In the realm of software development, creating a user-friendly and effective graphical user interface (GUI) is crucial. This is where Visual Basic (VB), a programming language developed by Microsoft, shines. Known for its ease of use and integration with the .NET framework, Visual Basic is a powerful tool for building intuitive and dynamic GUI applications.
The Role of Visual Basic in Modern Software Development
Visual Basic, with its rich history, has evolved significantly over the years, adapting to new technologies and frameworks. As a part of the .NET ecosystem, VB.NET offers a robust platform for developers to create versatile and scalable applications. The integration with .NET allows access to a comprehensive suite of libraries, enhancing the capabilities of VB in application development.
The Importance of GUI in Applications
The GUI serves as the bridge between your application and its users. It’s the first thing they interact with, making its design and functionality pivotal to the user experience. A well-designed GUI can significantly enhance usability, increase engagement, and improve the overall perception of your application. In the context of Visual Basic, the focus on GUI is evident from the language’s design and the tools provided in Visual Studio, which simplify the process of GUI creation.
Visual Basic’s Approach to GUI Development
Visual Basic adopts an event-driven programming model, making it particularly suitable for GUI development. This approach allows developers to create applications that respond to user interactions, such as clicks and key presses, in a straightforward manner. The Visual Basic Integrated Development Environment (IDE), primarily Visual Studio, offers a drag-and-drop interface for designing GUIs, making the development process more intuitive and less time-consuming.
Setting Up Your Development Environment
To embark on the journey of GUI development in Visual Basic, the first step is to set up a suitable development environment. Visual Studio, Microsoft’s integrated development environment (IDE), is the primary tool for Visual Basic development. It offers a comprehensive suite of features that cater to both novice and professional developers.
Configuring Visual Studio for Visual Basic
Visual Studio provides a streamlined experience for setting up your development workspace. When installing Visual Studio, ensure that you select the “.NET desktop development” workload. This includes all the necessary tools and libraries for Visual Basic development, especially for Windows Forms and WPF (Windows Presentation Foundation) applications.
Essential Tools and Extensions
Visual Studio offers various tools and extensions that can significantly enhance your development workflow. For GUI development in Visual Basic, key tools include:
- Windows Forms Designer: A visual design surface to drag and drop controls like buttons, text boxes, and labels onto your forms.
- Properties Window: Allows you to view and modify the properties of selected controls on your form in a user-friendly manner.
- Solution Explorer: Helps you manage and navigate through your project files and resources efficiently.
Visual Basic Code Example: Setting Up a Basic Form
In Visual Basic, creating a basic form with a button control can be done with minimal code. Here’s an example of how you can set up a simple form:
Public Class MyForm
Inherits Form
Private Sub InitializeComponent()
Me.SuspendLayout()
'
'MyForm
'
Me.ClientSize = New System.Drawing.Size(284, 261)
Me.Name = "MyForm"
Me.Text = "My First VB Application"
Me.ResumeLayout(False)
End Sub
End Class
This code snippet defines a new form MyForm
with a specific size and title. The InitializeComponent
method is where you would typically add your controls and set their properties.
Adding Controls Programmatically
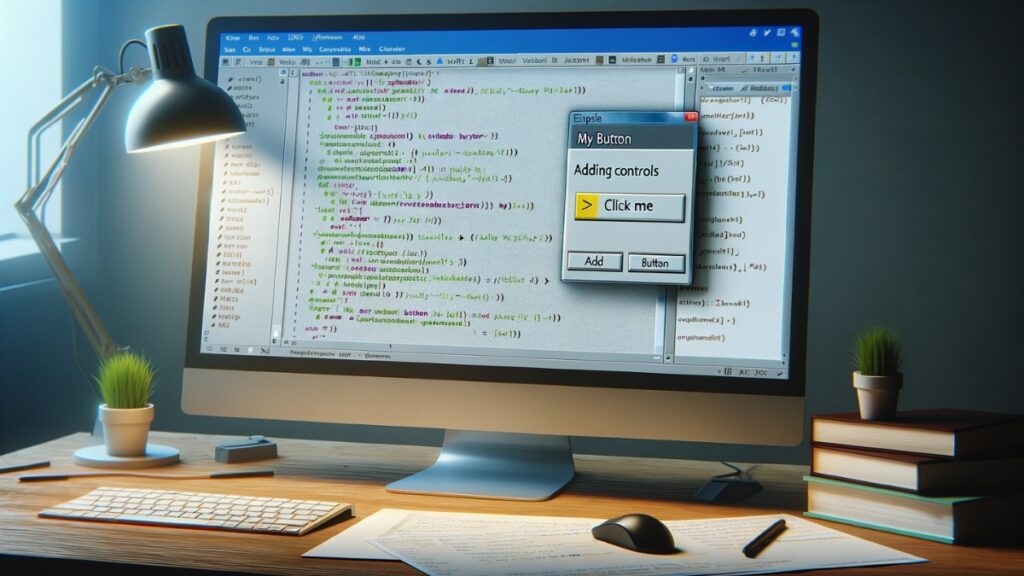
While the Visual Studio Designer allows for drag-and-drop control placement, sometimes you might need to add controls programmatically. Here’s an example of adding a button to MyForm
:
Public Class MyForm
Inherits Form
Private WithEvents MyButton As Button
Public Sub New()
InitializeComponent()
AddButton()
End Sub
Private Sub AddButton()
MyButton = New Button()
MyButton.Text = "Click Me"
MyButton.Location = New Point(100, 100)
AddHandler MyButton.Click, AddressOf MyButton_Click
Me.Controls.Add(MyButton)
End Sub
Private Sub MyButton_Click(sender As Object, e As EventArgs)
MessageBox.Show("Button Clicked!")
End Sub
End Class
In this example, a button named MyButton
is created with the text “Click Me”. It is positioned at a specific location on the form, and an event handler is added to respond to the button click event.
Understanding Basic Controls and Forms
In Visual Basic, the fundamental building blocks of a GUI application are forms and controls. A form acts as a container for various controls like buttons, text boxes, and labels, which are the interactive elements of the GUI.
Exploring the Toolbox
The toolbox in Visual Studio is your go-to place for all controls that you can add to your forms. Here’s a quick overview of some commonly used controls:
- Button: Triggers an action when clicked.
- TextBox: Allows users to input text.
- Label: Displays non-editable text, usually for labeling other controls.
- CheckBox: Represents an option that can be turned on or off.
- ComboBox: A drop-down list from which the user can select one of several choices.
Designing Forms
Forms in Visual Basic are highly customizable. You can set various properties such as size, background color, and text. Here’s an example showing how to customize a form’s properties programmatically:
Public Class MyForm
Inherits Form
Public Sub New()
Me.Text = "My Custom Form"
Me.Size = New Size(300, 200)
Me.BackColor = Color.LightBlue
End Sub
End Class
This code sets the title, size, and background color of the form.
Customizing Control Properties
Just like forms, controls have properties that can be set to change their appearance and behavior. Here’s an example of customizing a button:
Private WithEvents MyButton As Button
Private Sub AddButton()
MyButton = New Button()
MyButton.Text = "Submit"
MyButton.Size = New Size(100, 50)
MyButton.Location = New Point(100, 100)
Me.Controls.Add(MyButton)
End Sub
In this example, the button’s text, size, and location are set before adding it to the form.
Writing Code for User Interactions
The real power of GUIs in Visual Basic comes from responding to user actions, such as clicks and text input. Here’s how you can add a click event handler to the button:
Private Sub MyButton_Click(sender As Object, e As EventArgs) Handles MyButton.Click
MessageBox.Show("Submit button clicked!")
End Sub
This event handler displays a message box when the button is clicked.
Advanced Controls and Customization Techniques
Moving beyond the basic controls, Visual Basic in Visual Studio offers a range of advanced controls that enable more complex functionalities and customization. These controls can enhance the user experience by providing more interactive and dynamic interfaces.
Implementing Advanced Controls
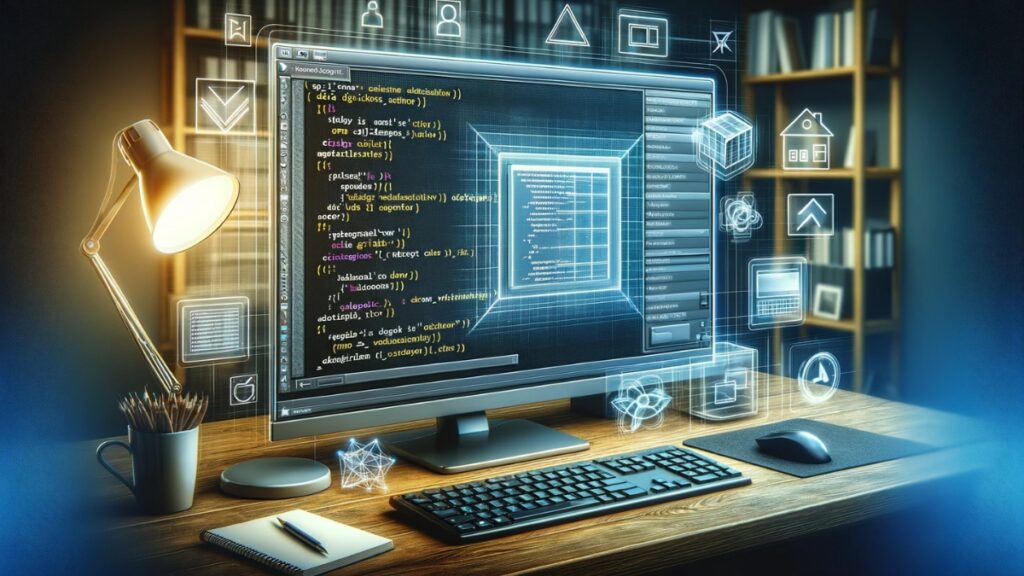
Some of the advanced controls you might use in your Visual Basic applications include:
- DataGridView: Displays data in a customizable grid format. It is commonly used for displaying, editing, and sorting tabular data.
- PictureBox: Used to display images in various formats.
- Panel: A container control that can hold other controls, useful for grouping elements.
Here’s an example of adding a DataGridView
to a form and customizing its properties:
Private WithEvents MyDataGridView As DataGridView
Private Sub AddDataGridView()
MyDataGridView = New DataGridView()
MyDataGridView.Size = New Size(300, 200)
MyDataGridView.Location = New Point(50, 50)
' Additional customization and data binding here
Me.Controls.Add(MyDataGridView)
End Sub
This code snippet demonstrates the creation of a DataGridView
control, setting its size and location, and then adding it to the form. Further customization can include setting data sources, column types, and styles.
Custom Graphics
For applications requiring custom graphics, Visual Basic provides the Graphics
class. This class can be used to draw shapes, text, and images directly on the form or other controls. Here’s an example of drawing a simple rectangle:
Protected Overrides Sub OnPaint(ByVal e As PaintEventArgs)
MyBase.OnPaint(e)
Dim g As Graphics = e.Graphics
g.DrawRectangle(Pens.Blue, New Rectangle(10, 10, 100, 50))
End Sub
This code overrides the OnPaint
method of the form and uses the Graphics
object to draw a blue rectangle.
Techniques for Customizing Look and Feel
Customizing the look and feel of your application is crucial for creating a unique and engaging user experience. This can be achieved by:
- Setting control properties like fonts, colors, and backgrounds.
- Using styles and themes for a consistent appearance across the application.
- Implementing custom rendering for controls.
Event-Driven Programming in Visual Basic
Visual Basic’s event-driven programming model is pivotal for creating interactive GUI applications. This model allows your application to respond to various events, such as button clicks, text changes, or mouse movements.
Understanding Events and Event Handlers
An event is a response to an action performed by the user or by the system. Event handlers are the blocks of code that execute in response to these events. For example, a button’s Click
event can have an event handler that executes when the button is clicked.
Here’s an example of handling a TextChanged
event for a TextBox
:
Private Sub MyTextBox_TextChanged(sender As Object, e As EventArgs) Handles MyTextBox.TextChanged
' Code to execute when the text changes
End Sub
In this snippet, the MyTextBox_TextChanged
method is an event handler that gets called whenever the text in the TextBox
changes.
Writing Code for User Interactions
Writing effective event handlers is key to making your application interactive. Here are some tips:
- Keep event handlers focused on their specific task.
- Avoid writing lengthy and complex code inside event handlers; instead, call other methods.
- Ensure that your UI remains responsive during long-running tasks by using asynchronous programming or background workers.
Debugging and Testing Your GUI Application
Debugging and testing are integral parts of the development process, especially in GUI applications where user interactions can lead to unexpected behaviors. Visual Basic in Visual Studio offers powerful debugging tools to help you identify and fix issues in your applications.
Best Practices for Debugging Visual Basic Applications
- Using Breakpoints: Breakpoints allow you to pause the execution of your application at a specific line of code. This is useful for examining the current state of your application and understanding the flow of execution.
Example of setting a breakpoint in code:
' Assuming this is a part of a larger code block
Dim i As Integer = 10
' Set a breakpoint on the next line to inspect the value of i
Debug.WriteLine(i)
- Watching Variables: The Watch window in Visual Studio lets you monitor the values of variables during debugging. You can see how the values change as you step through the code.
- Stepping Through Code: You can use the Step Over, Step Into, and Step Out features to control the execution of your code line by line. This is particularly helpful for understanding the behavior of event handlers and other methods.
Strategies for Effective Testing and Validation
- Unit Testing: Write unit tests to validate individual parts of your application. Visual Studio has built-in support for unit testing, allowing you to automate the testing of various components.
- UI Testing: Automated UI tests simulate user interactions with the GUI. These tests can help ensure that your application behaves as expected from a user’s perspective.
- Error Handling: Implement robust error handling within your application. This includes catching exceptions and, if necessary, informing the user in a non-technical manner.
Example of basic error handling:
Try
' Code that might throw an exception
Catch ex As Exception
MessageBox.Show("An error occurred: " & ex.Message)
Finally
' Code that runs after the try/catch block
End Try
- Performance Testing: Ensure that your application performs well, especially in scenarios with heavy user interactions or data processing.
Deploying and Maintaining Your Application
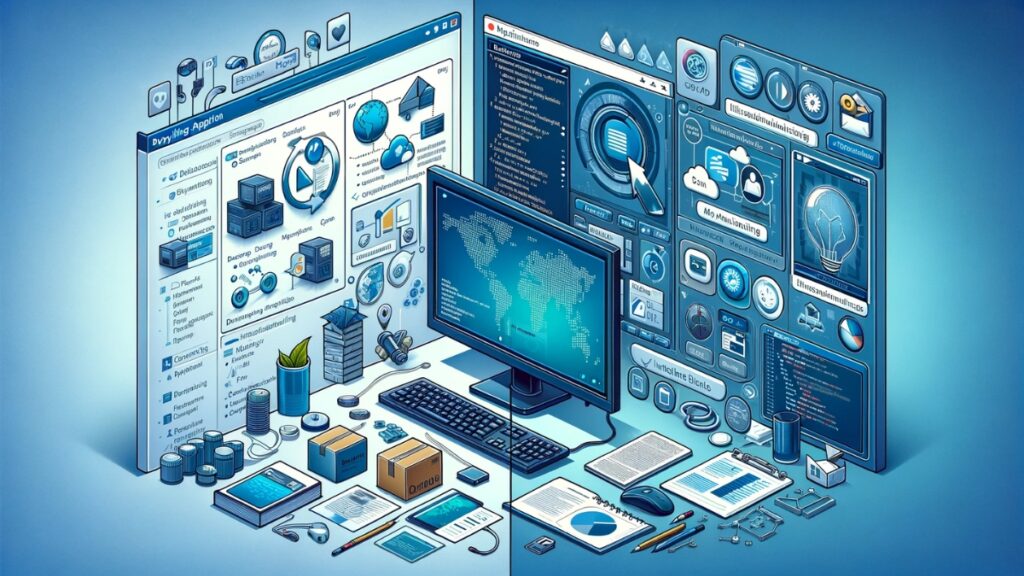
Once your GUI application is developed, tested, and ready for use, the next steps are deployment and maintenance.
Steps for Deploying a Visual Basic Application
- Compiling the Application: Compile your application into an executable file (.exe) using Visual Studio.
- Creating an Installer: Use tools like Microsoft’s Installer or third-party installer packages to create an installation program for your application.
- Distributing the Application: Distribute the application to users, which can be done via a website, physical media, or network deployment.
Tips for Maintaining and Updating Your GUI Application
- Regular Updates: Provide regular updates to fix bugs, improve performance, and add new features.
- User Feedback: Collect and analyze user feedback to understand how your application is being used and where improvements can be made.
- Monitoring and Analytics: Implement monitoring tools to track usage patterns, error reports, and performance metrics.
- Refactoring: Regularly refactor your code to improve readability and maintainability.
Additional Resources and Further Learning
Developing expertise in Visual Basic for GUI applications involves continuous learning and practice. Here are some resources and avenues for further exploration and learning:
Books
- “Programming in Visual Basic 2010” by Julia Case Bradley and Anita Millspaugh: This book provides a comprehensive introduction to Visual Basic, covering fundamentals and advanced topics.
- “Starting Out With Visual Basic” by Tony Gaddis and Kip Irvine: Ideal for beginners, this book introduces programming concepts in a step-by-step manner with plenty of examples.
Online Courses
- Microsoft Learn: Microsoft offers a range of free learning paths and modules for Visual Basic and .NET development Microsoft Learn for Visual Basic.
- Udemy and Coursera: Both platforms have various courses on Visual Basic, from beginner to advanced levels. These courses are often created by industry professionals and academics.
Tutorials and Documentation
- Microsoft Documentation: The official Microsoft documentation is an excellent resource for learning about Visual Basic and staying updated with the latest features Microsoft Docs for Visual Basic.
- VB.NET Tutorial: A comprehensive resource for tutorials covering different aspects of Visual Basic programming VB.NET Tutorial.
Community Forums and Groups
- Stack Overflow: A vital resource for developers, Stack Overflow has an active community for Visual Basic where you can ask questions and share knowledge Stack Overflow Visual Basic.
- GitHub: Explore repositories related to Visual Basic to see real-world applications and projects. This can also be a great way to contribute to open source projects GitHub Visual Basic.
Conferences and Meetups
- Microsoft Build: Microsoft’s annual conference often includes sessions on .NET and Visual Basic, offering insights into the latest developments.
- Local Developer Meetups: Check platforms like Meetup.com for local developer groups focusing on .NET and Visual Basic. These meetups can be a great way to network and learn from peers.
YouTube Channels
- Microsoft Visual Studio: The official Visual Studio YouTube channel provides tutorials, tips, and feature highlights Microsoft Visual Studio YouTube.
- Programming Knowledge: This channel offers a series of Visual Basic tutorials, useful for beginners and intermediate developers.
By leveraging these resources, you can enhance your skills, stay updated with the latest trends, and connect with a community of like-minded developers. Whether you’re just starting out or looking to deepen your expertise, the journey of learning Visual Basic for GUI development is continuous and rewarding.