Introduction to CSS Variables and Custom Properties
The evolution of web design and development has been marked by continuous innovation and the quest for greater efficiency and flexibility. In this realm, CSS (Cascading Style Sheets) has undergone significant transformation since its inception. One of the most notable advancements in recent years is the introduction of CSS variables and custom properties. These features represent a paradigm shift in how developers approach styling in web design, offering a level of dynamism and maintainability that was previously challenging to achieve.
What are CSS Variables?
CSS variables, also known as custom properties, are essentially entities that store values which can be reused throughout a stylesheet. They are easily identifiable by their double hyphen (–) prefix, for example, –main-color or –primary-font-size. These variables are declared within a CSS selector and can be applied globally or locally within the stylesheet.
Here is a basic example to illustrate the concept:
:root {
--main-color: #3498db;
--font-size: 16px;
}
In this snippet, –main-color is set to a blue color value, and –font-size is defined as 16 pixels. These variables can then be utilized anywhere in the stylesheet, providing a centralized control point for these values.
The Role of CSS Variables in Modern Web Design
The introduction of CSS variables marks a significant leap in the capabilities of CSS. Prior to their existence, achieving similar functionality required less efficient methods, such as SASS variables or extensive class definitions. CSS variables streamline these processes by allowing values to be stored and manipulated directly within the stylesheet.
One of the key benefits of CSS variables is their scope. Variables declared in the :root selector are globally accessible across the entire stylesheet, whereas those declared within specific selectors are only available within that scope. This scoping provides a powerful tool for developers to manage styles in a more structured and maintainable way.
Another advantage is the ease of integration with JavaScript, enabling dynamic updates to CSS values. This capability is essential for responsive design and interactive elements, such as changing themes or styles based on user interactions or environmental conditions.
Transforming Frontend Development
CSS variables and custom properties have revolutionized frontend development by introducing more efficient and manageable ways to handle styles. They facilitate the creation of dynamic themes, allow for scoped styling, and greatly improve code organization. By incorporating CSS variables into projects, developers can achieve flexible and responsive designs more effortlessly.
Defining and Implementing CSS Variables
After establishing the pivotal role of CSS variables in modern web design, it’s crucial to understand how to define and implement them. This section focuses on the practical aspects of using CSS variables to create efficient, maintainable, and scalable stylesheets.
Defining CSS Variables
CSS variables are defined by using the — prefix followed by a custom name and are typically declared within the :root selector for global accessibility. However, they can also be defined within specific selectors for localized use.
Here’s a simple example demonstrating the definition of CSS variables:
:root {
--main-color: #3498db;
--secondary-color: #2ecc71;
--font-size: 16px;
}
In this example, we’ve defined three variables: –main-color, –secondary-color, and –font-size. These variables are now accessible throughout the entire stylesheet.
Implementing CSS Variables in Styles
Once defined, CSS variables can be applied to any CSS property by using the var() function. This function allows you to insert the value of a variable into your styles.
body {
background-color: var(--main-color);
font-size: var(--font-size);
}
button {
background-color: var(--secondary-color);
}
In these styles, var(–main-color) and var(–secondary-color) are used to set the background colors for the body and button elements, respectively, while var(–font-size) sets the font size for the body.
Localizing CSS Variables
While global variables are powerful, CSS also allows for defining variables within specific selectors. These localized variables override global ones within their scope, enabling more granular control over styles.
.container {
--main-color: #e74c3c; /* Redefining main color for .container scope */
}
.container p {
color: var(--main-color); /* This will use the red color */
}
In this snippet, –main-color is redefined within the .container class, affecting all elements within this container.
Advantages of Using CSS Variables
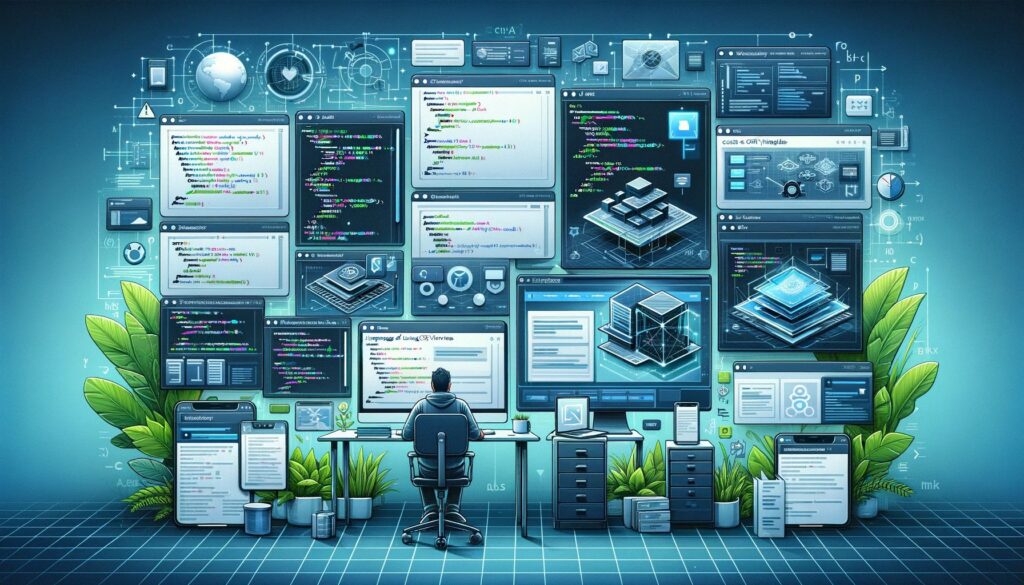
The implementation of CSS variables not only revolutionizes the way stylesheets are written but also brings a host of benefits to web development. These advantages contribute to more efficient, maintainable, and dynamic web design.
1. Enhanced Code Maintainability and Reusability
One of the primary benefits of CSS variables is the significant improvement in code maintainability. By defining a variable once and reusing it throughout the stylesheet, any changes made to the variable are automatically propagated wherever the variable is used. This feature drastically reduces the need for find-and-replace operations, minimizing the risk of errors and inconsistencies.
Consider the following example:
:root {
--main-font-size: 16px;
}
p {
font-size: var(--main-font-size);
}
In this scenario, changing –main-font-size in the :root will automatically update the font size for all paragraphs, illustrating the ease of maintaining and updating styles.
2. Dynamic Theming Capabilities
CSS variables empower developers to create dynamic themes. This is particularly useful for scenarios like theme switching (e.g., light and dark modes) or customizing themes based on user preferences. By manipulating CSS variables with JavaScript, developers can dynamically alter the appearance of a website without reloading the page.
Example of dynamic theme switching:
const root = document.documentElement;
root.style.setProperty('--main-color', 'darkblue');
This JavaScript code snippet demonstrates how a website’s main color can be dynamically changed, facilitating real-time theme customization.
Scoped Styling for Specific Components
Another significant advantage is the scoping of variables. CSS variables can be defined globally in the :root or locally within specific selectors. This scoping provides flexibility in styling individual components or sections of a webpage without affecting the global styles.
Example of scoped variables:
.banner {
--banner-text-color: white;
}
.banner p {
color: var(--banner-text-color);
}
In this example, the –banner-text-color is scoped to the .banner class, ensuring that only text within the banner is affected by this variable.
Real-world Example: Creating a Dynamic Button
To demonstrate the practical application of CSS variables, let’s create a dynamic button that showcases their power and flexibility. This example will provide insights into how CSS variables can be used to streamline the design process and enhance interactivity.
Step 1: Defining CSS Variables for the Button
First, we define the CSS variables for our button. These variables will control various aspects of the button’s appearance, such as its background color, text color, and hover effects.
:root {
--button-bg-color: #3498db;
--button-text-color: #ffffff;
--button-hover-bg-color: #2980b9;
}
In this snippet, we have defined three variables: the background color (–button-bg-color), the text color (–button-text-color), and the hover background color (–button-hover-bg-color) for the button.
Step 2: Styling the Button
Next, we use these variables to style the button. The var() function is used to insert the values of the variables into the button’s CSS properties.
.button {
background-color: var(--button-bg-color);
color: var(--button-text-color);
padding: 10px 20px;
border: none;
border-radius: 4px;
cursor: pointer;
transition: background-color 0.3s ease;
}
Here, the button is styled with the defined variables, ensuring that the look of the button is easily maintainable and can be changed by simply altering the variables.
Step 3: Adding Interactive Effects
To demonstrate the dynamic nature of CSS variables, we add a hover effect that changes the button’s background color.
.button:hover {
background-color: var(--button-hover-bg-color);
}
When the user hovers over the button, the background color transitions to the color defined in –button-hover-bg-color.
Advanced Techniques and Tips
As you become proficient in working with CSS variables, you can explore advanced techniques and best practices that will further enhance your web design and development projects. This section delves into some of these techniques, providing valuable insights into how CSS variables can be used effectively.
- Using CSS Variables in Responsive Designs
CSS variables are particularly useful in creating responsive designs. By defining variables for values like font sizes, margins, and padding, you can easily adjust these properties for different screen sizes. This eliminates the need for writing media queries for every style change, simplifying your CSS and making it more maintainable.
Consider the following example:
:root {
--font-size: 16px;
}
p {
font-size: var(--font-size);
}
@media (max-width: 768px) {
:root {
--font-size: 14px; /* Adjust font size for smaller screens */
}
}
In this example, the –font-size variable is adjusted for smaller screens using a media query, ensuring a responsive design without duplicating styles.
- Concatenating and Transforming Units with calc()
CSS variables can be used in conjunction with the calc() function to concatenate and transform units dynamically. This is particularly valuable for properties like width, height, and margin, where you may want to maintain proportions based on a variable.
:root {
--button-width: 200px;
--button-height: 50px;
}
.button {
width: var(--button-width);
height: calc(var(--button-height) + 10px); /* Adding 10px to the height */
}
Here, the –button-height variable is used within the calc() function to adjust the height of the button while maintaining its proportions.
- Local vs. Global Scoping of Variables
Understanding when to use local or global variables is crucial. Global variables declared in the :root selector are accessible throughout the entire stylesheet, making them suitable for widely used values like colors and fonts. On the other hand, local variables defined within specific selectors are scoped to those elements, providing isolation and preventing unintended style changes. - Preventing Overuse and Complexity
While CSS variables offer flexibility, it’s essential to use them judiciously. Overusing variables can lead to complexity in your stylesheets, making them harder to maintain. Reserve variables for values that are reused significantly or require dynamic changes. - Browser Compatibility and Performance Considerations
While CSS variables are well-supported in modern browsers, it’s essential to be aware of potential limitations. Some older browsers may not fully support CSS variables, so consider fallback styles when necessary. Additionally, extensive use of variables, especially with complex calculations, can impact performance, so test your styles on various devices and browsers.
Incorporating these advanced techniques and best practices into your CSS variable usage will enable you to create more efficient, maintainable, and responsive web designs. CSS variables offer a powerful toolset for frontend developers, and mastering these techniques will elevate your web development projects to new heights.
JavaScript Integration with CSS Variables
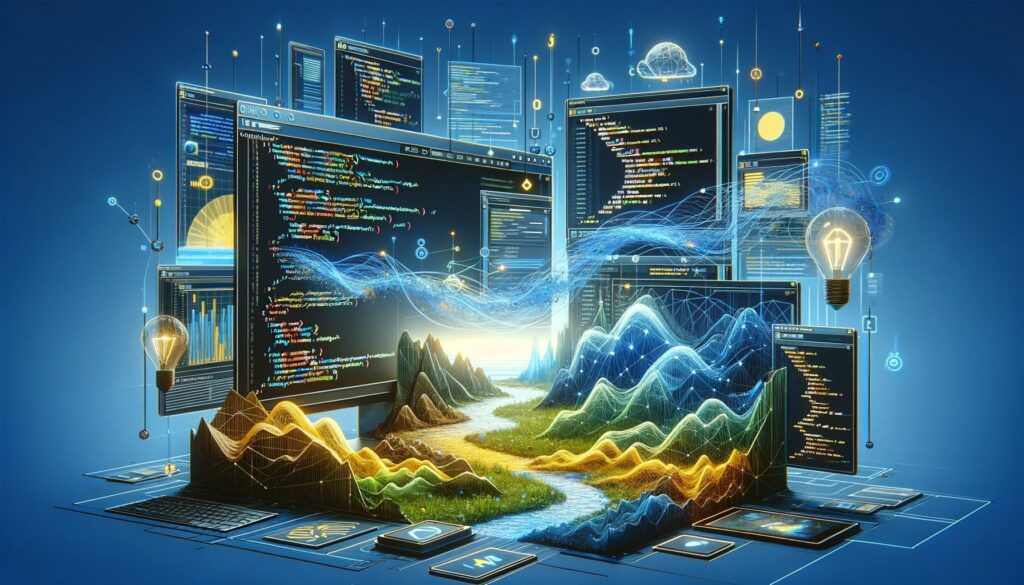
Integrating JavaScript with CSS variables opens up a world of possibilities for creating dynamic and interactive web interfaces. In this section, we will explore how JavaScript can be used to retrieve and update CSS variables dynamically, enabling real-time changes to styles and enhancing user experiences.
Retrieving CSS Variables with JavaScript
To interact with CSS variables in JavaScript, you can use the getComputedStyle method to retrieve the computed value of a variable. This allows you to access the current value of a CSS variable and use it in your JavaScript code.
Here’s an example of how to retrieve a CSS variable using JavaScript:
const root = document.documentElement;
const mainColor = getComputedStyle(root).getPropertyValue('--main-color');
console.log(mainColor); // Outputs the value of --main-color
In this code snippet, we access the –main-color variable and store its value in the mainColor variable for further manipulation.
Updating CSS Variables with JavaScript
Updating CSS variables with JavaScript enables dynamic changes to styles based on user interactions or other events. You can use the setProperty method to modify the value of a CSS variable.
const root = document.documentElement;
root.style.setProperty('--main-color', 'red'); // Change --main-color to red
In this example, we change the value of –main-color to ‘red’, which will affect all elements that use this variable in their styles.
Practical Example: Implementing a Dark Mode Feature
A common use case for JavaScript integration with CSS variables is implementing a dark mode feature. By toggling CSS variables, you can switch between light and dark themes easily. Here’s an example of how to achieve this:
const toggleDarkMode = () => {
const root = document.documentElement;
const currentMode = getComputedStyle(root).getPropertyValue('--mode');
if (currentMode === 'light') {
root.style.setProperty('--main-bg-color', 'black');
root.style.setProperty('--main-text-color', 'white');
root.style.setProperty('--mode', 'dark');
} else {
root.style.setProperty('--main-bg-color', 'white');
root.style.setProperty('--main-text-color', 'black');
root.style.setProperty('--mode', 'light');
}
};
// Attach the toggleDarkMode function to a button or user interaction
In this example, we define CSS variables for background color, text color, and mode. The toggleDarkMode function toggles between light and dark modes by changing the values of these variables. This provides a seamless and interactive way to switch themes on a website.
Benefits of JavaScript Integration
Integrating JavaScript with CSS variables offers several benefits, including:
- Real-time interactivity: Users can change styles on the fly, enhancing the user experience.
- Theme customization: Users can choose between different themes or modes, such as light and dark modes.
- Dynamic updates: Elements can change styles based on user interactions, device conditions, or other events.
- Improved accessibility: Users with specific preferences or accessibility needs can customize the website’s appearance.
By mastering JavaScript integration with CSS variables, you can create web interfaces that are not only visually appealing but also highly interactive and user-friendly.
Conclusion
In this article, we’ve uncovered the transformative power of CSS variables and custom properties in modern web design. CSS variables offer numerous advantages, including improved code maintainability, dynamic theming capabilities, and scoped styling. By integrating JavaScript with CSS variables, you can create dynamic and interactive web interfaces, such as implementing dark mode or real-time theme customization. These features enhance user experiences and accessibility. As you explore CSS variables, remember to follow best practices, such as scoping variables appropriately and considering browser compatibility and performance. Incorporating CSS variables into your web development toolkit opens up new possibilities for efficient, maintainable, and user-friendly designs. Embrace this technology to stay at the forefront of web design innovation.