The landscape of software development is continually evolving, adapting to new challenges and opportunities presented by the ever-changing technological environment. At the forefront of this evolution is the integration of Java with microservices architecture, a combination that has revolutionized the way developers and organizations approach complex application development.
Understanding Microservices Architecture
Microservices architecture is a design approach that structures an application as a collection of loosely coupled services. This methodology contrasts sharply with the traditional monolithic architecture, where an application is built as a single, unified unit. In a microservices architecture, each service is self-contained and implements a specific business functionality, operating independently from other services.
Key Characteristics of Microservices:
- Decentralization: Services are developed, deployed, and managed independently.
- Autonomy: Each service is responsible for a specific business function and operates independently.
- Resilience: The architecture is designed to be fault-tolerant, handling failures gracefully.
- Scalability: Services can be scaled horizontally, adding more instances as demand increases.
The Role of Java in Microservices
Java, with its robustness and portability, has become a popular choice for building microservices. The language’s ability to handle complex, enterprise-level applications makes it an ideal fit for the demands of microservices architecture. Java’s platform independence, reliability, and performance, along with a rich ecosystem of frameworks and tools, provide a solid foundation for developing scalable and resilient microservices.
Why Java for Microservices?
- Robustness and Stability: Java’s maturity and established presence in the enterprise space make it a reliable choice.
- Platform Independence: Java applications can run on any platform or operating system without modification.
- Rich Ecosystem: Java offers a plethora of frameworks like Spring Boot, Micronaut, and Quarkus that facilitate microservices development.
The Synergy of Java and Microservices
The synergy between Java and microservices lies in their mutual strengths. Java’s stable and scalable nature complements the modular and flexible design of microservices. This combination allows developers to build applications that are not only robust and efficient but also adaptable to changing business needs. Microservices architecture, with its emphasis on decentralized management and scalability, aligns perfectly with Java’s capabilities, making it a go-to choice for modern application development.
Evolution of Java in Microservices
The journey of Java in the realm of microservices is a tale of evolution and adaptation. Java, one of the most widely used programming languages, has continually evolved to meet the demands of modern software architecture, particularly microservices. This evolution marks a shift from traditional, monolithic application structures to more agile, scalable, and resilient system designs.
Historical Perspective and Java’s Emergence
Java’s journey in software development started as a robust, object-oriented language ideal for building large-scale enterprise applications. Its stability, security features, and cross-platform capabilities made it a preferred choice for many developers and organizations. However, as software development practices shifted towards more modular and scalable approaches, Java too adapted, embracing the principles of microservices.
Recent Trends in Java for Microservices
In recent years, Java has seen significant advancements, especially with the introduction of features like lambda expressions, stream API, and the modular system in Java 9. These features greatly align with the microservices architecture by promoting more readable, concise code and facilitating easier application scaling and maintenance.
For instance, lambda expressions in Java allow for more concise and readable code, which is vital in microservices for ease of maintenance and scalability. Consider a simple example where lambda expressions are used to filter a list of objects:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
In this example, lambda expressions simplify the process of filtering a list, making the code more readable and maintainable – qualities essential in a microservices environment.
Java’s Growing Significance in Microservice Applications
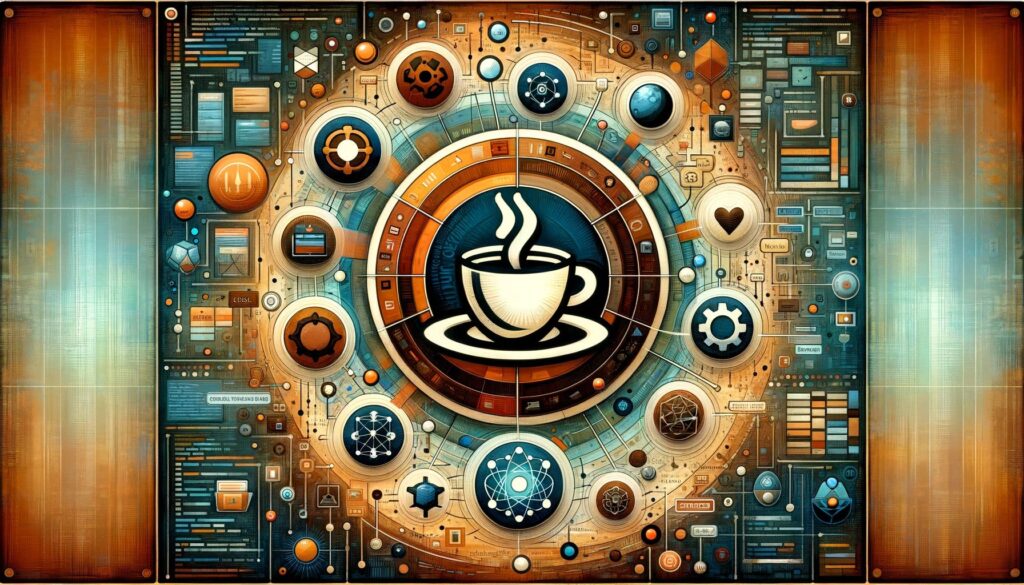
The growing significance of Java in microservices is also due to its extensive ecosystem of frameworks and libraries that simplify microservices development. Frameworks like Spring Boot, Micronaut, and Quarkus provide out-of-the-box support for creating microservices, including features for service discovery, configuration management, and routing, which are essential in a distributed environment.
For example, Spring Boot simplifies the development of microservices through its auto-configuration and standalone approach. A basic Spring Boot application can be set up with minimal configuration, as shown in the following code snippet:
@SpringBootApplication
public class MyMicroserviceApp {
public static void main(String[] args) {
SpringApplication.run(MyMicroserviceApp.class, args);
}
}
This code establishes a straightforward Spring Boot application, showcasing how Java frameworks make it easy to develop microservices.
Benefits of Using Java for Microservices
The adoption of Java in microservices architecture is not coincidental but a result of its numerous benefits that align well with the demands of this architectural style. Let’s explore these advantages and understand how Java facilitates efficient and robust microservices development.
Enhanced Scalability and Fault Tolerance
One of the primary benefits of using Java in microservices is its inherent scalability and fault tolerance. Java’s ability to handle large-scale applications makes it an ideal choice for microservices, which often require scaling individual components based on demand.
For example, in Java, creating a RESTful web service that can be independently scaled is straightforward with frameworks like Spring Boot. Below is a basic example of a REST controller in a Spring Boot application:
@RestController
@RequestMapping("/api")
public class MyRestController {
@GetMapping("/hello")
public ResponseEntity<String> sayHello() {
return ResponseEntity.ok("Hello, World!");
}
}
This simple REST controller can be scaled independently in a microservices architecture, demonstrating Java’s capacity for building scalable services.
Improved Flexibility and Faster Time-to-Market
Java’s flexibility, especially with its rich set of libraries and frameworks, accelerates development cycles, allowing for faster deployment and time-to-market of services. For instance, Java’s Maven or Gradle build tools streamline the build process, handling dependencies efficiently which is crucial in a microservices setup where services may have different dependencies.
Consider the following example of a pom.xml
file in a Maven-based Java project:
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-microservice</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<!-- Spring Boot Dependencies -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Other dependencies -->
</dependencies>
</project>
This Maven configuration showcases the management of dependencies, which is essential for the independent development of microservices.
Robustness and Platform Independence
Java’s platform independence is a significant advantage in microservices, allowing services to run on different environments without changes to the codebase. Java’s virtual machine (JVM) ensures that applications written in Java can run on any platform that supports the JVM, enhancing the portability of services.
For instance, a Java microservice packaged as a JAR file can be executed in various environments, ensuring consistency across development, testing, and production stages.
Challenges and Solutions in Java Microservices
While Java provides a robust platform for microservices, there are challenges that developers may encounter. These challenges, however, come with viable solutions that leverage Java’s capabilities.
Handling Increased Complexity and Startup Times
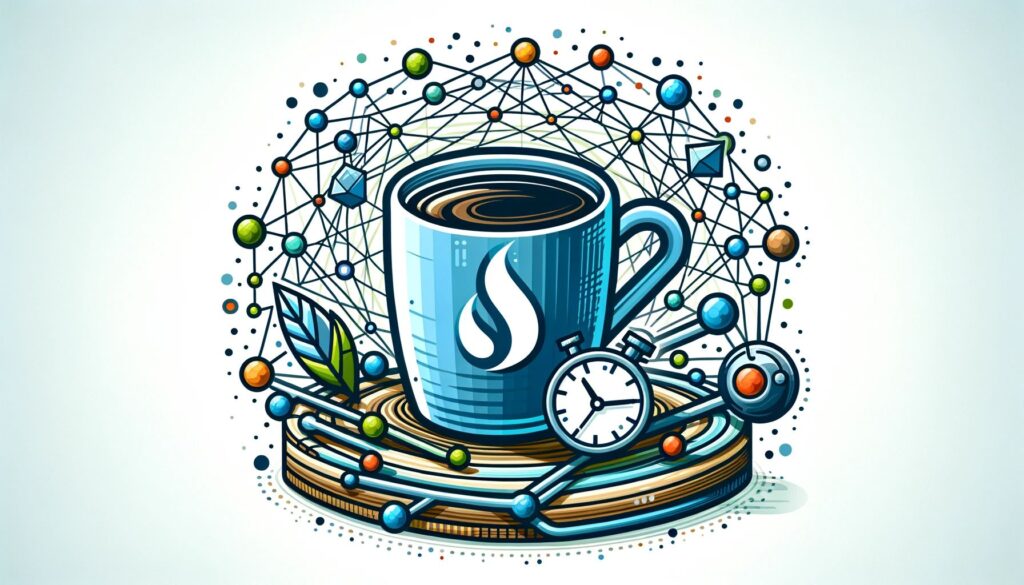
Microservices architecture inherently involves dealing with a complex network of services. Java applications, especially those using Spring Boot, can face longer startup times due to this complexity. To mitigate this, Java developers can optimize configuration and leverage lazy initialization.
For example, Spring Boot provides options to reduce startup time by configuring lazy initialization. This can be done by setting the property in the application.properties
file:
spring.main.lazy-initialization=true
This setting ensures that beans in the Spring context are only created when they are actually needed, reducing the startup time.
Best Practices for Deployment and Management
Deploying and managing a network of Java microservices can be challenging. Many organizations widely adopt containerization, utilizing tools like Docker, to streamline this process. Containerizing Java microservices ensures consistency across different environments and simplifies deployment.
Here is an example Dockerfile
for a typical Java application:
FROM openjdk:11
ADD target/my-microservice-app.jar my-microservice-app.jar
EXPOSE 8080
ENTRYPOINT ["java", "-jar", "my-microservice-app.jar"]
This Dockerfile
creates a Docker image for a Java application, which can then be run as a container, making deployment and scaling more manageable.
Java Frameworks and Tools for Microservices Architecture
The Java ecosystem is rich with frameworks and tools that significantly ease the development, deployment, and management of microservices. These frameworks not only simplify the coding process but also provide robust solutions for common challenges in microservices architecture.
Spring Boot
Spring Boot is one of the most popular frameworks for building microservices in Java. It simplifies the bootstrapping and development of new applications through auto-configuration, an opinionated setup, and a wide range of starter kits.
Here’s a basic example of a Spring Boot application with a REST controller:
@SpringBootApplication
public class MicroserviceApplication {
public static void main(String[] args) {
SpringApplication.run(MicroserviceApplication.class, args);
}
@RestController
class GreetingController {
@GetMapping("/greeting")
public String greet() {
return "Hello from Spring Boot Microservice";
}
}
}
This code demonstrates a simple Spring Boot application with a REST endpoint.
Dropwizard
Dropwizard is another Java framework specifically designed for developing high-performance RESTful web services. It bundles together stable, mature libraries from the Java ecosystem into a simple, light-weight package, making it ideal for microservices.
Below is a snippet to create a simple RESTful service in Dropwizard:
public class HelloWorldApplication extends Application<HelloWorldConfiguration> {
public static void main(String[] args) throws Exception {
new HelloWorldApplication().run(args);
}
@Override
public void run(HelloWorldConfiguration configuration, Environment environment) {
final HelloWorldResource resource = new HelloWorldResource(
configuration.getTemplate(),
configuration.getDefaultName()
);
environment.jersey().register(resource);
}
}
This example sets up a basic application using Dropwizard, highlighting its simplicity and efficiency.
Micronaut
Micronaut is a modern, JVM-based, full-stack framework for building modular, easily testable microservice applications. It is designed for service discovery, distributed tracing, and other microservices patterns.
Example of a Micronaut controller:
@Controller("/hello")
public class HelloController {
@Get("/")
public String index() {
return "Hello from Micronaut!";
}
}
This controller in Micronaut defines a simple endpoint for a microservice.
Design Patterns and Resilience in Java Microservices
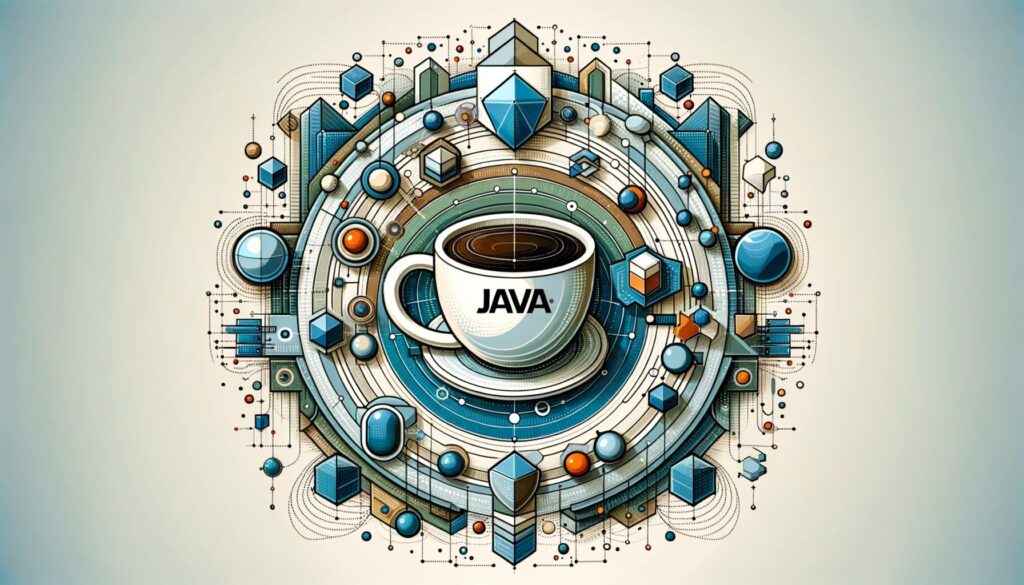
The success of microservices architecture depends not only on the choice of frameworks and tools but also on the effective application of design patterns to ensure the robustness and scalability of the system. Java, with its versatility, allows developers to implement various design patterns that enhance the resilience of microservices.
Key Principles of Microservices Architecture
Before delving into design patterns, it’s crucial to understand the fundamental principles of microservices architecture:
- Decentralization: Develop, deploy, and manage microservices independently of one another. This allows for greater flexibility and faster deployment cycles.
- Autonomy: Each microservice should be responsible for a specific business function and should be able to make decisions independently of other services.
- Resilience: Design microservices to gracefully handle failures, employing techniques such as circuit breakers, retries, and timeouts.
- Scalability: Design microservices to achieve horizontal scalability by adding more service instances as demand increases.
- API-driven: Microservices should communicate with each other through well-defined APIs. This allows for loose coupling between services and enables independent development and deployment.
Design Patterns for Building Resilient Systems
Several design patterns can be applied to microservices architecture to build resilient systems. Let’s explore some of these patterns along with code examples.
Circuit Breaker
We utilize the circuit breaker pattern to manage failures in a microservice. When a service fails, the circuit breaker switches to a fallback mode and returns a default response. This allows the service to continue to operate even when other services are not available.
Here’s an example of using the Netflix Hystrix library to implement a circuit breaker in a Spring Boot application:
@HystrixCommand(fallbackMethod = "fallbackMethod")
public String someMicroserviceCall() {
// Call the microservice here
}
public String fallbackMethod() {
return "Fallback Response";
}
In this code, @HystrixCommand
is used to create a circuit breaker around the someMicroserviceCall()
method.
Retry
We employ the retry pattern to address transient failures in a microservice. When a service fails, we retry the request a specific number of times before giving up. This can help reduce the impact of temporary failures on the overall system.
Here’s an example using Spring Retry to implement retry logic:
@Retryable(maxAttempts = 3, backoff = @Backoff(delay = 1000))
public String someMicroserviceCall() {
// Call the microservice here
}
In this code, the @Retryable
annotation specifies that the someMicroserviceCall()
method should be retried up to 3 times with a 1-second delay between retries.
Timeout
We use the timeout pattern to ensure a service doesn’t become unresponsive. When a service responds too slowly, we terminate the request and provide a default response. This helps prevent a single service from causing a bottleneck in the system.
@HystrixCommand(fallbackMethod = "fallbackMethod", commandProperties = {
@HystrixProperty(name = "execution.isolation.thread.timeoutInMilliseconds", value = "1000")
})
public String someMicroserviceCall() {
// Call the microservice here
}
In this code, the execution.isolation.thread.timeoutInMilliseconds
property is set to 1000 milliseconds, defining a timeout for the microservice call.
Conclusion
In the world of microservices architecture, Java stands as a robust and versatile choice, offering a multitude of benefits that align seamlessly with the demands of modern software development. Its scalability, flexibility, and platform independence make it an ideal language for developing microservices, enabling the construction of highly resilient and efficient systems.
Java’s ecosystem, including frameworks like Spring Boot, Dropwizard, and Micronaut, provides developers with the tools to simplify development, containerization, and orchestration. Moreover, the application of design patterns such as circuit breakers, retries, and timeouts enhances the resilience of Java-based microservices. Scaling, load balancing, and performance monitoring further solidify Java’s position as a top choice for microservices architects. As we look to the future, Java continues to evolve, offering new possibilities for serverless microservices, containerization, and AI integration, ensuring its enduring relevance in the ever-evolving landscape of microservices architecture.