In the rapidly evolving landscape of web development, Java and RESTful Web Services have emerged as two pivotal elements. These technologies, individually powerful and in combination, offer a robust foundation for building modern, scalable, and efficient web applications. As 2024 unfolds, it’s crucial to understand their significance, synergies, and the innovations they bring to the tech world.
Java: The Evergreen Backbone of Programming
Java, a language celebrated for its platform independence, robustness, and security, continues to be a cornerstone in the programming world. Java’s versatility allows it to adapt to new trends while maintaining its relevance and efficiency. Its object-oriented nature makes it ideal for a wide range of applications, from enterprise-level software to mobile apps.
In recent years, Java has seen significant updates, with the introduction of features like lambda expressions, stream API, and the modular system. These enhancements not only make Java more powerful but also improve the developer experience by simplifying coding and increasing readability. The latest versions, such as Java 17, have further cemented its place as a reliable and future-ready programming language.
RESTful Web Services: The Gateway to Seamless Interactions
RESTful Web Services play a critical role in the current era of interconnected applications. Representational State Transfer (REST) is an architectural style that defines a set of constraints for creating web services. RESTful services are characterized by their statelessness and ability to leverage existing protocols, primarily HTTP, making them an ideal choice for building scalable and flexible web APIs.
The RESTful approach simplifies the communication between client and server by using standard HTTP methods (GET, POST, PUT, DELETE). This simplicity, coupled with the ability to return data in various formats like JSON and XML, makes RESTful services widely used in building modern web applications, especially in microservices architectures.
The Convergence: Java and RESTful Web Services
The convergence of Java and RESTful Web Services is a testament to the evolution of web development practices. Java frameworks like Spring Boot, Jersey, and JAX-RS have streamlined the process of creating RESTful APIs, offering annotations and libraries to simplify routing, serialization, and deserialization. This integration has led to the creation of agile, responsive, and maintainable web services.
Moreover, Java’s strong support for concurrency, security, and database connectivity makes it a go-to choice for backend development in RESTful services. The language’s ability to handle complex business logic and interact with various database systems aligns perfectly with the needs of modern web services that prioritize data integrity and performance.
Navigating the Trends
As we delve deeper into 2024, it’s clear that both Java and RESTful Web Services will continue to shape the future of web development. Their combined strengths offer a powerful toolkit for developers to build sophisticated and scalable web solutions. The upcoming sections of this article will explore the latest trends, technologies, and practices that are influencing Java and RESTful Web Services, providing insights into how they will continue to evolve and dominate the web development arena.
The Rise of Containers in Java Applications
The integration of containers in Java application development represents a significant shift in how developers deploy and manage applications. Containers, such as those provided by Docker, offer a lightweight, efficient, and consistent environment for running applications, making them an ideal choice for Java developers seeking to improve scalability and efficiency.
Understanding Containers in Java Context
Containers encapsulate an application along with its dependencies, libraries, and other necessary components into a single package. This encapsulation ensures that the application runs uniformly and reliably across different computing environments. In the context of Java, containers offer several advantages:
- Isolation: Each container runs in isolation, ensuring that the application’s environment remains consistent, regardless of where it is deployed.
- Scalability: Containers can be easily scaled up or down, making them suitable for applications that experience variable loads.
- Efficiency: Containers share the host system’s kernel, unlike virtual machines that require a full operating system, leading to better utilization of system resources.
Practical Implementation: Containerizing a Java Application
To demonstrate the process of containerizing a Java application, consider a simple RESTful web service. The service is built using Spring Boot, a popular framework for creating stand-alone, production-grade Spring-based applications.
First, create a basic Spring Boot application. You can use Spring Initializr (start.spring.io) for this purpose, choosing Maven as the build tool and adding dependencies for Spring Web.
Here’s a simple REST controller for our application:
@RestController
public class HelloWorldController {
@GetMapping("/hello")
public String helloWorld() {
return "Hello, World!";
}
}
This controller exposes a GET endpoint /hello
that returns a simple “Hello, World!” message.
Next, create a Dockerfile in the root of the project. This file contains instructions for building the container image.
FROM openjdk:11
COPY target/hello-world-0.0.1-SNAPSHOT.jar hello-world.jar
ENTRYPOINT ["java","-jar","/hello-world.jar"]
This Dockerfile performs the following steps:
- Uses the
openjdk:11
image as the base. - Copies the compiled JAR file of the application into the container.
- Sets the container to run the application when it starts.
Compile the application using Maven:
mvn clean package
Build the Docker image:
docker build -t hello-world-java .
Run the container:
docker run -p 8080:8080 hello-world-java
The application will be accessible on localhost:8080/hello
.
Embracing Container Orchestration
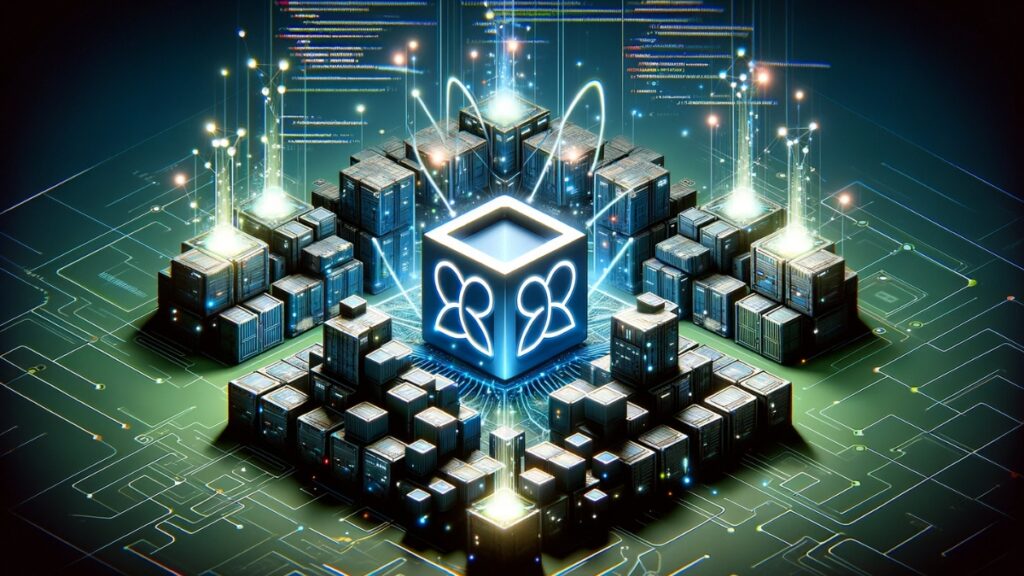
As the use of containers in Java development grows, the importance of container orchestration tools like Kubernetes becomes evident. These tools manage the lifecycle of containers, ensuring that applications are deployed, scaled, and managed efficiently in production environments.
Kubernetes, for instance, allows Java applications to be deployed in a highly available and scalable manner. It handles tasks such as load balancing, self-healing (restarting failed containers), and automated rollouts and rollbacks, making the management of containerized Java applications more straightforward and robust.
Embracing Reactive Programming in Java
Reactive programming in Java represents a paradigm shift towards more responsive and scalable applications. It is particularly relevant in scenarios where applications need to handle a large number of concurrent requests efficiently. The core concept revolves around building non-blocking, asynchronous applications that can better utilize resources and provide faster response times.
Reactive Programming Concepts in Java
In reactive programming, the focus is on data flows and the propagation of change. This means that when a data value changes, all the computations that depend on this data are updated in real time. Java provides several libraries and frameworks to facilitate reactive programming, such as Project Reactor, RxJava, and others.
Here’s a basic example using RxJava, a popular library for composing asynchronous and event-based programs using observable sequences:
First, include the RxJava dependency in your Maven pom.xml
file:
<dependency>
<groupId>io.reactivex.rxjava3</groupId>
<artifactId>rxjava</artifactId>
<version>3.x.x</version>
</dependency>
Then, you can create a simple observable and subscriber:
import io.reactivex.rxjava3.core.Observable;
public class ReactiveExample {
public static void main(String[] args) {
Observable<String> observable = Observable.just("Hello", "World");
observable.subscribe(
item -> System.out.println(item), // onNext
error -> error.printStackTrace(), // onError
() -> System.out.println("Completed!") // onComplete
);
}
}
This code snippet demonstrates creating an Observable
that emits “Hello” and “World”, and a subscriber that prints each emitted item. The subscribe
method has three parts: onNext
for handling each item, onError
for handling any errors, and onComplete
for handling the completion of the stream.
Advantages of Reactive Programming in Java
- Improved Performance and Scalability: By adopting non-blocking and asynchronous execution, Java applications can handle more tasks concurrently, optimizing CPU and memory usage.
- Responsive and Resilient Systems: Reactive programming allows Java applications to be more responsive and maintain stability even under heavy load conditions.
- Better Resource Management: Asynchronous execution means that threads are not blocked waiting for I/O operations, leading to better utilization of system resources.
Kotlin’s Impact on Java Development
The rise of Kotlin, a modern programming language developed by JetBrains, is significantly influencing Java development. Kotlin’s interoperability with Java allows developers to seamlessly integrate Kotlin code into existing Java projects, offering several benefits such as concise syntax and enhanced features for functional programming.
Kotlin and Java: Working Together
Kotlin runs on the Java Virtual Machine (JVM) and can be used alongside Java in the same project. This compatibility means developers can gradually adopt Kotlin in their Java applications, enjoying its modern features without a complete rewrite.
Here’s an example of how Kotlin simplifies code compared to Java. Consider a simple Java class for a Customer:
public class Customer {
private String name;
private int age;
public Customer(String name, int age) {
this.name = name;
this.age = age;
}
// Getters and Setters
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
The equivalent Kotlin class is much more concise:
data class Customer(var name: String, var age: Int)
In Kotlin, the data class
automatically generates getter and setter methods, as well as other useful methods like toString()
, equals()
, and hashCode()
.
Kotlin’s Functional Programming Features
Kotlin also supports functional programming, a paradigm that allows developers to write more expressive and concise code. For instance, Kotlin provides a range of built-in higher-order functions like map
, filter
, and reduce
which can be used to perform operations on collections more succinctly.
Here’s an example of using functional programming in Kotlin to process a list of numbers:
val numbers = listOf(1, 2, 3, 4, 5)
val doubled = numbers.map { it * 2 }
println(doubled) // Output: [2, 4, 6, 8, 10]
This snippet demonstrates how easily a list can be transformed in Kotlin, showcasing the language’s power in writing clear and concise code.
The Future of Kotlin and Java
Kotlin’s growing influence in the Java ecosystem is undeniable. Its compatibility with Java, along with its modern features, makes it an attractive option for new projects and for enhancing existing Java applications. Kotlin is not only prevalent in server-side development but also dominates Android development, further solidifying its position in the Java world.
Java’s Role in AI and Machine Learning
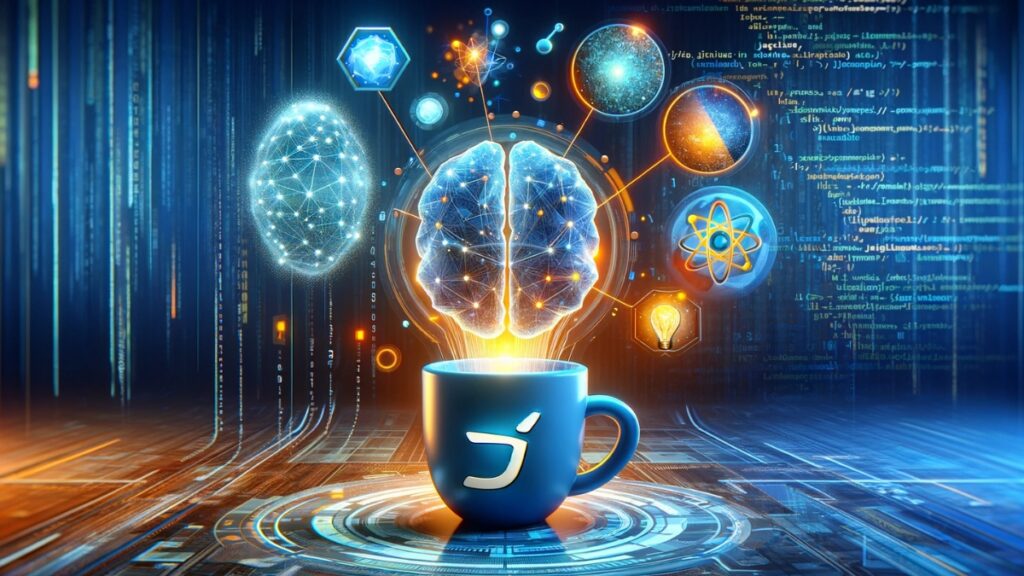
Java’s application in the realm of Artificial Intelligence (AI) and Machine Learning (ML) is expanding rapidly. Its platform independence, robustness, and ease of use make it a preferred choice for developing sophisticated AI algorithms and machine learning models.
Leveraging Java Libraries for Machine Learning
Several Java libraries facilitate AI and ML development. Libraries like Deeplearning4j, Weka, and Apache Mahout provide pre-built algorithms and tools that simplify the implementation of complex models in Java. For instance, Deeplearning4j allows building, training, and deploying neural networks.
Here’s a basic example using Deeplearning4j to create a simple neural network:
First, include the Deeplearning4j dependency in your Maven pom.xml
file:
<dependency>
<groupId>org.deeplearning4j</groupId>
<artifactId>deeplearning4j-core</artifactId>
<version>1.x.x</version>
</dependency>
Then, you can create a basic neural network model:
import org.deeplearning4j.nn.conf.NeuralNetConfiguration;
import org.deeplearning4j.nn.conf.layers.DenseLayer;
import org.deeplearning4j.nn.conf.layers.OutputLayer;
import org.deeplearning4j.nn.multilayer.MultiLayerNetwork;
import org.deeplearning4j.nn.weights.WeightInit;
import org.nd4j.linalg.activations.Activation;
import org.nd4j.linalg.learning.config.Adam;
import org.nd4j.linalg.lossfunctions.LossFunctions;
public class SimpleNeuralNet {
public static void main(String[] args) {
var conf = new NeuralNetConfiguration.Builder()
.weightInit(WeightInit.XAVIER)
.updater(new Adam(0.001))
.list()
.layer(new DenseLayer.Builder().nIn(4).nOut(3)
.activation(Activation.RELU)
.build())
.layer(new OutputLayer.Builder(LossFunctions.LossFunction.NEGATIVELOGLIKELIHOOD)
.activation(Activation.SOFTMAX)
.nIn(3).nOut(3).build())
.build();
MultiLayerNetwork model = new MultiLayerNetwork(conf);
model.init();
// Training code would go here
}
}
This code snippet sets up a simple neural network with one hidden layer. The network is configured with Xavier weight initialization, an Adam optimizer, and a softmax output layer using negative log likelihood as the loss function.
Java in Developing AI-driven Solutions
Java’s object-oriented nature and robust ecosystem make it well-suited for building complex AI-driven solutions. Its ability to handle large-scale applications and integrate with databases and web services makes it a strong candidate for enterprise-level AI applications.
In AI development, Java is often used for:
- Natural Language Processing (NLP)
- Data analysis and visualization
- Robotics and automation systems
Java’s strong community support and extensive libraries provide developers with the necessary tools to experiment and innovate in the AI and ML fields.
Innovations in Java: Project Loom and Native Compilation
Java continues to innovate, with Project Loom and native compilation being two key areas of advancement. These technologies address the limitations of traditional Java threading models and offer new ways to build efficient, high-performance applications.
Project Loom: Revolutionizing Concurrency in Java
Project Loom aims to introduce lightweight, user-mode threads (virtual threads) to Java, making concurrency much more accessible and efficient. These virtual threads are managed by the JVM rather than the operating system, enabling the creation of thousands of threads with minimal resource overhead.
Here’s a basic example of using virtual threads in Java, assuming Project Loom is available:
import java.util.concurrent.Executors;
public class VirtualThreadsExample {
public static void main(String[] args) {
var executor = Executors.newVirtualThreadPerTaskExecutor();
for (int i = 0; i < 10; i++) {
int taskId = i;
executor.execute(() -> System.out.println("Task " + taskId + " executed on virtual thread"));
}
executor.close();
}
}
This example creates a new virtual thread for each task, allowing multiple tasks to run concurrently with minimal overhead.
Native Compilation with GraalVM
Native compilation, particularly with GraalVM, enables Java applications to be compiled into native machine code. This approach enhances application startup time and reduces memory footprint.
A simple Java application can be compiled into a native executable using GraalVM’s native-image
tool. First, ensure GraalVM is installed and set up. Then, compile a Java application into a native executable as follows:
native-image -cp myapp.jar MyMainClass
This command will produce a native executable from the Java application myapp.jar
with the main class MyMainClass
.
Balancing Performance and Compatibility
While native compilation offers performance benefits, it also poses challenges, particularly with dynamic features like reflection. Developers need to carefully manage dependencies and understand the limitations of static analysis used in native compilation.
The Evolving Landscape of Java Frameworks
Java frameworks are continuously evolving to embrace the latest trends and technologies in the Java ecosystem. Frameworks like Spring Boot, Quarkus, and others are adapting to changes such as Project Loom and native compilation, ensuring that Java remains a top choice for enterprise application development.
Spring Framework: Adapting to Modern Java
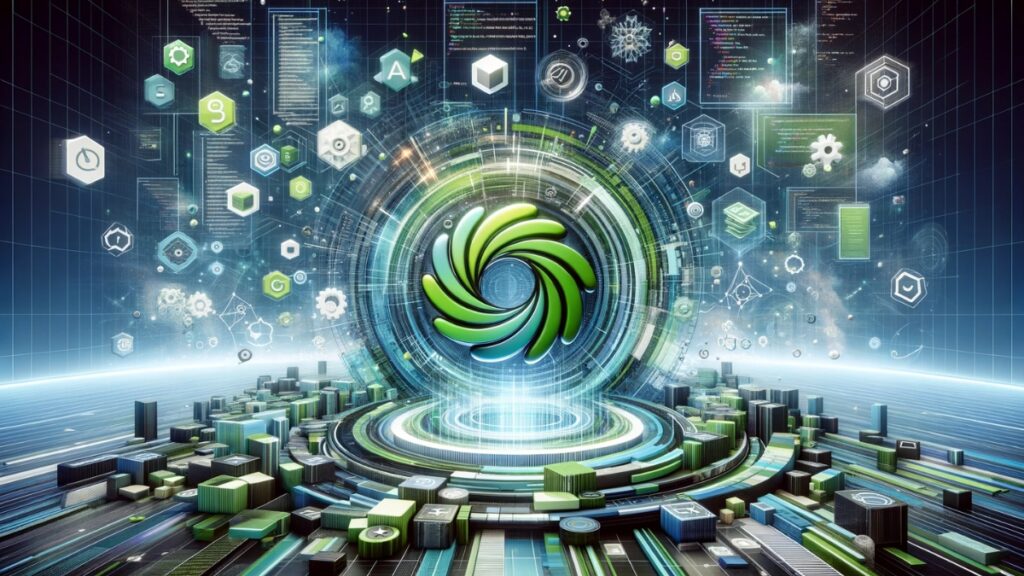
Spring Framework, particularly Spring Boot, has been instrumental in simplifying Java application development. With its extensive set of features and integrations, Spring Boot allows developers to create robust and scalable web applications with minimal boilerplate code.
Here’s a basic Spring Boot application example:
First, create a new Spring Boot project using Spring Initializr or manually with your preferred IDE.
Add the Spring Web dependency in your pom.xml
or build.gradle
file for Maven or Gradle, respectively.
Then, create a simple REST controller:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class SimpleController {
@GetMapping("/greet")
public String greet() {
return "Hello from Spring Boot!";
}
}
This controller defines a /greet
endpoint that returns a greeting message.
Run the application, and the service will be available at http://localhost:8080/greet
.
Quarkus: Java’s Supersonic Subatomic Framework
Quarkus is a modern Java framework designed for building efficient and scalable microservices and serverless applications. It is known for its container-first philosophy, making it a great choice for cloud-native Java applications.
A simple Quarkus application can be started by generating a new project from its website or using Maven:
mvn io.quarkus:quarkus-maven-plugin:create \
-DprojectGroupId=org.example \
-DprojectArtifactId=my-quarkus-app \
-DclassName="org.example.GreetingResource"
Then, add a REST endpoint:
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("/hello")
public class GreetingResource {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String hello() {
return "Hello from Quarkus!";
}
}
Run the application using:
./mvnw compile quarkus:dev
The service will be available at http://localhost:8080/hello
.
Frameworks and Project Loom
Frameworks are beginning to experiment with integrating Project Loom’s virtual threads, promising to simplify concurrency in Java applications further. This integration could lead to more straightforward and efficient handling of parallel processes within applications.
Future Directions: Java in Cloud Computing and Mobile Development
As Java continues to evolve, its role in cloud computing and mobile development is becoming increasingly significant. Java’s robustness, security features, and extensive ecosystem make it well-suited for these domains.
Java in Cloud Computing
Cloud-native development is a growing trend, and Java is adapting to meet these new demands. With cloud computing, applications are designed to take full advantage of cloud environments, which offer scalability, flexibility, and cost-efficiency.
Java’s integration into cloud platforms often involves frameworks like Spring Boot and MicroProfile, which offer tools and libraries specifically designed for building microservices and cloud-native applications.
For example, a Spring Boot application can be easily containerized and deployed to a cloud platform. Here’s how you can create a Docker image for a Spring Boot application:
First, add a Dockerfile to your project:
FROM openjdk:11
ARG JAR_FILE=target/*.jar
COPY ${JAR_FILE} app.jar
ENTRYPOINT ["java","-jar","/app.jar"]
Then, build the Docker image:
docker build -t my-spring-boot-app .
And finally, you can deploy this image to a cloud platform that supports Docker containers.
Java for Mobile Development
Java has been a cornerstone of Android app development since its inception. While Kotlin is now the preferred language for Android, Java remains widely used due to its vast developer base and existing codebases.
Developing an Android app in Java involves using Android Studio and the Android SDK. Here’s a simple example of an Android activity written in Java:
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
TextView textView = findViewById(R.id.text_view);
textView.setText("Hello, Android!");
}
}
This code sets up a basic activity with a TextView
displaying a greeting message.
Java’s Future in Cloud and Mobile
In cloud computing, Java is likely to see increased adoption in serverless architectures and platform-as-a-service (PaaS) solutions, providing more streamlined and efficient deployment models.
In mobile development, while Kotlin gains traction, Java’s large ecosystem and ongoing improvements ensure it remains a viable and popular choice for Android development.
Conclusion: The Future of Java and RESTful Web Services
As we navigate towards the future, Java’s enduring presence in the world of programming is unequivocally affirmed by its continuous evolution and adaptation to emerging trends. The integration of modern concepts like reactive programming, advancements in concurrency with Project Loom, and the move towards native compilation with GraalVM are testaments to Java’s resilience and capacity for innovation. These developments not only bolster Java’s performance and efficiency but also reinforce its relevance in the rapidly changing landscape of technology. Coupled with RESTful Web Services, Java is poised to maintain its stronghold in building versatile, scalable, and efficient web applications. The synergy between Java and RESTful Web Services is set to grow stronger, driven by the convergence of cloud computing, AI, and mobile development. This symbiosis ensures that Java remains a cornerstone in the creation of sophisticated, future-ready web solutions.
Looking ahead, the trajectory for Java and RESTful Web Services is marked by an exciting blend of stability and innovation. The ongoing enhancements in Java frameworks like Spring Boot and Quarkus, tailored for modern architectures, are paving the way for more agile and responsive web services. Java’s role in cloud computing and mobile app development continues to expand, promising new horizons in serverless architectures and cross-platform applications. As Java evolves to meet these new challenges, its integration with RESTful Web Services is expected to unlock unprecedented possibilities, shaping the future of web development in an era dominated by digital transformation and cloud-native technologies. The journey of Java, marked by its adaptability and robustness, is a clear indicator that its influence in the realm of web services will not only persist but thrive in the years to come.