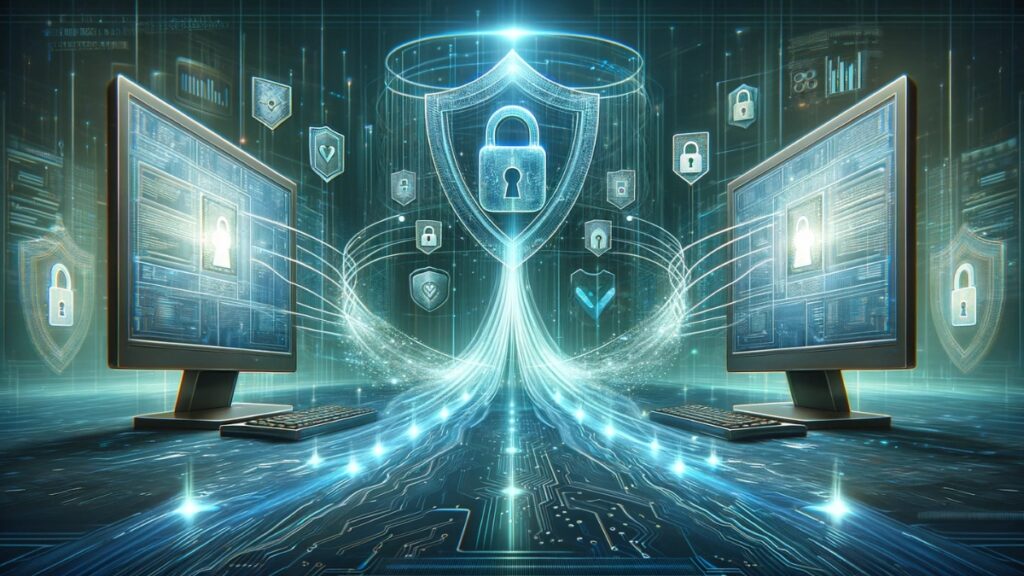
Securing data transmission is a crucial aspect of PHP security, primarily achieved through the implementation of SSL/TLS (Secure Sockets Layer/Transport Layer Security).
The Role of SSL/TLS in PHP Security
SSL/TLS provides an encrypted channel for data transmission between the client (user’s browser) and the server (your PHP application). This encryption is critical for protecting sensitive data such as login credentials, personal information, and financial transactions.
Configuring SSL Certificates for PHP Applications
1. Obtaining an SSL Certificate: You can get an SSL certificate from a Certificate Authority (CA). There are free options like Let’s Encrypt or paid options offering various levels of validation.
2. Configuring Your Web Server: After obtaining an SSL certificate, it needs to be installed and configured on your web server (e.g., Apache, Nginx).
- Example for Apache:
In the Apache configuration file (httpd.conf or apache2.conf), set up the Virtual Host to use SSL:
<VirtualHost *:443>
ServerName www.yourdomain.com
SSLEngine on
SSLCertificateFile /path/to/your/certificate.crt
SSLCertificateKeyFile /path/to/your/private.key
SSLCertificateChainFile /path/to/CA-bundle.crt
</VirtualHost>
3. Enforcing HTTPS in PHP:
Redirect all HTTP traffic to HTTPS. This can be done with an .htaccess file in Apache or similar configuration in other web servers.
- Example using .htaccess:
RewriteEngine On
RewriteCond %{HTTPS} off
RewriteRule ^(.*)$ https://%{HTTP_HOST}%{REQUEST_URI} [L,R=301]
Employing Encryption and Hashing
Encryption and hashing are vital techniques in PHP security, especially when it comes to protecting sensitive data like passwords and personal information.
Necessity of Encrypting Sensitive Data
Encryption transforms data into a secure form which can only be deciphered with the correct key. It’s essential for protecting data at rest (stored data) and data in transit (like during API calls).
Using Strong Hashing Algorithms for Data Integrity
Hashing is particularly crucial for password storage. Instead of storing plain text passwords, PHP applications should store hashed versions, which are virtually impossible to reverse-engineer.
Implementing Encryption and Hashing in PHP
1. Using PHP’s password_hash() for Passwords:
PHP provides the password_hash() function, which simplifies the process of hashing passwords using a strong algorithm like bcrypt.
- Example of password_hash():
$hashedPassword = password_hash("userPassword", PASSWORD_DEFAULT);
This line of code creates a secure hash of the user’s password.
2. Verifying Hashed Passwords:
When a user logs in, their entered password needs to be verified against the stored hash. PHP’s password_verify() function makes this simple.
- Example of password_verify():
if (password_verify('userPassword', $hashedPassword)) {
// Password is correct
}
This checks if the entered password matches the hashed version stored in the database.
3. Encrypting Data:
For encrypting sensitive data, PHP offers several functions and libraries. For example, you can use OpenSSL for robust encryption.
- Example using OpenSSL for Encryption:
$data = "Sensitive Data";
$encryption_key = openssl_random_pseudo_bytes(32);
$iv = openssl_random_pseudo_bytes(openssl_cipher_iv_length('aes-256-cbc'));
$encrypted = openssl_encrypt($data, 'aes-256-cbc', $encryption_key, 0, $iv);
Prepared SQL Statements to Prevent Injections
Prepared statements are a fundamental part of PHP security, primarily used to prevent SQL injection attacks, which occur when an attacker manipulates a SQL query through unsecured user input.
Importance of Using Prepared Statements
Prepared statements separate SQL logic from data, making it impossible for an attacker to inject malicious SQL through user input. They are a robust method for executing SQL queries safely, especially when handling user-provided data.
Example of Prepared Statements with PDO
1. Using PDO (PHP Data Objects):
PDO is a database access layer providing a uniform method of access to multiple databases.
- PDO Prepared Statement Example:
$pdo = new PDO('mysql:host=example.com;dbname=database', 'username', 'password');
$stmt = $pdo->prepare("INSERT INTO users (username, email) VALUES (:username, :email)");
$stmt->bindParam(':username', $username);
$stmt->bindParam(':email', $email);
$stmt->execute();
In this example, bindParam binds the PHP variables to the SQL query and ensures that the inputs are treated as strings, not executable SQL.
Example of Prepared Statements with MySQLi
1. Using MySQLi Extension:
MySQLi is a PHP extension designed to work with MySQL databases.
- MySQLi Prepared Statement Example:
$mysqli = new mysqli('example.com', 'username', 'password', 'database');
$stmt = $mysqli->prepare("INSERT INTO users (username, email) VALUES (?, ?)");
$stmt->bind_param("ss", $username, $email);
$stmt->execute();
This example uses bind_param, which binds the variables $username and $email to the placeholders ? in the SQL query.
Regular Security Audits and Code Maintenance
Regular security audits and maintaining up-to-date code are critical for ensuring the ongoing security of PHP applications. These practices help identify and fix vulnerabilities before they can be exploited.
Significance of Continuous Security Assessments
Regular security audits involve reviewing code, checking for known vulnerabilities, and testing the application for security flaws. This proactive approach helps in early detection of potential security issues.
Keeping PHP and its Libraries Up-to-Date
Using the latest versions of PHP and its libraries is crucial. Each update often includes patches for security vulnerabilities discovered in previous versions.
Secure Authentication and Session Management
Secure authentication and session management are crucial for safeguarding user identities and preventing unauthorized access to PHP applications.
Best Practices for User Authentication
1. Strong Password Policies:
Enforce strong password requirements to enhance account security. Utilize PHP’s password_hash() and password_verify() for secure password handling.
2. Multi-Factor Authentication (MFA):
Implementing MFA adds an additional layer of security. While PHP doesn’t directly support MFA, you can integrate third-party MFA services.
3. Session Security:
Securely managing sessions is vital to prevent session hijacking and fixation attacks.
- Example of Secure Session Code:
session_start();
// Regenerate session ID
session_regenerate_id();
// Set session cookie parameters
session_set_cookie_params([
'lifetime' => 0, // session cookie lifetime
'path' => '/',
'domain' => $_SERVER['HTTP_HOST'],
'secure' => true, // transmit cookie over HTTPS only
'httponly' => true // inaccessible to JavaScript
]);
This code snippet initiates a secure session, regenerates the session ID to prevent fixation, and configures the session cookie with secure parameters.
Secure Handling of Session Data
Handling session data securely involves a few key practices:
- Storing Minimal Data: Avoid storing sensitive information in session variables.
- Session Expiration: Implement an expiration policy for sessions to reduce the risk of unauthorized access.
- HTTPS Only Cookies: Ensure that session cookies are transmitted only over HTTPS connections.
Leveraging PHP Security Frameworks and Equipment
Modern PHP frameworks and equipment can significantly enhance the security of web applications by providing built-in functionalities and best practices.
How Frameworks Enhance Security
Frameworks like Laravel, Symfony, and CodeIgniter come with security features that handle common vulnerabilities. They offer functionalities such as CSRF protection, input validation, and more.
1. Using Laravel’s Security Features:
Laravel, for instance, has several security features out of the box:
- CSRF Protection: Laravel automatically generates and verifies CSRF tokens for each active user session, which helps protect against CSRF attacks.
- Code Example for CSRF Token in a Laravel Form:
<form method="POST" action="/profile">
@csrf
<!-- form body -->
</form>
This snippet includes a CSRF token in a Laravel form, which is validated upon form submission.
2. Utilizing Symfony’s Security Components:
Symfony provides a comprehensive security component that offers various user authentication and authorization mechanisms.
- Code Example for User Authentication in Symfony:
// Controller method in Symfony
public function login(AuthenticationUtils $authenticationUtils): Response
{
// get the login error if there is one
$error = $authenticationUtils->getLastAuthenticationError();
// last username entered by the user
$lastUsername = $authenticationUtils->getLastUsername();
return $this->render('security/login.html.twig', [
'last_username' => $lastUsername,
'error' => $error,
]);
}
This example demonstrates a basic user authentication setup in Symfony.
Using Devices for Security Analysis and Vulnerability Detection
Several devices are available to aid in PHP security:
- RIPS: A static code analysis tool dedicated to detecting PHP security vulnerabilities.
- PhpSecInfo: Provides a security overview of your PHP environment.
Conclusion
Securing PHP applications is an ongoing, multifaceted endeavor. By embracing best practices such as validating user input, employing encryption, using secure frameworks, and regularly conducting security audits, developers can significantly bolster the defense of their web applications. Keeping PHP versions updated and leveraging security devices further ensures robust protection. Ultimately, the commitment to continuous learning and adapting to emerging threats is key to maintaining a secure and reliable PHP environment.