Turning your program into an executable double-click program without the DOS command line.
Out of all the questions posed in java among the most frequently asked has to be:
“How can I turn my program into an executable double-click program without the DOS command line?”
In point of fact there are numerous solutions. The easiest way (assuming Windows) is to use a bat file which will be explained first. I really like this solution as it maintains the integrity of the java program and allows for simple adjustments to the code. The Sun solution is to use an ‘executable jar’, this can be a bit tricky the for the newcomer to get right first time and this is explained here on page 2. The executable jar is also a neat, simple and clean solution that has some persuasive advantages over the bat file. There are many other solutions too such as using 3rd party applications that are listed at the end of this article.
1. Copy, Paste and Compile these 2 Programs
// program 1: HelloWorld1.java
import javax.swing.JOptionPane;
public class HelloWorld1{
public static void main(String args[]){
JOptionPane.showMessageDialog(null,"Hello World.",
"Welcome to Java Programming",JOptionPane.INFORMATION_MESSAGE);
System.exit(0);
}
}
// program 2: HelloWorld2.java
import java.awt.*;
import java.awt.event.*;
public class HelloWorld2 extends Frame implements ActionListener {
public HelloWorld2() {
setLayout(new FlowLayout());
setSize(120, 120);
addWindowListener(new WindowCloser());
Button hello = new Button("Say Hi");
hello.addActionListener(this);
add(hello);
show();
}
public void actionPerformed(ActionEvent e){
if(e.getActionCommand().equals("Say Hi")) {
add(new Label("Hello World") );
validate();
}
}
public static void main(String[] args) {
HelloWorld2 frame = new HelloWorld2();
Image onFrame = Toolkit.getDefaultToolkit().getImage("Hi.gif");
frame.setIconImage(onFrame);
}
}
class WindowCloser extends WindowAdapter{
public void windowClosing(WindowEvent e){
System.exit(0);
}
}
Note: for the second program you will need a gif image. Right click on the image below, copy it and save it as “Hi.gif” in the same folder as HelloWorld2.java and HelloWorld2.class. Note also that there is also a file called WindowCloser.class that has been compiled, the use of 2 class files here is deliberate.
2. How to create an instant click *.bat file
Actually this one is very easy to do. All that is required here is that you open a simple text editor and then type in exactly the same thing as you would type in the DOS command line ie:-
“java HelloWorld1”
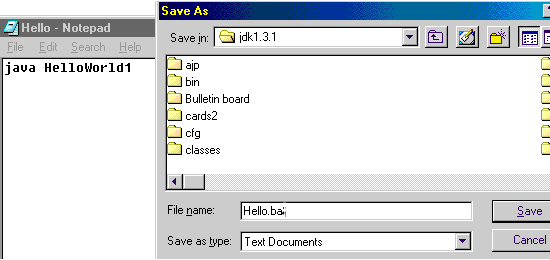
and then save this as “whateverNameYouWant.bat”. From here you can now ‘double click’ on the icon that looks like this in the file named MS-DOS Batch File below and the java file will open.

and you can also change the icon by right clicking the icon (*NB* This is how it works for Windows ’98) and then click on ‘properties’ — > ‘program’ — > and then ‘Change Icon…’ In this case a light bulb icon as shown below. Once you have the short-cut icon you can drag or copy this to the desktop or wherever you want, though note that the java class file(s) must be in the same folder as the *.bat file for this to work. You will also realise that a black DOS screen Window opens when the bat file is clicked upon, this can be useful to capture messages for the user or for your own debugging messages -however, here’s a little known and neat trick;-
line 1: @echo OFF
line 2: javaw MyApplication (done, save it again as: xxx.bat)
The black screen is a feature that does not occur at all with the executable jar file and this is something you can use to bundle all of your files together; images, sounds, class files etc for a really professional finish. This is explained in part two of this tutorial, please click on the link: ‘next page’ below.
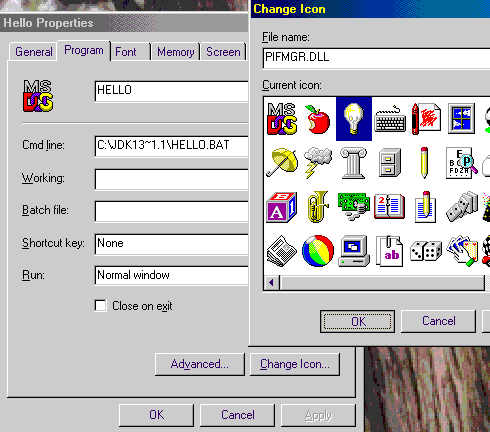
Part 2: How to jar
Jar is an acronym for Java Archive file format, a file type that enables you to bundle multiple files into a single program. First we will make a jar for program 1, ‘HelloWorld1.java’. Make sure that this compiles and runs in the usual way with the MS-DOS command. In order to make our jar the first thing we need to do is to write a Manifest File, this is a simple one line of text which will tell the JRE (Java Runtime Environment) interpreter where to find the java program with the main method signature.
Write this in a simple text editor such as Notepad exactly the same as shown here:
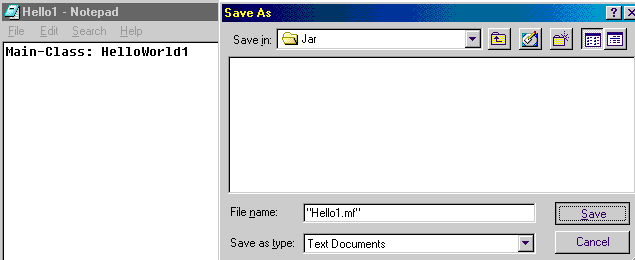
Note the following:
Main-Class: – though not case sensitive, this must have a hyphen and must have a colon.
Include a space: – you MUST include a space after the colon or you will get an error.
The java file: – the file name is the java file with the main method signature, eg “public static void main(String []args){“
(NB: do not include the ‘*.class’ or ‘*.java in the name)
Case sensitivity: – remember that java is case sensitive so the file is ‘HelloWorld1’ and not ‘helloworld1’.
Hit the enter key: – after the class file name (HelloWorld1), YOU MUST hit the key, so in effect this is actually a 2-line file.
Care when saving: – make sure the file is saved with an *.mf extension and not: Whatever.mf.txt as Notepad can sometimes have the annoying habit of associating any file extension not recognised with an additional ‘.txt’. The easiest way to ensure this is to use quotation marks (in Notepad only) when you save the file as shown above (Small techinical point: saving with an *.mft extension also works).
Next: go to MS-DOS and type this in the command line:
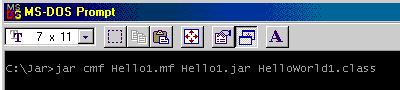
Lets go through that one at a time;-
1. First, be aware that “C:\Jar>” is merely the folder (named Jar) on the C-Drive where these files have been saved. You can save it wherever you wish, though having a seperate folder for your java files and another for your jar files is probably a good idea.
2. “jar”: calls upon the jar command in the JDK or SDK.
3. “cmf”: First ‘c’ means create a new and empty archive file for the jar file. Next ‘m’ means include a manifest file which is specified. Finally, ‘f’ specifies the named jar file to process, in this case ‘Hello1.jar’
(Note: for more information about these jar options see the Sun javadocs or for general information and further reading about jar files try one of the Sun tutorials on the subject)
4. “Hello1.mf”: this is the name of the manifest file that we saved above.
5. “Hello1.jar”: this is the specified name of the jar file that we will create.
6. “HelloWorld1.class”: the compiled java class file to include.
7. Hit the key and the jar file should be created, all being well it should also execute when it is double-clicked.
Program 2:
As stated earlier it is a good idea to create seperate folders for you various java or programming projects and this includes a folder for your jar files. In the case of program 2 there is more than one class file and one other file too, a gif file so the first thing we do here is to isolate them all in a single folder as you will see why in a moment.
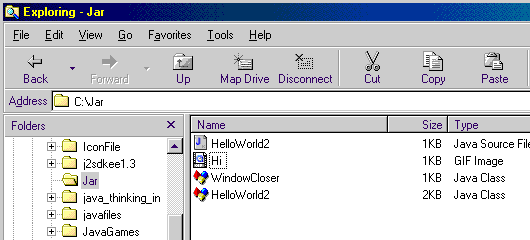
Remember that we will need a Manifest File as before, so open a simple text editor and type;-
Main-Class: HelloWorld2 (and remember to press the key)
… and then save this as ‘Hello2.mf’ (or some other suitable name) in the same folder as the other files.
Next the DOS Command in this case will be as follows:
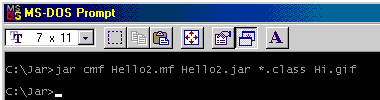
Note that here we simply write *.class, ie: include all of the class files in this folder and we could have also done the same with the gif file (ie, we could have written *.gif instead of Hi.gif) or any other of the file formats we might include, for instance *.wav files, *.jpg files, *.au files or whatever. Here is the final outcome of ‘Hello2.jar’ after double clicking the newly created icon.
The advantages of the jar file over and above the bat file are basically two-fold. In the first place you don’t get the ugly black DOS window rearing its head as your program executes and secondly all of the files: the class files, image data, audio data etc are bundled together with the jar file into one program. In fact you can try this, drag the ‘Executable Jar File’ to another folder or the desk top on its own and you will discover the independent wonders of our jar! If there is a downside to all this, then its the look of the default icon that the jar creates which has the look of a text file in my opinion, do not despair in this as you can easily create a great looking shortcut icon in the same way as we did with out bat file and with the assistance of other programs we can in fact do more (read on).
Executable java programs: some alternative options.
The other common solution is to turn our jar file into a Windows-based *.exe file. Before we go on, I feel somewhat obliged to point out that many java purists disapprove of such an approach and with some justification too. The point being (and it is a good one) is that java is a cross-platform, Operating System independent language and this is right at the heart of the java philosophy, so why change your solution to a narrow, platform specific OS such as a Windows *.exe file? Conversely there may be good reasons for this, for instance the Windows OS has a near monopoly for privately owned home PC’s anyway, so should we worry. There is also the view that “java programs are slow” and I would like to say something about this;-
- First the truth: java programs are not only compiled, but they are
also interpretted by your JVM. This is perhaps the price we have to pay
for platform independence and it will mean they can be slower than what
we might call native code, such as the language of C in a Windows
environment. - A classic feature of the java program is that they often, or even
usually slower to load. - I, personally speaking, have yet to write an application that
appears to run slowly. - Java programs will typically have a bigger memory (ie: RAM)
‘footprint’ and as such may not be suitable for older machines. - Many independent tests have proven java to be just as fast as native
code and in some cases even faster. It depends on what the application
is or what it does. - Programmers make programs that slow, not the language.
- As PC’s have become increasingly faster and more powerful (doubling
every 18 months according to Moore’s Law) the significance of this
difference in performance must also deminish. - The JVM’s have also improved significantly! Many developers have
noted better performances with the JVM/JRE 1.4 over the previous 1.3 and
there are promises of further improvements with 1.5 - If you think you are going to get a great performance hit by
converting your application to an *.exe Windows executable, think again.
This is not a good reason and you will probably be disappointed! - If the target platform does not have a JVM, then this may be
a good reason to make a native executable, it may also be a good idea to
ask your client to update their JVM, which is probably something they
need to do anyway and you could provide them with this on the CD you
distribute or with href=”http://java.sun.com/products/javawebstart/”>Java Webstart if
you are distributing your software via the Internet (You can also jar
the JVM/JRE with your program, though it is the opinion of this author
that this is a ‘dirty’ solution). - Finally note: some of the latest versions of Windows XP will treat
*.jar files as a zip file, this may be politics (and I suspect
that it is!) so you may need to change the file association from ‘zip’
to ‘javaw’. My personal preference for a solution to this is to use a
simple ‘wrapper’ in C so my clients have this as an alternative, which
is one of the options provided below
As with most things such matters boil down to individual choice, my personal preference is use a bat file for development (the test phase) and a jar file for distribution. Here in brief are your free options for converting your java file to an exe file
1. Duckware: allows you to package a Java application, along with its resources (like GIF, JPG, JNI DLLs, etc), into a single compressed 32-bit Windows EXE that targets multiple Java runtime environments (though this is not the same as a true exe file).
2. The GNU Project: is an Open-Source project is similar to the above that will convert java byte code to native machine code. Also highly recommended is Zerog as is the evaluation, trial versions Excelsior Jet and Native J: which will produce a pure exe file. The only ones that I’ve personally used is Duckware and Excelsior and with mixed results, but don’t let that put you off.
3. Windows J++. Shortly after the release of java, Windows came up with their own (proprietary) version of java called J++. This probably caused more confusion than improvement to the cause of good programming practices, though the solution is still available if you’re willing to work with java1 only. These days Microsoft are pushing their .NET platform and the C# language, which is a step in a better direction. Follow the link here to learn more of Microsofts own conversion tools.
4. Wrap it in C. Here is one for C programmers, the code below goes into the JNI (Java Native Interface) dll:
// Example: to call javac.exe : pass the path to cmd.exe in cmdproc,
// and command line stuff in buff: createProcess("_P_WAIT,
// "c:\\windows\\system32\\cmd.exe", "/c c:\\jdkbinpath\\javac.exe c:\\*.java");
// otherwise this won't work correctly. (credit to S. Greenfield)
JNIEXPORT jint JNICALL Java_Win32Native_createProcess (JNIEnv *env, jobject obj,
jint nMode, jstring cmdproc, jstring buff ){
STARTUPINFO si = {0};
PROCESS_INFORMATION pi = {0};
const char *str = env->GetStringUTFChars(cmdproc,0);
const char *str2 = env->GetStringUTFChars(buff,0);
ZeroMemory( & si, sizeof(si) );
si.cb = sizeof(si);
ZeroMemory( & pi, sizeof(pi) );
si.cb = sizeof( STARTUPINFO );
si.lpReserved = NULL;
si.lpReserved2 = NULL;
si.cbReserved2 = 0;
si.lpDesktop = NULL;
si.dwFlags = STARTF_USESHOWWINDOW;
si.wShowWindow = SW_HIDE; BOOL bCreateProcessStatus;
DWORD retval;
bCreateProcessStatus = CreateProcess( str, (LPSTR)str2, NULL, NULL, TRUE,
NORMAL_PRIORITY_CLASS, NULL, NULL, & si, & pi );
env->ReleaseStringUTFChars(cmdproc, str); env->ReleaseStringUTFChars(buff, str2);
if (bCreateProcessStatus) {
WaitForSingleObject( pi.hProcess, nMode );
GetExitCodeProcess( pi.hProcess, &retval );
CloseHandle(pi.hProcess);
CloseHandle( pi.hThread );
}
return retval;
} // end of file
Next, the java code that can be put into a jar for easy use in all your projects:
public class Win32Native {
public static int _P_WAIT = 0;
public static int _P_NOWAIT = 1;
public native int createProcess(int nMode, String strCommand, String strParams);
static {
System.out.println("Loading library...");
System.loadLibrary("Win32Native");
System.out.println("Loaded ok!");
}
public static void main(String[] args) {
Win32Native nat = new Win32Native();
nat.createProcess("_P_WAIT, "c:\\windows\\system32\\cmd.exe",
"/c c:\\jdkbinpath\\javac.exe c:\\*.java");
}
}
Note that the Win32Native.class is here for demo and testing purposes. This call to ‘createProcess’ would call cmd.exe passing the /c param and the path to the java compiler (javac.exe) and the path to the java files we wish to compile (c:\\*.java). The value returned is equal to what GetLastError() in the sytem would return.
It is beyond the scope of this article to provide full lessons in the C language. The purpose here is merely to illustrate that C (and C++ for that matter) can provide useful alternatives as a solution to providing for executable click to start java files and the above solution does not invoke the DOS black window either. If you are interested in this root, then a new language will need to be at least partly learnt and there are various free compilers available such as here or here.
And at the risk of delivering an information overload, here is a C Wrapper application at this link here (and credit to Sun JDC member alias ‘DevWizard’). As much as I do like executable jar files, this is a solution that I personally like very much simply because you can specify your icon independently of what is saved on the user hard drive as an *.ico file. Try it, its a nice one and really easy to do, just follow the instructions on the word docs in the zip file (and there we have it, all my tricks and secrets are revealed).
Finally, there was an interesting solution (supported by a number of big league players: IBM, SuSE, Apache, Borland and others) that came up with the Eclipse Project and what they termed as SWT (Standard Widget Toolkit) that used native widgets (ie: buttons, text areas, menu bars etc) to compile java-based code into native bytecode for desktop application development. A very good idea that provides yet another very credible alternative.
Whatever solution you come up with remember that there are many, many potential solutions and the jar as a solution is a very good one in itself, some might say at least as good as any other. The purpose of the tutorials has been primarily to provide instructions so that you will now know how to bat and jar; – happy clicking!