Linux shell scripting is an incredibly powerful tool in the arsenal of any system administrator, developer, or IT professional. At its core, shell scripting involves writing scripts, which are small programs, to automate a wide range of tasks in a Linux environment. These scripts are executed in the Linux shell, a command-line interface that interprets and executes commands given by the user or a script.
What is a Linux Shell?
The Linux shell is more than just a command prompt. It’s an environment where users interact with the operating system by typing commands. The shell interprets these commands and communicates with the Linux kernel, the core part of the operating system. There are various types of shells available, such as the Bourne-Again Shell (Bash), Korn Shell (Ksh), C Shell (Csh), and others. Among these, Bash is the most widely used and is the default on many Linux distributions.
The Role of Shell Scripting
Shell scripting plays a critical role in automating repetitive tasks, thus saving time and reducing the likelihood of human error. It’s used for a variety of purposes, from simple file manipulations to complex system management tasks. Scripts can automate the backup of data, monitor system performance, configure software, and much more.
Why is Shell Scripting Important?
- Efficiency: Automation of tasks ensures efficiency and consistency, especially for repetitive tasks.
- Cost-Effective: It reduces the need for manual intervention, thereby saving on labor costs.
- Flexibility: Scripts can be modified and repurposed for different tasks, providing great flexibility.
- Scalability: Shell scripts can easily be scaled up to handle larger tasks and systems.
- Powerful Toolset: The shell provides a range of tools and utilities that can be combined in scripts for powerful functionalities.
Understanding the Basics
Before diving into scripting, it’s essential to understand the basics of the Linux command line. This includes familiarity with common commands like ls (to list directory contents), cp (to copy files), mv (to move or rename files), and grep (to search text). A strong foundation in these basics is crucial for writing effective scripts.
Getting Started with Shell Script Basics
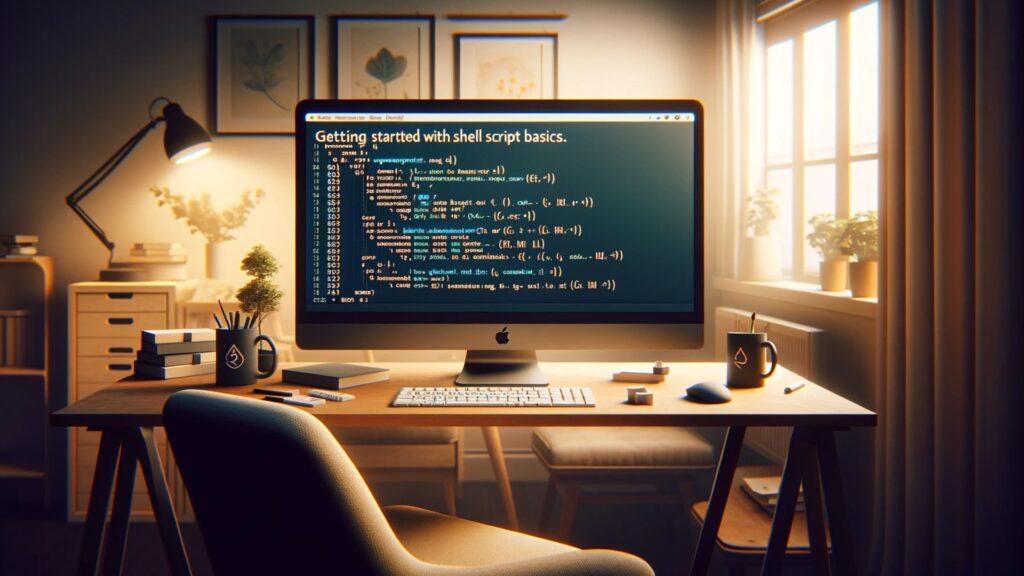
Understanding the Shell Environment
The shell environment in Linux is where users execute commands and scripts. It’s a powerful interface that interprets user inputs and communicates with the operating system’s kernel. When you start writing shell scripts, it’s important to choose the right shell. For most purposes, Bash (Bourne Again SHell) is a great choice due to its widespread availability and extensive feature set.
Basic Scripting Syntax and Commands
A shell script is a text file containing a sequence of commands. To start writing a script, open a text editor and begin with the ‘shebang’ line, which tells the system which interpreter to use. For Bash scripts, this line is:
#!/bin/bash
Creating Your First Script
Let’s create a simple script that outputs “Hello, World!” This example demonstrates basic scripting syntax:
1. Open a text editor and write the following lines:
#!/bin/bash
echo "Hello, World!"
2. Save the file as hello_world.sh.
3. Make the script executable with the command:
chmod +x hello_world.sh
4. Run the script:
./hello_world.sh
This script uses the echo command, which prints text to the terminal.
Variables in Shell Scripting
Variables are essential in scripting. They store data that can be used and manipulated throughout the script. Here’s an example of how to use variables:
#!/bin/bash
greeting="Welcome to Linux Shell Scripting"
echo $greeting
Control Structures: Conditional Statements and Loops
Control structures allow you to make decisions in your scripts or perform actions repeatedly.
If Statements:
#!/bin/bash
number=10
if [ $number -eq 10 ]; then
echo "The number is 10"
else
echo "The number is not 10"
fi
For Loops:
#!/bin/bash
for i in {1..5}; do
echo "Iteration $i"
done
Reading User Input
You can also prompt the user for input using the read command. Here’s an example:
#!/bin/bash
echo "Enter your name:"
read name
echo "Hello, $name!"
Shell Script Arguments
Arguments can be passed to a script from the command line. They are accessed inside the script as $1, $2, etc. Here’s a quick example:
#!/bin/bash
echo "First argument: $1"
echo "Second argument: $2"
Run this script with two arguments like ./script.sh arg1 arg2.
Automating Daily Tasks
Automating daily tasks with shell scripts not only saves time but also ensures accuracy and consistency in the execution of these tasks. Here, we’ll explore some common automation scenarios.
Basic File Management
One of the most common uses of shell scripts is for managing files and directories. Here’s a simple script to organize files by their extension:
#!/bin/bash
for file in *; do
if [ -f "$file" ]; then
ext=${file##*.}
mkdir -p "$ext"
mv "$file" "$ext"
fi
done
This script moves each file in the current directory into a subdirectory named after its file extension.
Automated Backup Script
Regular backups are crucial. Below is a basic script to compress and backup a directory:
#!/bin/bash
backup_dir="/path/to/directory"
backup_file="backup_$(date +%Y%m%d).tar.gz"
tar -czf $backup_file $backup_dir
echo "Backup of $backup_dir completed successfully."
Replace /path/to/directory with the path of the directory you want to back up. This script creates a compressed archive of the directory with a timestamp.
Monitoring Disk Usage
It’s often necessary to monitor disk usage to prevent running out of space. Here’s a script that checks disk usage and sends an alert if it exceeds a certain threshold:
#!/bin/bash
threshold=90
usage=$(df -h | grep '/dev/sda1' | awk '{print $5}' | sed 's/%//g')
if [ $usage -gt $threshold ]; then
echo "Warning: Disk usage is above $threshold%!"
fi
This script checks the disk usage of /dev/sda1. You should replace /dev/sda1 with the relevant disk partition.
Scheduling Tasks with Cron
To automate the execution of scripts, you can use Cron, a time-based job scheduler in Unix-like systems. Here’s how to schedule the disk usage script to run daily:
1. Open the crontab file:
crontab -e
2. Add the following line to schedule the script to run every day at 5 AM:
0 5 * * * /path/to/disk_usage_script.sh
Replace /path/to/disk_usage_script.sh with the path to your script.
Advanced Scripting Techniques
As you become more comfortable with basic shell scripting, you can start exploring advanced techniques that offer more power and flexibility.
Conditional Statements and Loops
Beyond simple if statements and for loops, shell scripting allows for more complex conditional and iterative operations.
Using Case Statements:
Case statements provide a more efficient way to handle multiple conditions. Here’s an example:
#!/bin/bash
read -p "Enter your choice (yes/no): " choice
case $choice in
yes|YES|y|Y)
echo "You chose yes."
;;
no|NO|n|N)
echo "You chose no."
;;
*)
echo "Invalid choice."
;;
esac
While Loops:
While loops are useful for performing an action until a certain condition is met. Here’s an example:
#!/bin/bash
count=1
while [ $count -le 5 ]; do
echo "Iteration $count"
((count++))
done
Functions and Modular Scripting
Functions in shell scripts help you modularize and reuse code. Here’s a simple function example:
#!/bin/bash
greet() {
echo "Hello, $1!"
}
greet "World"
This script defines a greet function that takes one argument and prints a greeting message.
Working with Arrays
Bash also supports arrays, which can be useful for handling lists of data. Here’s an example:
#!/bin/bash
fruits=("Apple" "Banana" "Cherry")
for fruit in "${fruits[@]}"; do
echo "Fruit: $fruit"
done
Advanced File Operations
Scripts can perform more complex file operations, like searching for files based on criteria or processing text files. Here’s a script that finds and lists all JPEG files larger than 1MB in the current directory:
#!/bin/bash
find . -type f -name '*.jpg' -size +1M
Combining Commands with Pipes
Pipes (|) allow you to use the output of one command as the input to another. This is a powerful feature for creating complex workflows. Here’s an example that lists the ten most frequently used commands:
#!/bin/bash
history | awk '{print $2}' | sort | uniq -c | sort -nr | head -n 10
These advanced techniques are essential for writing robust, efficient, and reusable scripts. They enable you to handle complex tasks and process data in sophisticated ways.
Error Handling and Debugging Scripts
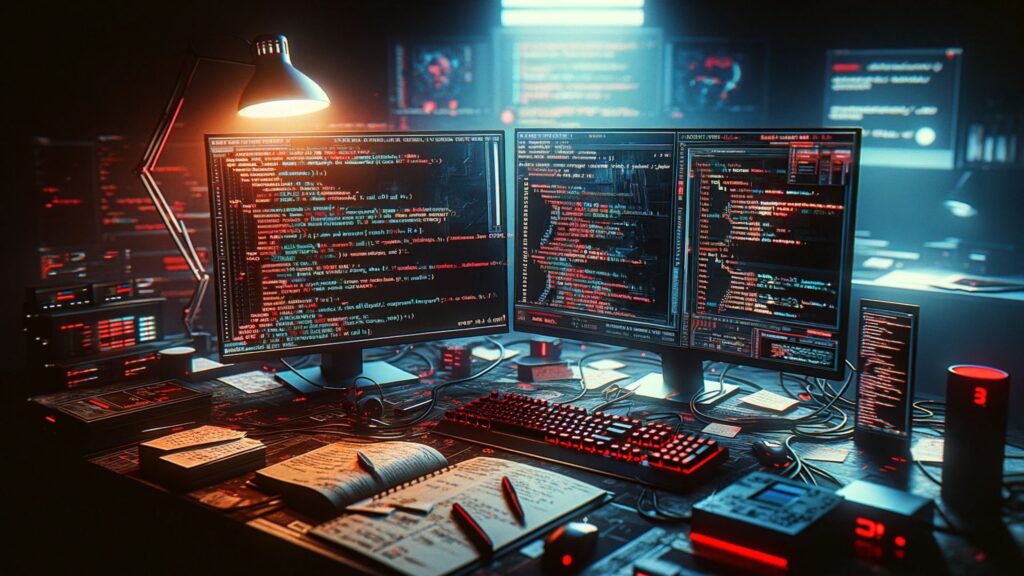
Writing scripts that handle errors gracefully and are easy to debug is vital for maintaining script reliability and ease of use.
Common Scripting Errors and Solutions
In scripting, common errors include syntax errors, command not found, permission issues, and logic errors. Here’s how you can address some of these:
Syntax Errors:
Syntax errors occur when the script contains invalid commands or command structures. A good practice is to run your script through a shell linter or checker, like shellcheck.
For example, running shellcheck myscript.sh can help identify syntax issues.
Command Not Found:
This error usually means the script is trying to execute a command that is not installed or is not in the PATH. Ensure all commands used in your script are available and properly referenced.
Permission Issues:
Scripts might fail to execute due to insufficient permissions. Make sure the script file is executable (chmod +x script.sh) and that it has the necessary access to all required files and directories.
Debugging Tools and Techniques
Debugging shell scripts can be challenging, but Bash provides some tools to make this easier.
Using set -x:
You can use set -x in your script to print each command and its arguments as they are executed. This is extremely useful for tracking the flow of execution and variables.
Example:
#!/bin/bash
set -x
echo "Starting the script"
mkdir new_directory
set +x
echo "Script completed"
Using trap for Error Handling:
The trap command allows you to catch signals and execute code when they occur. This is useful for cleaning up temporary files or rolling back changes if the script exits unexpectedly.
Example:
#!/bin/bash
trap 'echo "An error occurred. Exiting..."; exit 1' ERR
echo "This is a script with error handling"
false # This command will fail
echo "This line will not be reached"
The trap command here is set to catch any error (signal ERR) and execute the given echo statement.
Testing and Validation
Testing your script in a controlled environment before deploying it to production is crucial. Make sure to test with different inputs and scenarios to cover as many use cases as possible.
Optimizing Scripts for Performance
Performance optimization in shell scripting involves writing scripts that are not only functionally correct but also efficient in terms of resource usage and execution time.
Best Practices for Efficient Scripting
1. Avoid Using Unnecessary Commands:
Minimize the use of external commands within loops. Each command spawns a new process, which can be resource-intensive.
For example, consider this inefficient script:
# Inefficient due to repeated calls to an external command
for i in {1..100}; do
echo $i | grep "5"
done
A more efficient approach would be:
# More efficient as it uses built-in Bash features
for i in {1..100}; do
if [[ $i == *5* ]]; then
echo $i
fi
done
2. Use Built-In String Manipulation:
Whenever possible, use Bash’s built-in string manipulation features instead of external commands like sed or awk.
For instance, instead of:
echo $var | sed 's/foo/bar/'
Use:
${var//foo/bar}
3. Leverage Arrays and Associative Arrays:
Arrays can be more efficient for handling lists of data.
# Using an array
files=("file1" "file2" "file3")
for file in "${files[@]}"; do
echo "Processing $file"
done
Performance Improvement
Let’s consider a script that processes log files. An inefficient version might look like this:
#!/bin/bash
# Inefficient script for processing log files
for log in /var/log/*.log; do
grep "error" $log | awk '{print $5}' >> errors.txt
done
An optimized version could be:
#!/bin/bash
# Optimized script for processing log files
for log in /var/log/*.log; do
while read -r line; do
if [[ $line == *"error"* ]]; then
echo "${line}" | awk '{print $5}' >> errors.txt
fi
done < $log
done
This optimized script reduces the number of times grep and awk are called by using Bash’s built-in pattern matching.
Security Considerations in Shell Scripting
Ensuring the security of your shell scripts is as important as their functionality and performance. Security in scripting involves writing code that is not only resistant to external attacks but also does not unintentionally expose vulnerabilities.
Security Best Practices
1. Avoiding Command Injection:
Command injection is a critical security issue where an attacker can execute arbitrary commands on your system. Always validate and sanitize user inputs.
Instead of:
# Vulnerable to command injection
read -p "Enter filename: " filename
cat $filename
Use:
# Safer approach
read -p "Enter filename: " filename
cat "$(basename "$filename")"
This approach ensures that only the filename part is used, not any appended commands.
2. Handling Sensitive Data:
Be cautious when dealing with sensitive data. Avoid hardcoding passwords or sensitive information in scripts.
For handling passwords, use secure methods like read with the -s option:
read -s -p "Enter Password: " password
3. File Permissions and Ownership:
Set appropriate file permissions for scripts, especially those that perform critical system operations.
chmod 700 your_script.sh
This command sets the script to be readable, writable, and executable only by the file owner.
4. Using set -e for Error Handling:
The set -e option makes the script exit immediately if any command exits with a non-zero status, which can prevent unintended consequences.
# Enable strict error handling
set -e
Protecting Scripts from Common Vulnerabilities
- Path Injection:
Avoid using untrusted input for file paths or commands. Validate input paths and use absolute paths for executing commands. - Denial of Service (DoS):
Be aware of resource-intensive operations within scripts that could potentially be exploited to cause a DoS condition. - Privilege Escalation:
Be cautious with scripts that require elevated privileges. Ensure that they cannot be exploited to gain unauthorized access.
Integrating Shell Scripts with Other Tools
The power of Linux shell scripting is greatly enhanced when combined with other tools and applications. This integration can automate complex tasks, streamline processes, and bridge different systems.
Combining Shell Scripts with Software Applications
1. Integration with Git:
Shell scripts can automate various Git operations. For instance, a script to automate the process of pushing changes to a repository:
#!/bin/bash
git add .
git commit -m "Automated commit"
git push
This simple script adds all changes, commits them with a standard message, and then pushes to the remote repository.
2. Integrating with Cloud Services:
Scripts can interact with cloud service APIs. For example, using curl to interact with a cloud storage API:
#!/bin/bash
curl -X POST -H "Authorization: Bearer $API_TOKEN" -F "file=@/path/to/file" https://api.cloudservice.com/upload
This script uploads a file to a cloud storage service using its API.
Examples of Powerful Integrations
1. Data Processing with Shell and Python:
Combining shell scripts with Python for data processing can be very effective. For example, you could use a shell script to gather data files and then invoke a Python script for analysis.
#!/bin/bash
for file in /data/*.csv; do
python process_data.py "$file"
done
Here, the shell script loops through CSV files and passes them to a Python script for processing.
2. Automated System Updates:
A script can be created to automate system updates, integrating with package managers:
#!/bin/bash
apt-get update && apt-get upgrade -y
This script updates the package lists and upgrades all the packages on a Debian-based system.
Conclusion
Linux shell scripting is a versatile and powerful tool pivotal in automating tasks across various domains. From system administration to data processing, it enhances efficiency, reduces manual errors, and simplifies complex workflows. As technology evolves, the role of shell scripting remains significant, adapting to new challenges and integrating seamlessly with emerging tools. Its continued relevance in the future of computing is assured, making it an essential skill for professionals in the digital era.