Java is a powerful, high-level programming language that revolutionized the software development landscape. Developed by Sun Microsystems in 1995, Java was initially named Oak before being renamed due to trademark issues. Its design was driven by the principle of “Write Once, Run Anywhere” (WORA), emphasizing portability across different platforms. Java is an object-oriented language, which means it focuses on manipulating and accessing objects, rather than just using functions and procedures.
The History of Java
The development of Java can be traced back to the early 1990s when a team led by James Gosling at Sun Microsystems set out to create a language for digital consumer devices like set-top boxes and televisions. However, the language quickly found its niche in web development, thanks to its versatility and robustness. Over the years, Java has evolved through several versions, adding new features and enhancing performance with each release.
Java’s Role in Modern Technology
Today, Java is ubiquitous in the world of programming, underpinning a vast array of applications:
- Desktop Applications: Java is used to build cross-platform desktop applications. Software like the Acrobat Reader, media players, and various antivirus programs are common examples.
- Web Applications: Java plays a significant role in server-side applications. Websites and applications like LinkedIn, eBay, and others use Java for their back-end development.
- Enterprise Applications: Large-scale business applications, such as banking and financial services systems, often rely on Java for their complex requirements due to its security and robustness.
- Mobile Applications: Java is also a cornerstone in mobile application development, especially Android apps. The Android SDK uses Java as the base language for developing mobile apps.
- Embedded Systems: Java finds its use in embedded systems owing to its portability and efficiency. Applications range from SIM cards to television set-top boxes.
- Others: Java is also used in developing software for smart cards, robotics, and games, showcasing its versatility.
Setting Up Your Java Environment
Installing Java (JDK and JRE)
Before diving into Java programming, setting up the environment is crucial. The first step is to install the Java Development Kit (JDK), which is a software development environment used for developing Java applications. JDK includes the Java Runtime Environment (JRE), an implementation of the Java Virtual Machine (JVM), and other development tools.
- JDK: The JDK allows developers to create Java programs that can be executed and run by the JVM and JRE.
- JRE: The JRE is what runs Java programs. It includes the JVM, core libraries, and other components necessary for running Java applications.
Choosing an IDE for Java Development
An Integrated Development Environment (IDE) is essential for efficient coding, debugging, and testing of Java applications. Some popular IDEs for Java include:
- Eclipse: Widely used, it offers a vast array of features and plugins.
- IntelliJ IDEA: Known for its user-friendly interface and robust functionality.
- NetBeans: Provides an easy-to-use interface and is great for beginners.
Each IDE has its unique features and choosing one depends on personal preference and the specific needs of your project.
Writing Your First Java Program (“Hello Java”)
After setting up the JDK and choosing an IDE, you are ready to write your first Java program. The classic “Hello, World!” program is a simple yet effective way to understand the basic structure of a Java program.
Here’s a simple example:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, Java!");
}
}
In this program:
- public class HelloWorld defines a class named HelloWorld.
- public static void main(String[] args) is the main method where the program execution begins.
- System.out.println(“Hello, Java!”); prints the string “Hello, Java!” to the console.
To run this program, write the code in your chosen IDE, compile it, and execute it. You should see the output “Hello, Java!” in the console.
Java Syntax and Basic Programming Concepts
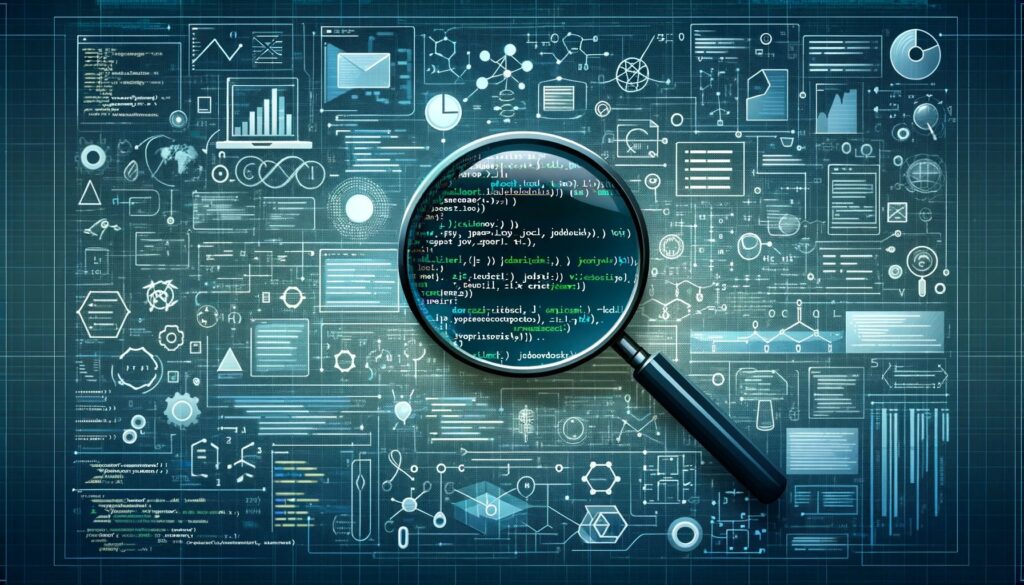
Understanding the syntax and foundational concepts of Java is essential for effective programming. Java’s syntax is similar to C++ but with greater emphasis on object-oriented design.
Understanding Java Syntax
Java syntax refers to the set of rules defining how a Java program is written and interpreted. Key elements include:
- Case Sensitivity: Java is case-sensitive, meaning that identifiers like variable names must be consistently cased.
- Class Names: The first letter of a class name should be uppercase (e.g., MyFirstClass).
- Method Names: A method name should start with a lowercase letter (e.g., myMethod()).
- File Name: The name of a Java file must exactly match the class name and have a .java extension.
Variables, Data Types, and Operators
Variables in Java are used to store data, and their type dictates the kind of data they can hold. Java supports various data types:
- Primitive Data Types: These include int, float, double, boolean, char, etc.
- Non-Primitive Data Types: These refer to objects and arrays.
Operators are symbols that perform operations on variables and values. Java includes:
- Arithmetic Operators: +, -, *, /, %
- Relational Operators: ==, !=, >, <, >=, <=
- Logical Operators: &&, ||, !
Conditional Statements and Loops
Conditional statements and loops control the flow of execution based on conditions.
- If-Else Statement: Allows executing different code blocks based on a condition.
- Switch Statement: A multi-way branch statement that executes one code block from multiple choices.
Loops are used for executing a set of instructions repeatedly:
- For Loop: Executes a block of code a certain number of times.
- While Loop: Repeats a block of code while a condition is true.
- Do-While Loop: Similar to the while loop, but the block of code is executed at least once before the condition is tested.
Sample Java Code: Basic Calculator
Here’s a simple Java program demonstrating variables, operators, and conditional statements:
import java.util.Scanner;
public class BasicCalculator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter first number: ");
double num1 = scanner.nextDouble();
System.out.println("Enter second number: ");
double num2 = scanner.nextDouble();
System.out.println("Enter an operator (+, -, *, /): ");
char operator = scanner.next().charAt(0);
double result;
switch (operator) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
result = num1 / num2;
break;
default:
System.out.printf("Error! Operator is not correct");
return;
}
System.out.printf("%.1f %c %.1f = %.1f", num1, operator, num2, result);
}
}
This program demonstrates a basic calculator that performs addition, subtraction, multiplication, and division based on user input. It illustrates the use of scanner for input, switch statement for conditional logic, and arithmetic operations.
Object-Oriented Programming in Java
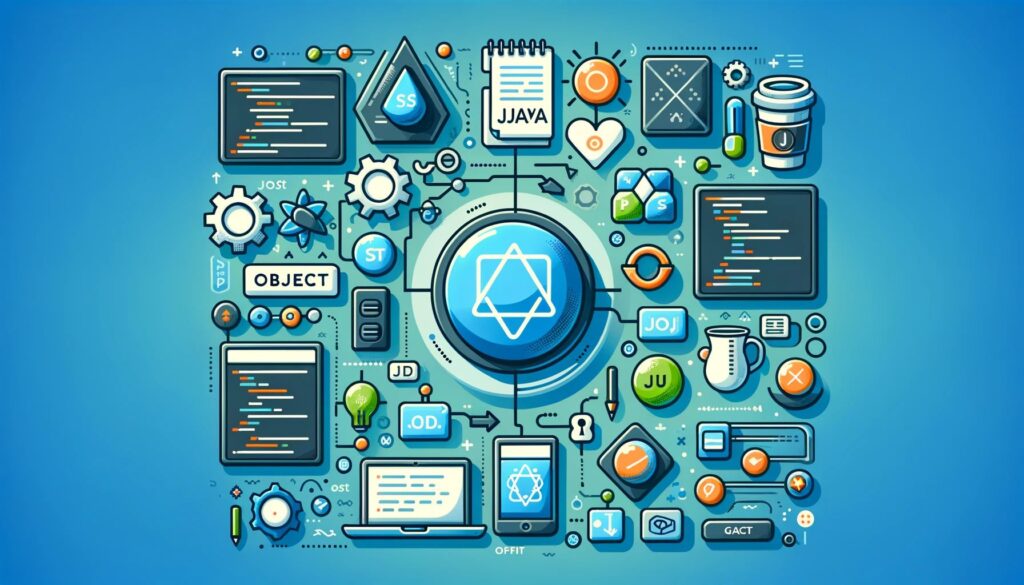
Object-Oriented Programming (OOP) is a paradigm centered around objects rather than actions, significantly influencing how Java applications are designed and written. Java’s OOP nature facilitates modular, flexible, and scalable software development.
The Four Pillars of OOP
- Encapsulation: This involves bundling the data (variables) and code (methods) that operates on the data into a single unit, known as a class. It restricts direct access to some of an object’s components, which is a means of preventing accidental interference and misuse of the methods and data.
- Abstraction: Abstraction means hiding complex implementation details and showing only the necessary features of the object. It reduces complexity and allows the programmer to focus on interactions at a higher level.
- Inheritance: This is a mechanism that allows one class to inherit the features (fields and methods) of another class. It provides a way for code reuse and establishes a relationship between different classes.
- Polymorphism: It allows methods to do different things based on the object it is acting upon. This means a single method can have different implementations.
Classes and Objects in Java
In Java, a class is a blueprint from which individual objects are created. A class contains fields (variables) and methods to describe the behavior of an object.
Here’s a basic example of a class and creating an object from it:
public class Car {
// Fields (Variables)
String color;
String model;
// Constructor
public Car(String color, String model) {
this.color = color;
this.model = model;
}
// Method
public void displayDetails() {
System.out.println("Car Model: " + model + ", Color: " + color);
}
public static void main(String[] args) {
// Creating Objects
Car car1 = new Car("Red", "Toyota");
Car car2 = new Car("Blue", "Ford");
// Calling method on the objects
car1.displayDetails();
car2.displayDetails();
}
}
In this example:
- Car is a class with two fields (color and model) and a method (displayDetails()).
- The Car constructor initializes the objects with the provided values.
- car1 and car2 are objects of the Car class, each with different attribute values.
Constructors and Methods
- Constructor: A constructor in Java is a block of code similar to a method that’s called when an instance of an object is created. It’s used to initialize the object.
- Method: A method is a collection of statements that are grouped together to perform an operation. Methods allow for code reusability and help in breaking down complex processes into simpler tasks.
Java Collections and Data Structures
Java Collections Framework provides a set of classes and interfaces for storing and manipulating groups of objects. These classes are essential for handling data efficiently and are crucial for any Java programmer.
Overview of Collections (List, Set, Map)
The Collections Framework includes various interfaces and classes:
- List: An ordered collection that allows duplicates. Elements can be accessed by their integer index.
- Examples: ArrayList, LinkedList
- Set: A collection that does not allow duplicates. It is mainly used for storing unique elements.
- Examples: HashSet, LinkedHashSet, TreeSet
- Map: An object that maps keys to values. A map cannot contain duplicate keys, and each key can map to at most one value.
- Examples: HashMap, TreeMap, LinkedHashMap
Understanding Generics
Generics enable types (classes and interfaces) to be parameters when defining classes, interfaces, and methods. This provides a way to reuse the same code with different inputs. The most common use of generics is with Collections.
Practical Examples of Using Collections
Let’s explore an example that uses a List and a Map:
import java.util.*;
public class CollectionExample {
public static void main(String[] args) {
// List Example
List fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Cherry");
System.out.println("Fruits List: " + fruits);
// Map Example
Map<String, Integer> stock = new HashMap<>();
stock.put("Apple", 50);
stock.put("Banana", 30);
stock.put("Cherry", 20);
System.out.println("Stock Map: " + stock);
}
}
In this example:
- The fruits list stores elements in insertion order and allows duplicates.
- The stock map associates a fruit name with its quantity in stock. Each key-value pair represents an entry in the map.
Advanced Java Features
Advanced features in Java enhance its capabilities, allowing for more sophisticated and efficient programming. Key advanced features include Exception Handling, Multithreading, and Lambda Expressions.
Exception Handling and Debugging
Exception handling in Java is a powerful mechanism that handles runtime errors, preventing the program from crashing. Java uses try-catch blocks to handle exceptions.
- Try Block: Contains code that might throw an exception.
- Catch Block: Catches and handles the exception.
Here’s an example:
public class ExceptionHandlingExample {
public static void main(String[] args) {
try {
int[] numbers = {1, 2, 3};
System.out.println(numbers[3]); // This will throw an ArrayIndexOutOfBoundsException
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("An exception occurred: " + e.getMessage());
}
}
}
In this code, attempting to access an index outside the bounds of the array triggers an ArrayIndexOutOfBoundsException, which is then caught and handled in the catch block.
Multithreading and Concurrency
Multithreading in Java is the capability to run multiple threads simultaneously. Threads can be used to perform complicated tasks in the background without interrupting the main program.
Example of creating a simple thread:
class MyThread extends Thread {
public void run() {
for (int i = 0; i < 5; i++) {
System.out.println(Thread.currentThread().getId() + " Value " + i);
}
}
}
public class MultithreadingExample {
public static void main(String args[]) {
MyThread t1 = new MyThread();
t1.start();
MyThread t2 = new MyThread();
t2.start();
}
}
This example demonstrates creating two threads that run concurrently, each printing a sequence of numbers.
Java Lambda Expressions and Functional Programming
Lambda expressions, introduced in Java 8, enable functional programming by providing a clear and concise way to represent one method interface using an expression. They are especially useful in collection libraries to iterate, filter, and extract data.
Example of a lambda expression:
List<String> names = Arrays.asList("John", "Jane", "Doe", "Smith");
names.forEach(name -> System.out.println(name));
This example uses a lambda expression with the forEach method to print each element in the names list.
Building Your First Java Application
Once you’ve grasped the basics and some advanced features of Java, you’re ready to start building your own applications. This section will guide you through designing and coding a simple Java project.
Designing a Simple Java Project
Before diving into coding, planning the application’s structure and functionality is crucial. For our example, let’s design a basic To-Do List application. It will include the following features:
- Add a new task
- View existing tasks
- Mark a task as completed
- Delete a task
Practical Coding Exercise
Now, let’s start building the To-Do List application. We’ll break it down into smaller parts for simplicity.
1. Define the Task Class: Each task in the to-do list will be an instance of this class.
public class Task {
private String name;
private boolean isCompleted;
public Task(String name) {
this.name = name;
this.isCompleted = false;
}
public void completeTask() {
isCompleted = true;
}
// Getters and Setters
public String getName() {
return name;
}
public boolean isCompleted() {
return isCompleted;
}
}
1. Create the To-Do List Class: This class will manage the tasks.
import java.util.ArrayList;
import java.util.List;
public class ToDoList {
private final List<Task> tasks = new ArrayList<>();
public void addTask(Task task) {
tasks.add(task);
}
public void completeTask(String taskName) {
tasks.stream()
.filter(task -> task.getName().equals(taskName))
.findFirst()
.ifPresent(Task::completeTask);
}
public void printTasks() {
tasks.forEach(task -> System.out.println(task.getName() + " - Completed: " + task.isCompleted()));
}
}
1. Implement the Main Class: This is where the application logic will reside.
public class Main {
public static void main(String[] args) {
ToDoList myToDoList = new ToDoList();
myToDoList.addTask(new Task("Learn Java"));
myToDoList.addTask(new Task("Write Java Article"));
myToDoList.addTask(new Task("Publish Article"));
myToDoList.completeTask("Learn Java");
myToDoList.printTasks();
}
}
In this application, we created a Task class to represent individual tasks, a ToDoList class to manage the tasks, and a Main class where we simulate adding and completing tasks.
Tips for Effective Java Programming
As you build your Java applications, remember these tips:
- Test as You Go: Regularly test your code to catch errors early.
- Keep It Simple: Start with a simple version of your application and incrementally add features.
- Understand the Java API: Familiarize yourself with Java’s standard libraries as they provide valuable resources.
- Follow Best Practices: Write readable and maintainable code by following Java best practices.
Resources and Continuing Your Java Journey
After building your first Java application, you might wonder what the next steps are in your Java programming journey. This section provides guidance on further resources and pathways to enhance your Java skills.
Recommended Books and Online Resources
1. Books:
- “Effective Java” by Joshua Bloch: This book offers best practices and tips for professional Java programming.
- “Java: The Complete Reference” by Herbert Schildt: A comprehensive guide covering the entire Java language.
2. Online Resources:
- Oracle’s Java Tutorials: Official tutorials that cover various aspects of Java.
- Codecademy’s Java Course: An interactive platform for learning Java from scratch.
Community Forums and Support
- Stack Overflow: A vital resource for any programmer. This Q&A site has a vast community of Java developers who can provide assistance and advice.
- GitHub: Exploring open-source Java projects can provide practical insights into real-world Java programming.
Next Steps in Advancing Your Java Skills
- Explore Advanced Topics: Delve into more complex areas of Java, like Java EE for enterprise applications, JavaFX for rich client applications, and Spring Framework for web applications.
- Contribute to Open Source Projects: Engaging with open-source projects can improve your coding skills and help you understand real-world software development.
- Build Your Own Projects: Continue to develop your own applications. The more you practice, the more proficient you will become.
- Stay Updated: Java is an evolving language. Stay updated with the latest versions and features.
Java is a vast and dynamic language, and there is always more to learn. With a solid foundation, you can continue to grow and evolve as a Java developer, taking on more complex projects and challenges. Remember, the journey of learning Java is continuous and rewarding. Keep exploring, coding, and sharing your knowledge with the Java community.
Conclusion
This guide has provided a comprehensive journey through Java programming for beginners. From the basic setup and syntax to advanced features and building your first application, it lays a solid foundation for aspiring Java developers. The journey into Java is ongoing, filled with continuous learning and practical application. Embrace the challenge, keep exploring new concepts, and join the vibrant community of Java programmers to further enhance your skills and knowledge.