In the realm of software development, performance is a cornerstone that can significantly impact the success and efficiency of applications. This is particularly true for Java, a widely used programming language renowned for its versatility, scalability, and robust feature set. Java’s performance, while generally reliable, can vary greatly depending on how well its applications are tuned. This introductory section delves into the critical importance of performance tuning in Java applications, highlighting the benefits it brings and the challenges it addresses.
Why Performance Tuning Matters in Java
- Enhanced Efficiency and Speed: At the core of performance tuning lies the goal of making Java applications run faster and more efficiently. This includes reducing the time taken to execute operations and improving the overall responsiveness of the application.
- Resource Optimization: Java applications often run in resource-constrained environments, making it crucial to optimize the use of available resources, such as CPU and memory. Effective performance tuning helps in maximizing the utility of these resources, thereby enhancing the application’s capability to handle larger workloads.
- Improved User Experience: In today’s digital age, users expect quick and seamless interactions with applications. Performance tuning ensures that Java applications meet these expectations, thereby enhancing user satisfaction and engagement.
- Scalability and Reliability: As applications grow in complexity and user base, they must scale efficiently. Performance tuning plays a pivotal role in ensuring that Java applications can scale without compromising on stability and reliability.
- Cost-Effectiveness: By optimizing performance, organizations can often avoid the need for additional hardware resources, leading to cost savings. Efficiently tuned applications can do more with less, which is particularly important in cloud-based and serverless computing environments where resource usage directly impacts cost.
Challenges in Java Performance Tuning
Despite its importance, performance tuning in Java is not without its challenges. These include:
- Complexity of the Java Ecosystem: Java’s vast ecosystem, encompassing various libraries, frameworks, and JVM (Java Virtual Machine) implementations, adds complexity to the performance tuning process. Different applications may behave differently under various configurations and environments.
- Balancing Trade-offs: Performance tuning often involves trade-offs. For instance, optimizing for speed might increase memory consumption, and vice versa. Finding the right balance that aligns with the application’s goals is a nuanced task.
- Continuously Evolving Standards: With the Java ecosystem continuously evolving, staying abreast of the latest performance optimization techniques and best practices can be challenging.
- Identifying Bottlenecks: Pinpointing the exact cause of performance issues, such as memory leaks, inefficient code, or database bottlenecks, requires in-depth analysis and understanding of the application and the Java platform.
Understanding JVM Internals and Garbage Collection
The Java Virtual Machine (JVM) is the engine that drives the performance of Java applications. One of its core components is the Garbage Collector (GC), which plays a pivotal role in memory management. To understand performance tuning in Java, it’s crucial to grasp the basics of JVM architecture and how garbage collection works.
The Role of the JVM in Java Performance
The JVM is responsible for executing Java bytecode, converting it into machine code at runtime. This process, known as Just-In-Time (JIT) compilation, is key to optimizing performance. The JVM also manages memory allocation for Java objects and handles their deallocation through garbage collection.
How Garbage Collection Works
Garbage collection in Java is the process of identifying and discarding objects that are no longer needed, freeing up memory resources. The JVM has several garbage collectors, each with its unique approach to managing memory. The choice of garbage collector can significantly impact application performance.
- Serial Garbage Collector: Suitable for applications with a small data set running on single-threaded environments.
- Parallel Garbage Collector (Throughput Collector): Optimized for multi-threaded applications, focusing on maximizing application throughput.
- Concurrent Mark Sweep (CMS) Collector: Designed to minimize application pause times, suitable for interactive applications.
- G1 Garbage Collector: Aims to provide a balance between throughput and pause time, ideal for applications with a large heap.
Tuning Garbage Collection
Tuning the garbage collector involves adjusting various parameters to optimize memory management for specific application needs. For example:
- Setting initial (
-Xms
) and maximum (-Xmx
) heap size: Determines the heap size allocated to the Java application. A larger heap can reduce the frequency of garbage collections but may increase pause times.
java -Xms512m -Xmx4g MyApp
- Selecting the right garbage collector: Use JVM flags like
-XX:+UseG1GC
to select a specific garbage collector, based on application requirements.
java -XX:+UseG1GC MyApp
Monitoring and Analyzing Garbage Collection
Monitoring the behavior of the garbage collector is crucial for tuning. Tools like VisualVM, JConsole, and Java Mission Control provide insights into garbage collection metrics, such as frequency, pause time, and memory reclaimed.
Analyzing Common Performance Issues in Java
Identifying and addressing common performance bottlenecks is essential for optimizing Java applications. These issues can range from inefficient code practices to improper resource management.
Memory Leaks
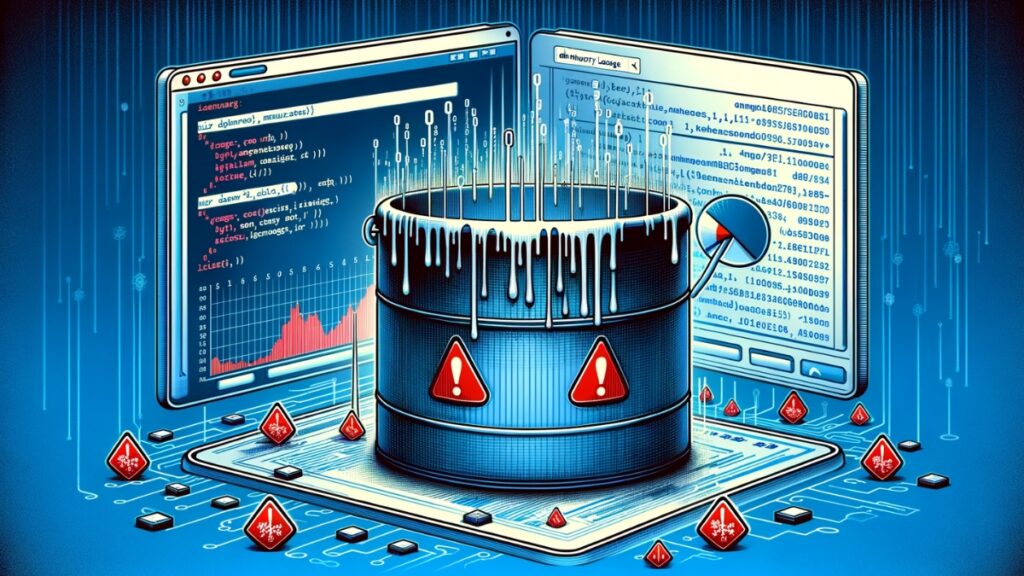
Memory leaks occur when objects are no longer used but are still referenced, preventing the garbage collector from reclaiming their memory. This can lead to OutOfMemoryError
. Tools like Eclipse Memory Analyzer (MAT) are useful for detecting memory leaks.
Example: An example of a potential memory leak is adding objects to a collection and not removing them when they’re no longer needed.
List<Object> leakyList = new ArrayList<>();
public void addToList(Object obj) {
leakyList.add(obj);
// Objects are never removed from leakyList, leading to a potential memory leak
}
CPU Utilization
High CPU usage can indicate inefficient code or concurrency issues. Profiling tools like JProfiler or VisualVM can help identify methods that consume excessive CPU time.
Example: An inefficient way of processing large data sets can lead to high CPU usage.
public int processData(List<Integer> data) {
// Inefficient processing logic here can lead to high CPU usage
return data.stream().mapToInt(Integer::intValue).sum();
}
Thread Contention and Locks
Poorly managed concurrency can lead to thread contention, where multiple threads compete for resources, leading to deadlocks or performance degradation.
Example: Synchronized methods or blocks can cause thread contention if not managed properly.
public synchronized void performTask() {
// Critical section code that may cause thread contention if accessed by multiple threads simultaneously
}
Inefficient Code
Inefficient algorithms or unnecessary object creation can significantly slow down application performance.
Example: Repeated string concatenation in loops should be avoided due to the immutability of strings in Java. Instead, StringBuilder
should be used.
StringBuilder sb = new StringBuilder();
for (int i = 0; i < largeNumber; i++) {
sb.append("some string");
}
Effective Memory Management Strategies
Efficient memory management is a cornerstone of Java application performance. Proper allocation, usage, and management of memory can lead to significant improvements in application responsiveness and scalability.
Heap Size Tuning
The heap is where Java stores objects at runtime. Configuring the heap size correctly is crucial for performance.
- Setting Initial and Maximum Heap Size: Use the
-Xms
and-Xmx
flags to set the initial and maximum heap size. This prevents frequent resizing of the heap, which can be costly.
java -Xms256m -Xmx1024m MyApplication
- Monitoring Heap Usage: Regular monitoring of heap usage can help in identifying the right size for your application, preventing both heap overflow and underutilization.
Object Pooling
Object pooling is a pattern used to manage a set of objects that are expensive to create and destroy. Reusing objects from a pool can significantly improve performance, especially for frequently used objects.
- Implementing Object Pooling: Apache Commons Pool is a library that provides an implementation for object pooling.
// Example of using Apache Commons Pool for object pooling
GenericObjectPool<MyObject> pool = new GenericObjectPool<>(new MyObjectFactory());
Garbage Collection Tuning
Choosing the right garbage collector and tuning its parameters can drastically improve performance, especially for applications with large heaps or specific latency requirements.
- Selecting a Garbage Collector: Use JVM flags to select an appropriate garbage collector based on your application needs.
java -XX:+UseConcMarkSweepGC -Xmx4g MyApplication
Optimizing Code for Better Performance
Writing efficient code is one of the most direct ways to improve the performance of a Java application. Optimizing code involves not just writing faster algorithms, but also adopting practices that minimize resource wastage.
Efficient Data Processing
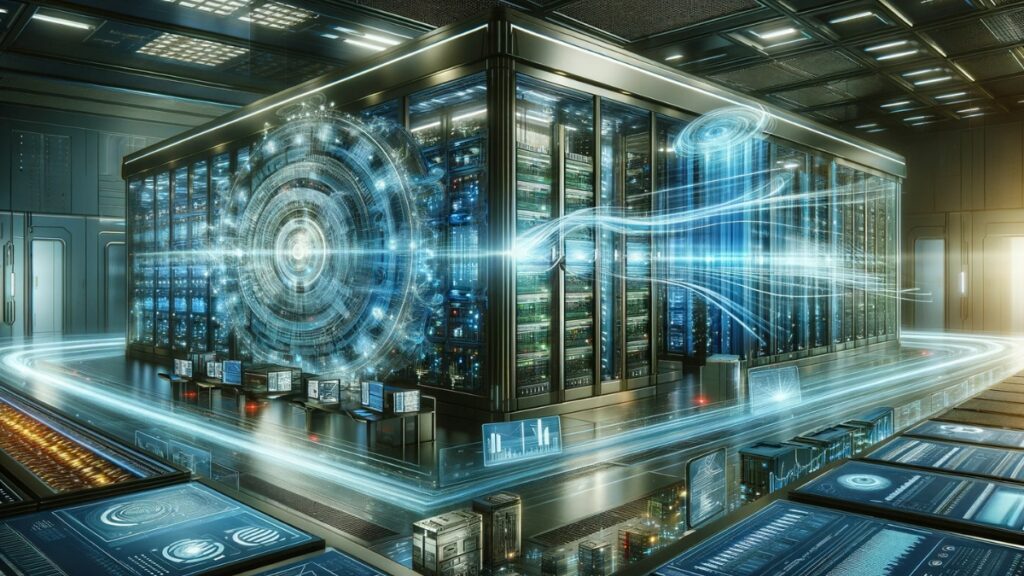
Leveraging Java’s built-in features, like Streams for parallel processing, can lead to significant performance gains, especially when dealing with large data sets.
- Example of Parallel Processing with Streams:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
List<Integer> results = numbers.parallelStream()
.map(this::processData)
.collect(Collectors.toList());
- In this example,
parallelStream()
allows for processing elements in parallel, potentially improving performance over traditional sequential processing.
Minimizing Object Creation
Reducing the frequency of object creation and destruction can lessen the load on the garbage collector and improve performance.
- Example of Using String Builders:
StringBuilder builder = new StringBuilder();
for (int i = 0; i < 100; i++) {
builder.append(i);
}
String result = builder.toString();
This approach is more efficient than concatenating strings in a loop, as it avoids creating multiple intermediate string objects.
Using Efficient Data Structures
Choosing the right data structure for the task can dramatically improve performance, especially in terms of time complexity for operations like searching, inserting, and deleting.
- Example of Choosing an Efficient Data Structure:
Set<Integer> hashSet = new HashSet<>();
hashSet.add(1);
hashSet.add(2);
// HashSet offers faster lookups compared to a list
boolean exists = hashSet.contains(1);
In this case, a HashSet
is used for faster lookups compared to a List
.
Leveraging Concurrency and Parallel Processing
Concurrency and parallel processing are powerful concepts in Java that, when used correctly, can significantly enhance the performance of applications, particularly in handling multiple tasks simultaneously or processing large amounts of data.
Concurrency in Java
Java provides several mechanisms for concurrency, including threads and the java.util.concurrent
package, which offer advanced utilities for concurrency management.
- Example of Using Threads:
Thread thread = new Thread(() -> {
// Task code goes here
});
thread.start();
This simple example demonstrates creating a new thread to perform tasks concurrently with other parts of the program.
- Example of Using
java.util.concurrent
:
ExecutorService executor = Executors.newFixedThreadPool(10);
executor.submit(() -> {
// Task code goes here
});
executor.shutdown();
The ExecutorService
creates a pool of threads and manages their lifecycle, allowing you to focus on task implementation.
Parallel Processing
Parallel processing enables the utilization of multiple cores of the CPU, leading to faster execution of suitable tasks, such as large-scale data processing.
- Example of Parallel Processing in a Collection:
List<String> items = Arrays.asList("apple", "banana", "cherry");
items.parallelStream().forEach(System.out::println);
This code snippet uses a parallel stream to process elements concurrently, leveraging multiple cores for performance gains.
Tuning Database Interactions
Optimizing how a Java application interacts with a database is a crucial aspect of performance tuning, particularly for applications that rely heavily on data retrieval and storage.
Efficient Database Connection Management
Managing database connections efficiently is key to enhancing performance. Connection pooling is a common technique used to reduce the overhead of creating and closing connections.
- Example of Using Connection Pooling:
DataSource dataSource = new HikariDataSource();
Connection connection = dataSource.getConnection();
// Use the connection for database operations
connection.close(); // Returns the connection to the pool, doesn't close it
In this example, HikariDataSource
from the HikariCP library is used for efficient connection pooling.
Optimizing SQL Queries
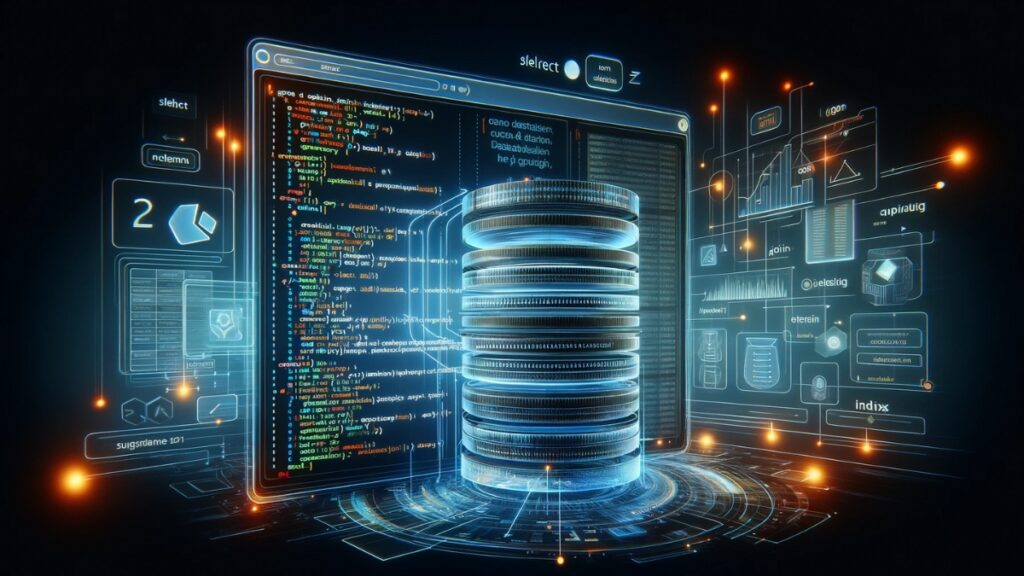
Writing efficient SQL queries is crucial for performance, especially in data-intensive applications. This includes selecting only necessary columns, avoiding complex joins when possible, and using indexes effectively.
- Example of an Optimized SQL Query:
String query = "SELECT id, name FROM users WHERE active = true";
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery(query);
// Process the resultSet
This SQL query is optimized to retrieve only the necessary columns and uses a simple condition for filtering.
Caching Frequently Accessed Data
Caching is an effective strategy to reduce database load by storing frequently accessed data in memory.
- Example of Implementing Caching:
Cache<Long, User> userCache = CacheBuilder.newBuilder()
.maximumSize(1000)
.build();
// Use the cache to store and retrieve User objects
Here, Google’s Guava CacheBuilder
is used to create a simple cache for storing user data.
Advanced Techniques: Profiling and JVM Tuning
For more sophisticated performance tuning, profiling Java applications and fine-tuning the JVM settings are indispensable techniques. They involve analyzing the runtime behavior of applications and adjusting the JVM’s operation to optimize performance.
Profiling Java Applications
Profiling is a process of analyzing an application to identify bottlenecks and performance issues. Java profilers can provide insights into CPU usage, memory consumption, thread activity, and more.
- Using a Profiler:
// Profiling is usually done through tools and not directly in code.
// However, you can initiate a heap dump programmatically, which can be analyzed later.
MBeanServer server = ManagementFactory.getPlatformMBeanServer();
HotSpotDiagnosticMXBean mxBean = ManagementFactory.newPlatformMXBeanProxy(
server, "com.sun.management:type=HotSpotDiagnostic", HotSpotDiagnosticMXBean.class);
mxBean.dumpHeap("heap_dump.hprof", true);
While the above snippet demonstrates how to programmatically trigger a heap dump for analysis, most profiling is done using external tools like JProfiler, YourKit, or VisualVM.
JVM Tuning
JVM tuning involves configuring JVM options to optimize performance, such as memory settings, garbage collection, and JIT compilation behaviors.
- Setting JVM Options:
# Example of setting various JVM options
java -XX:+UseG1GC -XX:MaxGCPauseMillis=200 -Xmx4g -Xms4g MyApplication
n this example, -XX:+UseG1GC
selects the G1 garbage collector, -XX:MaxGCPauseMillis=200
sets a target for maximum GC pause time, and -Xmx4g -Xms4g
configures the initial and maximum heap size.
Selecting and Configuring Garbage Collectors
The choice of the garbage collector (GC) in the JVM can have a significant impact on Java application performance. Each garbage collector has its own set of characteristics and is suited for specific types of applications.
Understanding Different Garbage Collectors
- Serial GC: Good for small applications with low memory footprint. Use
-XX:+UseSerialGC
to enable it. - Parallel GC: Ideal for medium to large applications where throughput is a priority. Enable with
-XX:+UseParallelGC
. - Concurrent Mark Sweep (CMS) GC: Suitable for applications requiring low pause times. Use
-XX:+UseConcMarkSweepGC
. - G1 GC: Designed for large heap sizes with predictable pause times. Enable with
-XX:+UseG1GC
.
Configuring Garbage Collector Settings
Fine-tuning GC settings can optimize performance, especially in terms of memory management and pause times.
- Example of Setting G1 GC with Specific Pause Time Goals:
java -XX:+UseG1GC -XX:MaxGCPauseMillis=100 -Xmx4g MyApplication
This command runs the application with the G1 garbage collector, aiming to keep garbage collection pauses under 100 milliseconds, and sets the maximum heap size to 4 GB.
Conclusion: Continuous Performance Improvement
In the dynamic world of software development, the pursuit of performance optimization in Java applications is an ongoing journey. The strategies and techniques discussed in this guide represent the foundation for achieving superior application efficiency, but they are just the beginning. Continuous performance improvement requires a commitment to vigilance, adaptability, and a proactive approach.
By embracing tools and practices for profiling, JVM tuning, and code optimization, developers can address current performance challenges and lay the groundwork for future scalability. Moreover, the careful selection of garbage collectors, efficient memory management, and smart database interaction practices ensure that Java applications remain responsive and reliable. In the ever-evolving landscape of technology, a dedication to continuous performance enhancement is not just a best practice; it is the key to staying ahead in the competitive world of Java development.