In the realm of web development, the integration of email functionality is a cornerstone feature, vital for a myriad of applications ranging from simple contact forms to complex notification systems. PHP, a server-side scripting language, stands out as a powerful tool for handling email-related tasks due to its flexibility, ease of use, and robust library support. In this section, we delve into the basics of PHP’s role in email handling and underscore the importance of email integration in modern web applications.
The Role of PHP in Email Handling
PHP’s inherent capabilities in managing emails stem from its server-side nature, allowing it to interact seamlessly with mail servers and protocols. This interaction is crucial for tasks such as sending out automated emails, managing user verification processes, and even handling newsletter subscriptions. PHP’s mail() function, for instance, is a straightforward yet powerful tool for sending emails. However, its simplicity can sometimes be limiting, especially when dealing with more complex email functionalities like attachments or HTML content, which is where additional libraries and frameworks come into play.
Importance of Email Integration
Email integration is more than just a feature; it’s a vital communication bridge between a web application and its users. Here are some key reasons why email integration is crucial:
- User Communication: Emails are a primary channel for communicating with users, be it for confirmation emails, password resets, or promotional content.
- Notifications and Alerts: Automated emails for notifications or alerts keep users informed about important updates or changes related to their interests or activities on the site.
- Marketing and Outreach: Email campaigns are a critical part of digital marketing strategies, allowing for targeted and personalized communication with potential or existing customers.
- Feedback and Support: Providing users with the ability to communicate through email enhances support and feedback mechanisms, essential for improving user experience and engagement.
PHP’s Flexibility with Email Protocols
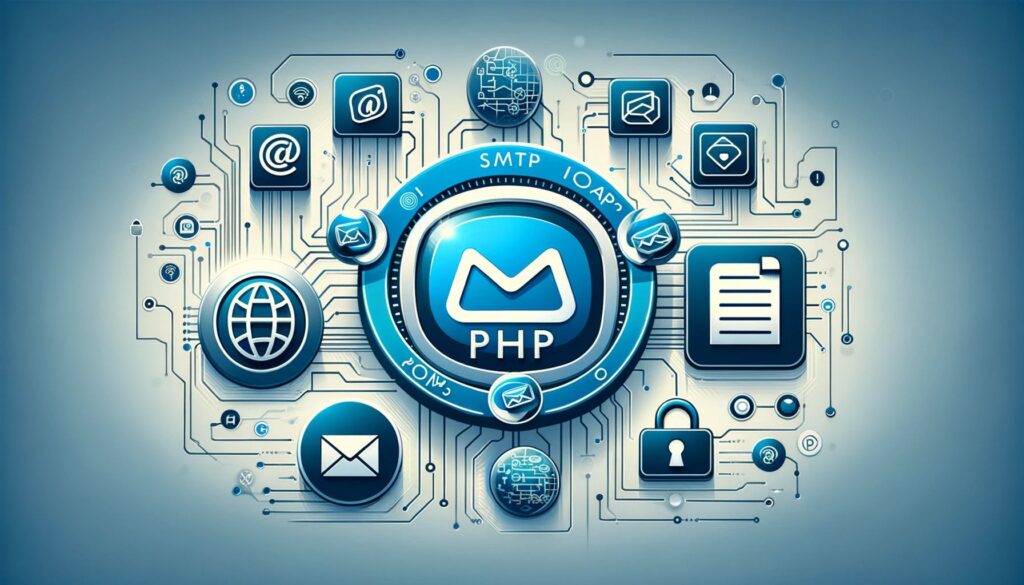
PHP’s adaptability with various email sending protocols (like SMTP, IMAP, and POP3) makes it a versatile choice for developers. While PHP’s native mail() function uses the server’s built-in mail system, more complex scenarios often require the use of SMTP (Simple Mail Transfer Protocol) for a more reliable and secure email delivery. Libraries like PHPMailer and SwiftMailer offer an extended range of functionalities, including SMTP support, which is essential for overcoming limitations of the native mail() function, especially in terms of security and deliverability.
Table: PHP Email Functions and Their Uses
Function | Description | Use Case |
---|---|---|
mail() | Sends an email | Simple text emails without attachments |
PHPMailer | A full-featured email creation and transfer class for PHP | Handling HTML content, attachments, and using SMTP |
SwiftMailer | A component-based library for sending emails | Complex email scenarios, batch sending, and advanced error handling |
Setting Up Your Environment for Email Sending
Configuring your PHP environment for email sending is a foundational step in leveraging PHP’s email capabilities. This setup involves choosing the right tools and libraries to ensure efficient and secure email delivery. Let’s explore how to configure PHP for sending emails and the considerations for selecting an appropriate PHP mail library.
Configuring PHP for Email Sending
The basic requirement for sending emails through PHP is a working mail transfer agent (MTA) on the server. This could be Sendmail, Postfix, or any other MTA that PHP can interact with. For most Linux servers, Sendmail comes pre-installed and PHP is configured to use it by default. However, for Windows servers or more customized setups, additional configuration might be necessary.
Here’s a simple example of sending an email using PHP’s mail()
function:
<?php
$to = '[email protected]';
$subject = 'Test Email';
$message = 'Hello, this is a test email from PHP!';
$headers = 'From: [email protected]';
if(mail($to, $subject, $message, $headers)) {
echo "Email sent successfully!";
} else {
echo "Email sending failed.";
}
?>
This script sends a basic email. However, it’s important to note that the mail()
function has limitations, especially in terms of security and advanced features like attachments and HTML content.
Choosing the Right PHP Mail Library
For more advanced email functionalities, it’s recommended to use a dedicated PHP mail library. Two popular choices are PHPMailer and SwiftMailer. These libraries provide a more robust and flexible way of sending emails, with support for SMTP, HTML emails, attachments, and better error handling.
PHPMailer is widely used due to its ease of use and comprehensive feature set. It supports sending emails via SMTP, which is more reliable and secure compared to PHP’s mail()
function. Here’s a basic example of sending an email using PHPMailer:
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\Exception;
require 'path/to/PHPMailer/src/Exception.php';
require 'path/to/PHPMailer/src/PHPMailer.php';
require 'path/to/PHPMailer/src/SMTP.php';
$mail = new PHPMailer(true);
try {
$mail->SMTPDebug = 2;
$mail->isSMTP();
$mail->Host = 'smtp.example.com';
$mail->SMTPAuth = true;
$mail->Username = '[email protected]';
$mail->Password = 'password';
$mail->SMTPSecure = 'tls';
$mail->Port = 587;
$mail->setFrom('[email protected]', 'Mailer');
$mail->addAddress('[email protected]', 'Joe User');
$mail->Subject = 'Here is the subject';
$mail->Body = 'This is the HTML message body <b>in bold!</b>';
$mail->send();
echo 'Message has been sent';
} catch (Exception $e) {
echo 'Message could not be sent. Mailer Error: ', $mail->ErrorInfo;
}
This code snippet demonstrates sending an HTML email using PHPMailer with SMTP. It includes error handling to catch any issues that might occur during the process.
By setting up your PHP environment correctly and choosing the right library, you can effectively manage email sending in your PHP applications. This setup not only enhances the functionality of your applications but also ensures a higher level of reliability and security in your email communications.
Crafting the Perfect Email with PHP
Creating an engaging and well-formatted email is crucial for effective communication. PHP offers the flexibility to craft both plain text and HTML emails. Let’s look at how to compose and format these types of emails using PHP.
Composing and Formatting Email Content
When sending emails, the content can either be in plain text or HTML. Plain text emails are straightforward and ensure compatibility with all email clients, but they lack styling and rich media capabilities. HTML emails, on the other hand, allow for a more visually appealing and engaging format.
Here’s an example of sending a plain text email using PHP:
<?php
$to = '[email protected]';
$subject = 'Simple Text Email';
$message = "Hello,\nThis is a simple text email.\nRegards,\nYour Website";
$headers = 'From: [email protected]';
mail($to, $subject, $message, $headers);
?>
For HTML emails, you need to set the Content-type
header to text/html
. This tells the email client that the email contains HTML content. Here’s an example:
<?php
$to = '[email protected]';
$subject = 'HTML Email';
$message = '<html><body>';
$message .= '<h1 style="color:#f40;">Hi there!</h1>';
$message .= '<p style="color:#080;font-size:18px;">This is an HTML email.</p>';
$message .= '</body></html>';
$headers = "MIME-Version: 1.0" . "\r\n";
$headers .= "Content-type:text/html;charset=UTF-8" . "\r\n";
$headers .= 'From: [email protected]' . "\r\n";
mail($to, $subject, $message, $headers);
?>
Handling HTML and Plain Text Emails
While HTML emails are more visually appealing, it’s important to cater to all users, including those who prefer or can only receive plain text emails. A good practice is to send emails in multi-part format, containing both HTML and plain text versions. This ensures that the email is readable regardless of the client’s capabilities or settings.
PHPMailer simplifies this process. Here’s how you can send a multi-part email with PHPMailer:
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\Exception;
require 'path/to/PHPMailer/src/Exception.php';
require 'path/to/PHPMailer/src/PHPMailer.php';
require 'path/to/PHPMailer/src/SMTP.php';
$mail = new PHPMailer(true);
try {
// Server settings
// ... [SMTP configuration as before] ...
// Recipients
$mail->setFrom('[email protected]', 'Mailer');
$mail->addAddress('[email protected]', 'Joe User');
// Content
$mail->isHTML(true);
$mail->Subject = 'Here is the subject';
$mail->Body = 'This is the HTML message body <b>in bold!</b>';
$mail->AltBody = 'This is the body in plain text for non-HTML mail clients';
$mail->send();
echo 'Message has been sent';
} catch (Exception $e) {
echo 'Message could not be sent. Mailer Error: ', $mail->ErrorInfo;
}
In this example, Body
contains the HTML version of the email, while AltBody
contains the plain text version. PHPMailer automatically handles the multi-part email sending process.
By carefully crafting your email content and considering both HTML and plain text formats, you can ensure that your messages are effectively delivered and presented to all your recipients, regardless of their email client or preferences.
Advanced Email Features in PHP
Expanding beyond basic email sending, PHP offers capabilities to include advanced features like attachments, inline images, and custom headers. These enhancements can significantly improve the functionality and professionalism of your emails.
Adding Attachments in PHP
Attachments are a common requirement for emails, whether it’s for sending documents, images, or other files. PHPMailer makes adding attachments straightforward. Here’s how you can attach a file to an email:
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\Exception;
require 'path/to/PHPMailer/src/Exception.php';
require 'path/to/PHPMailer/src/PHPMailer.php';
require 'path/to/PHPMailer/src/SMTP.php';
$mail = new PHPMailer(true);
try {
// ... [SMTP configuration as before] ...
// Add a recipient
$mail->addAddress('[email protected]');
// Attachments
$mail->addAttachment('/path/to/file.jpg'); // Add attachments
$mail->addAttachment('/path/to/file.pdf', 'new.pdf'); // Optional name
// Content
$mail->isHTML(true); // Set email format to HTML
$mail->Subject = 'Here is a subject';
$mail->Body = 'This is the HTML message body <b>in bold!</b>';
$mail->send();
echo 'Message has been sent with attachments';
} catch (Exception $e) {
echo 'Message could not be sent. Mailer Error: ', $mail->ErrorInfo;
}
Managing Email Headers and MIME Types
Custom headers and MIME types are essential for more complex email functionalities, like specifying content type, character set, and handling different parts of the email body. PHP allows you to set these headers manually, giving you control over how your email is processed and displayed by the recipient’s email client.
Here’s an example of setting custom headers in PHP:
$headers = "MIME-Version: 1.0" . "\r\n";
$headers .= "Content-type:text/html;charset=UTF-8" . "\r\n";
$headers .= 'From: [email protected]' . "\r\n";
$headers .= 'Cc: [email protected]' . "\r\n";
$headers .= 'Bcc: [email protected]' . "\r\n";
mail($to, $subject, $message, $headers);
Adding Inline Images
Inline images can make your HTML emails more engaging. Unlike attachments, inline images are displayed directly in the email content. PHPMailer simplifies embedding images inline. Here’s how you can do it:
$mail = new PHPMailer(true);
try {
// ... [SMTP configuration as before] ...
// Content
$mail->isHTML(true);
$mail->Subject = 'Email with Inline Image';
$mail->Body = 'This is the HTML message body with an inline image: <img src="cid:logo">';
// Embedding image
$mail->addEmbeddedImage('path/to/image.jpg', 'logo');
$mail->send();
echo 'Message has been sent with inline image';
} catch (Exception $e) {
echo 'Message could not be sent. Mailer Error: ', $mail->ErrorInfo;
}
In this code, addEmbeddedImage
is used to embed the image, and cid:logo
is used in the HTML body to reference the image.
By utilizing these advanced features, you can create more dynamic and functional emails, enhancing the overall experience for your recipients. Whether it’s through adding attachments, customizing headers, or embedding images, PHP provides the tools necessary to elevate your email communications.
Ensuring Email Deliverability
Ensuring that your emails actually reach the inbox of your recipients is a critical aspect of email handling in PHP. This involves understanding and implementing best practices to avoid spam filters and configuring email authentication methods like SPF and DKIM.
Tips to Avoid Spam Filters
Spam filters can block or redirect your emails to the spam folder, significantly affecting your communication. Here are some tips to improve the deliverability of your emails:
- Use a Reputable SMTP Service: Sending emails through a recognized SMTP server can improve deliverability as these servers are often trusted by email providers.
- Avoid Spammy Content: Words that are typically associated with spam should be avoided in both the subject line and the body of the email.
- Keep a Clean Mailing List: Regularly update your mailing list to remove invalid or unresponsive email addresses.
- Consistent Sending Patterns: Sudden spikes in email volume can trigger spam filters. It’s better to maintain a consistent email sending pattern.
Here’s an example of setting up PHPMailer with a reputable SMTP service:
$mail = new PHPMailer(true);
try {
// Server settings
$mail->isSMTP();
$mail->Host = 'smtp.example.com'; // Specify main and backup SMTP servers
$mail->SMTPAuth = true;
$mail->Username = '[email protected]';
$mail->Password = 'your_password';
$mail->SMTPSecure = 'tls';
$mail->Port = 587;
// ... [Rest of the email sending code] ...
} catch (Exception $e) {
echo 'Message could not be sent. Mailer Error: ', $mail->ErrorInfo;
}
Understanding SPF and DKIM Records
SPF (Sender Policy Framework) and DKIM (DomainKeys Identified Mail) are email authentication methods that help prevent email spoofing and improve deliverability.
- SPF: This is a DNS text entry that shows a list of servers that are allowed to send email for your domain. It helps in preventing others from sending emails on behalf of your domain.
- DKIM: This involves attaching a digital signature to your emails. This signature is verified against a public cryptographic key in your domain’s DNS records.
Configuring SPF and DKIM can be a bit technical, as it involves DNS management. Here’s a basic overview of how to set up these records:
- SPF Record Setup: Add a TXT record to your DNS settings. The value might look something like this:
v=spf1 include:_spf.example.com ~all
. - DKIM Record Setup: Generate a DKIM key pair, and add a TXT record for the DKIM public key in your DNS. The value will be a long string of characters, representing the public key.
Automating Email Tasks with PHP
Automation in email handling can significantly enhance the efficiency and effectiveness of your web application. PHP provides the capability to automate various email-related tasks, such as scheduling emails, setting up autoresponders, and managing email lists for bulk sending.
Scheduling Emails with PHP
Scheduling emails to be sent at a specific time can be crucial for various applications like reminders, follow-ups, or timed marketing campaigns. PHP does not have a built-in way to schedule emails to be sent later, but you can achieve this functionality by combining PHP with cron jobs (on Linux servers) or scheduled tasks (on Windows servers).
Here’s a basic approach to scheduling emails in PHP:
- Write a PHP Script to Send Email: Create a PHP script that contains the logic to send your email.
- Set Up a Cron Job or Scheduled Task: Schedule this script to run at your desired time. For example, a cron job can be set up using the following command in the crontab file:
0 9 * * * /usr/bin/php /path/to/your/email_script.php
- This cron job will run the
email_script.php
file every day at 9:00 AM.
Setting Up Autoresponders
Autoresponders are automated emails sent in response to certain actions or triggers, such as a new user registration or a customer inquiry. Here’s a simple example of setting up a basic autoresponder in PHP:
if(isset($_POST['email'])) {
$to = $_POST['email'];
$subject = "Thank you for contacting us!";
$message = "Hello, thank you for reaching out. We will get back to you shortly.";
$headers = 'From: [email protected]';
mail($to, $subject, $message, $headers);
}
This script sends a thank you email whenever it is triggered, typically after a user submits a form with their email address.
Managing Email Lists for Bulk Sending
Sending emails to a list of recipients is a common requirement for newsletters or announcements. PHP can handle bulk email sending, but it’s important to manage this carefully to avoid being flagged as spam.
Here’s a basic example of sending an email to multiple recipients using PHPMailer:
$mail = new PHPMailer(true);
try {
// ... [SMTP configuration as before] ...
$recipients = ['[email protected]', '[email protected]', '[email protected]'];
foreach ($recipients as $email) {
$mail->addAddress($email);
}
$mail->isHTML(true);
$mail->Subject = 'Newsletter';
$mail->Body = 'This is the content of your newsletter.';
$mail->send();
echo 'Newsletter sent to all recipients';
} catch (Exception $e) {
echo 'Message could not be sent. Mailer Error: ', $mail->ErrorInfo;
}
In this script, emails are sent to a list of recipients in a loop. It’s important to handle such operations with care to ensure server performance and email deliverability are not adversely affected.
By automating these email tasks, you can greatly improve the functionality and user experience of your PHP-based web application, ensuring timely and effective communication with your users.
Troubleshooting Common PHP Email Issues
When working with email sending in PHP, you might encounter various issues that can hinder the process. Understanding how to troubleshoot these common problems is essential for maintaining smooth email operations. Let’s look at some typical email sending errors in PHP and how to address them.
Debugging Email Sending Errors
One of the most common issues with sending emails in PHP is not receiving the emails at all. This could be due to several reasons, such as server configuration issues, incorrect email settings, or emails being marked as spam.
To debug this, follow these steps:
- Check Email Logs: Most servers keep logs of all email activities. These logs can provide valuable information about any errors or issues.
- Test with Simple mail() Function: Simplify your script to use the basic
mail()
function to see if the issue is with your script or the server setup. - Verify Email Headers: Incorrect headers can cause emails to be rejected or marked as spam.
- Use Error Reporting in PHPMailer: If you’re using PHPMailer, enable error reporting to get detailed error messages. Here’s how you can do it:
$mail = new PHPMailer(true);
try {
// ... [Your email sending code] ...
} catch (Exception $e) {
echo 'Message could not be sent. Mailer Error: ', $mail->ErrorInfo;
}
Best Practices for Logging and Monitoring
Implementing logging and monitoring for your email sending functionality is a good practice. This can help you track the emails sent, monitor for failures, and understand the overall performance of your email system.
Here’s a basic way to implement logging in your PHP email script:
function sendEmail($to, $subject, $message, $headers) {
if(mail($to, $subject, $message, $headers)) {
file_put_contents('email_log.txt', "Email sent to: $to \n", FILE_APPEND);
} else {
file_put_contents('email_log.txt', "Failed to send email to: $to \n", FILE_APPEND);
}
}
// Example usage
sendEmail('[email protected]', 'Test Subject', 'This is a test email.', 'From: [email protected]');
In this example, every attempt to send an email is logged into a file called email_log.txt
. This log file will include both successful sends and failures, which can be invaluable for troubleshooting.
By understanding how to troubleshoot common issues and implementing effective logging and monitoring strategies, you can ensure that your PHP email functionality operates reliably and efficiently, providing a better experience for both the developer and the end user.
Conclusion
In summary, mastering email handling and sending with PHP is a valuable skill for any web developer. Starting from setting up the environment for email sending, we explored crafting both plain text and HTML emails, and advanced features like attachments and inline images. We also covered essential practices for ensuring email deliverability, including avoiding spam filters and setting up SPF and DKIM records. Automation of email tasks, such as scheduling and managing bulk emails, enhances the functionality of your PHP applications. Finally, understanding how to troubleshoot common email issues and implementing logging and monitoring ensures reliable email communication. This comprehensive guide provides the tools and knowledge needed to effectively manage email operations in PHP, making your web applications more dynamic and user-friendly.